Understanding Wrapper Classes in Java Programming
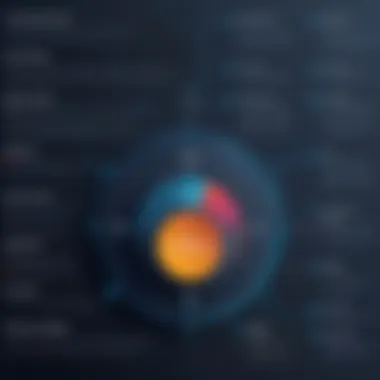
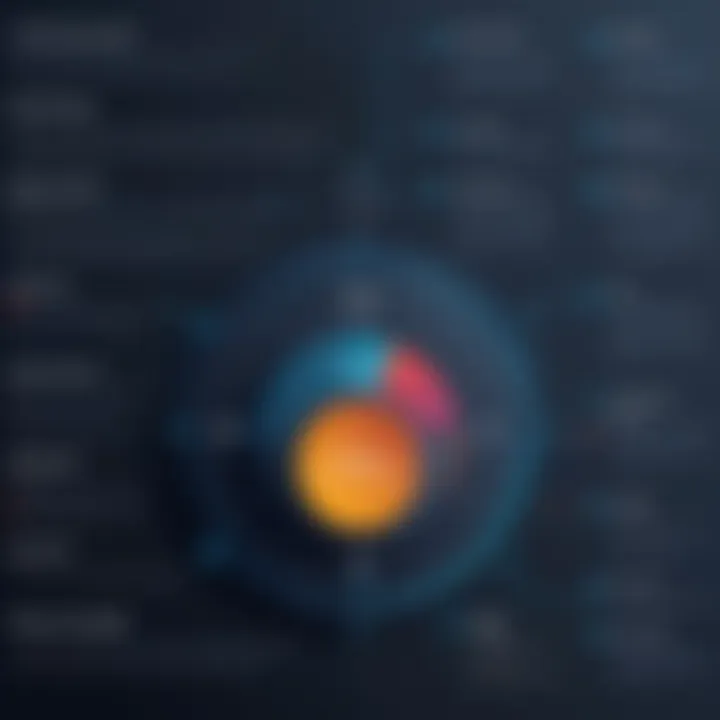
Intro
In the vast realm of software development, dozens of solutions exist to cater to the pressing needs for data manipulation and performance. Among these solutions, the Java programming language stands out as a powerful ally for programmers seeking flexibility and reliability. One may wonder: how do we manage and manipulate primitive data types effectively? This is where wrapper classes come into play, bridging the gap between simple data types and the rich world of objects.
Wrapper classes in Java are essentially object representations of primitive data types like , , , and others. Every time you find yourself needing to work with objects while handling these fundamental types, these wrappers fetch you from defeat. If Java is the vessel sailing through the seas of programming, then wrapper classes are the buoy, keeping you afloat amid the intricacies.
In this article, readers will embark on a journey of discovery, unveiling the variety of wrapper classes, exploring their capabilities, and comprehending their role in object-oriented programming. Through practical examples, we will also illustrate the implementation of these classes, equipping you with knowledge suitable for both newcomers and seasoned programmers.
Significance of Wrapper Classes
Wrapper classes prove essential in many scenarios, such as:
- Collections: Java Collections Framework requires objects, and here is where wrapper classes shine. You can't add directly to an , but you can add an object instead.
- Method Overloading: They allow method calls to be overloaded, enabling different types of data to be handled gracefully.
- Immutable Objects: Unlike primitives, wrapper classes create immutable objects, making them safer and less prone to unexpected changes during execution.
As we continue through this article, expect to gain insights not just into their functions, but also their drawbacks. After all, like two sides of a coin, understanding any technology includes recognizing its limitations. Stay tuned, for the next chapter unfurls the foundational aspects of coding, revealing simple principles that will build a solid ground for exploring wrapper classes further.
Intro to Wrapper Classes
When delving into the realm of Java, one must recognize the pivotal role that wrapper classes play in bridging the gap between primitive data types and the world of objects. Wrapper classes are not just auxiliary components; they significantly extend the capabilities of Java's primitive types, allowing developers to take full advantage of object-oriented programming principles. This article unpacks the various elements surrounding wrapper classes, emphasizing their benefits and considerations in programming.
Wrapper classes serve numerous purposes. They facilitate type safety in generic classes, promote better interaction with collections, and enable methods to handle objects rather than primitive data types. Understanding their functionality can act as a cornerstone for anyone working with Java, opening up avenues for more robust programming practices.
Additionally, as one navigates the intricacies of Java’s data handling, it's essential to consider the historical evolution of wrapper classes. The journey from primitive types to their encapsulated counterparts sheds light on how Java developers made strides to enhance functionality while ensuring the integrity and safety of data.
In this section, we will dissect the fundamental aspects of wrapper classes by exploring their definitions, purposes, and evolution, establishing a clear understanding for students and programming enthusiasts who aim to enrich their coding skill set.
Definition and Purpose
Wrapper classes in Java are essentially classes that encapsulate primitive data types. Each primitive type—such as int, char, and boolean—has a corresponding wrapper class: Integer, Character, and Boolean, respectively. This encapsulation allows primitive data types to be treated as objects, which is crucial for utilizing Java’s object-oriented features.
The main purposes of wrapper classes include:
- Object Representation: They convert primitives into objects whenever required, making it easier to work with data in object-oriented structures.
- Utility Methods: Each wrapper class comes equipped with useful methods for conversion, manipulation, and parsing of the primitive types, enhancing flexibility in coding.
- Working with Collections: Since collections in Java (like ArrayList and HashMap) cannot store primitives directly, wrapper classes become indispensable here, allowing the storage of primitive values in a more complex data structure.
Evolution of Wrapper Classes in Java
The evolution of wrapper classes in Java reflects the language's growth and adaptations to user needs. Initially, Java operated primarily on primitive data types, restricting the ways developers could interact with data. With the introduction of wrapper classes in Java 1.0, developers were granted new capabilities for object manipulation that were absent in earlier iterations of Java.
As Java progressed, new features were added. The autoboxing and unboxing mechanism introduced in Java 5 allowed seamless conversion between primitives and their corresponding wrapper classes, streamlining code and reducing boilerplate. This refinement not only improved the efficiency of writing Java code but also enhanced the overall performance of applications by minimizing unnecessary object creation.
"Wrapper classes have become a fundamental aspect of Java programming, evolving as the language has matured, creating a more intuitive programming environment."
In short, understanding the definition and the evolutionary journey of wrapper classes is crucial for anyone looking to harness the full potential of Java. It sets the stage for exploring their roles, types, and applications in the broader context of data manipulation and programming practices.
Understanding Primitive Data Types
To appreciate the utility of wrapper classes, one must first have a solid grasp on primitive data types. In Java, these primitive types form the backbone of data manipulation, providing a foundation for everything that follows. Understanding them is akin to learning the notes before playing a musical piece; without a strong melody of primitive types, you can’t expect the symphony of Java programming to resonate.
Overview of Primitive Types
Primitive data types in Java comprise a small but vital set of values. These types are:
- byte: An 8-bit signed integer, which can hold values from -128 to 127.
- short: A 16-bit signed integer, providing a range from -32,768 to 32,767.
- int: The most commonly used data type, a 32-bit signed integer, ranging from -2^31 to 2^31-1.
- long: A 64-bit signed integer, accommodating large values between -2^63 to 2^63-1.
- float: A 32-bit floating-point number, suitable for decimal values but with limited precision.
- double: A 64-bit floating-point number, offering double the precision of float, making it ideal for most mathematical calculations.
- char: A single 16-bit Unicode character, which can represent letters, digits, and symbols.
- boolean: A binary data type that can be either true or false.
These primitive types are crucial for storing basic data. Unlike objects, they do not possess methods or attributes, which means they're lightweight and generally faster in terms of performance.
Limitations of Primitive Data Types
While primitive types are beneficial, they come with a set of limitations that can hamper their usability in various programming scenarios:
- No Methods: Being basic data types, they lack methods. If you want to perform operations or manipulate the data, you need to resort to arrays or collections, which can be cumbersome.
- Fixed Size: Each primitive type has a defined size. This fixed size can be an issue when dealing with large data sets or when calculations exceed the type's capacity, leading to overflow or underflow situations.
- No Nullability: Primitive types cannot hold a null value. This can be problematic in situations where null is often required to signify the absence of data or an optional state. You can’t assign null to an integer, for instance, without using its corresponding wrapper class.
- Limited Operations: Many useful operations or functionalities that come with classes are absent from primitive types. For instance, you cannot directly convert a primitive data type to another type without explicit casting.
The absence of these features is where wrapper classes step in, filling the gaps that primitives leave behind. By converting primitives into objects with wrapper classes, you gain access to a wealth of additional methods and functionalities that make data manipulation far more intuitive.
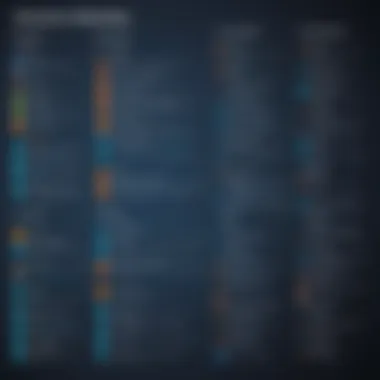
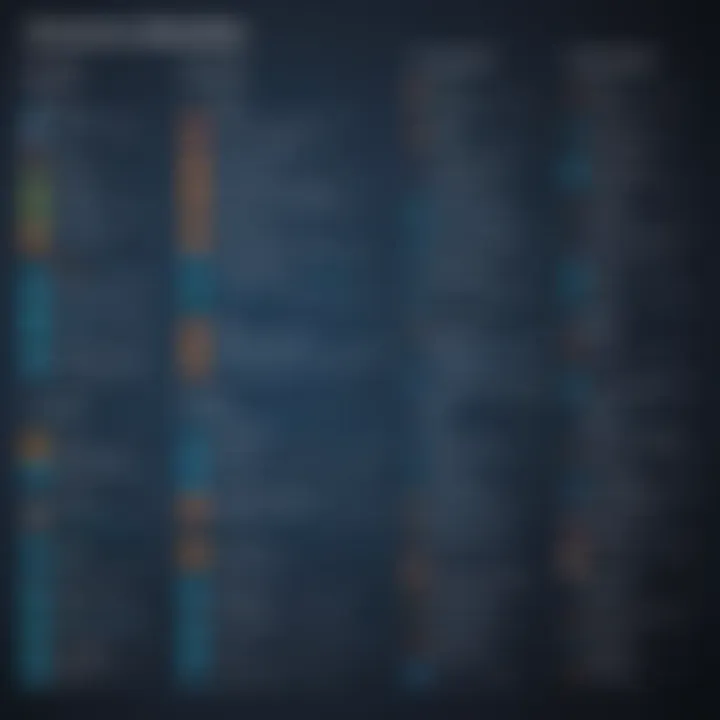
"Understanding these primal types is essential; they are the building blocks of any Java application. Without them, designing sophisticated programs becomes a Herculean task."
Grasping the importance of primitive data types simplifies the process of working with wrapper classes and enhances overall programming efficiency.
The Role of Wrapper Classes
In the world of Java programming, wrapper classes serve a crucial purpose, bridging the gap between primitive data types and the object-oriented nature of the language. Simply put, they allow developers to treat primitive types as objects, making it easier to explore their functionalities, especially in cases where an object orientation may be essential, such as when interfacing with collections or generics. Understanding this role is fundamental for anyone diving into Java.
Converting Primitives to Objects
When coding, you often encounter situations where you need to switch between a primitive data type and its corresponding object, and that’s where wrapper classes come into play. For instance, in Java, the primitive can be converted to an object using wrapping methods, either manually or automatically.
To take a closer look:
Here, the method converts a primitive integer into an Integer object. This conversion, known as boxing, allows objects to be stored in data structures that require reference types. In the context of programming, having these conversions can be beneficial when leveraging Java's Collections Framework, among other places.
However, it’s equally important to note the reverse process—unboxing—which returns the primitive data from its wrapped object form. For example:
The seamless conversion between objects and primitives is significant. This efficiency opens the door for developers to take full advantage of Java's features without sacrificing performance or design.
Benefits of Using Wrapper Classes
The introduction of wrapper classes in Java is not merely a design choice; numerous benefits arise from their use in various programming scenarios. Here are several key advantages:
- Null Value Representation: Primitives cannot be null, while wrapper classes can. This becomes particularly useful when working with collections or databases where a value may be absent.
- Enhanced Functionality: Wrapper classes come equipped with useful methods. For instance, the class includes methods like , which can be incredibly useful for converting strings into integers seamlessly.
- Support for Collections: Java’s collection framework works largely on objects. Hence, being able to use numbers and characters as objects broadens the possibilities when designing data structures like or .
- Autoboxing Convenience: Java simplifies the process of converting primitives to objects automatically through autoboxing. This feature allows smooth conversion with minimal boilerplate code, enhancing developer productivity.
"Wrapper classes are not just about making code work; they enrich it by adding flexibility and functionality that primitives can't provide alone."
Despite these advantages, it’s prudent for coders to bear in mind performance considerations when utilizing wrapper classes due to additional memory overhead. Balancing their use with performance needs is essential for effective programming in Java.
Types of Wrapper Classes in Java
In the realm of Java programming, wrapper classes serve a pivotal role in bridging the gap between primitive types and the object-oriented nature of the language. These classes not only broaden the programming toolkit but also enhance the flexibility and functionality of data manipulation. The importance of understanding the various types of wrapper classes cannot be overstated, as they allow developers to seamlessly incorporate primitive data types into more complex data structures like collections and generics. The interplay between wrapper classes and primitive data types is foundational for any aspirational Java developer, making it essential to delve into the specifics of each wrapper class.
Integer Wrapper Class
The Integer wrapper class transforms the traditional int type, enabling a range of functionalities that help in performing various operations. One primary advantage is the ability to use integers in collections, such as or , where objects are required. By boxing an int into an Integer, programmers can leverage the full power of Java's object-oriented programming capabilities. Additionally, the Integer class includes useful methods like which allows for the conversion of a string to an int.
Interestingly, one should note that Java caches Integer objects for values from -128 to 127. This means that if you create Integer objects in this range, Java will return the same object instead of creating a new one. Thus, performance implications can come into play when frequently creating Integer instances within this range.
Double Wrapper Class
The Double wrapper class acts similarly for the double primitive data type, allowing for enhanced numerical computations. This class becomes particularly handy when managing decimals, especially in cases like scientific calculations or financial applications where precision is crucial. The Double class provides many static methods, including and , which help in validating calculations.
Working with the Double class also introduces memory considerations. As a floating-point type, it can consume more memory compared to its primitive counterpart, which may matter in performance-critical applications. Being aware of the trade-offs when using Double instead of double is important for optimized coding.
Character Wrapper Class
Next, the Character wrapper class helps to wrap around the char primitive type, providing methods that process single characters. The functionalities offered by this class can be quite beneficial, such as checking if a character is a digit, letter, or whitespace. These operations allow developers to manipulate characters in a more robust manner.
Moreover, Character methods also include support for Unicode characters, which broadens the internationalization capabilities of Java applications. Therefore, using the Character class is a good practice when dealing with any text processing tasks involving individual characters.
Boolean Wrapper Class
Lastly, the Boolean wrapper class encapsulates the boolean data type, adding significant functionality to the true/false primitive. Beyond simply storing a value, the Boolean class provides articulations for handling logic within Java applications. One standout method is , which converts a string representation of a boolean into a boolean value.
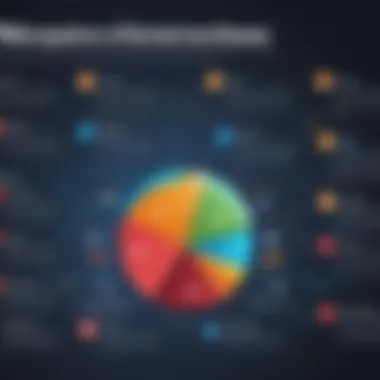
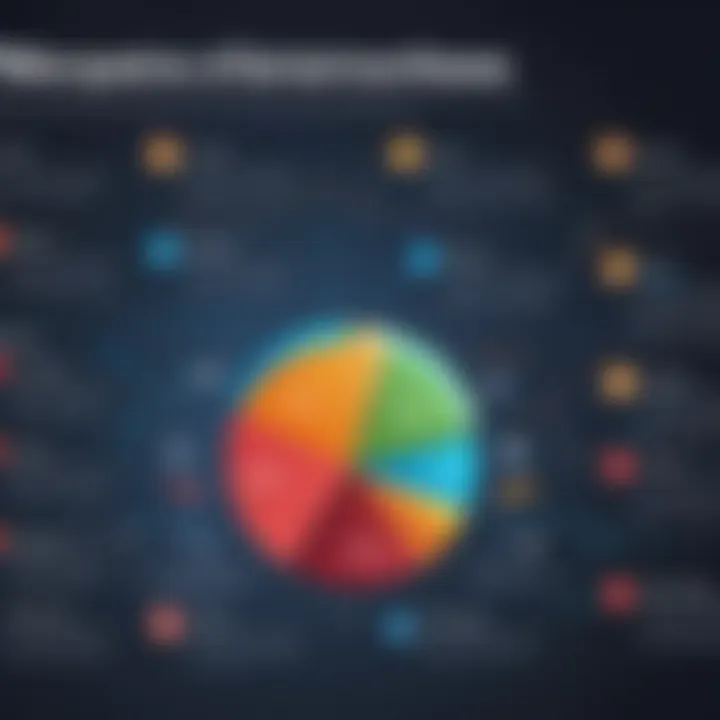
Using Boolean objects is often essential in collections and generics, as booleans cannot directly be used as objects. The understanding of this wrapper class is crucial for controlling flow in applications involving logical operations, such as in conditionals and loops.
By mastering these wrapper classes, Java developers can ensure a thorough understanding of both primitives and objects, unlocking new realms of flexibility and power in their programming prowess.
Boxing and Unboxing
The concepts of boxing and unboxing are foundational in understanding how wrapper classes function within Java. At first glance, they may seem like a simple technicality, but they are crucial for efficient programming. These processes are pivotal for converting primitive data types to their respective wrapper classes and vice versa, enabling object-oriented programming practices in Java. With Java heavily leaning on OOP principles, the significance of these processes becomes even more pronounced.
What is Boxing?
Boxing is the process of converting a primitive type into its corresponding wrapper class object. For example, take an primitive type. When it's boxed, it becomes an instance of the wrapper class. This capability allows the primitive data types to function as objects. It’s like taking a raw ingredient and placing it in a fancy package—the essence remains the same, but the presentation and potential for manipulation expand.
Example of boxing in Java:
In the example above, the primitive integer gets boxed into a object. This transformation enables developers to utilize methods defined in the class, enhancing the versatility of their code. Moreover, boxing automatically occurs when a primitive is assigned to a variable of its wrapper class type, thanks to Java's internal handling of such conversions.
Understanding Unboxing
Unboxing is the reverse operation of boxing; it’s the process of converting a wrapper class object back into its corresponding primitive type. Continuing from the previous example, if you have an object and you want to retrieve the primitive value it encapsulates, you perform unboxing. This step is essential when you're looking to utilize the original primitive functionality, as it allows developers to extract the fundamental data type without the overhead of the object structure.
Example of unboxing in Java:
This conversion brings the value back to its primitive form. Java provides a seamless way to unbox; if you directly assign an to an , Java auto-magically handles the conversion for you. Every time you work with collections, generics, or want to leverage methods that require objects, you'll find boxing and unboxing at play.
Key Point: Boxing and unboxing illustrate Java's ability to bridge the gap between primitive types and object-oriented programming, enhancing code flexibility.
In summary, understanding boxing and unboxing can demystify a significant aspect of using wrapper classes in Java. These processes not only enable you to manage data types more effectively but also make it easier to create scalable and maintainable code.
Practical Applications of Wrapper Classes
Wrapper classes play a vital role in Java programming, particularly when it comes to practical applications that leverage the strengths of both primitive data types and object-oriented design. Understanding how to effectively use these classes can enhance a programmer's ability to manipulate data more flexibly and efficiently. This section explores key ways in which wrapper classes are utilized, focusing on their significance in Java's collection framework and generics.
Using Wrapper Classes in Collections
In Java, collections are widely used data structures that facilitate the storage and management of groups of objects. A primary limitation of collections is that they cannot hold primitive data types directly. For example, if you want to store integer values in a list, you need to use the Integer class instead of just an int. This is where wrapper classes become indispensable.
When you work with collections like , , or , you actually store instances of wrapper classes. Here are some benefits of using wrapper classes in collections:
- Flexibility: Wrapper classes allow you to add, remove, and manipulate data with ease. You can store all sorts of numbers and characters in collections without worrying about losing their values during type conversion.
- Functionality: Many collection types provide built-in methods that require objects rather than primitives. Using Integer or Double is the key to unlocking these advanced features.
- Null Representation: Unlike primitives that cannot hold a null value, wrapper classes can, which is particularly useful when working with databases or APIs where nullable values are common.
"Utilizing wrapper classes in collections is not just a workaround; it's a strategy that allows you to build more robust Java applications."
A simple example demonstrating this is:
In this snippet, you can see how the is used to store objects, thus showcasing how wrapper classes enable the use of collections that inherently require objects.
Wrapper Classes in Generics
Generics introduce another layer of versatility in Java programming, enabling classes and interfaces to operate on types specified by the programmer. When it comes to generics, wrapper classes are often used for type parameters, which makes for more inclusive and dynamic code.
The advantages of integrating wrapper classes with generics include:
- Type Safety: Generics provide compile-time type checking, which enhances code reliability. By using wrapper classes as generic parameters, developers ensure that their code behaves predictably.
- Code Reusability: Methods and classes defined with generics can manage any of the wrapper types without modification. This means fewer duplicate methods or classes for different types, leading to cleaner, more maintainable code.
- Inheritance Support: Wrapper classes inherit from the class, allowing them to play nicely with generics, which require object types. Thus, every time you need an or , you're utilizing wrappers effectively.
Here's how a generic class might look:
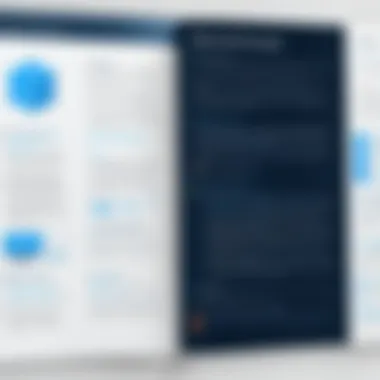
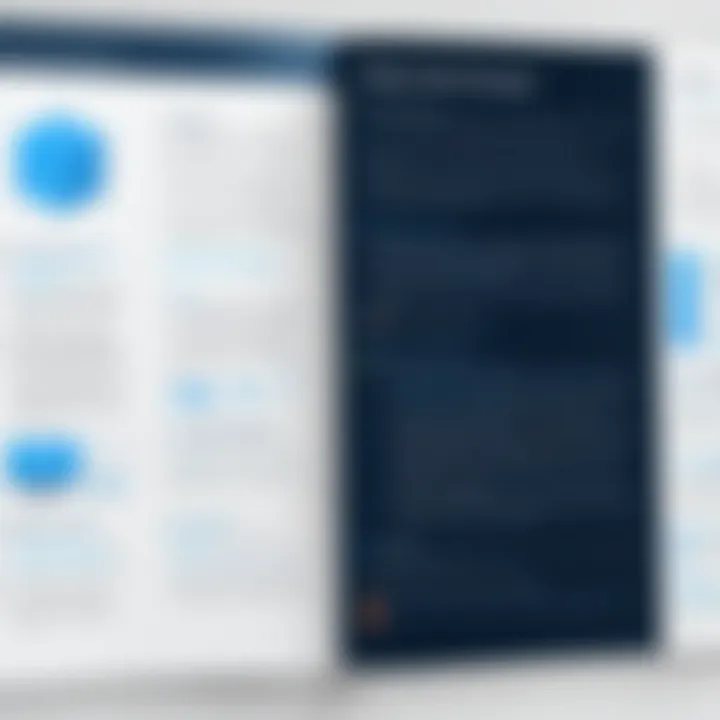
In this example, is a class that can work with any type, including Integer. This approach fosters versatility while keeping the core logic intact.
Performance Considerations
When delving into wrapper classes in Java, performance considerations are paramount. Understanding how these classes interact with the rest of the system can help programmers make informed choices that optimize applications. The use of wrapper classes can bring about several advantages, but they also present certain challenges related to performance and memory usage. In this section, we'll explore these implications in detail.
Memory Usage Implications
Wrapper classes play a significant role in memory management within Java applications. When you use primitive types, such as or , you’re working with simple, lightweight values. But once you box these primitives into their respective wrapper classes—like , , or —the scenario changes.
- Each wrapper object introduces an overhead that comes in the form of additional memory required to store the object’s metadata and its reference on the heap.
- The larger heap-based footprint can impact performance negatively, especially in memory-constrained environments. For example, if you're working with a myriad of objects instead of plain , you might notice a dip in performance due to increased garbage collection activity.
- In scenarios where memory efficiency is critical, minimizing the use of wrapper classes is advised. For smaller populations of data, this concern may not pose a significant issue. However, in large-scale data processing, these implications can add up quickly.
This small snippet illustrates the shift from a primitive to a wrapped primitive. Despite the convenience of , be mindful of how many instances you’re creating.
Comparative Analysis with Primitives
It’s crucial to understand how wrapper classes stack up against their primitive counterparts. The primary distinction lies not just in syntax but in functional capabilities and behaviors.
- Performance: Generally, primitive types are faster to execute because they are stored directly in memory, while wrapper classes must reference their memory location. This access level significantly elevates the execution time needed when performing arithmetic operations and logical evaluations.
- Functional Features: Wrapper classes come with built-in methods which allow you to perform various operations, such as conversion or parsing. For instance, converting a to an can be done with ease using unlike the primitive which has no such functionality.
- Nullability: Wrapper classes can be assigned , which provides flexibility in programming. This can support more complex applications where the absence of a value might be meaningful. However, working with null can lead to exceptions if not handled correctly, unlike primitives that will always hold a default value.
Remember: While wrapper classes enhance functionality, they impose a cost on memory and performance. Assessing these trade-offs is essential when making design decisions.
Overall, when choosing between wrapper classes and primitives, consider the trade-offs carefully. Often, using primitives can be more efficient in terms of both performance and memory, especially in tight loops or performance-sensitive applications. But if object equality, method functionalities, or collection compatibility is necessary, wrapper classes can be the better choice. The key is to strike the right balance for your unique coding scenario.
Common Misconceptions about Wrapper Classes
When diving into the world of Java and its features, wrapper classes frequently spark questions and confusion, particularly among new programmers. These misconceptions can lead to misunderstandings about how to effectively utilize wrapper classes in real-world applications. Setting the record straight is vital because clarity on this topic not only enhances understanding but also improves coding practices.
Wrapper Classes vs. Primitives
A significant misconception is that wrapper classes are always a better choice than primitive data types. It's also tempting to think that whenever you need an object, you should reach for a wrapper class. However, there’s more to the story.
Primitive types like , , or are more efficient in terms of memory and speed. They reside directly within the stack, allowing for faster manipulation compared to wrapper classes, which are objects stored in the heap. This creates overhead, distance, and some lag when invoking methods.
In many cases, wrapper classes are useful mainly when operations require the object nature of data types, such as in collections where only objects can be stored. For example:
- Efficiency: Primitive types are generally quicker to access.
- Flexibility: Wrapper classes can be used in scenarios where primitives can’t, like utilizing generics or collections.
By utilizing both properly, programmers can balance performance and capability effectively. It's all about choosing the right tool for the job.
Immutability of Wrapper Objects
Another common misconception circles around the idea that wrapper objects can be altered like their primitive counterparts. This confusion often stems from a misunderstanding of what immutability actually means in this context.
In Java, wrapper classes like , , , and are immutable. This means that once an object is created, its value cannot be changed. If you need a different value, you create a new object instead. For example:
Many developers mistakenly believe they can simply change the state of a wrapper object. This could lead to errors in coding and unwanted behavior in applications. Recognizing immutability helps in understanding how Java handles objects and can inform better design choices.
Important Point: The immutability of wrapper classes ensures thread safety, as any instance can safely be used across different threads without risk of unexpected changes.
Culmination
In this article, we have journeyed through the multifaceted world of wrapper classes in Java, uncovering their fundamental role in object-oriented programming. Understanding wrapper classes is not mere academic curiosity but a necessity for anyone serious about mastering Java. They offer a bridge between primitive data types and objects, allowing us to leverage the best of both worlds.
Summary of Key Points
Reflecting on the discussions we've had, a few key points stand out:
- Wrapper Classes Enable Object Manipulation: By converting primitive types into objects, wrapper classes allow developers to utilize the exhaustive capabilities of Java's object-oriented nature, such as in collections or when working with methods that require objects instead of primitives.
- Boxing and Unboxing: The ease of transitioning between primitives and their corresponding wrapper classes simplifies coding. Boxing automatically converts primitives into objects, while unboxing facilitates the opposite.
- Practical Applications: From enhancing collections to providing compatibility with generics, wrapper classes prove valuable in real-world applications.
- Performance Considerations: While wrapper classes offer flexibility, it’s crucial to consider their memory overhead compared to primitive types, which can have implications for performance, particularly in resource-constrained environments.
- Common Misconceptions: It’s important to clarify that while wrapper classes share the same data representation as their primitive counterparts, they offer additional functionality and ensure immutability.
These points elucidate why wrapper classes are indispensable in Java programming and how they enrich the development experience.
Future of Wrapper Classes in Java
Looking ahead, the role of wrapper classes in Java appears poised for growth, particularly as the language evolves and more developers engage with frameworks that rely on object-oriented principles. Current trends suggest a continuing emphasis on performance and efficiency.
- Potential Innovations: As Java incorporates functional programming features with Lambda expressions and streams, the usage of wrapper classes could transform even further. For instance, the integration with APIs might require deeper understanding and optimized use of wrapper structures.
- Growing Demand for Robust Applications: With an increasing push for complex applications, the reliability of wrapper classes to manage data types will be paramount. The future will likely see enhancements in extra functionalities or even new, specialized classes that further abstract or optimize the interaction with primitives.
- Educational Focus: As more educational institutions and coding bootcamps include masterclasses on Java, the foundational knowledge about wrapper classes will keep becoming crucial for learners of all levels.