Web Application Creation: A Comprehensive Guide
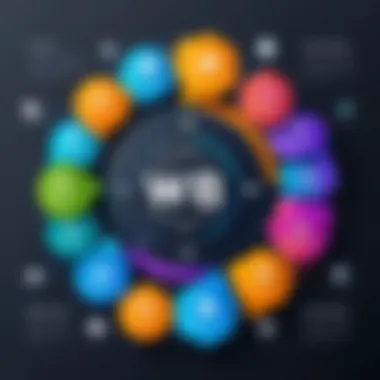
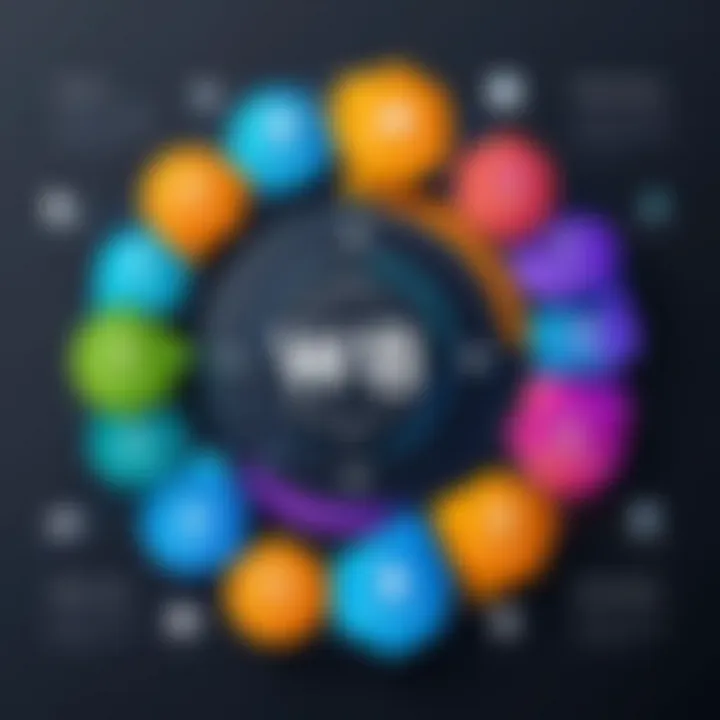
Intro
Creating a web application is a multifaceted process that requires both technical proficiency and a clear understanding of user needs. The design and implementation of web applications can largely define their success or failure in the marketplace. This guide aims to cover essential aspects of the process, making it approachable for beginners and intermediate learners.
Prologue to Programming Language
Understanding programming languages is fundamental for anyone attempting to build web applications. Languages like JavaScript, Python, and Ruby dominate the landscape due to their versatility and community support.
History and Background
The concept of programming languages has evolved significantly since its inception. Initially, there were low-level languages which closely matched machine code. However, as needs grew more complex, higher-level languages emerged to simplify code writing and enhance developer productivity.
Features and Uses
Programming languages differ widely in their features. Some key aspects include:
- Syntax and Semantics: This defines the rules for writing code.
- Abstraction Levels: Higher-level languages abstract more complexity from the user.
- Libraries and Frameworks: They provide reusable code for common tasks.
Popularity and Scope
Certain programming languages have gained traction due to their efficiency and ease of use. For instance, JavaScript is essential for web development. It allows creators to make interactive web pages, which are vital for user engagement.
Basic Syntax and Concepts
In learning any programming language, starting with the basic syntax and concepts will lay a strong foundation.
Variables and Data Types
Variables are placeholders for data. Different programming languages utilize various data types, including:
- Integers: Whole numbers.
- Strings: Textual data enclosed in quotes.
- Booleans: True/False values.
Operators and Expressions
Operators perform operations on variables and values. Types of operators include:
- Arithmetic Operators: Such as +, -, *, and /.
- Logical Operators: Such as && (and), || (or).
Control Structures
Control structures direct the flow of execution in code. Common structures are:
- If Statements: Allow conditional execution of a block of code.
- Loops: Such as for and while, used for repeated execution.
Advanced Topics
Once the basics are mastered, diving into advanced topics becomes necessary.
Functions and Methods
Functions are reusable blocks of code. They make programs modular and easier to maintain. Defining a function typically requires a name, parameters, and a body that contains the code to execute.
Object-Oriented Programming
Object-oriented programming (OOP) adds another layer of flexibility. Key concepts in OOP include:
- Classes: Blueprints for objects.
- Objects: Instances of classes.
- Inheritance: Mechanism to create new classes using existing ones.
Exception Handling
Error handling is crucial in application development. Using try-catch blocks can help manage exceptions and ensure the application does not crash unexpectedly.
Hands-On Examples
Practical application reinforces learning. Building sample projects can bridge the gap between theory and practice.
Simple Programs
Start with simple programs like a calculator or a to-do list app. They help instill fundamental programming concepts.
Intermediate Projects
Once comfortable, try creating a small web application with a front-end and back-end component. This could include user authentication and database connections.
Code Snippets
Here’s an example of a basic JavaScript function:
Resources and Further Learning
For continued growth in web application development, here are some valuable resources:
Recommended Books and Tutorials
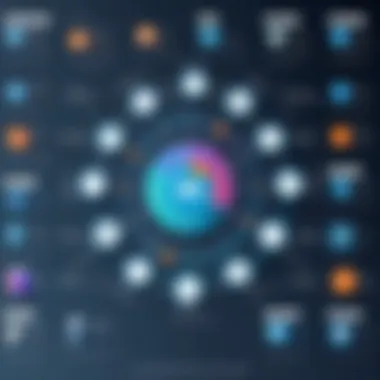
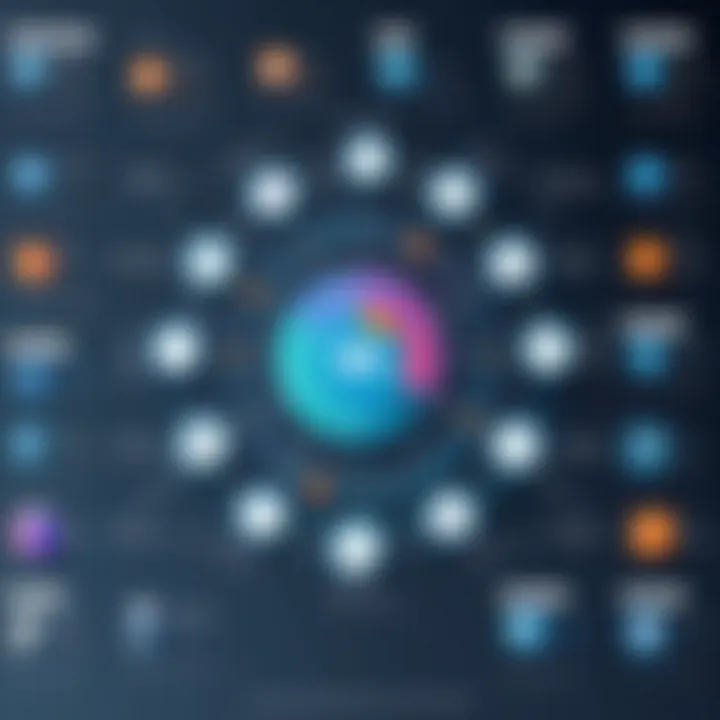
- "Eloquent JavaScript" by Marijn Haverbeke
- "You Don’t Know JS" by Kyle Simpson
Online Courses and Platforms
- Coursera offers various programming courses.
- FreeCodeCamp allows you to learn coding through projects.
Community Forums and Groups
Engaging with communities, such as Reddit or Stack Overflow, can provide additional support. These platforms are useful for troubleshooting and networking.
Understanding Web Applications
In today’s digital landscape, web applications play a crucial role. Understanding this topic is essential for anyone involved in tech development. A web application allows users to perform specific tasks via the internet. These applications create a seamless interaction between users and services, making them integral in the daily operations of both individuals and businesses.
The key aspects to study include their definitions, types, and functionalities. Knowing these elements encourages more informed decisions. By examining the structure and utility of web applications, learners can identify potential applications relevant to their needs. Additionally, comprehending the evolution of web technologies enhances strategic thinking.
Definition of Web Applications
A web application is a program that runs on a web server. Unlike traditional desktop applications, users do not need to install them on their devices. Instead, they access them via a web browser. This distinct feature provides flexibility. Users can interact with the app from anywhere, as long as they have an internet connection. Popular examples include Google Docs and online banking portals.
A web application functions through a combination of server-side and client-side processes. Server-side is responsible for data processing and storage, while client-side is all about user interaction. The interplay between these two environments enables dynamic content generation and a personalized user experience.
Common Types of Web Applications
There are various kinds of web applications, each serving different purposes. They can be categorized as follows:
- Single Page Applications (SPAs): These load a single HTML page and dynamically update as users interact. Frameworks such as Angular and React are frequently used to build SPAs.
- Dynamic Web Applications: These employ server-side languages to generate content dynamically. Examples include content management systems like WordPress.
- E-commerce Applications: Online stores like Amazon exemplify this type. They facilitate transactions and integrate payment systems.
- Social Networking Applications: Platforms like Facebook allow users to create profiles and share content. They focus on user interaction and community building.
- Progressive Web Applications ( PWAs): These combine the best of web and mobile apps, offering offline capabilities and high performance. They act like a native application but run in a web browser.
Each type comes with unique features and advantages, contributing to the web ecosystem’s diversity.
Planning the Web Application
Planning the web application is a crucial phase in the development lifecycle. This step provides clarity on the project’s direction and helps prevent issues that may arise later in the process. Effective planning allows developers to anticipate potential challenges and streamline the workflow. It is vital to approach this stage with a clear perspective and understanding of the project objectives.
Requirement Analysis
Requirement analysis involves identifying what the users need from the web application. This step is about gathering and documenting the specifications and ensuring every stakeholder’s voice is captured in the process. Engaging with users and conducting surveys during this stage is beneficial. It helps in collecting important information that shapes the application's features.
Moreover, a thorough analysis prevents any misalignment between what users expect and what is delivered. If requirements are overlooked, it can result in significant backtracking later on. Identifying technical and functional requirements early on also assists in estimating resources and timelines effectively.
Identifying User Needs
Identifying user needs goes hand in hand with requirement analysis. Understanding who will use the application is essential to tailor the design and functionality to fit the target audience. User personas are often created during this phase. These personas represent various user types and help in visualizing their challenges and goals.
Interviews and feedback sessions can also be useful tools for gathering insights. It is important to ask users about their preferences and frustrations with existing applications. By doing this, developers can create a solution that directly addresses these pain points. Keeping the user at the center of development ensures greater adoption and satisfaction once the application is launched.
Defining Objectives and Goals
Defining objectives and goals provides a roadmap for the project. These should align with the overall vision for the application and reflect both short-term and long-term aims. Well-defined goals help in measuring the success of the project post-launch.
Setting specific, measurable, achievable, relevant, and time-bound (SMART) goals is often recommended. For instance, a goal might be to increase user engagement by 20% within six months of launching the app. Clear objectives guide decision-making throughout the development process and help in resource allocation.
Choosing the Right Technology Stack
Choosing the right technology stack is fundamental in the development of a web application. It encompasses a combination of programming languages, frameworks, libraries, and tools that collectively determine how the application will be built. The choice of technology affects not only the efficiency of development but also the application’s performance, scalability, and maintainability. Understanding the specific requirements of the project is essential in making informed decisions.
When selecting a technology stack, several key factors should be taken into account. These include:
- Project requirements: Different applications require different technologies. For instance, a real-time data application might necessitate specific frameworks that support WebSocket technology.
- Team expertise: The existing skills within the development team can influence the technology decision. Sticking to familiar tools can enhance productivity and reduce learning curves.
- Community support: A strong community backing a technology can provide valuable resources, libraries, and help. Technologies with larger communities are often more reliable due to continuous support and updates.
- Future maintenance: Some technologies are easier to maintain than others. This is crucial in planning for long-term support and updates of the application.
The right technology stack can drastically speed up development and improve the overall user experience.
Frontend Technologies
Frontend technologies are instrumental in crafting the user interface of web applications. They are responsible for everything the user interacts with directly. Here are some primary technologies used in frontend development:
- HTML: HyperText Markup Language is the backbone of every web application. It structures the content.
- CSS: Cascading Style Sheets enhance the visual presentation of HTML elements. Responsive design is achieved through frameworks such as Bootstrap or Foundation.
- JavaScript: This programming language adds interactivity and dynamic content to applications. Popular libraries and frameworks like React, Angular, and Vue.js provide additional functionalities and streamline development.
A well-designed frontend enhances user satisfaction and drives engagement.
Backend Technologies
Backend technologies are crucial for server-side application logic and database management. They manage how data is created, stored, and accessed. Some widely used backend technologies include:
- Node.js: A JavaScript runtime that enables building scalable network applications.
- Python (Django or Flask): Known for its simplicity and readability, facilitating rapid development.
- Ruby on Rails: A framework that emphasizes convention over configuration, speeding up the development process.
- Java (Spring): A robust environment for building enterprise-grade applications.
Selecting an appropriate backend technology ensures security and performance.
Database Solutions
Databases are essential for storing and retrieving data in web applications. Choosing the right database solution can profoundly influence application performance and scalability. Two primary categories exist:
- Relational Databases: Systems like MySQL and PostgreSQL use structured query language (SQL) for defining and manipulating data. They are ideal for applications requiring strong consistency.
- NoSQL Databases: Solutions like MongoDB and Cassandra are more flexible and can handle unstructured data. They cater to applications with varying data structures and large scale data processing.
Consider the project scope and data requirements while choosing a database.
Considerations for API Integration
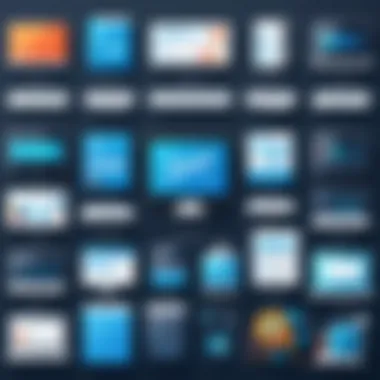
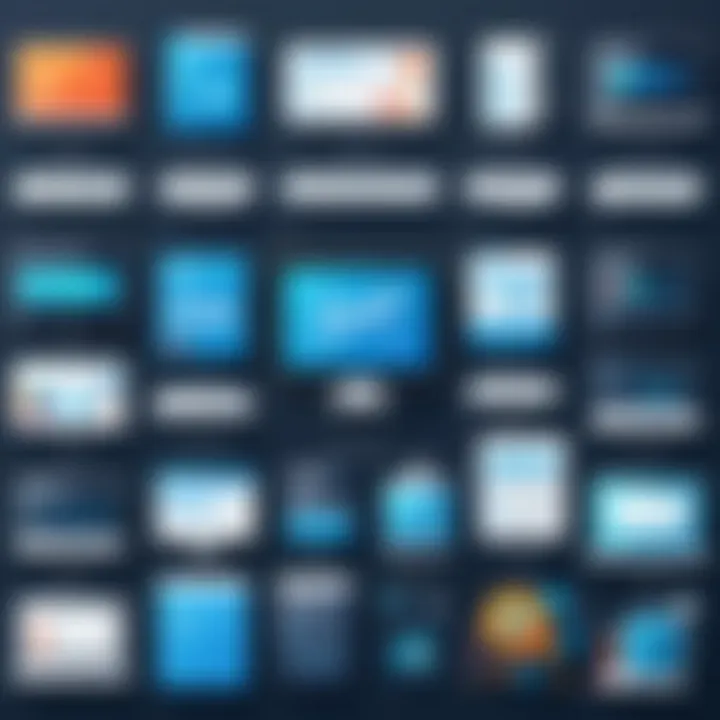
APIs (Application Programming Interfaces) allow different software components to communicate. Integration with APIs is a vital aspect of modern web applications. Effective API integration can provide enhanced functionalities such as:
- Data exchange: Facilitates interaction with external data sources or services (e.g., social media APIs).
- Modular architecture: Encourages separation of services, making the application scalable and easier to manage.
- Third-party services: Provides access to functionalities like payment processing or authentication without building features from scratch.
When selecting APIs, consider factors such as authentication methods, response times, and usage limitations.
A well-chosen technology stack lays the foundation for success in web application development.
Web Application Design Principles
Understanding web application design principles is crucial for creating effective and user-centric applications. These principles ensure that the application is visually appealing, easy to navigate, and functions well across various devices. Prioritizing these aspects can enhance user satisfaction, boost engagement, and ultimately drive better results. In the competitive landscape of web applications, ignoring design principles is a risk that can lead to user frustration and reduced functionality.
User Interface Design
User interface (UI) design plays an essential role in how users interact with a web application. It includes the layout, visual elements, and overall aesthetic appeal. A well-thought-out UI makes it easier for users to accomplish tasks. This includes careful consideration of color schemes, typography, and button placements.
To create a successful UI design, consider the following:
- Consistency: Maintain a uniform design throughout the application to enhance usability.
- Intuitiveness: Users should understand how to interact with the application without needing extensive instructions.
- Feedback: Provide users with immediate feedback for their actions to promote a seamless experience.
A well-designed user interface helps establish trust and connection with the audience, resulting in better retention and satisfaction.
User Experience Considerations
User experience (UX) encompasses all aspects of end-user interaction with the company, its services, and its products. This concept extends beyond simple aesthetic design. A compelling UX fosters positive feelings and satisfaction among users. To focus on UX, designers must consider various elements:
- Understanding User Goals: Prioritize the needs and expectations of users to align the application's function with its intent.
- Navigational Structures: Implement intuitive navigation paths for users, ensuring they can move through the application effortlessly.
- Accessibility: Ensure that the application is usable for all individuals, including those with disabilities. Using semantic HTML elements can improve accessibility.
Improving user experience leads to higher adoption rates, as users are more likely to return to an application they find easy and enjoyable to use.
Responsive Design Techniques
Responsive design is another essential principle of web application design. This approach ensures that the application performs well on a variety of devices, from desktops to smartphones. A responsive design eliminates the frustration of having to zoom in or scroll excessively on smaller screens. Techniques for achieving responsive design include:
- Fluid Grid Layouts: Utilize percentages for width rather than fixed pixel dimensions, allowing elements to scale smoothly across different screen sizes.
- Media Queries: Implement CSS media queries to apply different style rules based on device characteristics.
- Flexible Images: Ensure that images can resize within their containing elements without losing quality or style.
Responsive design not only improves overall user experience, but it also enhances search engine optimization, as search engines favor mobile-friendly sites.
Effective design is not just about making things pretty—it’s about making things work well.
In summary, web application design principles are an integral part of application development. They influence both user interface and user experience, which in turn affect user satisfaction and retention. By adopting these principles, developers and designers can create web applications that are not only functional but also enjoyable to use.
Development Best Practices
In the realm of web application creation, adhering to development best practices is essential. These practices ensure that the application is not only functional but also maintainable, scalable, and secure. Developers who integrate these practices into their workflow can enhance productivity and reduce the likelihood of issues arising in the future. Next, we will explore key elements such as version control systems, coding standards, and testing strategies which all play a crucial role in achieving high-quality web applications.
Version Control Systems
Version control systems (VCS) are crucial for managing code changes in web application development. Tools like Git allow multiple developers to collaborate efficiently. With Git, developers can track changes, revert to previous versions, and manage branches for feature development. This ensures that the main codebase remains stable while new features are being added.
Some benefits of using VCS include:
- Collaboration: Multiple developers can work on the same project simultaneously.
- History Tracking: Every change is recorded, allowing for easy reviews and rollbacks.
- Branching: New features can be developed in isolated branches, minimizing disruptions.
"A well-implemented version control system can prevent conflicts and streamline collaboration, making it a fundamental practice in modern web development."
Coding Standards and Conventions
Coding standards define a set of guidelines that developers agree to follow when writing code. This practice ensures that the codebase remains consistent and understandable, making it easier for everyone involved in the project. Languages like JavaScript, Python, and Ruby have established conventions that can be adopted alongside tools for automated linting and formatting.
Benefits of maintaining coding standards include:
- Readability: Code is easier to read and understand, facilitating collaboration.
- Maintenance: Consistent code is simpler to maintain and update.
- Error Reduction: Standards help reduce common errors and improve code quality.
New developers, in particular, benefit from following coding conventions. This guidance can accelerate their learning process and help integrate them into the team more quickly.
Testing Strategies
Testing is an integral part of web application development. A well-defined testing strategy can identify bugs and issues before they reach production. There are several types of testing techniques, including unit testing, integration testing, and user acceptance testing. Effective testing ensures the application works as expected and that user needs are met.
Aspects to consider when implementing testing strategies:
- Automated Testing: Implementing frameworks such as Jest or Selenium can automate repetitive tasks, enhancing efficiency.
- Test-Driven Development (TDD): Writing tests before coding encourages better design and reduces the number of bugs.
- Continuous Testing: Incorporating automated tests into the development pipeline aids in catching issues early in the lifecycle.
In summary, adopting development best practices like version control, coding standards, and effective testing will significantly enhance the overall quality of web applications. These practices not only lead to better software solutions but also foster a productive environment for development teams.
Deployment of Web Applications
Deployment of web applications is a crucial phase in the development lifecycle. It marks the transition of an application from the development environment to a live, user-facing scenario. Understanding this process is essential for ensuring that web applications function optimally post-launch. Moreover, successful deployment minimizes risks related to downtime and bugs.
Key considerations include:
- Stability: Ensuring the application behaves as expected.
- Scalability: The ability to handle varying loads efficiently.
- Security: Protecting data and complying with regulations.
Selecting the correct environment for deployment is foundational to achieving these goals. The subsequent sections will delve into the specifics of deployment environments and practices that facilitate a smoother transition.
Deployment Environments
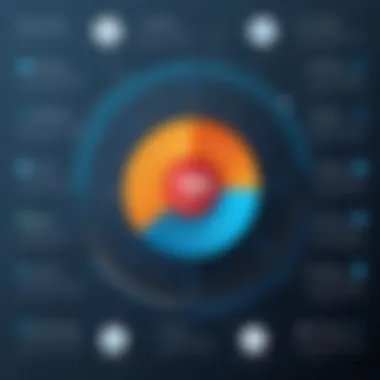
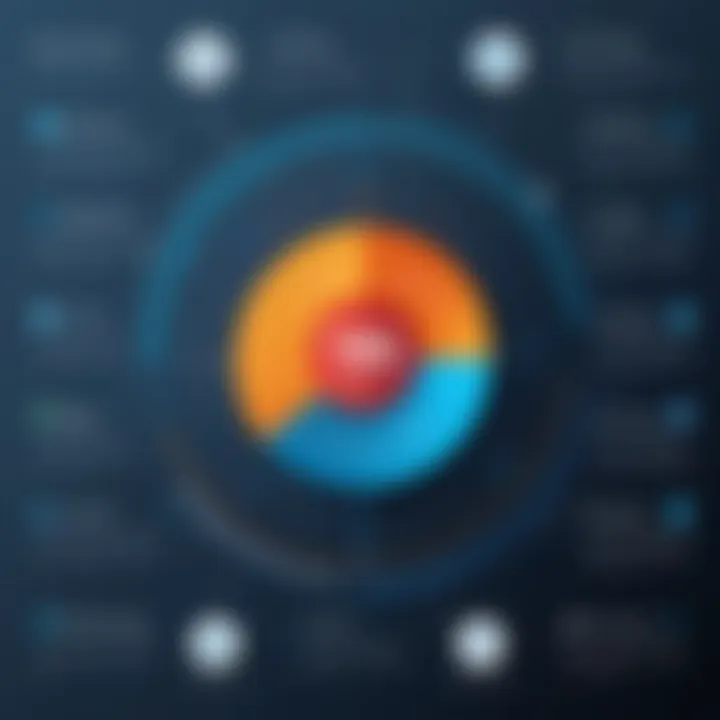
Deployment environments refer to the various settings where web applications are hosted and run. This may range from simple local servers for development and testing to complex cloud infrastructures for production. Each environment serves a distinct purpose:
- Development Environment: Used for building and testing new features, allowing developers to make changes without affecting live users.
- Staging Environment: Mirrors the production environment. It is used for final testing before deployment to ensure everything works properly once live.
- Production Environment: The live environment where the application is available to users. This environment must be stable and monitored continuously.
"Choosing the right environment can greatly influence the application’s performance and reliability, making it essential for developers to understand the nuances of each stage."
Maintaining a clear distinction between these environments is vital. It prevents errors during development and ensures users always experience a polished version of the application.
Continuous Integration and Continuous Deployment
Continuous Integration (CI) and Continuous Deployment (CD) are practices that contribute to effective deployment strategies. CI involves automatically testing changes in the code base before they are merged. This minimizes potential issues by catching errors early.
CD, on the other hand, refers to the release of code changes to production automatically after passing predefined tests. This tightens the feedback loop and ensures that updates can be delivered promptly.
Some advantages of implementing CI/CD include:
- Faster Deployment Cycles: Reduces the time between writing code and deploying it.
- Improved Code Quality: Continuous testing enhances code reliability.
- Greater Efficiency: Teams can focus on building features rather than managing deployment processes.
By integrating CI/CD practices into the development plan, teams can streamline their workflow and deliver applications that meet high-quality standards while responding quickly to user needs.
Ultimately, understanding the significance of deployment environments and CI/CD practices is essential for anyone involved in web application creation. These practices not only facilitate deployment but also lay the groundwork for ongoing maintenance and improvement of applications.
Post-Deployment Maintenance
Post-deployment maintenance is critical for the longevity and effectiveness of web applications. This phase ensures that applications remain functional and relevant after their initial launch. Regular maintenance contributes to improved performance, security, and user satisfaction. It is an ongoing process that involves addressing issues as they arise and implementing updates as needed, which can enhance the overall user experience. Without sustained maintenance, applications risk becoming obsolete, insecure, or buggy.
Regular Updates and Patching
Keeping software up to date is essential in web application development. Regular updates and patching can address vulnerabilities and bugs that might be exploited by malicious users. If left unchecked, these issues can compromise user data and overall application integrity.
Benefits of Regular Updates:
- Security: Updates often contain crucial security patches. Being proactive in applying these updates minimizes risks of breaches.
- Performance Enhancements: New versions may improve speed, efficiency, and compatibility with modern technology.
- New Features: Updates can introduce new functionalities that enhance the user experience and keep the application competitive.
To establish a solid updating routine, consider:
- Setting defined schedules for updates.
- Monitoring release notes from technology vendors.
- Testing updates in a staging environment before full deployment.
Monitoring Performance and Security
Once a web application is deployed, continuous monitoring is necessary. Both performance and security monitoring should be integral parts of post-deployment maintenance. By observing these factors regularly, developers can identify issues before they escalate.
Aspects of Monitoring:
- Performance Metrics: Track loading times, server uptime, and response times to ensure the application functions smoothly. Tools like Google Analytics or Application Performance Management (APM) solutions can assist in this regard.
- Security Threat Detection: Employ tools that scan for vulnerabilities and anomalies in real-time. Implementing firewalls, intrusion detection systems, and regular security audits can act as preventative measures.
Staying ahead of potential threats can save organizations from reputational damage and customer loss.
Overall, diligent post-deployment maintenance is not merely an afterthought but a strategic necessity in web application development. Engaging in regular updates and continuous performance and security monitoring fosters a resilient web application. This ongoing commitment ensures that the application not only serves its intended purpose but can adapt to changing technologies and user expectations.
Challenges in Web Application Development
Web application development is a multifaceted process. Various challenges accompany this endeavor. Addressing these challenges effectively is vital for a successful project. Understanding these hurdles can also lead to more efficient solutions. In this section, we will explore the main categories of challenges faced during web application development: technical challenges and user adoption barriers.
Technical Challenges
Technical challenges in web application development can significantly impact timelines and budgets. Some of the primary technical issues include:
- Scalability: As user demand increases, applications must scale effectively. If the architecture is not designed for growth, performance may degrade, leading to a poor user experience.
- Security: Cyber threats are a constant concern for any web application. Protecting user data and maintaining privacy is a legal and ethical obligation. Developers must stay updated on the latest security vulnerabilities and apply best practices to ensure safety.
- Integration: Many applications today rely on third-party services and APIs. Ensuring seamless integration while managing data flow can become complex, requiring additional development effort.
- Cross-Browser Compatibility: Different browsers may render applications differently. Developers need to continually test and modify their applications to ensure a uniform experience across platforms.
- Performance Optimization: Slow loading times can lead to user abandonment. Developers must find ways to minimize load times and ensure the application runs smoothly.
Overcoming these technical challenges often requires a solid understanding of the latest technologies and best practices. It is crucial to invest time in identifying potential issues early in the development cycle and to plan accordingly.
User Adoption Barriers
User adoption barriers can be just as detrimental as technical difficulties. Even the best web applications can fail if users do not engage with them. Here are common barriers to user adoption:
- User Interface Complexity: If an application is difficult to navigate, users may become frustrated. A straightforward, intuitive design is essential to encourage engagement.
- Lack of Education: Users may not fully understand the features or benefits of the application. Providing adequate documentation or tutorials can help users to see the value.
- Resistance to Change: Users often prefer familiar tools. If a new application requires significant changes in habits or processes, it may face initial resistance.
- Performance Issues: If users encounter performance problems, they are likely to abandon the application. Thus, addressing technical issues can also enhance user adoption rates.
- Limited Support: Users may feel discouraged if they encounter issues and cannot find help. Providing robust customer support can mitigate this barrier.
In summary, understanding challenges in web application development is crucial. Both technical challenges and user adoption barriers can influence the success of a web application. Focusing on these issues during the planning and ongoing development phases can lead to more adaptable and user-friendly applications. Building a web application is not just about the code; it is also about the people who will use it.
Future Trends in Web Application Development
The landscape of web application development continually evolves to meet the needs of users and businesses. Understanding future trends is crucial for developers, as these trends can impact everything from design choices to the technologies used. By staying informed, developers can adopt practices that enhance efficiency, improve user satisfaction, and ensure long-term success.
Emerging Technologies
Emerging technologies are reshaping the web application environment. Some of the most notable advancements include:
- Artificial Intelligence (AI): Integrating AI into web applications enhances user interaction. AI algorithms improve personalization, automate tasks, and optimize performance. For instance, chatbots can handle customer inquiries around the clock.
- Progressive Web Apps (PWAs): PWAs blend web and mobile app experiences. They work offline and load quickly, providing an improved user experience. This capacity to function without a continuous internet connection broadens user access.
- WebAssembly: WebAssembly enables high-performance applications on the web. It allows developers to run code written in multiple languages, resulting in better performance for complex applications.
- Serverless Architecture: This approach allows developers to build and deploy applications without managing servers. It reduces operational costs while streamlining the deployment process. This model enhances scalability and flexibility for web applications.
Investing time in familiarizing with these technologies is beneficial. They can strengthen a developer’s toolkit and increase the impact of the applications created.
Shifts in User Expectations
As technology advances, user expectations continue to shift dramatically. Users anticipate more engaging, faster, and more intuitive web applications. Key shifts include:
- Speed and Performance: In today's fast-paced digital world, users expect applications to respond instantly. A delay of even a few seconds may lead to frustration and abandonment.
- Mobile-First Design: Mobile devices dominate internet access. Developers must prioritize mobile-first design, ensuring websites function seamlessly on all screen sizes.
- Security and Privacy: With the rise in data breaches, users are increasingly concerned about their personal information. Web applications must implement stringent security measures to protect user data effectively.
- Customization and Personalization: Users prefer tailored experiences. Applications that adapt content and services to individual preferences are more likely to succeed in retaining users.
Meeting these shifting expectations becomes essential for lasting user engagement. Harnessing feedback and user data can guide developers in making informed decisions.
"The future of web application development relies on our ability to adapt to technological advancements and user expectations."
Overall, acknowledging and responding to these future trends can significantly affect the effectiveness of web applications. Staying informed and proactive allows developers to navigate the evolving landscape with confidence.