Effective Email Address Validation Using JavaScript
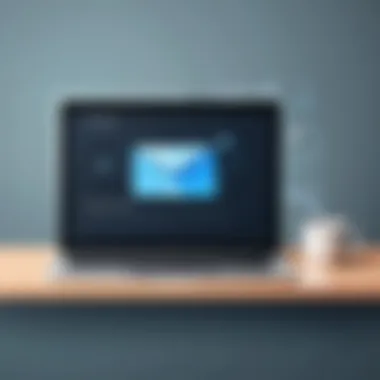
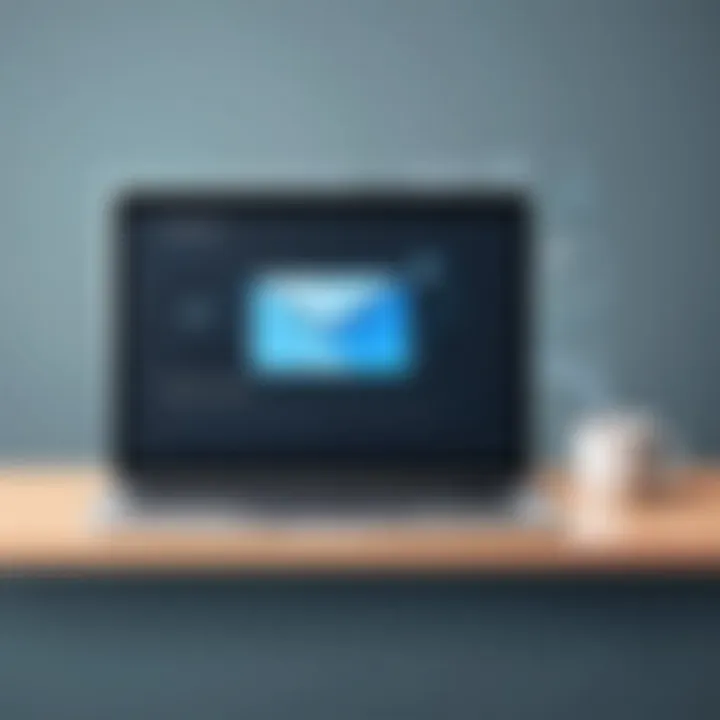
Intro
Understanding how to validate email addresses is key in today's digital age. Email is a primary means of communication, and ensuring the format is correct is crucial for web applications. JavaScript, the brain behind many interactive web experiences, provides robust methods for this task.
The significance of email validation goes beyond merely checking the syntax. It can enhance the user experience by preventing errors and streamlining processes. In web applications, incorrect inputs can lead to frustration, resulting in higher dropout rates. Therefore, validating emails effectively is not just a technical necessity; it's about delivering a smooth experience for users.
This article explores the methods and nuances of email validation using JavaScript. We will cover the fundamentals, such as the typical formats of email addresses, and delve into advanced techniques like using regular expressions. By doing this, readers will gain practical insights that can be applied directly to their projects.
Foreword to JavaScript
History and Background
JavaScript emerged in the mid-1990s, crafted by Brendan Eich while he was at Netscape. Originally known as Mocha, it quickly transformed into an essential web language. Unlike other programming languages that focus solely on server-side tasks, JavaScript brings interactivity and dynamism to web pages, playing an integral role in shaping modern web development.
Features and Uses
JavaScript boasts a unique combination of features:
- Versatile: It works seamlessly across all major web browsers.
- Event-driven: It can respond to user actions like clicks and keyboard inputs, creating responsive interfaces.
- Rich ecosystem: JavaScript offers numerous libraries and frameworks, such as React and Angular, expanding the possibilities for developers.
These characteristics make JavaScript a top choice for client-side scripting. As the web evolved, so did JavaScript, now also powering server-side applications with the help of Node.js.
Popularity and Scope
The popularity of JavaScript is undeniable. It consistently tops surveys regarding the most-used programming languages. With a vibrant community supporting it through forums like Reddit and developer-friendly resources, itās no wonder that new developers are encouraged to learn it.
Basic Syntax and Concepts
Variables and Data Types
At its core, JavaScript employs versatile data types. From strings that can house text to numbers used in calculations, understanding these is the foundation of any project. Letās take a quick look:
- String: Represents textual data. Example:
- Number: Represents numeric values. Example:
- Boolean: Represents true or false values. Example:
Operators and Expressions
Operators manipulate values to perform calculations or comparisons. JavaScript includes:
- Arithmetic Operators: For basic math tasks, like , , , and .
- Comparison Operators: Such as or for checking equality or difference.
Control Structures
Control structures allow control of the flow in the program. statements, loops, and case constructs guide the logic and handling of different situations, especially useful when validating email addresses.
Advanced Topics
Functions and Methods
Functions encapsulate code to perform specific tasks, making the code reusable and organized. For email validation, a function might check if the provided email matches a valid regex pattern.
Object-Oriented Programming
JavaScript supports object-oriented programming. This allows for the creation of objects that can represent users or form submissions, enhancing the organization and modularity of the code base.
Exception Handling
Part of keeping a smooth user experience is handling errors gracefully. JavaScript includes statements to manage exceptions, critical when validating inputs that might not adhere to expected formats.
Hands-On Examples
Simple Programs
Imagine a simple email validation script:
This function uses regex to test if the email is valid, returning or accordingly.
Intermediate Projects
Creating a full form that includes email validation can be a great project. You can add input fields for the user to enter their email, then call the function when a user submits the form.
Code Snippets
Learning through snippets is beneficial. Here's how one might proceed on an input field change:
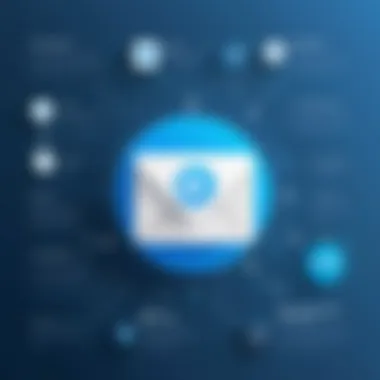
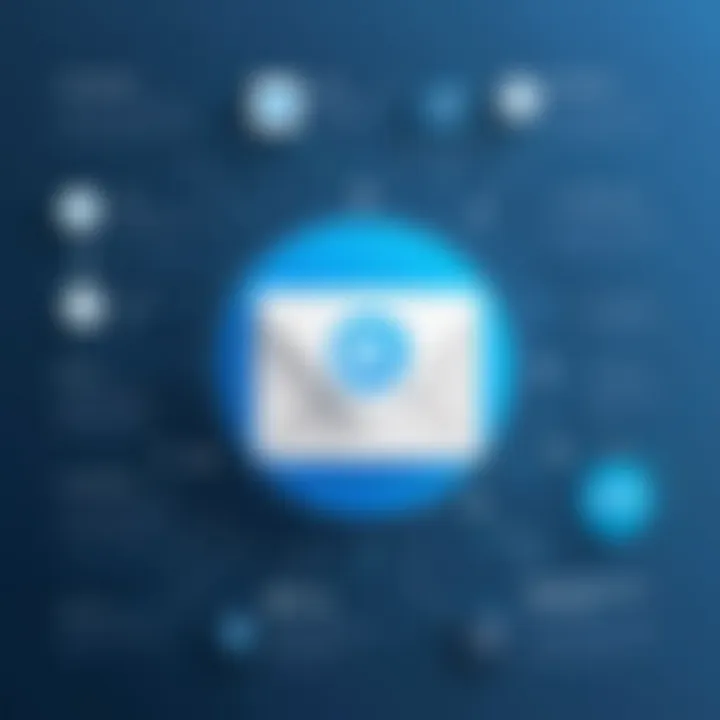
Resources and Further Learning
Recommended Books and Tutorials
Several resources can help you dive deeper into JavaScript and email validation. Websites like Wikipedia and Britannica offer introductory content.
Online Courses and Platforms
Platforms such as Coursera and freeCodeCamp provide structured courses to advance your skills further.
Community Forums and Groups
Engaging with communities can enhance learning. Consider joining groups on Facebook or platforms like Stack Overflow where you can ask questions and share experiences.
Prelims to Email Validation
In today's digital landscape, validating email addresses is no longer a mere afterthought; it's a critical component of robust web applications. With most interactions happening online, a valid email is often the gateway for user registration and communication. Ensuring that an email address adheres to accepted formats not only fosters effective communication but also enhances user experiences. When users sign up on a platform or receive notifications, there's an underlying expectation that their email will receive pertinent information without issues. The importance of valid email addresses extends beyond correctness; it influences the legitimacy and trustworthiness of the service being offered.
Importance of Valid Email Addresses
Valid email addresses serve various functions. For one, they prevent communication failures. Imagine a user enrolling for a newsletter but specifying their email incorrectly. If the address is invalid, that user won't receive critical updates or offers, resulting in frustration.
Furthermore, a reliable email validation process can significantly reduce fraud. Businesses often rely on the accuracy of user information to segment their audience and tailor content. An incorrect email can skew analytics and lead to misguided marketing strategies, wasting resources.
Beyond functionality, valid email addresses enhance the user experience. Users who encounter seamless interactions without error messages are more likely to feel confident and satisfied, which translates to higher retention rates. Thus, validating email addresses is not merely a technical necessityāitās about creating an environment where users feel valued and respected.
"Every mistake in communication is a lost opportunity to connect."
Challenges in Email Validation
While the importance of validating email addresses is clear, the road to effective validation is riddled with challenges. One major hurdle is the sheer variety of email formats accepted. With a plethora of service providers, from Gmail and Yahoo to custom business domains, the range of valid configurations can be dizzying. Some users might utilize uncommon characters or formats that standard validation mechanisms fail to recognize.
Another challenge involves balancing strictness and flexibility. An overly restrictive validation process can alienate users. Consider this: if the validation script rejects a legitimate email simply because it lacks certain punctuation or domain suffix, it throws a wrench into the user experience. Users tend to abandon forms that are too critical of input.
Moreover, issues of internationalization pose a unique challenge. With the advent of global communication, email addresses are now beginning to include non-Latin characters, which many. classic validation patterns do not account for. Launching a validation system that successfully navigates these complexities requires a nuanced approach, blending technical precision with a keen understanding of user behavior.
Overview of JavaScript for Validation
JavaScript plays a crucial role in validating email addresses, serving as a bridge between user intentions and the technical requirements of web applications. Its ubiquity ensures that web applications can perform validations swiftly and efficiently, both enhancing user experience and reducing the likelihood of errors during data entry. But what makes JavaScript so appealing for this purpose? Let's delve deeper into its importance.
Why Use JavaScript?
There are several compelling reasons to choose JavaScript for email validation. Here are a few points to consider:
- Immediate Feedback: JavaScript operates on the client side, which means users receive instant feedback as they type. This real-time validation is crucial in preventing form submission with incorrect data and can guide users to correct mistakes before they even hit the submit button.
- Resource Efficiency: By performing validation on the client side, you reduce server load. This can speed up the process, making it less burdensome for your server. Hence, users enjoy quicker interactions with your application.
- User Engagement: A web application that anticipates user errors and addresses them promptly fosters a more engaging experience. Instead of getting frustrated by unfriendly error messages post-submission, users can smoothly complete their tasks if the application guides them through it.
"Client-side validation acts like a friendly conversational partner, ensuring that the input process feels less like a chore and more like a guided interaction."
Client-Side vs Server-Side Validation
Understanding the roles of client-side and server-side validation is fundamental in creating robust and user-friendly web applications. Hereās a closer look:
- Client-Side Validation: As previously mentioned, this occurs within the userās browser. It checks the email format as the user inputs the information. This method increases response speed and reduces unnecessary server requests.Advantages of Client-Side Validation:
- Server-Side Validation: This type of validation occurs after data is sent to the server. Even if front-end checks are skipped or bypassed, server-side validation acts as a final filter to ensure the integrity of the data before it interacts with the database.Advantages of Server-Side Validation:
- Immediate response to user input.
- Reduces server load and network traffic.
- Enhances user interaction by guiding input in real-time.
- Adds a layer of security; malicious users cannot bypass it.
- Ensures that data integrity is maintained regardless of client-side failures.
In summary, embracing both validation strategies is essential for a full-spectrum, user-centric approach to email validation. While JavaScript on the client side handles initial checks, server-side validation serves as a safety net, confirming that the data aligns with the required standards ā because an ounce of prevention is worth a pound of cure.
Regular Expressions Explained
Regular expressions, or regex, serve as the backbone for validating email addresses in JavaScript. They allow us to define a search pattern that can identify valid email structures amidst a sea of potential mistakes. The significance of this topic lies in its ability to enhance the reliability and functionality of web applications, especially when it comes to handling user-submitted data.
When dealing with email validation, regex provides a concise way to construct complex validation rules without adding unnecessary complexity to your code. This dual natureāboth a tool for precision and an advocate for clarityāmakes regex an invaluable part of a developerās toolkit. By utilizing regex correctly, you significantly reduce the risk of accepting invalid email formats, which could hamper communication and user engagement.
Basics of Regular Expressions
At its core, a regular expression is a sequence of characters defining a search pattern. This pattern is then applied to strings to identify matches. For instance, the regex describes a simple email structure, where:
- matches any word character (letters, digits, or underscores)
- indicates one or more occurrences of the preceding element
- signifies the literal character '@'
- is an escape sequence for the period, crucial for representing domain separators.
Understanding the individual components of a regex is essential, especially when creating valid email patterns. Regex operates like a puzzle; knowing how to piece together its elements is key to achieving a successful outcome.
Creating a Regex for Email Validation
When creating a regex pattern specifically for email validation, there are certain considerations to keep in mind. A comprehensive regex might look something like this:
Letās break it down:
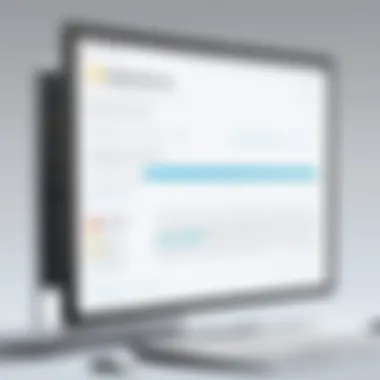
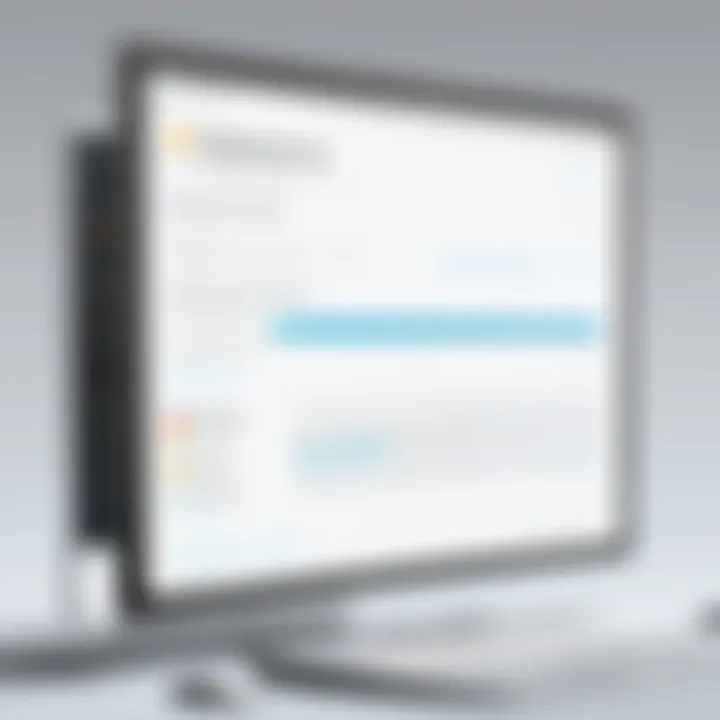
- asserts the start of the string.
- captures the local part of the email, allowing various characters.
- establishes the required separator.
- represents the domain name portion.
- denotes the domain separator.
- asserts the end of the string.
This regex presents a balance of strictness while still allowing legitimate email formats to pass through.
Common Regex Patterns for Emails
There are several common patterns you might see for validating emails, each varying in complexity. Here are a few:
- Flexible Pattern:
Regex for email validation can vary greatly based on context and the level of strictness required. Itās always advisable to test your regex against various email examples to ensure it functions as expected.
In each example above, the regex has its unique rules regarding what it accepts. Depending on your needs, you may choose a pattern that allows for special characters in the email or restricts certain input types entirely. Pondering over these choices is crucial for achieving the best user experience.
Implementing Email Validation in JavaScript
Implementing email validation in JavaScript is not just a technical necessity; it's a pivotal aspect of enhancing user experience and ensuring data integrity. An effective validation system can catch errors before they hit the server, saving both time and resources. When users input their email addresses incorrectly, it can lead to frustration, missed communications, and overall inefficiency within web applications.
The process doesnāt merely involve checking if an email has a general format with an '@' and some characters before and after, but dives deeper into user interaction. Itās about creating a seamless experience where users are guided, informed, and in control of the data they provide. Beyond just validation, itās a chance to set the tone for how robust and user-friendly your application can be.
This section outlines the importance of effectively implementing the validation process through a step-by-step approach, followed by a piece on utilizing event listeners for instant feedback. Letās get a bit more granular.
Step-by-Step Validation Process
When laid out clearly, a step-by-step validation process can make things easier both for developers and users. Hereās a general approach you might consider:
- Define Valid Email Format: Start with a foundational understanding of what a valid email looks like. This usually follows the standard regex expressions with a typical structure, such as . In addition, keep in mind companies often have different formats that need updating in validation regimens.
- Write JavaScript Validation Function: Create a function that incorporates regex to test the incoming input against your defined valid structure. Hereās a simple example:
- Integrate Into HTML Forms: Connect your validation function to form elements via event listeners. This back-and-forth communication makes for a smooth validation experience.
- Test Valid and Invalid Inputs: Run various test cases to highlight the robustness of your validation function. Ensure that so-called edge cases like "user@domain..com" are trotted out for assessment.
- Display Errors: If validation fails, it's crucial to show articulate and helpful error messages. Instead of simple alerts, consider inline messages that help the user correct their input.
This method contributes to a systematic validation framework that ensures efficiency and clarity. A stepwise approach like this minimizes user errors and enhances the application's credibility.
Using Event Listeners for Input Validation
Once you have a validation function in place, incorporating event listeners for real-time validation ensures that users donāt have to wait until they submit the form to know if they made a mistake. This element is essential for maintaining an engaging user experience.
- Hook the Listener: Attach an event listener on the input field to monitor for changes or when the user leaves the field (commonly known as event). Hereās an example:
- Display Validation Result: Provide immediate feedback in the form of an error message or a visual indicator (like a red border) on the input field. This makes the input process easy and error-free.
- Capture Possible Changes: Keep in mind that the user might edit their input. As such, it's essential to verify the input each time itās altered. Listening for events like can enhance this checking mechanism.
"Real-time feedback leads to satisfied users and robust data management."
To wrap it up, implementing email validation in JavaScript does more than just ensure accuracy in user inputs. Itās about creating an environment where users feel supported and informed. With proper event listener integration, the application can easily provide an interactive and pleasant experience, helping users not only input correctly but also understand the process as they move along.
Testing Email Validation Logic
Testing your email validation logic is not just an afterthought; itās the crux of ensuring that your web application functions smoothly in real-world scenarios. Imagine a scenario where a user registers with an incorrect email. It can spell doom not just for the user experience but also for your app's credibility. Having a reliable testing mechanism allows developers to effectively squash bugs and ensure consistent performance across different conditions. By rigorously testing various inputs, youāll not only catch errors but also refine your validation logic for optimal performance.
Common Test Cases
When you think about validating emails, itās essential to consider various common scenarios that users will likely throw at your form. Running through these test cases can help sharpen your validation skills:
- Valid email formats: Make sure to test different variations of legitimate emails such as:
- Invalid email formats: Itās equally crucial to scrutinize formats that should be flagged. Test cases should include:
- Empty or missing fields: Users may submit forms without filling in the email address. Check how your validation handles:
- Long email addresses: Validating emails that are unusually long or contain unusual characters helps ensure your logic is versatile. For instance:
- user.name@example.com
- user+tag@example.co.uk
- first.last@subdomain.example.com
- user@.com
- @example.com
- user@exam_ple.com
- (Empty input)
- (Input with spaces only)
- verylongusername@verylongdomainname.com
"A great test suite can serve as documentation, showcasing expected behavior and edge cases."
Each of these cases contributes to a more resilient validation system. Automating tests can save time and ensure that any modifications in your validation logic won't inadvertently break existing working features.
Handling Edge Cases
Edge cases are the wild cards of software development. They emerge when conditions under normal testing parameters suddenly change. Ignoring these scenarios can be as good as inviting chaos into your application. Below are a few key edge cases that merit attention when validating email addresses:
- International domain names: Since users worldwide may utilize your application, be sure to validate emails with non-Latin characters such as:
- Unusually formed email addresses: Account for complex structures that may still be technically valid:
- Multiple consecutive dots: Users might try to be clever and input something like:
- test@exƤmple.com
- user@Ł Ų«Ų§Ł.Ų„Ų®ŲŖŲØŲ§Ų±
- ""@example.com
- user@domain.com (a period must not be the first or last character)
- user..name@example.com
These edge cases remind you to further scrutinize your regex patterns and your validation flow, making deficiencies glaringly obvious. Focusing on these points can enhance the robustness of your email validation, leading to fewer user frustrations and smoother operations on your platform.
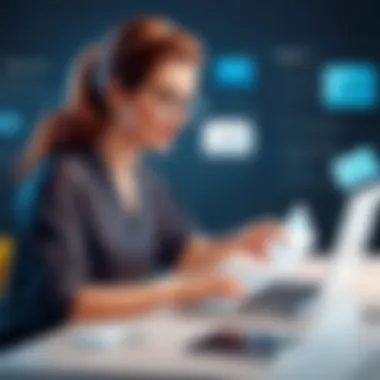
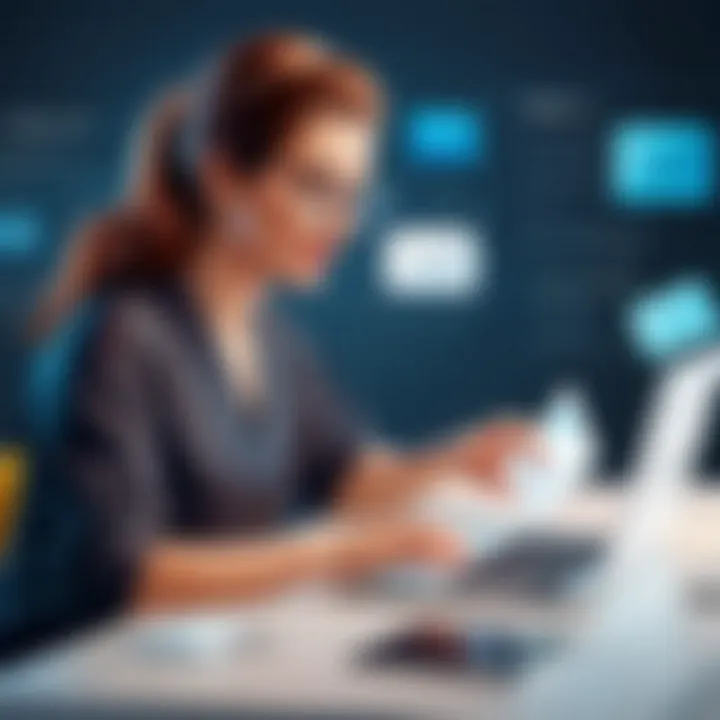
User Experience Considerations
When it comes to validating email addresses through JavaScript, user experience should sit on the throne of your priorities. Properly executed validations not only ensure that an email entered is accurate but also contribute to a smoother interaction with your forms. If users encounter confusing messages or hurdles during the sign-up or contact process, they'll likely toss in the towel before completing the form. Thus, focusing on user experience in email validation also aligns perfectly with the broader strategies of user retention and satisfaction.
Providing Real-Time Feedback
Feedback, in any interactive scenario, acts as a compass. Users crave guidance to know if they're on the right path or headed for a dead end. Implementing real-time feedback when a user inputs their email address can drastically improve their experience. As they type, validating their input on-the-fly helps catch mistakes immediately, instead of waiting until submission time. This proactive approach saves both the userās time and your serverās resources.
- Immediate Error Correction: If a user types something like "example@gmail.con" instead of "example@gmail.com", real-time validation can flag this error instantly. Instead of receiving a cold and often frustrating error message post submission, users receive an immediate nudge to correct their mistake.
- Visual Indicators: Think about integrating color changes, icons, or even brief messages adjacent to the email field. For instance, showing a green checkmark next to a correctly formatted email can provide a sense of accomplishment, while a red exclamation icon signals that something's amiss. It creates a more inviting interaction.
As noted, these small nudges can enhance user engagement and increase trust in your site. When users see that the system is working alongside them, they're likely to feel more comfortable.
Designing Intuitive Forms
The form design plays a critical role in user experience, especially when validating email addresses. A well-structured form is like a roadmapāit guides users smoothly from point A to B without unnecessary distractions. Simple things make a big difference:
- Logical Flow: Ensure that the email address input field appears in a logical sequence, well after gathering necessary information like name or username. If users see an email field at the wrong spot, confusion may arise, possibly leading to an abandoned form.
- Clear Labels: Labels need to be clear and concise. Instead of a vague āEmailā, consider something like āEnter Your Email Address.ā It communicates more directly what you expect from users.
- Placeholder Text: Using placeholder text within the input fields can also guide users on what format to use. Something like "yourname@example.com" indicates the expected input clearly, reducing the chances of mistakes.
- Error Messages: If errors do occur, explain them simply and kindly. Instead of saying āInvalid email,ā try āPlease enter a valid email format, like user@example.com.ā This not only informs the user but does so in a more human way.
In summary, prioritizing user experience when validating email addresses in JavaScript can transform a frustrating task into a seamless one. By implementing real-time feedback and designing intuitive forms, you create an environment that respects users' time and intelligence, which can significantly enhance their journey through your digital landscape. Focus on these aspects, and you'll foster an environment where users feel valued and understood.
Remember: The goal is to let users navigate your forms with ease, ensuring that every interaction is as smooth as butter.
Common Pitfalls in Email Validation
Email validation is an essential part of web form development, yet many developers overlook crucial details that can lead to frustrated users and compromised data integrity. Understanding the common pitfalls in email validation helps in crafting reliable and user-friendly applications. By avoiding these mistakes, we can improve both the accuracy of the validation process and the overall experience for users filling out forms.
Over-restrictive Patterns
Creating an overly restrictive regex pattern is a frequent misstep many developers take when validating email addresses. While it might seem prudent to include every possible criterion to ensure that only the "perfect" email formats are accepted, this often leads to unnecessary friction for the user. For instance, insisting on the presence of specific characters or domains can lock out legitimate users.
For example, if a validation pattern requires emails to end with a specific top-level domain such as .com or .org, anyone trying to enter an email address from a lesser-known domain will face rejection. This can not only alienate users but also skew data collection, leaving significant gaps in understanding user interaction.
"Remember, the goal of email validation is to strike a balance between filtering out bad input while allowing those who might not conform to the strict ideal of a 'valid' email to still participate."
In essence, validation should be smart enough to allow variations while still catching obvious mistakesālike missing an '@' or a period. We should aim for patterns that cover common use cases without going overboard.
Ignoring Internationalization
As our world becomes more interconnected, overlooking email validation for international users is a mounting issue. Different regions may have unique email formats or characters that need to be accepted to avoid excluding global users. For example, some non-Latin characters can be part of email addresses, reflecting local cultures or languages.
If developers stick to only Latin characters in their validation regex, they may inadvertently shut out a portion of potential users. Not accounting for the fact that international email addresses can include complex character sets could lead to failed registrations or transactions.
Make sure your email validation includes internationalized domain names and other global formats. Itās not only about respecting diverse user bases but also about making sure that your services are accessible to a broader audience. As more businesses operate online, inclusive design will become not just a nice-to-have but a necessity.
In summary, avoiding over-restrictive patterns and considering internationalization can lead to a smoother and more welcoming user experience. As we create more inclusive web applications, recognizing and addressing these common pitfalls will position developers to build more effective email validation systems.
Future Trends in Email Validation
As technology evolves at a breakneck pace, the methodologies for validating email addresses are also on the cusp of transformation. The future of email validation is entwined with advancements in programming practices, user experience design, and innovative integration of machine learning. In this section, we will explore what changes are on the horizon, focusing on the significance of adopting new standards and the exciting integration of AI into our validation processes.
Adopting New Standards
Anytime companiesābig and smallāthink about enhancing their applications, new standards always come into play. These standards ensure that user data, particularly email addresses, are not just valid but also adhere to the latest security protocols and best practices. The emergence of RFC 5321 and RFC 6531 drew attention to authenticating domains and providing support for international email addresses. This emphasizes that the email landscape is constantly shifting.
- Protecting User Data: New standards often focus on encryption and privacy. Sticking to these ensures that the validation process does not compromise user data, keeping it safe from spies and thieves lurking in the digital shadows.
- Enhancing User Experience: By adopting new ways to validate, interfaces can become more intuitive. Standards that promote real-time validation can reduce errors before users even hit 'submit,' leading to better interactions.
In addition to improving security, adopting standards also sets the stage for more inclusivity. As a world that's becoming increasingly interconnected, it's imperative that email validation accommodates diverse character sets, allowing users from different cultural backgrounds to seamlessly engage with digital platforms.
"New standards in email validation not only enhance security but also broaden access, ensuring we don't leave anyone behind."
Integration of AI in Validation Processes
Looking forward, artificial intelligence is becoming a game-changer in multiple domains, and email validation is no exception. With AI integrated into validation processes, we're talking about a leap forward in accuracy and efficiency. Hereās how AI is reshaping email validation:
- Smart Learning: AI algorithms can learn from massive datasets, refining the validation process over time. This means that adjustments can be made based on emerging patterns, resulting in continual enhancement of accuracy.
- Predictive Validation: Utilizing machine learning allows for anticipating user inputs before they happen. If a user consistently submits an incorrect email structure, the AI can proactively suggest corrections.
- Fraud Prevention: By analyzing patterns and behaviors, AI can flag unusual activities that might indicate fraudulent email submissions, providing an additional layer of security. This advantage cannot be ignored, especially in environments sensitive to data breaches.
The integration of AI also sets the stage for a more adaptive approach to validation, addressing problems like over-restrictive patterns that often thwart legitimate users. By gradually learning from the data it processes, the technology can respond in real-time to users' unique validation needs while minimizing unnecessary barriers.
As we venture into the next era of email validation, it's clear that the fusion of new standards and AI has the potential to create a smoother, more secure experience for users. Keeping pace with these advancements not only keeps applications relevant but also emphasizes a commitment to user-centric development.
Ending
In the realm of web development, the ability to validate email addresses is not just a courtesy; itās a fundamental necessity. A well-implemented email validation mechanism ensures that applications can effectively interact with users while safeguarding against potential inputs that could disrupt functionality. It works behind the scenes, yet its impact resonates profoundly throughout the user experience.
Importance of Proper Email Validation
In this article, we've unpacked various facets of email validation using JavaScript. The techniques and best practices discussed highlight the balance between user convenience and data integrity. A validated email enhances communication effectiveness and reduces unnecessary frustration during user registration or account setups.
- An accurate email validation process confirms that user inputs are viable, leading to fewer bounce rates and better overall engagement.
- By employing regex patterns in JavaScript, developers can customize validation rules suited to their specific audience, promoting international inclusivity and adherence to local standards.
- The potential risks associated with over-restrictive patterns can thwart genuine users, which underscores the necessity of a thoughtful approach in creating these validations.
Benefits of Engaging Email Validation Practices
Implementing email validation strategies not only preserves the sanctity of user data but also plays a role in optimizing applications for better performance. It enhances user interactions, reduces errors, and promotes trust between the user and the application. As we consider future advancements, the prospect of integrating AI into validation processes presents a fascinating evolution that could predict and adapt to user behavior, leading to an even more refined experience.
In summary, proper email validation ensures the reliability of interaction between users and applications, fosters an atmosphere of professionalism, and diminishes the likelihood of technical hiccups. By adhering to best practices while being cognizant of common pitfalls, developers can create robust systems that respect and enhance the user's journey. This will set a foundation for more advanced features, enriching the overall landscape of web applications.