Unveiling the Intricacies of Binary Trees: A Programming Exploration
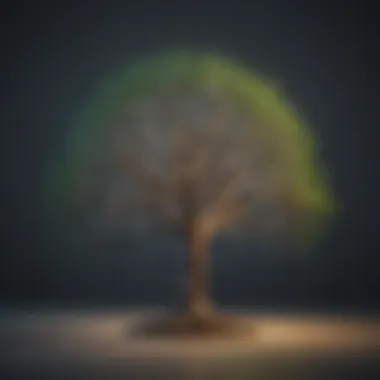
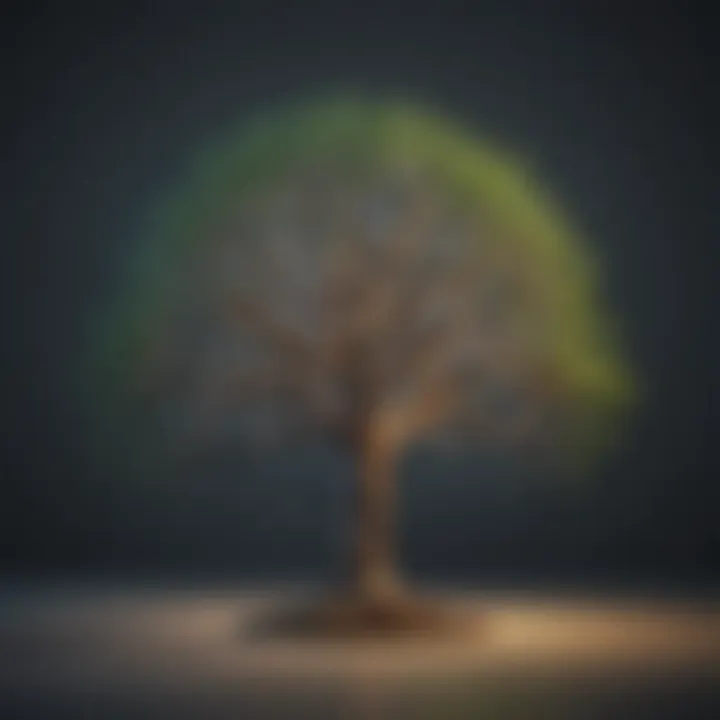
Introduction to Binary Trees in Programming
Binary trees are a fundamental data structure in the realm of computer science, offering a versatile and efficient way to store and manage data. Understanding the intricacies of binary trees is crucial for anyone delving into the world of programming. This section will provide a concise overview of binary trees, their importance, and their widespread applications.
Basic Concepts of Binary Trees
Nodes and Leaves
Binary trees comprise nodes, each of which can have a maximum of two children. The topmost node is known as the root, with nodes beneath referred to as children. Leaves are nodes that do not have any children. Exploring these fundamental components lays the groundwork for comprehending the structure of binary trees.
Traversal Techniques
In programming, traversing a binary tree involves systematically visiting each node exactly once. There are various methods for traversal, including in-order, pre-order, and post-order traversal. Each technique offers a unique way to access and process the nodes within the binary tree.
Binary Search Trees
A specialized form of binary tree, binary search trees maintain a specific order of nodes, making search operations more efficient. The left child of a node contains a value less than the parent, while the right child contains a value greater. Understanding this ordering principle enhances the understanding of binary trees' practical applications.
Advanced Concepts and Applications
Balanced vs. Unbalanced Trees
Balanced binary trees maintain a symmetrical structure, ensuring optimal performance for various operations. On the other hand, unbalanced trees can lead to decreased efficiency due to uneven distribution of nodes. Exploring the differences between these tree types sheds light on optimizing data structure performance.
Binary Tree Operations
From insertion and deletion to searching and updating nodes, binary trees facilitate a multitude of operations essential for data manipulation. Understanding these operations is vital for harnessing the full potential of binary trees in programming.
Practical Implementations and Case Studies
Tree Traversal Algorithms
Implementing tree traversal algorithms, such as Depth-First Search (DFS) and Breadth-First Search (BFS), provides practical insights into how binary trees can be utilized to solve real-world issues. Hands-on examples and code snippets showcase these algorithms in action, offering a tangible approach to learning.
Application in Database Management
Binary trees play a crucial role in database management systems, enabling efficient storage and retrieval of data. Exploring how binary trees are integrated into database structures elucidates their significance in optimizing large-scale data operations.
Further Learning Resources
Recommended Readings
For those seeking to deepen their knowledge of binary trees, recommended books such as 'Introduction to Algorithms' by Thomas H. Cormen et al. offer comprehensive insights into advanced algorithms and data structures. These resources serve as valuable assets for individuals aiming to master binary tree concepts.
Online Courses and Platforms
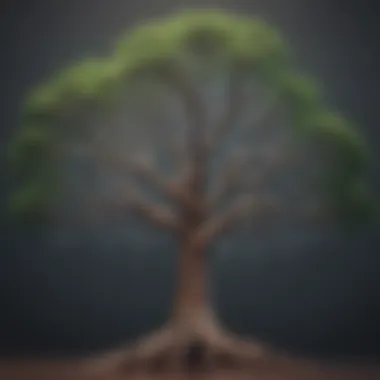
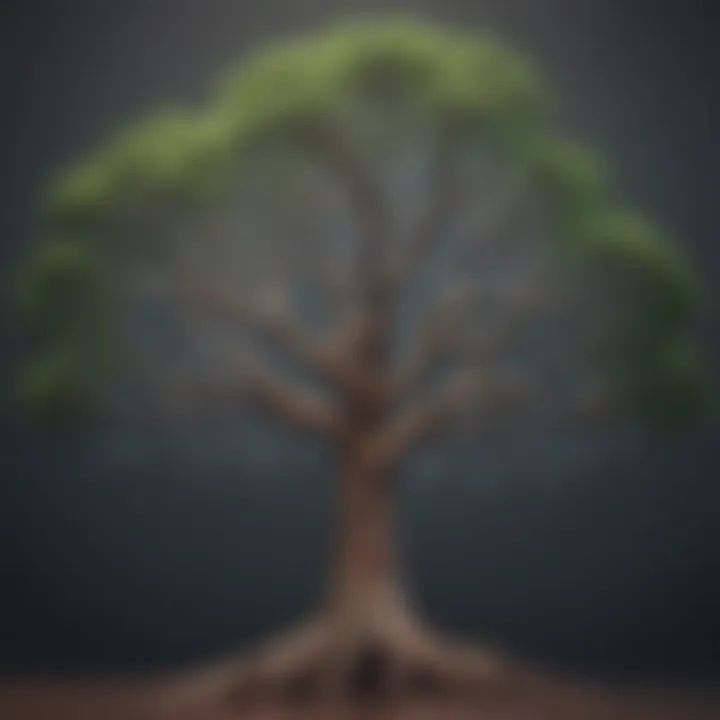
Platforms like Coursera and edX provide specialized courses on data structures and algorithms, including in-depth modules on binary trees. Enrolling in these courses can enhance one's understanding of binary trees through interactive lessons and practical exercises.
Community Engagement
Engaging with programming communities like Stack Overflow and GitHub allows individuals to collaborate, exchange ideas, and seek guidance on binary tree implementations. Joining relevant forums and groups fosters a sense of community and encourages continuous learning and growth in programming skills.
Introduction to Binary Trees
Binary trees stand as a cornerstone in computer science, playing a pivotal role in organizing and managing data efficiently. Understanding the essence of binary trees is fundamental to grasping complex algorithms and data structures. In this article, we delve deep into the intricacies of binary trees, exploring the various types, traversal techniques, and applications that underpin the very foundation of programming.
Definition and Characteristics
Node Structure
The node structure of binary trees defines the building blocks of these hierarchical structures. Each node encapsulates data and references to its left and right children nodes, forming the core functionality of binary trees. This arrangement allows for streamlined data access and manipulation, facilitating efficient operations like searching, insertion, and deletion. The simplicity and clarity of the node structure make it a preferred choice for implementing various tree-based algorithms and applications.
Binary Tree Rules
Binary tree rules encompass the principles governing the organization and traversal of binary trees. These rules dictate the arrangement of nodes, ensuring that each node has at most two children: a left child and a right child. Adhering to these rules maintains the binary tree's integrity and enables efficient data retrieval and manipulation. By following established binary tree rules, programmers can implement optimal solutions for a wide range of computational problems.
Types of Binary Trees
Full Binary Tree
A full binary tree exemplifies a tree structure in which each node has either zero or two children. This characteristic simplifies the tree's representation and traversal, offering a balanced structure that enhances algorithmic efficiency. The completeness of the full binary tree facilitates quick data access and ensures optimal space utilization, making it a popular choice for scenarios requiring consistent search and retrieval operations.
Complete Binary Tree
A complete binary tree exhibits a structure where all levels, except possibly the last, are fully filled, and the nodes are as left as possible. This compact arrangement streamlines the storage and retrieval of data, minimizing access times for essential operations. The completeness of a complete binary tree guarantees optimal performance in scenarios demanding uniform data distribution and efficient memory usage.
Perfect Binary Tree
A perfect binary tree represents a specialized case of a full binary tree where all internal nodes have two children, and all leaf nodes are at the same level. This uniform distribution of nodes ensures a complete and balanced structure, facilitating expedited data operations and traversal. The regularity of a perfect binary tree optimizes memory allocation and access patterns, making it a preferred choice for scenarios requiring consistent and predictable performance.
Binary Tree Traversal Techniques
Binary tree traversal techniques play a crucial role in the realm of binary trees. It involves visiting each node in a specific order to perform various operations on the tree. The significance of mastering traversal techniques lies in the efficiency of data retrieval and manipulation processes. By understanding these techniques, programmers can navigate through binary trees systematically, ensuring optimal performance and accurate results. Factors such as the type of traversal, recursive algorithms, and iterative methods contribute to the overall effectiveness of binary tree operations.
Inorder Traversal
Algorithm
The algorithm for inorder traversal defines the sequence of steps followed to visit each node methodically. It ensures that nodes are processed in the correct order, starting from the left subtree, then the root, and ending at the right subtree. The effectiveness of this algorithm lies in its simplicity and efficiency in handling various tree structures. Implementing the inorder traversal algorithm enhances the predictability of node access, enabling programmers to leverage ordered data output for diverse programming requirements.
Implementation Example
An implementation example of inorder traversal showcases the practical application of this technique in traversing binary trees. By demonstrating how nodes are accessed and processed according to the inorder sequence, programmers gain a tangible understanding of the algorithm in action. This example provides a hands-on experience in applying inorder traversal to real-world programming scenarios, highlighting its advantages in facilitating data retrieval, manipulation, and analysis within binary tree structures.
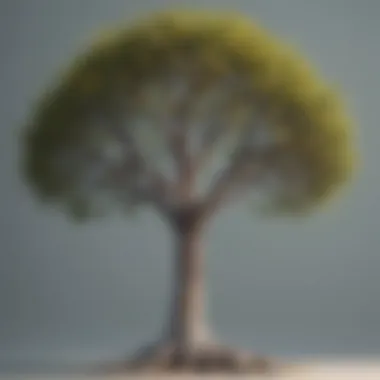
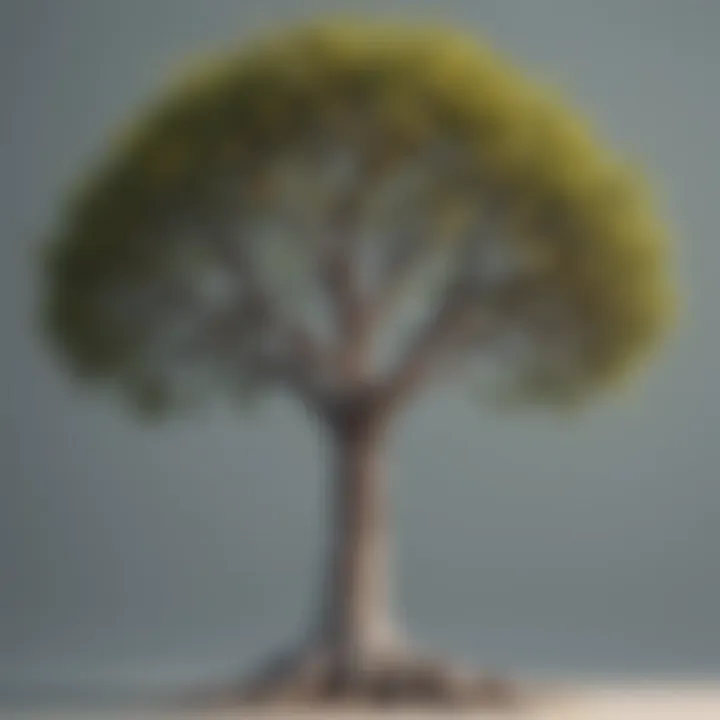
Preorder Traversal
Explained
Preorder traversal is another essential binary tree traversal technique. It involves visiting the root node first, then the left subtree, followed by the right subtree. This approach is valuable for scenarios where a node's parent must be processed before its descendants. Preorder traversal's unique characteristic lies in its ability to establish the tree's hierarchy from the top-down, making it suitable for tasks like creating expression trees and copying the structure of a tree.
Algorithmic Approach
The algorithmic approach to preorder traversal defines how nodes are accessed and processed in a specific order. By starting from the root and moving towards the subtrees, this method ensures systematic traversal of the entire tree structure. The strategic nature of the algorithmic approach enhances procedural efficiency, enabling programmers to execute operations such as tree construction, serialization, and evaluation with precision and clarity.
Postorder Traversal
Sequential Explanation
Postorder traversal presents a distinctive way of traversing binary trees. It involves visiting the left and right subtrees before the root node. This sequencing enables programmers to process a node's descendants before the node itself, making it advantageous for tasks like memory cleanup and evaluating arithmetic expressions. The sequential explanation characteristic of postorder traversal ensures that the tree's leaves are prioritized, promoting effective processing of nodes based on their dependencies and relationships.
Recursive Implementation
A recursive implementation of postorder traversal showcases how this technique can be efficiently applied to navigate through binary trees. By employing recursion to access nodes in the correct order, programmers can streamline the traversal process and handle complex tree structures effectively. This implementation exemplifies the recursive nature of postorder traversal, illustrating how it simplifies tree operations and facilitates the management of hierarchical data within programming contexts.
Balanced vs. Unbalanced Binary Trees
In the realm of binary trees, understanding the distinction between balanced and unbalanced trees is crucial. Balanced trees offer efficient search operations and ensure that the tree remains symmetrically structured. On the other hand, unbalanced trees can lead to skewed structures, affecting the overall performance of tree-related operations. By exploring the differences between these tree types, programmers can make informed decisions on the most suitable tree structure for their specific applications.
Balanced Binary Trees
Concept Overview
Balanced binary trees aim to maintain a consistent depth for all branches, ensuring that search operations are efficient and predictable. This characteristic makes them ideal for scenarios where quick search and retrieval of data is paramount. The self-balancing property of these trees facilitates smoother data operations and reduces the likelihood of performance bottlenecks, offering a balanced approach to managing information.
AVL Trees
AVL trees, a type of self-balancing binary tree, guarantee that the height difference between nodes' children is minimal. This balancing act ensures that the tree remains in optimal condition, promoting faster search times and overall operational efficiency. While requiring additional overhead to maintain balance, AVL trees excel in scenarios where search speed is a priority, making them a popular choice in a variety of programming applications.
Red-Black Trees
Red-black trees, another self-balancing tree variant, introduce color-based rules to ensure the tree's equilibrium. By adhering to specific guidelines regarding node coloring and structure, red-black trees provide a balance between structural stability and operational speed. Despite some complexities in implementation, their ability to maintain balance during dynamic data changes makes them a valuable asset in scenarios demanding adaptability and efficiency.
Unbalanced Binary Trees
Causes
Unbalanced binary trees typically result from skewed insertions or deletions, leading to disproportionate tree structures. The primary cause could be uneven distribution of nodes or improper tree balancing techniques. Such imbalance can hinder search performance and slow down data retrieval processes, highlighting the importance of addressing and rectifying these underlying causes to maintain optimal tree functionality.
Effects
The effects of unbalanced trees manifest in slower search operations, increased complexity in tree traversal, and reduced overall efficiency. Asymmetric structures can impact the scalability and responsiveness of tree-based algorithms, compromising the intended benefits of using binary trees. Understanding the consequences of unbalanced trees emphasizes the significance of proactive measures to prevent or mitigate imbalance, ensuring consistent and reliable performance in programming implementations.
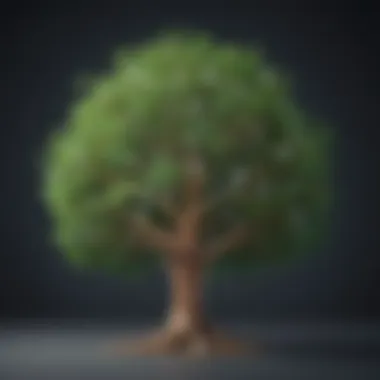
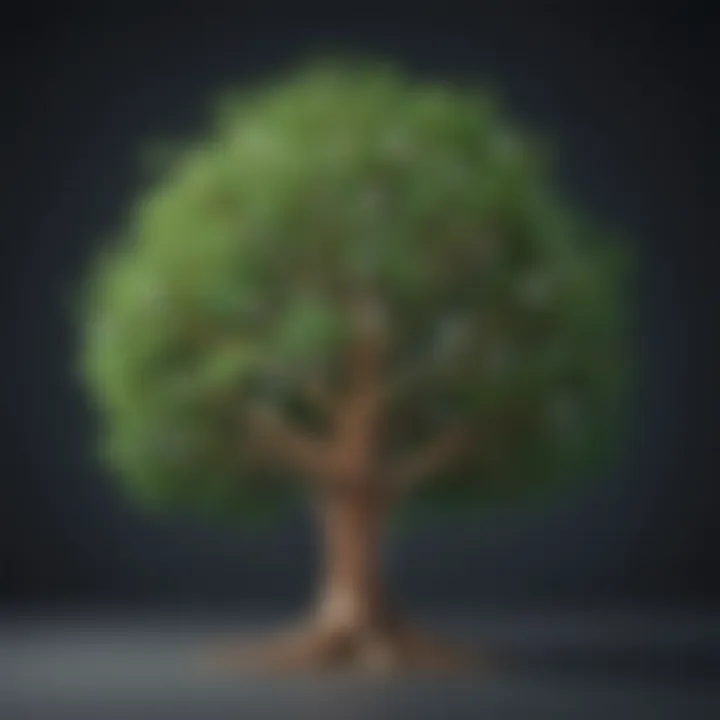
Binary Tree Applications
In the expansive realm of programming, Binary Tree Applications play a pivotal role in data structuring and manipulation. These applications provide a sophisticated means of organizing data efficiently, offering quick access and modification capabilities. Binary Search Trees facilitate swift search operations, making them ideal for tasks that require rapid retrieval of information. Additionally, their versatility extends to insertion and deletion functions, ensuring seamless data management. The heap data structure, encompassing both Max Heap and Min Heap, offers specialized ways to prioritize data elements based on their significance. Max Heap structures data in a way that the highest value is easily accessible, while Min Heap ensures quick retrieval of the smallest value. This Article highlights the importance of understanding these applications in optimizing programming tasks and enhancing algorithmic efficiency.
Binary Search Trees
Search Operation:
Within the domain of Binary Search Trees, the Search Operation stands out as a fundamental feature driving efficient data retrieval. Its ability to swiftly locate specific elements within a sorted hierarchy significantly enhances the overall efficiency of data processing tasks. The Search Operation's logarithmic time complexity makes it a popular choice for scenarios where speed is a critical factor. One unique characteristic of the Search Operation is its reliance on the binary tree structure, enabling a balanced and efficient search process. While offering remarkable advantages in terms of retrieval speed and simplicity, the Search Operation may face limitations in handling unsorted data sets, potentially leading to suboptimal performance in such cases.
Insertion and Deletion:
Insertion and Deletion operations in Binary Search Trees are essential for maintaining data integrity and structure. These operations allow for the seamless addition and removal of elements within the tree, adjusting its layout dynamically. The key characteristic of Insertion and Deletion lies in their ability to preserve the binary search tree properties while modifying its contents. This makes them popular choices in scenarios requiring frequent data updates. One unique feature of these operations is their adaptability to changing data requirements, ensuring the tree remains balanced and efficient. Despite their effectiveness in maintaining tree consistency, Insertion and Deletion operations may incur additional overhead in cases of complex restructuring, impacting overall performance.
Heap Data Structure
Max Heap:
The Max Heap component of the heap data structure focuses on organizing data in a way that the highest value element is at the root, allowing for quick access to the most significant information. This characteristic makes Max Heap an efficient choice for priority-based operations, where identifying and processing the maximum value is crucial. Max Heap's unique feature lies in its hierarchical arrangement, ensuring that priority elements are readily accessible for processing. While offering significant advantages in terms of priority handling and retrieval speed, Max Heap may exhibit limitations when dealing with dynamic data sets, requiring periodic restructuring to maintain optimal performance.
Min Heap:
In contrast to Max Heap, Min Heap organizes data to position the smallest element at the root, facilitating rapid access to minimum values within the dataset. This aspect makes Min Heap a valuable tool for applications where the identification and processing of minimum values are paramount. The unique characteristic of Min Heap lies in its structured hierarchy, ensuring efficient retrieval of minimal elements. Despite its advantages in handling minimum value operations with ease, Min Heap may face challenges in scenarios demanding frequent structural modifications, potentially impacting its operational efficiency.
Huffman Coding
Compression Technique
Huffman Coding stands out as a sophisticated Compression Technique renowned for its ability to efficiently minimize data size without compromising information integrity. This technique's key characteristic lies in its utilization of variable-length codes to represent characters, ensuring optimal storage utilization. Its popularity stems from the capacity to reduce overall data volumes significantly, making it an ideal choice for tasks requiring efficient data transmission and storage. One unique feature of Huffman Coding is its adaptability to varying data distribution patterns, effectively compressing data based on frequency occurrences. Despite its prowess in data compression, Huffman Coding may pose challenges in scenarios with irregular data distributions, potentially impacting compression ratios and decoding processes.
Challenges in Binary Tree Implementation
In the domain of computer science, understanding the challenges associated with binary tree implementation is crucial for programmers aiming to build efficient and reliable systems. When delving into the complexities of binary trees, developers encounter various hurdles that require thoughtful consideration and strategic solutions. By examining the intricacies of binary tree implementation challenges, individuals can elevate their programming skills and enhance the performance of their applications.
Memory Management
Dynamic Memory Allocation
Dynamic Memory Allocation plays a pivotal role in the realm of binary tree implementation by enabling programs to allocate memory at runtime, offering flexibility and efficiency in managing memory resources. This dynamic approach allocates memory as needed, optimizing resource utilization and enabling programs to adapt to varying data requirements. Its key characteristic lies in its ability to allocate memory based on program demands dynamically, reducing wastage and improving memory efficiency. Dynamic Memory Allocation stands as a popular choice in this article due to its versatility and adaptability, allowing developers to optimize memory usage and enhance application performance. However, one must carefully manage memory deallocation to prevent memory leaks and maintain system stability.
Garbage Collection
Garbage Collection contributes significantly to the efficiency and reliability of binary tree implementation by automating the deallocation of unused memory, preventing memory leaks and enhancing program stability. This essential process automatically identifies and recycles unreferenced memory blocks, streamlining memory management and reducing the likelihood of memory-related errors. The key characteristic of Garbage Collection lies in its automated approach to memory deallocation, simplifying memory management tasks for programmers. Its popularity in this article stems from its ability to improve productivity by handling memory cleanup tasks, allowing developers to focus on core programming logic. However, the overhead associated with Garbage Collection mechanisms may impact performance in resource-constrained environments, necessitating careful consideration in implementation.
Complexity Analysis
Time Complexity
Time Complexity analysis plays a crucial role in evaluating the efficiency and performance of algorithms associated with binary tree operations, providing insights into the computational cost of processing data. By assessing the time required for algorithms to execute based on input size, developers can optimize algorithm design and improve program scalability. The key characteristic of Time Complexity lies in its quantification of algorithm performance in relation to input size, guiding developers in selecting efficient algorithms for binary tree operations. Its relevance in this article rests on its ability to inform algorithm choice and enhance program efficiency. However, analyzing Time Complexity demands a deep understanding of algorithmic analysis and may require trade-offs between time and space efficiency in algorithm selection.
Space Complexity
Space Complexity analysis is essential for evaluating the memory requirements of algorithms and data structures used in binary tree implementation, guiding developers in optimizing memory usage and storage efficiency. By quantifying the amount of memory space a particular algorithm consumes based on input size, programmers can make informed decisions to minimize memory overhead and enhance system performance. The key characteristic of Space Complexity lies in its measurement of memory utilization by algorithms, guiding developers in designing space-efficient data structures. Its significance in this article lies in its ability to optimize memory usage and prevent resource depletion. However, managing Space Complexity may involve balancing trade-offs between time and space efficiency, requiring careful consideration in algorithm design and implementation.