Comprehensive Guide to TSP Programming Techniques
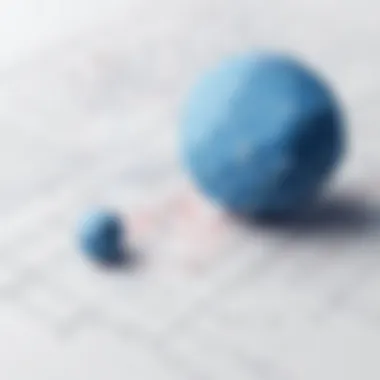
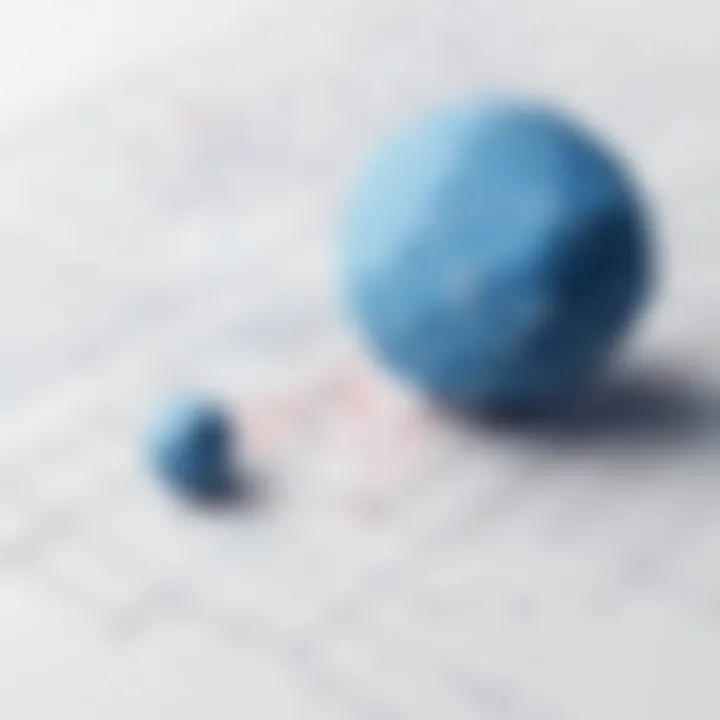
Intro
The Traveling Salesman Problem (TSP) presents an intriguing challenge and has broad applications in numerous sectors. Understanding TSP programming not only aids in grasping theoretical concepts in computer science but also equips individuals with problem-solving techniques relevant across various domains. In this section, we will venture into the foundational elements of programming languages relevant to this challenge, which serve as the backbone of TSP programming solutions.
Intro to Programming Language
Programming languages are the tools that enable developers to communicate with computers, provide instructions, and solve complex problems, including TSP. The variety and architectural differences among languages have significant impact on how problems are addressed in practice.
History and Background
The evolution of programming languages traces back to the 1940s with early machines needing specific commands. Over the decades, programming evolved significantly, leading to languages that abstract complicated rules into simpler components. Each language's development reflects the technological needs and preferences of its time. By exploring TSP through several programming languages, one can better appreciate both the problem and the syntactical and structural elements involved.
Features and Uses
Programming languages come equipped with key features that elevate their utility in TSP, including:
- Syntax and Semantics: Understanding how language rules dictate code structure helps in properly implementing TSP solutions.
- Libraries and Frameworks: Many languages provide robust libraries that simplify the implementation of algorithms relevant to TSP. Examples include Python's NetworkX and C++'s STL.
- Performance Optimization: Certain languages allow for speed enhancements, critical when dealing with TSP's computational cyttery.
Popularity and Scope
Some languages become particularly favorable for tackling TSP challenges, owing to their syntax simplicity or available libraries. Most notable among these are:
- Python: Renowned for being beginner-friendly while having extensive resources for scientific computations.
- Java: With a focus on portability and applicability for large-scale systems.
- C++: Valued for performance efficiency, crucial when implementing demanding algorithms.
Basic Syntax and Concepts
The fundamental components of any programming language form the basis for program creation. Individuals interested in TSP programming should understand several basic syntax constructs, including:
Variables and Data Types
Variables are named storage locations in memory which hold values that can vary. Understanding data types—integers, floats, strings, and arrays—enables effective storage of the various facets of a TSP instance.
Operators and Expressions
Operators are symbols that tell the program to perform certain operations. They can be arithmetic (like + and -) or logical (like && and ||), crucial for processing the data relevant to the TSP problem.
Control Structures
Control structures such as loops and conditionals dictate the flow of tsks in a program. This understanding allows programmers to explore all paths in a solution space effectively, an approach vital to solving TSP optimally.
Advanced Topics
Those wishing to dive deeper might benefit from mastering advanced concepts.
Functions and Methods
Functions encapsulate code into reusable blocks. They can significantly offer clarity in implementing specific algorithms for TSP.
Object-Oriented Programming
OOP introduces concepts like classes, encapsulation, and inheritance. Implementing TSP through OOP can structure code logically and manage complexity.
Exception Handling
Implementing robust error management is crucial in TSP solutions to prevent crashes due to unreachable routes or invalid data.
Hands-On Examples
Programming proficiency grows with practice. Beginners can strengthen their skills through structured examples. Projects at a different scale foster deeper comprehension of coding decision-making.
Simple Programs
Construct basic TSP algorithms using descriptive variable names and well-structured functions.
Intermediate Projects
Consider developing projects using external libraries that can enable visualization functions to better illustrate TSP methods.
Code Snippets
Here’s a small Python function snippet that demonstrates a brute-force search to solve TSP:
Resources and Further Learning
Numerous materials are available for those interested in deepening their understanding of TSP programming:
- Recommended Books and Tutorials: Books focusing on algorithms often cover TSP alongside other computational subjects.
- Online Courses and Platforms: Coursera, edX provide specialized courses for structured learning.
- Community Forums and Groups: Participating in platforms like Reddit can help learners connect and find valuable resources.
Engaging with a community fosters mutual learning and growth in programming skills essential for tackling challenges such as TSP.
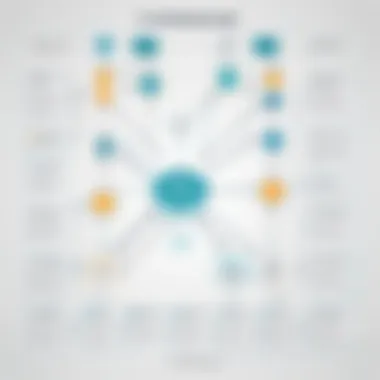
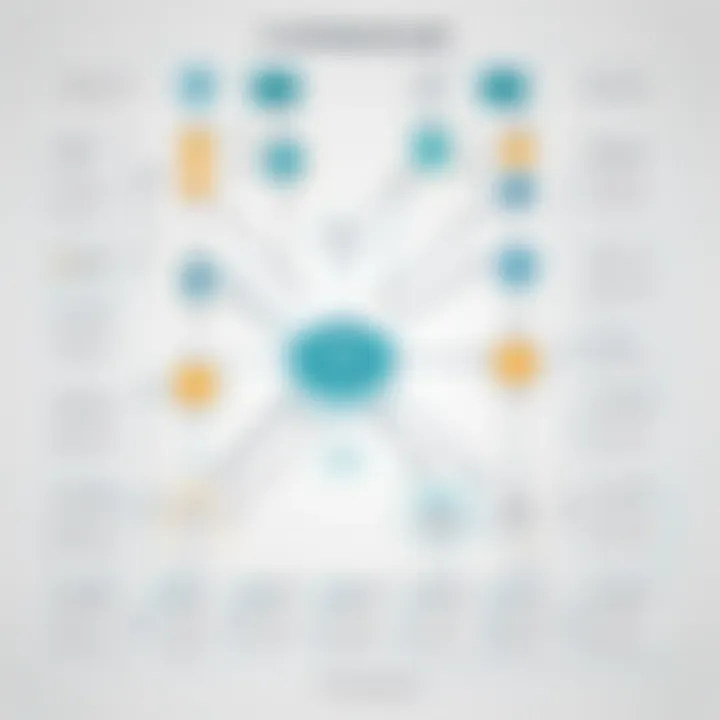
Understanding TSP programming requires a strategic approach to grasp programming fundamentals while practically applying them. Through this comprehensive exploration, individuals can cultivate a strong foundation and grow their capabilities effectively.
Preface to TSP
The Traveling Salesman Problem (TSP) represents a fundamental challenge in the fields of optimization, algorithms, and computer science. Understanding its introduction is vital as it sets the foundation for exploring its far-reaching implications and applications. This section aims to explain the core aspects of TSP and why it deserves attention in both theoretical and practical contexts.
Definition of TSP
The Traveling Salesman Problem involves a salesman who must visit a set of cities exactly once and return to the origin city while minimizing the total travel cost. This problem can be visually represented as a weighted graph, where nodes signify cities and edges represent travel costs between them. Mathematically, our goal is to find the shortest possible Hamiltonian cycle in this graph.
The complexity of TSP arises from the requirement to explore various permutations of city visits to determine the optimal path. Due to this requirement, the TSP is categorized as an NP-hard problem, suggesting that no known polynomial-time solution exists. Understanding TSP goes beyond just knowing the definition; it involves grappling with its computational challenges and the myriad of algorithms devised to tackle it.
Historical Background
The origins of the Traveling Salesman Problem date back to the 18th century, although it gained formal recognition as a mathematical problem in the 1930s. In 1930, mathematicians like Karl Menger studied geometric properties related to paths, establishing a base necessary for contemporary research into TSP.
The progression of computing technology during the mid-20th century brought TSP into the limelight of algorithm theory. In 1954, Michael Held and Richard Karp developed a dynamic programming algorithm that approached the TSP in more manageable terms, laying the groundwork for subsequent methods.
As computing capabilities expanded, researchers ventured into the uncharted territories of heuristics and approximation algorithms designed for tackling TSP more efficiently. The importance of TSP only increases with today's demand for optimization in logistics, transportation, and network design.
The Traveling Salesman Problem challenges thinkers in optimization and algorithms, presenting real-world applications accompanied by formidable computational complexities.
By exploring TSP's definition and historical context, we can appreciate its significance in computer science and adjacent disciplines. In the next sections, we shall delve deeper into its practical relevance and how it relates to device programming.
Relevance of TSP in Computer Science
The Traveling Salesman Problem (TSP) occupies a significant position in computer science. Its study not only enhances understanding of computational challenges but also reveals intricacies inherent in optimization problems. Understanding the relevance of TSP is essential for those who aspire to master programming and its applications for real-world scenarios.
Optimization Problems
In the realm of computer science, optimization problems are fundamental. These problems involve finding the best solution from a large set of feasible solutions. TSP provides a clear case of optimization where the goal is to deliver a route that minimizes the total distance traveled by a salesperson visiting each city exactly once and returning to the origin city.
TSP serves as a benchmark for algorithm performance. It assists in evaluating various algorithms' efficacy, allowing researchers to fine-tune approaches for solving similar complex problems. The characteristics of TSP establish it as an NP-hard problem. This classification indicates that the computational effort increases exponentially with the number of cities, making it an exemplar for understanding computational limitations and the development of new heuristics.
Key Elements of TSP as an Optimization Problem:
- Distance Minimization: The primary goal remains to minimize distance or travel cost.
- Feasibility in Routes: Each route must be viable, touching every city exactly once.
- Algorithm Benchmarking: Performance comparisons can pinpoint strengths and weaknesses in different approaches for solving optimization tasks.
Real-World Applications
The influence of TSP stretches well beyond academic exploration; it permeates numerous sectors and applications around the globe. Real-life scenarios can often mirror TSP's challenges. Logistics and transportation companies frequently encounter problems akin to TSP when planning efficient delivery routes. Understanding TSP’s relevance in these contexts fosters greater insight into operational efficiencies.
Notable applications of TSP include:
- Logistics and Distribution: Delivery services like UPS and FedEx utilize similar paths to maximize efficiency. Effective route planning minimizes fuel costs and delivery times.
- Manufacturing Processes: Several industries use TSP principles to streamline the movement of machinery and tools, reducing motion waste.
- VLSI Design: In electronics, TSP helps improve circuit designs where wires must reach multiple points, optimizing layout and reducing material use.
- Genomics: Certain bioinformatics applications involve data sequence assemblies that resemble TSP.
In recognizing these applications, it's clear that mastering TSP programming equips students with transferable problem-solving skills valued in many high-tech environments.
Understanding the relevance of TSP across multiple domains creates a foundation for programmers aiming to leverage this knowledge for innovative solutions. Computers and algorithms have the potential to reshape tasks ranging from simple route mapping to more intricate problem-solving across various fields.
Formulating the TSP
Formulating the Traveling Salesman Problem (TSP) is a fundamental task for understanding how to approach the optimization involved. This stage is critical as it lays out the structure upon which solutions are built. A clear formulation will help programmers grasp the problem's requirements and ultimately improve their programming skills. The significance of this stage lies in its ability to create a foundation for further exploration of algorithmic, computational, and graphical considerations relevant to TSP. It aids in identifying essential elements that will guide the choices made later in the developmental phases.
Basic Problem Structure
In order to solve TSP efficiently, a programmer must first identify its basic structure. The problem can be summarized as finding the shortest possible route that visits a set of cities exactly once and returns to the origin city. At its core, three primary components make up the TSP's problem structure: cities, distances, and path.
- Cities: These represent the nodes in a problem, typically visualized as points on a map.
- Distances: This component represents the two-way connections. It can be defined in real numbers depending on actual distances on a map, or mathematically in other contexts, such as costs or time taken.
- Path: Refers to the route taken to connect all the cities. The main goal is to minimize the total length of this path.
The importance of defining these components is paramount as it directly influences the methods used for solving TSP.
Understanding how each element interacts will determine the approach and efficiency of potential solutions. Misinterpreting the structure could lead to inaccurate formulations, thereby complicating the entire problem-solving endeavor.
Graph Representation
Applying graph theory fundamentals can greatly enhance the process of formulating the TSP. Graph representation models cities as vertices and distances as edges in a weighted graph. This visualization plays a crucial role in representing relationships and distances more intuitively.
A basic overview of representing TSP in graph terms could be considered as follows:
- Vertices: Represent each city that must be visited.
- Edges: Connect the cities, weighted by the distance or cost of traveling using a particular path.
- Weighted Graph: Each edge in the graph has a defined weight, which can represent distance, time, or cost.
With this representation, we can utilize various graph algorithms, enhancing our capabilities for solving TSP effectively.
To help visualize this, here is an example of a simple code snippet for an adjacency matrix used to represent a graph in Python:
This code framework illustrates how programmers can represent TSP in a structured form. Modifying the adjacency matrix is an essential first step in developing algorithms that will be tackled in the next sections. Recognizing these graphs assists programmers in visualizing the routes better and developing more refined solutions to TSP.
Algorithmic Approaches to TSP
The field of algorithmic approaches to TSP is essential in overlaying practical solutions over the conundrum that this problem presents. Understanding these approaches helps in determining how one can leverage different technological methods to solve the TSP challenge effectively. There are distinct classes of algorithms, each with unique emphasis, benefits, and drawbacks. Throughout this discussion, precise methods will be defined, and their roles in introducing practicality in finding answers to TSP issues will be analyzed.
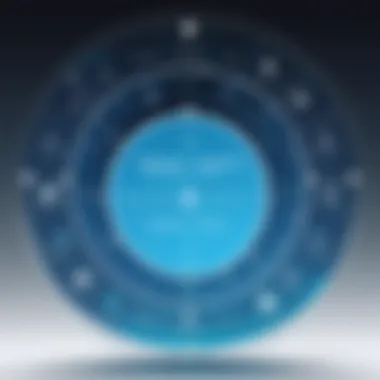
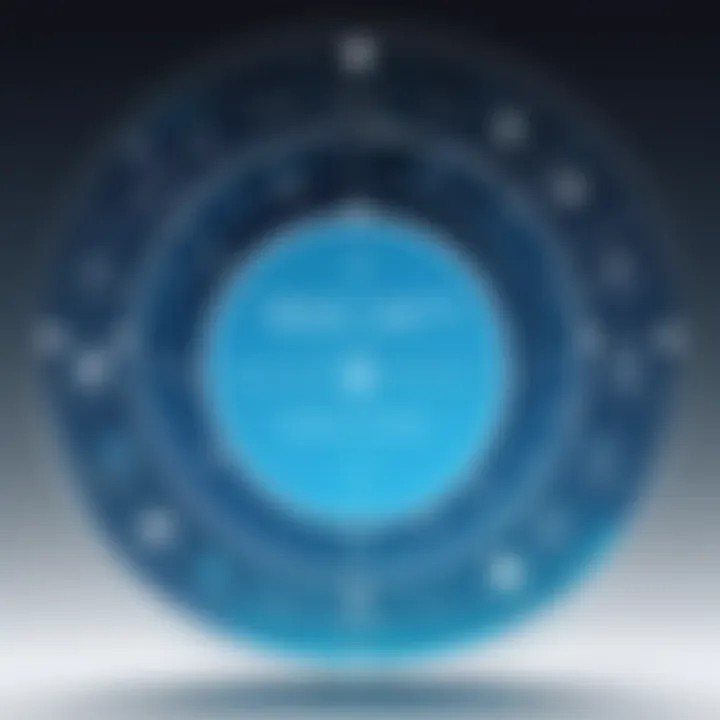
Exact Algorithms
Exact algorithms aim to guarantee the optimal solution for the Traveling Salesman Problem, most suitable for cases involving a limited number of cities due to their computational intensity.
Exhaustive Search
Exhaustive search evaluates every reachable possibility within the problem's constraints. Its contribution is straightforward: by calculating the total distance of all permutations of routes, it enables users to figure out the very shortest path available. This approach is beneficial because it delivers a verified optimal solution in all tested variants. However, the main disadvantage is its inefficiency – as the number of cities expands, the variations grow factorially, leading to potential performance issues. It can be specifically compelling when finding an exact solution is critical regardless of associated time costs, such as in smaller datasets where every node must be addressed holistically.
Dynamic Programming
Dynamic programming offers an insightful edge. Instead of computing different phases repeatedly, it utilizes memorization of the solutions already computed. This makes it a favorable candidate for generating results within a more reasonable time frame. It notably tackles subproblems by breaking down the main problem fly, allowing for significantly quicker processing than exhaustive search paths when grappling with the limited repeated states. Nevertheless, its primary consideration involves adjusting memory due to the added complexity necessary for storage.
Approximation Algorithms
Approximation algorithms strive for efficiency by yielding near-optimal solutions, sacrificing guaranteed accuracy for more manageable computation. These algorithms can be operational for larger datasets and thus are valuable in more extensive applications.
Greedy Method
The greedy method simplifies picking the immediate next best path. It iteratively selects the nearest neighbor yet may fail to yield the final overall best solution. While this approach exhibits low speed in time complexity, it has drawbacks when optimal paths are fundamental, often leading to suboptimal completions since it neglects overall assessments. Nonetheless, it remains popular for handling situations where immediate estimations or deployments are more practical than striaght optimal solutions.
Christofides' Algorithm
Christofides' algorithm fixes inconsistencies left by simpler approximation methods by ensuring triangle inequalities. This unique implementation goes a step further to provide an optimal solution within a given factor, building on earlier greedy results while compensating losses in solutions and providing preventable onlongation of cumulative costs. Its complexity distinguishes effective organizations requiring computational reinforcement and laying up precisions in comparisons relative to other heuristic methods that can drift away from specificity altogether.
Heuristic Methods
Heuristic methods aid in guiding computations towards more approachable routes rather than optimal solutions, favoring real-time scenarios.
Genetic Algorithms
Genetic algorithms mimic natural selection through evolving principles over generations of candidates, which may greatly increase efficiency for finding optimal solutions. They tackle vast and complex landscapes making it possible to conduct extensive searches where average algorithms suffer from diminishing returns. However, the entire framework depends heavily on fitness-based evaluations, and it revolves around adequate configurations for slight setbacks in rate wherein deduction from variations often leads arbitrarily towards oscillation. Thus, effective adaptation in terms of structuring the mutation deals profound with skillful tuning especially during convergence.
Simulated Annealing
Simulated annealing is along the lines of genetic implementations, favoring solutions according to temperature variables slideshow which adapts operational processes based openness thanks to probabilistic definitions throughout iterations. An appreciable aspect is the positive accord providing systemic variation without defining a straightline prior derived growth source altering genetic population takeaways. Nonetheless, a dependent sequence mishap may freeze adjustments briefly leading to potential consolidation overlaps or stagnation on clearer routes across comprehension journeys ahead.
Understandably, the thorough evaluations of these varied algorithmic approaches equip threshold decision-making processes with more intuitive technological selections, improving comprehension pathways across broader representations for computing layer guidelines.
Implementing TSP Solutions
Implementing TSP solutions is a significant aspect of understanding and applying the Traveling Salesman Problem in programming. The ability to create effective algorithms and solutions is necessary to solve real-world optimization questions. This section emphasizes programming languages, programming techniques, and detailed guides crucial for students and practitioners keen to explore TSP.
Programming Languages Suitable for TSP
Python
Python is a widely recognized programming language known for its simplicity and versatility. Its syntax is straightforward, making it accessible for those new to programming. Python provides extensive libraries, such as NumPy and SciPy, that streamline processing and data manipulation. These libraries greatly ease complex matrix operations that are often encountered in TSP.
- Key Characteristic: High readability and ease of learning.
- Contribution to the Topic: Python's widespread community support ensures numerous resources for TSP algorithms, from academic papers to online forums.
- Unique Feature: Libraries designed for mathematical computations.
Pythons advantages in TSP include quick prototyping capabilities, but one should be cautious of performance issues in highly complex problems when compared to lower-level programming languages.
++
C++ boasts features that leverage both high-level and low-level programming. It has efficient memory management, which is crucial for solving large instances of TSP using complex algorithms. C++ allows for more control over hardware optimization, leading to faster computation times for intensive algorithms.
- Key Characteristic: Offers a mix of efficiency and control.
- Contribution to the Topic: Valuable for implementing performance-critical TSP algorithms, particularly for exact methods.
- Unique Feature: Supports object-oriented programming.
C++ is powerful but can add complexity for beginners. The learning curve exists, but its performance in competitive programming is widely noted.
Java
Java, a robust and portable language, is well-suited for larger projects where portability is essential. Its write-once-run-anywhere feature allows TSP solutions to be executed on different platforms without modification. Java has built-in libraries that handle collections efficiently, which aligns well with graph databases that are common in TSP-solving strategies.
- Key Characteristic: Platform independence and an extensive ecosystem.
- Contribution to the Topic: It is especially useful for developing applications that require reliable frameworks.
- Unique Feature: Automatic memory management through garbage collection.
While Java provides solid performance, it may have a slower execution compared to C++. It is still a choice for organizations focusing on cross-platform performance.
Step-by-Step Implementation Guide
Creating solutions for TSP involves an organized approach right from problem formulation to executing algorithms. Below is a simplified step-by-step guide for implementing TSP.
- Define the Problem: Clearly outline to whether it's a symmetric or asymmetric TSP.
- Choose data structures: Often, an adjacency matrix or adjacency list will be useful for representing the graph of cities.
- Select Algorithm: Based upon the complexity and specifics of your TSP case, choose an exact algorithm or a heuristic method.
- Write Code: Determine which programming language suits you (Python, C++, Java) and start implementation in a modular approach.
- Test the Solution: Use small example cases and gradually increase the inputs.
The implementation of TSP solutions is cultivated through significant practice, as theoretical knowledge is only a part of this complex subject.
- Optimize the Algorithm: Review the code for possible enhancements or optimizations.
- Documentation: Ensure your code is well-documented for future reference and collaboration.
Through clear guidelines and orderly steps, implementation of TSP solutions becomes not only feasible but also an intriguing approach to understanding various programming paradigms.
Analyzing TSP Solutions
Understanding the complexities of the Traveling Salesman Problem (TSP) necessitates an in-depth analysis of the solutions derived. Analyzing TSP solutions entails evaluating how effective an algorithm is based on various performance metrics and complexity analyses. This structured inquiry is critical, as it enables practitioners to identify the strengths and limitations of different approaches. Whether one is a beginner wishing to grasp fundamental concepts or an intermediate coder aiming to enhance their skill set, delving into solution analysis remains paramount.
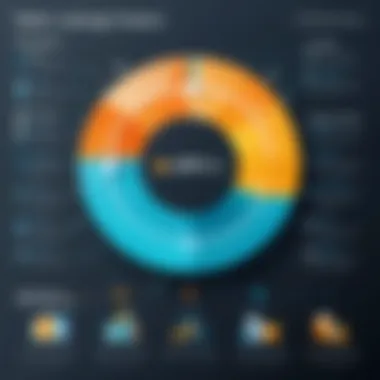
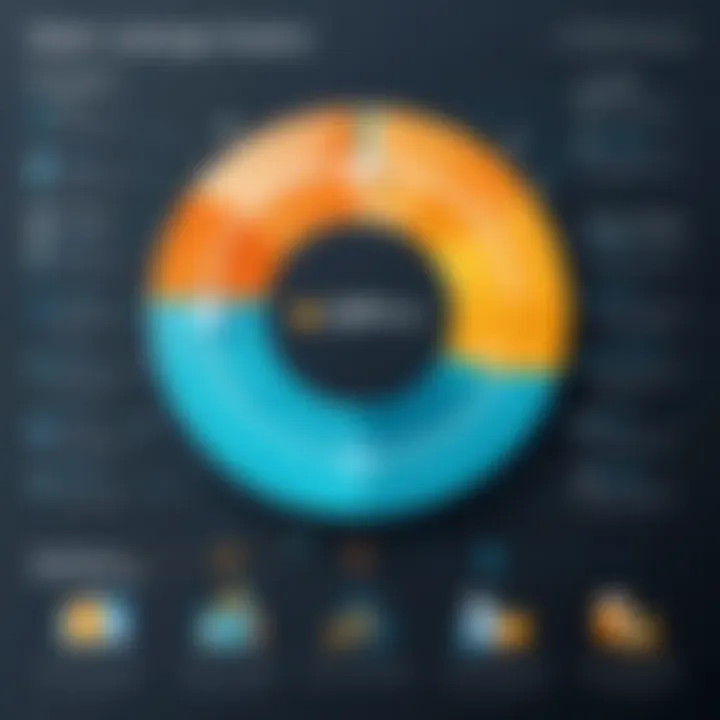
Performance Metrics
Performance metrics are the criteria used to assess the quality of TSP solutions. These metrics highlight the algorithm's efficiency, accuracy, and overall performance. Key metrics to consider include:
- Optimality: It refers to whether the solution provides the best possible route based on the iteration and approach used. Algorithms that yield the optimal solution are often more desirable. However, they may require significant time to reach.
- Execution Time: This metric captures how long it takes for an algorithm to deliver a solution. It is crucial to note that, depending on the algorithm—whether an exact technique or a heuristic—the execution time can vary significantly.
- Solution Quality: Solution quality can be evaluated in terms of distance traveled, cost-effectiveness, or resource utilization. A solution with lower overall costs is generally preferable.
- Scalability: As the number of cities increases, a solution's ability to maintain performance without degrading is critical. A scalable solution effectively adapts to larger datasets accordingly.
Comparing these performance metrics permits developers to select the right algorithms for specific applications. A good grasp of these elements helps inform better choices, enhancing your TSP programming experience.
Complexity Analysis
Complexity analysis examines the computational demands of algorithms used in TSP solutions. By understanding how resource consumption scales with input size, developers can make more informed decisions regarding which method to apply.
This analysis focuses primarily on:
- Time Complexity: It addresses how the execution time of an algorithm increases as the number of inputs—or in TSP, cities—grows. Algorithms with higher-dimensional complexities typically become infeasible in practice as input size increases.
- Space Complexity: This considers the amount of memory that an algorithm requires concerning the input size. An algorithm with lower space complexity generally proves more useful, especially in resource-limited environments.
These two dimensions combine to form a comprehensive view of an algorithm's efficiency. Understanding complexity can drive the choice of sophisticated techniques that effectively counteract the intrinsic inefficiencies inherent to higher-order combinatorial problems like TSP.
Key takeaway: A thorough analysis of TSP solutions aids not only in understanding performance but also in strategic planning for future works.
Challenges in Solving TSP
The Traveling Salesman Problem (TSP) presents considerable challenges for those seeking efficient solutions. Understanding these challenges is crucial, as they expose the inherent complexity of the problem and the significant limitations that practitioners must navigate. When aiming to apply programming techniques to TSP, recognizing these elements will inform better approaches and foster improved algorithm development.
Computational Limitations
The TSP is classified as NP-hard. This classification inherently links to the computational limitations faced in uncovering efficient solutions. Exact methods, such as exhaustive search, suffer as the number of cities increases, making it impractical for large datasets.
- Exhaustive Search: This method evaluates all possible routes, leading to a time complexity of O(n!). As the dataset grows, the processing time spikes exponentially. For example, a problem with just 20 cities results in over 2 billion possible routes. Computing this is unfeasible within a reasonable timeframe.
- Dynamic Programming: While it reduces time complexity, it still cannot bypass exponential growth. The notable Held-Karp algorithm, a dynamic programming solution, functions in O(n^2 * 2^n), which limits utility for datasets beyond a certain size.
Several aspects aggravate these limitations:
- Resource Consumption: Solving TSP entails considerable time and space resource allocation.
- Algorithm Efficiency: Without scalable solutions, the algorithm styles must be judiciously selected based on the specific problem instance.
Understanding computational limitations not only underscores the significance of improved approaches but also shapes how practitioners should set realistic expectations when solving TSP in practical applications.
Scalability Issues
Another considerable challenge arises when addressing scalability in TSP solutions. As problems expand in size, adjustments to algorithms must become equally agile. Scalability entails the ability of a method to adapt and solve larger-sized instances while maintaining efficiency.
Key elements influencing scalability include:
- Algorithm Design: Algorithms like Christofides' Algorithm or Genetic Algorithms may yield reasonable results for moderate-sized TSP instances. Their success diminishes when scaled up due to increased computation overheads.
- Heuristic Effectiveness: Although heuristics are designed specifically to offer quicker solutions, their results can become inconsistent across diverse datasets.
- Infrastructure Expense: As one applies TSP solutions to various fields—logistics, telecommunications, and even AI—diverse computing configurations need to be established. This can be both costly and timing-intensive for companies or individuals aiming to deploy scalable systems.
Balancing between exact precision and pragmatic adaptability is central to managing unto fundamental scalability hurdles. With computational constraints and scalability issues fused in tandem, there remains substantial work to undertake in TSP programming. Only through creating tailored strategies can the possibilities of TSP begin to meet real-world demands.
Future Trends in TSP Research
Exploring future trends in Traveling Salesman Problem (TSP) research presents an opportunity to enhance the understanding of this fundamental computational problem. TSP is more than just a theoretical construct; it has practical implications in diverse fields. Among the numerous future trajectories, two areas stand out: emerging technologies and interdisciplinary applications. Both elements will not only drive the advancement of TSP research but also optimize problem-solving capabilities and expand its utility in various contexts.
Emerging Technologies
Emerging technologies are redefining how algorithms are developed and implemented. With the instances of complex computations growing, the advent of quantum computing is, perhaps, the most transformative technology relevant to TSP analysis. This technology can compute numerous possibilities at a speed unmatched by traditional computers. Quantum algorithms like Grover’s Algorithm could significantly shorten the time it takes to find optimal or near-optimal routes in solving TSP.
In addition to quantum computing, artificial intelligence (AI) and machine learning are making strides in TSP. These algorithms analyze vast datasets and improve their own performance over time as they are exposed to new information. Techniques such as reinforcement learning are of particular interest as it allows for dynamic problem solving in TSP, responding to changes in parameters in real-time. This approach not only provides adaptability to various problem strategies but also extends TSP applications in autonomous systems, like drones in delivery services.
Another trend to consider is cloud computing. Distributed systems can manage complex calculations, offering scalability that localized systems cannot. This setup allows researchers to more efficiently share resources and process large data more effectively, all while reducing costs associated with heavy computational needs.
Interdisciplinary Applications
Interdisciplinary applications are crucial to the practical relevance of TSP. Areas such as logistics, biotechnology, and urban planning now increasingly rely on methods rooted in TSP solutions. Transportation and delivery services optimize their routes to maximize efficiency and minimize costs, simultaneously addressing real-world issues of sustainability.
Moreover, TSP insights are finding a place in the analysis pertaining to biological systems. For instance, genetic sequencing may utilize similar path optimization strategies to identify more efficient routes for biological information flow. Thus, the understanding of TSP is essential in optimizing intricate biosystems, showcasing its broader implications in life sciences.
In environmental studies, TSP frameworks facilitate tackling issues such as wildlife corridor planning for animal migration or finding optimal routes for aqueducts and irrigation systems in agriculture. These examples illustrate the colliding paths of mathematics, computer science, and ecology, evidencing the breadth of TSP’s relevance.
Ending
The conclusion of this article synthesizes the essential concepts discussed throughout the sections covering TSP programming. Understanding the Traveling Salesman Problem goes beyond just a theoretical exercise; it has real-world implications in optimization and logistics. This section serves to emphasize the importance of all the elements covered, ranging from algorithms to practical implementations, and the challenges faced by programmers dealing with TSP.
In summarizing the key points, readers should recognize how TSP represents a fundamental challenge in computational theory. It brings forth the significance of algorithmic efficiency and embraces the diversity of approaches, whether through exact algorithms like dynamic programming or heuristic and approximation methods.
Moreover, learning to implement TSP solutions in various programming languages not only provides an understanding of the problem itself but also enhances their overall programming proficiency. This improvement comes from engaging with the methodologies, challenges, and analytical practices necessary to tackle TSP's inherent complexity.
Therefore, the conclusion isn't just a mere wrap-up; it prompts continued interest in TSP programming, encouraging learners to explore advanced topics and applications. As fields like AI and machine learning grow, the principles derived from TSP are bound to have increasing relevance.
"The exploration of TSP not only sharpens logical skills but cultivates a problem-solving mindset that serves well beyond the realm of theoretical computing."
Summary of Key Points
- TSP is a cornerstone problem in optimization and computer science.
- It illustrates various algorithmic approaches, including exact, approximation, and heuristic methods.
- Implementing solutions involves multiple programming languages like Python, C++, and Java.
- Effective performance metrics are vital for evaluating solutions.
- The challenges of computational limitations and scalability impact real-world applications of TSP.
- Future research trends offer new horizons and applications for TSP in emerging technologies.
Final Thoughts on TSP Programming
In reflecting upon TSP programming, it's clear that it stands as an exemplary challenge worth mastering. TSP encapsulates the intriguing blend of complexity and solvability that characterizes many optimization problems in computer science. As readers conclude this journey, they should appreciate TSP not merely as a computational puzzle but as a gateway into deeper learning and innovative applications.
Successful navigation through TSP equips programmers with vital skills. Enhancing logical thinking, instilling a keen sense to analyze complex problems, and engaging with diverse algorithmic strategies are intrinsic benefits gained. Furthermore, knowing the practical applications in sectors such as logistics, manufacturing, and network design elevates the understanding of its framework.
Endeavor to keep exploring, because the realms of TSP will unveil new challenges and opportunities in programming, pushing boundaries of problem-solving and analytical reasoning.