Unraveling the Intricacies of Recursion in Coding
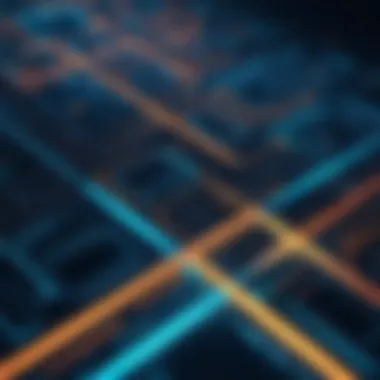
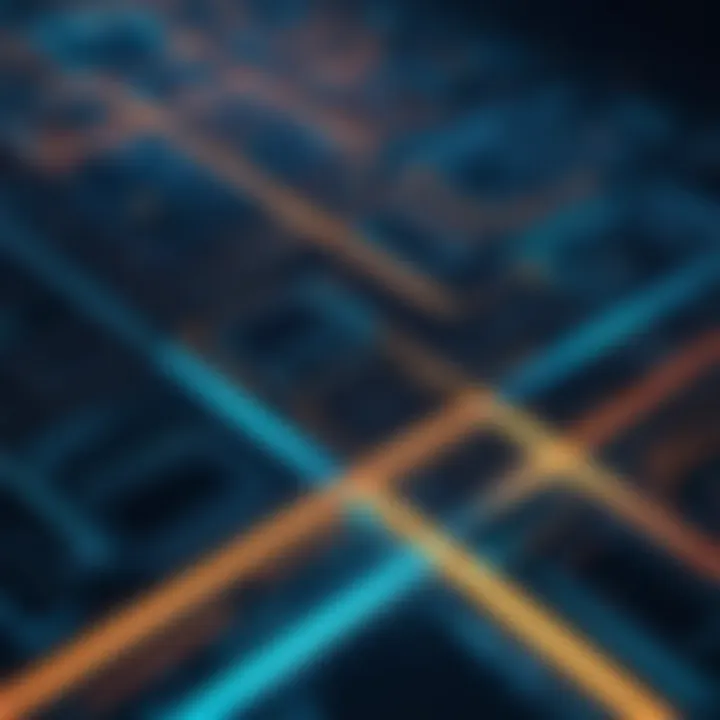
Introduction to Recursion in Coding
Recursion is a fundamental technique in the world of coding where a function can call itself to tackle and resolve problems. This method may seem complex initially but delving into it can unveil its multiple applications, advantages, and potential challenges. The process of recursion involves a function repetitively breaking down a problem into smaller, more manageable parts until a base condition is met. Through this extensive guide, we will explore the intricate concept of recursion, shedding light on its significance and relevance in the realm of programming.
Basics of Recursion
When diving into recursion, understanding the core concepts and syntax is crucial to grasp its essence fully. Variables and data types play a pivotal role in recursion, ensuring that the correct information is being processed and manipulated within the recursive function. How operators and expressions are utilized within recursive functions can significantly impact the efficiency and effectiveness of the code. Control structures also come into play, dictating the flow of the recursive process and determining when and how the function calls itself.
Exploring Advanced Recursion Topics
Moving beyond the fundamentals, advanced topics in recursion delve into more intricate aspects of coding. Functions and methods in recursion introduce the concept of modularization, allowing for code reusability and organization. Object-Oriented Programming (OOP) principles can be applied to recursion, enhancing code readability and scalability. Exception handling in recursion is crucial for error management, ensuring that the program can gracefully handle exceptions and maintain its functionality.
Practical Application of Recursion
To solidify understanding, hands-on examples are essential in illustrating how recursion can be implemented in real-world scenarios. Simple programs can demonstrate the basic structure and functionality of recursive functions, laying the foundation for more complex projects. Intermediate projects showcase the versatility and power of recursion in solving intricate problems, honing problem-solving skills and analytical thinking. Code snippets offer concise yet potent demonstrations of recursion in action, providing a compact visualization of its application.
Resources for Recursion Learning
For further exploration and learning, a curated list of resources is invaluable in enhancing one's proficiency in recursion. Recommended books and tutorials offer in-depth explanations and practical exercises to reinforce understanding. Online courses and platforms provide interactive learning experiences, catering to diverse learning preferences and styles. Community forums and groups create a collaborative environment for sharing knowledge, seeking help, and engaging with like-minded individuals passionate about recursion in coding.
Introduction to Recursion
In the vast realm of coding, an essential technique that stands out is recursion. At its core, recursion allows functions to call themselves, paving the way for elegant problem-solving strategies. This article embarks on a detailed journey to unravel the nuances of recursion in coding, shedding light on its applications, advantages, and potential challenges within the realm of programming.
Definition of Recursion
The basic concept of a function calling itself
The fundamental essence of recursion lies in a function's ability to invoke itself, creating a loop of self-replicating calls within the code. This recursive behavior empowers programmers to tackle complex problems by breaking them down into simpler, more manageable tasks. Embracing the recursive nature of functions opens doors to innovative solution designs and efficient problem-solving techniques.
Recursive functions in programming
Within the programming landscape, recursive functions play a pivotal role in streamlining processes and enhancing code flexibility. By allowing functions to call themselves, recursive functions offer a structured approach to problem-solving, emphasizing the importance of base cases and termination conditions. While recursion can be a powerful tool in a programmer's arsenal, it also demands meticulous attention to detail to prevent issues such as infinite loops and excessive memory consumption.
History of Recursion
Origins of recursion in mathematics
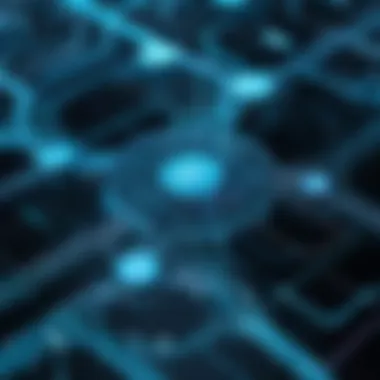
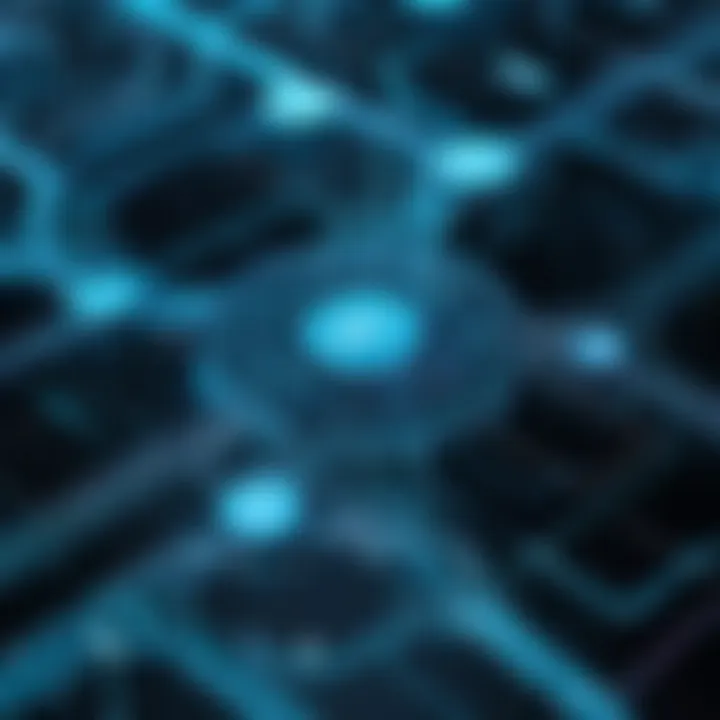
The origins of recursion can be traced back to its roots in mathematics, where the concept of self-referential algorithms first took shape. Building on mathematical foundations, recursion gradually found its way into the realms of programming, offering a novel perspective on problem-solving methodologies. The evolution of recursion from theoretical frameworks to practical applications underscores its transformative impact on algorithmic processes.
Evolution of recursion in programming languages
As programming languages evolved over time, recursion emerged as a valuable paradigm that revolutionized the coding landscape. The incorporation of recursive techniques in programming languages ushered in a new era of problem-solving efficiency, allowing developers to approach challenges with a fresh mindset. The evolution of recursion in programming languages signifies a significant leap towards intricate, yet systematic coding practices.
Understanding Recursive Functions
Recursion in coding plays a vital role in solving complex problems by allowing a function to call itself iteratively. This section serves as a crucial aspect of the article by delving deep into the mechanics and significance of recursive functions. It sheds light on how recursive functions break down problems and create efficient solutions. Understanding Recursive Functions is a cornerstone in programming, offering a unique approach to problem-solving that is both elegant and effective. Readers will gain a comprehensive understanding of the importance and applications of recursive functions in coding.
Structure of Recursive Functions
Base case and recursive case
In the realm of recursive functions, the base case and recursive case are pivotal elements that determine the flow and logic of the function. The base case acts as the stopping condition, preventing infinite recursion and providing a clear endpoint for the function. On the other hand, the recursive case encapsulates the iterative nature of the function, allowing it to call itself until the base case is reached. The synergy between the base case and recursive case is fundamental in ensuring the function's proper execution and termination. While the base case offers stability and closure to the function, the recursive case enables repetition and problem decomposition, making them essential components in recursive function design.
Termination condition in recursion
The termination condition in recursion serves a similar purpose as the base case, defining when the recursive calls should stop. It acts as a fail-safe mechanism to prevent infinite loops and control the function's behavior. By imposing a termination condition, programmers can regulate the recursion depth and avoid stack overflow errors. The termination condition enhances the predictability and reliability of recursive functions, ensuring that they execute within memory constraints and deliver accurate results. However, miscalculating or omitting the termination condition can lead to erratic behavior and computational inefficiency, underscoring the critical role it plays in recursive function implementation.
Examples of Recursive Functions
Fibonacci sequence
The Fibonacci sequence stands as a classic example of recursive functions, showcasing the beauty and simplicity of recursive problem-solving. By defining the sequence recursively with base cases for 0 and 1, each subsequent number is the sum of the two preceding ones. This elegant approach illustrates how recursion can efficiently generate complex sequences through iterative self-referencing. While the Fibonacci sequence demonstrates the elegance and compactness of recursive solutions, it also highlights the potential drawbacks of recursive overhead and repetitive calculations in certain scenarios.
Factorial calculation
Factorial calculation exemplifies another common application of recursive functions, especially in mathematical computations. By defining the factorial recursively as the product of a number and the factorial of its predecessor, recursive functions can compute factorials with concise logic. This methodical approach simplifies factorial calculations and showcases the power of recursion in handling repetitive tasks efficiently. However, factorial calculation through recursion may encounter limitations in handling large values due to stack limitations, necessitating optimization techniques or iterative alternatives for improved performance and scalability.
Benefits of Recursion
Recursion entails significant benefits in the realm of coding, offering a unique approach to problem-solving by allowing functions to call themselves. The utilization of recursion provides programmers with a powerful tool to tackle complex issues effectively and elegantly, delving into the core of problem structures to derive solutions with innate efficiency. By breaking down intricate problems into smaller, manageable tasks, recursion facilitates a structured and systematic approach to programming conundrums, enabling programmers to unravel convoluted issues with precision and clarity. Through the elegant design of recursive solutions, programmers can create streamlined and refined code that not only addresses immediate challenges but also lays the foundation for scalable and adaptable programming practices.
Simplifying Complex Problems
Breaking down problems into smaller tasks
Breaking down problems into smaller tasks forms the crux of recursion, allowing programmers to deconstruct overwhelming challenges into more manageable components. This method streamlines the problem-solving process, guiding programmers through a step-by-step analysis that dissects intricate issues into simpler elements. By compartmentalizing complexities into bite-sized tasks, programmers can navigate through daunting problems systematically, leading to a more efficient and targeted resolution approach. Despite its effectiveness in simplifying complex problems, breaking down tasks can sometimes introduce the risk of overlooking overarching patterns or relationships within the problem, requiring programmers to strike a balance between granularity and comprehensive understanding.
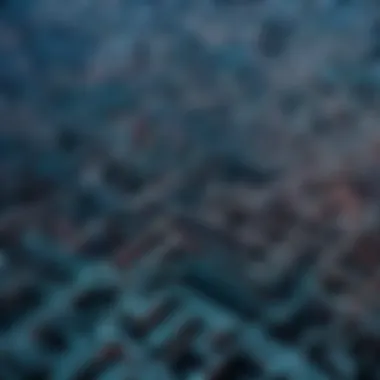
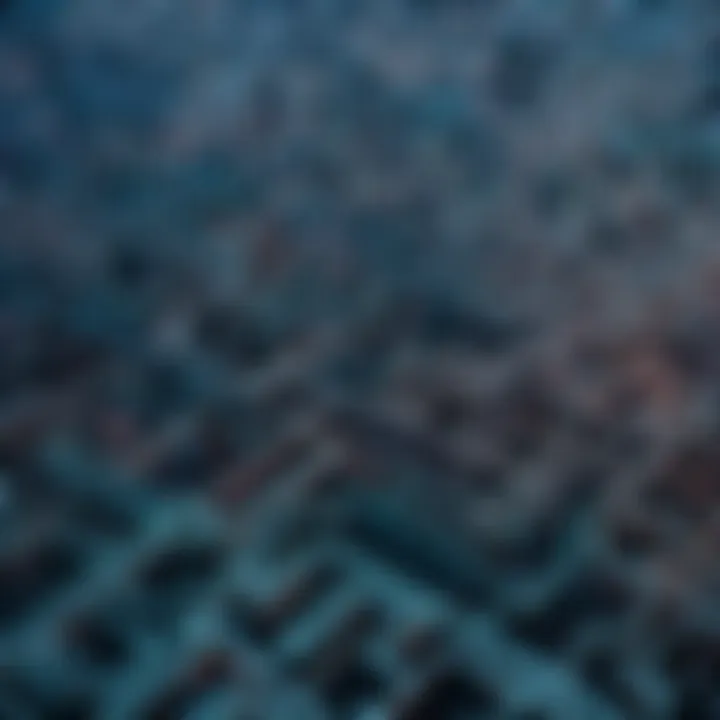
Elegant solution design using recursion
The elegant solution design using recursion epitomizes the finesse and sophistication embedded in recursive programming approaches. This aspect emphasizes the aesthetic appeal of recursive solutions, emphasizing not only the functional efficiency but also the elegance in code structure and logic flow. Through the utilization of recursive methodologies, programmers can architect solutions that transcend mere functionality, embodying a harmonious blend of efficiency, clarity, and aesthetic appeal. This design philosophy promotes the creation of codes that are not only effective in solving problems but also reflective of a meticulous and strategic approach to algorithmic design, elevating the programming experience to a realm of artistry and precision.
Efficient Memory Management
Reducing memory usage with recursive calls
Efficient memory management stands as a pivotal benefit of utilizing recursion in programming, particularly through the reduction of memory usage achieved by recursive calls. By optimizing the memory allocation and deallocation processes within recursive functions, programmers can minimize the overall memory footprint of their programs, enhancing system efficiency and performance. This reduction in memory usage not only facilitates smoother program execution but also contributes to overall system stability and resource allocation efficiency, ensuring optimal utilization of available memory resources.
Impact on program performance
The impact of recursion on program performance is undeniably profound, influencing the efficiency and speed of code execution significantly. Through well-optimized recursive functions, programmers can harness the inherent power of recursion to enhance program performance, leveraging recursive calls to streamline processes and enhance computational speed. However, while recursion can bolster program performance, it is essential for programmers to exercise caution in optimizing recursive functions to prevent potential performance bottlenecks, ensuring that recursive methodologies enhance, rather than impede, the overall efficiency and responsiveness of their programs.
Challenges of Recursion
Recursion in coding introduces various challenges that programmers need to navigate effectively to optimize their algorithms. Understanding the potential obstacles helps in enhancing problem-solving skills and program efficiency. By addressing the challenges head-on, developers can improve their coding practices and produce more robust and effective solutions. The section on Challenges of Recursion delves into the critical aspects that programmers encounter when utilizing recursive techniques. It offers valuable insights into the complexities of recursion and equips readers with essential knowledge to overcome these hurdles proficiently.
Risk of Stack Overflow
Issues with infinite recursion
Infinite recursion poses a significant risk in programming, leading to a stack overflow when a function calls itself indefinitely without reaching a proper termination condition. This aspect of recursion can cause memory leaks and program crashes, impacting the overall stability of the software. Understanding how to identify and rectify infinite recursion is crucial for maintaining the integrity of code and preventing system failures. By exploring the nuances of issues with infinite recursion, programmers can implement safeguards to mitigate this common pitfall in recursive functions.
Optimizing recursive functions
Optimizing recursive functions is essential to enhance performance and efficiency in coding projects. By refining the algorithms and structure of recursive functions, developers can reduce overheads and improve the execution speed of their programs. This optimization process involves streamlining recursive calls, implementing proper base cases, and minimizing redundant computations. Through strategic optimization techniques, programmers can achieve optimal results and elevate the overall quality of their recursive implementations.
Complexity and Readability
Difficulty in tracing recursive calls
Tracing recursive calls can be challenging due to the dynamic nature of function invocations within the recursive paradigm. Understanding the flow of recursive functions and tracking parameter values across multiple recursive levels requires careful attention to detail and logical reasoning. This aspect of recursion adds a layer of complexity to code comprehension, necessitating thorough testing and debugging protocols to ensure accuracy and consistency. By acknowledging the difficulty in tracing recursive calls, programmers can adopt systematic approaches to manage complexity effectively and enhance the readability of their code.
Alternative approaches for clarity
In situations where recursive solutions may introduce readability challenges, exploring alternative approaches can offer clarity and simplicity in problem-solving. By considering iterative methods or utilizing data structures like stacks and queues, programmers can devise alternative strategies to achieve the desired outcomes efficiently. This flexibility in approach empowers developers to choose the most suitable solution based on the nature of the problem and the project requirements. Incorporating alternative approaches for clarity ensures that code remains accessible and easy to maintain, promoting a streamlined development process.
Best Practices for Recursion
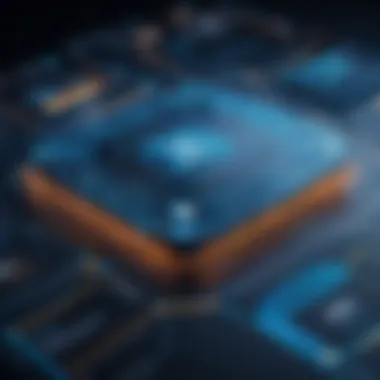
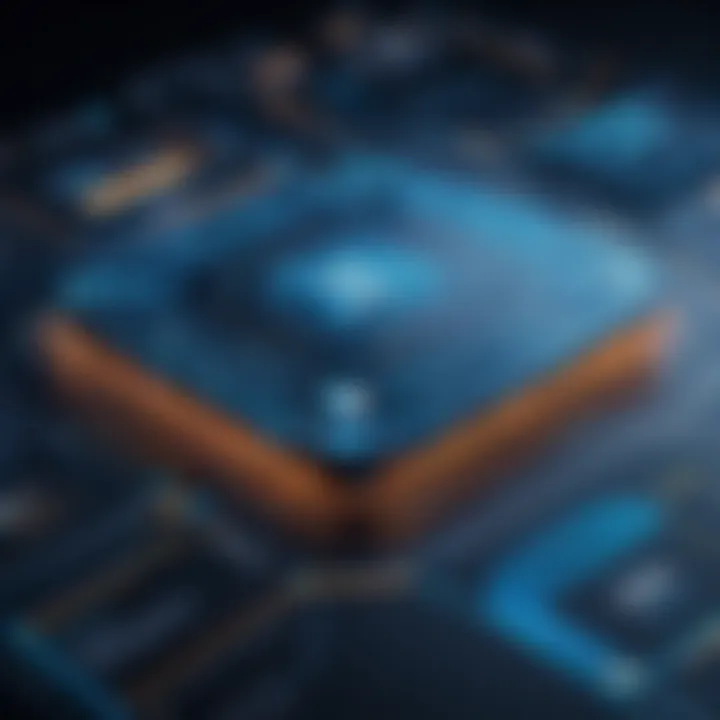
Recursion, a fundamental concept in programming, requires meticulous adherence to best practices to ensure efficient problem-solving and code elegance. In this section, we delve into the critical aspects of implementing recursion effectively. By following these practices, programmers can enhance their problem-solving skills and optimize program performance. Clear Problem Analysis plays a pivotal role in developing robust recursive functions, emphasizing the significance of strategic problem breakdown and base case definition. Crafting recursive solutions demands meticulous planning and strategic thinking to ensure optimal outcomes.
Clear Problem Analysis
Identifying suitable problems for recursion
Identifying suitable problems for recursion is pivotal in leveraging the technique's power effectively. By selecting problems that exhibit recursive patterns or can be broken down into smaller, similar subproblems, programmers can harness recursion's strength to its fullest potential. The key characteristic of Identifying suitable problems for recursion lies in recognizing tasks that involve self-referential repetition, aligning well with the recursive nature of the method. This selection is advantageous as it simplifies complex challenges by dividing them into manageable iterations, fostering a structured problem-solving approach within the context of this article.
Defining base cases effectively
Defining base cases effectively serves as the cornerstone of successful recursion, ensuring that recursive functions terminate at a specific condition. This aspect is crucial as it prevents infinite loops and guides the function to return the expected results. The key characteristic of effective base case definition is providing clear instructions for the termination of recursive calls, anchoring the algorithm in a defined endpoint. Effectively defined base cases are beneficial in maintaining program efficiency and preventing logical errors, contributing significantly to the overall success of recursive implementations detailed in this article.
Optimization Techniques
Tail recursion and its advantages
Tail recursion, an optimization technique in recursion, involves performing recursive calls as the final operation in a function. This approach streamlines memory management and enhances code readability by eliminating the need to store intermediate results between recursive calls. The key advantage of tail recursion lies in its potential to improve program efficiency and reduce memory overhead, making it a favorable choice for recursive algorithms detailed within this article.
Memoization for efficient recursion
Memoization, a dynamic programming technique, aids in optimizing recursive functions by storing and reusing previously computed results. By memorizing intermediate solutions, memoization eliminates redundant calculations and enhances overall performance. The unique feature of memoization lies in its ability to expedite recursive computations by eliminating repetitive work, thus optimizing recursive processes presented in this article.
Conclusion
Recursion is a fundamental concept in programming, offering a unique approach to problem-solving by allowing functions to call themselves. Through its exploration in this article, we have uncovered the significance of recursion in breaking down complex problems and designing elegant solutions. The consideration of base cases and termination conditions highlights the structured nature of recursive functions, contributing to their efficiency in memory management. Despite the challenges like stack overflow risks and concerns about readability, mastering recursion opens up new possibilities in algorithmic thinking.
Summary of Recursion
Recap of Key Concepts
The recap of key concepts in recursion emphasizes the essence of dividing problems into manageable tasks and the necessity of defining clear base cases for recursive functions. This technique showcases its prowess in tackling intricate problems by simplifying them into smaller, more digestible components. The recursive nature of this approach aids in creating elegant and efficient solutions, promoting a deeper understanding of algorithmic structures within the programming domain.
Impact of Recursion on Problem-Solving
The impact of recursion on problem-solving lies in its ability to offer a fresh perspective on approaching complex issues. By allowing functions to call themselves, recursion provides a recursive chain of solutions that lead to a more streamlined problem-solving process. This recursive nature enhances the logical flow of solutions, resulting in a harmonious approach to various programming challenges.
Future Perspectives
Advancements in Recursive Algorithms
The advancements in recursive algorithms signify a continuous evolution in the application of recursion within programming. By enhancing the efficiency and performance of recursive functions, these advancements pave the way for more sophisticated problem-solving techniques. The optimization of recursive algorithms aims to address memory management concerns and optimize the overall execution of recursive functions.
Integration of Recursion in Modern Programming
The integration of recursion in modern programming approaches emphasizes the relevance of this concept in contemporary coding practices. By seamlessly incorporating recursive functions into traditional programming paradigms, developers can harness the power of recursion to create innovative solutions. This integration not only enhances the versatility of programming languages but also promotes a deeper understanding of algorithmic design principles.