Exploring Prim's Algorithm for Minimum Spanning Trees
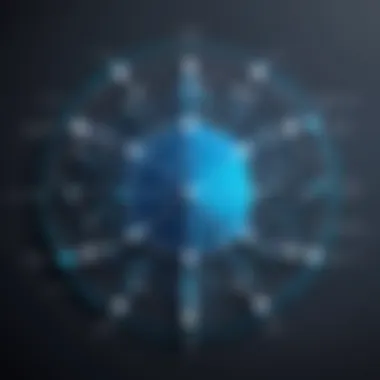
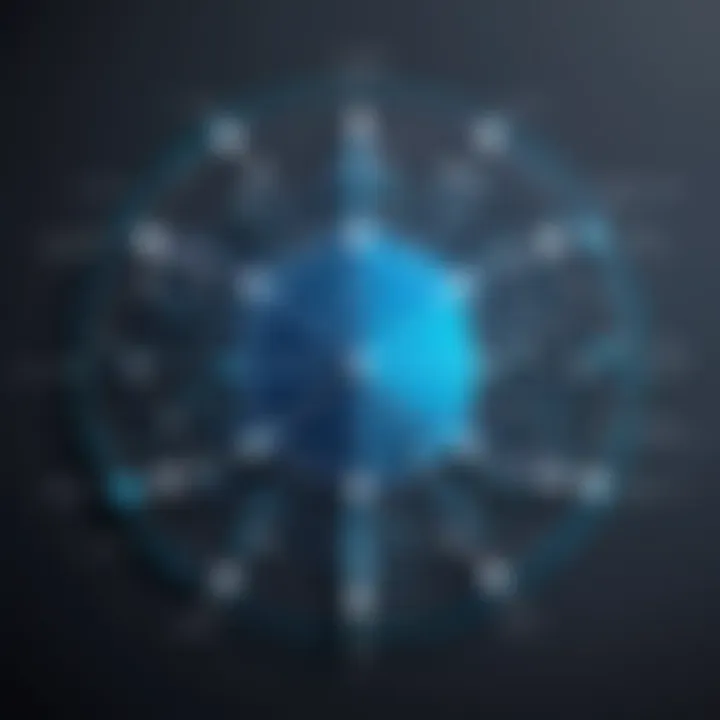
Intro
Prim's Algorithm is a cornerstone of graph theory, particularly in its application to constructing minimum spanning trees (MSTs). Understanding it is crucial for any budding programmer interested in data structures and algorithms. In simplest terms, a minimum spanning tree of a connected graph is a subset of the edges that connects all vertices with the least possible total edge weight, without forming any cycles. This can come in handy in various real-world applications like network design, resource management, and even road construction.
The beauty of Prim’s algorithm lies in its simplicity and efficiency. Using a greedy approach, it gradually builds the MST by adding edges one by one. Essentially, it starts with a single vertex and repeatedly adds the smallest edge that connects a vertex in the tree to a vertex outside the tree. But, it’s not just about the steps involved; understanding the when and why behind them is vital for grasping the algorithm.
This article will unpack the essence of Prim's algorithm by diving into its historical background, theoretical foundation, and real-world applications. We’ll also draw comparisons with Kruskal’s algorithm to provide a well-rounded perspective. By the end of this exploration, students and programming enthusiasts will find themselves well-equipped to implement the algorithm in languages like Java and C++.
Key Points to Discuss:
- The theoretical underpinnings of Prim's Algorithm.
- A step-by-step guide to its execution.
- An analysis of its time complexity.
- Practical applications in various domains.
- Comparisons with algorithms like Kruskal's.
Join us on this journey into the world of graphs and learn how Prim’s algorithm can sharpen your programming skills and enhance your understanding of data structures.
Prelims to Graph Theory
Graph theory, a vital component of mathematics and computer science, serves as the underlying framework for various real-world applications. Understanding graph theory is essential as it enables one to tackle problems related to connectivity, relationships, and optimization in numerous domains. In this article, we aim to focus on one specific aspect of graph theory—minimum spanning trees—using Prim's Algorithm as our primary tool.
Graphs, at their core, are mathematical structures that comprise vertices and edges. They provide a visual representation of complex relationships in a simplified manner. Herein lies the beauty of graph theory: it allows for the modeling of systems ranging from social networks to computer networks and transportation systems.
As we delve into the specifics of Prim's Algorithm, it becomes crucial to grasp the fundamental concepts of graph theory. This groundwork lays a pathway for understanding how we can efficiently find the minimum spanning tree within a given graph. With this integral knowledge, we can appreciate the significance and the mechanics of Prim’s Algorithm better.
Definition of Graphs
A graph is essentially a collection of nodes, which are referred to as vertices, and these nodes are interconnected by edges that represent relationships or pathways. This simple yet powerful concept translates into many practical applications. The nodes can signify anything from cities in a map to points in a network, while the edges reflect the connections between them whether it be a direct route or an association of some kind.
Types of Graphs
Graph theory encompasses various types of graphs, each serving distinct purposes. Here, we break them down into three primary categories:
- Directed Graphs: In a directed graph, the edges have a specific direction. This means that if there's a connection from vertex A to vertex B, it doesn’t imply that you can travel from B back to A. This characteristic allows directed graphs to reflect one-way streets or similar scenarios where directionality matters. They're commonly used in tasks such as web page linking, where the direction of the links is crucial.
- Undirected Graphs: Contrary to directed graphs, undirected graphs have edges that do not have a direction. If there's a path between two vertices A and B, it means you can travel both ways. This type is beneficial for modeling relationships like friendships in social networks or two-way streets in a city. The equality of travel in both directions adds simplicity and is often more intuitive in various contexts.
- Weighted Graphs: When edges in a graph carry weights, they are termed as weighted graphs. These weights could represent distances, costs, or any metric you wish to consider, adding another layer of complexity to your analysis. In practical scenarios, such as road networks where the distance is a critical factor, weighted graphs provide a nuanced understanding of optimization problems by allowing the measurement of shortest or most cost-effective paths.
Importance of Spanning Trees
Spanning trees are essential in ensuring that all the vertices in a graph are connected without any cycles. They are especially significant for network optimization, minimizing redundancy while maintaining connectivity. In the larger context of graph theory, finding a minimum spanning tree is crucial as it provides the most efficient way to connect all points, an endeavor paramount in applications like telecommunications, electrical grid design, and transportation engineering.
"In a minimum spanning tree, there's no shortcut—just the wisest path."
Understanding spanning trees not only gives insight into efficiency and optimization but also reveals an elegant way to manage and structure various interconnected systems. As such, we set the stage for a deeper exploration of Prim's Algorithm, which is uniquely designed to handle these graphs and their properties.
Understanding Minimum Spanning Trees
Minimum spanning trees (MST) play a critical role in graph theory and have vast applications across various fields. The concept revolves around selecting a subset of edges from a connected, undirected graph that connects all the vertices without cycles, ensuring the total edge weight is minimized. This foundational principle not only aids in efficient resource management but also serves as a springboard for more complex algorithms.
Understanding MST allows programmers to appreciate how to simplify network design or optimize routing problems. It's a key topic for developing algorithms that can handle real-world issues such as determining the least expensive setup for connections, whether that’s in telecommunications or transportation systems. Moreover, recognizing the nuances of MST bridges theoretical computations and practical applications, which can enhance students' and professionals’ problem-solving capabilities significantly.
Definition and Characteristics
A minimum spanning tree of a given weighted graph is a tree such that:
- It includes every vertex in the graph.
- The sum of the weights (costs) of the edges in the tree is minimized.
- There are no cycles present in the tree.
This definition sets the groundwork for many algorithmic strategies. The characteristics that make MSTs particularly interesting involve properties like uniqueness; some graphs have multiple MSTs with the same total weight, while others have a unique solution. Understanding this is crucial for executing algorithms like Prim’s and Kruskal’s effectively. Furthermore, an MST is a subset of the original graph, which showcases how trimming down data can lead to new insights and functionality.
Properties of Minimum Spanning Trees
Minimum spanning trees possess several properties that make them integral to algorithm design:
- Connectedness: MST inherently contains all vertices, ensuring that there are no isolated nodes.
- Acyclicity: Since a spanning tree is always acyclic, it prevents the looping back of paths, which would unnecessarily bloat the total cost.
- Edge Cut Property: If an edge is removed, an MST will still hold true as long as the remaining structure can continue to connect all vertices.
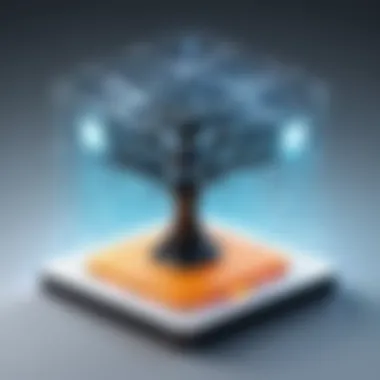
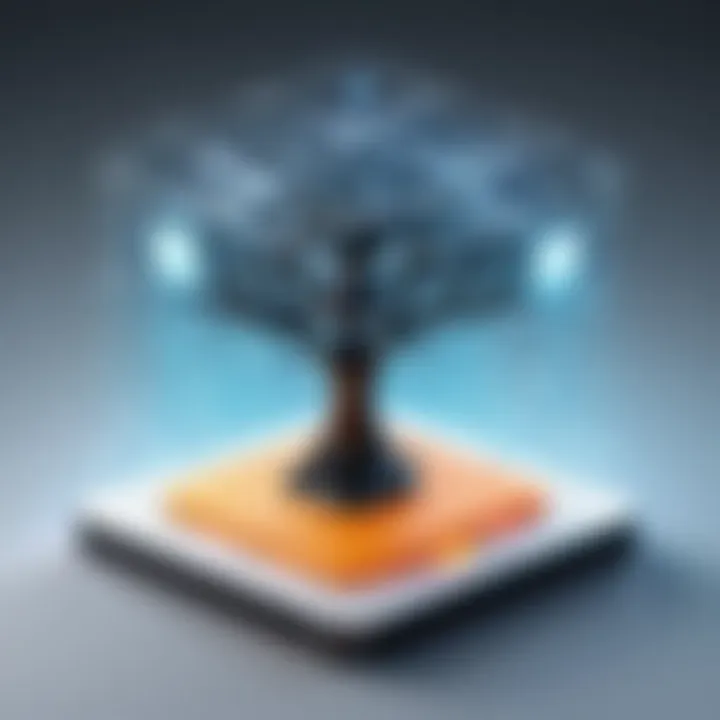
These properties guide the optimization process in both computational and real-world scenarios, making them a favorite among engineers and computer scientists alike.
Real-World Applications
In many applications, the principles behind minimum spanning trees find their utility in diverse fields:
Network Design
Network design utilizes MST concepts to create efficient connections in communication networks, data transmission channels, and electrical grids. The goal is to minimize costs while maximally connecting nodes, ensuring data can move seamlessly without unnecessary expenditure on redundant paths. A notable aspect of network design is its emphasis on scalability and reliability: as networks grow, the ability to maintain an efficient weight structure is crucial.
One outstanding feature is its adaptability; network designs can shift dynamically with changes, such as adding new nodes. The advantage here is clear: minimizing resource expenditure while maximizing reach ultimately improves efficiency and effectiveness in the long run.
Cluster Analysis
Cluster analysis employs MST principles in data mining and statistics to effectively group similar data points while maintaining a low cost of interaction. This method allows analysts to visualize data interrelationships in a condensed manner. A key characteristic of cluster analysis is its objective to identify patterns or anomalies within data sets, like categorizing customer behavior or disease patterns.
The unique feature of using MST in clustering is its systematic approach, as it focuses not just on grouping but also on maintaining proximity while minimizing distances. The advantage of this method is it can yield clear, actionable insights from complex datasets without overwhelming the user with unnecessary information.
Transportation Problems
Transportation problems leverage MSTs to optimize routes and reduce transportation costs. Whether it's routing delivery trucks or managing supply chains, the task is to find the minimal path that reaches all destinations without incurring high costs. This field’s significant characteristic is the demand for efficiency amid complexity; various routes and options often complicate decision-making.
The unique feature of applying MSTs here is that it helps simplify the complexities involved in route optimization. By streamlining decisions for real-world problems, it not only saves money but also time for businesses dealing with logistics. The challenge, however, lies in balancing between routes and constraints, which can sometimes lead to suboptimal solutions if not carefully analyzed.
These applications showcase how understanding minimum spanning trees not only helps in theoretical computer science but also enhances practical implementations, emphasizing the broader impact of this essential concept.
Detailed Explanation of Prim's Algorithm
In this section, we will unravel the intricacies of Prim's Algorithm, shedding light on its significance in graph theory and its role in constructing minimum spanning trees. This algorithm serves as a vital tool in various fields of computer science, especially for those delving into data structures and optimization problems. Understanding the detailed workings of this algorithm not only enhances programming skills but also sharpens critical thinking and problem-solving abilities.
Algorithm Overview
Prim's Algorithm is designed to find a minimum spanning tree (MST) for a weighted undirected graph. The essence of this algorithm lies in its greedy approach, always opting for the edge with the smallest weight that connects a vertex to the growing spanning tree. It starts with a single vertex and incrementally builds up a tree by continually adding the cheapest edge from any vertex already in the tree to a vertex outside of it until all vertices are included.
This method is particularly effective when dealing with dense graphs because it notices the weight of edges directly to reach a conclusion swiftly. The simplicity and efficiency of Prim's Algorithm make it a reliable choice for developers and analysts. The algorithm guarantees an MST if the graph is connected, emphasizing its robustness in real-world applications.
Step-by-Step Process
Initialization of Nodes
The first step in Prim's Algorithm is the Initialization of Nodes. Here, you select a starting vertex and mark it as part of the growing spanning tree. This decision is crucial because it sets the foundation for how subsequently connected nodes are chosen. The beauty of this phase lies in its straightforwardness; every node begins marked unvisited except the selected starting vertex.
- Key Characteristic: One defining feature is the simplicity of starting with any vertex, which allows flexibility.
- Advantages: Because any vertex can be chosen arbitrarily, it becomes easy to implement, catering well to various types of graph structures.
- Unique Feature: The algorithm's incremental design urges revisiting vertices that can yield minimum weight edges, thus making it dynamic in its approach.
Selection of Edges
Next comes the Selection of Edges, where the algorithm seeks the least weight edge that connects the growing tree to a new vertex. At each stage, the minimum edge connecting any vertex in the tree to one outside of it is added.
- Key Characteristic: The greediness of this step defines Prim's methodology, as it prioritizes local optimum choices in hopes of reaching a global optimum.
- Advantages: It effectively narrows down choices at each iteration, making the computational process more efficient.
- Unique Feature: This focus on the edge weights often leads to a faster convergence, especially in sparse graphs, where fewer edges translate to fewer calculations.
Revisiting Nodes
Finally, we delve into Revisiting Nodes, a crucial aspect of the algorithm. After selecting an edge and adding a vertex to the tree, the algorithm checks again for edges that lead to newly added vertices to ensure that edge selection remains optimal.
- Key Characteristic: This functionality ensures that the algorithm does not overlook cheaper options after adding a new vertex.
- Advantages: It enhances adaptability, keeping the spanning tree as lightweight as possible in real time.
- Unique Feature: The algorithm's flexibility allows refreshment of node connections that can lead to better options – an invaluable trait for complex graphs.
"With every edge selected, Prim's Algorithm ensures an evolving yet coherent construction of the minimum spanning tree."
By following this systematic approach, Prim's Algorithm elegantly navigates through nodes and edges to construct an efficient minimum spanning tree. This detailed understanding lays the groundwork for practical applications and will be explored through various programming implementations in subsequent sections.
Time Complexity Analysis
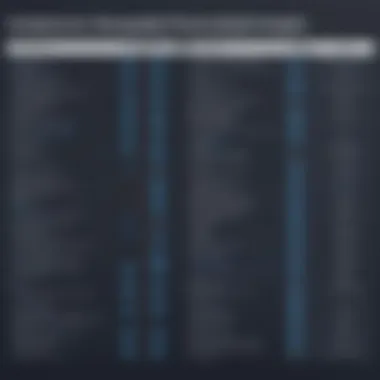
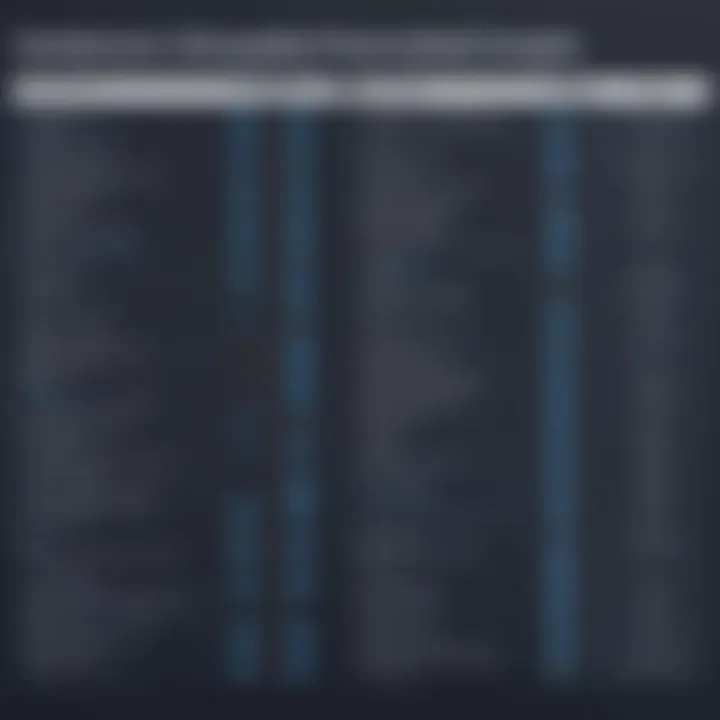
Time complexity analysis forms the backbone of understanding algorithms like Prim's Algorithm for Minimum Spanning Trees. Taking a deep dive into this topic ensures that learners grasp how efficient these algorithms are, especially as the size of the input grows. When programming, one must often consider not just the outcome but how long it takes to get there. Emphasizing time complexity equips one with the tools to discern between different algorithms and choose the most effective one for a given problem. This section will navigate through complexity notation and how Prim's Algorithm stacks up against its counterparts.
Understanding Complexity Notation
Complexity notation provides a standardized way to express the performance characteristics of algorithms. It’s essential to understand these notations, as they encapsulate how an algorithm’s run-time scales with respect to the input size. Two primary forms of notation come into play here:
- Big O Notation: This describes the upper limit of the running time, providing a worst-case scenario overview.
- Omega (Ω) Notation: This represents the lower bound, indicating the best-case performance.
- Theta (Θ) Notation: This notation gives a tight bound, meaning that it provides both upper and lower bounds.
These terms may sound technical, yet they simplify discussions on performance, allowing programmers to communicate clearly about an algorithm's efficiency.
Big O Notation for Prim's Algorithm
When assessing Prim's Algorithm, it’s often put through the lens of Big O notation to evaluate its efficiency. The core operation in Prim's Algorithm is the repeated selection of the minimum weight edge, which involves checking all edges connected to the growing minimum spanning tree. If a priority queue is used, the overall time complexity is typically represented as O(E log V), where E indicates the number of edges, and V is the number of vertices in the graph.
Comparison with Other Algorithms
In the realm of graph algorithms, comparing Prim's with others like Kruskal’s reveals crucial insights. Kruskal's Algorithm also has a time complexity of O(E log E) under optimal conditions. This makes Prim's Algorithm a competitive alternative, especially when one considers the structures of the graphs.
- Special Characteristics: Prim's tends to be more efficient on dense graphs compared to Kruskal’s, which shines in sparse graphs. This characteristic makes it a beneficial choice when dealing with weighty connections in graphs with high vertex counts.
Contextual Performance Evaluation
Taking the complexity further, evaluating Prim's Algorithm in context yields valuable insights. Depending on the topology of the input graph, the perceived performance can shift dramatically.
- Key Strengths: Prim's excels in cases where the graph is dense and allows for faster edge access due to better connectivity. Using data structures like Fibonacci heaps can further optimize its time complexity to O(E + V log V), which showcases its adaptability.
- Weaknesses: On the flip side, in sparse graphs, it may lag behind Kruskal’s, making it vital to choose the right algorithm based on the nature of the problem at hand.
Understanding the interplay of these factors arms learners with the know-how to deploy the right algorithm that fits their specific needs.
"The choice of the right algorithm is like a key to unlock possibilities in programming."
In summary, a solid understanding of time complexity enhances programming effectiveness. With insights into Big O notation and comparative performance analysis, one can approach challenges in graph theory with confidence.
Implementing Prim's Algorithm
Implementing Prim's Algorithm is crucial for anyone working with graph theory and minimum spanning trees. It brings theory into practice, allowing students and programmers to grasp the concept of these structures through hands-on experience. With a solid understanding of how Prim's Algorithm operates, learners can develop their skills in programming languages such as Java and C++. This practical implementation serves as a bridge between abstract concepts and real-world applications.
Prim's Algorithm in Java
Code Structure and Design
When designing Prim's Algorithm in Java, the code structure needs to be clear, efficient, and easy to follow. The emphasis on modularity stands out significantly here. Each part of the algorithm can be separated into functions or classes, which helps with readability and maintenance. A typical characteristic is the use of priority queues that manage edges based on weight. This choice enhances the algorithm’s efficiency while also adhering to Java's object-oriented principles.
One unique feature of this design is how Java's collection framework simplifies managing data structures. This convenience can lead to quicker development times, although it may also bloat the code with too many abstracted features. Keeping code lean while using Java's robust features is a balance every programmer must tackle.
Stepwise Implementation
The stepwise implementation of Prim's Algorithm in Java provides a structured pathway to tackle problems systematically. Highlighting clarity in code execution is paramount. Breaking down the algorithm into distinct steps – initializing nodes, selecting edges, and revisiting nodes – showcases a logical progression. This segmented approach allows learners to grasp each part without getting overwhelmed.
Java’s strong typing system means that each step can be carefully monitored for errors, which is a boon for beginners. However, this strictness can also lead to verbosity, making the code appear cumbersome to some.
Prim's Algorithm in ++
Code Structure and Design
In C++, the code structure of Prim's Algorithm is often characterized by the use of vectors and pairs. This method promotes efficiency in managing edge lists and weights, which are core to the algorithm's function. The use of C++’s Standard Template Library (STL) allows developers to leverage built-in data structures, making the implementation both swift and straightforward.
One advantage of this structure is how it integrates seamlessly with performance optimizations. Yet the flexibility of ++ can lead to tangible complexities, and managing memory becomes an essential consideration that developers must not overlook.
Stepwise Implementation
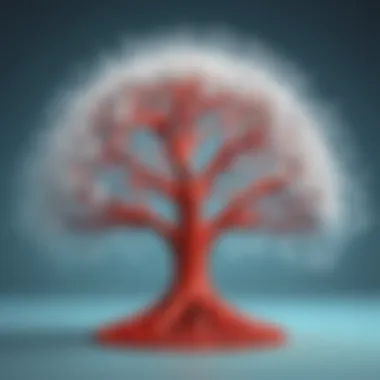
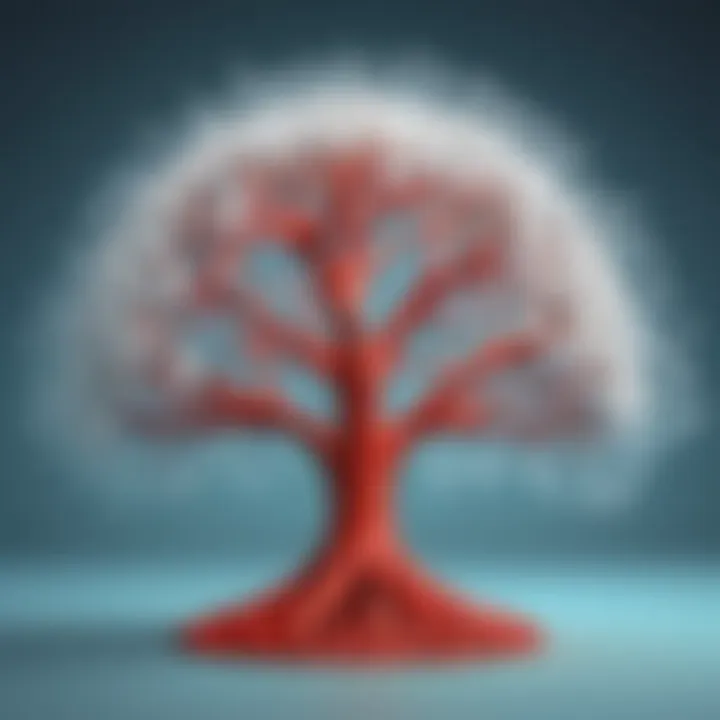
C++ excels in stepwise implementation thanks to its low-level capabilities. As programmers begin breaking down Prim's Algorithm, they can exploit features like manual memory management. Each step of the algorithm – from the initialization of nodes to handling edge cases – can be meticulously tailored to suit specific needs.
This hands-on approach can significantly boost understanding and control, but it demands a higher level of understanding from the programmer. Newcomers might find themselves grappling with pointers and memory leaks if they don't tread carefully.
Mastering the implementation of Prim's Algorithm is not merely about coding; it's about cultivating a deeper understanding of how to manipulate data structures efficiently. Each programming language has its own nuances that allows for diverse methodologies in execution.
In summary, implementing Prim's Algorithm in Java and C++ reveals different strengths and nuances inherent to each language, making them suitable for various educational purposes and practical applications.
Comparison with Other Algorithms
Understanding how Prim's Algorithm fits within the larger landscape of algorithms for finding minimum spanning trees is crucial for programmers and computer scientists alike. This section sheds light on the relevance of comparing Prim's Algorithm with alternatives, such as Kruskal's Algorithm, as it enables learners to grasp the nuanced differences and advantages of each method. Moreover, these comparisons unveil the scenarios where one algorithm proves more advantageous than the other.
Kruskal’s Algorithm
Kruskal's Algorithm is a powerful approach for constructing minimum spanning trees, particularly in cases where the graph is sparse. Unlike Prim's, which builds the tree from a single vertex outward, Kruskal's operates by sorting all the edges in the graph and adding them one by one, ensuring that no cycles are formed. This makes Kruskal’s algorithm highly suitable for certain types of graphs where quick access to edge weights can be beneficial.
Differences and Similarities
Algorithmic Approach
The algorithmic approach of Prim’s Algorithm centers on growing the minimum spanning tree from a single vertex, expanding it gradually by adding the nearest neighbor. This method is characterized by its clarity and directness, making it particularly digestible for those new to graph theory. In contrast, Kruskal’s emphasizes a more global perspective; it considers all potential edges from the start, allowing it to efficiently handle disconnected components.
- Key Characteristic: Prim’s is easily implemented with an adjacency list, whereas Kruskal's often requires a priority queue for optimal performance.
- Unique Feature: Prim's can be implemented using a greedy strategy which provides immediate feedback on tree growth, while Kruskal's entirely depends on edge sorting, which may be less intuitive for beginners.
- Advantages: Prim’s is often faster for dense graphs due to its localized expansion, while Kruskal's excels in handling sparse graphs.
Performance Metrics
When delving into performance metrics, both algorithms have their strengths. Prim's Algorithm typically operates in O(E log V) time complexity when using priority queues, making it efficient for dense graphs. On the other hand, Kruskal’s Algorithm has a time complexity of O(E log E) since it requires sorting edges, which can occasionally lead to slower performance on edges-heavy problems.
- Key Characteristic: In terms of execution, a sparse graph will generally favor Kruskal's efficiency but could overwhelm it if many edges need inspecting.
- Unique Feature: Prim's can adapt easily to changes in graphs if a single edge or vertex is modified, while Kruskal's requires complete re-evaluation, potentially slowing down the process.
- Advantages: The adaptability of Prim's gives it a robust edge in dynamic applications where the graph structure might change often, whereas Kruskal's maintains a steadfast performance in static environments with a fixed edge set.
The choice between Prim’s and Kruskal’s ultimately boils down to the specific characteristics of the graph at hand and the particular requirements of the application in question.
Common Challenges in Implementing Prim's Algorithm
Prim's Algorithm appears straightforward on the surface, but like getting lost in a maze, things can easily become complex when you dive deeper. A robust understanding of the common challenges that may arise during its implementation is vital. These challenges not only test your skills but also enhance your intuitive grasp of graph-theoretical concepts. Addressing these hurdles can make a significant difference in your ability to apply the algorithm effectively.
Handling Edge Cases
In practical programming, edge cases can feel like the thorn in your side. They often pop up unexpectedly, leaving you scratching your head. For Prim's Algorithm, edge cases typically involve graphs that present unique structures or conditions. Some common edge cases to consider include:
- Graphs with no edges: In such a scenario, Prim's cannot function. Handling an empty graph gracefully is paramount.
- Singular nodes: A graph with only one node and no edges represents a trivial case, yet it shouldn't cause errors in your implementation.
- Nodes with equal edge weights: When multiple edges have the same weight, the algorithm might select any of them. Ensuring consistency in these situations can affect your results.
Navigating these edge cases often involves writing additional checks in your code. This extra planning improves robustness and can save time later. A well-planned approach to handling edge cases makes your toolset more versatile in tackling various graph structures.
Debugging Strategies
Debugging Prim's Algorithm can be as intricate as weaving a tapestry. When something goes awry, you need a clear plan to unravel the issues. Here are some strategies to consider:
- Verbose Logging: Implement detailed logging to track the algorithm's steps. Printing changes in the priority queue and selections made can shed light on where it veers off course.
- Visual Representation: Sometimes seeing is believing. Utilize graph visualization tools to depict how the algorithm progresses. Identifying the edges selected at each step can help pinpoint where issues emerge.
- Unit Testing: Before diving into complex graphs, test your implementation with very simple examples. This granular approach helps ensure your foundational logic is sound.
- Code Review: Sometimes, a fresh set of eyes can spot issues you've overlooked. Engaging with peers in code review can reveal paths for improvement that you may not have considered.
In essence, addressing challenges while implementing Prim’s Algorithm involves a conscientious mindset and a systematic approach. By focusing on edge cases and incorporating solid debugging techniques, you're on your way to mastering not just the algorithm but also the broader realm of graph theory. Remember, persistence and adaptability are your best allies in programming.
Finale
Prim's Algorithm comes in handy countless times in various real-world applications, from network design to transportation logistics, allowing professionals to solve practical complications effectively. This is where the significance of recognizing its workings shines through. By being well-acquainted with not just its step-by-step process but also its complexity and edge case handling, one can avoid potential pitfalls when implementing the algorithm.
The beauty of this algorithm lies in its straightforward approach while remaining powerful in terms of performance. This makes it an excellent starting point for those diving deep into data structures and algorithms. Furthermore, by comparing it with Kruskal’s Algorithm, readers gain a broader perspective of the algorithmic landscape, helping them choose the right tool for their specific problems.
Ultimately, students embarking on their programming journey can find immense value in the knowledge imparted here. The skills learned while mastering Prim’s Algorithm can become building blocks for more advanced topics in computer science, making this worthwhile to study.
"The power of understanding comes not only from learning algorithms but from applying them wisely in our programming endeavors."
Summary of Key Points
- Prim's Algorithm is crucial in creating efficient Minimum Spanning Trees from graphs.
- It provides practical solutions applicable to various fields including network design and transportation.
- Understanding its workings enables programmers to avoid common pitfalls in implementation.
- Comparisons with Kruskal’s Algorithm enrich understanding and competency in selecting algorithms.
- The educational insights gained form the basis for advancing in the broader area of computer science.
Thus, the exploration of Prim’s Algorithm contributes significantly to a programmer's toolkit, equipping them with knowledge and skills applicable in both theoretical and practical realms.