Understanding Polymorphism in Java: Concepts and Examples
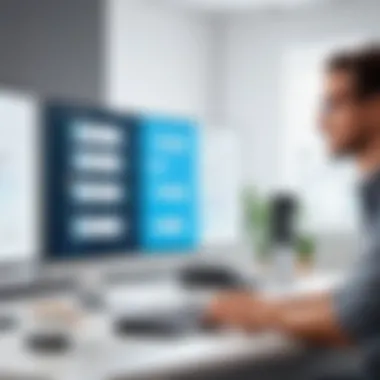
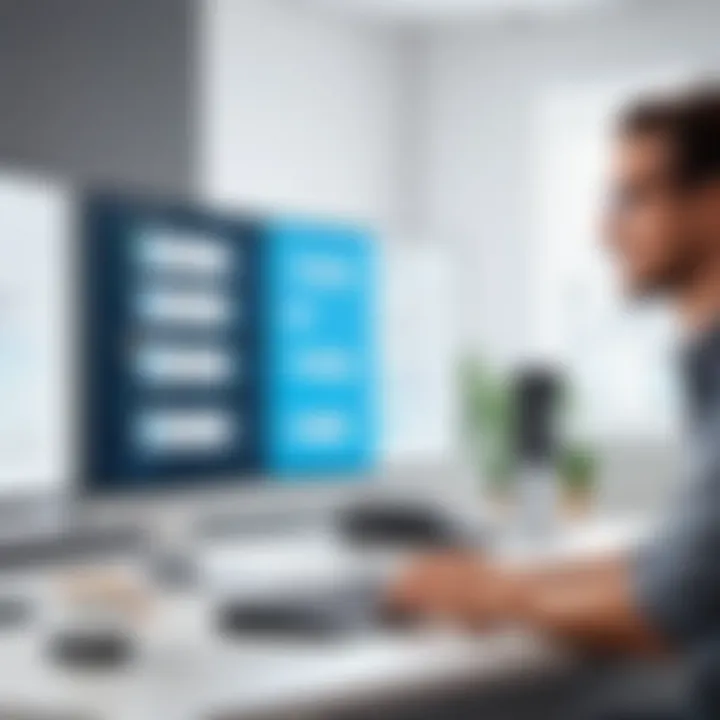
Intro
When one dives into the world of programming languages, it’s a bit like opening a treasure chest filled with tools and techniques designed to build software. Understanding the core principles of a language sets the pace for further explorations, especially when we talk about a concept as pivotal as polymorphism in Java. Polymorphism essentially allows methods to do different things based on the object that it is acting upon. It's like how a single word, say "run," can imply different actions, whether it's running a race or running software.
History and Background
Java first popped onto the scene in the mid-90s and was initially designed for interactive television, though it found its home in the world of web development. Over the years, Java became a go-to language for many due to its robustness and simplicity. In a programming world often marred by complexity, Java emerged with a promise of flexibility and compatibility. This was no small feat, and as programmers began adopting it in droves, it solidified itself as one of the leading languages.
Features and Uses
Java is renowned for its platform independence—often touted as "write once, run anywhere." But that’s not the only feather in its cap. Some features include:
- Object-Oriented: Everything in Java is an object, which helps in organizing complex code.
- Rich API: Massive sets of libraries and tools simplify tasks.
- Automatic Memory Management: The garbage collector takes care of memory, lessening the burden on developers.
- Multi-threading: Java can handle multiple tasks at once, improving efficiency.
Popularity and Scope
It’s hardly a surprise that Java tickles the fancy of programmers worldwide, from beginners to seasoned pros. It’s widely used in enterprise software, Android app development, and large systems programming. Given the growth of data handling and cloud computing, Java's relevance isn’t withering; if anything, it’s only intensifying. The vast community also fosters collaboration and knowledge sharing, making it a great choice for learners.
Polymorphism in Java
Now that we’ve got the basics about Java, let’s hit the ground running into the specifics of polymorphism. As mentioned earlier, polymorphism plays a dual role in enhancing flexibility in code and facilitating easier maintenance. It allows methods to operate on objects of different classes, improving the adaptability of the code.
Static and Dynamic Polymorphism
Polymorphism isn’t a monolith; it branches into two primary types: static (or compile-time) and dynamic (or run-time).
Static Polymorphism: This happens when a method is called based on how many arguments it takes or their type. Method overloading is a classic example. For example:
Here, the same method name operates differently based on the parameter types.
Dynamic Polymorphism: On the flip side, this occurs when a method call is resolved at run-time. It’s typically associated with method overriding, where a subclass provides a specific implementation of a method that is already defined in its parent class. Consider:
In this case, the correct method is chosen based on the object instance, not the declaration type.
"Polymorphism is a principle that teaches us to invest in adaptability for complex scenarios."
As we peel back the layers of polymorphism, it's essential to grasp how this principle can significantly streamline coding processes, making it easier to manage and expand programs when new features need incorporation.
End
Understanding Polymorphism
Polymorphism stands as a cornerstone in the edifice of object-oriented programming, particularly within the Java language. It allows developers to interact with objects more abstractly, which can simplify code and make systems more modular and comprehensible. At its core, polymorphism enables different classes to be treated as instances of the same class through a common interface, which can lead to more flexible and reusable code. In a world where time and resources are limited, being able to leverage polymorphism can save substantial effort in both development and maintenance phases.
Moreover, understanding polymorphism is essential for grasping more advanced programming concepts and design patterns. Getting a firm handle on this topic unlocks the door to smart, efficient coding practices that are not only effective but also maintainable over time. Through discovering the various facets of polymorphism, learners also cultivate a mindset of thinking in terms of objects and interactions, laying the groundwork for more robust software engineering skills.
Definition of Polymorphism
Polymorphism, a term derived from Greek, literally means "many forms." In programming, particularly in Java, it refers to the ability of a single function or method to operate on different kinds of objects. Essentially, it allows methods to do different things based on the object that it is acting upon, despite appearing identical in call.
For example, a method named could be used for various shapes such as circles, squares, or triangles. Each of these shapes would implement the method uniquely, yet the caller remains blissfully unaware of the underlying implementation details.
Here's a quick code snippet illustrating this:
Historical Context
Polymorphism’s roots can be traced back to early programming languages but gained traction and formalization with the advent of object-oriented programming in the 1980s. Languages like Smalltalk and C++ moved the concept of polymorphism to the forefront by allowing methods to be overloaded and overridden, enhancing the flexibility of code. Java, which debuted in 1995, took these principles and incorporated them seamlessly, allowing developers to write robust, maintainable code. By supporting both compile-time (static) and runtime (dynamic) polymorphism, Java has fostered an environment where code can be more versatile and less prone to errors. Today, polymorphism remains not just a concept from programming lore, but a vital practice in modern application development.
Importance in Object-Oriented Programming
In object-oriented programming, polymorphism plays a pivotal role. First, it promotes code reusability and efficiency. Instead of writing boilerplate code for similar functions, polymorphism helps in achieving the same functionality via a single interface, thereby minimizing redundancy. Further, it enhances the flexibility of code by allowing methods to be extended without changing their core functionalities.
Additionally, polymorphism supports a clear hierarchy in code structure. It allows for clear, organized classification of classes and their associated behaviors, which can be crucial in larger systems. Moreover, it reduces the complexity of code, making it easier to read and maintain.
If you want a system that is easy to extend and maintain, embrace polymorphism. This is how you foster more resilient code bases.
By exploring these elements of polymorphism, one can appreciate how this concept streamlines programming in Java, making it an invaluable tool for any developer's toolbox.
Types of Polymorphism
In the realm of Java and object-oriented programming, understanding the distinct types of polymorphism is vital. It enhances the code's versatility, allowing for both efficiency and readability. Identifying when to utilize each type can significantly inform design decisions and optimize performance in applications. Let's dive into the two main categories: compile-time and run-time polymorphism.
Compile-Time Polymorphism
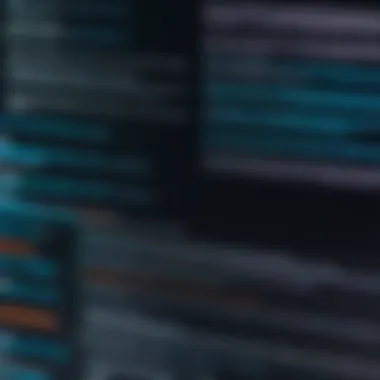
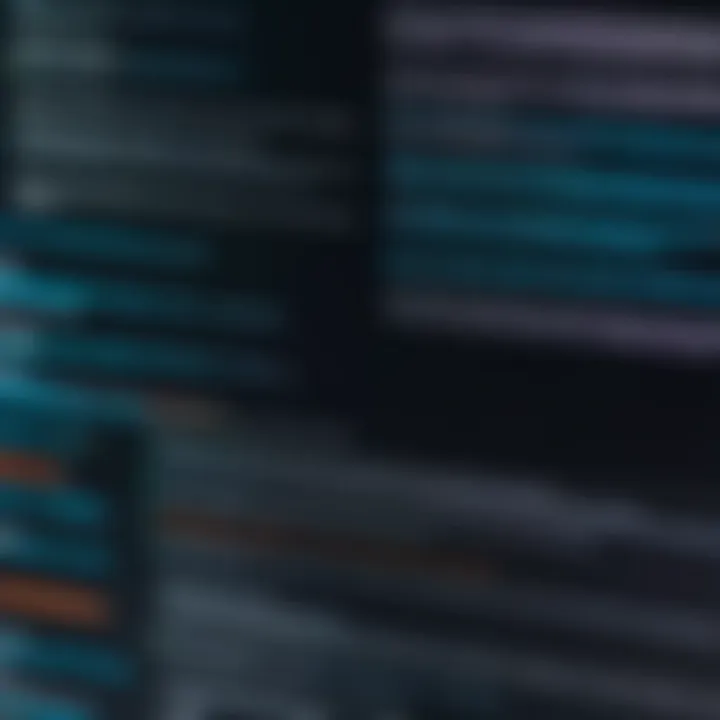
Compile-time polymorphism, often referred to as static polymorphism, occurs when the method to be invoked is determined at compile time. It primarily springs from two concepts: method overloading and operator overloading. Both concepts allow developers to write more intuitive code while reducing redundancy.
Method Overloading
When discussing method overloading, we tap into a hallmark of compile-time polymorphism. This is where methods share the same name but differ in parameters — either in the number of parameters, their types, or both. The main benefit of this approach is that it provides clarity. Developers can use a single name to represent different functionalities, making the code cleaner and easier to manage.
Consider a simple example:
Here, the method is overloaded to handle both integers and doubles. A unique feature of method overloading is that it prioritizes dimensionality over naming, which often makes the code self-explanatory. However, its disadvantage lies in potential ambiguity, especially when the parameters of overloaded methods are too similar or the types are not distinct enough, leading to confusion during reading and debugging.
Operator Overloading
Although Java doesn’t support operator overloading in the same way languages like C++ do, it’s worth acknowledging its concept as part of compile-time polymorphism. In languages that support it, operator overloading allows programmers to define new behaviors for existing operators based on the operands' types. For example, you could customize the behavior of for custom classes.
The key characteristic here is flexibility; it lets developers extend the language’s capabilities naturally. Despite its allure, operator overloading can lead to code that is difficult to understand. Misuse can result in expressions that are hard to read, reducing maintainability and increasing the learning curve for new developers.
Run-Time Polymorphism
In contrast to compile-time polymorphism, run-time polymorphism, or dynamic polymorphism, unfolds during the program’s execution. This is achieved through method overriding, where a subclass provides a specific implementation of a method that is already defined in its superclass.
Method Overriding
Method overriding plays a crucial role in facilitating run-time polymorphism. It allows a subclass to offer a specific version of a method that’s more suitable for its context. The core of this practice is rooted in the principle of substitution, enabling objects to be treated as instances of their parent class.
Here’s a simple illustration:
In this scenario, both the class and the class have a method named . When you call the method on a reference, the overridden method in the subclass executes, showcasing the beauty of polymorphism. This ability is highly beneficial as it promotes flexible code that can easily adapt to changing requirements. However, a potential pitfall is the increased complexity in understanding class hierarchies, which can often make debugging a bit of a challenge.
Dynamic Method Dispatch
Dynamic method dispatch is the mechanism behind run-time polymorphism. It ensures method calls are resolved at runtime based on the object’s type, rather than the variable type. This is a crucial feature because it gives flexibility in how methods and operations are handled.
The unique edge of this approach is that it makes your code extensible and adaptable. However, it can also lead to performance drawbacks when dealing with large hierarchies, or when heavy reliance on polymorphism increases method resolution overhead.
In sum, understanding both compile-time and run-time polymorphism is essential for Java enthusiasts. It not only fosters better coding practices but elevates the ability to create reusable and maintainable code. As we navigate these types, recognizing the specific advantages and potential drawbacks lends clarity to their implementation.
Implementing Polymorphism in Java
In the realm of coding, particularly in Java, implementing polymorphism holds a significant place. It acts like a glue that binds various coding paradigms together, allowing programmers to create more flexible and maintainable code. Polymorphism enables the same function or method to behave differently based on the object that it is acting upon. As such, it not only enhances code clarity but also fosters a programming environment where changes and modifications can be made with minimal impact on existing code structures. This section seeks to explore the critical aspects of implementing polymorphism in Java, including the ways it can be executed effectively and the benefits it brings to the coding process.
Method Overloading in Practice
Method overloading stands at the forefront when discussing polymorphism in Java. This technique allows multiple methods to have the same name but different parameter lists within the same class. It showcases how a single action can be performed in different ways, depending on the inputs provided. This not only makes method names more intuitive but also enhances code readability.
Example of Overloaded Methods
When we talk about overloaded methods, consider a simple scenario of a class named . In this class, you might have multiple methods:
In this example, the method is overloaded with various signatures. What makes this beneficial is its clarity; if a developer sees , they instantly understand it's an addition operation, despite differences in arguments. The unique feature here is flexibility in handling various data types and numbers of parameters. However, one must be cautious, as excessive method overloading can lead to ambiguity and confusion, particularly if methods have similar signatures.
Common Use Cases
Common use cases for method overloading are found in many libraries and APIs. For instance, the method in Java's class. It can take different argument types. This versatility allows developers to handle various output scenarios seamlessly.
The real charm of method overloading lies in its ability to simplify the code and reduce name collisions. Yet, this approach does require a careful thought process to avoid making the code base more convoluted than it needs to be. Therefore, use it wisely by ensuring methods are logically related.
Method Overriding Explained
While method overloading fosters similarities, method overriding introduces a refreshing contrast. This feature allows a subclass to provide a specific implementation of a method already defined in its superclass. It underscores the principle that a child class can redefine behaviors that it inherits.
Base and Derived Classes
Consider a superclass named with a method . A subclass might override this method to return "Bark", while a class might return "Meow". This ability to change how a method behaves in subclasses is fantastic for writing clean and logically sound code.
One must note that overriding promotes a clear inheritance hierarchy. While beneficial, it's also important to ensure that overridden methods maintain the same method signature. If not, the system could throw unexpected errors, making debugging a tad harder than it ought to be.
Example of Overridden Methods
Here's a brief Java snippet illustrating this:
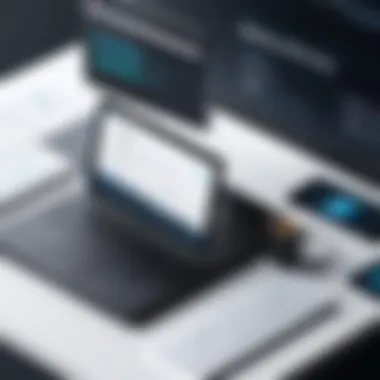
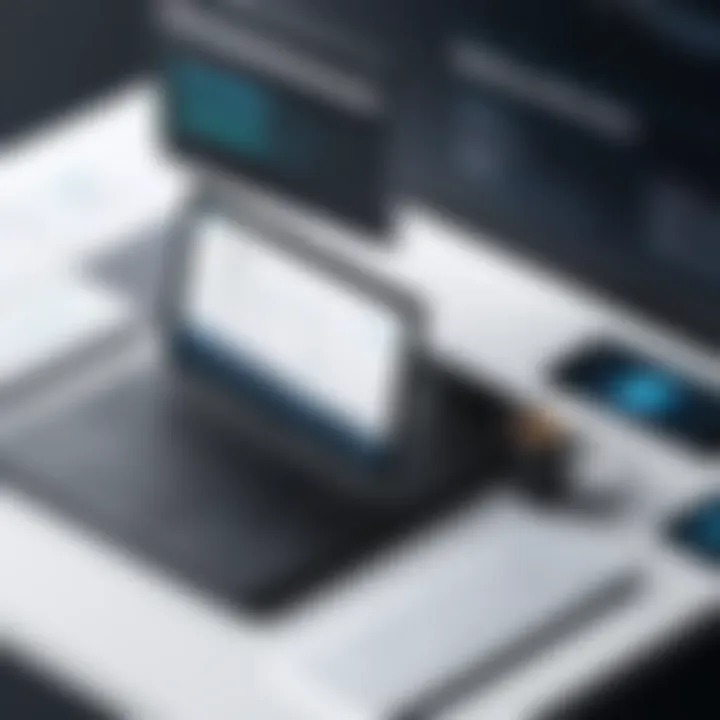
In this example, both and adapt the method as their own. This not only reflects the versatility of polymorphism but also demonstrates how it encourages a structured code approach. However, while this customization enriches functionality, it may introduce complexity if a method is overridden in many subclasses. It’s critical to balance this flexibility with maintainable code.
Benefits of Polymorphism
Understanding the benefits of polymorphism is pivotal for grasping its fundamental role in Java programming and object-oriented design. Polymorphism allows code to be more adaptable and streamlined, which is increasingly important as software systems grow in complexity. The advantages can be appreciated through various lenses: code reusability, flexibility and scalability, and ease of maintenance. Each of these elements plays a critical part in the journey from novice ideas to robust applications.
Code Reusability
One of the standout features of polymorphism is its ability to promote code reusability. Instead of writing new code from scratch, Java developers can extend existing class functionalities through method overriding or overloading. In practice, this means that many classes can share the same interface or base class while implementing their own unique behavior. For example:
- If we have a base class named , each animal type like or can override methods like , thus retaining the same method signature but providing distinct implementations.
This leads to less redundancy and more concise code, as similar functionalities are encapsulated in a single method. Employing polymorphism in your design can drastically reduce the lines of code, making programs less cluttered and easier to navigate. The use of polymorphism can be a game changer for large scale applications where consistency is crucial.
Flexibility and Scalability
The flexibility and scalability of an application can make or break its success, and polymorphism offers this promise. In practical terms, if a developer wants to add new features or modify existing ones, polymorphism allows for such enhancements without a complete rewrite of the application.
- With polymorphic code, you can introduce new classes that extend current functionalities or replace old ones with little effort. This flexibility allows for future growth without the hassle of backtracking.
- Suppose you have a method that accepts an type and processes its sound. If a new class like is added later, it can simply inherit the base class with minimal changes required in the existing method. This leads to a more adaptable codebase that can grow alongside user requirements.
Through polymorphism, systems become scalable, allowing developers to add new functionalities over time without endlessly repeating or altering existing code.
Ease of Maintenance
Finally, ease of maintenance is one of the overlooked advantages of polymorphism. In any software project, bugs and inconsistencies will arise. Here, polymorphism simplifies debugging because it centralizes related code and reduces interdependencies.
- If a particular method needs changing, it can be done at a single point of reference (the method in the base class), thereby affecting all derived classes accordingly. This not only streamlines the debugging process but also minimizes the risk of introducing new errors when changes are made.
- The idea of having multiple functions activated via one method also aids in isolated behavior testing. When base and derived classes are designed well using polymorphism, the maintenance becomes less burdensome, making life easier for a programmer over the long haul.
As you can see, benefiting from polymorphism touches different facets of software engineering, lifting the complexity off your shoulders. It's about working smarter, not just harder, in the dynamic world of programming.
Common Challenges with Polymorphism
Polymorphism, while invaluable in object-oriented programming, does bring its share of hurdles. Understanding these challenges helps developers leverage polymorphism effectively. This section will cover key obstacles that programmers, especially novices, might face when working with polymorphism. We'll discuss debugging complex hierarchies, potential performance issues, and the risks associated with overusing polymorphism. Each of these components plays a significant role in how polymorphism is implemented and understood.
Debugging Complex Hierarchies
Debugging is an inevitable aspect of programming. The complexity of hierarchies that polymorphism introduces can make this task even trickier. When multiple classes inherit from a single base class and override its methods, pinpointing the source of a bug can be like finding a needle in a haystack. Take this example:
In such cases, if the wrong method is executed, tracking down why it happened requires a deep dive into both the base and derived classes. A common debugging approach involves using logging or breakpoints to follow the execution flow, but the process remains cumbersome.
Key points to consider when navigating these complexities include:
- Test Hierarchies Thoroughly: Use unit tests to evaluate not just the method outputs but also interactions among classes.
- Utilize IDE Features: Most modern IDEs have debugging tools that allow stepping into methods to observe runtime behavior.
Potential Performance Issues
Another pitfall pertains to performance. When polymorphism is employed, especially in extensive applications, it can sometimes hinder performance due to the overhead of method calls. For instance, virtual method lookups might slow down the execution. It's similar to this analogy: if one person is fetching a drink for everyone at a party, there will be a long wait compared to each person just getting their own drink.
For instance, when a derived class has to frequently call overridden methods, the time taken can stack up. The Java Just-In-Time (JIT) compiler does optimize, yet the concern remains, particularly in scenarios demanding high-performance computing. Developers can keep performance in check by:
- Profiling the Application: Regularly track method call times and identify bottlenecks.
- Avoid Unnecessary Overriding: Sometimes it’s better to keep base methods simple and focused.
Overuse of Polymorphism
Lastly, overuse of polymorphism is a challenge that often creeps in. While polymorphism promises flexibility, it can lead to spaghetti code if not managed correctly. This happens when developers feel inclined to create every possible variation of behavior using polymorphism, leading to a myriad of subclasses and interfaces that can be difficult to manage.
Such overextension can obscure the original intent of code. It may feel tempting to use polymorphism for every single case, but it’s not always the best solution. Here are a few guiding principles to avoid crossing into an overuse trap:
- Stick to Clear Interfaces: Simplifying interfaces and keeping them concise makes it easier to understand underlying relationships.
- Limit Subclass Count: Don’t go creating subclasses arbitrarily. Ask yourself if the abstraction truly adds value.
Polymorphism in Interfaces and Abstract Classes
Polymorphism in programming languages, especially in Java, derives significant power from its interfaces and abstract classes. These constructs not only enrich the flexibility of the language but provide vital frameworks that facilitate the implementation of polymorphism. Here, we delve into their roles, comparative analysis, and best practices. Understanding these concepts is crucial for developers aiming to create scalable and maintainable code.
Role of Interfaces
Interfaces serve as contracts in Java, defining a set of methods that implementing classes must provide. They allow various classes—potentially from completely different class hierarchies—to adhere to the same method signatures. This is particularly useful when striving for flexibility within a system. Because multiple classes can implement the same interface, programmers can easily swap out implementations without the rest of the code finding out. This technique is quite handy when you're working in a collaborative environment where different teams develop different parts of the project.
Using interfaces can prevent the dreaded "spaghetti code", as developers can write code against interface types rather than specific implementations. This limits dependencies and provides a clearer path for modifications in the long run. Besides, interfaces can accommodate a myriad of functionalities while ensuring that core methods retain consistency.
Abstract Classes and Their Use
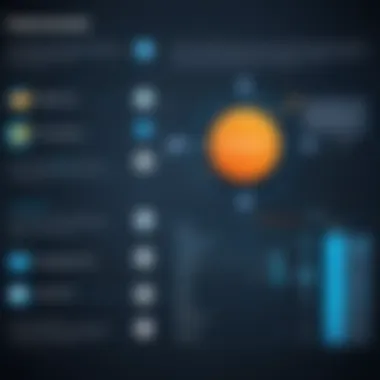
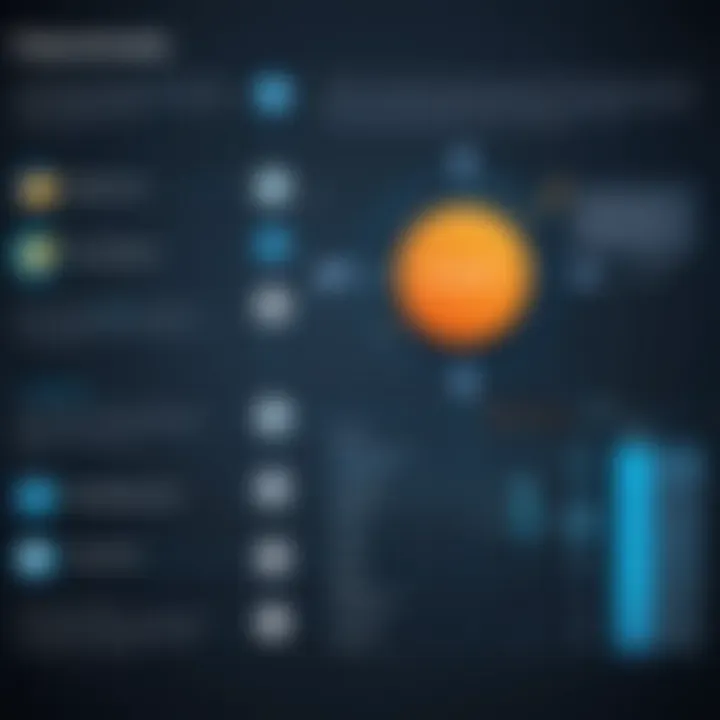
Abstract classes offer a middle ground between a full-fledged class and an interface. While abstract classes can define some behavior through concrete methods, they also allow for abstract methods—those without an implementation. When another class inherits from an abstract class, it inherits both the concrete methods and the promise to implement the abstract ones.
The key advantage of abstract classes is their ability to promote code reuse. For instance, if multiple subclasses share common behaviors, an abstract class can house these shared methods and fields, thereby abiding by the DRY (Don't Repeat Yourself) principle. Still, abstract classes differ from interfaces in one significant way: a class can only inherit from a single abstract class but can implement multiple interfaces. This characteristic leads to an interesting design dilemma where developers need to consider whether an abstract class or an interface serves their goals better.
Comparative Analysis
Interfaces vs Abstract Classes
When examining the comparison between interfaces and abstract classes, it’s evident that both serve vital purposes in the realm of polymorphism but come with distinct attributes.
A key characteristic of interfaces is their compositional nature. They allow disparate classes to collaborate effectively, paving the way for greater flexibility in design. Whether it’s a fish swimming or a car driving, interfaces can bundle unrelated behaviors into a unified whole.
On the other hand, abstract classes shine in providing a shared foundation. The uniqueness here is that they allow for partial implementation in a way that an interface can’t. Abstract classes act as a backbone for closely related classes, supporting default behaviors while ensuring that subclasses adhere to specific standards. So, if you’re building a framework where classes are tightly coupled, using abstract classes is commonly a more beneficial choice.
In short, if your requirement leans toward behavior specification and you foresee various classes potentially working together, interfaces could be your best bet. But if there’s common ground among the classes you've got in mind, abstract classes can save time and reduce redundancy.
Best Practices
In employing interfaces and abstract classes effectively, certain best practices stand out. First, favor interfaces when you require a collection of methods to be implemented independently of class hierarchies. This tends to lead to cleaner, more maintainable code, especially in larger systems.
On the flip side, use abstract classes when shared code or default method implementations are essential, making it simpler to keep everything connected. Establishing clear lines between when to use which can significantly contribute to a program’s architecture.
- Keep interfaces focused on behavior only.
- Ensure abstract classes encapsulate shared state wherever applicable.
- Avoid unnecessary complexity—don't mix responsibilities.
Ultimately, striking a solid balance between interfaces and abstract classes empowers programmers to utilize polymorphism to its fullest while ensuring that code remains elegant and manageable.
Understanding when and how to use interfaces versus abstract classes is fundamental for any Java developer aiming to handle real-world programming challenges effectively.
Real-World Applications of Polymorphism
Polymorphism is not just an abstract concept; it finds its roots in practical applications all around us, significantly impacting how we design and implement software. In the real world, polymorphism enhances system flexibility, allows for the dynamic invocation of methods, and promotes code maintainability. This is particularly beneficial in scenarios where developers deal with complex systems that require adaptability and robustness. Understanding where and how polymorphism is applied emphasizes its relevance and importance in modern programming practices, especially in Java.
Design Patterns Utilizing Polymorphism
Strategy Pattern
The Strategy Pattern is often heralded as a hallmark of designing algorithms independently from their context. By isolating these algorithms in different classes and defining a common interface, developers can substitute one strategy for another without modifying the client code. This characteristic not only promotes clean code organization but also directly reflects polymorphism at work. In cases where you have different models for sorting, for example, each sorting algorithm can be encapsulated in its strategy class, allowing the system to choose the appropriate one at runtime.
The standout feature of the Strategy Pattern lies in its ability to shift between behaviors dynamically, promoting greater adaptability. When you consider the multitude of sorting algorithms available, it becomes clear why this pattern is so favored: the flexibility it offers encapsulates multiple behaviors without complicating the overall code structure. However, developers should be cautious as it can lead to an explosion of classes if overused—ensuring balance in design complexity is key.
Factory Pattern
The Factory Pattern plays a vital role in Java application design by managing the creation of objects. Rather than instantiating objects directly, a factory method encapsulates the logic for object creation, allowing it to return objects of varying classes that share a common interface. This flexibility allows clients to work with an array of classes without needing to know the specifics of their implementation. For example, you might have a GraphicsFactory that generates different shapes (Circle, Square, etc.) based on parameters given.
The main advantage of the Factory Pattern is that it adheres to the Open/Closed Principle—code remains open for extension but closed for modification. A developer can add new concrete classes without altering existing code, which can significantly reduce the likelihood of introducing errors into a well-functioning system. The downside is, however, that it might add a level of indirection that some developers find unnecessary if their projects are straightforward.
Polymorphism in Frameworks
Java Collections Framework
Addressing polymorphism in the Java Collections Framework illuminates its designers' foresight. The framework is built upon interfaces, making it exceedingly versatile. Collections like List, Set, and Map are all interfaces that can be implemented by various classes. That means you can write code that is agnostic of the specific collection type being used, enhancing code reusability. When developers switch from one collection type to another—like moving from an ArrayList to a LinkedList—the changes are contained, emphasizing how polymorphism supports adaptability.
The capacity to treat different collections uniformly is a significant attribute. However, developers must be judicious in their choice of collection based on performance considerations. For instance, using a HashSet for frequent lookups is beneficial, though it might not be the best for situations where order is essential.
JavaFX
JavaFX showcases the power of polymorphism in building graphical user interfaces. With its component model, JavaFX allows developers to create complex applications with various UI controls like buttons, lists, and text fields. Each control can be treated as an instance of a common interface, allowing for seamless integration and swapping. This feature enables developers to enhance their applications without having to overhaul their foundational architecture, showcasing dynamic behavior that adds to usability.
One of the unique traits of JavaFX is its emphasis on the scene graph. This hierarchical representation of UI components allows for efficient rendering and event handling. Though its complexity can sometimes discourage beginners, understanding this hierarchy exemplifies how polymorphism can facilitate GUI construction. However, as applications grow in size, managing this scene graph can become cumbersome, requiring thoughtful design planning.
"Polymorphism enables software to grow and adapt, allowing developers to build flexible systems that respond to change efficiently."
Future of Polymorphism in Programming
The landscape of programming is always evolving. As new paradigms emerge, the role of concepts like polymorphism shifts and expands. In this section, we delve into the future of polymorphism in programming, examining its relevance in the advancing world of technology, particularly focusing on object-oriented programming and its interactions with functional programming.
Trends in Object-Oriented Programming
In the realm of object-oriented programming (OOP), polymorphism remains a pillar of design. As systems grow more complex, the necessity for flexible code that can adapt to changes without being rewritten is paramount. Current trends indicate that developers are increasingly leaning towards polymorphic patterns that support scalability and maintainability.
- Modular Design: The rise of modular programming encourages developers to use polymorphism effectively. This approach allows different modules to interact seamlessly through common interfaces, making the overall system more robust.
- Microservices Architecture: Within microservices, individual services often have varied implementations of the same functionality. Polymorphism becomes essential here, as it allows for a single interface to dictate multiple implementations depending on context.
- Increased Use of APIs: As APIs proliferate across applications, polymorphism will likely underpin the design patterns that facilitate interaction between disparate systems. This helps to standardize communication while allowing diverse implementations.
Overall, the focus on reusability and clear contracts between components ensures that polymorphism will remain crucial in OOP as we move forward.
Impact of Functional Programming
While object-oriented practices continue to thrive, functional programming has carved out its own significant space in software development. Its impact on polymorphism is notable, particularly in how it influences OOP. Here are some points of convergence between functional and object-oriented paradigms:
- Higher-Order Functions: Functional programming often employs functions that operate on other functions, akin to polymorphic behavior. This emphasizes flexibility and reusability, which can lend new insights into designing polymorphic systems in Java.
- Immutability and State Management: As functional programming promotes immutability, there’s an increasing trend toward using functional techniques alongside polymorphic designs. This change could minimize side-effects often related to polymorphism, making it safer and more predictable.
- Combining Approaches: Many developers are now using a hybrid approach. A system may leverage both object-oriented and functional aspects, encouraging flexible polymorphism through interfaces from OOP while benefiting from functional utilities, like map and reduce functions, to manage collections of objects more effectively.
"Flexibility in design is paramount—polymorphism ensures that the methods we write can evolve as our understanding of problems deepens."
In light of these advancements, students and budding programmers should stay abreast of the ongoing trends in both object-oriented and functional programming to truly harness the full potential of polymorphism.