Understanding PL/SQL: A Comprehensive Overview
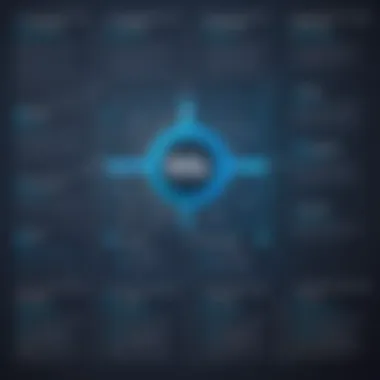
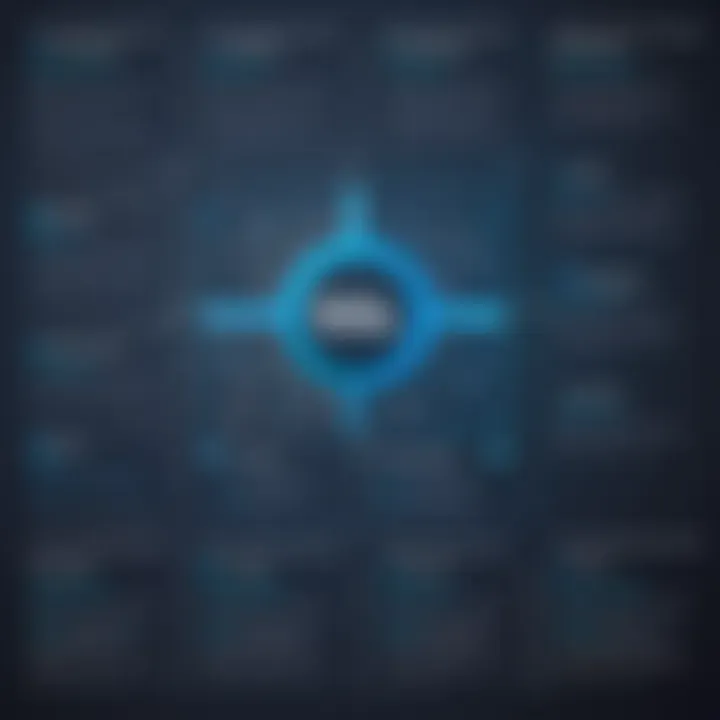
Intro
PL/SQL is essential in the world of database management. This procedural language extends SQL by adding features that support complex business logic and programming capabilities. It offers a means to integrate SQL with procedural constructs, resulting in effective data processing and management. Understanding PL/SQL can significantly benefit those engaged in database-driven applications.
History and Background
The journey of PL/SQL began in the late 1980s, developed by Oracle Corporation. It was designed to address the shortcomings of SQL and to provide a robust programming interface for database interactions. Over the years, it has evolved to keep pace with advancements in technology, becoming a mainstay in the Oracle ecosystem. PL/SQL empowers developers to create efficient, reliable applications with optimized queries.
Features and Uses
PL/SQL includes several key features that enhance its utility:
- Block Structure: Code is organized into blocks, which improves readability and maintainability.
- Exception Handling: This feature allows for graceful handling of errors, ensuring that applications run smoothly.
- Integration: It integrates seamlessly with SQL, enabling developers to write complex queries and manage large datasets easily.
Typical uses of PL/SQL include:
- Building applications that require intensive data manipulation.
- Creating stored procedures and functions to encapsulate business logic.
- Developing triggers that automatically respond to database events.
Popularity and Scope
The popularity of PL/SQL can be attributed to its efficiency and versatility. Many large enterprises utilize it for its ability to handle vast amounts of data effectively. The scope of PL/SQL continues to expand as it adapts to modern database needs, particularly in cloud services and analytics.
PL/SQL's combination of SQL with procedural programming creates a powerful tool for application development.
Basic Syntax and Concepts
Understanding the basic syntax of PL/SQL is crucial for new learners. Each code block starts with the keyword , is followed by an section where the main logic resides, and ends with an statement.
Variables and Data Types
PL/SQL supports various data types, including:
- NUMBER: For numerical values.
- VARCHAR2: For variable-length strings.
- DATE: For storing date and time.
Defining a variable requires specifying its type, like this:
Operators and Expressions
Operators in PL/SQL are similar to those in other programming languages:
- Arithmetic Operators: +, -, *, /
- Relational Operators: =, >, , >=, =
- Logical Operators: AND, OR, NOT
These operators are used to create expressions that can be evaluated to produce results.
Control Structures
Control structures dictate the flow of execution in a PL/SQL program. Common types include:
- IF Statements: Conditional execution based on boolean expressions.
- LOOPS: For executing a block of code multiple times.
- CASE Statements: For choosing between different execution paths based on conditions.
Advanced Topics
As one progresses in PL/SQL, understanding advanced topics becomes necessary.
Functions and Methods
PL/SQL allows the creation of user-defined functions and methods. These are reusable blocks of code designed to perform specific tasks and return values. Using functions can simplify code management and boost performance.
Object-Oriented Programming
PL/SQL also supports Object-Oriented Programming principles. It enables developers to define objects, use inheritance, and create encapsulated data structures. This feature enhances code organization and reuse.
Exception Handling
Proper error management is critical in any application. PL/SQL has robust mechanisms for exception handling, allowing developers to manage runtime errors effectively. Using the block, errors can be caught and handled without crashing the application.
Hands-On Examples
Practical examples play a significant role in learning. Starting with simple programs helps build a strong foundation.
Simple Programs
A basic PL/SQL block might look like this:
Intermediate Projects
As you become familiar, you can attempt to create stored procedures to manage data. For instance:
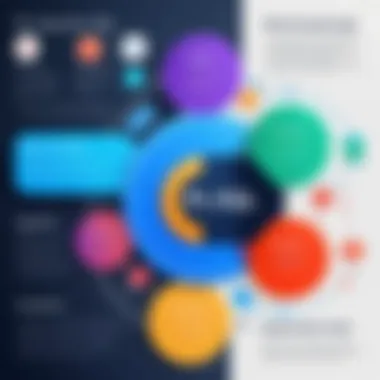
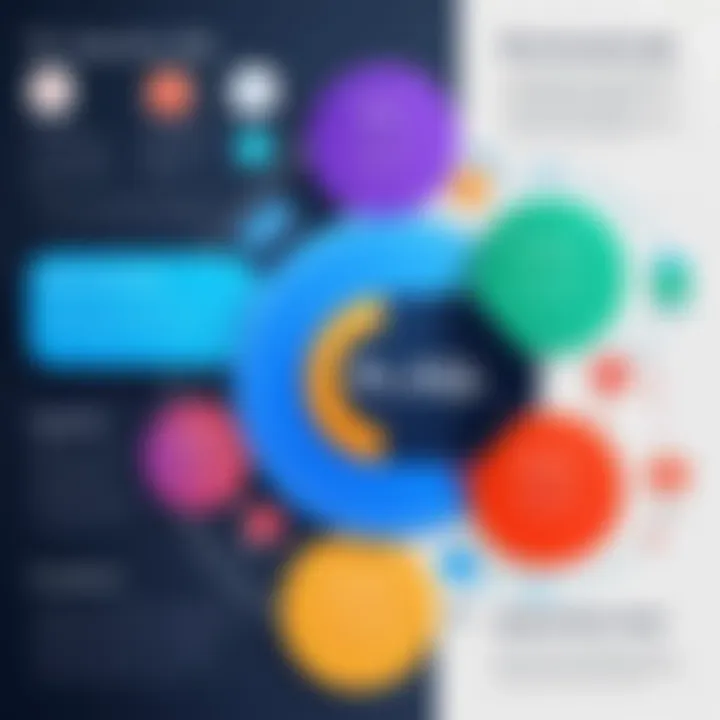
Code Snippets
Utilizing snippets accelerates learning. For instance, a loop that prints numbers:
Resources and Further Learning
To deepen your understanding of PL/SQL, consider these resources:
- Recommended Books and Tutorials: Look for books dedicated to PL/SQL programming, such as "Oracle PL/SQL Programming" by Steven Feuerstein.
- Online Courses and Platforms: Websites like Coursera, Udemy, and LinkedIn Learning offer comprehensive courses on PL/SQL.
- Community Forums and Groups: Engage with communities on platforms like Reddit and Facebook to share knowledge and get support from fellow learners.
In summary, mastering PL/SQL equips developers with the skills to create robust applications and handle data effectively in a database environment. As you explore further, remember to apply these concepts practically to solidify your understanding.
Preamble to PL/SQL
In the realm of database management, PL/SQL occupies a significant position. It is crucial as it combines the power of procedural programming with SQL. This combination allows developers to write complex and efficient database applications. PL/SQL enhances the capabilities of SQL, enabling various programming constructs that are essential for data manipulation and retrieval.
Since database systems are fundamental for storing, retrieving, and managing data, understanding PL/SQL is key. This language facilitates the use of control structures, modular programming, and error handling, which are vital for robust application development.
Moreover, organizations benefit from using PL/SQL due to increased performance and improved maintainability of database code. This has made PL/SQL an important tool for software developers working with Oracle databases.
Definition of PL/SQL
PL/SQL stands for "Procedural Language extensions to SQL". It is a block-structured language, designed specifically for Oracle databases. The language enhances SQL by providing additional procedural features, such as loops, conditions, and exceptions handling.
This enables developers to encapsulate SQL statements into procedures and functions, improving code modularity and reuse. The integration of PL/SQL with SQL allows for seamless execution of SQL queries within procedural code, leading to more efficient data handling and processing.
History and Evolution
PL/SQL was introduced by Oracle Corporation in the early 1990s. Initially, it was developed to provide an interface between SQL and Oracle's application development environments. The language evolved significantly over the years, keeping pace with the growing demand for robust database applications.
As technology advanced, so did PL/SQL. New features were added, enhancing its functionality and performance. In the latest iterations, features such as object-oriented programming support and improved optimization techniques have made PL/SQL a vital component of modern database applications.
PL/SQL continues to evolve alongside Oracle's database offerings, ensuring that it remains relevant in the ever-changing landscape of software development.
Core Features of PL/SQL
The section on core features of PL/SQL is crucial to comprehending its functionality and significance in a practical context. Understanding these features allows developers to leverage the full potential of PL/SQL in database management and application development. Each characteristic contributes to making PL/SQL a robust, efficient, and reliable tool for programmers.
Procedural and Declarative Nature
A notable aspect of PL/SQL is its procedural and declarative nature. While SQL is mainly declarative—focused on what data is to be retrieved or manipulated—PL/SQL extends this by allowing procedural programming. This means developers can write scripts that include variables, control structures, and logic statements to dictate how data operations are performed.
This feature includes:
- Control Structures: These are essential for directing the flow of program execution. They include the conditional statements like and loops like and . This versatility enables developers to form complex logical decisions and to handle different scenarios efficiently.
- Variable Declaration: Developers can introduce variables to store temporary data. This is essential for managing states or calculations without losing prior data.
- Sequential Execution: Unlike SQL, where commands execute separately, PL/SQL executes commands in a block, providing a cohesive processing experience.
The combination of these elements allows for more complex operations that are not possible with SQL alone. It leads to more dynamic applications.
Integration with SQL
Another vital feature of PL/SQL is its integration with SQL. Since PL/SQL is an extension of SQL, it can seamlessly utilize SQL commands within its programming. This makes it easier to perform database operations by embedding SQL statements directly in PL/SQL blocks.
Key benefits include:
- Direct SQL Access: Developers do not need to switch contexts between SQL and PL/SQL. This access simplifies coding practices and reduces the potential for errors.
- Bulk Data Operations: PL/SQL can process multiple records at once, improving performance significantly compared to processing rows one at a time with traditional SQL.
- Transaction Handling: PL/SQL supports transaction control statements such as COMMIT, ROLLBACK, and SAVEPOINT, providing developers with the tools to manage database states effectively.
Having this integration simplifies the work of the programmer while ensuring efficient data management, leading to faster application response times.
Support for Modular Programming
PL/SQL promotes support for modular programming, allowing developers to organize their code into manageable modules. These modules can be procedures, functions, or packages. This modularity offers several advantages:
- Reusability: Code blocks can be reused across different applications. This not only saves time but also minimizes redundancy.
- Maintenance: Modular code is easier to maintain. If a particular module has a flaw, it can be modified independently without affecting other parts of the program.
- Collaboration: Teams can work simultaneously on different modules, enhancing productivity and speeding up the development process.
Overall, the modular structure is foundational for building scalable applications. It allows teams to develop complex systems in manageable portions.
"The ability to combine procedural logic with declarative SQL makes PL/SQL particularly powerful in application development."
In summary, the core features of PL/SQL—its procedural and declarative blend, SQL integration, and modular programming support—are essential elements that define its capability as a language. These aspects make PL/SQL not just a functional extension but a critical language for any developer working within the Oracle ecosystem.
Advantages of Using PL/SQL
PL/SQL offers a range of advantages that enhance database management and application development. Its capacity to integrate with SQL while providing a procedural programming approach creates a robust environment for developers. Understanding these benefits is crucial for anyone looking to optimize their use of databases.
Improved Performance
One of the primary advantages of using PL/SQL is its improved performance in executing database operations. This is largely due to its capability to execute multiple SQL statements in a single execution. Instead of sending individual SQL statements to the database, developers can encapsulate the statements within PL/SQL blocks. This significantly reduces the number of context switches between the SQL engine and the PL/SQL engine, leading to more efficient resource utilization.
Moreover, PL/SQL supports bulk processing which allows users to process multiple rows at a time. For example, using the statement helps in applying the same SQL operation to a series of rows. The reduction in the number of database calls translates to faster execution times. This is particularly advantageous for applications that handle large datasets or perform complex operations.
Enhanced Security Features
Security is often a primary concern in database management. PL/SQL incorporates enhanced security features directly into its structure. Developers can employ procedures and functions to limit access to sensitive data while allowing controlled interaction with the database. This method of encapsulating business logic and data access ensures that only authorized users can manipulate critical components.
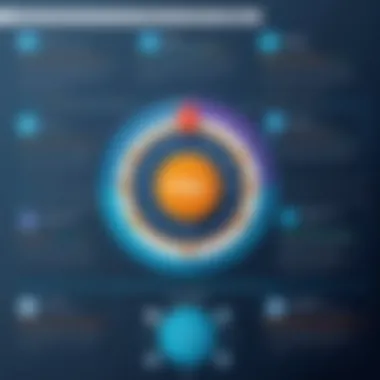
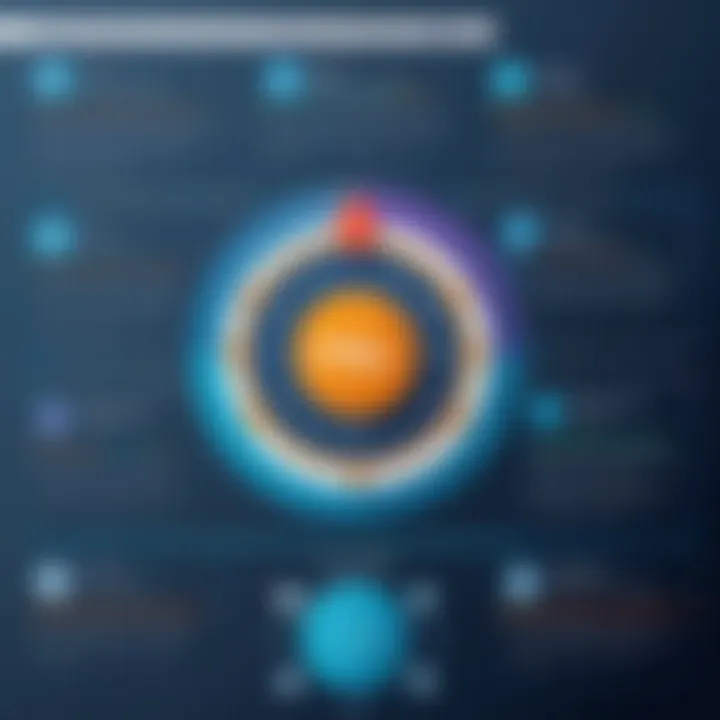
Additionally, PL/SQL provides functionalities such as binding variables that help in reducing the risk of SQL injection attacks. By utilizing bound variables, developers can ensure that user inputs are treated as data rather than executable code. This adds another layer of security and protects the integrity of the database.
Error Handling Mechanisms
Robust error handling is essential for any programming language, and PL/SQL excels in this area. The language has built-in exception handling features that facilitate the identification and management of runtime errors. Developers can define custom exception handlers to deal with specific errors effectively. This capability allows for more predictable application behavior and enhances overall reliability.
Using the block, developers can separate error handling code from the main logic, which enhances readability and maintainability. Additionally, PL/SQL allows users to raise exceptions explicitly, giving developers fine control over error management.
"Error handling in PL/SQL is not just about catching errors; it is about creating robust applications that can adjust to unexpected situations and continue functioning smoothly."
Components of PL/SQL
The concept of PL/SQL is underpinned by several key components. These include blocks, variables, control structures, and cursors. Each of these elements contributes significantly to the functionality and versatility of PL/SQL. Understanding how each component works is crucial for effective programming and enhances the overall performance and maintainability of PL/SQL code.
Blocks: Structure and Syntax
PL/SQL programs are organized into blocks. A block is a logical unit of code that groups together related declarations and operations. This structure is essential for defining the scope of variables and controlling the execution flow. Each block consists of three sections: the declaration section, where variables and constants are defined; the executable section, which contains the code to execute; and the exception handling section, where errors are addressed.
The syntax of a PL/SQL block is straightforward:
Using blocks effectively helps encapsulate code, improving readability and maintainability. Each block can be nested within another, allowing for complex program structures.
Variables and Constants
In PL/SQL, variables and constants are essential for storing data that can be manipulated during execution. Variables can change their values throughout the program, while constants are fixed and do not change once defined. This distinction is important for developers, as it affects memory usage and performance.
Variables are declared with a specific data type, such as INTEGER, VARCHAR2, or DATE. An example of variable declaration is:
Creating variables and constants thoughtfully ensures that the code is not only functional but also efficient. Correct use of data types plays a crucial role in maintaining database integrity, as well as in preventing runtime errors.
Control Structures
Control structures are vital for guiding the flow of execution in any PL/SQL program. They allow programmers to make decisions and execute code conditionally. The primary control structures include IF statements, CASE statements, and loop constructs.
For example, an IF statement can be structured as follows:
Loops, such as FOR and WHILE loops, enable repetition of code until a certain condition is met. Utilizing these control structures effectively enhances logic flow and decision-making within the program.
Cursors and Exception Handling
Cursors in PL/SQL act as pointers to the result set of a query. Cursors can be explicit or implicit. Explicit cursors are defined by the user, providing more control over the data fetched. Implicit cursors are managed automatically by PL/SQL when executing a SELECT statement without an explicit cursor declaration.
Using cursors allows for row-by-row processing of records, which is often required in applications that deal with complex datasets.
Exception handling is another critical aspect of PL/SQL programming. It ensures that programs can gracefully handle runtime errors. The ability to handle exceptions allows developers to log errors, notify users, and continue executing other parts of the program without crashing.
A simple example of exception handling is:
"Implementing proper exception handling and cursor management is key to developing robust PL/SQL applications."
Common Use Cases for PL/SQL
PL/SQL serves a vital role in various applications within database management. Understanding its common use cases is crucial for anyone looking to fully leverage the power of this language in their development projects. This section explores three primary use cases: database triggers, stored procedures and functions, and data validation and manipulation.
Database Triggers
Database triggers are a powerful feature of PL/SQL. They are special procedures that automatically execute in response to certain events on a database table or view. For instance, triggers can activate when a row is inserted, updated, or deleted. This feature is significant because it helps enforce business rules at the database level, ensuring data integrity without requiring changes to application code.
One common use of triggers is to maintain audit trails. When a significant action occurs, such as a data update, a trigger can log the change into an audit table, capturing who made the change and when it happened. This enhances security and accountability.
Here are some advantages of using database triggers:
- Automatic Execution: Triggers run automatically, reducing the need for manual intervention.
- Enhanced Data Integrity: They ensure that specific rules are followed consistently.
- Centralized Logic: Businesses can encapsulate logic at the database level, making it easier to manage.
Despite these benefits, developers must use triggers carefully. Overusing them can lead to performance issues or complicated interactions between triggers and the application logic.
Stored Procedures and Functions
Stored procedures and functions are another essential use case for PL/SQL. Both are essentially named blocks of PL/SQL code that can be executed later. The key difference lies in how they are called and how they handle parameters. A stored procedure performs actions but does not return a value, while a function computes a value and must return it.
These constructs are valuable for several reasons:
- Reusability: Once written, stored procedures and functions can be reused across multiple applications or queries, promoting consistency.
- Performance: They can execute faster, as the database processes the code once and stores the execution plan.
- Improved Security: By limiting direct access to database tables, developers can provide controlled access through procedures or functions.
Example:
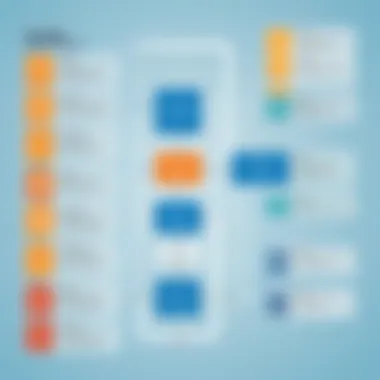
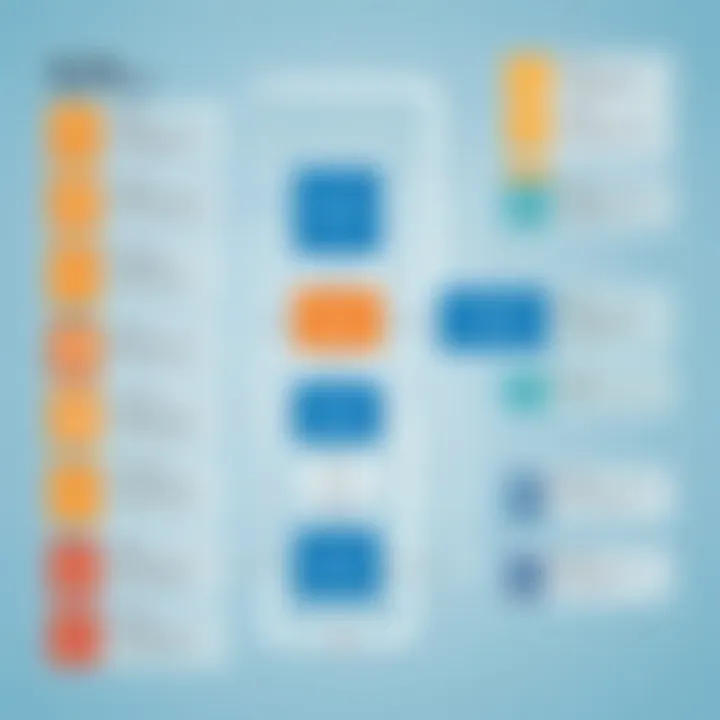
This simple example demonstrates how a stored procedure helps implement a salary update without exposing the underlying table directly to users.
Data Validation and Manipulation
Data validation and manipulation are critical tasks in any database application. PL/SQL provides robust features for ensuring that the data entering the database meets specific criteria. This is done through checks in procedures or triggers that validate incoming data against business rules before committing it to the database.
For instance, developers can write procedures that validate data formats, ensuring that email addresses are correctly formed or that date entries fall within a specified range. This kind of validation can prevent incorrect data from ever entering the system, which is often more efficient than correcting errors after the fact.
In addition to validation, PL/SQL enables complex data manipulation tasks. With built-in functions, developers can transform data as needed before insertion or updating processes.
To sum up, the common use cases for PL/SQL not only demonstrate its importance but also highlight how it enhances database operations. By implementing database triggers, stored procedures, functions, and ensuring data validation, developers can create robust database solutions that maintain data integrity and improve overall efficiency.
Best Practices in PL/SQL Programming
Best practices in PL/SQL programming are essential for maintaining high-quality code that is both efficient and easy to manage. By adhering to these practices, developers can produce programs that not only function correctly, but also perform well in production environments. This section will outline critical aspects of PL/SQL code, focusing on techniques that optimize performance, mitigate errors, and ensure maintainability.
Code Optimization Techniques
Optimizing PL/SQL code is crucial for improving execution speed and resource usage. Here are several key optimization techniques:
- Use Bulk Operations: Instead of processing rows one at a time, utilizing bulk operations, like and , can drastically reduce context switches between SQL and PL/SQL. This can lead to significant performance gains.
- Minimize Context Switching: Every time PL/SQL calls SQL, it incurs a context switch. Minimizing the frequency of these switches by grouping SQL statements within a single block can enhance performance.
- Avoid Unnecessary Computations: Conduct calculations only when required. Store results in variables when they will be reused within the block to avoid redundant calculations.
- Use Proper Indexing: Ensure that the database tables used in your queries are indexed appropriately. Proper indexing allows for more efficient data retrieval.
By applying these techniques, developers can create efficient PL/SQL code that responds better to business needs.
Effective Error Handling
Error handling in PL/SQL is vital to the reliability of applications. An effective approach allows developers to anticipate issues and react accordingly, ensuring that operations can proceed smoothly. Here are important strategies for handling errors in PL/SQL:
- Use EXCEPTION Blocks: By using handling, developers can catch runtime errors and define specific actions for different error types. This helps to keep the application running and provides valuable feedback for troubleshooting.
- Log Errors: Keeping a record of errors encountered can aid in diagnosing issues. Consider writing error logs to a dedicated error table or a log file.
- Graceful Failures: Design the program to fail gracefully. This can involve rolling back transactions or notifying users without exposing sensitive error details.
- Reraise Exceptions When Needed: After handling a specific error, it may be appropriate to reraise the exception to allow higher-level routines to react appropriately. Be mindful of what data needs to be preserved during this process.
Creating a solid error handling strategy not only enhances the robustness of the program but also improves the user experience.
Documentation and Code Maintenance
Good documentation and code maintenance practices are fundamental aspects of PL/SQL programming. They make codebases easier to read and maintain over time. Here are practices to keep in mind:
- Commenting: Regularly comment on your code to explain complex logic or assumptions. This is necessary for anyone who might maintain the code in the future, including yourself.
- Consistent Naming Conventions: Establish and adhere to a set naming convention for variables, functions, and procedures. This helps others understand the purpose of each component at a glance.
- Version Control: Utilize version control systems to track changes in code. This allows for easy rollback to earlier versions as needed and facilitates collaboration among multiple developers.
- Regular Refactoring: Periodically review your code and refactor to improve clarity and performance. Removing unused code or optimizing sections that have become less efficient over time is beneficial.
Good documentation and maintenance not only simplify the debugging process but also promote team collaboration and knowledge transfer.
"Good code is its own best documentation."
By following these best practices, PL/SQL developers can enhance code efficiency, reliability, and maintainability, ultimately resulting in better applications.
PL/SQL in the Oracle Ecosystem
PL/SQL holds a pivotal role within the Oracle ecosystem, providing a robust environment for extracting maximum value from Oracle databases. Its procedural nature enhances SQL's capabilities, making it indispensable for developers and database administrators. Understanding PL/SQL's significance starts with recognizing how it seamlessly integrates with various Oracle tools and applications, enhancing both functionality and efficiency in data handling.
Integration with Oracle Databases
PL/SQL’s integration with Oracle databases is foundational to its functionality. The language was designed to work closely with Oracle's SQL, allowing for complex operations within a singular environment. This tight integration means that developers can execute multiple SQL statements as a single block, which leads to better performance and reduced network traffic. The language's ability to handle large data volumes and execute business logic on the database server puts less strain on client applications.
Key Benefits of PL/SQL Integration:
- Performance: Batch processing reduces the number of context switches between the SQL and PL/SQL engines, boosting execution speed.
- Network Efficiency: Less data movement between the client and server enhances application responsiveness.
- Security: Business logic executed on the server side minimizes exposure to SQL injection attacks compared to client-side processing.
This integration fosters the need for thorough understanding. PL/SQL provides access to Oracle features like triggers and stored procedures, allowing developers to automate tasks and implement complex business rules directly in the database.
Role of PL/SQL in Oracle Applications
Within Oracle applications, PL/SQL serves as the backbone for many functionalities. It is widely used in ERP systems, CRM platforms, and various custom applications that require robust data handling capabilities. PL/SQL streamlines process automation, system integration, and enhances user experience by enabling efficient data retrieval, manipulation, and security implementations.
"PL/SQL is not just a programming language; it is a crucial part of Oracle's software ecosystem that facilitates higher levels of efficiency and interactivity."
Common Roles of PL/SQL in Oracle Applications:
- Business Logic Implementation: Ensures that data handling aligns with business rules and processes.
- Data Validation: Guarantees that data integrity is maintained through checks and constraints embedded within PL/SQL code.
- Performance Enhancements: Supports optimization techniques that improve overall application performance by executing complex queries closer to the data.
Learning PL/SQL is essential for those working within the Oracle environment, as it unlocks the full potential of its applications. Whether it is for developing new applications or maintaining existing ones, a thorough grasp of PL/SQL is vital for success in leveraging Oracle technologies.
Ending and Future of PL/SQL
The conclusion of this article emphasizes the vital role PL/SQL plays in the realm of database management and application development. By examining its core principles, advantages, and use cases, we gain an understanding of how this programming language not only enhances efficiency but also reinforces security. PL/SQL stands out for its procedural capabilities that seamlessly integrate with SQL, making it a preferred choice for developers working in Oracle environments. This section aims to tie together key themes and provide insights into what lies ahead for PL/SQL.
Summary of Key Takeaways
To summarize, here are the critical points presented in this article:
- Robust Integration: PL/SQL's integration with SQL allows for powerful database transactions and data manipulation.
- Efficiency: Through procedural programming features, PL/SQL enhances performance, offering bulk processing that minimizes database round-trips.
- Error Handling: Integrated error handling mechanisms contribute to robust software solutions, reducing potential downtimes.
- Security Models: PL/SQL includes strong security features like encapsulation, which help protect sensitive data.
- Support for Modular Programming: The ability to create procedures, functions, and packages encourages code reusability and organization.
- Versatile Use Cases: From database triggers to complex data validations, PL/SQL serves various purposes in real-world applications.
These takeaways encapsulate the essence of PL/SQL and its significance in the software development lifecycle.
Emerging Trends in PL/SQL
Emerging trends in PL/SQL reflect the evolving landscape of database management. As organizations continue to demand efficient data processing methods, the future of PL/SQL looks promising. Some noteworthy trends include:
- Cloud Integration: With the increasing adoption of cloud technologies, PL/SQL will likely evolve to support cloud-based databases like Oracle Cloud.
- Compatibility with Big Data: As businesses leverage big data technologies, the integration of PL/SQL with platforms like Hadoop is becoming crucial.
- Optimized Performance: Continuous improvements in PL/SQL are focusing on optimizing execution times and resource management.
- AI and Automation: Utilizing artificial intelligence to streamline query processing and automate routine tasks will be a significant trend.
These developments suggest a forward-thinking approach, ensuring PL/SQL remains relevant in a rapidly changing technological environment. As such, developers and students working with PL/SQL must stay informed about these trends to leverage the language effectively.