Node.js Databases: Architecture and Best Practices
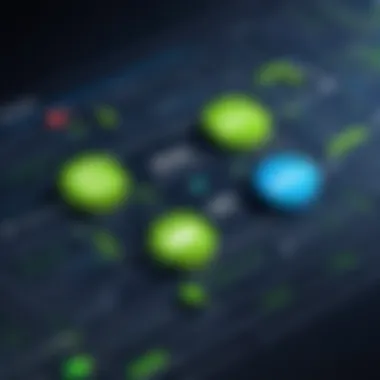
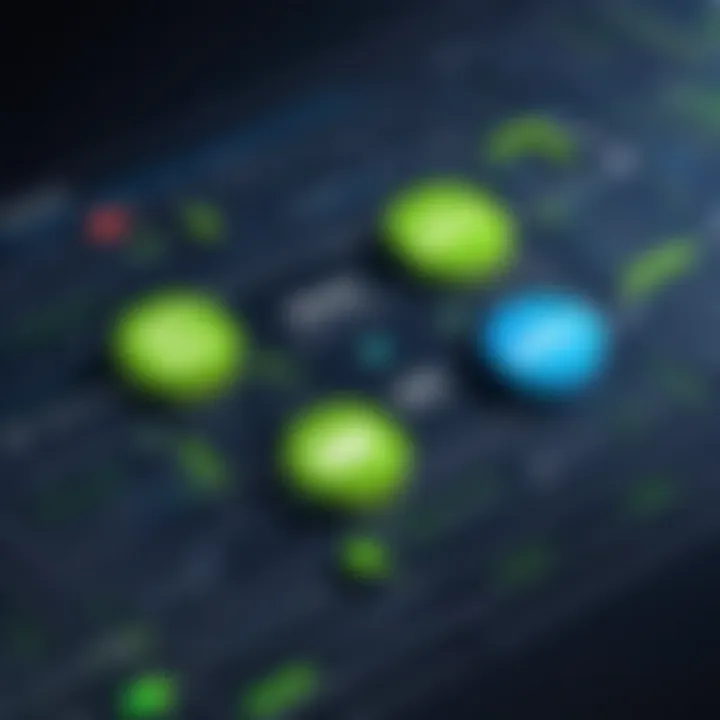
Intro
Node.js has emerged as a powerful platform for server-side development, known for its non-blocking architecture and event-driven design. A key aspect of its robustness lies in how it integrates with various databases. This article aims to clarify that integration, exploring architectural choices, database options, and optimal practices. Understanding these elements is crucial for developers interested in enhancing their backend solutions with Node.js.
Importance of Databases in Node.js
When building applications, data management becomes central to their functionality. The choice of a database can heavily influence performance and scalability. Node.js developers must ensure that the selected database complements Node.js's capabilities while also meeting the application's specific requirements.
In this regard, we'll examine how different types of databases, including SQL and NoSQL options, can be leveraged effectively within Node.js environments.
"Choosing the right database can significantly impact your application’s performance and maintainability."
Overview of Topics
This article will traverse various facets of Node.js databases:
- Architectural considerations for optimal database integration.
- A comparison of popular database solutions suited for Node.js, including MongoDB, PostgreSQL, and Redis.
- Practical implementation strategies and best practices that ardent users can apply.
By the end of this discussion, readers should have a nuanced perspective on how to select and implement databases in their Node.js projects. This understanding facilitates not just enhanced performance but also a more seamless data management experience.
Foreword to Node.js and Database Integration
In the ever-evolving landscape of web application development, understanding the integration of Node.js with various database systems stands as a foundational skill. Node.js, with its non-blocking, event-driven architecture, presents unique opportunities and challenges when connecting to databases. This section sets the stage to explore these dynamics alongside the critical role databasess play in applications today.
Overview of Node.js
Node.js is a runtime environment that allows developers to execute Javascript on the server side. Its asynchronous nature is particularly suitable for building scalable network applications. It utilizes a single-threaded model, which can handle many connections simultaneously, making it a preferred choice for modern application development. The lightweight structure enables developers to create fast and efficient web services.
Node.js comes equipped with a rich ecosystem of libraries and frameworks, simplifying the development process. This capacity to integrate with various databases enhances its functionality, enabling seamless operation across multiple types of applications. The synergy between Node.js and databases is vital for developers seeking optimized solutions for data handling.
The Importance of Databases in Applications
Databases are the backbone of most web applications, acting as repositories for data storage, retrieval, and management. The significance of databases transcends mere storage; they facilitate data integrity, consistency, and security. In an era where data-driven decisions underpin business success, the relationship between Node.js and databases cannot be overstated.
Moreover, an effective database management system underpins user experience and application performance. As applications scale, the demand for efficient data access and manipulation increases. The choice of database and proper integration with Node.js can influence application responsiveness and resilience. Developers must, therefore, understand the implications of their database choices and how they interact with Node.js.
"A well-integrated database can accelerate application development and ensure data consistency, impacting user satisfaction and operational efficiency."
In summary, gaining insights into Node.js and database integration is essential for developers at any stage of their careers. By navigating these initial considerations, one can appreciate the broader context of this article, which aims to provide a detailed examination of various database options and best practices.
Types of Databases Compatible with Node.js
Understanding the types of databases compatible with Node.js is crucial for developers looking to build efficient applications. Each database type offers unique features and benefits that can significantly influence the application's performance, scalability, and ease of use. When considering database options, developers must evaluate factors such as data structure, query complexity, and consistency requirements. This section delves into the primary types of databases that can seamlessly integrate with Node.js, enabling developers to make informed decisions.
Relational Databases
Relational databases, like PostgreSQL and MySQL, are structured around tables with rows and columns. This format allows for clear relationships between distinct data points. For Node.js applications, relational databases provide strong consistency and a well-defined schema. This is crucial when working with transactions or when data integrity is paramount. Node.js interacts with these databases through various drivers and Object-Relational Mapping (ORM) tools, such as Sequelize or TypeORM. These tools allow developers to work with database entries as JavaScript objects, simplifying CRUD operations.
The use of SQL queries in relational databases can be a strong advantage, as they support complex join operations and allow for detailed data analysis. However, working with relational databases also requires careful planning of the database schema, which can be a drawback if the application demands rapid iteration.
NoSQL Databases
NoSQL databases, such as MongoDB and CouchDB, differ fundamentally from relational databases. They do not require a predefined schema, which grants developers flexibility in their data models. This is particularly useful for applications that handle unstructured data or require rapid scalability. In Node.js, the use of NoSQL databases allows for efficient handling of JSON-like data structures, which are native to JavaScript.
Beyond flexibility, NoSQL databases can handle large volumes of data across distributed systems. This makes them suitable for applications like real-time analytics and social media platforms. However, developers must be cautious, as NoSQL databases often trade off ACID (Atomicity, Consistency, Isolation, Durability) properties in favor of performance and scalability.
In-memory Databases
In-memory databases, such as Redis, store data directly in the system's memory rather than on disk. This leads to exceptionally fast data access speeds, making them ideal for high-performance applications. For example, caching frequently accessed data reduces load time and enhances user experience significantly. Node.js can easily integrate with in-memory databases, using libraries like which allows the management of data in real time.
Despite their performance advantages, in-memory databases may not be suitable for all data types, particularly if persistent storage is necessary. Additionally, since data is stored in RAM, it can be lost on system failure unless backups are properly configured. Consequently, they are often used alongside other database types to optimize overall performance while managing data durability.
Popular Databases Used with Node.js
In the realm of Node.js development, the selection of a proper database is crucial. The choice of database affects the performance, scalability, and data integrity of applications. With a multitude of database options available, it's essential for developers to understand each one's characteristics, benefits, and integration nuances with Node.js.
MongoDB
MongoDB is a widely-used NoSQL database known for its flexibility and scalability. It stores data in JSON-like format, which aligns well with JavaScript's objects. This synergy facilitates a seamless data interchange between the Node.js application and the database. MongoDB's document-based model allows developers to reveal complex data structures without predefined schemas. It is particularly advantageous in environments where application needs evolve rapidly.
Some benefits of using MongoDB include:
- High availability through built-in replication and automatic sharding.
- Scalability requires horizontal expansion, allowing developers to add additional servers as data needs increase.
- Ease of use, especially for JavaScript developers, reduces the learning curve significantly.
PostgreSQL
PostgreSQL is an advanced relational database management system. It emphasizes extensibility and SQL compliance. PostgreSQL supports complex queries, transactions, and offers powerful data integrity features. Developers sometimes prefer it for applications requiring complex data interactions and transactions.
Advantages of PostgreSQL include:
- Strong data integrity through ACID compliance.
- Support for advanced queries and indexing options, leading to better performance.
- Extensibility, allowing users to create custom data types and functions.
This makes PostgreSQL an excellent choice for applications where data relationships are paramount. However, it may involve a steeper learning curve compared to NoSQL options.
MySQL
MySQL is another popular relational database choice within the Node.js ecosystem. It has been in the market for many years and has earned a reputation for reliability and stability. MySQL is widely used in various web applications and has extensive community support.
Key features of MySQL include:
- Efficient performance, particularly for read-heavy operations.
- Widespread adoption, which ensures that many hosting services offer MySQL support.
- Rich tools and support, which includes various client libraries for Node.js integration.
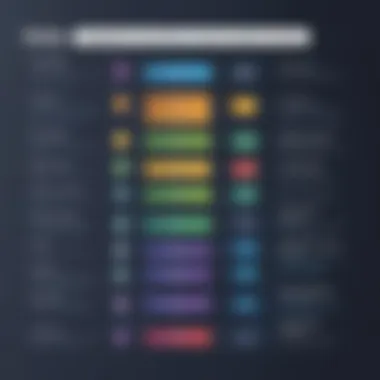
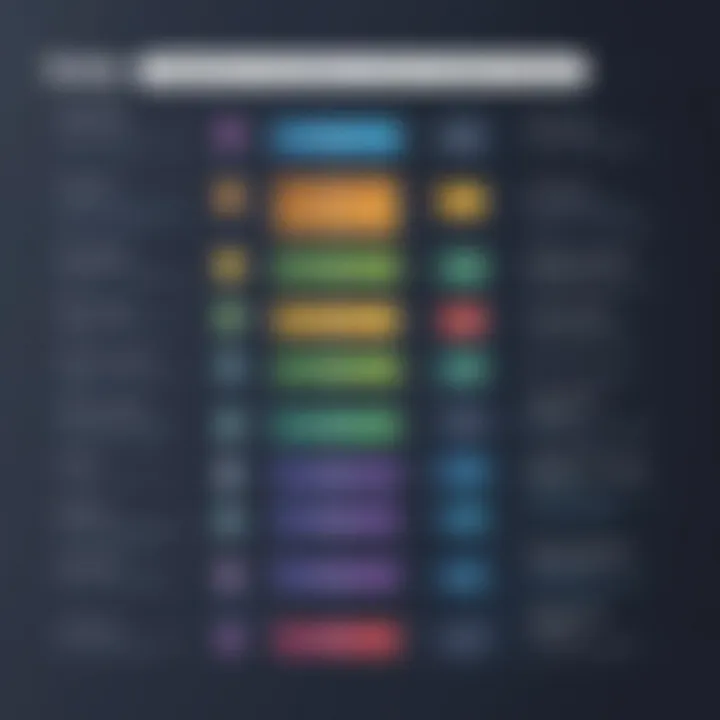
This entrenched position and support can ease deployment and maintenance in many commercial environments.
Redis
Redis is a versatile in-memory data store. Although it is primarily known for caching, it can also be used as a database, message broker, and task queue. Redis provides fast access to data due to its in-memory storage mechanism, making it suitable for applications demanding high performance.
Benefits of Redis include:
- Ultra-fast performance, enabling applications to handle a large number of transactions per second.
- Rich data types like lists, sets, and hashes enhance its usability.
- Pub/Sub capabilities enable message broadcasting among multiple clients, useful in real-time applications.
In summary, the choice of database can heavily influence the architecture and performance of Node.js applications. Understanding these popular databases can guide developers in making informed decisions that best suit their project requirements.
Connecting Node.js to Databases
The integration of Node.js with databases plays a crucial role in developing robust applications. Node.js operates on a non-blocking, event-driven architecture, making it an ideal choice for database connections. The ability to interact with databases efficiently determines the performance and scalability of the application. This section will look at important elements of connecting Node.js to databases, the benefits, and various considerations to keep in mind.
Database Drivers and ORMs
Database drivers are essential for establishing communication between Node.js applications and databases. Each database type has its specific driver that allows Node.js to send and receive data effectively. For instance, is commonly used for PostgreSQL, while is popular for working with MongoDB. These drivers manage queries and handle connection pooling, which enhances performance.
Object-Relational Mappers (ORMs) provide a higher level of abstraction. They simplify database interactions by allowing developers to work with JavaScript objects instead of raw SQL queries. is a well-known ORM for Node.js, supporting various databases, including MySQL, PostgreSQL, and SQLite. Using an ORM can speed up development time and improve code readability, making it easier to manage database resources. However, it is important to consider that using ORMs may add a slight overhead, which can affect performance in some cases.
Configuration and Setup
Setting up a database connection in Node.js requires several steps. Developers need to install the appropriate driver or ORM and configure it according to the application's requirements. Typically, this involves specifying connection details such as the database host, port, username, and password. Here’s a basic example of how to configure a connection using for PostgreSQL:
This snippet illustrates how to establish a connection and handle potential errors. Configuration might also include setting options for connection pooling, which is essential for managing multiple requests efficiently without overwhelming the database.
Architectural Patterns for Database Integration
Architectural patterns form the backbone of how applications are designed to interact with databases. For developers working with Node.js, utilizing the correct architectural pattern is crucial for creating scalable, maintainable, and efficient applications. These patterns allow for a clear separation of concerns, making it easier to manage code, enhance testing processes, and improve collaboration among team members. The adoption of suitable architectural patterns can lead to better application performance and easier updates when business requirements change.
When integrating databases with Node.js, two primary architectural patterns emerge: Model-View-Controller (MVC) and Microservices Architecture. Each has its advantages and considerations. Understanding these patterns helps developers choose the right structure for their applications based on specific needs and existing constraints.
Model-View-Controller ()
The Model-View-Controller (MVC) pattern delineates the application into three interconnected components: the model, the view, and the controller. This separation facilitates a more organized approach to handling data and user interactions. The model represents the data and the logic behind it. The view is responsible for displaying the information to users, while the controller acts as a mediator between the model and the view, updating both when necessary.
Benefits of
- Separation of Concerns: This pattern allows developers to work on different components independently, enhancing collaboration.
- Reusability: With clear boundaries, components can be reused across different parts of the application or even in different projects.
- Maintainability: Changes to one part of the application, such as transitioning to a new database system, can be done with minimal impact on the other components.
Considerations for
When implementing the MVC pattern in a Node.js environment, the following points should be considered:
- Framework choice: Some popular Node.js frameworks that support MVC include Express.js and Sails.js. Choosing the right framework can streamline development.
- Database integration: Ensure the models have robust schema definitions and data validation to prevent inconsistencies.
Microservices Architecture
Microservices architecture takes a different approach by breaking down applications into smaller, independent services. Each service focuses on a specific functionality and communicates with others over APIs. This modular design is beneficial for scalability and allows teams to work on different services simultaneously.
Benefits of Microservices
- Scalability: Each microservice can be scaled independently based on its requirements, leading to more efficient resource management.
- Flexibility in Technology Stacks: Different services can employ various databases or programming languages, allowing teams to choose the best tools for each task.
- Resilience: If a service fails, it doesn’t necessarily bring down the entire application, enhancing overall system reliability.
Considerations for Microservices
While the microservices architecture is powerful, it poses challenges:
- Service discovery and orchestration become essential to manage interactions between services effectively.
- Database management can become complex. Each service may require a different data storage solution, and considerations about data consistency and integrity must be addressed.
In summary, both the Model-View-Controller and Microservices Architecture provide insightful frameworks for integrating databases with Node.js. The choice of pattern will largely depend on the application's specific requirements, existing team structures, and future growth potential. Adopting these architectural patterns can lead to more efficient development cycles, improved user experiences, and robust application performance.
"Choosing the right architectural pattern is fundamental for a successful application. It determines how well components can interact and evolve over time."
Understanding these patterns equips developers with the knowledge to make informed decisions that align with both current and future project needs.
Data Access Patterns in Node.js
Data access patterns play a crucial role in how applications manage data with databases in Node.js. These patterns provide a structured approach, ensuring that developers can maintain, scale, and integrate their data access methods effectively. Understanding these patterns helps streamline the development process and enhances the overall performance and reliability of applications.
Utilizing appropriate data access patterns can lead to numerous benefits, such as:
- Separation of Concerns: Developers can encapsulate data access logic away from the core business logic. This makes it easier to manage changes in one part of the system without affecting others.
- Better Testability: Patterns like the repository can simplify unit testing since they allow for easy mocking of data access layers.
- Easier Maintenance: A well-defined pattern can reduce the complexity of data access code, making it easier for teams to maintain and improve over time.
When choosing a data access pattern in Node.js, it’s essential to consider the specific needs of the application and the complexity of data interactions. Embracing these patterns equips developers not only with a solid foundation for their work but also makes future enhancements more manageable and less error-prone.
Repository Pattern
The repository pattern abstracts the data layer, providing a simplified interface to communicate with the database. By doing this, it allows developers to work with data as if they were dealing with a collection of objects rather than raw database queries. This pattern is particularly effective in applications that require complex business logic or multiple data sources.
Benefits of the Repository Pattern include:
- Encapsulation: All data access logic is centralized, which helps improve code organization.
- Flexibility: This pattern provides the option to switch the underlying data source without changing the business logic. For instance, switching from MongoDB to PostgreSQL can be done with minimal changes to the overall application.
- Consistency: It promotes a consistent interface for data access, ensuring that data is retrieved and manipulated in predictable ways.
Implementing the repository pattern can be done easily in Node.js. Here’s a simple example:
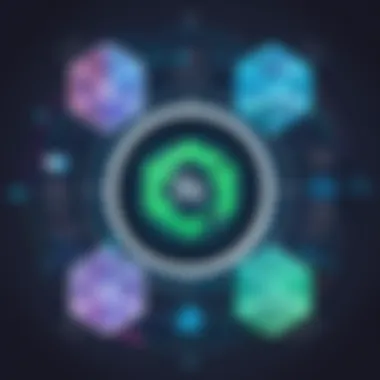
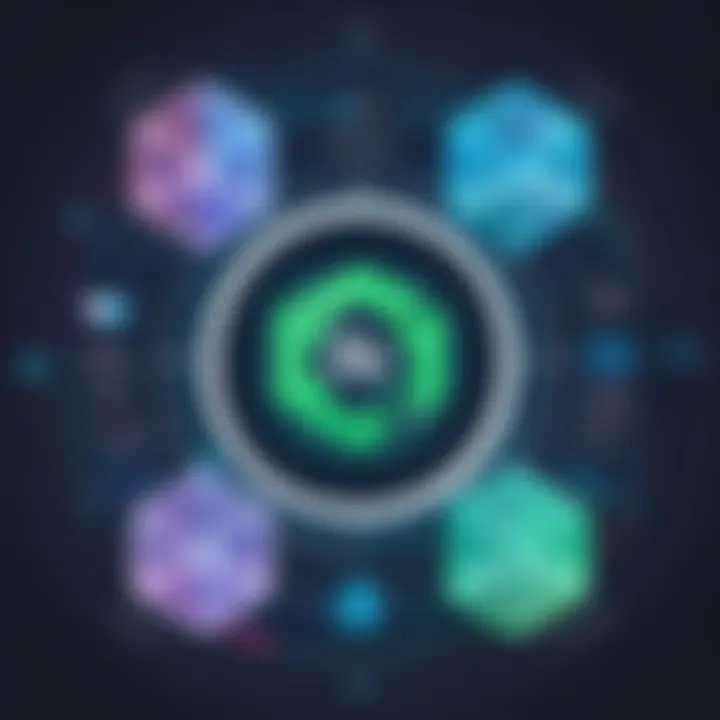
In this example, the interacts with a database but keeps the data access logic away from the rest of the application. This allows for easy testing and clear separation of responsibilities.
Data Mapper Pattern
The data mapper pattern serves as a bridge between the database and the application’s domain objects. Rather than having entities take responsibility for their persistence, the data mapper transfers data between objects and the database while keeping them independent. This results in a clean separation between the application logic and the data storage.
The primary advantages of the Data Mapper Pattern are:
- Decoupling: Domain objects don’t need to worry about how data is stored or retrieved. This results in a cleaner design and better adherence to the Single Responsibility Principle.
- Reduced Code Duplication: Since all data interactions are managed by the data mapper, it reduces redundancy in the codebase.
- Easier Testing: Testing becomes more straightforward because the data mapper can be mocked or stubbed in isolation to test the application logic.
Here is a brief illustrative example:
In this code snippet, the takes care of mapping the raw data from the database into a object. This allows developers to focus on using the domain model rather than worrying about how it interacts with the database directly.
Both the repository and data mapper patterns help shape the data access strategy in Node.js. Utilizing either approach can significantly enhance the clarity, maintainability, and testability of applications.
Error Handling and Debugging
In any software development process, error handling and debugging are key elements that contribute to application stability. This holds true for applications built with Node.js that interact with various databases. Error handling ensures that the application can respond to unexpected situations without crashing. Debugging, on the other hand, is about identifying the source of an error to fix it effectively. For developers, these practices are essential for creating reliable applications and a good user experience.
Focusing on these concepts enables developers to grasp important design patterns and workflow changes necessary to handle potential issues more gracefully. Recognizing errors early, instead of during production, can save considerable time and resources, allowing for smoother deployments and fewer problems in live environments.
Moreover, a thorough understanding of error handling techniques empowers developers to build robust applications that can manage and respond to database-related errors, facilitating an overall higher level of application security and performance. Errors can originate from various sources such as database connection failures, incorrect queries, or permission issues. Thus, being prepared to address these matters is a priority.
Common Error Types
When dealing with databases in a Node.js application, several common error types can arise. Such errors may include:
- Connection Errors: Issues with establishing or maintaining a connection to the database. These can occur due to network failures or incorrect connection details.
- Query Errors: Mistakes in SQL syntax or invalid parameters can lead to failed queries. Understanding how to log and handle these effectively is critical.
- Timeouts: When a query or connection takes too long, it can generate timeout errors. Handling these involves setting appropriate timeout durations and managing retries.
- Data Validation Errors: When input does not meet predefined criteria or constraints in the database, errors arise. Proper data validation on the server side can help capture these early.
- Authentication Errors: Incorrect credentials or insufficient permissions can prevent access to the database, resulting in errors.
It is crucial for developers to categorize and log these types of errors, as they will influence how the application behaves and how user feedback is managed. Prioritizing error handling not only leads to improved stability but also aids in maintaining user trust in the application.
Tools for Debugging
Debugging is a critical part of the development process for any application, including those using Node.js with databases. Several tools and techniques are available to assist developers in tracing and fixing issues. Some of these include:
- Node.js Built-in Debugger: Using the built-in debugger with Node.js allows developers to set breakpoints and step through code to investigate errors in detail.
- Console Logging: Simple yet effective. Inserting statements at critical parts of the application can help track data flow and spot issues.
- Third-party Debugging Tools: Tools like Node Inspector or Chrome DevTools provide more advanced debugging capabilities, allowing developers to visualize the code execution and find problems more easily.
- Error Monitoring Services: Using services like Sentry or Loggly can help in tracking errors in real-time, enabling developers to respond quickly and analyze issues as they arise.
- Testing Frameworks: Implementing testing frameworks such as Mocha or Jest can help catch errors during development before deployment, ensuring a robust application.
Securing Database Connections
Securing database connections is fundamental in the realm of backend development, particularly when working with Node.js. As applications scale and handle sensitive data, ensuring the integrity and confidentiality of database connections becomes increasingly critical. The potential for unauthorized access or data breaches necessitates a robust focus on security. By implementing proper authentication and authorization measures, alongside strategies to prevent common vulnerabilities, developers can create a secure data environment.
Authentication and Authorization
Authentication is the process that verifies the identity of users or systems trying to access the database. This step confirms whether the individual trying to establish a connection has the appropriate credentials. Authorization follows authentication and involves granting permissions on what actions authenticated users can perform in the database.
A few essential points include:
- Use Strong Passwords: Ensure that the credentials used for connecting to the database are strong. This reduces the risk of brute force attacks.
- Environment Variables: Store sensitive information, like database passwords, in environment variables. This practice helps in keeping hard-coded secrets out of your source code.
- Multi-Factor Authentication (MFA): Implementing MFA adds an extra layer of security, making it harder for attackers to gain unauthorized access even if they have the correct password.
Implementing comprehensive authentication and authorization can safeguard your database from various exploits. Use libraries and frameworks that support robust security features, which can help streamline this process.
Preventing SQL Injection Attacks
SQL injection attacks occur when attackers manipulate the input data to execute arbitrary SQL code within your database. These attacks can lead to unauthorized viewing of data, deletion of records, or even full database compromise. Thus, prevention strategies are vital to maintain the integrity of any application.
Some effective measures to protect against SQL injection include:
- Parameterized Queries: Also known as prepared statements, they ensure that SQL code and data are processed separately. This method protects against unwanted SQL code execution.
- Input Validation: Always validate user inputs. This includes checking format, length, and data types against expected parameters.
- Least Privilege Principle: When creating database users, ensure they have the minimum permissions required for their tasks. This limits potential damage from a successful attack.
"Implementing basic security practices can significantly reduce your risks of data breaches and attacks."
Taking proactive measures against SQL injection and ensuring proper authentication protocols can enhance the security posture of your Node.js applications. This forms a backbone for secure data management, ultimately contributing to the reliability and integrity of the systems in which these databases serve.
Performance Optimization Techniques
Performance optimization is a critical aspect when working with databases in Node.js applications. As developers aim for faster responses and improved efficiency, it becomes essential to employ various techniques that ensure the application runs smoothly. Enhancing performance can lead to better user experiences and increased scalability. This section will discuss two vital techniques: connection pooling and data caching strategies. Both techniques help minimize latency and conserve resources, which is particularly important in high-traffic environments.
Connection Pooling
Connection pooling is a method used to manage database connections more efficiently. Instead of creating a new connection for each request, a pool of connections is maintained and reused. This reduces the overhead associated with establishing connections, which can be time-consuming.
Some key benefits of connection pooling include:
- Reduced Latency: Since connections are reused, the wait time for establishing a new connection is eliminated.
- Resource Management: Limits the number of active connections to the database, which prevents overloading and ensures that resources are used efficiently.
- Improved Throughput: With faster connection handling, applications can manage higher loads, which is crucial for scaling solutions.
To implement connection pooling in Node.js, you can use popular libraries such as for PostgreSQL or for MySQL. Here is an example code snippet using :
This approach allows multiple requests to be handled efficiently, leading to noticeable performance improvements.
Data Caching Strategies
Data caching is another important technique for optimizing database performance. By storing frequently accessed data in memory, the need for repeated database queries is minimized. This speeds up data retrieval and reduces the load on the database.
There are several strategies for implementing data caching:
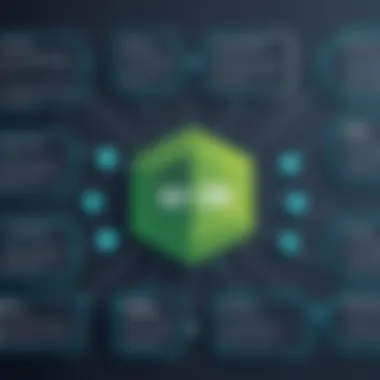
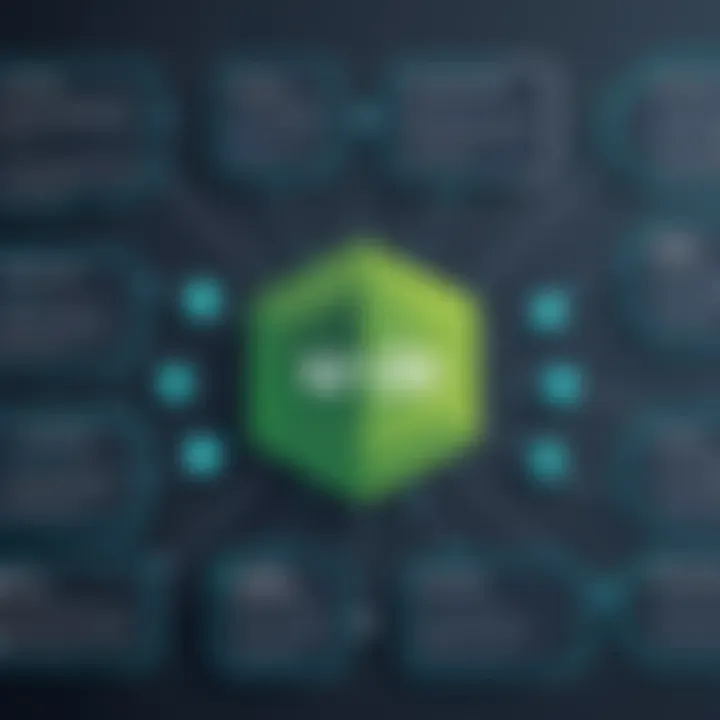
- In-memory Caching: Use systems like Redis or Memcached to store data temporarily. This allows for quick access to data without querying the database every time.
- Client-side Caching: Store data on the client side using tools such as local storage or session storage. This reduces the need for server interaction, which can improve response times.
- Cache Expiration: Implement expiration times for cached data. This ensures that stale data does not persist and that fresh data is fetched from the database as needed.
Using caching effectively can significantly reduce database load and improve application performance. It's essential to balance caching strategies with data accuracy to ensure that users are always working with the latest information. With the right techniques in place, Node.js applications can achieve optimal performance, making them more responsive and reliable.
"Performance optimization is not just about speed; it's also about responsible resource management."
Integrating connection pooling and data caching into Node.js applications can lead to noticeable enhancements in performance. By understanding and applying these techniques, developers can craft more efficient applications that meet user demands effectively.
Testing Integration with Databases
Testing the integration of databases with Node.js applications is vital. It ensures that the interaction between the application and the database operates smoothly. There are several key aspects to consider when testing this integration. These include identifying issues before they affect users, ensuring data integrity, and validating that the application meets its performance requirements.
Effective testing can significantly reduce bugs and errors that otherwise disrupt functionality and harm the user experience. Developers should not ignore the importance of having a robust testing strategy in place. Each test performed provides a safety net that can catch integration flaws.
Unit Testing
Unit testing focuses on individual components of the application. In the context of database integration, this means testing functions or methods that interact with the database directly. By isolating these components, developers can ensure that each piece of their code works correctly before being combined with other parts of the application.
To ensure thorough testing of database-related functions, developers might consider several practices:
- Mocking Database Calls: This practice allows testing the logic of functions without the need for an actual database connection. Tools like Sinon.js can help achieve this.
- Test-Driven Development (TDD): This approach involves writing tests before writing the actual code. TDD helps in maintaining high code quality and reliability, which can be particularly useful when building complex database interactions.
- Testing for Edge Cases: Developers should test scenarios such as empty databases, invalid inputs, or connection failures. These edge cases often expose weaknesses in the logic used to manage database interactions.
Here is a simple example of a unit test using Jest that checks if a function retrieves data correctly:
Integration Testing
Integration testing, on the other hand, examines how different modules or services work together. This stage is crucial for identifying issues that occur when combining various parts of the application, especially when those parts interact with external systems such as databases.
Here are some common considerations for integration testing in this context:
- Real Database Connections: Unlike unit testing, integration tests often use real databases. This ensures that dependencies are accurately represented in the tests, leading to reliable outcomes.
- End-to-End Scenarios: Integration tests may cover user stories from start to finish, ensuring data flows correctly through various components. This can include creating a user, retrieving user data, and updating it, all in one test script.
- Database Migrations: Testing how new database schemas interact with existing code is crucial, especially when deploying updates. Integration tests should verify if the application still works after changes.
As shown, both unit and integration testing serve different but complementary roles. They provide valuable insights into the functionality and performance of Node.js applications interacting with databases. It is worthwhile to invest time and effort into building a comprehensive testing strategy.
Best Practices for Database Management in Node.js
The management of databases in Node.js applications necessitates careful consideration of various best practices. These guidelines are essential for ensuring efficient data handling and integrity, crucial for the performance of applications. Proper database management leads to faster response times, improved scalability, and a reduction in maintenance overhead. As applications grow, following these practices becomes increasingly important, so neglecting them can result in significant issues down the line.
Data Validation and Sanitization
Data validation and sanitization stand at the forefront of secure and efficient database management. When an application accepts input from users, there is always a risk of unexpected data formats or malicious attacks. This makes it paramount to validate the incoming data before processing it.
Key aspects of data validation include:
- Type Checking: Ensure that the data types of inputs are consistent with what the database expects. For example, if an age field is expected to be an integer, accepting string or floating-point values can lead to application errors.
- Range Checks: For numerical values, check if the input falls within acceptable limits. For instance, a user’s age must be a positive integer.
- Format Validation: This is essential for fields that have specific formats, such as email addresses or phone numbers. Regular expressions can be employed to enforce these formats.
Sanitization focuses on cleaning the data to remove or alter any harmful elements. This step is particularly important in the context of database interactions to mitigate threats such as SQL injection. Applying a library like or incorporating built-in sanitization features from database frameworks can aid in hardening input against malicious content.
Monitoring and Logging
Monitoring and logging are crucial in maintaining the health of Node.js applications that rely on databases. Adequate monitoring allows developers and system admins to detect performance issues before they escalate to critical failures. Logging provides an essential audit trail, assisting in troubleshooting and forensic analysis following incidents.
Benefits of effective monitoring and logging include:
- Performance Insights: Tracking response times and query performance helps in identifying bottlenecks in database interactions. This data can inform decisions about query optimization or infrastructure scaling.
- Error Detection: Automated alerts can be set up to notify developers of unusual events or errors in real-time. This proactive approach minimizes downtime and enhances user experience.
- Comprehensive Audit Trails: Maintaining logs of database transactions and user interactions enables developers to backtrack and understand what went wrong, aiding in improving system reliability for the future.
To implement monitoring, tools like New Relic or Datadog can be integrated into the application to provide real-time insights. For logging, using Winston or Morgan in a Node.js application allows for flexible logging options, such as outputting to files or external monitoring services.
"Proper data handling is not just about efficiency; it is key to safeguarding against vulnerabilities that could jeopardize the entire application."
Following these best practices can enhance database management in Node.js applications, providing a foundation for building robust, efficient, and secure web applications.
Future Trends in Node.js Database Management
The landscape of database management continues to evolve rapidly. As developers seek improved performance and scalability, understanding these trends becomes essential. For those utilizing Node.js, staying in tune with future developments can enhance application effectiveness and user experience. The following sections delve into emerging technologies and cloud-based solutions, providing insights into how these advancements can be leveraged in Node.js environments.
Emerging Technologies
Emerging technologies are reshaping how databases function and interact with programming languages like Node.js. Some key innovations include:
- Graph Databases: Technologies like Neo4j showcase how relationships can be pivotal in data structuring. Node.js can interface efficiently with these databases, allowing for advanced queries and insights into complex data connections.
- Time-Series Databases: As IoT and real-time analytics grow, time-series databases such as InfluxDB gain momentum. Node.js applications can tap into this trend to analyze data streams over time, providing valuable insights in real-time scenarios.
- Artificial Intelligence Integration: The infusion of AI into databases is becoming prominent. With Node.js, developers can build applications that leverage AI-driven data analysis, making decision-making processes more streamlined and intelligent.
Understanding these technologies is not only beneficial but vital. They enhance the ability of Node.js applications to manage diverse data types effectively, optimize performance, and enable complex functionalities that meet user demands.
Cloud Databases and Serverless Architectures
The rise of cloud databases and serverless architectures marks a significant shift in how applications are built and deployed. These trends offer several advantages:
- Scalability: Cloud databases like Amazon DynamoDB provide on-demand scaling. This flexibility is invaluable for Node.js applications that may experience variable traffic. Developers can easily adjust resources without worrying about underlying infrastructure.
- Cost Efficiency: Serverless architectures reduce costs by allowing developers to pay only for the resources they consume. This model is particularly appealing for startups and small businesses that need to optimize their budgets while building applications.
- Focus on Development: With cloud solutions, developers can focus on writing code rather than managing servers or databases. This can lead to faster development cycles and quicker deployment of new features.
As cloud technologies continue to advance, Node.js will likely integrate even more seamlessly with various cloud services, ensuring that developers can harness their benefits effectively.
Ending
The conclusion is a crucial component of this article as it encapsulates the key themes discussed throughout and underscores the relevance of understanding Node.js databases. In a world where data drives decision making, a solid grasp of database integration with Node.js becomes essential for developers. It influences not only how applications perform but also their scalability and maintainability.
Recap of Key Takeaways
- Diverse Database Options: Node.js supports various database types, including relational and NoSQL options, each with unique strengths. Developers should choose based on the specific requirements of their projects.
- Architectural Patterns: Understanding architectural patterns such as MVC and microservices is vital for effectively integrating databases with Node.js. These patterns help organize code, making it cleaner and easier to maintain.
- Performance Considerations: Techniques like connection pooling and data caching significantly enhance performance and help in managing resources efficiently.
- Security Measures: Securing database connections through proper authentication and authorization means that data remains protected, minimizing risks of breaches.
- Testing and Debugging: Robust testing strategies, including unit and integration tests, are essential for maintaining functionality and reliability in complex applications.
- Best Practices: Implementing data validation, monitoring, and logging are key for effective database management, ensuring data integrity and application stability.
Resources for Further Learning
For those interested in deepening their understanding of Node.js and database integration, several resources may assist:
- Wikipedia: Offers a broad overview of Node.js, covering its features and use cases.
- Britannica: Provides insights into Node.js architecture and its applications in web development.
- Reddit: Engages with a community of developers sharing knowledge and experiences about Node.js.
- Facebook: A platform for asking questions and discussing advancements and updates related to Node.js.
Understanding the interplay between Node.js and diverse database options equips developers with the necessary tools to create powerful applications. As trends continue to evolve, the knowledge gained here will increasingly prove invaluable.