Understanding MVC Architecture: A Comprehensive Guide
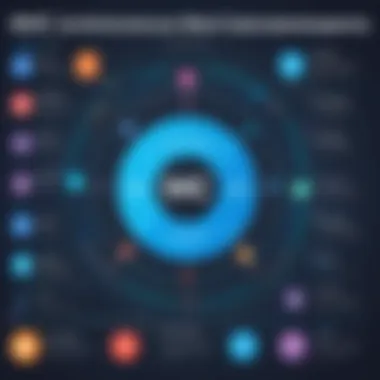
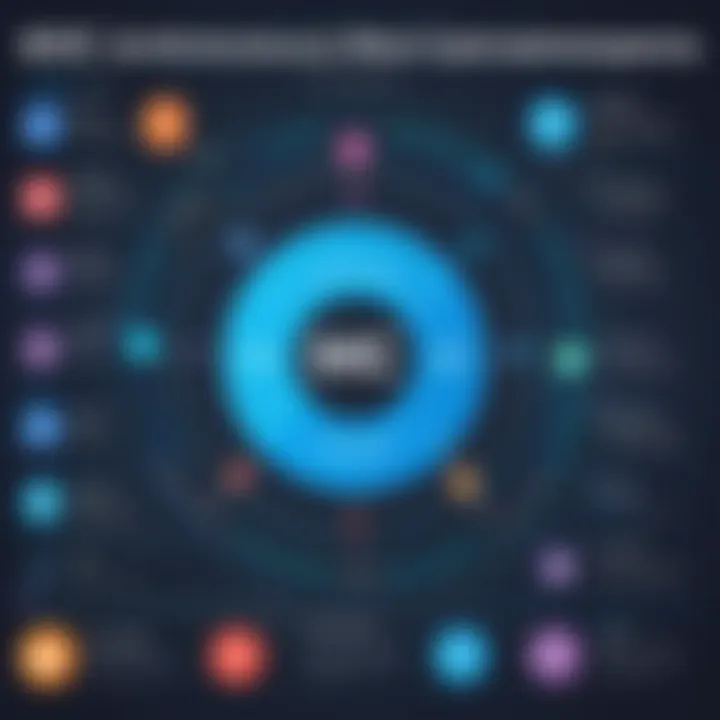
Intro
In the ever-evolving world of software development, the Model-View-Controller (MVC) architecture stands as a beacon of organization and clarity. It provides a structured way to divide an application into three interconnected components that handle different aspects of user experience and data management. This separation fosters a more modular approach to programming, allowing developers to work more efficiently and effectively tackle challenges that arise during the application's life cycle.
Key Points of Discussion
Throughout this comprehensive guide, we will journey through the intricacies of MVC architecture. We’ll break down each component—Model, View, and Controller—illustrating how they interact. Also, we will explore various programming languages that implement MVC, hinting at the framework's universality. Additionally, you'll find detailed discussions about the benefits of MVC, including its role in promoting code reusability, maintainability, and ease of testing.
At the end of this article, our aim is crystal clear: equip seasoned developers and eager learners alike with vital knowledge to implement MVC in their programming endeavors.
Let’s embark on this journey to demystify MVC architecture. The road ahead is lined with insights that will arm you with a deeper understanding of not just MVC, but also the principles that guide efficient coding.
Intro to Architecture
In the world of software development, efficient structuring of code is key. Without optimal organization, projects can become unwieldy, hard to maintain, and downright chaotic. This makes understanding the Model-View-Controller (MVC) architecture immensely important. MVC serves as an architectural pattern that simplifies the development process by dividing an application into three interconnected components. Each part specializes in its own concern, thus promoting clean code and enhancing viability for both growth and alteration.
By embracing MVC, developers gain clarity in their coding practices. For example, rather than having business logic and user interface tightly coiled together, each can evolve independently. This isolation provides an unmistakable benefit: easier debugging and testing.Consequently, the application becomes more resilient and adaptable to change, allowing developers to pivot quickly without stumbling over previous design choices.
With that background, let’s dive deeper.
Defining
MVC stands for Model-View-Controller. Breaking it down, the Model handles data and business logic, the View takes care of what the user interacts with, and the Controller acts as the intermediary between the two. This clear distinction allows developers to focus on a specific concern without the clutter of navigating unrelated code. The Model is about the application's state, the View is the visual representation, and the Controller processes user input.
Imagine a restaurant: the Model would be the kitchen where the meals are prepared, the View would be the dining area where customers experience the ambiance and savor their dishes, while the Controller is akin to the waiter, taking orders and ensuring a smooth service from kitchen to table. This analogy captures the essence of how MVC operates.
Historical Context
The origins of MVC trace back to the late 1970s. Initially conceptualized within the Smalltalk programming environment, MVC was established as a way to streamline user interface development. The goal was to separate concerns—the data model from the presentation layer and the control logic. This separation made it easier for developers to manage programs as they grew in complexity.
By the mid-1990s, web technologies began adopting MVC principles. Rails, developed in 2004, popularized MVC in the web context. As web applications became more sophisticated, frameworks like Ruby on Rails harnessed MVC, marking a turning point in how developers approached coding. Understanding its roots provides insight into why MVC has stood the test of time and remains a preferred choice in software architecture today.
Components of Architecture
The Model-View-Controller (MVC) architecture serves as a fundamental framework in software design. In this section, we will explore the three core components of MVC: the Model, the View, and the Controller. Each of these plays a vital role in how an application is structured and functions. Understanding these components is not just an academic exercise; it's crucial for building scalable, maintainable, and efficient software.
Model Explained
The Model is at the heart of the MVC architecture. It represents the data and the rules that govern access to and updates of this data. Think of it as the engine of a car; without it, the car won’t move.
The Model is responsible for:
- Data Management: It directly manages the data, whether it's fetching from a database or pushing updates.
- Business Rules: It encapsulates the business logic, ensuring that any changes made coincide with the set guidelines of the application.
- State Representation: The Model holds the current state of the application, which is crucial for processes and interactions.
A well-structured model can significantly improve code organization. For instance, using a simple yet effective approach like the Active Record pattern allows you to instantiate objects that directly map to database records. Consequently, this alignment paves the way for clearer and more expressive code. Here’s a snippet to illustrate a basic model in Python:
View Explained
The View is what the user interacts with. This component takes the data from the Model and presents it visually. It’s akin to the presentation layer in a business meeting. You want to engage your audience, not dull them to sleep.
The View's responsibilities include:
- Presentation Logic: The View handles how data is displayed, relying on templates to render the interface.
- User Interaction: It captures user input and interaction, which is then sent to the Controller for processing.
- Separation from Logic: Keeping the View separate from the Model helps maintain clarity and aids in future changes.
Using frameworks like Django, you can easily create views that dynamically pull from your models, allowing even novice developers to whip up functional interfaces in no time. A simple HTML template could look like this:
Controller Explained
The Controller acts as a middleman between the Model and the View. If the Model is the engine and the View the display, then the Controller is the steering wheel that directs where the application goes.
The Controller performs several essential functions:
- Input Handling: It receives user inputs from the View and processes them, passing relevant commands to the Model.
- Application Flow: The Controller decides what happens next; based on input, it may call upon different methods in the Model.
- Updating the View: After the Model is updated, the Controller will guide the View to refresh based on the new data.
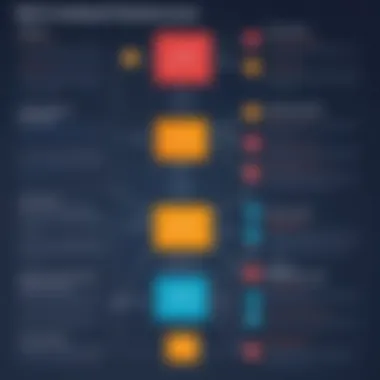
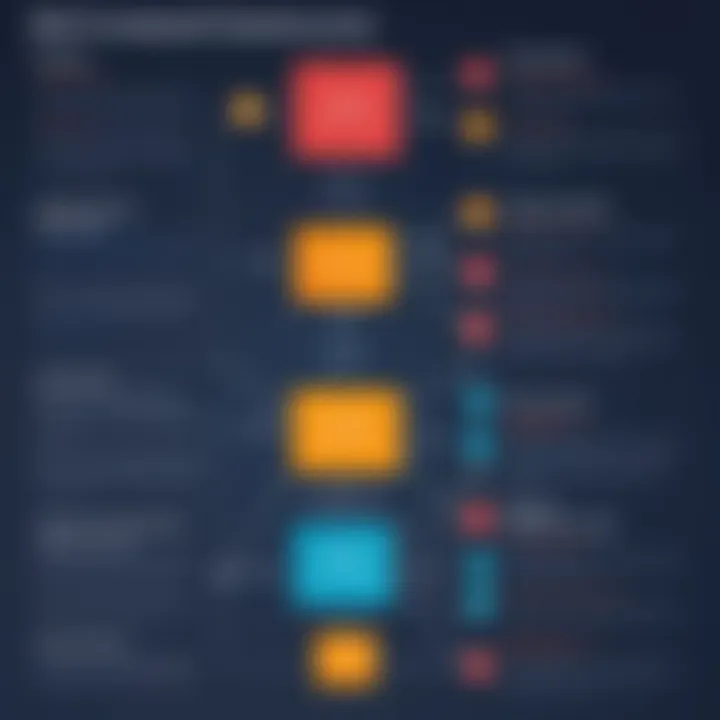
Often, Controllers are designed to handle various routes and endpoints in web applications. For instance, a Controller method in a RESTful API for updating user data might look like this:
Key Insight: Emphasizing the separation of concerns in MVC helps prevent tangled code and enhances maintainability.
Understanding these components is just a stepping stone. Mastering how they interrelate can elevate your programming skills significantly. By grasping their roles, you'll be better equipped to implement and innovate using MVC architecture.
The Role of Each Component in
In the Model-View-Controller (MVC) architecture, each component has a unique role that contributes to the overall functionality and performance of applications. This section dives deep into these roles, shedding light on their significance, advantages, and practical considerations. Grasping the interplay of each component is crucial for developers who aim to leverage MVC to its fullest potential.
Model: Data Management
The Model serves as the core of any MVC application. It's where the application's data is stored, manipulated, and processed. Think of it as the backbone, housing the essential data that drives the entire application. It communicates directly with the database, ensuring that data operations, like creating, reading, updating, and deleting, happen seamlessly.
*"A well-structured model can simplify the complexities of data transactions, providing a clear pathway for data handling."
One of the prime benefits stems from the separation of concerns. Developers can modify the data logic in the Model without touching other components, thus minimizing the chances of introducing bugs elsewhere in the application. Moreover, as the application scales, having a robust Model allows for straightforward adjustment and enhancement, which is vital for long-term maintainability.
View: User Interface
The View is the visible part of the application—the front end. It displays the data provided by the Model and sends user commands to the Controller. In essence, the View presents the information in an understandable format, transforming raw data into a user-friendly display.
In a well-designed MVC framework, the View operates independently from both the Model and the Controller. This ensures that any changes made in the layout or presentation don’t affect the underlying data or the logic that processes user input. The use of disjointed templates to render UI elements is a common practice here.
To illustrate, when a user interacts with a button on the interface, they expect a corresponding reaction. The View must seamlessly represent this in a way that is coherent to the user, often relying on libraries or frameworks like React or Vue.js to make that interaction visually appealing.
Controller: The Mediator
The Controller acts as the glue between the Model and the View. It interprets user inputs from the View and translates them into operations on the Model. This interaction supports the flow of data and keeps the components finely tuned.
When a user clicks a button, for instance, the Controller reads that action, determines the logical processing required, and updates the Model accordingly. Afterward, it instructs the View to refresh or alter the UI to reflect the updated data. Thus, every exchange of information between the Model and View is channeled through the Controller.
In a nutshell, the Controller harmonizes the functions of the Model and View, ensuring that user actions lead to the correct responses. An important caveat is to avoid letting the Controller bloat with too much logic; this can lead to a tangled mess that defeats the MVC's purpose. Keeping it slim and focused is paramount.
This holistic understanding of how each component operates within the MVC framework is vital. The Model, View, and Controller not only facilitate clear organization of code but also promote a cleaner workflow. This architecture is particularly valuable for new developers or those learning programming languages, as it provides clarity amid complex processes.
Benefits of Architecture
Understanding the benefits of MVC architecture is crucial for developers, both experienced and those just dipping their toes in programming. MVC, short for Model-View-Controller, establishes a clear framework that not only enhances the design of applications but also promotes a more organized approach to coding.
When one thinks about the intricacies of software development, various aspects come into play, from user experience to code maintainability. MVC structures everything in a way that facilitates these goals. In this section, we'll take a closer look at three significant benefits: enhanced separation of concerns, increased flexibility and maintenance, and facilitating parallel development.
Enhanced Separation of Concerns
The concept of separation of concerns is a pillar of good software design. In the case of MVC, it's like drawing a line in the sand between different aspects of an application. The Model handles data, the View is responsible for displaying that data, and the Controller coordinates how the two interact.
To illustrate, consider a simple web application for managing tasks. The tasks themselves—their status, due date, and descriptions—are represented by the Model. When a user adds or updates a task, it's the Controller that processes these commands and updates the Model accordingly. Meanwhile, the View displays this information to the user.
This separation allows developers to make changes in one layer without adversely affecting the others. For instance, if there's a bug in the presentation of tasks, one can debug the View without touching the logic in the Controller or the data layer in the Model. This is essential for both efficiency and debugging, ensuring fewer headaches down the line.
Increased Flexibility and Maintenance
Flexibility in design is key for evolving technology and user needs. MVC architecture supports this flexibility by allowing developers to swap out or update components independently. For example, if a better framework emerges for the View layer, replacing it won’t necessitate a complete rewrite of the Model or Controller. This easy interchangeability saves time and resources and keeps the project nimble in a fast-paced tech environment.
Moreover, ongoing maintenance becomes more manageable with MVC. The project can grow as features are added, and diverse development teams can work on various components simultaneously. This approach minimizes the need for constant communication and coordination, which can bog down productivity. In fact, it becomes more of a breeze to enhance functionality or fix bugs over time, as the structure is sound from the get-go.
Facilitating Parallel Development
One cannot underestimate the advantages of parallel development. In simple terms, multiple developers can work on different parts of the application at the same time without stepping on each other's toes. This is where MVC shines. For instance, while one developer focuses on optimizing the Model for better data handling, another can fine-tune the View for improved user experience.
This aspect becomes increasingly critical when working on larger projects involving numerous team members. Each role can focus entirely on their area—be it data handling, user interaction, or business logic—resulting in a more efficient workflow.
Some organizations find that embracing the MVC pattern leads to faster development cycles, which can directly translate into quicker time-to-market for new features. As the saying goes, "many hands make light work,” and in the MVC framework, this saying rings true.
Implementing in Various Languages
The Model-View-Controller (MVC) architecture is not just a theoretical concept; it’s a practical framework that can be implemented across various programming languages. Understanding how to implement MVC in different environments enhances a developer's versatility and contributes significantly to their coding efficiency. Each language has its nuances, which can influence design decisions, performance, and even the developer’s experience. This section provides a detailed exploration of how MVC can be implemented in three prominent programming languages: Java, C#, and Python.
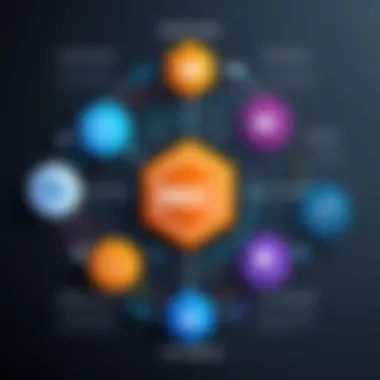
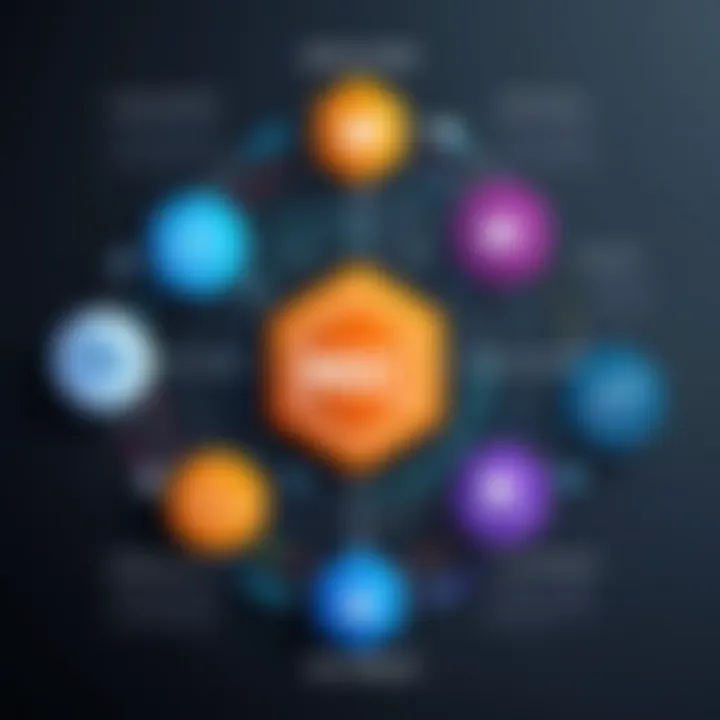
in Java
Java is a robust language commonly used for large-scale enterprise applications. Its strong typing and object-oriented nature make it a great fit for MVC.
Key Features of in Java:
- Spring Framework: A popular framework that allows easy implementation of MVC based applications. It helps manage dependencies and promotes better code organization.
- Separation of Concerns: By utilizing Java’s convention-over-configuration approach, developers could easily separate the model, view, and controller. This can be seen in a typical Spring application where Controllers handle web requests, Models represent data, and Views display the output.
- Annotations: With features like @Controller, @RequestMapping, and @ModelAttribute, Java MVC frameworks simplify the coding process, making it elegant and less verbose.
A simple example of a controller in Spring might look like this:
in
C# is another language widely used in enterprise environments, especially when leveraging the .NET framework. MVC implementation in C is primarily facilitated by ASP.NET MVC.
Benefits of in #:
- Rapid Development: The use of scaffolding in ASP.NET allows developers to create setups quickly, especially for CRUD operations.
- Powerful Web API: C# MVC has strong integration with Web API, making it easier to create RESTful services.
- Built-In Features: Built-in support for dependency injection and tools for routing and model binding make the implementation straightforward.
For example, a simple action method in a C# MVC controller could look like this:
in Python
Python’s elegant syntax and dynamic nature make it another popular choice for implementing MVC, especially with frameworks like Django and Flask.
Considerations for in Python:
- Django’s MTV: It employs a variant known as Model-Template-View (MTV). The emphasis remains on data and logic separation, maintaining the essence of MVC.
- Flask Flexibility: This lighter framework allows developers to structure their applications as they see fit while adhering to the MVC principles.
- Community Support: A large community and extensive documentation make learning and implementation easier even for those new to the language.
Here's how a simple Flask view function could be written:
Understanding and implementing MVC in these various languages strengthens the foundations of efficient and scalable software development. The choice of framework or language can greatly impact the application's adaptability and ease of maintenance. With each offering its own set of strengths, developers should select tools that best meet their project needs.
"The choice of framework can either liberate or constrain the development process."
As seen, knowing how to navigate and leverage MVC in Java, C#, and Python opens diverse pathways in application design and architecture.
Common Challenges with Implementation
MVC architecture certainly brings a lot of benefits to software development, but it isn't without its challenges. Understanding these obstacles becomes crucial, especially for early-career developers who seek to harness the full potential of this framework. The difficulties associated with MVC can affect scalability, maintainability, and even the developer experience itself. Addressing these challenges not only enhances the efficiency of the MVC model but it also empowers developers to create applications that are easier to update and manage in the long run.
Complexity in Small Applications
When developing small applications, the MVC architecture can sometimes feel like using a sledgehammer to crack a nut. The overhead introduced by creating distinct Model, View, and Controller components might outweigh the simplicity that small projects typically demand. In a simple app, programmers might find themselves trudging through unnecessary boilerplate code and having to navigate multiple files for what could have been accomplished in a single script.
For example, if a tiny fruit store app is being built, having separate model classes for storing fruit data, views for rendering the interface, and a controller to handle user input could seem excessive. This setup can result in fast-paced iterations slowing down owing to the cumbersome structure. Here, the architecture may inadvertently introduce layers of unnecessary complexity, leading to absurdity.
- Less Overhead: In small apps, developers might prefer a leaner, more straightforward coding approach.
- Development Time: Creating distinct components can extend development time, resulting in potential risks of project delays.
- Initial Setup: The initial setup for MVC often requires more time than simply writing straightforward code.
Though complexity can arise in small applications, it’s important to remember that, in many cases, an MVC structure can still yield clearer long-term benefits, especially if the app is intended to scale or evolve.
Overhead of Design
The overhead associated with MVC design pertains to both the amount of code generated and the cognitive load required to develop within this architecture. One of the key points to consider is that introducing three distinct parts—Model, View, and Controller—means programmers must manage the interactions between these components carefully. For inexperienced developers, this can lead to confusion and mismanagement, potentially resulting in bugs that can be a headache to troubleshoot.
Moreover, as applications grow, so do the dependencies among components. Managing these dependencies effectively requires a good understanding of the architecture and solid planning skills. A real-world scenario can be seen with the Spring Framework in Java, where managing various components can lead to what is often termed the “pulling hair” phenomenon. Additionally, if a developer fails to implement good architecture practices, they may face a situation where the code becomes tightly coupled, resulting in nightmare scenarios for testing and debugging.
- Increased Code Base: The requirement for specialized components often leads to a larger codebase.
- Learning Curve: New developers may find they need to invest significant time to fully understand how to implement MVC correctly.
- Potential for Errors: As the application grows, mismanagement of MVC components can result in an increase in bugs and errors.
Adapting to these overheads can be challenging, but with practice and familiarity, the initial complexity often transitions into a more manageable structure, allowing developers to focus on building robust applications.
Important Insight: The challenges presented by MVC architecture should not dissuade developers from using it; rather, recognizing these issues can lead to better practices and a deeper understanding of how to leverage MVC successfully.
Real-World Examples of Applications
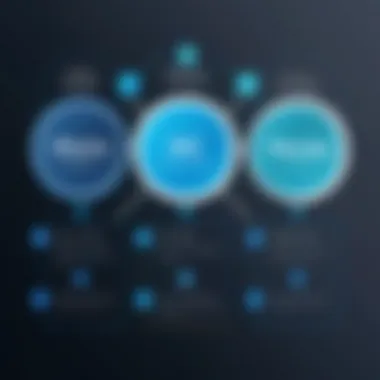
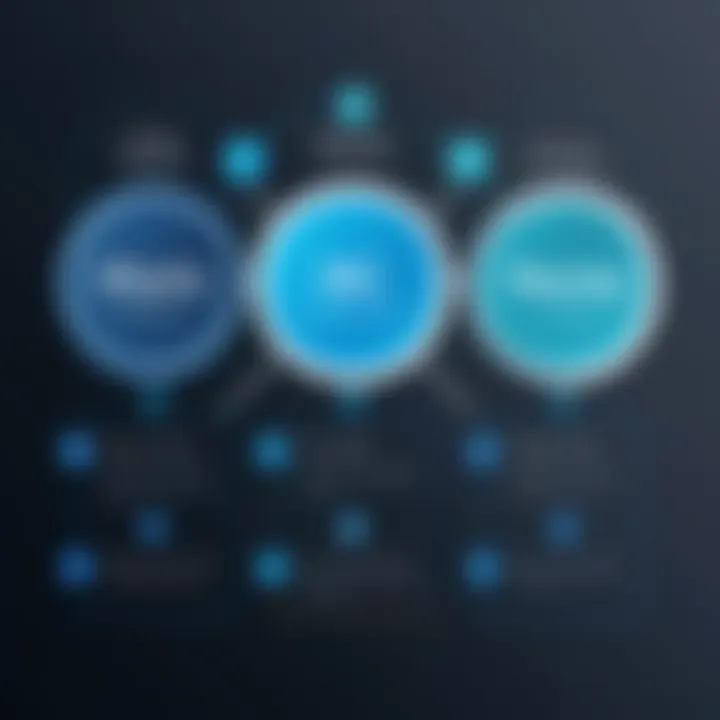
Understanding real-world examples of MVC applications is not just an academic exercise but a vital part of grasping how this architecture improves coding efficiency and organization. By examining practical implementations, one can appreciate the advantages it offers in terms of scalability, maintainability, and clarity in design. Simply put, knowing how MVC is applied in the field can be the difference between a successful project and one that's just a tangled web of code.
MVC architecture shines in many applications today. Let’s break down two prominent frameworks that use this structure effectively.
Case Study: Rails Framework
The Ruby on Rails framework stands as a quintessential example of MVC in action. Rails employs the MVC pattern to ensure that developers work within a clean and structured environment. Each component plays its role:
- Model: In Rails, models manage the data of the application. For instance, consider a blog application—here, a BlogPost model would handle database interactions like creating, reading, updating, and deleting posts.
- View: The view in Rails is responsible for displaying the information from the models. It uses templates to present data. When you think of rendering views with ERB files, you can see how MVC separates these concerns effectively.
- Controller: The controller acts as the bridge between models and views. It processes user input and interacts with models to fetch or modify data then forwards this data to the view for display. In our blog example, the PostsController might have actions like , , and for handling requests regarding blog posts.
Rails not only simplifies creating web applications but also lets developers adhere to best practices, making it a fine choice for beginners as well as seasoned professionals. Utilizing conventions over configuration has increased productivity, allowing developers to focus on the actual logic instead of boilerplate code.
"Rails emphasizes convention over configuration, making it easier to follow best practices without getting lost in specifics."
in AngularJS
AngularJS, another heavyweight in the world of web applications, adopts the MVC architecture to enhance its performance and usability. Whereas Rails handles server-side processes, AngularJS takes a different route, focusing on client-side rendering and interactive user experiences.
- Model: In AngularJS, the model is represented primarily through JavaScript objects. These objects carry data properties that the application works with. Let's say we are building a shopping cart application. The model might include products, their quantity, and user preferences—all handled within the Angular framework.
- View: Views in AngularJS use HTML combined with Angular's directives, allowing real-time updates to the UI based on the model's state. Changes to the model directly reflect in the view without complex DOM manipulations, showcasing the elegance of this architecture.
- Controller: The controller in AngularJS connects the model and view. It listens for events in the UI and manipulates the model accordingly. For our shopping cart, the ShoppingCartController could manage actions like adding or removing items from the cart, ensuring users see current data promptly.
In many ways, AngularJS offers a more dynamic approach to MVC by leveraging the power of the browser. This separation allows developers to create highly interactive and responsive applications that are not only user-friendly but also stand the test of time.
In summary, real-world implementations of MVC like Rails and AngularJS illuminate how this architecture can substantially enhance software development. They exemplify the principles of separation of concerns while providing a framework for developers to create robust and scalable applications. Knowing these examples equips aspiring programmers with the tools needed for success.
Advanced Concepts in Architecture
In the world of software development, MVC architecture serves as a strong backbone, but like any framework, it evolves. Advanced concepts in MVC architecture push the boundaries of traditional practices, allowing developers to harness modern methodologies effectively. Here, we’ll explore how MVC interacts with microservices and RESTful APIs, both pivotal in today's agile and scalable applications.
with Microservices
Microservices have gained traction as a preferable architecture in the realm of distributed systems. When combined with the MVC framework, microservices enable a more modular approach. This means that different components of an application can be developed, deployed, and scaled independently.
This independence not only provides flexibility but also enhances maintainability. Imagine a large application where the user interface is tightly coupled with the backend. Any modification in the backend would mean debugging the entire system. In contrast, with microservices, you can tweak the model or the controller without affecting the view. This leads to a quicker turnaround time for updates and bug fixes, promoting a smooth user experience.
- Benefits of MVC with Microservices:
- Scalability: Teams can scale specific services based on demand. If a certain feature receives traffic spikes, that microservice can be scaled without affecting the whole application.
- Fault Isolation: If one service fails, the rest can continue to operate. This does not just preserve functionality but also enhances the application's reliability.
- Diverse Technology Stack: Different microservices can be built using different technologies suitable for that service’s functionality, providing the ability to leverage the best tools available.
Implementing MVC with microservices isn't without its challenges. Developers must navigate complexities such as inter-service communication, database management, and ensuring consistent data across services. Yet, the payoff often outweighs these hurdles, making it a sought-after approach in the development arena.
Integration of with RESTful APIs
The advent of RESTful APIs has transformed how applications communicate over the web. Integrating MVC with RESTful APIs is a game changer in creating seamless and efficient data exchanges between the client and server. By leveraging HTTP methods and resource representations, MVC can effectively decouple the user interface from backend logic.
This integration allows developers to build applications where the view can call specific resources to fetch or manipulate data without having to know the intricacies of the server-side logic. The controller acts as a middleman, processing requests and directing them to the appropriate models.
"The great thing about RESTful APIs is that they allow for a more organized and maintainable codebase, which is a boon when working with large applications."
Key advantages of merging MVC concepts with RESTful APIs:
- Consistency: RESTful APIs provide a standard way to interact with the backend, ensuring consistent communication protocols across various services or components.
- Statelessness: Each client request is treated as an independent transaction, enhancing performance as the server does not need to store session information for clients.
- Interoperability: Any system capable of communicating via HTTP can interact with the application, opening doors to integrating various platforms and technologies.
As large scale applications grow, MVC integrated with RESTful APIs provides a robust structure. The components are clear-cut, interactions are straightforward, and the development process becomes more intuitive.
The End and Future Trends in
The conclusion of an article serves not only as a wrap-up but also as a beacon for future endeavors in the realm of software architecture. In the case of MVC architecture, understanding its evolution and application trends is crucial for any budding or experienced developer. Notably, this architecture method presents advantages such as systematic organization, ease of testing, and adaptability for team collaboration. Given these merits, the MVC approach has remained a go-to solution in many development landscapes.
Summarizing Key Insights
From our discussions, several key points stand out about MVC architecture:
- Separation of Concerns: Each MVC component plays a defined role, leading to clearer code and maintainable systems.
- Flexibility: Its structure allows for easy updates and enhancements without major overhauls.
- Cross-Platform Utility: MVC installations are found in a myriad of programming paradigms, providing robust support across various languages.
As developers navigate this landscape, they should ponder how these insights apply to their own projects. Will employing MVC enhance your project's clarity? Will it facilitate smoother collaboration among peers? Addressing these questions encourages thoughtful implementation.
The Evolution of
The path that MVC has taken over the years speaks volumes about adaptability and progress. Since its inception, developers have continuously refined MVC principles to cater to the demands of modern software development. For instance, the rise of microservices and serverless architectures has prompted an evolution in how MVC is implemented.
As we look to the future, trends hint at MVC adapting further to incorporate responsive design methodologies and real-time data processing capabilities. The use of GraphQL alongside MVC shows promise by allowing efficient data querying, reducing payloads and enhancing performance.
Furthermore, tools like React and Vue.js have emerged as front-runners in implementing MVC-based strategies while optimizing user experience and interactivity. The incorporation of these tools presents a shift, emphasizing not just backend MVC but also front-end integration.
In summary, as MVC continues to adapt, future trends will likely yield more streamlined architectures that seamlessly integrate diverse programming components, much to the benefit of software architects and developers. This ensemble of history, insights, and forward-looking approaches creates a vibrant context for future innovations in MVC.