Understanding Local Datetime in Programming
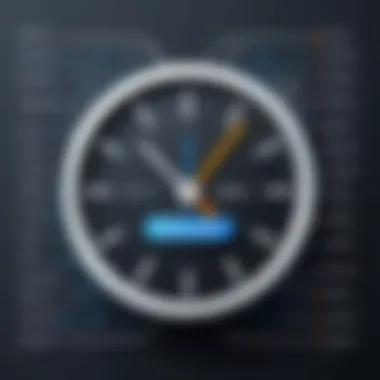
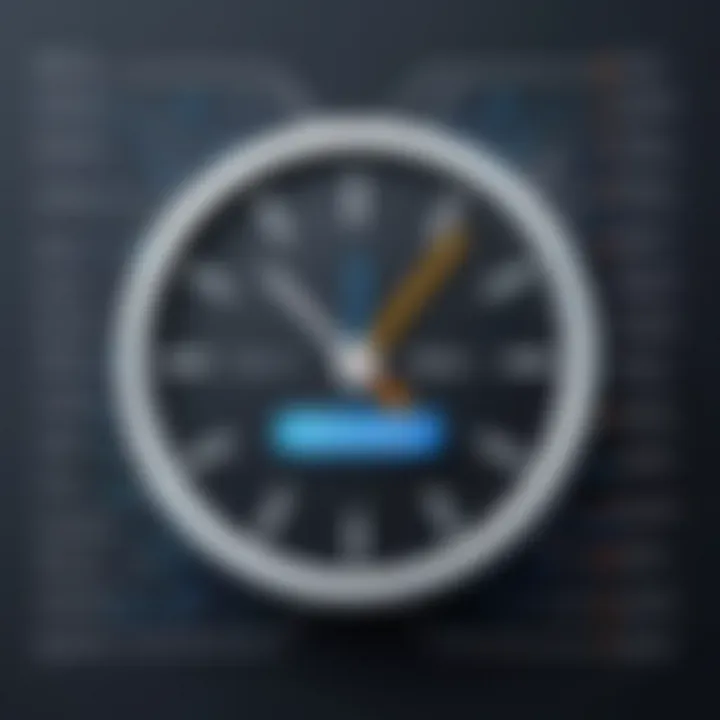
Intro
In the broad world of programming, the management of date and time can prove to be a tricky subject. Let's face it: we live in a time where calculation precision is paramount, and not knowing whether you are working with UTC or local time can lead to a multitude of problems.
When a program doesn't handle local datetime correctly, it's akin to trying to find your way in a labyrinth without a map. Only one wrong turn can take you miles away from your intended destination. Hence, understanding local datetime is not just a nice-to-have skill; it's crucial.
Here, we will explore the nuances of local datetime, emphasizing its significance in various programming languages. By breaking down the concepts in digestible chunks, we aim to arm beginners and intermediate programmers with the tools necessary to handle this topic with confidence.
"A stitch in time saves nine."
This old saying rings true in programming, especially when it comes to dealing with time.
The journey ahead will delve into how local datetime functions, best practices for managing it efficiently, and common pitfalls to avoid. Through this exploration, you will learn not just to grasp local datetime concepts but to wield them adeptly across environments.
Get ready, for we are setting sail on an enlightening voyage through the intricate yet rewarding realm of local datetime management.
Foreword to Local Datetime
In todayâs increasingly interconnected world, grasping the concept of local datetime becomes not just useful, but essential. Local datetime serves as the backbone for numerous applications, affecting everything from scheduling events to tracking user activities across different regions. Without understanding it, one might find themselves lost in the tangled web of timezones, leading to confusing results in their applications.
Definition of Local Datetime
Local datetime refers to the representation of date and time in a specific time zone. Unlike Coordinated Universal Time (UTC), which is a global standard and does not change with daylight saving time, local datetime is defined based on the geographical location of the user or system. It essentially houses the concept of time tailored to social practices, locale-specific habits, and variations in daylight, thus making it more relatable and easier to manage when programming. For example, when someone in New York schedules a meeting at 3 PM, that time is relative to New York's local timezone, not UTC.
Importance in Programming
Understanding local datetime is crucial in programming for several reasons:
- User Experience: A well-informed program can adjust times based on user location, providing a smoother and more relatable experience. Think about a travel app that shows flight departure times in the user's timezone.
- Data Integrity: Different systems may process dates and times differently. To maintain consistency, integrating local datetime helps to reduce ambiguity.
- Error Reduction: If developers neglect local context, they risk making an application that behaves erratically, especially when communicating with users globally. Miscommunication may arise if, say, an online store sends out a promotion at a time that doesn't align with Eastern Standard Time.
For beginners, acknowledging these factors during development can prevent future headaches down the line. In this way, local datetime is not just another technical concept; itâs an integral part of creating effective and user-friendly software.
Differences Between Local and UTC Time
Differentiating between local datetime and UTC time is a fundamental aspect that requires attention. Here are key points to consider:
- Time Zone Variability: Local datetime can vary based on time zones and the inclusion of Daylight Saving Time, while UTC remains static.
- Format: Local datetime may include additional data such as timezone information, making it richer in content compared to the more straightforward UTC format.
- Practical Applications: Local datetimeâs immediate relevance manifests in applications like calendars, where users expect to see their dates and times formatted according to local customs, not a generic UTC.
Local datetime enables applications to provide accurate information that aligns with usersâ real-world contexts, enhancing overall efficiency.
Understanding these differences helps programmers make informed decisions in their applications and systems, ensuring that they cater to a diverse array of users effectively. When you start to think about local datetime, you're not merely considering the numeral values; youâre tuning into the worldâs rhythm, understanding how various users interact with time.
Local Datetime in Programming Languages
Working with local datetime is a crucial element in programming, especially when developing applications that interact with users across various time zones. Understanding how to manage local datetime effectively can mitigate a host of potential issues such as miscommunication in scheduling, data inconsistencies, and poor user experience.
Java Local Datetime
Utilizing the java.time Package
The introduction of the java.time package in Java 8 is a significant advancement in handling date and time. This package offers comprehensive classes and methods to manage local datetime. One key characteristic that stands out is its ability to create immutable date-time objects, which ensures thread safety in programming. By using this package, developers can avoid common pitfalls associated with mutable date and time operations.
A unique feature of the java.time package is the class, which represents a date-time without a time zone. This is particularly beneficial for applications where the time zone is not essential, such as logging or local calculations. However, itâs crucial to remember that using this feature without considering time zone information can lead to inconsistencies if the application scales to a global audience.
Creating and Formatting LocalDateTime
Creating and formatting is straightforward, thanks to methods available in the java.time package. The method can be used to get the current date and time, while allows developers to create specific date-time instances. This makes it convenient to work with specific date ranges, catering to various application needs.
Furthermore, formatting options provided by the class enhance flexibility. It allows developers to format into string representations that are readable and suitable for user interfaces. However, misconfiguring the formatter can lead to formatting errors, which could confuse users if the date appears in an unexpected format.
Comparing Local DateTimes
Comparing instances provides useful functionality for developers needing to establish relationships between date-times, like determining if one event occurs before or after another. The , , and methods are handy in these scenarios.
However, a downside to relying solely on for comparisons is that it lacks time zone context. Comparing times from different time zones might lead to erroneous conclusions about the order of events without converting them into a consistent time zone first. Thus, careful implementation is vital to ensure accurate comparisons across varying contexts.
Python Local Datetime
Working with datetime Module
Pythonâs module serves as a powerful tool for managing local datetime, making it a favored choice for many developers. This module allows easy creation, manipulation, and formatting of date and time objects. A notable characteristic is the versatility it provides through both the class and its more specialized alternatives like and .
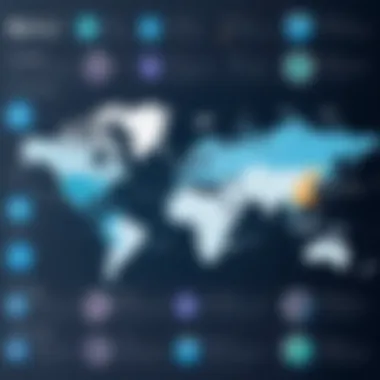
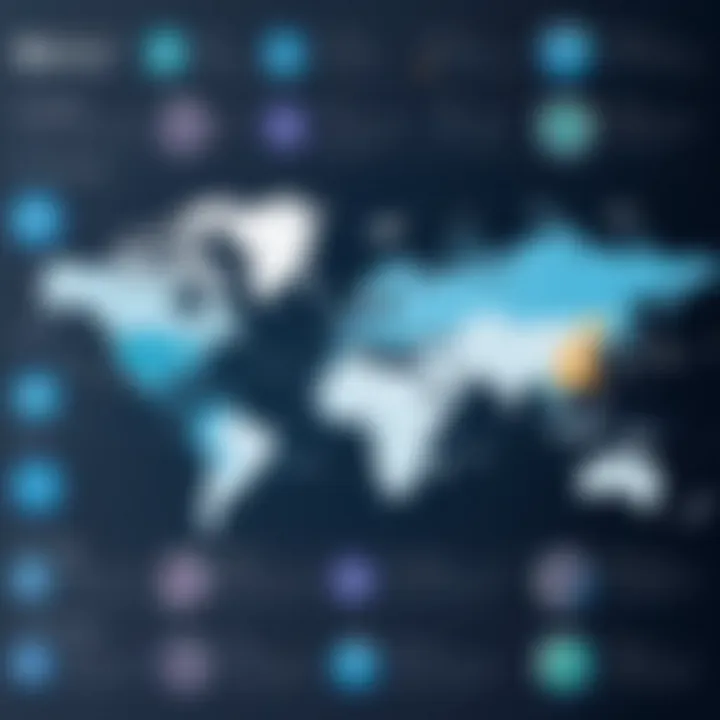
The moduleâs biggest advantage lies in its simplicity. Developers can create a object with just or , making it user-friendly for beginners. However, a caveat is the lack of built-in timezone support in the naive objects, which can lead to confusion when manipulating time if the developer does not account for zones.
Timezone Handling in Python
Handling time zones is crucial for applications that span multiple locations. Python provides the library for this purpose, allowing adjustment and conversion of naive datetime objects into timezone-aware objects.
One of the most significant features of is the ability to enable or convert datetime objects into different time zones easily. For example, effectively offers local time adjusted for New York.
However, while resolves many issues, it adds complexity into a code that may be unnecessary for some projects. Understanding this library comes with a learning curve, which is a consideration for those just getting their feet wet in programming.
Formatting Options for Local Datetime
The method in Python permits developers to format datetime objects into human-readable strings. Unique formatting features allow a variety of formats to be easily generated, such as for standard output.
This versatility is highly beneficial for applications that require user-facing dates and times, ensuring clarity and readability. The potential downside is that if date formatting is not handled consistently throughout the application, it could lead to confusion or misinterpretation by users and developers alike, especially in internationalization contexts.
Local Datetime
Understanding DateTime Structure
C#âs structure is essential for any developer working with local datetime. This structure encapsulates date and time in a single object, providing both attributes for the date and methods for manipulation. A defining feature is its capacity to represent dates and times with a precision of ticks, or 100 nanosecond intervals, making it advantageous for high-precision applications.
The ease of manipulation is another hallmark of the structure. Using methods like , developers can seamlessly manage dates while minimizing the error risk involved in manual calculations. However, one downside is that the structure lacks built-in timezone support, meaning developers may need to integrate additional libraries or implement their solutions when dealing with global applications.
Converting Between Time Zones
In C#, converting between different time zones can be effectively achieved using the class. This class allows developers to define time zones and perform conversions seamlessly, which is critical for applications that handle users from different regions.
One key feature is the method that helps adjust datetime when switching between time zones. While this offers flexibility and ease of use, developers must be cautious of daylight savings time changes that might impact the correct offset.
Manipulating Local DateTime Values
Manipulating values in C# can be done through various methods embedded within the structure. The methods such as enable conversion to UTC time, ensuring consistency across different regions.
While these methods are beneficial, programmers should avoid direct manipulations without thorough understanding, as incorrect assumptions about time zones or local changes (like daylight savings) can lead to significant issues in data integrity.
++ Local Datetime
Utilizing ctime Library
The library in C++ provides functionality for handling date and time operations. This library is fundamental yet basic, making it a good place for beginners to start understanding datetime concepts. One of the perimeter features is the ability to retrieve system time via , which returns the current time based on the system clock.
While this library serves well for straightforward tasks, it does not provide advanced features compared to other languages. Its simplicity can be double-edged; though easy to grasp for beginners, limitations might impede more complex applications requiring intricate datetime manipulations.
Creating and Formatting Dates
Creating and formatting dates in C++ often revolves around using structures like , which contains date and time representations. This approach, while clear, can be more verbose than necessary; developers may need several lines of code to produce formatted date strings.
One appealing factor is the compatibility with C-style strings and file operations, making it easy to integrate into varied applications that require file date logs. However, the verbosity can make it cumbersome for quick operations, especially in more extensive programs.
Time Zone Support in ++
Time zone handling in C++ is a challenging area due to the standard libraryâs limitations. While the library provides a foundation, it lacks built-in support for time zones. This absence means developers often rely on external libraries, which can add complexity to projects.
Although this enhances functionality, it requires learning additional tools, which may overwhelm newcomers. Itâs critical for developers to weigh the pros and cons of their specific project needs against the potential learning curves involved.
Understanding local datetime in programming is not just about creating and formatting dates; itâs about building reliable applications that respect users' time and location.
Best Practices for Managing Local Datetime
Managing local datetime is not just a trivial matter; it plays a significant role in ensuring applications function smoothly, particularly as they scale. Understanding best practices around this topic helps developers avoid common pitfalls such as discrepancies in time reporting, misalignments in transactions, or worse, data corruption stemming from incorrect datetimes. In this section, we will explore how to effectively manage local datetime, with a focus on practical elements like time zone management, error handling, and performance consideration.
Dealing with Time Zones
Understanding Time Zones
Time zones are a way to talk about the time in different places on Earth. Each zone has a standard offset from Coordinated Universal Time (UTC). This feature is crucial because it allows for accurate programming and database handling of datetime values. A dependable understanding of time zones enables applications to serve users correctly based on their physical locations.
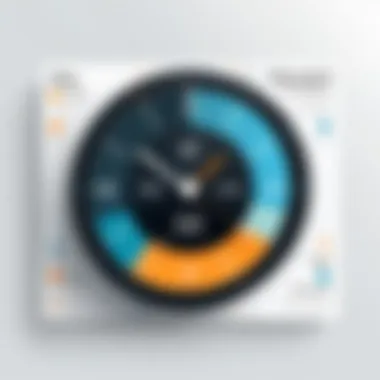
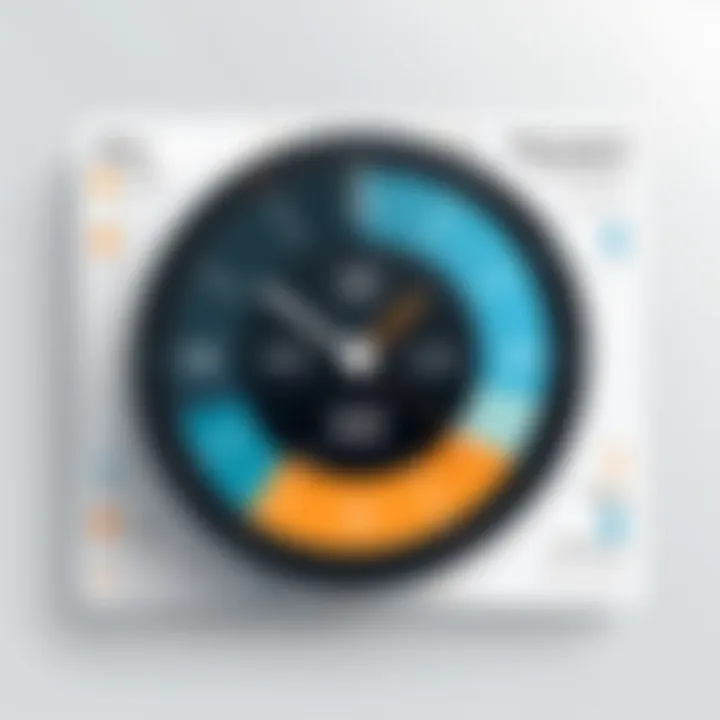
One might say that time zones are like a box of chocolates; you never know which one youâll get until youâre deep in the project. The primary benefit is that it helps in scheduling and organizing functionalities for applications used across different geographical regions. Yet with every benefit comes a unique challenge. Time zones can lead to confusion, especially when daylight saving changes are in play, and not all regions follow this practice. Being aware of these nuances makes dealing with time zones a double-edged sword.
Implementing Time Zone Conversions
Conversions between time zones is a pivotal task when dealing with datetime across diverse cultures and locales. This involves changing a time from one zone to another correctly, ensuring that the logic behind the moments absolutely holds. This task maintains relevance in applications, especially in scheduling, where appointments across time zones can lead to missed meetings if handled improperly.
Utilizing libraries like in Python or the package in Java can simplify this process. However, the unique feature of properly implemented time zone conversions is that they keep your application in sync without a hitch, allowing for smooth user interactions.
The drawbacks can include computational overhead. If not handled carefully, time zone conversions can slow down the execution of applications, particularly those requiring frequent datetime calculations.
Best Practices in Multi-Time Zone Applications
When developing applications that span multiple time zones, a good practice is to always store datetime values in UTC and convert to the userâs local time only during data presentation. This approach minimizes errors related to daylight savings changes, which can differ significantly.
A notable characteristic here is the importance of a consistent strategy in displaying local time. Keeping track of user preferences in timezone settings is essential. Meanwhile, this practice helps developers avoid any unsightly bugs during updates or changes, helping to maintain a high standard of quality in application performance.
However, managing user preferences can lead to increased complexity, which needs to be carefully designed to avoid bugs when users' preferences might change.
Error Handling in DateTime Operations
Common Errors
Errors in datetime operations often arise due to improper handling of leap years, daylight saving time transitions, and input format mismatches. These common errors can trip up even seasoned programmers. A solid understanding of how datetime works across programming languages is essential to minimize these pitfalls.
Recognizing and preemptively addressing these inaccuracies can save developers from facing useless headaches down the line, ensuring user experiences are seamless.
Error Handling Strategies
To manage errors effectively, itâs crucial to employ certain strategies, such as implementing a standard format for datetime input across the board. Relying on exception handling can also catch and resolve errors before they severely impact users.
These strategies are beneficial because they provide a safety net; thus, discouraging situations where an application could crash due to datetime mismanagement.
Importance of Validations
An area of high importance is validations during datetime input and operations. Validating data helps catch issues before they propagate through an application. Implementing checks to see if an input string is a proper datetime formation can save significant trouble later on.
With a high level of validation, developers can be confident that they are working with accurate data, resulting in fewer bugs and a higher-quality user experience. Yet, while validations are beneficial, they do add layers to the coding process which could complicate things slightly if not implemented cautiously.
Performance Considerations
Memory Management
Local datetime manipulations are memory-intensive operations, particularly when handling lists of dates, timestamps, or time zone data. Efficiently managing memory can lead to significant performance gains. Utilizing native datetime types instead of more complex structures whenever possible helps conserve memory.
This focus on memory means that when dealing with local datetime, less is often more, as simple implementations can yield better application performance.
Efficient Algorithms for DateTime Calculations
Utilizing efficient algorithms for datetime calculations is another aspect that can dramatically enhance performance. For example, the difference in days between two dates may appear straightforward but algorithms need optimization, especially when calculating across time zones.
Employing cutting-edge techniques can lead to time savings in an application, making it more responsive to user interactions. However, the trade-off could sometimes include an increased learning curve related to implementing and understanding these algorithms.
Reducing Complexity in Time Operations
Reducing complexity is a key task while managing datetime. When algorithms become overly intricate, they may lead to increased chances of errors occurring. To counter this, simplifying the logic behind datetime operations, using helper functions can often clarify the steps involved, allowing for cleaner, easier-to-read code.
By minimizing complexity, developers not only enhance application performance but also improve the maintainability of the codebase.
Managing local datetime is more than just coding; itâs about understanding how the world ticks and ensuring your applications resonate with local user's expectations.
Advanced Topics in Local Datetime
Understanding advanced topics in local datetime is crucial for developers who want to master the nuances of handling time effectively in their applications. Local datetime isn't just about the exact hour or minute; it weaves deeper into how data is stored, retrieved, and manipulated across various time zones and cultures. Each aspect contributes to the overarching goal of providing a seamless user experience, ensuring that every user sees the correct time, irrespective of their location.
Using Local Datetime in Databases
Storing Timestamps
Storing timestamps is a fundamental aspect in databases. This helps to capture events accurately in a system. The primary characteristic of storing timestamps is that it preserves the exact moment an event occurs. Timestamps are a popular choice because they allow for precise records, making them invaluable in applications where knowing the exact time is critical, such as logging or transactional systems.
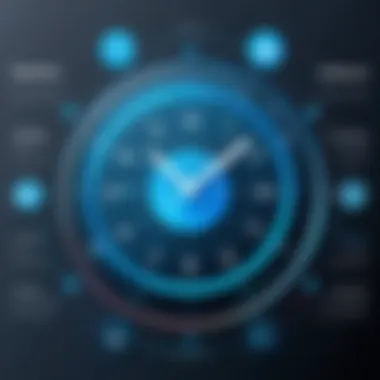
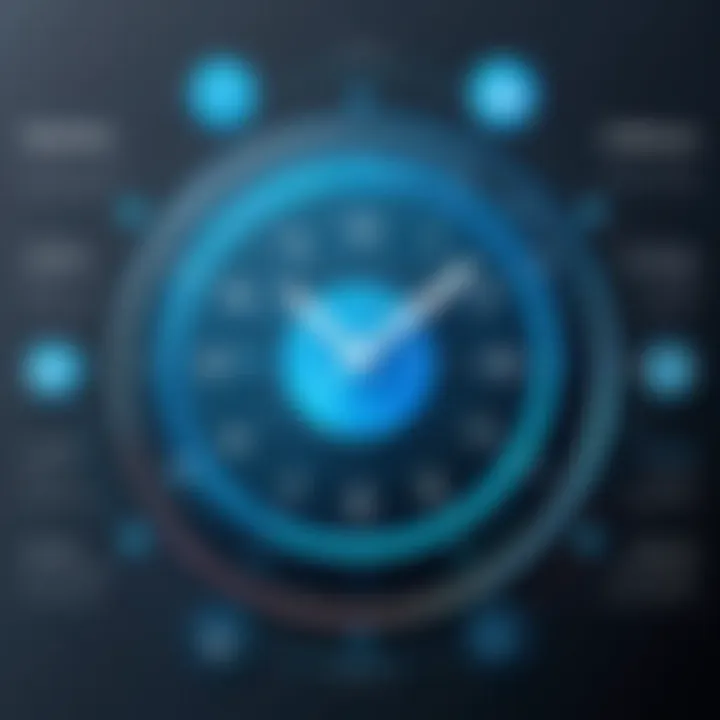
A unique feature of timestamps is their ability to express moments in time universally, regardless of local timezone, aiding in consistent data retrieval across platforms. However, one disadvantage might be that if a system does not adequately handle time zone conversions, it could lead to confusion or errors in interpreting the time.
Retrieving and Querying Local Datetime
Retrieving and querying local datetime is equally significant. This process entails fetching date and time data from databases with respect to a user's local context. The key characteristic here is that it should present data in a familiar way, considering the users' local time settings.
The benefit of querying local datetime is that it helps in creating user-centric applications - users can see timestamps in a format that makes sense to them. Unique to querying is the ability to filter and sort data based on local time, but a challenge arises in ensuring that all related events are accurate and logically coherent across different zones.
Dealing with Timezone-Aware Columns
Dealing with timezone-aware columns addresses the complexity of having data that changes context based on location. The key characteristic of timezone-aware columns is that they store date and time information along with its associated timezone offset. This is particularly beneficial in applications that involve a diverse user base spread across various regions.
A unique feature of this approach is the ability to offer localized time representation dynamically. The downside, however, could be the increased complexity in implementation and understanding, which may lead to mismanagement if not handled properly.
Localization and Internationalization
Impact of Locale on DateTime
The impact of locale on datetime is an essential consideration when developing software for global audiences. Locale specifies the geographical, political, or cultural region, influencing how date and time are presented. Its significance lies in adapting applications to meet specific cultural expectations, enhancing user satisfaction.
This characteristic ensures that dates and times are formatted correctly, aligning with local conventions. The unique feature is its ability to present this information in relatable formats, making it appealing and easy for users to understand. However, managing multiple locales can also complicate development, necessitating consistent testing across different environments.
Formatting for Different Cultures
Formatting for different cultures is bound to the notion of making applications accessible and user-friendly across borders. This involves knowing and applying various date formats as they differ widely from one culture to another. The principal characteristic here is adaptability; applications must adjust how they display dates and times based on cultural standards.
A unique feature of this formatting is its utility in promoting inclusivity, enabling users from diverse backgrounds to navigate through the dates easily. Nonetheless, the challenge remains in ensuring all formats are correctly implemented, as errors could lead to misunderstandings or frustrations among users.
User Customization and Preferences
User customization and preferences provide the flexibility that many users desire. This aspect focuses on allowing users to select their preferred date and time settings within an application. The highlight of this feature is personalization - aligning the application with individual user choices can foster a greater connection to the product.
A beneïŹcial trait of customizable settings is that it enhances user engagement, as individuals feel more in control. However, it can also lead to increased complexity in application design and backend management, as developers will need to cater to various preferences thoughtfully.
Future Trends in Local Datetime Management
Emerging Libraries and Tools
Emerging libraries and tools signify a shift towards more efficient and sophisticated methods for managing datetime functions. They often provide enhanced capabilities over older models, making programming more accessible. A significant characteristic of these libraries is streamlined functionality; developers can accomplish what once required extensive lines of code with just a few variables.
Unique to these emerging tools is their focus on handling complex datetime functions, reducing the risk of human error. But a major disadvantage could be the learning curve involved. Adapting to new methods requires time and effort, which can delay project timelines.
Impact of Cloud Computing
The impact of cloud computing on local datetime management is substantial as it offers scalable and flexible solutions. By leveraging cloud systems, applications can manage datetime operations more efficiently. This characteristic allows seamless access to data from anywhere with an internet connection.
A unique feature is the ability to synchronize events across multiple time zones automatically, which can be a game changer for global applications. However, this also introduces potential challenges, such as connection reliability and data latency issues that could complicate time-sensitive transactions.
Anticipating Changes in Standards
Anticipating changes in standards is pivotal for future-proofing software applications. As datetime norms evolve, staying ahead of those changes can provide a competitive advantage. The essence of this aspect is flexibility; developers who adjust practices in anticipation can mitigate risks before they arise.
A unique feature of staying ahead is maintaining consistency in user experience amidst change. Yet, the challenge here is in accurately predicting which changes to prioritize, given that not all shifts will have the same level of impact across different systems.
In summary, delving into advanced topics surrounding local datetime unveils layers of complexity that are often overlooked, yet essential for creating robust applications tailored for a global audience.
Finale
The conclusion of this article holds significant weight as it ties together the vast array of topics weâve explored throughout our journey into local datetime. This isnât just a simple wrap-up; itâs where we reflect on the critical elements that help programmers wield local datetime effectively in their projects. For those learning programming languages, understanding how local datetime interacts with their applications can mean the difference between a finely-tuned solution and a codebase riddled with time-related bugs.
Recap of Key Concepts
In this section, we summarize some of the core concepts covered:
- Definition of Local Datetime: Local datetime is essentially the time representation of a specific location, crucial for application logic where regional settings matter.
- Importance in Programming: Itâs vital for ensuring that applications run smoothly across different time zones and locales.
- Differences Between Local and UTC Time: This distinction matters when you need universal time coordination against the backdrop of local time specifics.
More than just an overview, understanding these concepts lays the foundation for proper manipulation and management of date and time in software. Without this knowledge, tasks like scheduling or timestamping can quickly spiral into a quagmire of confusion.
Final Thoughts on Local Datetime
As we wrap up, itâs essential to consider the long-term implications of what has been discussed. The robustness of applications fundamentally hinges on how well date and time are handled. The importance of local datetime will only grow as technology continues to expand globally, intersecting myriad time zones and cultures.
This is just the tip of the iceberg; emerging libraries and tools are set to revolutionize how local datetime is utilized. It is crucial for programmersâespecially the newbies and intermediate folksâto not only grasp these concepts but also stay updated with trends that shape local datetime management in software development.
"Emerging tools will redefine how we view and manipulate time within our applications, making it as crucial as any coding language itself."
By keeping a focus on these ideas, youâll not only build better applications but also embrace the concept of programming as a global endeavorâwhere time, quite literally, is of the essence.