Understanding Linked Lists: A Comprehensive Guide
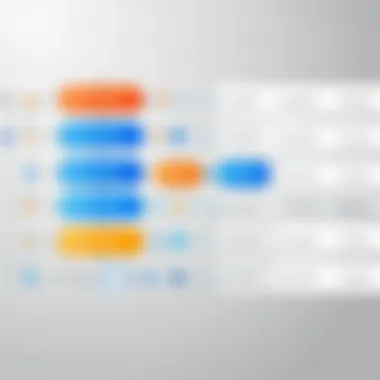
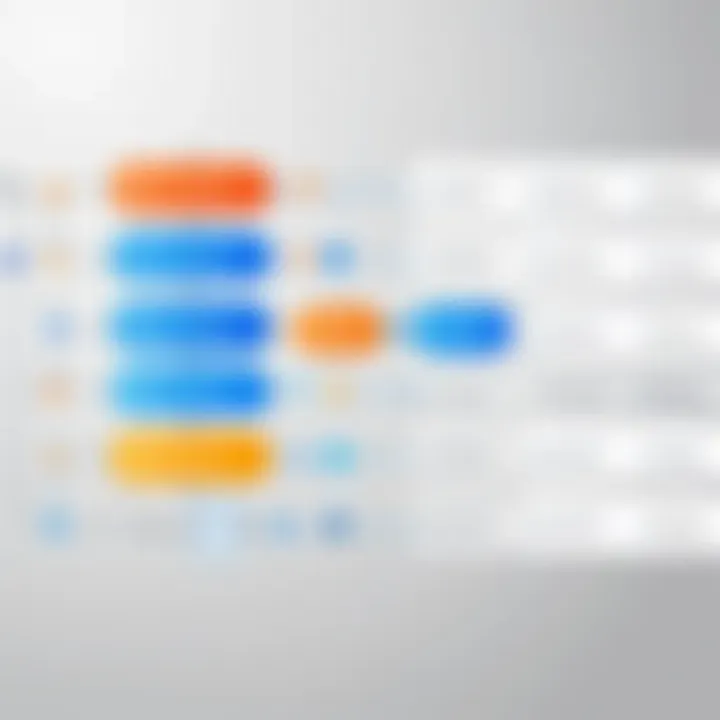
Intro
Linked lists are a cornerstone of computer science, playing a pivotal role in how we organize and manage data. They might seem unassuming at first glance, yet they power everything from basic sorting algorithms to complex graphic representations. If you've dabbled in programming, you're likely familiar with arrays and their rigid structure. But linked lists offer a different approach, one that's flexible and dynamic, catering to a variety of needs in software development.
Understanding linked lists requires grasping several fundamental concepts. Each linked list is composed of nodes, where each node contains data and a reference to the next node – or in some cases, the previous one. This setup facilitates efficient insertion and deletion operations, which can be cumbersome in arrays.
In this guide, we will not only dissect the structure of linked lists but also explore their types, advantages, and disadvantages. We’ll walk through practical applications in various programming languages, ensuring you have a well-rounded understanding of how and when to use linked lists effectively.
"Data structures are the building blocks of software. Mastering them enhances your problem-solving skills in programming."
As we progress, prepare to dive into some coding examples, as seeing these concepts in action provides valuable insights into their utility. Let’s embark on this journey to enhance your programming prowess through a detailed exploration of linked lists.
What is a Linked List?
In the realm of computer science, the term "Linked List" isn’t just a fancy jargon thrown around in programming classes; it serves a foundational role in how data is organized and accessed. Understanding what a linked list is can open doors to better coding practices and algorithm designs. In this section, we'll unpack the definition and the comparative strengths of linked lists, peeling back the layers on why this data structure deserves a spotlight.
Definition of a Linked List
A linked list is a linear data structure comprised of a sequence of elements, each known as a node. Each node contains two main parts: the data itself and a reference (or pointer) to the next node in the sequence. This structure allows for a fluid arrangement of data, where the next node's position isn't necessarily contiguous in memory, unlike arrays that store data at consecutive memory locations.
Think of a linked list as a chain of paperclips, each holding a piece of paper. Each paperclip (node) not only holds the paper (data) but also the information on where the next paperclip is located. This approach brings several advantages:
- Dynamic Size: Linked lists can grow and shrink in size easily, as nodes can be allocated and deallocated in real-time to suit needs.
- Non-contiguous Memory: Since data isn’t stored in contiguous memory locations, it minimizes memory fragmentation.
Comparison with Other Data Structures
When placing linked lists under the microscope against other data structures, the distinctions become clear. Each structure has its hefty purpose, yet linked lists stand out in specific contexts.
Consider arrays, for example:
- Memory Allocation: Arrays require a predetermined size and allocate memory accordingly. In contrast, linked lists indulge in dynamic memory allocation.
- Insertion and Deletion: Adding or removing elements from an array can be cumbersome, generally necessitating shifting items. Linked lists, however, simplify this process significantly; nodes can be added or removed without disrupting the entire structure.
But they also have their drawbacks when juxtaposed with such structures:
- Access Time: For accessing an element, arrays shine thanks to their indexed nature. Linked lists have a linear access time complexity because you must traverse the nodes sequentially to get to your desired element.
Moreover, stacks and queues, while they can be implemented via linked lists, may not always gain advantages. If random access isn't a priority, linked lists become quite appealing as they offer fluidity to sorting and grouping tasks, adapting as your application grows.
"Linked lists are like roads between towns—some might be winding, while others are direct, but each helps you get from one place to another, often with a bit more flexibility in route."
Understanding linked lists is not merely about learning terms or definitions—it is about grasping how they change the data handling game and carving pathways for modern programming. As we dig deeper into linked lists in this guide, it becomes evident how critical this structure is for efficient software development.
Core Components of a Linked List
Understanding linked lists requires a good grasp of their core components. These elements form the bedrock of linked lists and understanding them benefits programming and data structure manipulation. When working with linked lists, recognizing how their main parts function together can illuminate much of their versatility and appeal.
Nodes and Links
At the heart of any linked list are the nodes. Each node is like a small box, holding specific data and pointing to the next box in the list. This linking is what sets a linked list apart from other data structures like arrays. Picturing nodes as beads on a string helps visualize this - the beads are the nodes, and the string is the link that holds them together.
A node usually consists of two main parts: a data field and a pointer (or reference). The data field stores the value, which can be anything from numbers to complex objects, while the pointer directs where the next node is located. This arrangement allows linked lists to be dynamic in nature.
One of the key benefits of nodes and links is flexibility. Unlike arrays, which have a fixed size, linked lists can grow and shrink as necessary. This dynamic size allocation can significantly optimize memory usage. You can add or remove nodes without the need for massive data shifting - a real boon for performance!
"Flexibility in data structures often leads to more efficient algorithms, allowing programmers to tackle complex problems with ease."
Head and Tail Nodes
A complete understanding of linked lists isn't just about the nodes themselves, but also about how they interact as a group, notably through the head and tail nodes. The head node acts as the entry point to the linked list; it holds the data of the first node in the sequence. If you're trying to access or manipulate the list, you often begin at the head. Think of it like the first domino in a long line - push it, and the rest follow.
On the other end of the spectrum lies the tail node. It represents the last node in the list, crucial for various operations, such as finding the length of the list or adding a new node at the end. If you lose track of the tail, you could end up treading on thin ice; you wouldn't be able to navigate the list efficiently.
Identifying the head and tail helps in optimizing operations like insertion and deletion. In some types, especially circular linked lists, these definitions can blur; the tail, after all, can loop back to point toward the head. This characteristic can lead to unique advantages in specific applications, especially in systems requiring continuous cycling through data.
Types of Linked Lists
Understanding the various types of linked lists is crucial for any budding programmer or computer science student. Each type comes with its own unique characteristics, benefits, and use cases. By having a firm grasp on these differences, developers can choose the most appropriate structure for their specific needs, ultimately enhancing program efficiency and performance.
Singly Linked List
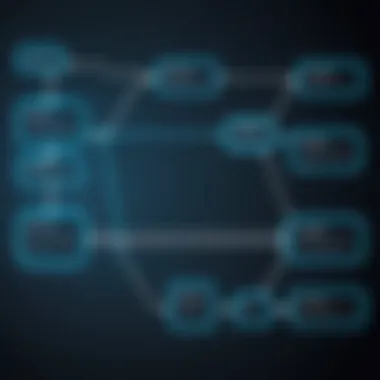
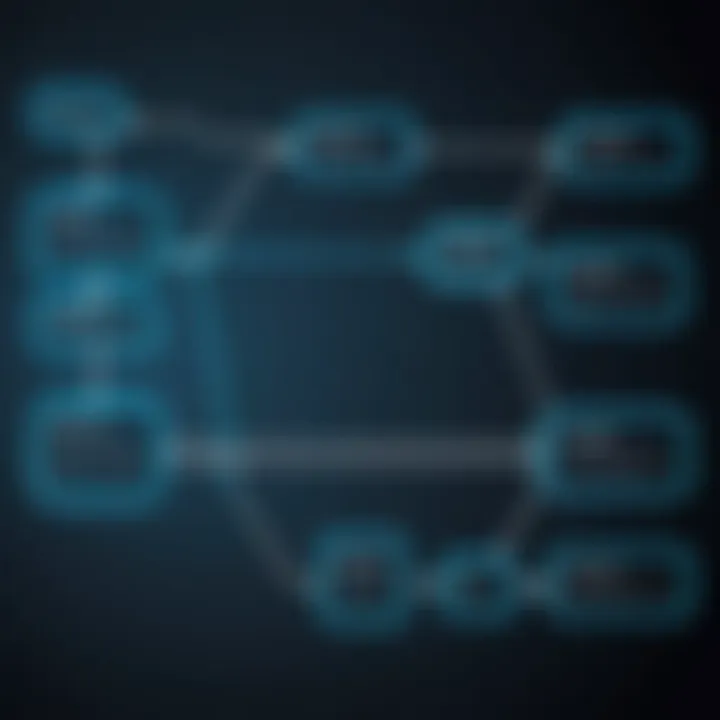
A singly linked list is often the foundational type of linked list most beginners encounter. It consists of a sequence of nodes, where each node contains two components: a data field that holds the actual value and a pointer that indicates the next node in the sequence. This structure allows for a straightforward and efficient way to traverse through the list.
One of the standout features of a singly linked list is its simple design. You can easily add or remove elements without needing to shuffle around existing nodes, which is a significant advantage over static arrays. However, you can only move forward through the list, meaning operations like reverse traversals can be cumbersome.
Here are some notable points to consider regarding singly linked lists:
- Memory Efficiency: There is little overhead; each node only needs to store a reference to the next node.
- Insertions and Deletions: Adding or removing elements from a linked list can typically be done in constant time, provided you have a reference to the node involved.
- Use Cases: Singly linked lists are suitable for stack implementations and simple data organization scenarios.
Doubly Linked List
Doubly linked lists take things a step further by introducing an additional pointer in each node. In a doubly linked list, every node has three elements: the data field, a pointer to the next node, and a pointer to the previous node. This bi-directional linking enables more flexible traversal.
The ability to traverse both forward and backward gives this type of linked list a higher level of versatility. Insertions and deletions become more convenient since you don't need to maintain a reference to specific nodes for operations at both ends of the list. However, this added functionality does introduce additional memory overhead since each node now stores two pointers instead of one.
Key aspects of doubly linked lists include:
- Bi-directional Traversal: This allows algorithms that rely on moving forwards and backwards through data.
- More Complex Structure: The additional pointer requirements necessitate more memory and slightly more complex logic for operations.
- Applications: Doubly linked lists work well for building complex structures like navigation systems in software or when implementing playlists in media applications.
Circular Linked List
A circular linked list is typically a more advanced version that can be either singly or doubly linked. In this structure, the last node points back to the first node instead of pointing to null, creating a looped effect. This arrangement allows for continuous traversing of the list without reaching an endpoint.
Circular linked lists are particularly useful in scenarios where you want to keep cycling through the items in the list, such as in round-robin scheduling or when implementing circular buffers. Another interesting aspect is that you can treat the list as if it had no endpoints, which can simplify certain algorithms.
Consider these important points about circular linked lists:
- Endless Traversal: You can iterate through the list without encountering a null pointer at the end, leading to potential efficiencies in certain algorithms.
- More Complex Insertions and Deletions: Care must be taken during operations to maintain the integrity of the circular reference.
- Useful Scenarios: Circular linked lists might be used in applications like multiplayer gaming turn management or even in continuous data streams.
In summary, understanding these types of linked lists equips programmers with versatile tools to tackle a variety of programming challenges. They each bring distinct benefits and trade-offs, making the choice of which to use dependent on the specific requirements of a project.
Advantages of Linked Lists
When diving into the world of data structures, linked lists stand out with a set of advantages that can greatly benefit the programming community. Their unique properties can address challenges that static data structures like arrays often face. Let's take a closer look at some of these advantages.
Dynamic Size Allocation
One of the most significant perks of using linked lists is their ability to allocate memory dynamically. Unlike arrays, which require you to define their size upfront, linked lists can grow or shrink as needed. This characteristic is particularly beneficial in scenarios where the amount of data is unpredictable.
Imagine a shopping cart in an online store. As customers add or remove items, the linked list representing that cart can seamlessly adjust without the need to allocate new memory or resize existing structures. In stark contrast, with arrays, if you hit your size limit, you might find yourself scrambling to create a new, larger array and move items over, which is cumbersome and time-consuming.
"With linked lists, one can manage data without the headaches of memory management typical of static arrays."
This flexibility means you only use as much memory as you need at any given time, leading to a more efficient use of system resources. The application scenarios are immense, from handling user connections in applications to accommodating varying sizes of data entries.
Ease of Insertion and Deletion
Another noteworthy advantage of linked lists is the ease with which you can insert and delete elements. In an array, if you want to insert or remove an element, you may need to shift other elements around to maintain order. This could result in considerable overhead, especially when dealing with large datasets.
On the other hand, with linked lists, adding or removing an element involves simply adjusting pointer references. For instance, if you need to insert a new node, you only need to change a few links to make the new node point to the next node in line, and adjust the previous node’s link accordingly.
This can significantly enhance the efficiency of operations such as enqueueing and dequeueing in a queue, or pushing and popping in a stack, where frequent insertions and deletions are the norms. You can think of it almost like shifting pieces in a game of chess; moving one piece doesn’t require reconfiguring the entire board.
Disadvantages of Linked Lists
When delving into data structures, it's essential to strike a balance. While linked lists come with a multitude of advantages, one cannot ignore the challenges they bring, especially when compared to other structures like arrays. Understanding these drawbacks is vital if you're looking to enhance your programming skills and make informed decisions in your coding journey.
Memory Overhead
One cannot underestimate the impact of memory overhead when working with linked lists. Each node in a linked list requires not only storage for the data but also memory for pointers, which link the nodes together. In a typical singly linked list, you have one pointer per node, whereas a doubly linked list necessitates two pointers per node.
This additional memory requirement means that linked lists can become unwieldy, especially when you're managing large data sets. For instance, if you have numerous small data entries, the memory cost of storing pointers can swell, leading to inefficient use of your system's resources.
Moreover, as each node is allocated individually in memory, it can lead to increased fragmentation. Over time, sporadic gaps of free memory might appear, making it harder for the system to find contiguous space for large arrays or other structures, which is important for performance optimization.
It’s vital to keep in mind that while linked lists are designed for dynamic memory allocation, their memory footprint can sometimes be counterproductive, particularly when compact storage is a priority.
Linear Search Time Complexity
Another significant downside stems from the search operation itself. When you want to find a specific element in a linked list, you're likely in for a lengthy process. Since linked lists do not provide direct access to their elements (unlike arrays), you'll often find yourself performing a linear search. This means you must start at the head and traverse each node until you find the target value.
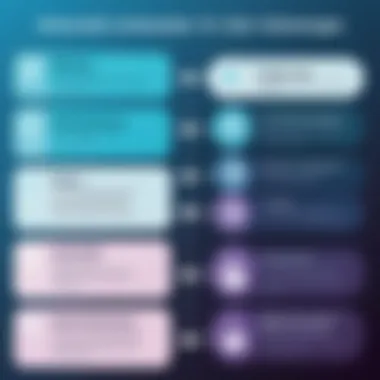
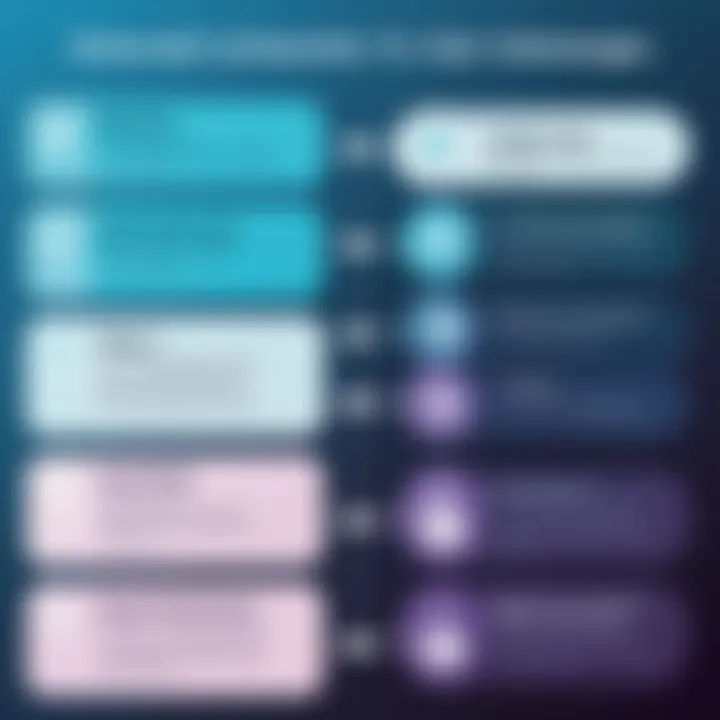
The time complexity for such a search is O(n), which can be a slog when dealing with large datasets. In contrast, data structures like arrays allow you to access elements directly via their index, leading to much more efficient search times, typically O(1) for indexed access.
Thus, in scenarios that demand frequent lookups, linked lists can become a bottleneck. When performance is key, especially in applications where quick access to data can mean the difference between success and failure, understanding this limitation is crucial.
In summary, while linked lists provide flexibility and dynamic sizing, their drawbacks regarding memory consumption and search efficiency are noteworthy. Recognizing these issues allows programmers to evaluate whether a linked list is genuinely the best fit for their project's requirements.
Implementation in Programming Languages
When it comes to understanding the underlying mechanics of linked lists, examining their implementation in various programming languages is essential. Each language offers a unique take on how to structure and manipulate this data type, which can fundamentally affect performance, memory management, and usability. By diving into implementation details in C, C++, and Java, developers can appreciate not just the versatility of linked lists but also the nuances that come with them. Depending on the language's features, certain characteristics might shine brighter; for example, memory control in C can be strikingly different from Java's built-in garbage collection.
Linked List in
C is often hailed as the quintessential language for system-level programming. When it comes to implementing linked lists, C provides low-level access that lets developers fine-tune their data structure for optimal performance.
A simple linked list in C would involve defining a structure to represent the node, along with pointers pointing to the next node. Here’s a basic example:
In this snippet, we're defining a with an integer field and a pointer to the next . The beauty of implementing linked lists in C lies in the control you have over memory allocation; it’s up to you to manage how memory is utilized, which can be both a boon and a bane. Remember, the only way to create and free nodes is by using functions like and , making it imperative to keep an eye on memory leaks.
Linked List in ++
Transitioning to C++, one notices added advantages due to the language's object-oriented characteristics. Thus, implementing a linked list can be done via classes, encouraging encapsulation and improved readability.
Consider a class for a singly linked list:
In this case, we’re creating a class and a class that manages the head pointer. The use of constructors allows for more flexibility and ease when initializing nodes. This captures an object-oriented spirit that many programmers prefer, taking advantage of features like polymorphism and inheritance, which can be leveraged when expanding the data structure’s functionalities.
Linked List in Java
Java simplifies many aspects of linked list implementation through its robust standard libraries which provide built-in classes such as . However, learning to implement a linked list manually can provide valuable insights.
A basic implementation would look something like this:
In Java, constructors are used much like in C++, but with automatic memory handling, you do not need to worry as much about freeing memory, as Java’s garbage collector takes care of it. This can reduce the risk of memory management errors but also shrouds the developer from perceiving the intricacies involved with linked lists.
Each of these approaches demonstrates that understanding linked lists isn’t merely about the coding itself; it’s about recognizing how the language’s features impact both the structure and the efficiency of your implementation.
"A solid grasp of linked lists can unfold new layers of understanding in programming beyond simply knowing how to implement them."
By studying linked lists in C, C++, and Java, aspiring programmers learn to navigate the waters of memory management, object-oriented design, and standard library efficiency.
Common Operations on Linked Lists
When working with linked lists, understanding the basic operations is crucial. These operations often define how effectively a linked list can be used and how well it can perform in various scenarios. Inserting new nodes, deleting existing ones, and traversing the list to access data are the three pillars of manipulating linked lists. Each operation plays a significant role in managing data efficiently and allows programmers to utilize the full power of linked lists in their algorithms.
Insertion
Insertion is a fundamental operation when dealing with linked lists. This process involves adding new nodes to the list. The method of insertion can vary depending on the type of linked list. Here are some important considerations:
- Types of Insertion: You can insert at the beginning, the end, or at a specific position. For example, if you want to insert at the front, you need to adjust the head of the list to point to the new node.
- Efficiency: Inserting a node in a linked list is relatively efficient compared to other data structures like arrays, as there’s no need to shift elements. For a singly linked list, insertion generally takes O(1) time if done at the head.
- Example:Let's say you have a linked list with values 5 -> 10 -> 15. If you wish to insert a node with value 7 at the beginning, the process is straightforward. You create a new node for the value 7, point this new node's next field to the current head (which is 5), and update the head to now point to the new node. Hence, your new list becomes 7 -> 5 -> 10 -> 15.
This simplicity of node addition is one of the advantages of linked lists and sets the stage for more complex operations.
Deletion
Deletion in a linked list is another vital operation, allowing you to remove nodes as required. The process can be tricky, requiring careful adjustments to maintain the integrity of the list. Below are considerations:
- Node Positioning: Like insertion, deletion can occur at the head, tail, or any specific position. The most common scenario is deleting the head node, which involves changing the head reference to the next node in the list.
- Memory Management: Proper memory deallocation is essential to avoid memory leaks. This is something that might not be as pressing with arrays, but in linked lists, failing to free up deleted nodes leads to wasted space.
- Example:Suppose you have a linked list of 10 -> 20 -> 30 -> 40, and you want to delete the node containing 20. You would access the node with value 10 and change its next pointer to refer to the node with value 30 instead. Consequently, your list would now look like 10 -> 30 -> 40.
Deleting nodes enables dynamic adjustments to the list’s structure, making linked lists adaptable and efficient for various data manipulation applications.
Traversal
Traversal is the means by which nodes in a linked list are accessed sequentially. This operation is essential for reading data, performing functions, or conducting searches within the list. Key points to remember include:
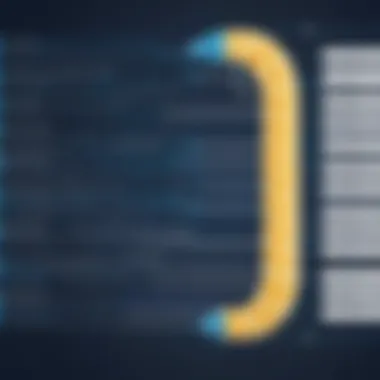
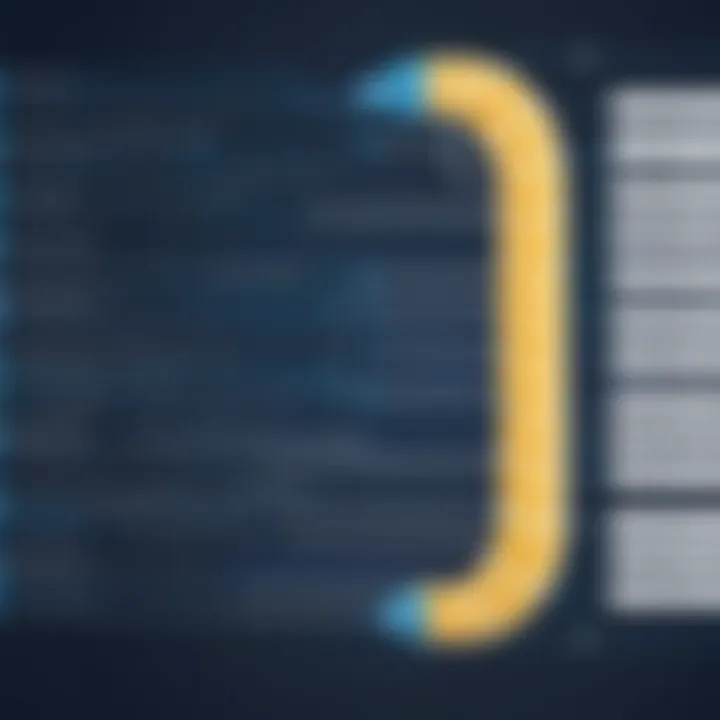
- Linear Access: In a singly linked list, you can only traverse in one direction—from head to tail. This can be limiting compared to other structures like arrays, where random access is possible.
- Utility in Algorithms: Traversal is often used in algorithms for searching or processing data. The time complexity for traversing a linked list is O(n), where n is the number of nodes.
- Example:Imagine you're checking the values in a linked list containing 1 -> 2 -> 3. You start at the head (1), read the value, and follow the next pointers until you reach the end of the list. This method is how you might implement a search operation or simply print the contents of a linked list.
"Traversal in linked lists can feel like reading a book, page by page, providing each piece of information step by step."
Practicing these operations—insertion, deletion, and traversal—forms a solid foundation for understanding linked lists and their application in coding and algorithm development.
Real-world Applications of Linked Lists
Linked lists stand as a pillar in computer science due to their flexibility and efficiency. Their applications spread across various fields, giving programmers and developers many powerful tools to enhance performance and improve resource allocation. In this section, we delve into two prominent applications of linked lists: dynamic memory allocation and implementing stacks and queues.
Dynamic Memory Allocation
When it comes to programs that demand a flexible amount of memory, linked lists shine brightly. Unlike arrays, which have fixed sizes, linked lists can grow or shrink as needed. This ability is crucial in scenarios where the size of the data cannot be predetermined.
Imagine running a social media application. Users join and leave the platform sporadically. Using arrays for user profiles would either waste space or lead to complex resizing efforts. With linked lists, each user profile can be created as a node in the list, making the system more dynamic and efficient.
Here are some essential benefits of dynamic memory allocation using linked lists:
- Efficient use of memory: They use only the memory required at a given time.
- Reduction of fragmentation: Since memory can be allocated and freed without predefined size, it minimizes wasted space.
- Easy reallocation: When a certain set of data no longer needs to be stored, it can simply be removed with less hassle compared to an array.
"In linked lists, each node points to the next, allowing quick memory reallocation that keeps programs running smoothly."
Implementing Stacks and Queues
Linked lists are also instrumental in the implementation of stacks and queues, two fundamental data structures used extensively in various programming tasks. Stacks follow a last-in, first-out (LIFO) order, while queues operate on a first-in, first-out (FIFO) basis. What makes linked lists ideal for them is their inherent flexibility to allow for efficient insertion and deletion operations.
In the case of a stack, elements are added to and removed from the same end, known as the top. By employing a linked list, adding or removing an item from the stack becomes easy and quick, often requiring only a couple of pointer adjustments. Similarly, for queues, linked lists enable efficient operations since elements can be added at the tail and removed from the head without the need for shifting elements, a necessity when using arrays.
To summarize the advantages of using linked lists for stacks and queues:
- No need for fixed size: You aren’t limited by a predetermined size, making them memory efficient.
- Speedy operations: Operations like push and pop for stacks or enqueue and dequeue for queues are typically O(1), translating to much faster performance.
- Simple implementation: The working of stacks and queues involves straightforward pointer manipulations that are easy to debug and maintain.
In a world increasingly reliant on performance and memory management, linked lists prove their worth time and time again. Whether you're allocating memory dynamically or managing data in stacks and queues, the adaptability of linked lists leads to robust and efficient software solutions.
Best Practices in Working with Linked Lists
Working with linked lists comes with its own set of hurdles, and understanding best practices is crucial for both efficiency and effectiveness in programming. Linked lists are distinct in their structure, offering flexibility, but this also brings about peculiar challenges in their management and functions. By adhering to certain best practices, one can not only ensure smooth operations but also enhance performance significantly. Here are essential considerations that every programmer should keep in mind when dealing with linked lists.
Memory Management Considerations
Memory management is a key aspect when it comes to working with linked lists. Given that these data structures dynamically allocate memory for each node, proper memory handling can make or break your application.
- Avoid Memory Leaks: When inserting or deleting nodes, ensure that you release any memory that is no longer in use. Not freeing memory leads to leaks, which can slow down your application over time. Leveraging languages like C or C++ requires explicit memory management. Using after a node's removal can avert memory wastage.
- Utilize Smart Pointers: In languages like C++, employing smart pointers can simplify memory management by automating the deletion process once the pointer goes out of scope. This reduces the chances of leaks and dangling pointers.
- Consider Fragmentation: Linked lists can lead to fragmentation of memory, which is especially pertinent in systems with limited memory resources. If you expect that nodes will be frequently added and removed, think about your memory allocation strategy ahead of time.
Proper memory management can enhance your program's performance and longevity. Not giving it due diligence could lead to complications down the line.
Choosing the Right Type of Linked List
Choosing the right type of linked list is another critical decision that can significantly influence both the performance and scalability of your application. Each linked list type has its merits and demerits, and the selection often hinges on the specific requirements of the task.
- Singly Linked Lists: These are the simplest form of linked lists, where each node points to the next. If your operations primarily involve low overhead on insertions and deletions, singly linked lists often suffice. However, iterating backward isn't possible, which might be a limitation in some applications.
- Doubly Linked Lists: These allow traversal in both directions. If your application demands frequent back-and-forth traversal or manipulations from both ends, a doubly linked list may be your best bet. However, they come at a cost of higher memory overhead due to the extra pointer.
- Circular Linked Lists: Here, the last node points back to the first node. This can be advantageous in scenarios requiring continuous looping through data, such as in music playlist management. Assess if your use case justifies this more complex structure.
When making a selection, it’s essential to factor in aspects such as:
- The frequency of insertions and deletions.
- Memory limits.
- The complexity of operations missing depending on traversal direction.
Consider running through a few scenarios where each type of linked list shines or suffers to ensure your choice aligns perfectly with your application’s needs.
Future Trends and Developments
The landscape of computer science is constantly evolving, and linked lists are not excluded from this transformation. Their adaptability has opened doors to new frontiers, making them integral not just in classic applications but also in emerging technologies. As we look ahead, the trend toward data-driven applications signifies that linked lists will play a vital role in handling dynamic data structures efficiently.
Linked Lists in Modern Applications
In the world of web development and mobile applications, linked lists are a backbone of many popular libraries and frameworks. From managing user sessions to implementing data streams, these structures are crucial in maintaining data integrity and improving the performance of applications. A perfect example is how the JavaScript ecosystem employs linked lists in libraries such as React. Here, they are utilized for managing the states effectively, allowing for a smoother and more responsive user experience.
Furthermore, in the realm of machine learning and artificial intelligence, linked lists are becoming pivotal in organizing vast amounts of data efficiently. With the sheer volume of data being generated, the ability to traverse and manipulate it without a fixed size is invaluable. Algorithms utilizing linked lists enable data to be processed more dynamically, catering to real-time analysis, which is essential for applications ranging from online recommendations to predictive modeling.
Comparative Analysis with Newer Data Structures
Comparing linked lists with newer data structures reveals both strengths and weaknesses. While linked lists offer excellent flexibility, they lag in speed when it comes to random access compared to structures like arrays or hash tables. However, the tradeoff is often worth it, especially when considering insertion and deletion efficiencies.
In data-heavy applications, hybrid structures are emerging that combine the benefits of linked lists with other concepts. Take the skip list, for instance; it utilizes linked lists but also includes pointers at various levels to enhance efficiency in search operations. This blend of traditional and modern techniques exemplifies how linked lists continue to adapt.
Additionally, as we embrace concurrent programming, the potential for lock-free linked lists holds promise for performance improvement. Efforts are underway to refine these structures to operate reliably in multi-threaded environments, void of the usual pitfalls encountered in more traditional implementations.
"In the digital age, being able to manage data effectively is akin to navigating the seas; the right structures will steer you clear of the whirlpools of inefficiency."