Understanding JavaScript Prototypes and Inheritance
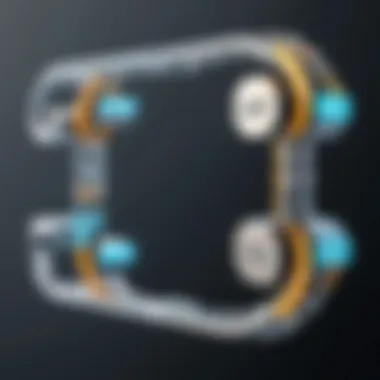
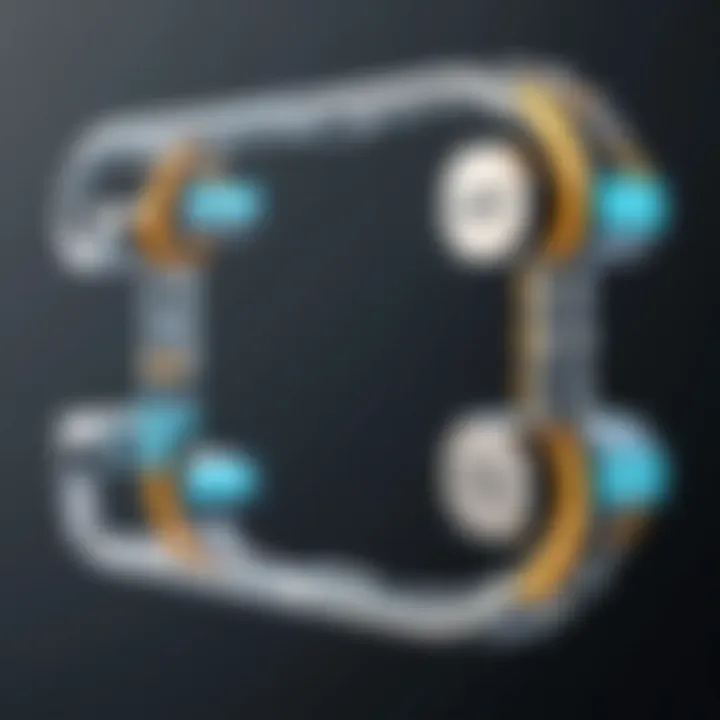
Intro
JavaScript, as a programming language, has grown tremendously since its inception in the mid-90s. Contrary to what some might believe, it isn’t merely a tool for adding interactivity to web pages but rather a full-fledged programming environment that supports complex applications. At the core of its functionality lies the concept of prototypes, which plays a crucial role in how inheritance is facilitated in this language. Understanding prototypes is essential for anyone looking to write more efficient and robust JavaScript code.
History and Background
When Brendan Eich devised JavaScript in 1995, he intended for it to be a lightweight language that could manipulate HTML documents. Over the years, however, it has evolved significantly. One of the most notable advancements is its support for object-oriented programming, albeit in a unique way compared to classical OOP languages like Java or C++. JavaScript's prototype-based inheritance, which uses prototypes as the basis for object creation and property sharing, sets it apart and lends a different flavor to how developers approach coding in this environment.
Features and Uses
JavaScript's features extend far beyond simple syntax. A few central elements include:
- Dynamic Typing: Types are checked at runtime, providing flexibility.
- First-Class Functions: Functions can be assigned to variables, passed as arguments, and returned from other functions.
- Event-Driven Architecture: Essential for handling user interactions seamlessly on web applications.
Due to these features, JavaScript has found usage in various fields from web development to server-side scripting and even in game development.
Popularity and Scope
Today, JavaScript undoubtedly stands as one of the most popular programming languages, frequently topping the charts of developers' favorites. With the advent of frameworks like React, Angular, and Node.js, its application has exploded well beyond the browser. According to surveys and usage stats (like on sites like reddit.com), JavaScript continues to dominate the development landscape.
Basic Syntax and Concepts
Understanding JavaScript basics prepares you for tackling more complicated concepts like prototypes. A brief overview follows:
Variables and Data Types
In JavaScript, you can declare variables using , , or . Each has its own scope and application.
- : Function-scoped variables, more prone to errors due to hoisting.
- : Block-scoped; ideal for loops or blocks.
- : Also block-scoped; meant for immutable variables.
Data types include:
- Number: Represents both integers and floats.
- String: Textual data enclosed in quotes.
- Object: Collections of key-value pairs.
Operators and Expressions
JavaScript supports various operators that facilitate data manipulation, such as arithmetic, comparison, and logical operators. Expressions combine these operators to produce values.
Control Structures
Control structures like , , and guide the flow of execution. Using these structures, JavaScript allows branching and looping through code, critical for developing dynamic applications.
Advanced Topics
As you understand the fundamentals, you’ll encounter more intricate concepts such as functions and prototypes.
Functions and Methods
Functions in JavaScript can be declared in several ways, including function expressions and arrow functions. Each type has its nuances, especially regarding the handling of the keyword in methods, which refers to the object that invokes the function.
Object-Oriented Programming
While JavaScript isn’t a classical OOP language, it supports OOP principles. The language employs a prototype-based model which differs from class-based systems. Understanding this model is vital, as it allows one to comprehend how inheritance operates in JavaScript.
Exception Handling
Error management done right can safeguard applications from unforeseen bugs. JavaScript adopts a conventional approach using the , , and blocks. This helps manage exceptions gracefully, ensuring a better user experience.
Hands-On Examples
To grasp the essence of prototypes, practical examples will be beneficial.
Simple Programs
A basic example might involve creating objects and using their prototype to add methods or properties shared across instances. For instance, consider:
Intermediate Projects
Moving beyond simple examples, you can explore more elaborate projects that utilize prototypes to manage complex data structures or to create libraries.
Code Snippets
Here’s one snippet demonstrating prototype chaining:
Resources and Further Learning
For those yearning to delve deeper into JavaScript prototypes and overall programming:
- Recommended books like "Eloquent JavaScript" provide great insights into the language’s intricacies.
- Online courses on platforms such as facebook.com and britannica.com offer hands-on learning.
- Joining community forums like reddit.com can also serve as a great way to gain knowledge from fellow learners and experts alike.
Understanding prototypes in JavaScript opens up a full new world of possibilities for a programmer. With it, one can harness the power of inheritance and create more scalable and maintainable code.
Preface to Prototypes in JavaScript
In the realm of JavaScript, where everything revolves around objects, understanding prototypes serves as a key cornerstone for mastering the language. Prototypes provide a mechanism for inheritance and shared behavior among objects. This is not just an abstract concept; it's the glue that holds the fabric of JavaScript's object-oriented nature together. By diving into prototypes, you empower yourself to write more efficient, reusable, and maintainable code.
When you create an object in JavaScript, it doesn’t exist in isolation. Each object can have its own hidden treasures in the form of properties and methods that can be shared. That’s where prototypes come into play. They allow individual objects to access methods and properties from a prototype object, promoting code reuse and minimizing memory usage. In contexts where performance is crucial, leveraging prototypes can lead to significant improvements, especially in applications with multiple objects needing similar functionalities.
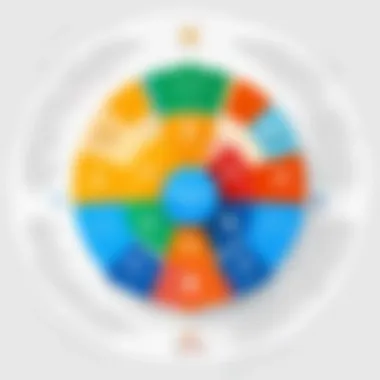
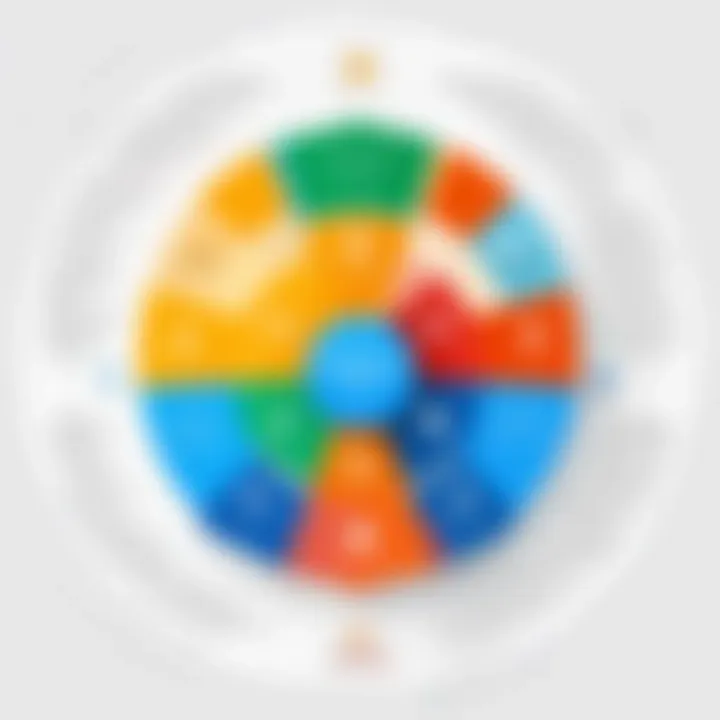
Defining Prototypes
A prototype is essentially a blueprint that defines shared properties and methods for a group of objects. When you create an object, it can automatically inherit from another object’s prototype. This means that if you call a method on an object, JavaScript first checks if that method exists directly on the object. If it doesn't, JavaScript looks at the prototype to see if it can find that method. This creates a linked structure called the prototype chain.
You can think of prototypes as a family tree where objects inherit traits from their ancestors. For instance, if you have a prototype called , and a object that extends from , the would inherit any methods defined on the prototype. This not only preserves consistency but also fosters an environment where changes made to the prototype can ripple through all derived objects, saving you countless hours in debugging.
The Importance of Prototypes
Understanding the importance of prototypes is vital for anyone learning JavaScript. They are not just a nice-to-have; they are a fundamental aspect of the language's design. By utilizing prototypes effectively, one can achieve:
- Efficiency: Reduces memory consumption by allowing multiple instances of objects to share methods rather than each instance maintaining its own copy.
- Flexibility: Modifying the prototype affects all objects linked to it, making it easier to implement updates or bug fixes at a single point.
- Enhanced Code Organization: Encourages a cleaner separation of functionality, establishing a clear hierarchy among different object types.
"Prototypes are JavaScript's way of enabling inheritance and organizing code in a logical manner, pivotal for effective object-oriented programming."
By grasping these concepts early on, students and budding developers can build a foundation that will assist them in tackling more complex scenarios later on. Prototypes illuminate the intricate dance of inheritance, allowing programmers to write cleaner, more efficient code, and ultimately debug with greater ease.
The Prototype Chain
The prototype chain is a fundamental concept in JavaScript that allows for inheritance and property sharing between objects. It's like a secretive road map; the more you understand its structure, the more effectively you can navigate the language's complexities. The prototype chain enables objects to access properties and methods from other objects, making your code not only more efficient but also cleaner and less repetitive.
Understanding the Hierarchy
At the core of the prototype chain lies the idea of a hierarchy. Each object in JavaScript has a prototype from which it can inherit properties and methods. When a property is accessed on an object, JavaScript first looks at that object itself. If it doesn't find the property there, it continues searching on the object's prototype, and then on that prototype's prototype, and so forth. This continues until it either finds the property or reaches the end of the chain, which is .
Here’s a simple analogy: think of it like a family tree. Your direct ancestors are your immediate family, but you also carry traits from grandparents and great-grandparents, branching further back.
Here's a clearer illustration:
In this example, is an instance of , inheriting the method from . This demonstrates the hierarchy in action—the instance is able to access methods defined on its prototype.
Behavior and Functionality
Understanding how the prototype chain behaves is crucial for effective programming in JavaScript. When dealing with prototype properties or methods, it’s important to note that any changes made to the prototype affect all instances.
- Efficiency: Since methods are stored in the prototype, they are shared among all instances. This means that instead of each object carrying its own copy of a method, they refer to a single location in memory. This conserves resources and improves performance, much like using a communal toolbox when fixing things instead of each person having their own.
- Dynamic Nature: The prototype chain's flexibility allows developers to modify or add properties and methods on the fly. Adding a method that’s accessible to all objects of a certain type can be done simply by adding it to the prototype. Here's an example:
With just this addition, every instance of can now use the method, showcasing the dynamic nature of the prototype chain.
However, it ’s crucial to navigate prototype manipulation with caution. Overwriting properties can lead to unexpected behavior, as you might inadvertently alter the behavior for all instances. The shared nature of prototypes can thus become a double-edged sword if not handled judiciously.
Remember: Changes to the prototype affect all instances sharing that prototype, so think carefully before implementing changes that could have wide-ranging impacts.
Creating Prototypes
Creating prototypes is at the very core of how JavaScript manages object behavior and inheritance. This section sheds light on how prototypes are not just a fancy term in the programming lexicon but an essential building block for structuring your JavaScript applications. The ability to create prototypes offers flexibility, encourages code reusability, and aligns with best practices in object-oriented programming.
Constructor Functions
At the crux of prototype creation lies the concept of constructor functions. These are special functions that you use to initialize new objects. Think of it as a blueprint for your objects. When you call a constructor function with the keyword, it creates a fresh object, and sets the value of inside the function to that new object.
Consider this basic example:
Here, is a constructor function. However, it’s not just a function; it serves as a prototype. You can add properties and methods to the prototype, making them accessible to every instance of that gets created. For example:
This method can be called on any instance created from , like so:
This approach helps in achieving code efficiency. You write the method once and use it across multiple instances. Hence, constructor functions facilitate the creation of objects while also enabling shared functionality through the prototype.
Object.create() Method
Another powerful way to create prototypes is through the method. Unlike constructor functions, which create a prototype chain by linking to another function, allows for direct creation of an object with a specific prototype object. This is particularly useful when you want to create a new object that inherits from an existing object but doesn’t need the overhead of a constructor function.
Here's how it works:
In this example, is a new object that inherits properties and methods from . This technique can be especially handy for setting up complex object hierarchies or when you want to establish a clear structure without the need for many constructor functions.
Utilizing not only provides a cleaner syntax but also enhances readability.
"Creating prototypes through constructor functions and the method paves a way for efficient, maintainable, and modular JavaScript code."
Prototype Properties and Methods
In JavaScript, understanding prototype properties and methods is paramount. The concept underscored in prototyping mirrors how inheritance works in real-world systems. Prototypes hold not just data but also behavior through their methods. This allows one to efficiently manage code and promote reusability. The focus on these components can significantly elevate the architecture of your JavaScript applications.
One key point about properties in prototypes is that they can be shared among instances of objects, reducing memory footprint. When you define a property in a prototype, any object created with that prototype will have access to it. This is crucial for incorporating shared behaviors or states without unnecessarily duplicating data within each object.
An additional consideration is how prototype methods help maintain clean and organized code. Instead of defining functions inside constructor calls, you can define methods directly on the prototype. This promotes a clear separation of concerns and enhances the readability of your code by keeping functionality tied closely to the objects it pertains to.
"By using prototypes effectively, you unlock the potential of JavaScript's object-oriented capabilities, allowing for efficient code management and simpler debugging processes."
Defining Properties
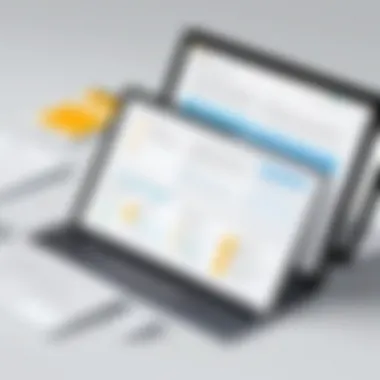
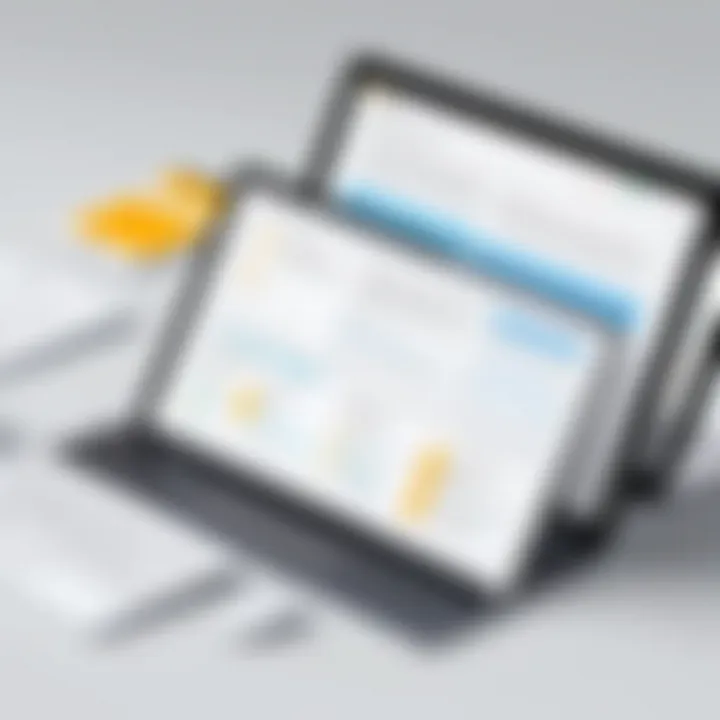
Defining properties on prototypes is easy in JavaScript, but knowing when and how to implement them is where the real skill lies. Adding properties to a prototype involves adjusting the constructor function or utilizing the method for direct assignments. For example, consider a scenario where you have a object:
This establishes a default value for that all instances will reference. If you create multiple cars, they won't each need to store this value—saving memory.
This practice leads to a more crystalline architecture. However, one must keep in mind that properties defined directly on the prototype can be overridden by instance properties. Thus, when managing object states, the layer of consistency in your architecture may sometimes be challenged.
Adding Methods to Prototypes
Adding methods to prototypes is a hallmark of JavaScript’s efficiency. Rather than having duplicated methods in every object, by placing functions on the prototype, you conserve memory and promote clarity.
Below is an illustrative example using the object:
Now, any car can utilize the method without bloating individual instances. You can call it like so:
Additionally, adding methods this way allows for easy modifications and extensions later on. For instance, if you wanted to enhance your honking method, changing it in one place automatically reflects in all instances:
This retains functionality across the board while keeping your code elegant. Remember, a cleaner and optimized codebase not only benefits immediate development but also enhances future maintainability.
Inheritance in JavaScript
Inheritance in JavaScript plays a crucial role in how objects relate to one another. It’s akin to a family tree where every object can inherit properties and methods from its predecessor. This construct not only fosters code reusability but also enhances maintainability, allowing developers to create a more dynamic and efficient coding environment.
Prototypal Inheritance Explained
At its core, prototypal inheritance is JavaScript's built-in mechanism for enabling one object to inherit from another. Unlike classical inheritance seen in other programming languages, which tends to adhere to rigid class structures, JavaScript operates through a more flexible prototype system. Every JavaScript object has a prototype, which is itself an object. This means when you attempt to access a property or a method that doesn’t exist on your current object, JavaScript checks the prototype chain until it finds it or concludes with a if it reaches the end.
To illustrate, imagine you have a parent object, , that has a method called . If you create a new object, , which inherits from , your object will automatically gain access to the method without the need to redefine it.
Here’s a simple code example:
This example shows the basic essence of prototypal inheritance, where inherits from . Understanding this mechanism is vital for developers as it lays the groundwork for crafting complex systems where objects communicate and share behaviors seamlessly.
Composition over Inheritance
While prototypal inheritance provides a straightforward approach to object reuse, it’s important to recognize the concept of composition in programming as a robust alternative. Composition emphasizes assembling objects from smaller, reusable components rather than inheriting from a single parent. This method can lead to more maintainable and flexible code, especially when working on large applications.
One of the main benefits of composition is that it allows for greater versatility when combining behaviors without being tied down by the constraints of hierarchy. For instance, suppose you have two objects, and . Instead of creating a deep hierarchy that combines both behaviors into a single class, you can simply mix and match them as needed:
- enables the ability to run.
- allows for barking behavior.
When you compose these objects together, you can produce various entities that share different combinations of functionality without the baggage of the inheritance chain.
In summary, both prototypal inheritance and composition have their place in JavaScript. While inheritance provides a useful way to extend objects, composition often yields a more scalable and manageable solution for extensive codebases. Developers should understand when to use each technique to maximize the efficiency and clarity of their code.
Common Misconceptions
Understanding prototypes in JavaScript often comes with a slew of misconceptions. These misunderstandings can lead to confusion and ineffective programming practices. It's essential to address these issues head-on to provide clarity and empower learners. Misconceptions not only hinder one’s grasp on the subject but can also perpetuate ineffective programming habits, which could lead to frustrating debugging sessions later on. By tackling these misunderstandings, we can foster an environment where developers of all skill levels can thrive.
Misunderstanding Object References
One prevalent misconception is the understanding of object references in JavaScript. Many learners believe that when they assign an object to another variable, they are creating a separate copy of that object. In reality, JavaScript handles objects by reference, not by value. This means when two variables refer to the same object, changes made through one variable will affect the other variable as well.
For example, consider this code:
In this case, becomes a reference to . When we update , it updates too since they are pointing to the same object in memory. This often leads to confusion, especially for those coming from languages that handle object copying differently.
Thus, it's important to emphasize that modifying an object via one reference affects all references to that object. This insight underpins many concepts in JavaScript, including manipulation of prototypes. Understanding object references is key to becoming proficient in JavaScript's object-oriented nature.
The 'this' Keyword in Prototypes
Another critical area of misunderstanding involves the 'this' keyword when working within prototypes. The usage of can feel perplexing because its value is determined by the context in which a function is called, rather than where it is defined.
For instance:
Here, refers to the instance of that was created (i.e., ). Many beginners mistakenly believe that will always point to the object where the function resides. This leads to confusion, especially when using callback functions or when the method is detached from the instance.
In the case of detached methods:
In this situation, no longer points to , as is called in a different context, causing it to be undefined. To cope with this, one could use functions like or arrow functions which have a different handling of .
Understanding the nuances of is vital for effective prototype usage in JavaScript. Learning how context impacts the binding is crucial for novices and can greatly improve code quality and function functionality.
Key Insight: Always be mindful of the context in which a function is called to predict the value of . It can save a lot of head-scratching in the long run.
Performance Considerations
When diving into JavaScript prototypes, it's crucial not to overlook performance considerations. The way prototypes are utilized can significantly impact both memory usage and efficiency. Understanding these elements equips developers with the tools to write not only functional but also performant code. With modern JavaScript applications growing in complexity, the importance of keenly observing these factors cannot be overstated.
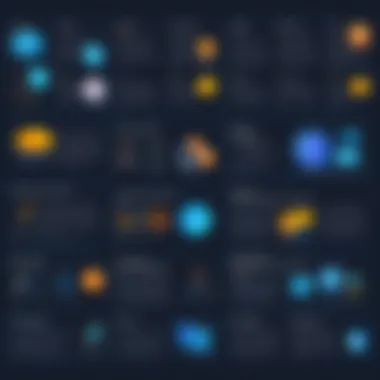
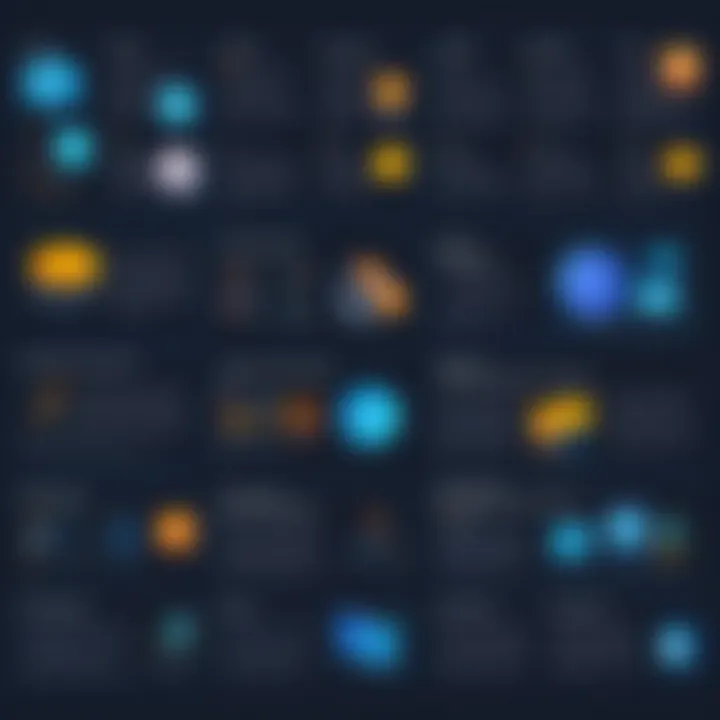
Memory Usage and Efficiency
Memory management is a key player in software development. Particularly in JavaScript, the use of prototypes allows for a more efficient way of handling memory when creating objects. Unlike creating multiple instances of an object with the same methods, prototyping enables a single method to be shared across all instances. This drastically reduces the memory footprint.
For instance, consider a scenario where you’re building a large-scale application that involves numerous objects with similar behaviors. If each object has its own copy of methods, memory can be exhausted pretty quickly. Using prototypes, however, means that each instance references the same method from its prototype, saving heaps of memory. Here’s a quick breakdown of how this works:
- Reduced Memory Footprint: Storing methods on the prototype eliminates the need for redundancy across instances.
- Faster Object Creation: New objects can be created faster, as they inherit properties and methods directly from the prototype.
- Easy Maintenance: Changes made to the prototype will automatically reflect in all instances, making updates more efficient.
Prototype Pollution Risks
Despite the benefits, embracing prototypes comes with its own set of risks—one of which is prototype pollution. This vulnerability occurs when an attacker manipulates an object’s prototype, allowing them to inject properties and methods that shouldn't be accessible or modifiable. This situation can lead to unexpected behavior and potential security flaws.
Here are some key points to keep in mind about prototype pollution:
- Increased Attack Surface: When properties are added or altered on the prototype, it opens doors for unintended consequences, leading to instability.
- Data Leakage: Sensitive data may become accessible if proper checks aren’t in place to limit what can be added or modified in prototypes.
- Difficult Debugging: Tracing issues back to prototype modifications can be tricky, making debugging a real challenge.
"Understanding the balance between utilizing prototypes for efficiency and guarding against risks can greatly enhance code quality and security."
To mitigate these risks, it's essential to implement strict control mechanisms and validation checks when working with objects and their prototypes. Here’s how you can protect your code:
- Use : This can prevent the addition of new properties to an object, effectively locking it down.
- Object.assign or Object.create: Utilize these methods with care to ensure that you’re not unwittingly exposing your prototypes to pollution.
- Linting Tools: Employ tools to catch potential vulnerabilities before they find their way into production.
In summary, the careful utilization of prototypes can lead to significant performance gains regarding memory efficiency. However, it's equally critical to stay alert to the pitfalls posed by prototype pollution. By addressing these aspects, you can craft high-quality JavaScript applications that are both effective and secure.
Best Practices for Utilizing Prototypes
In the realm of JavaScript, embracing the art of prototypes can augment one’s programming skills dramatically. Understanding how to optimize the use of prototypes not only boosts performance but also contributes to cleaner, more maintainable code. This section will dissect various best practices that every programmer should adopt to wield prototypes proficiently.
Avoiding Constructor Noise
Constructor noise refers to the clutter that can accumulate in the constructor functions when properties and methods are defined directly within them. Each time you create a new instance, the constructor gets loaded with all its properties and methods, which can lead to inefficient memory use. This practice can become problematic especially when dealing with a large number of instances, potentially slowing down the entire application.
To mitigate this, leveraging prototypes to define methods rather than having them inside the constructor is crucial. Here’s a simple example:
By moving the method to the prototype, all instances of share the same method instead of having it recreated for each individual dog.
By centralizing method definitions, you reduce memory consumption and enhance code clarity.
Moreover, maintaining a leaner constructor function allows for better organization. It becomes easier to track what properties the constructor is responsible for, helping future developers (or future you) grasp the logic without wading through methodological clutter.
Inheriting Safely
Inheriting safely emphasizes the idea that not all inheritance patterns are beneficial or relevant in every situation. JavaScript, with its prototypal inheritance, can lead to potential pitfalls if not handled properly. When extending objects, it's essential to ensure that inherited properties do not lead to unpredictable behaviors, which can occur if any side effects from the parent object's methods leak into child objects.
To safely inherit, consider using Object.create() which creates a new object with a specified prototype. This helps in crafting a clear inheritance structure. An illustration would look like this:
This approach keeps the method streamlined and prevents unintended method overrides. It is also fundamental to understand the limitations of inheritance. Rather than creating a deep hierarchy, sometimes opting for composition over inheritance brings more flexibility to your design, allowing objects to be mixed and matched without suffering the pitfalls of a rigid inheritance structure.
Practical Examples
When it comes to the practical application of JavaScript prototypes, there’s much to gain from understanding how they operate under the hood. Practical Examples not only reinforce the theory behind prototypes but also illustrate their real-world utility. Through examples, students and budding programmers can see the concrete benefits of leveraging prototypes in their code. This section is vital as it bridges the gap between theoretical knowledge and hands-on coding experience.
One key benefit of practical examples is problem-solving. By observing how prototypes can be used to create custom objects, or how they function within widely-used libraries, learners can develop a deeper grasp of how JavaScript is structured. Moreover, practical applications foster an instinctive feel for optimal coding practices, allowing programmers to write cleaner, more efficient code.
Creating a Custom Object
To create a custom object in JavaScript using prototypes, one can define a constructor function. This function acts as a blueprint for the objects created from it. For instance, let’s consider a simple example where we want to create a custom object. Here’s how you might go about it:
In the above example, we defined a constructor function that sets up the and properties. The method is added to the prototype, allowing all instances of to share this functionality. Not only does this conserve memory, but it also enforces consistency across similar objects.
Using Prototypes in Libraries
Another major application of prototypes is their use in JavaScript libraries. A well-known example is jQuery, which relies heavily on prototype-based inheritance. By using prototypes, jQuery extends built-in objects like and , adding methods that enhance functionality. This approach leads to cleaner, more maintainable code.
For example, jQuery has a method called that applies a function to each item in an array. Here's a quick look:
This way of extending base objects allows programmers to use additional capabilities without modifying the originals, thus maintaining stability and predictability in their code. It's worth noting how using prototypes in libraries promotes a more modular style of programming, enabling the reuse of code and functionalities across different projects.
Leveraging prototypes effectively transforms how objects behave in JavaScript, leading to more intuitive and maintainable code.
By delving into practical examples, programmers are better equipped to apply the principles of prototypes in their own work. As seen, understanding prototypes clarifies not just how to create objects, but how to enhance them efficiently, paving the way for skillful programming.
Culmination
Understanding the nuances of prototypes in JavaScript is no small feat, yet it's a crucial part of mastering the language. Prototypes serve as the backbone of JavaScript's inheritance model, allowing for the sharing of methods and properties among various objects without consuming excessive memory. This efficiently designed system can significantly optimize performance in larger applications, allowing developers to write cleaner, more maintainable code.
Recap of Key Points
As we wrap up this exploration of JavaScript prototypes, let's revisit some fundamental ideas:
- Prototypes are foundational: They form the basis for inheritance, allowing objects to share properties and methods seamlessly. This takes away the redundancy of creating multiple copies of the same properties.
- The prototype chain: Each object carries a reference to its prototype, leading to a chain of prototypes that can affect property and method lookup, enabling developers to create complex hierarchies.
- Performance matters: Understanding memory usage and prototype pollution is essential for writing efficient code. Carelessly adding properties to prototypes can lead to significant memory consumption in large applications.
- Practical application is key: Using prototypes effectively in libraries and custom objects can enhance functionality and code organization.
"To master any programming language, one must first understand its core principles." This sentiment rings especially true for JavaScript's prototype system.
Encouragement for Further Learning
Prototypes are just one piece of the JavaScript puzzle. As you delve deeper into the language, consider exploring associated topics:
- Asynchronous JavaScript: Understand how to handle asynchronous operations effectively.
- Functional programming concepts: Many of these ideas tie back into the principles of JavaScript, shaping your understanding of the language.
- Explore ES6 features: New syntax and enhancements continue to evolve JavaScript, promising even greater capabilities.
Books, online courses, and forums like reddit.com are valuable resources for furthering your understanding. Don't hesitate to seek out community support as you advance through more complex aspects of JavaScript. This journey is just beginning—embrace it!