Mastering JavaScript: A Detailed Exploration
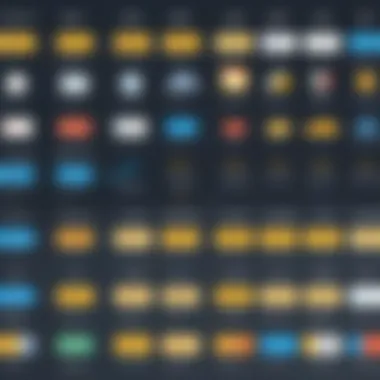
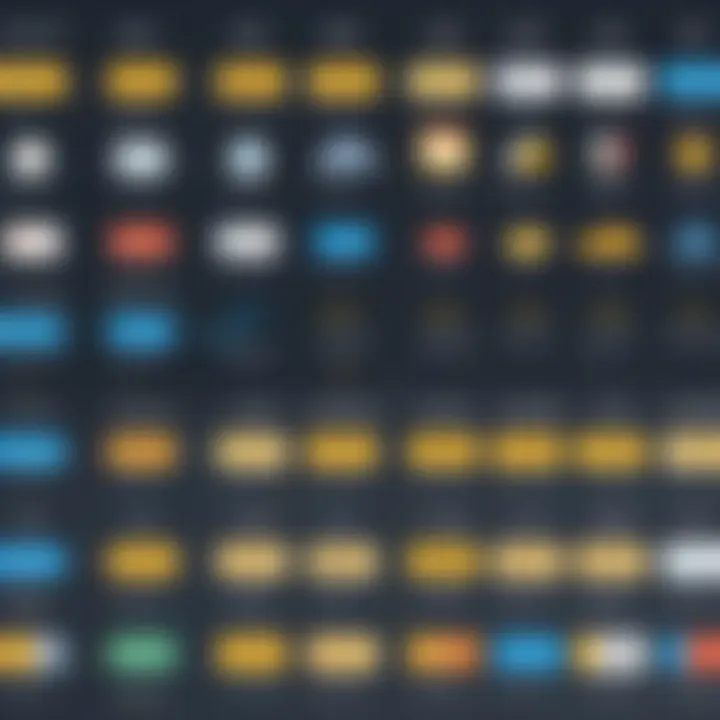
Intro
JavaScript, often simply referred to as JS, has become an integral part of the modern web landscape. It empowers users to create interactive websites, build web applications, and even develop server-side solutions. But, how did it all begin? To fully grasp JavaScript’s impact, it’s crucial to take a step back and explore its roots, key attributes, and the vast applications it nurtures.
History and Background
JavaScript was born in the mid-nineties, a time when the internet was still in its infancy. Developed by Brendan Eich while he was at Netscape Communications Corporation, the language was initially called Mocha, then renamed to LiveScript before finally becoming JavaScript. Its inception happened around 1995, coinciding with a growing need for client-side processing. Rather than relying solely on server-side technology, web developers needed a way to enhance user experience directly within the browser.
As its usage grew, standards were established. The European Computer Manufacturers Association (ECMA) standardized the language in 1997, which led to the development of ECMAScript. This standardization allowed for uniform implementation across different browsers. Fast forward to today, JavaScript has evolved far beyond its original conception, thanks to frameworks and libraries like React, Angular, and Node.js.
Features and Uses
JavaScript is lauded for its versatility and ease of use. Here are some notable features:
- Dynamic and weakly typed: Variables in JS can hold various data types, which gives developers flexibility.
- Event-driven programming: This allows for asynchronous programming, making it suitable for interactive websites.
- Prototype-based: JavaScript uses prototypes for inheritance, a departure from classical inheritance seen in languages like Java.
- Cross-platform capabilities: Developers can build applications that run across different devices and platforms.
The uses of JavaScript are ubiquitous, ranging from adding interactive elements to web pages, creating mobile applications, to powering server-side technologies with Node.js.
Popularity and Scope
Today, JavaScript holds the title as one of the most popular programming languages in the world. According to Stack Overflow’s Developer Survey, it consistently ranks among the top languages that developers use and love. This popularity is driven by a variety of factors:
- Community support: A vast community surrounds JavaScript, fostering growth through open-source projects and libraries.
- Continuous updates: Regular updates to the ECMAScript language ensure JavaScript doesn’t stagnate, adapting to modern development needs.
- Job opportunities: With its rising demand, there’s no shortage of career paths for developers proficient in JavaScript.
With such a strong presence in the programming community, JavaScript continues to shape the future of web development, making it imperative for learners to understand its nuances. Whether you’re just starting your programming journey or looking to solidify your skills, this exploration delves into the essential concepts and practices that will guide you in mastering JavaScript.
Preamble to JavaScript
JavaScript sits at the core of modern web development, acting as the linchpin between interactive designs and robust functionality. Knowing its underpinnings is fundamental for anyone aspiring to navigate the landscape of programming. As you delve into JavaScript, you don't just learn a language; you unearth a versatile toolkit that shapes the very fabric of web experiences.
Historical Background
JavaScript made its debut in 1995, created by Brendan Eich while he was a part of Netscape Communications. Initially known as Mocha, it morphed into LiveScript before settling on the name we know today. At that time, web pages were static, lacking the dynamism that users have come to expect. JavaScript emerged as a answer to this need, allowing developers to craft functionalities that would enhance usability and engagement.
Its rise can be attributed to its ease of learning and immediate applicability. Independent web developers adopted it quickly, transforming the web from a simple collection of pages to a rich interactive platform. Over the years, JavaScript has seen monumental growth, influencing and being influenced by the emergence of frameworks and the ever-evolving needs of developers.
JavaScript vs. Other Languages
When you throw JavaScript into the mix alongside other programming languages, it’s not exactly an apples-to-apples comparison. For instance, compared to Python, which is often lauded for its readability, JavaScript tends to be a bit more chaotic due to its flexible nature. You can write clean, structured JavaScript; however, it can also descend into a quagmire of complexity if not handled properly.
Java and C# embrace a more structured approach with strong typing, whereas JavaScript opts for a more fluid property, allowing types to change dynamically. This might make it seem daunting for newcomers, but it also opens the door for rapid development and prototyping.
JavaScript has evolved into a mecca for asynchronous programming, allowing it to efficiently handle tasks like data fetching or user input without slowing down the entire application. This is a significant advantage when you compare it to traditional languages that block execution until a task is completed.
- JavaScript is often embraced for front-end development with frameworks such as React, Angular, and Vue.js that enhance the user experience.
- It boasts vibrant community support and a plethora of resources, making it approachable for new developers.
"The difference between JavaScript and other programming languages can be likened to the difference between a Swiss Army knife and a single instrument; versatile yet potentially overwhelming."
In essence, while JavaScript may present unique challenges, the rewards of mastering it—such as creating responsive web applications—make it extremely worth the effort.
Key Features of JavaScript
JavaScript stands out in the programming landscape due to its unique features that not only define its functionality but also significantly enhance the overall programming experience. Understanding these key features is essential for anyone delving into the world of JavaScript, as they form the backbone of how the language operates and interacts with various environments, particularly web browsers. These features not only empower developers to create dynamic web applications but also allow for flexibility and creativity in coding solutions.
Dynamic Typing
One of the most striking characteristics of JavaScript is its dynamic typing feature. In many programming languages, data types must be explicitly defined, which can sometimes hinder the pace of development. With JavaScript, variables can be declared without a predefined type, allowing developers to change the type of variable as per the requirements of their code.
For instance, consider a simple snippet:
This flexibility can speed up the development process significantly. However, it’s not all rainbows and butterflies. Dynamic typing can lead to unexpected bugs when a variable is unexpectedly changed or misused later in the program. It requires developers to be vigilant and sometimes results in debugging challenges. Therefore, while dynamic typing is beneficial, the responsibility lies heavily on the developer to manage types carefully.
First-Class Functions
JavaScript treats functions as first-class citizens, which means they can be treated just like any other object. This is a powerful concept that allows functions to be assigned to variables, passed as arguments, and even returned from other functions. This capability enables a range of programming paradigms including functional programming, callbacks, and higher-order functions.
To illustrate, let’s take a look at this snippet:
In the example above, is a function that generates a greeting message. It can be passed to another function, , demonstrating the first-class nature of functions within JavaScript. This enhances modularity and reusability in code, contributing to cleaner and more maintainable scripts.
Prototype-Based OOP
Unlike many languages that use class-based object-oriented programming (OOP), JavaScript employs a prototype-based approach. This means objects can inherit directly from other objects. Each object has a prototype, which is itself an object, and it can share properties and methods from its prototype. This flexibility enables developers to create rich and intricate structures while avoiding some of the pitfalls associated with classical inheritance.
For example:
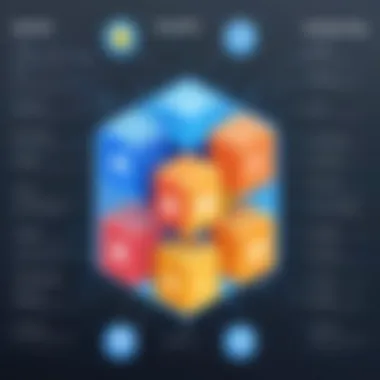
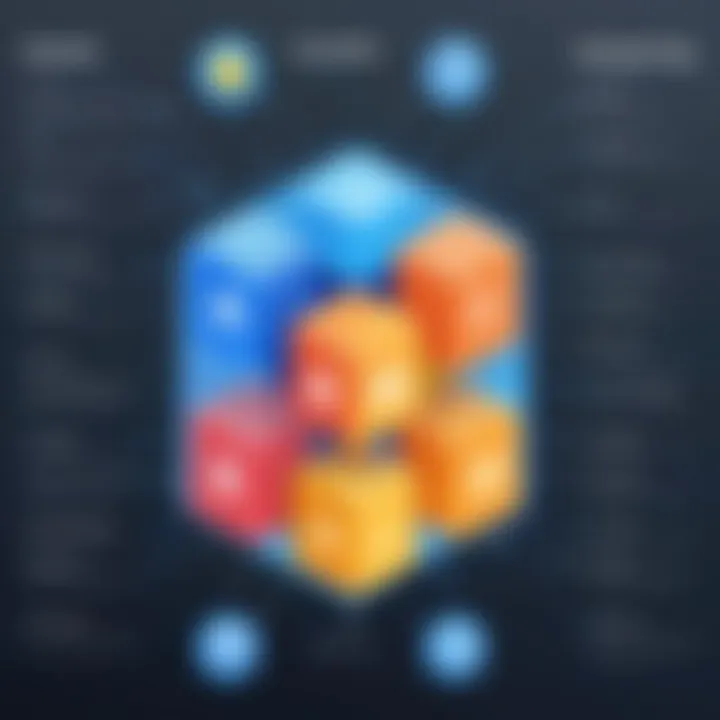
In this case, the function is a constructor. By adding methods to , we allow any instance of to access those methods. This prototype-based structure is not just syntactically simpler but also provides memory efficiency by sharing methods across instances, instead of creating multiple copies.
By harnessing these key features, JavaScript developers can leverage the language's capabilities to craft responsive and efficient web applications. Understanding dynamic typing, first-class functions, and prototype-based object-oriented programming is pivotal for mastering the art of JavaScript.
In summary, the features discussed play a significant role in making JavaScript a versatile and powerful language. Recognizing their implications helps learners navigate the complexities of programming in JavaScript while equipping them with the tools necessary for effective web development.
The Role of JavaScript in Web Development
JavaScript plays a pivotal role in modern web development. It’s as central to websites today as bread is to a sandwich. In simple terms, JavaScript enables interactive capabilities on web pages, which is crucial for enhancing user experience. Without it, web pages would be static, and users would find themselves clicking through dull, unresponsive content. In this section, we’ll explore the various facets of JavaScript within web development, including its primary applications, benefits, and key considerations.
Client-Side Scripting
Client-side scripting is where JavaScript shines bright. It allows code to execute in the user’s web browser rather than on a server, leading to quicker interactions. For instance, think about how online forms behave—when you enter your details and click submit, JavaScript can validate that information instantly without needing to communicate with a server and wait for a response.
Here’s a quick rundown of the advantages of client-side scripting:
- Faster Feedback: Immediate responses to user actions.
- Reduced Server Load: Less data sent back and forth means the server has to do less work.
- Improved User Experience: A smoother interface makes navigating the web more manageable and enjoyable.
Asynchronous Programming
Asynchronous programming in JavaScript is a game changer. Traditional programming flows in a linear way, causing the web to become unresponsive when waiting for data retrieval. Here, JavaScript’s ability to handle asynchronous tasks comes into play. Techniques like AJAX (Asynchronous JavaScript and XML) or Fetch API allow developers to load data in the background without disrupting the user experience.
For example, when you search for something on a website, JavaScript can fetch results and update the page dynamically, eliminating the need for a full page refresh. Advantages include:
- Enhanced Performance: Seamless updates make applications feel quicker.
- Better Resource Management: Only the data required is fetched, minimizing bandwidth usage.
Frameworks and Libraries
In the ever-evolving landscape of web development, JavaScript frameworks and libraries have emerged to simplify the development process. They’re like a toolbox packed with ready-made solutions, allowing developers to avoid reinventing the wheel. Let’s break down a few prominent ones.
React
React, developed by Facebook, has gained a massive following due to its component-based architecture. It allows developers to break down complex user interfaces into small, reusable components. This modularity is a major advantage, facilitating easier maintenance and scalability of applications.
Also noteworthy is React’s virtual DOM, which optimizes rendering processes, leading to faster updates and efficient performance. However, while React is popular, it often comes with a steep learning curve for beginners.
Angular
Angular, on the other hand, is a robust framework endorsed by Google. It provides a comprehensive solution for building dynamic web applications. One of its key features is two-way data binding, meaning that any changes in the user interface immediately reflect in the application data and vice versa. This responsive behavior allows for fluid user experiences.
Nevertheless, Angular can sometimes feel overwhelming due to its extensive feature set and intricate syntax, making it less accessible for those just dipping their toes into web development.
Vue.js
Lastly, we have Vue.js, known for its ease of integration and progressive nature. Developers can slowly adopt Vue.js into their projects without needing a complete overhaul of existing code. Its lightweight and flexible design is appealing, especially for creating smaller applications or parts of larger ones.
Vue.js encourages readability and maintainability in code, but it does not have as large a community or as many third-party resources compared to React and Angular.
"Choosing the right framework can significantly impact your development speed and the performance of your application"
As we traverse through JavaScript’s realm in web development, it's clear that it isn’t just a language but a powerful tool, shaping how users interact with the web. Its various applications—from client-side scripting to asynchronous programming, and the utilization of frameworks—make it an essential component for modern developers.
Understanding the JavaScript Syntax
JavaScript syntax is the set of rules that define a correctly structured JavaScript program. Like the rules of grammar in a language, syntax is crucial in programming as it dictates how programmers write the language to ensure that their code executes as desired. Understanding JavaScript syntax is not merely about learning how to write code; it opens the doors to debugging, improving readability, and enhancing collaboration. In a world where a single misplaced character can derail an entire project, mastering these components is non-negotiable for developers.
Variables and Data Types
Variables in JavaScript serve as containers for storing data. Think of them as labeled boxes where you can keep your items in an organized manner. The types of values that can be held by these variables range widely, from numbers and strings to more complex structures like arrays and objects. Familiarizing oneself with data types is essential since JavaScript uses dynamic typing, which allows variables to hold different types of data at different times.
Underneath the surface, understanding what type of data your variable holds can influence how you manipulate that variable. For instance, you can't simply concatenate a string to an integer without converting one or the other. This flexibility can be advantageous, but it can also be a double-edged sword if you don’t pay attention to the data types you are working with.
Control Structures
Control structures are fundamental in directing the flow of your JavaScript code. They allow you to make decisions and execute different blocks of code based on certain conditions. There are two primary forms of control structures in JavaScript: If-Else Statements and Loops.
If-Else Statements
If-Else statements serve as the decision-makers in your code, akin to navigating through a maze with forks in the path. When faced with a condition, these statements give you the power to specify different actions. The simplicity of their design is what makes them a popular choice in many scenarios.
- Key Characteristics: The clear structure of If-Else statements allows for easy readability, making it simpler for developers to understand what conditions lead to what outcomes.
- Unique Feature: You can chain multiple conditions together using , enhancing the complexity of your decision-making flow.
- Advantages: The ability to articulate different paths based on varying conditions offers unparalleled flexibility. Conversely, if an If-Else chain becomes too lengthy, it can lead to messy code that is difficult to manage and read.
Loops
Loops are designed to execute a block of code repeatedly until a specified condition is met. They are particularly useful when you need to perform the same action multiple times, like processing items in a list. The most common types of loops in JavaScript are the loop and loop, akin to a runner completing laps around a track until told to stop.
- Key Characteristics: One of the most significant features of loops is their iterative nature, which leads to less redundancy in your code and increases efficiency.
- Unique Feature: Loops can also be combined with control structures for enhanced functionality, allowing you to execute them based on conditions.
- Advantages: The undeniable simplicity and efficiency of loops can, however, introduce complexity if you’re not careful. An uncontrolled loop, or an infinite loop, can halt or crash your program. Careful management of loop conditions is essential to prevent this.
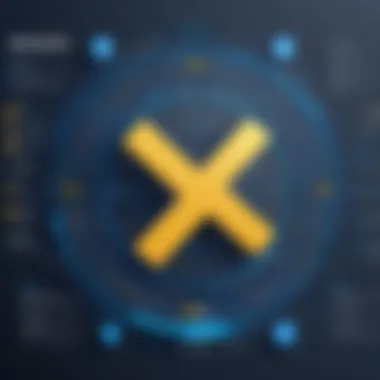
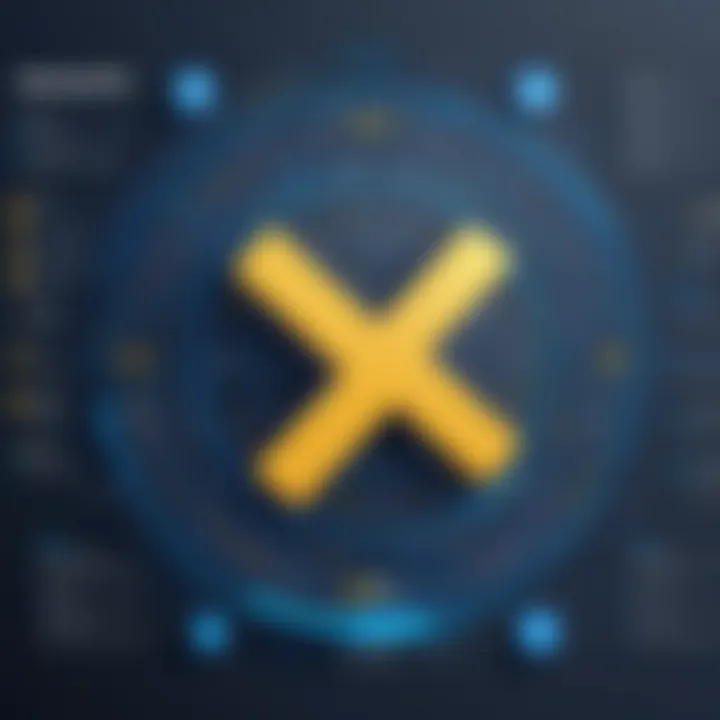
Functions and Scope
Functions in JavaScript encapsulate blocks of code and allow for their reuse throughout your scripts, facilitating modular programming. When you define a function, you’re essentially teaching your program a new skill.
Moreover, understanding scope—how variables are accessed within functions or blocks of code—is vital. JavaScript uses function scope for variables defined within functions, while variables declared outside are typically accessible throughout your script. This understanding can assist developers in managing variable lifespan and prevent unwanted behavior in more complex applications.
Mastering JavaScript syntax equips you with the foundational tools required for proficient coding, serving not only as a launchpad for your programming journey but also as a bastion against common pitfalls.
Practical Applications of JavaScript
JavaScript plays a pivotal role in the programming landscape, especially when it comes to practical applications. It's not just a bunch of syntax or theoretical constructs; it's a language that breathes life into websites and applications, making them interactive and user-friendly. For students and learners venturing into the realm of programming, understanding these practical applications is crucial. They illuminate how JavaScript translates to real-world scenarios and why it's indispensable in web development.
Building Interactive Websites
In today's digital age, an interactive website is no longer a luxury. It's a standard expectation. Users today are looking for more than just static pages. They want an engaging experience, and JavaScript is the heart that pumps that engagement.
With JavaScript, developers can introduce dynamic content, animations, and real-time updates without having to reload an entire webpage. Ever wondered how a comment section updates without refreshing? That's done using JavaScript’s powerful capabilities. Here’s why building interactive websites with JavaScript is so significant:
- Enhanced User Experience: JavaScript can create a seamless flow, allowing users to interact with the content. For instance, users can fill forms, navigate galleries, or play videos without interruptions.
- Responsive Designs: Websites can adapt to different screen sizes and orientations. Using JavaScript in conjunction with CSS, developers can ensure a site looks great on both mobile and desktop.
- Event Handling: Whether it's clicking a button or scrolling a page, JavaScript can respond to user actions in real-time. This immediate feedback is crucial in fostering user engagement.
"An interactive website isn't just a trend; it's a necessity in capturing user attention and providing value."
Developing Web Applications
As we move deeper into the era of web applications, JavaScript emerges as a key player in developing robust, functional applications that run on browsers. Unlike traditional software that requires installation, web applications can be accessed from any device with an internet connection. The importance of JavaScript in this domain cannot be overstated:
- Single Page Applications (SPAs): Frameworks such as React and Angular allow developers to create SPAs that load a single HTML page and dynamically modify it without page reloads. This results in an efficient, app-like experience.
- Real-Time Applications: JavaScript enables developing applications that need real-time data, like messaging platforms or collaborative editing tools. With libraries like Socket.IO, developers can manage real-time events with ease.
- Cross-Platform Development: With the advent of Node.js, it’s possible to use JavaScript on the server side as well. This means developers can create full-stack applications using one language, simplifying the development process.
Utilizing APIs
Application Programming Interfaces (APIs) are the bread and butter of modern web development. They enable different systems to communicate with each other effectively. JavaScript’s role in utilizing APIs can’t be overlooked:
- Data Fetching: JavaScript can make requests to external APIs to fetch data asynchronously, meaning web applications can maintain interactivity while gathering information. For instance, pulling in user data from platforms like Facebook or Twitter enriches the user experience.
- Integration of Services: Developers can easily integrate third-party services, like payment gateways or mapping services, using JavaScript. This capability is instrumental in developing feature-rich applications.
- Dynamic Content: Using APIs, data can be loaded on demand. This removes the need to load entire datasets initially. Instead, data is fetched as needed, enhancing performance and user experience.
JavaScript, when woven into the fabric of applications, is what transforms the mundane into the remarkable. The implications of its practical applications are vast, and for those venturing into the programming realm, it’s essential to recognize its significance in shaping not just websites, but the very future of web-related technologies.
Advanced Concepts in JavaScript
Diving into the advanced concepts of JavaScript can feel like peeling an onion. Each layer reveals something deeper about the language, imparting a richer understanding for developers. This section is crucial because it bridges the gap between foundational knowledge and more sophisticated programming techniques. Grasping these concepts allows for more efficient code, enhances performance, and encourages a more elegant approach to solving problems. These elements aren't just academic; they are real-world skills that shape how developers interact with JavaScript to build complex applications.
Closures
Closures in JavaScript can be seen as a unique way that functions manage their scope. When you create a function inside another function, the inner function gains access to variables in its parent function, even after the outer function has finished executing. This behavior can sometimes seem a bit like magic.
For example, if you have a function that returns another function:
Here, is not accessible directly from outside , but the inner function can still manipulate it. This makes closures invaluable for data hiding and encapsulation. It's a handy pattern for creating private variables in JavaScript, promoting better modular programming.
Promises and Async/Await
Promises represent a critical evolution in handling asynchronous operations in JavaScript. They relieve the burden of callback hell, which happens when too many nested callbacks make code hard to read and maintain. A promise can hold a value that may be available now, or in the future, or never. It can be in one of three states: pending, fulfilled, or rejected.
Consider a simple promise:
This approach promotes cleaner code by separating the handling of success and failure conditions. Furthermore, async/await syntax builds on promises. It allows asynchronous code to be written in a way that resembles synchronous code, making it much easier to read and manage errors. By using , you can pause execution until the promise settles:
ES6 Features
ECMAScript 6, or ES6, introduced several game-changing features that modernized JavaScript. Among these are arrow functions and template literals, which not only streamline the syntax but also improve the functionality of the language.
Arrow Functions
Arrow functions provide a concise syntax for writing function expressions. They help eliminate the need for the keyword and do not bind their own , which can be extremely handy in many situations. Consider this example:
The above example is not just shorter; it also keeps the context from the surrounding lexical scope, thus avoiding common pitfalls associated with traditional functions where can change.
One might wonder why developers find them such a beneficial choice. Because they reduce verbosity without sacrificing clarity, they quickly became popular in app construction — especially where inline functions are needed.
Template Literals
Template literals, on the other hand, revolutionize string handling in JavaScript. They allow embedding expressions within string literals, making it easier to create complex strings without the tedious concatenation:
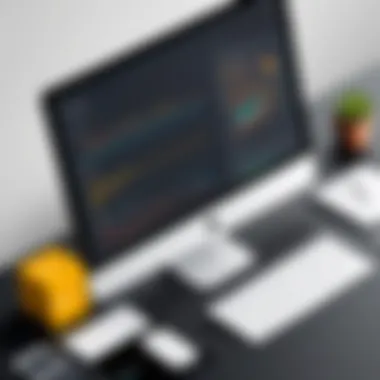
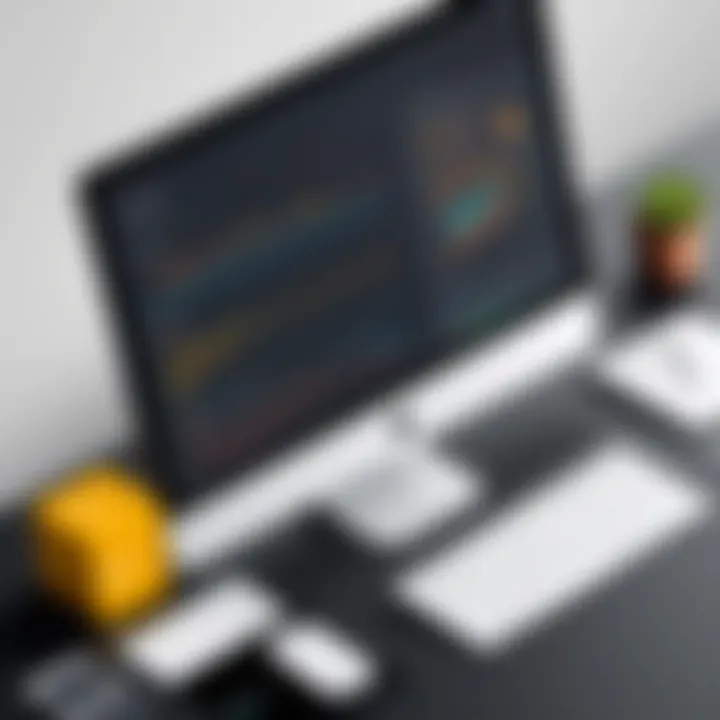
This feature notably improves readability and maintainability. Additionally, template literals support multi-line strings without the worry of adding line breaks or using the concatenation operator.
In summary, both these features enhance the development experience. They provide more readable, concise, and maintainable code, which is a cornerstone of modern JavaScript development.
Best Practices for JavaScript Development
In the ever-evolving world of web development, the significance of adopting best practices in JavaScript cannot be overstated. These principles not only enhance the quality of the code but also improve the productivity of developers, making it essential knowledge for anyone delving into the language. By adhering to best practices, one can ensure maintainability, readability, and ultimately a smoother development process.
Code Organization
Keeping your code organized is like keeping a tidy workspace. When your code is structured well, you can easily navigate, debug, and extend it in the future. This involves more than just pretty formatting; it’s about maintaining a clear hierarchy and using consistent naming conventions. A solid approach is to adopt a modular structure where each module or component has a specific function.
- Use descriptive names: Rather than naming variables or , use names that indicate their purpose. It not only helps with readability but makes it easier for others (or future you) to understand what the code is doing at a glance.
- Break it down: Divide large code files into smaller, manageable pieces. This can involve separating functions into different files or using object-oriented principles to encapsulate related functionality.
Additionally, leveraging tools like ES6 modules can help in bundling and organizing code effectively, making it modular without losing clarity.
Debugging Strategies
Even the best developers encounter bugs. The key lies in how you deal with them. Debugging can be painstaking, yet it is an invaluable skill for any JavaScript developer. Here are effective strategies to streamline that process:
- Use the Console: The built-in function is your best friend. It allows you to inspect variable values and program flow right from the browser's console.
- Leverage Debugging Tools: Modern browsers come equipped with powerful debugging tools. For instance, Chrome DevTools provides a suite of features such as breakpoints, call stacks, and comprehensible logging which can provide invaluable insights into how your code is executing.
- Stay Systematic: Isolate problems by commenting out sections of code and re-evaluating functionality incrementally. This approach often reveals the root cause by eliminating possibilities one by one.
"The act of debugging is akin to detective work; methodically unravel the threads until the truth is revealed."
Performance Optimization
JavaScript can often be criticized for performance, especially in large applications. However, knowing how to optimize code can make a significant difference in user experience. Here are some fundamental practices:
- Minimize DOM Manipulations: Direct interaction with the DOM can be costly in terms of performance. Whenever possible, cache DOM elements, batch updates, or utilize frameworks that employ virtual DOM strategies like React.
- Avoid Memory Leaks: Keeping your memory usage in check is crucial. Leaks can occur when references to outdated objects linger in memory unnecessarily. Regularly freeing up resources and utilizing tools to analyze memory can help keep your application smooth.
- Load Scripts Asynchronously: Loading scripts asynchronously can enhance page load speeds and execution times, improving user experience.
By integrating these best practices into JavaScript development, you are not just writing code, but creating a robust foundation for scalable and maintainable applications. This, in turn, will result in smoother development processes and enhance the enduring quality of your work.
JavaScript in the Era of Modern Development
JavaScript has evolved into a vital element of contemporary software development, extending far beyond its humble beginnings as a client-side scripting language. The significance of JavaScript in today's landscape cannot be understated. Its ability to create dynamic, interactive user experiences has made it essential for web development. But it doesn't stop there. The emergence of frameworks and libraries offers developers the tools needed to construct complex applications more efficiently. Additionally, JavaScript is no longer confined to the browser—it's making waves on the server-side as well, thanks to technologies like Node.js.
In understanding JavaScript’s modern relevance, especially for students and aspiring developers, it’s crucial to grasp several key components that highlight its versatility and broad application.
"JavaScript is the only programming language that can run in the browser and server seamlessly, unlocking a new frontier for developers."
The Rise of JavaScript Frameworks
Frameworks such as React, Angular, and Vue.js have transformed how developers approach web application development. These frameworks provide structured environments that enhance productivity and facilitate the implementation of best practices.
- React: Its component-based architecture allows for reusable UI components, making it easier to manage large applications.
- Angular: Favored for its powerful data binding capabilities and built-in solutions, Angular is an all-encompassing framework.
- Vue.js: Known for its simplicity, Vue.js allows developers to incrementally adopt its functionality, making it an attractive option for both small and large projects.
The impact of these frameworks has been profound; they streamline development processes and improve code maintainability. More than just tools, they have spurred entire communities of developers, fostering collaboration and shared knowledge.
JavaScript and Server-Side Rendering
Server-side rendering (SSR) has also gained traction in recent years. Unlike traditional client-side rendering, which loads a blank HTML page and populates it subsequently with JavaScript, SSR sends a fully rendered page to the client. This approach enhances performance and improves SEO.
Frameworks like Next.js integrate SSR capabilities, offering developers the dual advantage of a robust framework along with enhanced page load speeds and better search engine visibility. Allowing search engines to easily crawl pages improves the likelihood of better rankings, which is crucial in an increasingly competitive web.
The Future of JavaScript
Looking ahead, the trajectory of JavaScript seems destined for further evolution, embracing emerging technologies and methodologies.
Here are a few areas where we can expect growth:
- TypeScript Adoption: With the growing complexity of applications, TypeScript—JavaScript's statically typed sibling—has gained popularity for its error-reducing features.
- WebAssembly: This technology aims to enhance web applications' performance by enabling languages like C++ and Rust to run in the browser, potentially changing the interaction model between JavaScript and other programming languages.
- Artificial Intelligence and Machine Learning: As AI becomes increasingly important, JavaScript libraries geared towards these areas, such as TensorFlow.js, are likely to see greater use.
These advancements not only promise to make JavaScript a more powerful language but also ensure that it remains relevant and indispensable in the developer toolkit.
In summary, the prevailing trends indicate that JavaScript will continue to shape the future of software development, providing vast opportunities for those willing to learn and adapt in this fast-paced environment.
Ending
As we wrap up our comprehensive exploration of JavaScript, the significance of this language in the realm of web development becomes crystal clear. JavaScript is more than just a tool for creating dynamic content; it has become foundational in shaping the internet as we know it today. The language's versatility allows for a wide range of applications, from enhancing user interfaces to powering complex web applications and everything in between.
Summarizing Key Points
In this article, we’ve taken a deep dive into various aspects of JavaScript. From understanding the language's historical background to dissecting critical features such as dynamic typing and first-class functions, we laid the groundwork for a thorough comprehension.
- Historical Background - JavaScript's evolution over the years provides essential context, revealing how its unique qualities differentiate it from other programming languages.
- Key Features - The language's flexibility, like prototype-based OOP and functional programming paradigms, empowers developers to adopt numerous styles according to their needs.
- Role in Web Development - The shift towards client-side scripting and asynchronous programming highlights how JavaScript revolutionized user experience.
- Syntax and Structure - Understanding variables, control structures, and functions equips new programmers with tools to write effective code.
- Practical Applications - From interactive websites to robust web applications and API integration, the real-world applications of JavaScript are limitless.
- Advanced Concepts - Grasping closures, promises, and ES6 features prepares developers for modern coding challenges.
- Best Practices - Learning effective error handling, debugging strategies, and performance optimization techniques is vital for writing maintainable code.
- Modern Development - The discussion on frameworks, server-side rendering, and future trends illustrates JavaScript's evolving landscape.
These key points provide a framework for understanding not just how JavaScript works, but why it matters deeply in today's tech-driven world.
Encouraging Continued Learning
Knowledge is like a vast ocean, and diving deeper into JavaScript will only enrich your understanding and mastery of programming. Continuous learning is essential in this field, where technologies and best practices evolve rapidly. Here are some suggestions for ongoing education:
- Engage with Online Communities: Platforms like reddit.com offer lively discussions and shared experiences that can enhance your learning curve.
- Explore Advanced Topics: Once comfortable with the basics, venture into frameworks like React, Angular, and Vue.js, which can dramatically increase your productivity.
- Stay Current: Follow resources like britannica.com for the latest developments in JavaScript and related technologies.
- Practice, Practice, Practice: Build projects that excite you. Whether it's a simple to-do list app or a complex web application, practical experience serves as the best teacher.
In summary, JavaScript is an indispensable tool in a developer's toolkit, and understanding its nuances can significantly impact a career in tech. There's always more to learn, so encourage yourself to explore new horizons and become well-versed in this dynamic programming language.
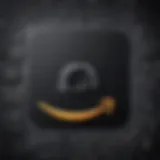
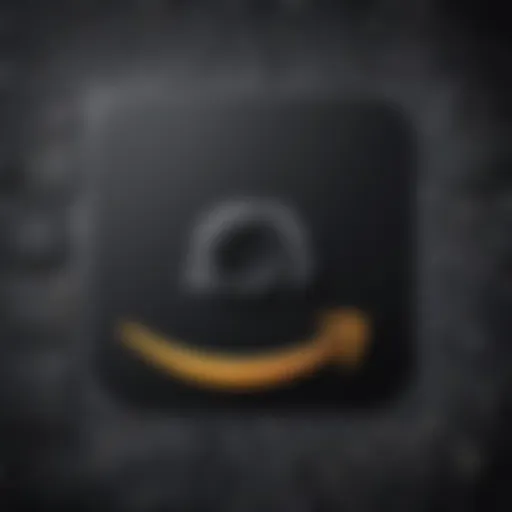