Mastering Object-Oriented Programming in Java: A Comprehensive Guide
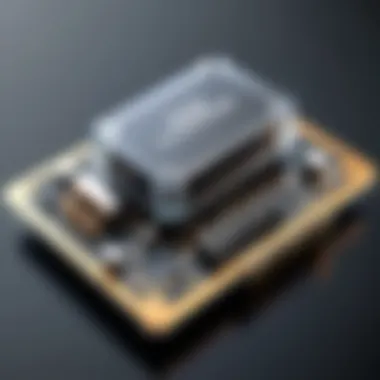
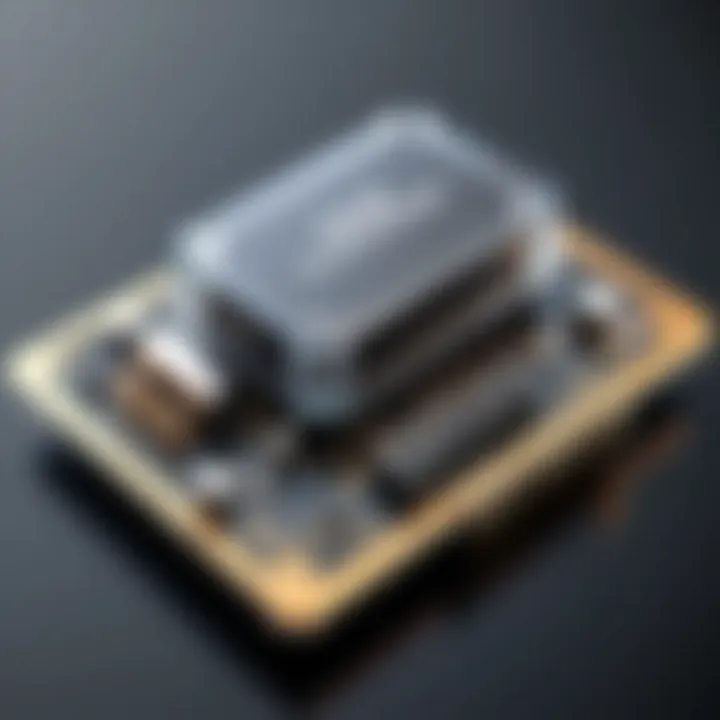
Introduction to Programming Language
Java, a versatile and robust programming language, has been instrumental in shaping the digital landscape with its rich history and background. Originally developed by Sun Microsystems in 1995, Java has surged in popularity due to its platform independence, making it a top choice for building enterprise-level applications. The language boasts extensive features, from its object-oriented approach to its emphasis on security and efficiency, cementing its significance in software development. Java's widespread use extends across web applications, mobile development, and big data processing, reflecting its broad scope and relevance in the tech industry.
Basic Syntax and Concepts
To grasp Java's essence, understanding its basic syntax and concepts is paramount. Variables in Java store data, each with a specific data type defining its characteristics and usage. Operators and expressions manipulate data or variables, executing logical or mathematical operations. Control structures like loops and conditional statements govern the flow of a program, enabling developers to execute code conditionally or iteratively, enhancing program efficiency and functionality.
Advanced Topics
Delving further into Java unveils advanced topics crucial for mastery. Functions and methods encapsulate segments of code, promoting reusability and modular design. Object-oriented programming (OOP) in Java emphasizes code organization around objects, fostering code scalability and flexibility. Exception handling mechanisms address runtime errors, ensuring program integrity and providing graceful error management within applications.
Hands-On Examples
Practical application solidifies Java proficiency, manifested through simple programs, intermediate projects, and code snippets. Simple programs elucidate fundamental concepts through concise, illustrative code implementations. Intermediate projects challenge developers with complex tasks, honing problem-solving skills and creativity. Code snippets offer ready-to-use segments for various functions, aiding in efficiency and promoting best coding practices.
Resources and Further Learning
For continuous growth, accessing resources and further learning materials is indispensable. Recommended books and tutorials provide in-depth insights, serving as comprehensive guides for Java learners of all levels. Online courses and platforms offer interactive learning experiences, equipping enthusiasts with practical skills and knowledge. Community forums and groups foster collaboration and knowledge sharing, creating a vibrant ecosystem for Java developers to engage, learn, and grow.
Introduction to Object-Oriented Programming (OOP)
In the realm of computer programming, Object-Oriented Programming (OOP) stands as a foundational concept essential for any aspiring developer. It serves as a robust framework that promotes organized code structure and reusability, key aspects that streamline the development process. Understanding OOP principles is akin to mastering the fundamental building blocks of modern software engineering. In this article, we unravel the intricacies of OOP in the context of Java, shedding light on its significance and application in the programming landscape.
Overview of OOP Principles
Inheritance
Inheritance functions as a paramount feature of OOP, offering a mechanism for deriving new classes from existing ones, thereby facilitating code reuse and promoting a hierarchical structure within a codebase. This principle allows for the propagation of attributes and behaviors from a parent class to its subclasses, fostering a relationship of 'is a' among objects. Despite its advantages in promoting code reusability and establishing logical connections between entities, overuse of inheritance can lead to complex hierarchies, potentially complicating maintenance and future modifications.
Polymorphism
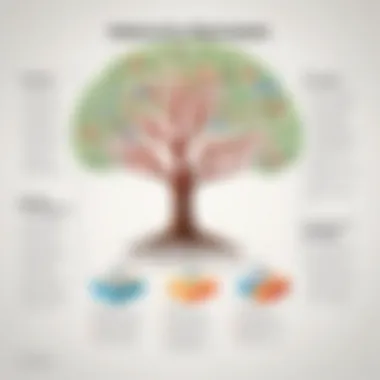
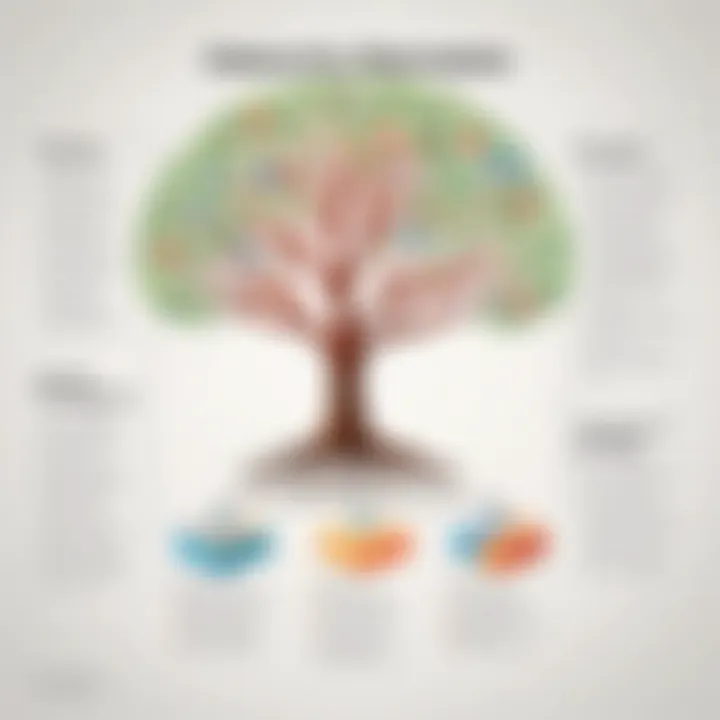
Polymorphism, a cornerstone of OOP, enables objects to exhibit multiple forms based on their context. Through method overloading and overriding, polymorphism empowers developers to write more concise and flexible code that adapts to diverse situations. This feature enhances code readability and maintenance while promoting adaptability and extensibility. However, excessive use of polymorphism without careful consideration may introduce ambiguity and hinder code comprehension.
Abstraction
Abstraction underscores the process of modeling real-world entities as simplified representations, focusing on essential attributes and behaviors while hiding implementation details. By creating abstract classes and interfaces, developers can define blueprints that guide class implementations, promoting code standardization and modularity. While abstraction fosters code flexibility and scalability, improper abstraction levels can impede code understanding and hinder maintenance efforts.
Encapsulation
Encapsulation encapsulates data and methods within a class, restricting external access and promoting data integrity. This principle enhances code security, reusability, and maintainability by enforcing access controls and reducing dependencies between classes. However, overly restrictive encapsulation can hinder code extensibility and testing, underscoring the need for a balanced approach to information hiding.
Benefits of OOP
Code Reusability
Code reusability serves as a pivotal advantage of OOP, allowing developers to leverage existing code components in new contexts efficiently. By encapsulating reusable functionalities into classes and objects, developers can minimize redundancy, enhance productivity, and expedite development cycles. However, over-reliance on code reusability can lead to tight coupling and diminished code modularization, necessitating careful design considerations.
Modularity
Modularity epitomizes the decomposition of a system into cohesive and encapsulated modules that encapsulate specific functionalities. This principle promotes code organization, reusability, and maintainability by isolating components, fostering independent development, and simplifying system enhancements. Yet, excessive module independence can undermine code cohesion and lead to integration challenges, highlighting the importance of striking a balance between modularity and interconnectivity.
Flexibility
Flexibility in OOP manifests as the ability of a system to adapt to changing requirements seamlessly without necessary code refactoring. This trait enables developers to modify and extend software functionalities efficiently, accommodating evolving needs and ensuring software longevity. Nonetheless, excessive flexibility can introduce complexity and overhead, necessitating a cautious approach in balancing adaptability with code simplicity and clarity.
Key Concepts of OOP in Java
Object-Oriented Programming (OOP) in Java is a fundamental concept that forms the backbone of efficient and scalable software development. Understanding the key concepts of OOP is essential for programmers aiming to write structured and maintainable code. In this article, we delve into the core principles of OOP in Java, focusing on encapsulation, inheritance, polymorphism, and abstraction. By grasping these concepts, readers can elevate their Java programming skills and tackle complex coding challenges effectively.
Class and Object
Definition and Implementation
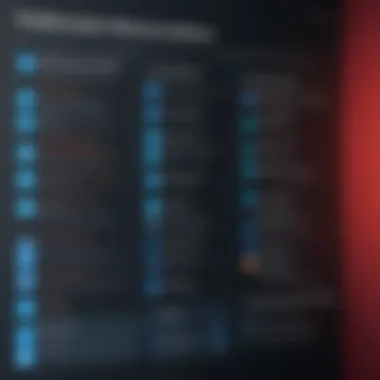
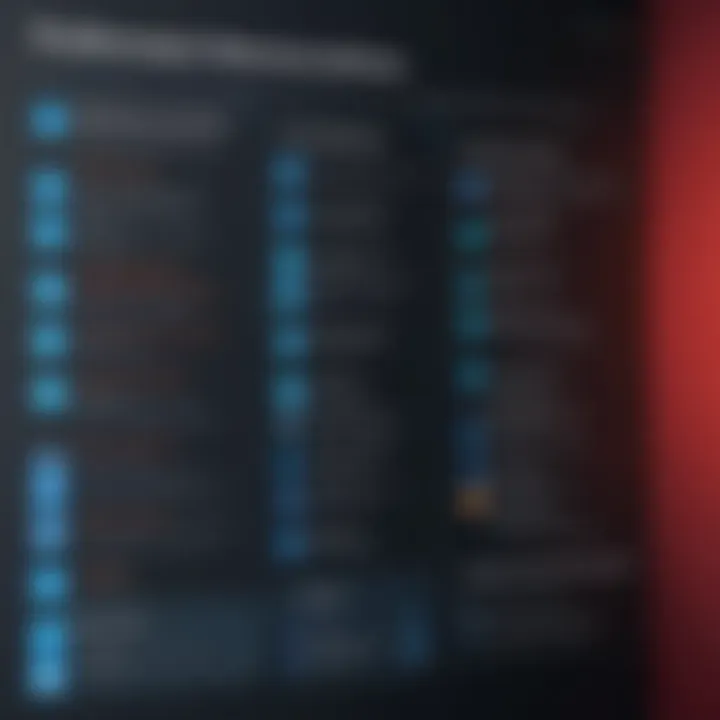
Class and object play a pivotal role in OOP as they define the structure and behavior of software entities. A class serves as a blueprint for creating objects, encapsulating data attributes and methods. By instantiating objects from classes, developers can instantiate multiple instances with distinct properties, fostering code reusability and modularity. The flexibility of defining classes and creating objects enhances code organization and promotes a more manageable development process in Java.
Relationship between Class and Object
The relationship between class and object in Java is symbiotic, where classes act as templates for object instantiation. Objects are instances of classes, inheriting the attributes and behaviors defined within the class. This relationship enables the implementation of inheritance, polymorphism, and encapsulation, crucial components of OOP. By understanding the interplay between classes and objects, programmers can design robust and scalable Java applications that adhere to OOP principles.
Inheritance in Java
Types of Inheritance
Inheritance is a key mechanism in Java that allows a class to inherit properties and behaviors from another class. Through inheritance, developers can establish a hierarchical relationship between classes, promoting code reuse and enhancing the extensibility of software components. Java supports various types of inheritance, including single, multilevel, hierarchical, and hybrid inheritance, offering programmers the flexibility to design class structures tailored to specific application requirements.
Method Overriding
Method overriding in Java enables a subclass to provide a specific implementation of a method defined in its superclass. This feature facilitates runtime polymorphism, where the method to be executed is determined dynamically based on the object's type. By overriding methods, programmers can customize the behavior of inherited methods in subclasses, tailoring functionality to suit unique requirements. Method overriding enhances code flexibility and promotes the adherence to the open-closed principle in OOP.
Super Keyword
The keyword in Java refers to the superclass of a subclass and is used to invoke superclass methods, constructors, and variables. By leveraging the keyword, developers can access superclass elements within a subclass, facilitating method overriding and constructor chaining. This keyword is essential for managing method conflicts, accessing hidden superclass members, and ensuring seamless interaction between parent and child classes in Java inheritance hierarchies.
Polymorphism and Method Overloading
Compile-Time and Runtime Polymorphism
Polymorphism is a core concept in OOP that allows objects to be treated as instances of their parent class. Compile-time polymorphism, achieved through method overloading, enables the implementation of multiple methods with the same name but different parameters. Runtime polymorphism, achieved through method overriding, facilitates dynamic method invocation based on the object type at runtime. By embracing polymorphism, Java programmers can write flexible and extensible code that adapts to varying runtime conditions and supports diverse object behaviors.
Examples of Method Overloading in Java
Method overloading in Java enables the declaration of multiple methods with the same name but different parameters. This feature enhances code readability and simplifies method invocation, catering to diverse input scenarios without sacrificing clarity. By providing examples of method overloading in Java, programmers can elucidate the versatility and conciseness of this feature, demonstrating how method signatures can vary based on parameter types, numbers, and order.
Abstraction and Interface
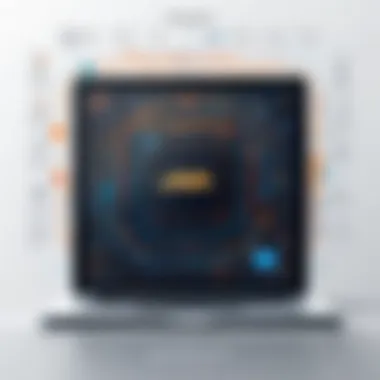
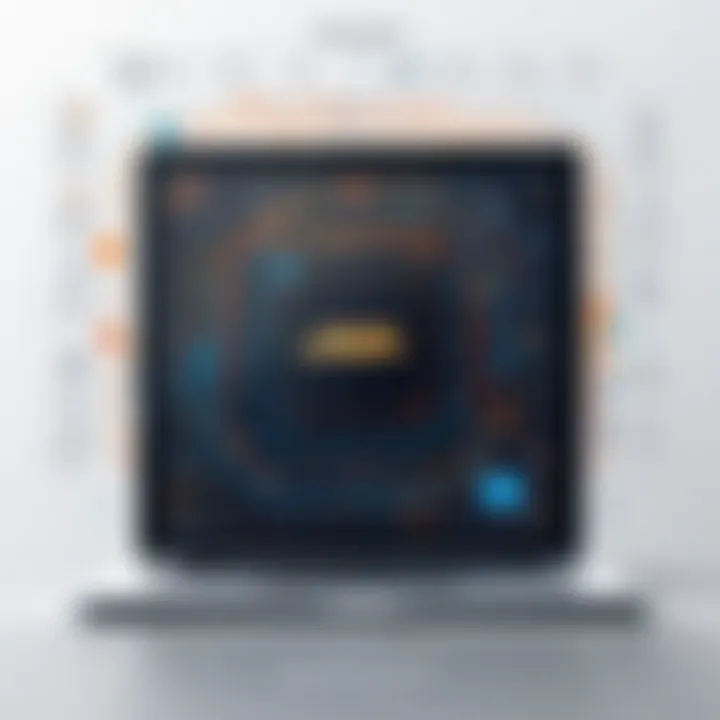
Abstract Classes
Abstract classes in Java serve as blueprints for defining common characteristics and behaviors shared by multiple classes. By declaring methods as abstract, developers establish a contract that subclasses must fulfill, promoting code consistency and abstraction of implementation details. Abstract classes enable the definition of template methods and abstract member variables, guiding subclasses in implementing specific functionality while maintaining a unified interface. By embracing abstract classes, programmers can design flexible class hierarchies and enforce consistent behavior across related classes.
Interfaces in Java
Interfaces in Java define abstract behaviors that classes can implement, enabling the realization of multiple inheritance and behavioral contracts. By providing a way to specify method signatures without revealing implementation details, interfaces facilitate code modularity and interoperability. Java interfaces promote code flexibility and extensibility, allowing classes to implement multiple interfaces and achieve polymorphic behavior. By incorporating interfaces into Java programming, developers can enhance code adaptability and design robust systems that adhere to OOP principles.
Encapsulation and Access Modifiers
Private, Protected, Public, Default
Encapsulation in Java encapsulates data and methods within a class, restricting direct access and ensuring data security and integrity. Access modifiers such as private, protected, public, and default control the visibility and accessibility of class members, regulating interactions between class components. Private members are accessible only within the class, while protected members can be accessed within the package and subclass. Public members are accessible from any class, promoting code reusability, while default members have package-level visibility. By utilizing access modifiers, programmers can enforce encapsulation, protect sensitive data, and establish clear boundaries between class components.
Encapsulation Benefits
Encapsulation in Java offers several benefits, including data security, code maintainability, and modular design. By encapsulating data within classes and exposing interfaces for interaction, developers safeguard data integrity and prevent unauthorized access. Encapsulation promotes code organization and reduces dependencies between classes, facilitating system scalability and maintenance. Additionally, encapsulation enhances code readability and facilitates collaborative development, as team members can work on isolated components without affecting the overall system functionality. By leveraging encapsulation, Java programmers can streamline development processes, enhance code quality, and build robust and secure applications.
Common Interview Questions on OOP in Java
In this section of the article, we delve into the significance of addressing common interview questions relating to Object-Oriented Programming (OOP) in Java. Understanding the ins and outs of these questions is paramount for individuals preparing for Java interviews and seeking to solidify their grasp on OOP principles. By delving into prevalent topics like inheritance, polymorphism, abstraction, and encapsulation through interview questions, readers can enhance their problem-solving skills and critical thinking abilities within the context of Java programming. Navigating through these questions not only aids in interview preparation but also enriches comprehension of OOP concepts, enabling a holistic approach towards Java programming.
Explain Inheritance with an Example
Inheritance serves as a foundational concept in Object-Oriented Programming, particularly significant in Java programming. Essentially, inheritance allows a class to inherit attributes and methods from another class, facilitating code reusability and promoting a hierarchical structure within a program. Understanding this concept is crucial to establish relationships between classes, promoting efficiency and maintaining a streamlined coding process. To illustrate, consider a scenario where a superclass 'Vehicle' encompasses general attributes like 'speed' and 'fuel type', while subclasses like 'Car' and 'Motorcycle' inherit these attributes. This example showcases how inheritance streamlines code implementation, enhances readability, and fosters a structured approach to Java programming.
What is the Difference Between Overloading and Overriding?
Distinguishing between method overloading and method overriding is pivotal in Java programming to ensure the correct utilization of these two concepts. Method overloading involves defining multiple methods in the same class with the same name but different parameters. On the other hand, method overriding occurs when a subclass provides a specific implementation of a method that is already defined in its superclass. Mastery of these distinctions is crucial as method overloading enhances code flexibility and readability by catering to varying method functionalities, while method overriding promotes customization and specialization within class hierarchies, thereby enriching the scalability and adaptability of Java programs.
Why Should We Use Abstraction in Java?
Abstraction serves as a fundamental principle in Java programming, emphasizing the concept of concealing implementation details and solely focusing on essential functionalities. Employing abstraction enhances code modularity, promotes code maintainability, and simplifies complex systems by delineating the core functionalities of a class from its implementation details. By utilizing abstract classes and interfaces, developers can enhance code scalability and flexibility, allowing for easier modifications and expansions within a program. Embracing abstraction in Java programming facilitates code structuring, eases debugging processes, and augments code understandability, thereby fostering efficient and scalable Java programs.
Discuss the Role of Encapsulation in OOP
Encapsulation plays a pivotal role in Object-Oriented Programming by encapsulating data within classes and controlling access to that data through methods. This mechanism enhances data security, prevents external interference, and promotes data integrity within a program. By encapsulating data, developers can minimize complexities, enhance code reliability, and reduce dependencies between classes, thereby facilitating code maintenance and promoting code robustness. With access modifiers like private, protected, public, and default, encapsulation enables developers to regulate data access levels, fortify data security, and uphold data consistency, thereby fostering a secure and scalable programming environment.