Understanding Interface Samples in Programming
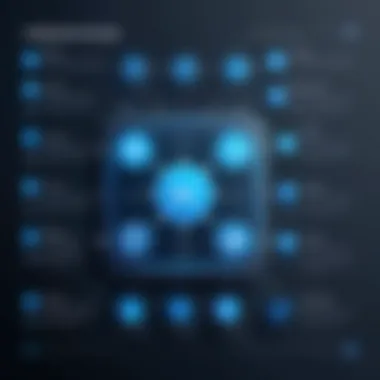
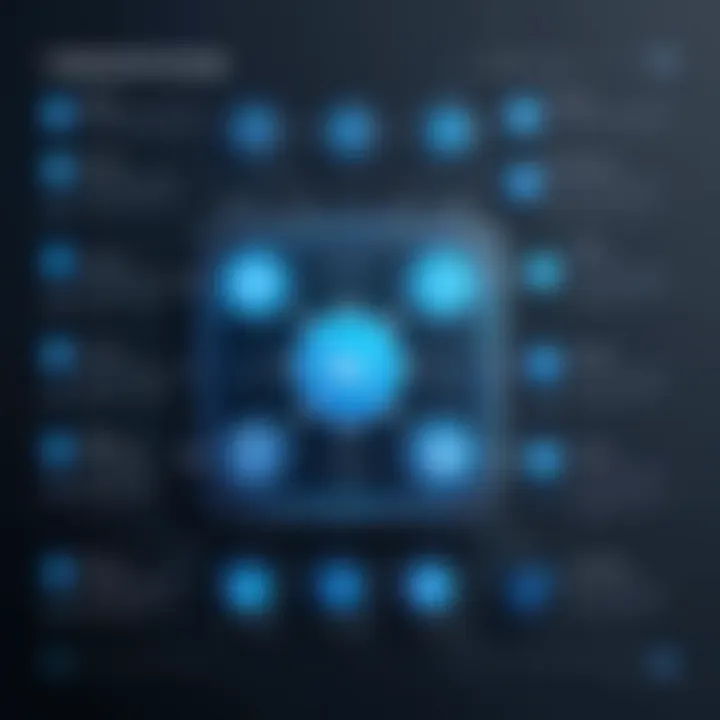
Intro
The rapidly evolving landscape of programming necessitates a deep understanding of various components that facilitate structure and organization in code. One such component is the interface, a crucial element that appears in numerous programming languages. This article seeks to illuminate the essential features, practical applications, and influence of interface samples within software development.
In programming, interfaces serve as a contract, outlining the methods and properties entities must implement. They promote consistency and reuse of code while ensuring that different parts of a program can interact seamlessly. This exploration will delve into what interfaces are, how they function, and the advantages they confer in various programming environments.
As we step through this topic, expect to uncover the role of interfaces in enhancing software architecture, comparing their characteristics with related constructs like abstract classes, and evaluating best practices in their design and implementation. Furthermore, we will touch upon future trends that may shape how interfaces are utilized in evolving coding paradigms.
Preamble to Interfaces
Interfaces are a crucial concept in programming. They play a significant role in defining behavior and establishing contracts between various components of a software system. Understanding interfaces helps developers create more organized and maintainable code. In this section, we will explore the definition of an interface and provide a historical overview. This exploration will set the stage for a deeper understanding of interfaces in later sections.
Definition of an Interface
An interface, in programming, is a specification that outlines a set of methods and properties that a class must implement. It acts as a blueprint that enforces a certain structure on the implementing classes. For example, in Java or C#, an interface can define methods without providing concrete implementations. Classes that implement this interface need to provide the actual behavior for those methods.
This approach fosters loose coupling between components. When one component relies on an interface, it remains agnostic of the specific implementations. This leads to systems that are easier to modify and extend. Furthermore, interfaces enable polymorphism, allowing objects to be treated as instances of their interfaces, and facilitating flexibility in code.
Historical Context of Interfaces
The concept of interfaces in programming dates back to the early days of object-oriented programming. Languages such as Simula and Smalltalk pioneered the idea of defining behaviors separately from their implementation. The adoption of interfaces became mainstream with the introduction of languages like Java in the mid-1990s. Java popularized the use of interfaces in a structured manner, allowing developers to define multiple interfaces a class could implement, thus promoting a more modular architecture.
As programming paradigms have evolved, interfaces have adapted to accommodate new methodologies, such as functional programming. In many modern languages, interfaces are integral to design patterns and are used to facilitate interaction between disparate parts of a system. Understanding this historical context is essential for grasping the significance and current applications of interfaces in contemporary programming.
The Purpose of Interfaces in Programming
Interfaces play a crucial role in programming, serving as a contract for what a class can do, without revealing how it does it. This abstraction is vital in creating systems that are easier to manage and adapt over time. In this section, we will explore three primary purposes of interfaces: Separation of Concerns, Facilitating Polymorphism, and Enhancing Code Reusability. Each of these benefits highlights the significance of interfaces in modern software design.
Separation of Concerns
Separation of concerns is a fundamental principle in software engineering. Interfaces help achieve this by delineating specific functionalities, allowing individual components to focus on their tasks without overlap. For example, when designing a user authentication system, you might create an interface for authentication. This interface would declare methods like and . Different classes can then implement this interface uniquely according to the authentication mechanism needed, such as via OAuth or a simple username-password scheme. By using interfaces, responsibilities are clearly outlined, reducing the chance of conflicting implementations.
This leads to cleaner and more maintainable code. It also allows developers to alter the underlying implementations without impacting the components that rely on these interfaces. If a new authentication method is desired in the future, the developer can create a new class that implements the same interface while leaving existing functionalities intact.
Facilitating Polymorphism
Polymorphism is another central concept in programming that interfaces support effectively. It enables a single interface to be implemented by multiple classes, allowing for different behaviors to be assigned at runtime. When programming, for instance, you can have a interface with a method . Classes like , , and can implement this interface, each providing a unique implementation of the method.
This leads to code that is more flexible and easier to extend. Clients using the interface can call the method without knowing the specific type of shape they are working with. This concept is particularly powerful in collections where various objects can be processed uniformly based on a common interface.
Enhancing Code Reusability
Code reusability is a critical aspect of software development, especially in large systems. Interfaces contribute to this by allowing developers to define common behaviors while enabling different implementations. For instance, if you define an interface with methods for and , you can create multiple implementations: one for a relational database, another for a NoSQL database, and still another for in-memory storage.
This set-up allows for easy swapping of implementations as per project needs. If a project requirement changes from using a SQL database to an in-memory solution for faster access times, you only need to implement a new class without altering the logic that interacts with .
In summary, the design and purpose of interfaces in programming serve as a crucial backbone for clean and effective coding practices. They facilitate separation of concerns, promote polymorphism, and enhance code reusability. Understanding these purposes not only aids in writing clear code but also empowers developers to respond swiftly to changing requirements and emergent technologies in the field.
Interface Samples Across Different Languages
Understanding interface samples within different programming languages is essential for any developer. The notion of interfaces transcends languages, yet varies in syntax and implementation. This section aims to reveal the importance of interfaces, how they are utilized across popular programming languages, and the benefits they provide to code structure and maintainability.
Interface Implementation in Java
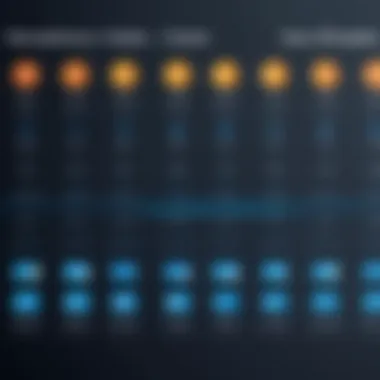
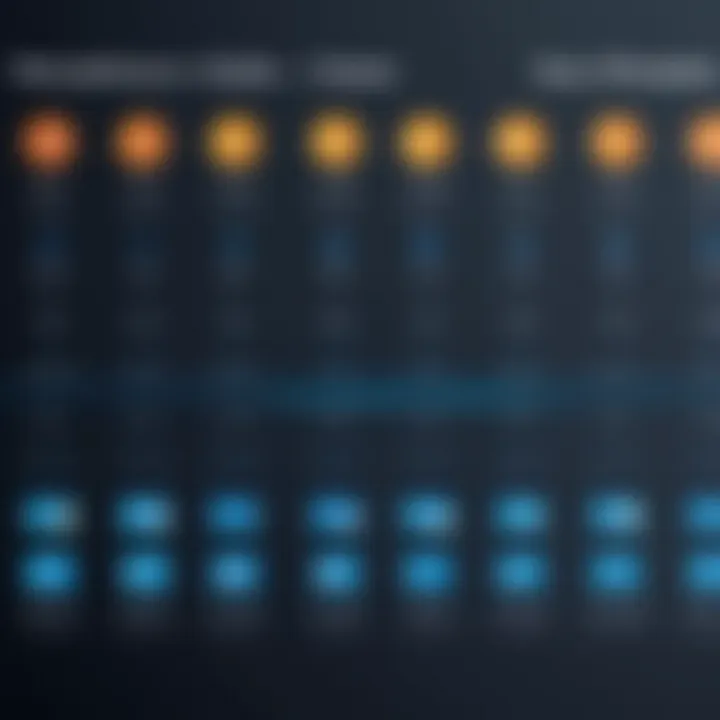
Basic Syntax
The basic syntax of interfaces in Java consists of defining an interface keyword followed by the interface name. The methods declared within an interface do not have bodies. Their implementation must be provided in the classes that implement the interface. This structure helps to define a contract that classes must fulfill. Its simplicity is a key feature. It establishes a clear guideline on how classes should interact. However, the constraint of not having method implementations can be seen as a disadvantage for developers who want to encapsulate some functionality.
Example: Animal Interface
The Animal interface is an illustrative example, defining two methods: and . This interface can be implemented by different classes such as Dog, Cat, etc., each providing specific details on how these methods operate. This adaptability makes the Animal interface popular among developers. However, the limitation comes from the fact that it requires each class to independently implement methods.
Practical Use Cases
Practical implementation of the Animal interface can be seen in various scenarios, such as in games or applications requiring a variety of entities with different behavior. Utilizing the interface promotes code reusability, as multiple classes can implement the same interface. Nonetheless, the potential for over-engineering arises if developers create interfaces that are not sufficiently general.
Implementing Interfaces in
Syntax Overview
In C#, an interface is defined similarly to Java. It uses the interface keyword. C# also allows for explicit interface implementation, where the interface methods are implemented only on the interface type. This adds an additional layer of specificity and is particularly useful in complex systems. The syntax promotes clarity, as all methods must be defined in the implementing class, ensuring adherence to the defined contract.
Example: IShape Interface
The IShape interface exemplifies how to establish a geometric structure with method for . Any class representing a shape such as Circle or Square must implement this method, enforcing a consistent API. This consistent behavior is advantageous as it allows for interchangeable use of different shape objects, but may sometimes require more detailed implementation than necessary, increasing the complexity.
Common Practices
In C#, adhering to common practices guide developers in creating clear and maintainable interfaces. Naming conventions, such as prefixing interfaces with an 'I', help signify that a type is an interface. This promotes readability within the codebase. However, designers should avoid creating large interfaces to prevent making them cumbersome for developers to implement.
++ Interface Implementation
Virtual Functions
C++ interfaces are primarily implemented using virtual functions. These functions allow polymorphism, letting derived classes override them. This leads to flexibility and dynamic behavior in applications. The unique characteristic of C++ interfaces is this capability to create a common interface while allowing for differentiation in behavior. However, using too many virtual functions can result in performance overhead due to dynamic dispatch.
Example: Shape Interface
The Shape interface can serve as a blueprint for creating various geometric shapes. This interface demands the implementation of methods related to shape characteristics. Utilizing this interface leads to consistent implementation across different shape types, which enhances maintainability. Yet, having numerous concrete implementations of the interface can sometimes complicate code and lead to maintenance challenges.
Best Practices
Best practices for designing interfaces in C++ recommend keeping them simple and sharply focused. It is preferable to ensure an interface only defines what is necessary for a particular functionality. This helps maintain clarity and usability. Unnecessarily complex interfaces can lead to confusion and hinder effective programming.
Comparative Analysis of Interfaces and Abstract Classes
Understanding the differences between interfaces and abstract classes is essential for software developers aiming to build scalable, maintainable applications. Both serve important roles in defining contracts for derived classes while emphasizing the commonalities and differences between them facilitates better programming practices. This section will delve into key distinctions, practical applications, and considerations surrounding the use of interfaces and abstract classes, which are foundational concepts in object-oriented programming.
Key Distinctions
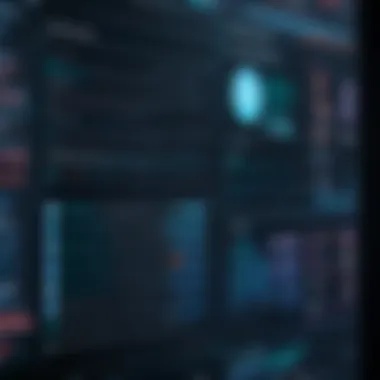
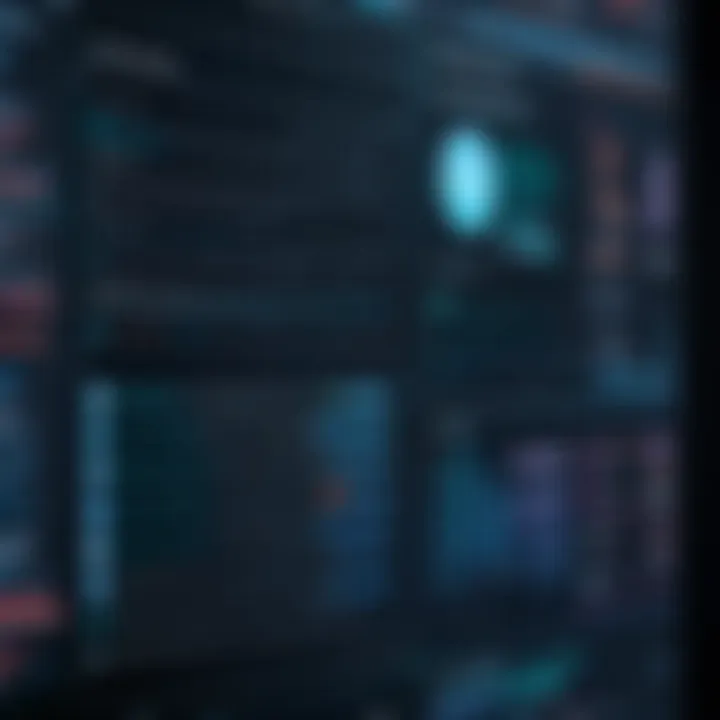
When comparing interfaces and abstract classes, several characteristics take precedence. Here are the major points of distinction:
- Nature of Implementation: An interface exclusively defines method signatures without providing any implementation. In contrast, an abstract class can define methods with implementations, offering a greater scope of encapsulation.
- Multiple Inheritance: Interfaces in languages like Java and C# support multiple inheritance, allowing a class to implement numerous interfaces. However, an abstract class can only be inherited directly by one class. This singular inheritance can restrict flexibility in certain designs.
- Access Modifiers: Interfaces typically do not include access modifiers since all members are public by default. Abstract classes can utilize various access modifiers, providing control over visibility and access levels for class members.
- Fields and Properties: Abstract classes can contain fields, properties, and constructors, while interfaces traditionally do not maintain state or reusability of properties. However, some languages are starting to support default implementations in interfaces.
Overall, these distinctions illustrate that abstract classes may offer more powerful features when the need for shared state or behavior exists, making them suitable for specific use cases.
When to Use Interfaces vs. Abstract Classes
The decision-making process for choosing between interfaces and abstract classes is often context-based. Here are several guidelines:
- Use Interfaces When:
- Use Abstract Classes When:
- You want to define a contract that multiple classes can implement, ensuring that different implementations share a common set of capabilities without needing to derive from the same base class.
- You need to support multiple inheritance of type, enabling a class to incorporate behavior from numerous sources.
- A clean separation between capabilities is needed, especially when there’s no shared code.
- You want to reuse code among closely related classes, as abstract classes allow for shared behavior and state across derived classes.
- Your design calls for constructors or instance variables, making the extra functionality of abstract classes beneficial.
- You expect to evolve your base class over time, potentially introducing new methods with default behavior without breaking existing implementations.
In summary, the decision to use an interface or an abstract class is influenced by the goals of the software design, expected future changes, and specific requirements of the problem domain.
"Selecting the appropriate tool for abstraction can shape the future of your code structure significantly."
By understanding these principles, developers can enhance their design strategies, ensuring the choice aligns with the broader architectural vision.
Common Pitfalls in Interface Design
In software development, designing interfaces is often a critical step that can define the success or failure of a project. Errors in this area may lead to increased complexity and maintenance challenges. Therefore, recognizing common pitfalls is essential for developers aiming for effective and clean code design. Understanding these issues offers insights that contribute to better programming practices.
Overcomplication of Interfaces
An interface should provide a clear and straightforward contract to implementers. However, many developers fall into the trap of overcomplicating interface design. This normally occurs when additional methods, unnecessary parameters, or overly complex types are introduced unnecessarily.
A good interface is one that allows for implementation without confusion.
When interfaces become too complex, they may no longer serve their intended purpose. Instead of promoting modularity and reusability, they become hard to maintain and prone to errors. To avoid this complication, strive for simplicity in interface design. Focus on:
- Clarifying the primary responsibilities of the interface.
- Limiting the number of methods to only those that are essential.
- Using descriptive names that clearly indicate purpose and functionality.
By adhering to these guiding principles, the designed interfaces can remain effective, transparent, and user-friendly.
Ignoring Versioning Issues
As software evolves, so do the requirements and functionalities that interfaces need to provide. Unfortunately, ignoring versioning issues could lead to significant obstacles. If interface modifications are not managed carefully, they may break existing implementations relying on older versions.
Proper versioning ensures that changes in interfaces do not adversely affect dependent code. Consider implementing strategies such as:
- Establishing clearly defined version numbers for interfaces.
- Using default methods in languages like Java to minimize disruption when adding new functionality.
- Offering backward compatibility to allow older implementations to function as expected.
Best Practices for Interface Design
Effective interface design is vital in programming, as it directly influences code quality and maintainability. Adhering to established best practices creates interfaces that are intuitive, efficient and easy to understand. Also, it helps developers avoid confusion, errors and future conflicts. Essential components include simplicity in structure and meaningful naming.
Maintaining Simplicity
Simplicity is a cornerstone of effective interface design. When interfaces are straightforward, they are easier to implement and understand. Complexity can lead to misinterpretation, increased bugs and greater difficulty in maintaining the code.
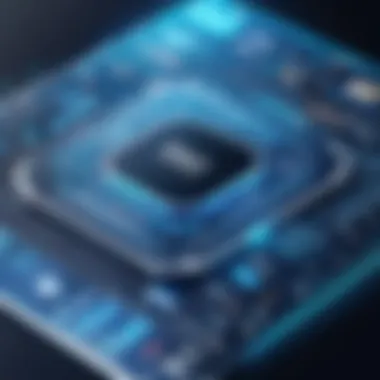
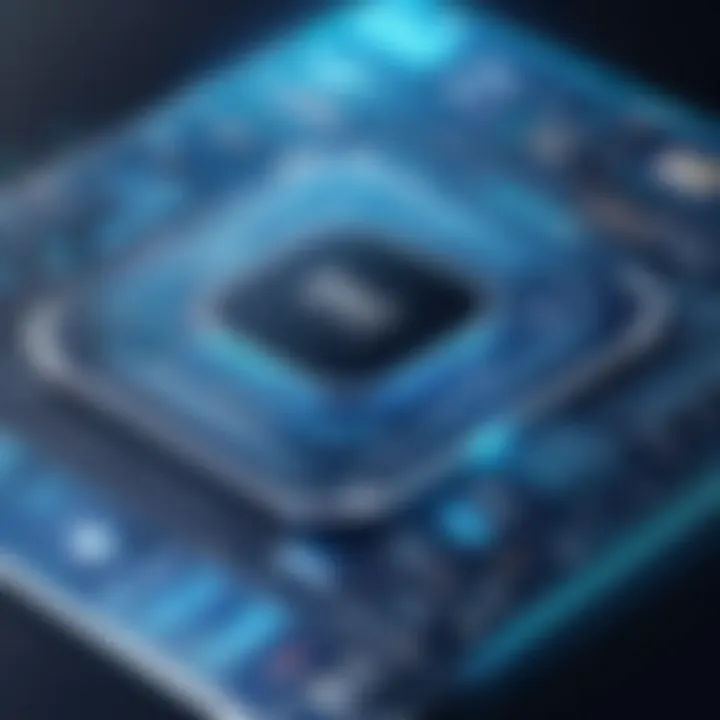
The following points exemplify the importance of maintaining simplicity:
- Limit Interface Size: Smaller interfaces with fewer methods are generally easier to comprehend. This promotes quicker learning and implementation.
- Focus on Cohesion: Group related functionality to enhance clarity. Each interface should define a single responsibility; this makes it more manageable.
- Avoid Unnecessary Methods: If a method does not serve a clear purpose, it should be omitted. This aids in reducing clutter and confusion.
Developers looking to create interfaces should continuously ask themselves how they can streamline their designs. Simplicity should not compromise functionality but rather enhance it.
Utilizing Meaningful Names
Meaningful names are a necessary feature in interface design. Names should communicate the purpose and functionality of the interface clearly. Ambiguous or overly technical names can result in misunderstandings or misuse by other programmers who interact with the interface. Here are some considerations:
- Descriptive Naming: Use full words instead of abbreviations. For instance, is better than . The more descriptive, the better it aids understanding.
- Consistent Naming Conventions: Maintain consistency throughout your naming schema. This enhances familiarity and predictability. The use of prefixes can signal an interface, such as the in .
- Relevance: Names should reflect the functionality. For example, if an interface controls drawing shapes, a name like is apt.
Incorporating meaningful names is significant for long-term code sustainability. It fosters collaboration among multi-disciplinary teams where clarity is key.
"Good design is as little design as possible." – Dieter Rams
Adopting these best practices in interface design can greatly improve the structuring of code. Simplicity and meaningful names ensure that interfaces are user-friendly and yield more robust applications.
The Future of Interfaces in Software Development
The future of interfaces in software development is vital for several reasons. As technology advances, programming paradigms evolve, and interfaces are at the forefront of this evolution. Designers and developers increasingly prioritize modularity, flexibility, and the seamless integration of systems. These priorities make understanding how interfaces will adapt essential for anyone engaged in software development. This section highlights the ongoing trends in interface design and the influence of emerging technologies on their future.
Trends in Interface Design
Interface design is becoming more user-centered and context-aware. Developers are focusing on creating interfaces that are not just functional but also intuitive and accessible. Some key trends include:
- Micro-interfaces: Breaking down complex interfaces into smaller, more manageable components.
- Flexible APIs: As software ecosystems grow, the API interfaces must adapt to serve various client demands efficiently.
- Design Systems: These systems ensure consistency in design across different applications, making interfaces easier to use and implement.
The rise of cloud computing and mobile applications also contributes to these trends. Efforts to streamline user experience across multiple devices necessitate that interfaces evolve continuously. With the growing emphasis on user experience, it becomes necessary for developers to adopt these trends to remain competitive.
Impact of Emerging Technologies
Emerging technologies like artificial intelligence, machine learning, and the Internet of Things are poised to reshape how interfaces are designed and used. These technologies enable smarter interactions, where interfaces can anticipate user needs based on patterns and preferences. Some impacts include:
- Dynamic Interfaces: Interfaces will adapt in real-time to the user’s actions and preferences.
- Voice Interfaces: The increasing use of voice commands means that traditional interfaces must integrate voice recognition technology seamlessly.
- Augmented Reality (AR) and Virtual Reality (VR): These technologies create new dimensions for interfaces, allowing for a more immersive experience. Designing interfaces for such environments presents unique challenges and opportunities.
Consideration: As new technologies influence interface design, developers must remain adaptable. Embrace change while ensuring that interfaces maintain usability.
In summary, the future of interfaces is promising and filled with potential. As interfaces evolve, understanding new trends and the impact of advanced technologies will be crucial for developers. Engaging with these changes ensures that one can design interfaces that meet the evolving needs of users in a dynamic technological landscape.
Closure
The conclusion of this article serves a critical purpose in synthesizing the key takeaways established throughout the discussion on interface samples in programming. It allows readers to step back and reflect on the material covered. This reflection reinforces the significance of interfaces within various programming languages and their essential role in creating robust software architectures.
The primary benefits of interfaces include their ability to promote code reusability, enhance maintainability, and facilitate clearer communication between different parts of a software application. Interfaces act as contracts that ensure implementing classes adhere to expected behaviors, thereby minimizing runtime errors.
Additionally, considering the challenges in interface design, as outlined in earlier sections, is vital. It highlights the need for developers to be mindful of maintaining simplicity and avoiding unnecessary complexity, which can lead to confusion and diminished effectiveness in software development.
Overall, the conclusion ties the article together, offering a comprehensive summary that gives clarity on the unique advantages of using interfaces.
Recap of Key Points
- Definition: Interfaces define a set of methods that other classes can implement, serving as a blueprint for behavior without dictating how the methods should be executed.
- Importance: They separate concerns, facilitate polymorphism, and enhance code reusability.
- Language Comparisons: Different programming languages approach interfaces in their own ways, such as Java, C#, and C++. Each has its syntax and use cases that reflect its language's philosophy.
- Best Practices: Keeping interfaces simple, using meaningful names, and anticipating future changes can improve overall design quality.
- Trends: Staying updated with trends in technological advancements affects how interfaces are designed and utilized.
Final Thoughts on Interfaces
Interfaces can be deemed as essential constructs in modern software development. They provide a structural backbone that allows developers to define how their classes should interact without enforcing specific implementations. This property leads to extensive benefits, such as increased flexibility and easier maintenance of code.
As software development evolves, the understanding and application of interfaces must keep pace with emerging technologies. Developers should remain vigilant, adapting their approach to incorporating interfaces that not only fit current paradigms but also anticipate future advancements in programming practices. By doing so, they can ensure their code remains understandable and efficient.