Understanding Hibernate: A Guide for Programmers
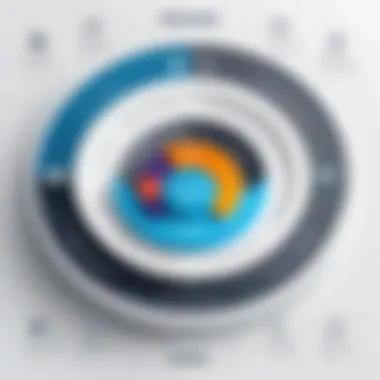
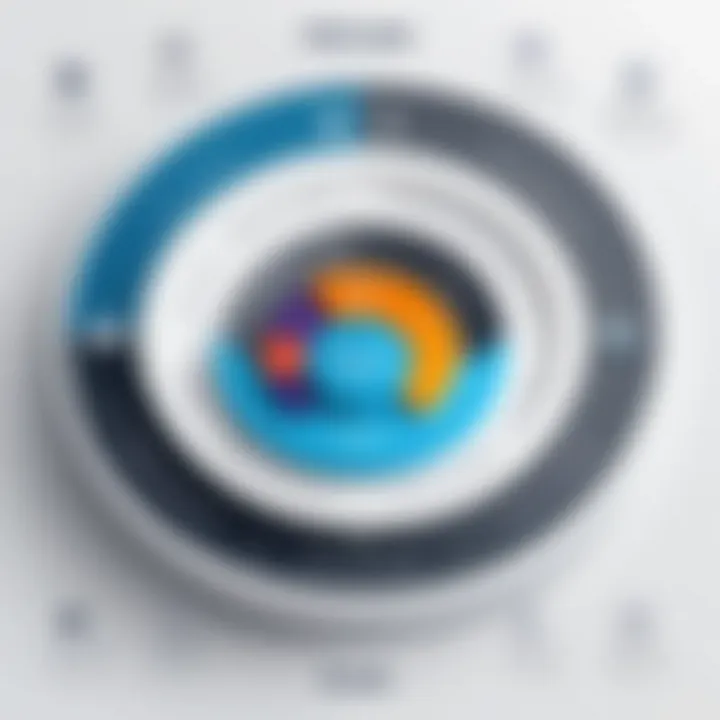
Preamble to Programming Language
Hibernate is not a programming language, but rather a framework for the Java programming language. This framework simplifies the interaction between Java applications and databases. Understanding Hibernate requires an understanding of Java, as they are closely linked. In this section, we will provide a brief overview of programming in Java and how Hibernate fits into this landscape.
History and Background
Java became popular in the 1990s as a platform-independent language. It provided a robust environment for building applications that run on any device equipped with a Java virtual machine. Hibernate was introduced later in 2001 as a solution to some of the challenges that developers faced when dealing with databases in Java. The idea was to reduce the complexity involved in database handling through an Object-Relational Mapping (ORM) approach.
Features and Uses
Hibernate offers several features that make it indispensable for Java developers. Some key features are:
- ORM capabilities: This allows developers to work with Java objects rather than SQL queries.
- Caching mechanisms: By reducing direct database access, Hibernate can improve application performance.
- Transaction management: Hibernate supports transaction management, which ensures data integrity.
- Support for multiple databases: With Hibernate, developers can work with different database systems without changing their code.
These features make Hibernate suitable for various applications, from small projects to large enterprise systems.
Popularity and Scope
The adoption of Hibernate has grown significantly over the years. Many Java-based applications use it for database operations. According to surveys from various developer forums, including Reddit, Hibernate consistently ranks among the top frameworks for Java developers. Its community support, alongside extensive documentation on platforms like Wikipedia and Britannica, contributes to its popularity. This framework has a significant scope, extending into various domains such as web applications, mobile apps, and even microservices.
"Hibernate is essential for Java developers looking for efficient database management."
In summary, the understanding of Java programming is fundamental to leveraging Hibernate effectively. This guide will take a closer look at Hibernate, its architecture, practical uses, and the challenges developers may encounter while working with it.
As we proceed, we will examine basic concepts and syntax associated with Hibernate, which will provide a more profound understanding and application of this powerful framework.
Intro to Hibernate
Hibernate is an essential Java framework that simplifies the process of working with databases. In the world of software development, managing data effectively is crucial. This section will introduce the core concepts of Hibernate, elucidating its significance for programmers.
What is Hibernate?
Hibernate is an object-relational mapping (ORM) framework for the Java programming language. It allows developers to interact with a database using object-oriented programming practices. With Hibernate, you can map Java classes to database tables. This means that you do not need to write complex SQL queries for basic CRUD (Create, Read, Update, Delete) operations. Instead, you can manipulate Java objects, and Hibernate handles the underlying database communication.
This abstraction simplifies database operations significantly. For example, when you save an object using Hibernate's API, it automatically translates this action into a SQL insert statement, reducing the effort required to implement data access layers. This bridge between Object-Oriented Programming and relational databases makes Hibernate a popular choice among Java developers.
The Need for Hibernate in Java Development
Java developers often face challenges when integrating applications with databases. These challenges include dealing with complex SQL queries, ensuring data consistency, and managing connections efficiently. Hibernate addresses these issues through several means:
- Efficiency: Hibernate reduces boilerplate code. By utilizing annotations or XML configurations, programming becomes faster and more efficient.
- Flexibility: It supports various databases, which means that developers are not locked into a specific database vendor. This adaptability is significant for projects that might evolve over time.
- Transactions: Hibernate provides built-in transaction management features. This functionality ensures that data integrity is maintained, even in the presence of errors.
- Caching: With Hibernate's caching capabilities, frequent database queries can be minimized, which leads to performance improvements.
Hibernate Architecture
The architecture of Hibernate is crucial to its functionality and performance. Understanding the core structure helps programmers to maximize Hibernate's capabilities in Java applications. The architecture is designed to facilitate object-relational mapping (ORM), enabling developers to interact with the database using Java objects instead of SQL statements. This abstraction simplifies complex operations, improving both development speed and application maintainability.
Key benefits of Hibernate's architecture include:
- Loose Coupling: The separation of the database logic from the application logic allows for easier modifications without impacting the other components.
- Database Independence: Hibernate can work with various database management systems, making it versatile and minimizing vendor lock-in.
- Improved Performance: Through features like caching and connection pooling, Hibernate optimizes database access, enhancing application performance.
When considering Hibernate architecture, it's important to recognize the layers involved. Each layer has specific responsibilities which contribute to the overall functionality of the framework.
Core Components of Hibernate
Hibernate's core components are vital for its operation. Understanding these components can simplify many aspects of Java development. The primary components include:
- Session Factory: This is the main access point for Hibernate functionalities. It creates sessions and manages the lifecycle of objects.
- Session: Represents a single unit of work with the database. A session is used for retrieving and storing objects.
- Transaction: Manages the transactional context of operations involving multiple sessions. It ensures data integrity and maintains rollback features in the event of errors.
A Session Factory is typically configured once, whereas sessions are created and opened as needed. This distinction allows for efficient management of resources while working with the database.
Understanding Hibernate ORM
Object-Relational Mapping is the central feature of Hibernate. This concept transforms data between incompatible type systems in object-oriented programming languages like Java. Hibernate's ORM capabilities allow you to treat database records as Java objects, abstracting the complexity involved in writing SQL queries.
Some essential aspects of Hibernate ORM include:
- Entity Classes: These are simple Java classes mapped to database tables. Each instance corresponds to a row in the table.
- Hibernate Query Language (HQL): A powerful query language that permits operations on objects instead of database tables, aiding in the creation of database-agnostic applications.
- Lazy Loading: This feature fetches objects and their relationships on-demand, which can help in optimizing resource usage.
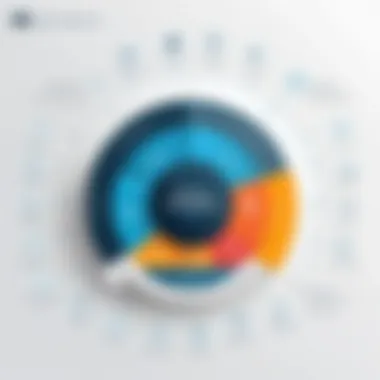
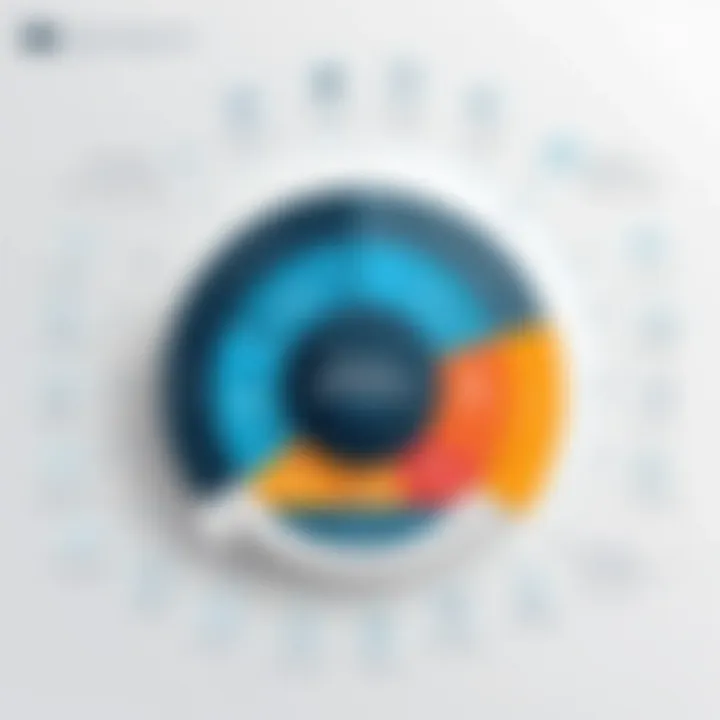
Additionally, the ORM layer simplifies complex data interactions. Developers can focus on writing business logic rather than dealing with data access code that can be cumbersome.
"Hibernate's ORM makes managing database operations seamless, allowing developers to concentrate more on application logic."
Setting Up Hibernate
Setting up Hibernate is a crucial step in using this framework effectively within Java applications. This section covers the elements necessary for installation, the role of configuration files, and how to implement Hibernate into your project. By understanding these components, developers can ensure a smooth integration of Hibernate, which allows for more efficient database management and operations.
Requirements for Installation
To begin with Hibernate, it is essential to meet certain requirements. Your development environment must support Java, typically version 8 or higher. Hibernate relies on various libraries that need to be included in your project. The core Hibernate library is Hibernate Core, which enables the Object-Relational Mapping (ORM) functionality. Further, you need a JDBC driver specific to the database you are using, such as MySQL Connector/J for MySQL databases.
It is also necessary to have an Integrated Development Environment (IDE) that supports Java, such as Eclipse or IntelliJ IDEA. Furthermore, Maven or Gradle can facilitate dependency management.
Proper setup ensures that learning and using Hibernate becomes an efficient process. Missing dependencies can lead to confusion and delays in development.
Configuration Files Overview
Configuration files are vital for Hibernate as they define how your application connects to the database and how Hibernate should operate. The primary file is usually named . This XML file contains essential settings such as the database connection URL, username, password, and Hibernate properties like dialect and show_sql options.
In addition to the core configuration file, you can create mapping files in the form of XML to define entity classes and their relationships to database tables. These mapping files will help Hibernate understand how to translate Java objects into database tables and vice versa.
When configuring Hibernate, pay attention to avoid mistakes in your properties, as this could lead to runtime exceptions. Syntax errors in XML files can also lead to misconfigurations.
Implementing Hibernate in Your Project
Once your environment is prepared and configuration files are set, it is time to implement Hibernate in your project. Start by including the necessary Hibernate libraries in your build configuration. If you are using Maven, you can add Hibernate dependencies in the file as follows:
After adding dependencies, the next step involves initializing a object. This object is essential for creating sessions through which all database operations occur. Here is a simple example to showcase the initialization of :
Through the , you can open sessions to perform operations like saving, updating, and retrieving entities from the database. Understanding how to manage sessions properly is critical, as it influences the performance of your application.
By following these steps and understanding the requirements, configuration, and implementation, developers can confidently set up Hibernate in their projects.
Hibernate Features
Understanding Hibernate's features is essential for leveraging its full potential in Java programming. These features not only simplify database interactions but also enhance performance and scalability in applications. Below, we delve into three core components of Hibernate's functionality: Data Mapping, Caching Mechanisms, and Transaction Management.
Data Mapping
Data mapping is one of Hibernate's pivotal features. It allows developers to define how Java objects relate to database tables. This mapping is usually defined using XML or annotations within Java classes. Mapping properties enable automatic conversion between database records and Java objects. This eliminates the need for writing manual SQL queries and helps to reduce boilerplate code.
Using Hibernate, programmers can easily map complex relationships between entities, including one-to-one, one-to-many, and many-to-many relationships. For example, the mapping between an entity and a entity can be seamlessly handled, thus promoting a clear data structure.
In Hibernate, the concept of the Object-Relational Mapping (ORM) framework comes into play. ORM enables interactions with the database via Java objects. A typical example of data mapping using annotations is shown below:
Data mapping not only fosters cleaner code but also aligns closely with Java's object-oriented paradigm, making the transition between object and relational databases smoother.
Caching Mechanisms
Caching in Hibernate is vital for maximizing performance. It reduces the number of database hits required, thus improving the overall application response time. Hibernate supports two levels of caching: first-level cache and second-level cache. The first-level cache is associated with the session and operates as a basic, in-memory cache that stores objects within a session lifecycle. As such, whenever the same entity is requested within a session, Hibernate retrieves it from the first-level cache.
On the other hand, the second-level cache is a more sophisticated caching system that spans across sessions, storing entities, collections, and associations. This feature is especially useful in applications with high read frequency but low write operations.
Some considerations when using Hibernate's caching mechanisms include:
- Configuration Complexity: Setting up caching correctly can require more intricate configuration.
- Data Freshness: With second-level caching, ensuring data consistency becomes crucial, particularly when multiple applications interact with the same database.
Proper implementation of caching can greatly reduce the load on database servers and optimize performance in Java applications.
Transaction Management
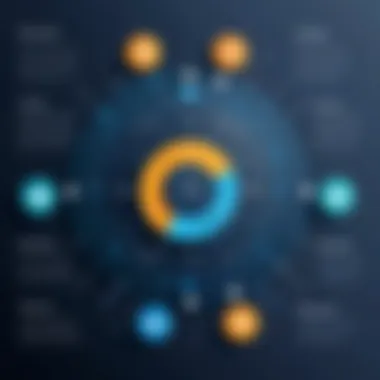
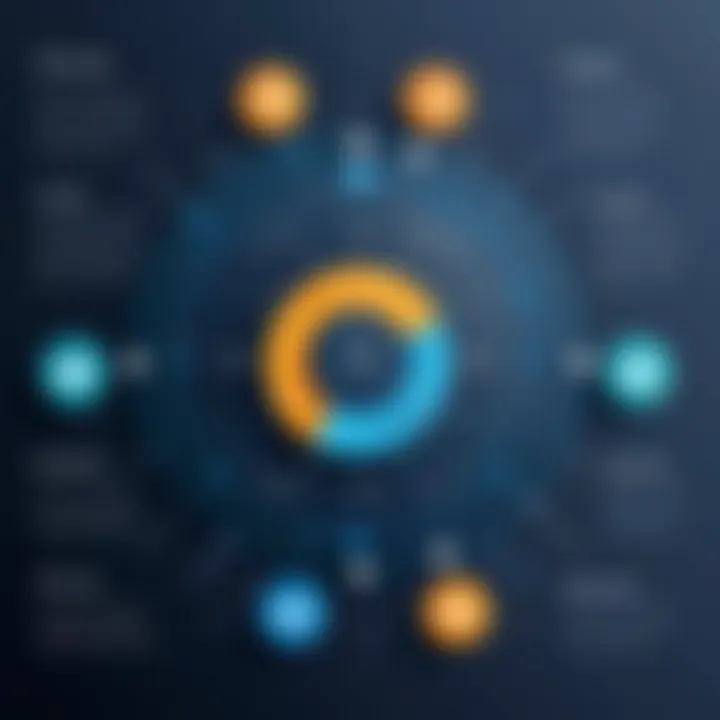
Transaction management in Hibernate is another critical feature that assists in ensuring data integrity and consistency. Hibernate provides a well-defined api for managing transactions across various operations, making it easier for developers to implement complex transaction scenarios like rollback or commit.
Hibernate allows developers to operate in both programmatic and declarative transaction management styles. Using JTA (Java Transaction API) or JDBC, developers have the flexibility to choose what best suits their application architecture.
This structured approach to transaction management includes:
- Atomicity: Transactions ensure that a series of operations complete successfully or not at all.
- Consistency: Data remains in a valid state throughout the transaction lifecycle.
- Isolation: Transactions are processed independently, preventing interference.
- Durability: Once a transaction is committed, the changes persist in the database.
Employing these mechanisms helps prevent data anomalies and ensures reliable database operations. Proper transaction management is crucial for applications requiring high levels of data accuracy and reliability, such as in banking or financial sectors.
"Utilizing effective transaction management is crucial for maintaining data integrity in any robust application."
Common Use Cases of Hibernate
The Common Use Cases of Hibernate are essential for understanding its practical applications in Java development. Through this section, readers will see how Hibernate facilitates various database operations, integrates well with other frameworks, and manages complex queries efficiently. Each use case demonstrates the advantages Hibernate provides, making it a vital tool for developers.
Working with Database Operations
Hibernate simplifies the process of interacting with databases. It abstracts the low-level JDBC API, allowing developers to focus on their application logic rather than the complexities of SQL. This abstraction is crucial when managing CRUD (Create, Read, Update, Delete) operations, enabling smoother interactions with the database. Hibernate's rich API allows for easy data manipulation.
- Entity Mapping: Hibernate maps Java objects to database tables. Developers annotate their Java classes with Hibernate annotations, eliminating the tedious work of writing SQL for simple operations.
- Session Management: Hibernate manages sessions efficiently. Each session represents a single unit of work with the database, making it easier to organize transactions and interactions.
- Batch Processing: It supports batch processing, which improves performance by allowing multiple operations to be executed in a single round trip to the database.
Using these features, Hibernate enables developers to handle database operations without needing extensive SQL knowledge. This is especially beneficial for those new to programming or those who prefer to work with higher-level abstractions.
Integrating Hibernate with Spring Framework
Integrating Hibernate with the Spring Framework exemplifies one of the most powerful use cases for this ORM tool. Spring offers a comprehensive framework for building Java applications. By combining the capabilities of both Spring and Hibernate, developers achieve a robust infrastructure for enterprise applications.
- Transaction Management: Spring provides declarative transaction management which can encompass Hibernate sessions, allowing developers to manage transactions in a more straightforward manner.
- Dependency Injection: This principle helps to reduce dependencies in code. Developers can inject Hibernate sessions into Spring-managed beans, promoting cleaner designs.
- Configuration: The integration allows for simplified configuration management. With Spring's configuration files or annotations, developers can easily specify how to connect to databases using Hibernate.
Such integration enhances productivity, especially in larger applications where managing different components efficiently is crucial. This use case is valuable for developers looking to build well-structured applications without being bogged down by configuration overhead.
Handling Complex Queries
Handling complex queries becomes manageable with Hibernate's Query Language (HQL) and Criteria API. As applications grow, the complexity of SQL queries tends to increase. Hibernate addresses this challenge effectively.
- Object-Oriented Querying: HQL allows developers to write queries in an object-oriented way, making them more intuitive for Java developers. This means they can use Java classes and properties instead of table names and column names.
- Criteria API: This API provides a programmatic way to create queries. It allows developers to construct queries dynamically, which is useful for scenarios where conditions can change depending on user input or application state.
- Lazy Loading: Hibernate supports lazy loading, which can optimize performance for complex queries by loading associated entities only when they are required.
Overall, managing complex queries is essential for applications that interact with large datasets. Hibernate’s capabilities in this area enhance performance and usability.
"Hibernate and Spring integration is a step towards minimalist architecture in enterprise applications, simplifying complexity considerably."
Through these common use cases, programmers can fully exploit Hibernate's capabilities to enhance their Java applications. From straightforward database operations to sophisticated query handling, Hibernate proves to be an indispensable framework in the programmer's toolkit.
Pros and Cons of Using Hibernate
Hibernate has become one of the most widely used frameworks among Java developers for its ability to simplify database interactions. However, like any technology, it comes with its own set of advantages and limitations. Understanding these pros and cons is essential for programmers considering Hibernate for their projects.
Advantages of Hibernate
- Simplified Database Interactions: One of the main benefits of Hibernate is its abstraction of database interactions. Developers can work with Java objects instead of SQL queries. This can reduce boilerplate code and increase productivity.
- Automatic Schema Generation: Hibernate can automatically generate and update the database schema based on the Java object model. This feature ensures that the database structure stays in sync with the application code, reducing manual errors.
- Caching Mechanisms: Hibernate comes with powerful caching capabilities. It supports first-level caching by default, and second-level caching can be configured. This feature can significantly improve application performance by reducing database access.
- HQL (Hibernate Query Language): Hibernate provides a powerful query language that is object-oriented and allows developers to express queries in terms of Java objects rather than database tables. This can improve readability and maintainability of the codebase.
- Support for Complex Data Types: Hibernate provides built-in support for complex data types and relationships, such as one-to-one and many-to-many mappings. This flexibility allows for easier modeling of real-world scenarios within the database.
- Integration with Spring Framework: Hibernate integrates seamlessly with the Spring Framework, providing enhanced functionality and making it a preferred choice for enterprise applications.
Potential Limitations
While Hibernate offers various advantages, potential limitations should also be considered:
- Learning Curve: For programmers new to Object-Relational Mapping (ORM), there might be a learning curve associated with understanding Hibernate’s many features and configurations. It can take time to become proficient in using its capabilities effectively.
- Performance Overhead: Abstracting database access comes with some performance overhead. Hibernate can generate suboptimal SQL queries that might slow down application performance. Developers need to profile and optimize queries manually for critical performance areas.
- Configuration Complexity: The configuration of Hibernate can be complex, especially in large applications. Finding the right settings for caching strategies, transaction management, and ORM settings can be challenging.
- Debugging Challenges: Debugging Hibernate can be complicated, owing to its abstraction. Understanding what happens under the hood requires familiarity with its internal processes, which may not always be straightforward.
- Dependency on ORM: Relying heavily on Hibernate can sometimes lead to developers not writing raw SQL queries. This might reduce the ability to leverage some of the advanced SQL features and optimizations that traditional relational databases offer.
In summary, while Hibernate provides substantial benefits, such as streamlined database operations and support for complex data relationships, it also presents challenges like performance considerations and a steep learning curve. Careful evaluation of both the advantages and potential limitations is crucial for programmers deciding whether to implement Hibernate in their development projects.
Best Practices in Hibernate Development
To maximize the efficiency and effectiveness of Hibernate in your projects, understanding and applying the best practices in Hibernate development is essential. These practices not only enhance the performance of your applications but also ensure maintainability and scalability in the long run. Adhering to these best practices can prevent common pitfalls that developers face when working with Hibernate, such as performance bottlenecks and complex data handling issues.
Optimizing Database Queries
Optimizing database queries is a crucial aspect of ensuring that your Hibernate application performs at its best. Poorly written queries can lead to significant performance issues, negatively impacting user experience and system resources. Here are some considerations for optimizing your queries:
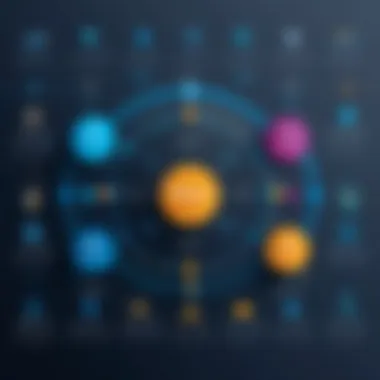
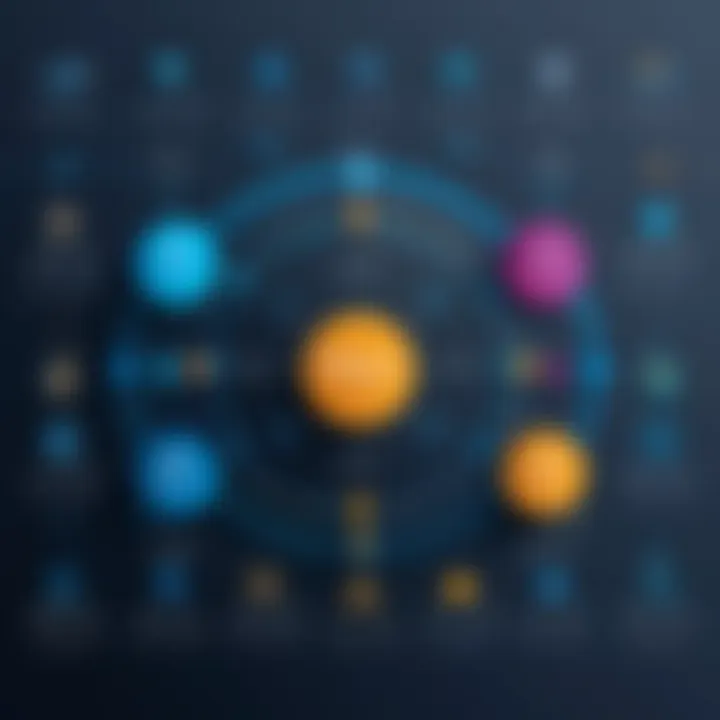
- Use HQL and Criteria Queries: Hibernate Query Language (HQL) provides an object-oriented way to write queries. It abstracts SQL syntax and allows you to work directly with your entities, facilitating easier maintenance. Criteria queries, on the other hand, offer a programmatic way of constructing queries and can dynamically adjust based on user input or application state.
- Fetch Strategies: Understand the fetch types in Hibernate. Using fetching may lead to unnecessary data loading while fetching can optimize performance by loading data only when necessary. Choose wisely based on use cases.
- Batch Processing: If you are dealing with a large number of records, consider using batch processing to minimize the number of database round trips. This can significantly reduce the load time for transactions.
- Caching: Implement second-level caching judiciously. This can reduce database access frequency, enhance performance, and speed up data retrieval. Options like Ehcache can be configured as a second-level cache in Hibernate.
Implementing these strategies can lead to faster and more efficient database interactions, ultimately improving the overall performance of your application.
Effective Error Handling Strategies
Error handling is another vital aspect when using Hibernate. Given that it functions as a bridge between an application and the database, developers must implement strategies for identifying and managing exceptions effectively. Here are some best practices to follow:
- Use Proper Exception Handling: Hibernate provides a set of exceptions that can be managed to handle different scenarios. Familiarize yourself with these exceptions such as , , and . Handling these appropriately will provide clear insights into issues as they arise.
- Logging: Implement comprehensive logging strategies to track errors and application behavior. Make use of logging frameworks like Log4j or SLF4J to log error messages (including stack traces) at strategic locations within your application. This can be invaluable when troubleshooting issues.
- Transaction Management: Ensure that you manage transactions effectively. Using the annotation can simplify transaction handling in a Spring environment. Always roll back transactions in case of a failure to maintain data integrity.
- Graceful Failures: Ensure your application can gracefully recover from failures. This includes providing user-friendly error messages and maintaining a seamless user experience even when errors occur.
By incorporating these error handling strategies, developers can create robust applications that can withstand runtime issues without affecting overall functionality.
Successfully implementing best practices in Hibernate leads not just to smoother transactions, but also a more enjoyable development experience.
Real-World Applications of Hibernate
Understanding the real-world applications of Hibernate is crucial for grasping its significance in contemporary software development. Hibernate is not just a tool for data persistence; it is a framework that brings efficiency and scalability to Java projects. By employing Hibernate, developers can achieve clean database interactions while reducing boilerplate code and enhancing productivity. This section emphasizes specific elements, benefits, and considerations regarding Hibernate's practical applications in different scenarios.
Case Studies in Industry
Numerous organizations have adopted Hibernate for its robust capabilities in managing data. For instance, Netflix utilizes Hibernate in their online platform to streamline database operations. The company benefits from Hibernate's caching mechanisms which support high traffic without sacrificing performance. By leveraging these features, Netflix can ensure seamless streaming experiences for millions of users simultaneously.
Another example is eBay, which employs Hibernate to handle multiple database actions efficiently. The complexity of their data architecture demands a reliable ORM (Object-Relational Mapping) solution. Hibernate allows eBay to maintain data integrity while enabling flexible querying abilities that adjust dynamically to user requirements.
These case studies illustrate how large-scale companies integrate Hibernate to solve real-world challenges, focusing on performance optimization and operational efficiency.
Hibernate in Enterprise Solutions
In the realm of enterprise applications, Hibernate serves as a viable solution for many development teams. Large organizations often face the challenge of integrating numerous databases with bespoke applications. By using Hibernate, these enterprises can achieve a consistent approach across different modules and legacy systems.
A significant advantage is in transaction management. Hibernate supports distributed transactions, which is vital in ensuring that all operations succeed or fail together. This function is particularly important in financial systems, where data consistency is paramount.
Furthermore, Hibernate’s ability to map complex Java objects to relational databases makes it a preferred choice in enterprise environments where data models can become intricate and unwieldy. It simplifies data handling and facilitates quicker response times for inquiries and reporting.
"Aggressive performance tuning of Java applications often relies on utilizing appropriate frameworks like Hibernate, which can optimize interactions with relational databases considerably."
In summary, the real-world applications of Hibernate showcase its role as a cornerstone technology in today's software solutions. Organizations benefit from its ability to simplify their data operations while promoting maintainability, scalability, and performance.
Troubleshooting Common Hibernate Issues
Troubleshooting is a crucial skill for any programmer working with Hibernate. As with any framework, errors and performance lags can occur. Understanding how to address these issues effectively allows developers to maintain application stability and improve user experience. In this section, we will explore common errors and ways to resolve performance bottlenecks, ensuring that your development process is smooth and rewarding.
Identifying Common Errors
Hibernate provides a powerful abstraction layer over relational databases. However, with this power comes the potential for various errors. Developers must be vigilant in identifying these common pitfalls.
- Mapping Issues: Incorrectly defined mappings between Java classes and database tables can lead to runtime errors. Ensure your entities are annotated properly. Review your configuration for discrepancies in data types.
- Session Management Errors: Improper handling of Hibernate sessions can result in lazy loading errors. It’s important to manage the session lifecycle correctly, especially in web applications.
- Transaction Problems: Without proper transaction management, you may face data integrity issues. Ensure that transactions are committed or rolled back as necessary.
Some tools can assist in identifying these errors. Hibernate provides a logging mechanism that can be configured to output error details. It's advisable to enable SQL logging for better visibility into what Hibernate is doing behind the scenes. This can help pinpoint where issues arise during the database operations.
Resolving Performance Bottlenecks
Performance optimization in Hibernate is essential for keeping applications responsive and efficient. Several strategies can be employed to tackle performance issues.
- Batch Processing: Adjust the number of records fetched or updated in a single session. For instance, using batch size configuration can significantly enhance performance.
- Query Optimization: Avoid using HQL or Criteria queries that return more data than necessary. Use projections to fetch only what your application needs. Also, analyze query execution plans to identify slow-performing queries.
- Caching: Implement effective caching mechanisms. Hibernate supports level 1 and level 2 caching. Activating second-level cache can drastically reduce database load for frequently accessed data.
By adopting these strategies, you can significantly enhance the performance of your Hibernate-based applications.
Tip: Regularly monitor your application’s performance and adjust settings based on actual usage patterns and requirements. This proactive approach can prevent issues from escalating.
Ending
In wrapping up our exploration of Hibernate, it’s crucial to reflect on the central themes discussed in this article. Hibernate is not merely a tool; it is an essential framework that revolutionizes how developers interact with databases in Java applications. It abstracts away many complexities, allowing for a more straightforward approach to object-relational mapping (ORM). This ability is paramount in today’s fast-paced development environment where efficiency and performance are key.
Summary of Key Points
Throughout this guide, we have dissected the fundamental elements of Hibernate, from its core architecture to its practical applications. The depth of its features, such as caching mechanisms and transaction management, showcases why it remains a preferred choice among developers. Here are the key points summarized:
- Core Architecture: Hibernate's architecture primarily revolves around the SessionFactory and Session, making it crucial for efficient data manipulation.
- Features: Data mapping, caching, and comprehensive transaction management empower developers to maintain data integrity and optimize performance.
- Real-World Applications: Whether in integrating with frameworks like Spring or in executing complex queries, Hibernate shines in real-world usage scenarios.
- Best Practices: Adhering to established best practices can significantly enhance Hibernate performance, opening doors for scalable applications.
- Troubleshooting: Knowing common pitfalls and how to resolve them can save considerable time and resources.
Future of Hibernate in Java Development
As we look ahead, Hibernate is set to continue evolving. With the rise of cloud-based applications, the demand for seamless database integrations will drive further enhancements in Hibernate. Additionally, as Java itself progresses, Hibernate will likely adapt to new paradigms and practices in software development. The ongoing emphasis on decoupled architectures, such as microservices, will likely influence Hibernate’s design and capabilities.