Understanding Google Authentication for Android Apps
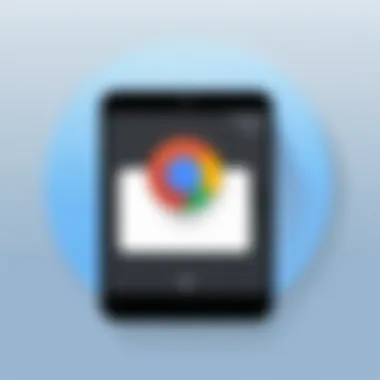
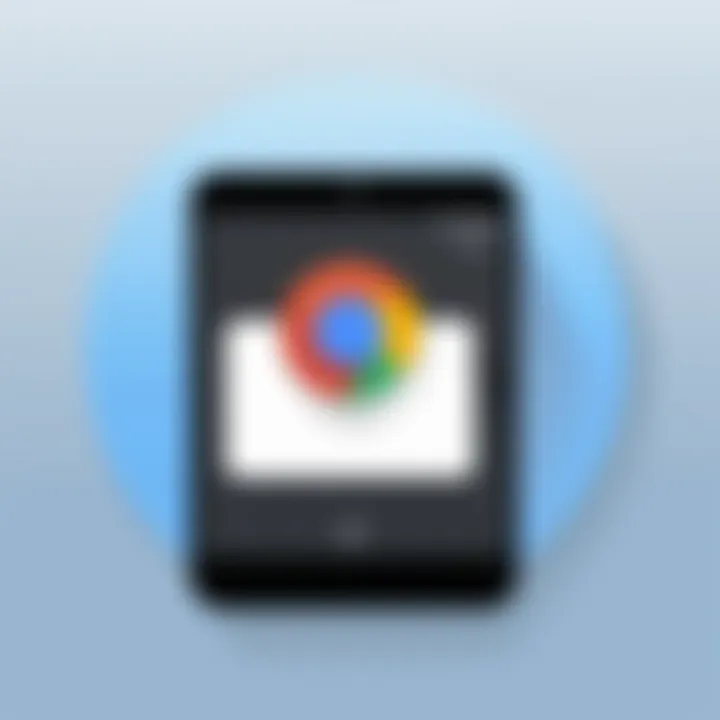
Intro
In today's digital age, user authentication is vital for securing applications. Google Authentication offers a robust solution tailored for developers integrating it within Android applications. This article provides a detailed examination of Google Authentication, its benefits, and the methods for integration. It aims to educate developers and those interested in secure programming practices.
Overview of Google Authentication
Google Auth serves as a means for users to access applications securely. By leveraging their Google accounts, users enjoy a streamlined login experience. Many applications use this strategy for several reasons:
- Security: Enhanced security features protect sensitive user data.
- Familiarity: Users are familiar with Google’s login interface, making it a comfortable choice for many.
- Convenience: One-click sign-ins reduce the friction often associated with account setups.
Understanding these advantages lays the groundwork for integrating Google Auth into an Android application.
Steps for Integration
To successfully integrate Google Authentication, developers need to follow a specific set of steps. These can be broken down as follows:
- Set Up Firebase Project: Start by creating a new Firebase project. Firebase simplifies the integration process for Google Auth.
- Enable Google Sign-In: In the Firebase console, enable the Google Sign-In method under the Sign-In method tab.
- Add Dependencies: Include necessary libraries such as in your project's file.
- Implement Code: Write the necessary code to handle authentication in your Android application's activity or fragment.
Here is an example code snippet:
- Test Authentication: Conduct thorough testing to ensure functionality before deploying.
Common Challenges
While implementing Google Authentication, developers may encounter challenges. Here are a few common issues and their solutions:
- API Key Issues: Ensure the API key is correctly set up and enabled in the Google Developers Console.
- User Experience Concerns: Customize the login interface to match app branding while keeping it recognizable to users.
- Error Handling: Implement adequate error handling to manage failed sign-in attempts gracefully.
A well-implemented authentication system is essential for user trust and data security.
Best Practices
When working with Google Authentication, adhering to best practices can significantly enhance the security and usability of the application:
- Regularly Update Libraries: Ensure that you are using the latest versions of libraries to avoid security vulnerabilities.
- Implement Two-Factor Authentication: Encourage users to activate two-factor authentication for added security.
- User Data Protection: Always prioritize user data protection in any authentication process.
Understanding the complexities involved in integrating Google Auth into an Android application is crucial. This overview provides developers with a clear pathway to implement secure authentication while acknowledging challenges and best practices along the way.
By focusing on specific implementation details and the essence of Google Authentication, this article will empower developers with the necessary knowledge to enhance their applications significantly.
Prelude to Google Authentication
In the modern digital landscape, where security breaches occur frequently, user authentication has become a cornerstone of application development. Google Authentication plays a vital role in strengthening the security framework of Android applications. It provides developers an efficient way to ensure that user identities are verified with a trusted system, which is crucial for safeguarding sensitive information such as personal data and payment details.
Understanding Google Authentication facilitates a smoother user experience while allowing developers to implement robust security measures. This article emphasizes the significance of Google Auth, its core mechanics, and how it can be integrated seamlessly into Android applications. By leveraging this authentication method, developers can enhance user trust, ultimately boosting engagement and retention.
Overview of Authentication Mechanisms
Authentication mechanisms evolve to meet the challenges posed by cyber threats. At the forefront is OAuth 2.0, the foundation of Google Authentication. OAuth 2.0 allows third-party services to exchange information on behalf of a user without exposing sensitive credentials. Other notable mechanisms include Basic Authentication, where credentials are sent with each request, and JSON Web Tokens, which enable efficient stateless authentication. These approaches illustrate the diversity of solutions available for user authentication, with Google Auth standing out due to its ease of integration and extensive support.
Importance of Secure Authentication
Security is paramount in today's digital age. With increasing instances of data breaches, employing secure authentication methods is not optional but a necessity. Secure authentication minimizes vulnerabilities, ensuring that only authorized users gain access. Google Authentication employs multi-factor authentication, which adds an additional layer of security by requiring users to provide something they know and something they have.
"Utilizing secure authentication practices safeguards user data and fosters user trust."
Additionally, secure authentication can significantly reduce instances of account breaches and fraud, ensuring a safer user experience in applications. Given the rise of mobile devices, protecting user information with technologies like Google Auth is more important than ever.
As users become more aware of the implications associated with account security, implementing strong authentication measures is not just a backend requirement but a front-line strategy to enhance user satisfaction and loyalty.
Understanding Google Auth
Understanding Google Authentication is crucial for developers working with Android applications. It provides a streamlined and secure way to manage user identities, facilitating various functionalities that bolster user experience. Employing Google Auth enhances security, as it minimizes the risks associated with traditional username and password combinations. It also allows developers to leverage Google's extensive identity verification infrastructure, providing users with a reliable mechanism to authenticate themselves.
Implementing Google Auth creates several benefits. Firstly, it enhances user engagement by simplifying the sign-in process. Users can avoid the hassle of creating and remembering complex passwords. Instead, they can use existing Google accounts, thus making the entry barrier lower. This convenience often translates to higher conversion rates in applications, as users are more likely to engage when the process is straightforward.
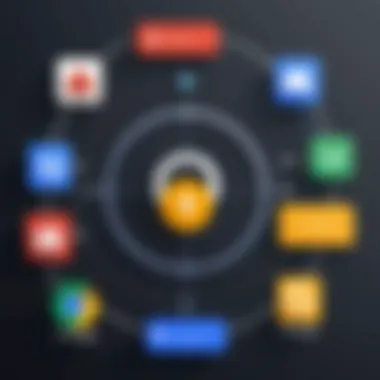
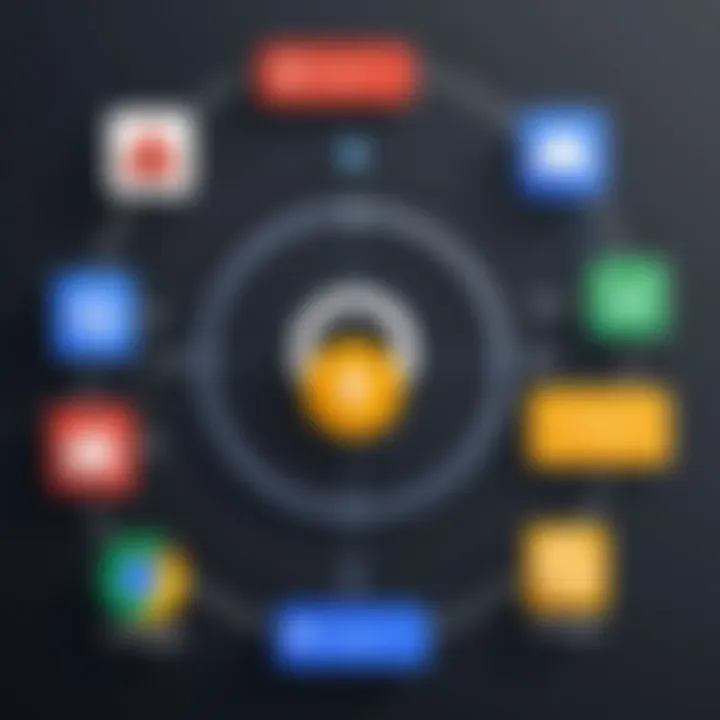
Moreover, Google Auth supports various social features that an application might want to implement. It allows for access to users' Google services, providing rich data that can improve the application functionality. For instance, access to Google Drive can enable users to store data in the cloud seamlessly.
Overall, understanding Google Auth is not only beneficial for ensuring secure authentication but also for augmenting user experience and expanding application capabilities. This knowledge is essential for modern development practices and can critically influence the success of an Android application.
What is Google Authentication?
Google Authentication is a secure identity verification process that allows users to log in to applications using their Google accounts. It is part of the Google Identity platform, designed to facilitate easy and secure sign-ins. This authentication mechanism leverages OAuth 2.0, enabling third-party applications to access user information without exposing sensitive details, such as passwords.
With Google Authentication, users can authenticate across multiple platforms with a single sign-in. This can be particularly beneficial in scenarios where users switch between devices or applications. By employing this method, developers can ensure that users have a hassle-free experience, minimizing friction when accessing crucial app functions.
Core Features of Google Auth
Google Auth comes with a set of core features designed to enhance both security and user experience. Some of these features include:
- OAuth 2.0 Protocol: This is a key component that allows secure delegated access. It enables applications to authenticate and authorize users without requiring them to share their passwords.
- Single Sign-On (SSO): Users can sign in once and gain access to multiple services without repeated logins. This increases user retention and satisfaction.
- Two-Factor Authentication (2FA): A layer of security is added when users need to verify their identity through a secondary method, like a text message or authentication app.
- Easy Integration: Google provides comprehensive libraries and APIs, which make it straightforward for developers to integrate authentication services into their applications.
Implementing these features allows developers to create a secure environment that safeguards user data while enhancing usability. The efficiency and security of Google Auth align closely with modern app development standards, making it an essential integration for Android developers.
Setting Up Google Auth in an Android Project
Setting up Google Auth in an Android project is a crucial step for any developer looking to enhance user experience and security. This process allows Android applications to utilize Google’s secure and efficient sign-in mechanisms. Implementing Google authentication not only simplifies the login process for users, but it also leverages Google’s robust security infrastructure, protecting sensitive user data against various threats. The significance of this setup flows from its impact on user trust, ease of access, and overall app credibility.
Prerequisites for Implementation
Before diving into the technical aspects of implementing Google Auth, certain prerequisites must be met. First, developers should have an active Google account. This account is essential for accessing the Google Cloud Console, where they will create a new project for their application. Furthermore, a basic understanding of Android development is necessary. Familiarity with Android Studio is also recommended. It is important to ensure that the Android device or emulator used for testing has Google Play services installed and updated.
Configuring Google Console
Configuring Google Console is the next significant step. This process involves several steps:
- Create a New Project: Go to the Google Cloud Console, sign in, and create a new project. This is where your app will be associated with its corresponding APIs.
- Enable Google Sign-In API: Once the project is created, enable the Google Sign-In API to allow your app to authenticate users through their Google accounts.
- Set Up OAuth Consent Screen: Define how your app will be presented to users during the sign-in process, including branding information and permissions requested.
- Create Credentials: Generate OAuth 2.0 credentials for your project. This includes obtaining a client ID and client secret, essential for the authentication process.
By following these steps, developers ensure the application is properly configured to communicate with Google’s authentication services.
Adding Required Dependencies
After configuring Google Console, the next step is to add necessary dependencies to the Android project. This involves modifying the file to include the Google Play Services libraries, specifically the library. Here’s how it can be done:
This dependency is crucial for enabling Google Sign-In within your application. Always check for the latest version to ensure compatibility and receive updates and improvements.
"Integrating Google Auth is more than just a technical requirement; it is about enhancing user experiences and building trust through secure authentication."
By understanding these foundational aspects, developers place themselves on the right path to leveraging the advantages of Google Auth effectively.
Implementing Google Auth in Android
Implementing Google Auth in Android applications is an integral aspect of ensuring secure and efficient user authentication. It allows developers to protect user data and streamline the sign-in process. The implementation of Google Auth not only enhances user experience through quicker access but also improves application security. By integrating this authentication solution, developers can leverage Google's extensive infrastructure to manage user identities. This article will delve into several vital components of implementing Google Auth, focusing on creating sign-in options, designing the sign-in user interface, and handling sign-in results effectively.
Creating Sign-In Options
Creating effective sign-in options is crucial for user engagement and ease of use. Google provides various sign-in methods that developers can utilize, such as using a Google account or incorporating multi-factor authentication to enhance security. When defining sign-in options, it is essential to understand the target audience and their preferences. By providing a familiar and seamless sign-in experience, apps can reduce friction and encourage user retention. Developers need to use the Google SignInOptions class to configure these sign-in methods. The configuration can be customized to include email addressing, profile access, or even token scopes for specific data permissions.
Building the Sign-In UI
Once sign-in options are established, the next step involves building the sign-in interface. A well-designed user interface (UI) is essential for guiding users through the authentication process. This means not only creating visually appealing layouts but also ensuring that the interactions are intuitive. Google's Auth UI provides predefined methods, such as the SignInIntent, which simplifies UI implementation. Developers should ensure that their sign-in UI reflects the overall app branding while remaining user-friendly. Providing clear messages about what users need to do at each step can significantly improve the user experience. Key aspects to consider include access to help buttons and clear error messaging should any problems arise during sign-in.
Handling Sign-In Results
Handling the outcomes of a sign-in process is critical for maintaining a smooth user experience. After users attempt to sign in, developers need to implement a method to process the results securely. This often involves using the onActivityResult method to handle the response from the Google Sign-In API. Developers should check if the sign-in was successful and then either proceed to the next steps, such as fetching user data or displaying an error message.
It is essential that developers properly manage the sign-in results to ensure scenario coverage, whether success or failure. Implementing appropriate feedback messages will help inform users of their status. This can include displaying confirmation of successful authentication or asking users to retry when issues arise.
"A seamless sign-in experience not only enhances usability but also boosts user trust in the application."
In summary, implementing Google Auth in Android applications is vital for creating a secure and user-friendly experience. This ensures that developers can manage user identities efficiently, reducing the risks associated with user authentication.
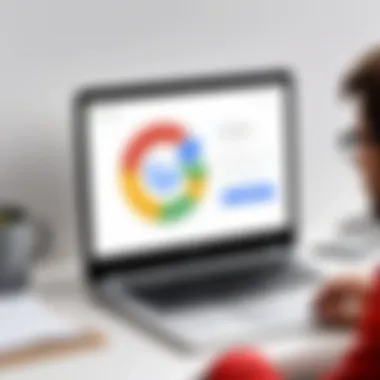
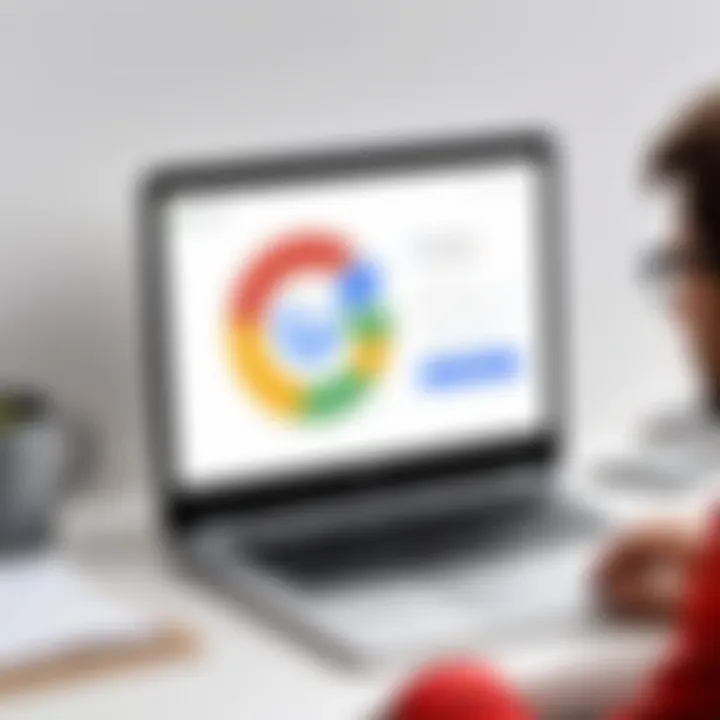
User Session Management
User session management is a critical component in the realm of mobile application development. Particularly when dealing with Google Authentication, understanding how to effectively manage user sessions can enhance the security and overall user experience in your Android applications. It enables developers to maintain user state, ensuring that users do not have to authenticate every time they engage with the app. This promotes a seamless experience while also addressing security concerns inherent in mobile applications.
Maintaining User Sessions
To maintain user sessions, developers typically utilize session tokens or management tools provided by Google. These methods allow applications to remember user credentials, effectively keeping them logged in, until a particular circumstance, such as session expiration or manual log-out, disrupts that state.
The benefits of efficient session management include:
- Improved User Experience: Users can engage with the application without repeated log-ins. This enhances user satisfaction and retention.
- Reduced Server Load: By keeping sessions active for longer, developers minimize the number of authentication requests sent to servers. This results in less strain on backend infrastructure.
- Flexible Security Options: Developers can implement timeout policies that require re-authentication after certain periods of inactivity. This provides a balance between convenience and security.
When implementing session management, consider the storage of session tokens. These tokens should be securely stored, possibly in SharedPreferences or encrypted storage, to mitigate any potential security vulnerabilities that may arise from unauthorized access.
Signing Out Users
The signing-out process is as crucial as maintaining user sessions. It serves multiple purposes, including enhancing security and ensuring that personal information is not accessible to unauthorized users. Proper sign-out mechanisms prevent issues such as data leaks or unauthorized access if the device is shared.
To facilitate a sign-out process using Google Authentication, developers can implement the following steps:
- Clear Session Data: Upon sign-out, it's vital to remove any cached tokens or session information from memory. This ensures that the application does not continue to act as if the user is still logged in.
- User Interface Feedback: Provide clear feedback indicating that the user has successfully signed out. This reinforces the action and helps mitigate confusion.
- Redirect to Login Screen: After signing out, redirect users to the login screen where they can enter their credentials again if they wish to log back in.
To illustrate the sign-out process in code, here’s a simple example:
Effective user session management and sign-out processes are essential to maintaining both user satisfaction and application security. Developers must not overlook these elements when integrating Google Authentication into their apps.
Overall, user session management lays the groundwork for a secure and user-friendly app experience. Balancing accessibility with security requires thoughtful consideration and implementation of appropriate methods.
Best Practices for Google Auth Implementation
Implementing Google Authentication in Android applications demands attention to best practices. Adopting strong practices not only enhances security but also improves user experience. Ensuring a seamless login process fosters trust and encourages repeated app usage. Given the rising threats from cyber attacks, developers must prioritize best practices in Google Auth. These standards can shield applications from vulnerabilities and provide a resilient authentication mechanism. The following sections delve into critical best practices for developers.
Ensuring Secure Data Transmission
Secure data transmission is a fundamental aspect of any authentication process. When users sign in, sensitive information such as credentials are exchanged. Developers must employ transport layer security (TLS) to safeguard this data from potential interception.
Here are key strategies to ensure secure data transmission:
- Use HTTPS: This protocol encrypts the data exchanged between the client's device and the server. It creates a secure tunnel, which prevents unauthorized access to user information.
- Validate SSL Certificates: Proper validation helps confirm that the application communicates with the intended server. Without this step, developers risk exposing users to man-in-the-middle attacks.
- Regular Security Audits: Conducting audits can help identify weak spots in the security framework. It is vital to update frameworks and libraries regularly, as vulnerabilities can emerge over time.
Implementing these practices ensures that user credentials and other sensitive data remain protected throughout the authentication process.
Leveraging Token Expiration
Token expiration acts as a defense mechanism against unauthorized access. In Google Authentication, tokens often have a limited lifetime. Developers must utilize this feature to enhance application security.
Here are some considerations regarding token expiration:
- Set Appropriate Expiry Times: Tokens should not last indefinitely. Generally, short-lived tokens enhance security by reducing the risk of token theft. However, they should balance user convenience with security.
- Implement Refresh Tokens: If a token expires, users should not be required to sign in again every time. Refresh tokens allow seamless access without frequent re-authentication while maintaining security protocols.
- Monitor Token Activity: Keeping track of token usage can alert developers to unusual patterns. If a token is used from multiple locations simultaneously, it may indicate an issue that requires immediate attention.
By leveraging token expiration adequately, developers can significantly bolster the security posture of their applications while ensuring that user experience does not suffer.
Following these best practices not only helps in securing the authentication process but also builds user confidence, reassuring them that their data is handled with care.
Troubleshooting Google Auth Issues
Troubleshooting Google Auth issues is a crucial aspect of integrating authentication in Android applications. While Google Auth is designed to be user-friendly, challenges can arise during implementation or runtime that can hinder user experience. Understanding these issues is essential for developers to maintain a seamless authentication process. This section will cover common errors one might encounter and methods to resolve configuration issues that often plague new integrations.
Common Errors with Google Sign-In
When implementing Google Sign-In, developers may face various common errors. Some of the frequent issues include:
- Permissions Not Set: Ensure the necessary permissions are declared in the Android manifest. Missing permissions can lead to authentication failures.
- SHA-1 Certificate Fingerprint Issues: The SHA-1 fingerprint must be correctly configured in the Google Cloud Console. Mismatched fingerprints can result in authentication errors during sign-in.
- Wrong Client ID: If the client ID configured in the project does not match the one in the Google Developer Console, access will be denied. This is a common error that can be easily overlooked.
- User Cancellation: If a user chooses not to sign in, a cancellation error occurs. This can happen for various reasons during the sign-in process, and it is important to handle it gracefully in the app.
- Network Issues: Sometimes, connectivity problems may prevent the app from reaching Google's servers. Always check the status of network connections when debugging authentication issues.
Developers must carefully handle these errors to provide meaningful feedback to users. This enhances the user experience and reduces frustration.
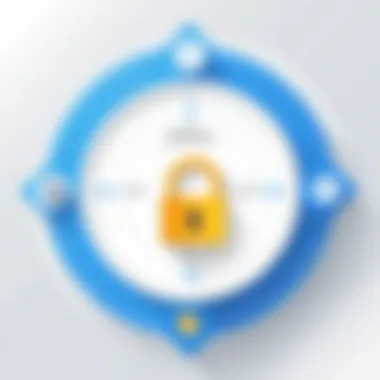
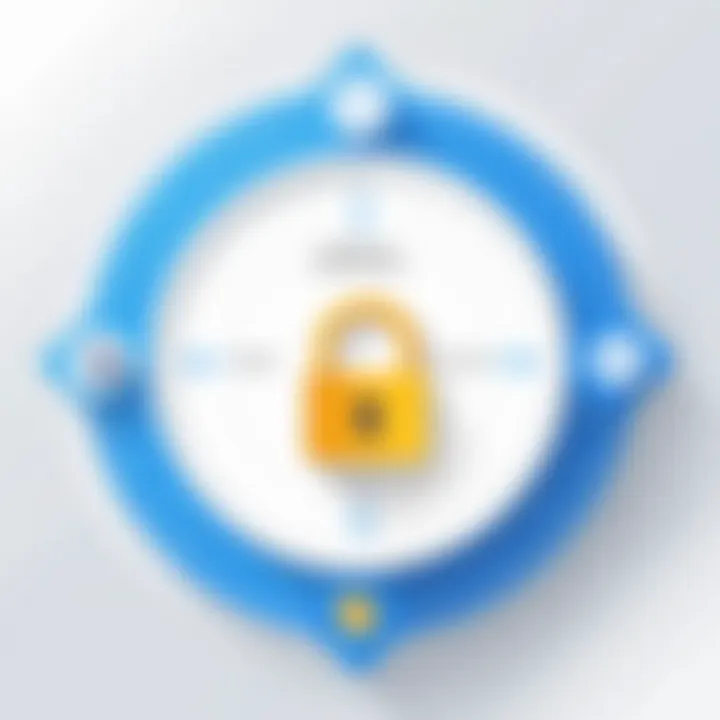
Resolving Configuration Issues
Configuration issues can often result from improper setup in the Google Cloud Console or in the Android project settings. Here are some strategies for resolving these issues:
- Verify API Access: Check if the required APIs are enabled in the Google Cloud Console. Access to the Google Sign-In API is essential for authentication.
- Examine OAuth Consent Screen: Ensure the OAuth consent screen is configured correctly with required details. This includes setting up the application name, logo, and other required fields for a smooth user experience.
- Check Package Name: The package name in the Google Developer Console should match the one in your Android project. Any mismatch will lead to authentication issues.
- Inspect JSON Configuration: The configuration file (google-services.json) must be placed in the correct location within the app. This file contains vital information needed for authentication processes.
- Update Dependencies: Always ensure that the Google Play Services dependencies are up to date in your Gradle file. Using deprecated or outdated versions can lead to unexpected errors.
By attentively reviewing each aspect of the configuration, developers can eliminate many common pitfalls. A strategic approach to troubleshooting will significantly improve the overall user experience and make the app more reliable.
"Debugging is being the detective in a crime movie where you’re also the murderer." - Unknown
Comparing Google Auth with Other Authentication Solutions
When discussing authentication mechanisms, it is vital to understand the landscape of existing solutions. This section will compare Google Auth with other prominent options, specifically Facebook Auth and traditional Email-Password systems. Each method has its own strengths and weaknesses, influencing developers' choices based on application requirements, user preferences, and security considerations.
Google Auth vs. Facebook Auth
Both Google and Facebook provide solid authentication frameworks, but there are several nuances that set them apart.
- User Base:
Google Auth leverages the vast user base of Google accounts, which are ubiquitous across various services. In contrast, Facebook Auth primarily attracts users heavily involved in social media. - Security Standards:
Google places a strong emphasis on security. With features like two-factor authentication, Google may appeal more to security-conscious users. Facebook also has security measures, but incidents in the past have raised concerns about user data protection. - Integration Complexity:
Implementing Google Auth often provides a more straightforward process due to strong documentation and community support. Facebook’s APIs can sometimes be more complex, leading to potential hurdles during integration. - Permissions and Data Access:
Google Auth gives applications access to a limited set of user data, maintaining user privacy. On the other hand, Facebook usually encourages apps to request broad permissions to enhance user engagement, which can be a double-edged sword.
"When you choose an authentication method, consider the specific needs of your app as well as your user audience. Balance between security, user experience, and data privacy is key."
Google Auth vs. Email-Password Auth
The traditional email-password method has been a standard for years, but it faces challenges that newer methods like Google Auth can address.
- User Experience:
Google Auth provides a seamless signing experience, especially for users with existing Google accounts. Email-password authentication often leads to user frustration due to common issues like forgetting passwords and account recovery challenges. - Security:
Google Auth incorporates modern security protocols that reduce the risk of common vulnerabilities such as phishing. Email-password authentication is inherently weaker, as it depends on user-selected passwords, many of which may be weak or reused across different services. - Management Complexity:
Developers using Google Auth can offload much of the authentication workload to Google’s infrastructure. In contrast, managing password storage, encryption, and recovery mechanisms remains the responsibility of the developers when using email-password systems. - Regulatory Compliance:
Oftentimes, Google Auth complies with various regulations like GDPR out of the box due to its infrastructure. With email-password systems, developers must ensure their mechanisms meet such regulations themselves.
Future Trends in Authentication
The landscape of user authentication is rapidly evolving due to advances in technology and the growing need for secure systems. In this section, we will explore the future trends in authentication, particularly their significance in enhancing security and user experience in Android applications. Understanding these trends is vital for developers. They must anticipate changes and adapt their applications accordingly.
Emerging Authentication Technologies
Several emerging technologies are shaping the future of authentication. These technologies aim to address the weaknesses of traditional methods and increase security against hacking attempts. Some noteworthy technologies include:
- Blockchain: This decentralized technology is gaining traction as it provides a secure way to verify identities without relying on a central authority. With blockchain, every transaction is recorded on a public ledger, reducing the chances of fraud.
- Smart Cards: These small devices can store cryptographic keys, making authentication faster and more secure. Smart cards are especially useful in corporate environments where secure access is critical.
- Two-Factor Authentication (2FA): While already common, 2FA continues to evolve. New methods, such as time-based one-time passwords and hardware tokens, enhance security by requiring multiple forms of verification.
The adoption of these technologies can provide better security and a smoother user experience. For developers, staying updated on these trends can lead to more robust authentication systems in their applications.
The Rise of Biometric Authentication
Biometric authentication is increasingly becoming a popular method for verifying identities. It utilizes unique biological traits such as fingerprints, facial recognition, and iris scans to grant access.
The advantages of biometric authentication are substantial:
- Convenience: Users find it easier to use their fingerprints or face instead of remembering complex passwords.
- Accuracy: Biometrics are harder to replicate compared to passwords, making unauthorized access more difficult.
- Speed: Authentication is faster. A simple scan or touch is often all that is needed.
However, developers should consider some challenges related to biometric authentication:
- Privacy Concerns: Users may be hesitant to share their biometric data. Clear communication regarding data usage is essential.
- False Acceptance/ Rejection Rates: No biometric system is entirely foolproof. Developers must choose reliable technologies to minimize errors.
As biometrics continue to advance with improvements in machine learning and data processing, they present an excellent opportunity for Android applications to enhance security measures.
The future of authentication is not just about improved security. It also focuses on creating a seamless user experience.
Ending
The conclusion serves an essential purpose within this article, tying together the central themes discussed throughout the various sections. Summarizing the critical aspects of Google Authentication for Android applications is important in reinforcing the knowledge gained by the reader. Understanding the mechanisms, advantages, and implementation practices helps developers create secure and user-friendly applications.
Recap of Key Points
In this article, we examined:
- The fundamental concepts behind Google Authentication, highlighting its definition and core features.
- The steps required to set up Google Auth in an Android project, starting from the prerequisites to the configuration in Google Console.
- The process of implementing Google Auth, including creating sign-in options, building the sign-in UI, and handling results effectively.
- User session management practices, emphasizing the importance of maintaining and signing out users securely.
- Best practices for ensuring secure data transmission and leveraging token expiration to enhance security.
- Troubleshooting methods for common errors and configuration issues.
- A comparative analysis of Google Auth with other authentication solutions, such as Facebook Auth and email-password methods.
- The future trends in authentication, focusing on emerging technologies and biometric solutions.
Concisely stating these points allows readers to understand the scope of Google Auth implementation in Android applications.
Final Thoughts on Google Auth
Amid the growing focus on cybersecurity, utilizing Google Authentication is no longer just an option but a necessity for Android developers. The emphasis on secure authentication cannot be overstated. Google Auth not only simplifies the sign-in process for users but also reduces the risks associated with managing passwords. As developers continue to enhance their applications, integrating robust authentication methods like Google Auth can significantly increase user trust and engagement. The insights shared in this article equip developers with practical knowledge to navigate the complexities of secure user authentication.
"The effectiveness of an application is often measured by its security; understanding Google Auth is a step towards greater protection for both developers and users."
By adopting these practices, developers can position themselves at the forefront of secure application development.