Understanding Function Calls in Python: A Comprehensive Guide
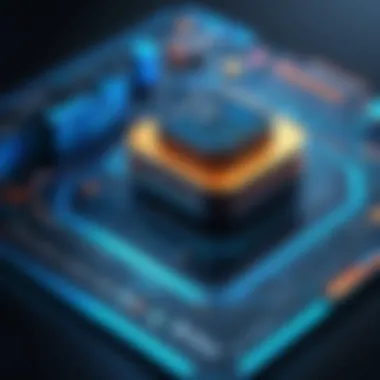
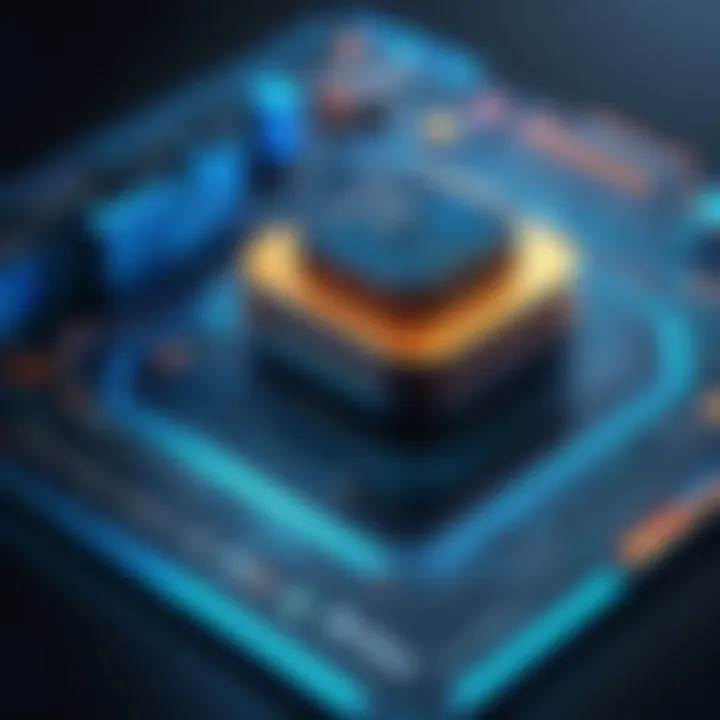
Intro
Importance of Function Calls
Function calls are significant because they help streamline code. By breaking down tasks into functions, programmers can avoid redundancy and improve code organization. Functions can handle various parameters and return different types of values, making them versatile tools in programming. This guide aims to equip you with the knowledge necessary for effective function utilization in Python.
Structure of the Article
- Defining Functions: Understanding how to create a function, including syntax and conventions.
- Function Parameters: Exploring different types of arguments and how to manage them.
- Return Values: Discussing ways to return data from functions.
- Advanced Concepts: Including higher-order functions and lambda expressions.
- Practical Applications: Hands-on examples and coding projects to solidify concepts.
- Learning Resources: Recommendations for further learning to deepen your programming skills.
Looking ahead, readers can expect a thorough breakdown of each aspect of function calls at a level suitable for beginners and intermediate programmers. This insight will help reinforce fundamental strategies and integrate them into real-life coding scenarios.
Intro to Functions in Python
Functions are a fundamental aspect of programming in Python. They provide a structured way to group a set of related instructions that perform a specific task. This organization not only makes code more readable but also facilitates reuse and maintenance. Understanding functions is critical for anyone looking to improve their coding skills or create complex programs.
Functions in Python can be defined using a simple syntax, but mastering them requires more than just knowing how to write them. One must grasp the different ways functions can be invoked, the significance of parameters, and the handling of return values. This article will cover these essential topics in a comprehensive manner.
In programming, the use of functions allows for better management of code. They can reduce redundancy by eliminating the need to write the same code multiple times. Functions can also enhance collaboration in coding projects by allowing developers to work on different parts of a program independently.
Additionally, understanding functions deepens one’s problem-solving abilities. It enables programmers to break down complex problems into smaller, more manageable parts. By studying functions thoroughly, one can gain insights into higher-level programming concepts that build upon these basics.
"Well-crafted functions lead to clean and efficient code, making programming a more gratifying endeavor."
In summary, gaining knowledge about functions lays a solid groundwork for further exploration of Python. Mastery of functions empowers students and programmers alike to write organized, effective code and become proficient in the language.
Defining a Function
Defining a function in Python is a fundamental concept that serves various key purposes in programming. Functions are blocks of reusable code that perform a specific task. They help organize code into manageable, logical sections, making it easier to read and maintain. The act of defining a function enables programmers to encapsulate functionality and reuse it throughout their projects, thus reducing redundancy and improving efficiency.
Moreover, functions allow for better abstraction in programming. By using functions, developers can focus on the high-level operations of their code rather than getting bogged down in the details of the implementation every single time. This enhances problem-solving skills and facilitates collaborative work, as different programmers can work on separate functions independently.
When defining a function, one must adhere to certain criteria, including a clear naming convention. A well-named function communicates its purpose effectively and contributes to the overall readability of the code. Furthermore, considering the need for parameters and return values during the definition stage is essential for the function's utility.
In summary, defining a function is not merely about writing code; it is about increasing the clarity, efficiency, and maintainability of software applications. This significantly impacts both development speed and code quality.
Calling a Function
Understanding how to call a function is crucial for any Python programmer. It is the mechanism that activates the code that resides within a function. When you call a function, you essentially instruct the interpreter to execute its body. This section covers the basic elements of function calls, explains the benefits, and discusses considerations that can shape how you effectively employ this programming feature.
Basic Function Call
A basic function call in Python involves using the function name followed by parentheses. The parentheses can enclose arguments that the function requires. For example, if you have defined a function named , you would call it as follows:
In this example, a function is instructed to execute with two parameters. It's essential to remember that the function must be defined before you call it. Attempting to call a function before it's defined will result in a . This basic understanding sets the stage for following the more complex aspects of function calls, notably how to pass information effectively.
Using Positional Arguments
Positional arguments are a straightforward way to provide input to functions. They rely on the order in which parameters are defined in the function. For instance, in a function defined as , the first argument corresponds to , and the second to . Hence, calling will assign 3 to and 4 to . If you change the order while calling the function like , the output changes accordingly, as the positional values are crucial to how the function processes inputs.
Using these arguments effectively enhances clarity. They foster readable code, as it's easier to discern which arguments correspond to which parameters through their sequence. However, this method can lead to confusion if a function has many parameters. Developers must remember the order or consult the function definition regularly.
Using Keyword Arguments
Keyword arguments improve the clarity of function calls, particularly with functions that have multiple parameters. When calling a function with keyword arguments, you specify a parameter name followed by its value. For example, if you have the same function, you can call it using:
In this case, it does not matter the order of the arguments, as you are referring to each parameter by name. This makes the call more readable and reduces the probability of making mistakes. Furthermore, keyword arguments allow you to skip optional parameters, which helps in navigating functions with numerous inputs. As a best practice, combining positional and keyword arguments can be advantageous, particularly when reading and maintaining code.
Return Values in Functions
Understanding return values in functions is crucial for anyone looking to grasp the full potential of Python programming. Return values determine what a function sends back to the caller after execution. This is important as it allows programmers to manage data flow in applications effectively. Functions without return values might appear less useful since they would not contribute any output after processing. This would limit their reusability and effectiveness in various scenarios.
The significance of return values can be seen in multiple aspects. Firstly, they enhance modularity by allowing you to break down complex problems into simpler, reusable components. This clarity in function design leads to improved maintainability of code. Secondly, return values provide a straightforward means to pass data between functions, thus promoting smoother integration within larger codebases. Lastly, understanding how to handle return values leads to more efficient memory management, as you can control when and how data is retained in memory.
Understanding Return Statements
A return statement in Python is where a function concludes its execution and sends a value back to the point where it was called. It serves as the exit point of a function, marking where control returns to the caller. The basic syntax of a return statement is simple:
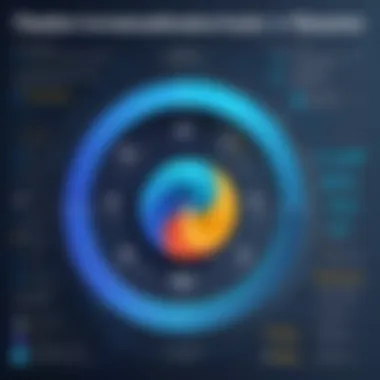
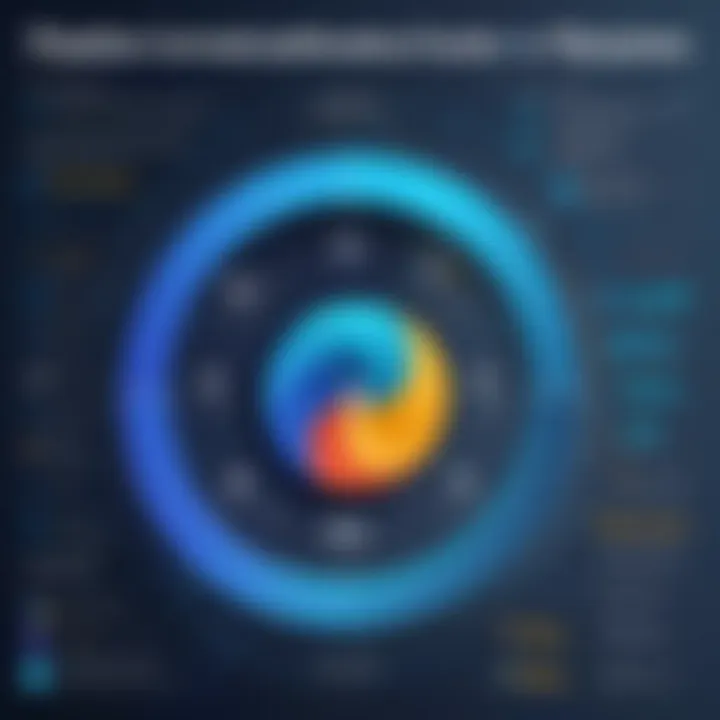
Here, can be any valid Python object such as a number, string, list, or even another function. When the return statement is executed, the function ceases to run further statements and exits, allowing the returned value to be used elsewhere in the program.
Consider the following example:
In this example, the function computes the sum of and , then uses the return statement to send this value back. The value stored in can now be utilized in other calculations or displayed, showcasing the importance of return values effectively.
Returning Multiple Values
Python's functions can return more than one value simultaneously. This is done by separating the values with a comma in the return statement. Returning multiple values is beneficial in numerous contexts, such as when different data outputs are needed at once.
When returning multiple values, Python encapsulates them into a tuple, which can be unpacked later. Here’s an example:
The function computes both the minimum and maximum values from the provided list. The function returns two values, both accessible to the caller, demonstrating how Python simplifies handling multiple outputs easily.
This ability to return multiple values enhances flexibility and makes functions more powerful and expressive.
In summary, understanding return statements and the capability to return multiple values are foundational elements in maximizing the utility of functions in Python. This knowledge reflects on how data is handled within your program, allowing for better code organization and reusability.
Scope of Variables
Understanding the scope of variables is crucial in Python programming. It refers to the visibility or accessibility of variables in different parts of the code. The scope determines where a variable can be used, modified, or referenced. This concept becomes particularly significant when dealing with functions, as it affects the behavior of those functions and the data they utilize.
The two main types of variable scopes in Python are local and global. Each type serves its purpose and comes with its rules, which can impact how developers structure their programs. For beginners, grasping the differences can lead to fewer errors and more efficient code.
Moreover, understanding scope can help prevent unintended side effects during function execution. A common pitfall in programming is conflicting variable names, which can arise if a global variable is shadowed by a local variable within a function. This makes it vital to comprehend how variable scopes interact with function calls and data handling.
Local vs Global Variables
Local variables are defined within a function and can only be accessed from within it. They exist in memory only while the function is running. Their lifespan is limited, which is practical for managing temporary data. For example, consider the following code:
In contrast, global variables are defined outside of any function and can be accessed anywhere in the script, including inside functions. They maintain their value throughout the runtime of the program. However, they can be modified using the global keyword, which we will discuss next. It is important to be careful with global variables since excessive use can lead to code that is hard to debug and maintain.
Using Global Keyword
When a function needs to modify a global variable, Python requires the use of the global keyword. This keyword tells the interpreter to use the variable defined in the global scope. Without it, any assignment to that variable within the function will create a new local variable instead of modifying the global one.
Here is an example to illustrate this:
Overusing global variables can lead to complicated relationships between components of a program. Good practice suggests limiting their use and maintaining the clarity of function interactions.
"Understanding variable scope is not just about preventing errors; it's about writing clean, maintainable code that is easy to follow and debug."
Lambda Functions
Lambda functions are a significant concept in Python, providing programmers with a powerful tool for creating quick, small functions without the necessity of formally defining them using the standard keyword. This section aims to elucidate the importance of lambda functions, their syntax, and their practical applications.
Definition and Syntax
A lambda function in Python, also known as an anonymous function, is defined using the keyword. It can take any number of parameters but can only have a single expression. The result of this expression is automatically returned. The syntax for a lambda function is straightforward:
For example, a simple lambda function that adds two numbers could be written as:
In this case, and are the parameters, and is the expression that computes the sum when the function is called. This compact form allows for constructing functions that may not need a dedicated name or formal structure, enhancing code readability and maintainability in specific contexts.
When to Use Lambda Functions
Lambda functions are particularly useful in scenarios where a full function definition would be unnecessary or impractical. Here are some situations where you might consider using lambda functions:
- Short-lived Functions: If a function will be used only once, defining it with can be overkill. A lambda provides a convenient one-liner.
- Functional Programming: Lambda functions work seamlessly with higher-order functions such as , , and . They allow transformations and operations on iterables without the need for verbose code.
- Callbacks: In certain cases, especially when working with graphical user interfaces or asynchronous programming, lambda functions can serve as quick callbacks, reducing boilerplate code.
Higher-Order Functions
Higher-order functions are an essential concept in Python programming, allowing developers to write more flexible and reusable code. Understanding this topic enriches a programmer's skillset by introducing ways of manipulating functions like any other data type. In essence, a higher-order function can either accept one or more functions as arguments or return a function as its result. This ability to work with functions in a more abstract manner opens many paths for functional programming techniques.
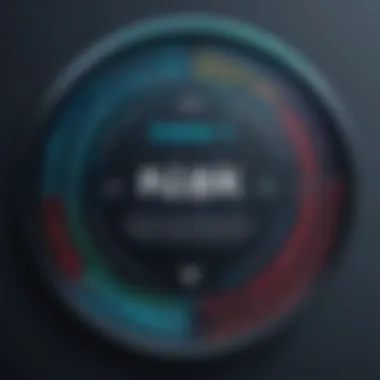
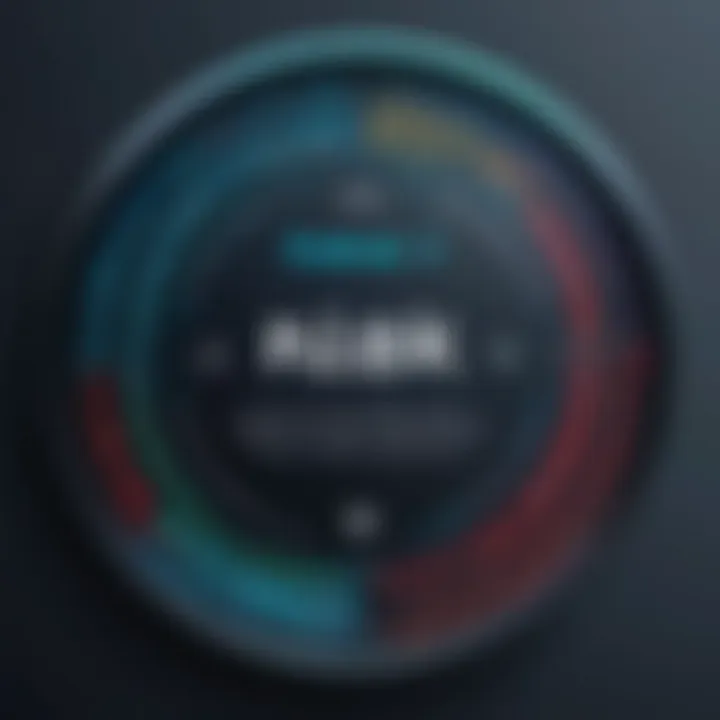
Definition and Examples
A higher-order function, as defined, operates on another function. Common built-in examples in Python include , , and . Each of these functions takes a function as an argument and applies that function to a set of data.
For instance, consider the following example that illustrates the use of the function:
In this code, takes a lambda function (a type of anonymous function) as an argument, which squares each number in the list. The result is a list of squared numbers.
This ability to manipulate functions allows for cleaner code and greater separation of concerns. It simplifies the programming logic, because functionality can be encapsulated within functions that can be reused across the program.
Functions as Arguments
One of the transformative aspects of higher-order functions is the ability to pass functions as arguments to other functions. This concept enhances modularity and increases code reuse.
For example, consider a function that accepts another function to apply it on a list:
In this snippet, takes two parameters: a function and a list. It applies the function to every item in the list, showcasing the flexibility and reusability of functions when used as arguments.
By leveraging higher-order functions in your programming, you unlock numerous possibilities for designing more abstract and generic solutions. This can lead to more maintainable and readable code, especially in larger and more complex projects.
Anonymous Functions
Anonymous functions, also known as lambda functions in Python, are an important concept that facilitates the creation of small, quick functions without the need for a formal definition. This topic is crucial in understanding how functions can be used flexibly and efficiently in programming. The use of anonymous functions can greatly enhance code clarity and brevity, especially when dealing with functions that are simple and only needed temporarily.
They are defined using the keyword, followed by a list of parameters, a colon, and an expression. Unlike regular functions defined using , lambda functions do not have a name, which is where the term "anonymous" comes from. They are particularly useful as throwaway functions, where a full function definition would be unnecessarily verbose.
Characteristics of Anonymous Functions
Anonymous functions have several key characteristics that distinguish them from regular functions:
- Concise Syntax: They are defined in a single line. This makes them easy to write and read when the functionality is straightforward.
- No Name: As mentioned, they do not require a name. This is beneficial in cases where the function is only used once or twice in the code.
- Limited Functionality: Lambda functions can only contain a single expression. This limits their complexity but makes them suitable for simple operations.
- Immediate Context: They are often used in conjunction with higher-order functions, such as , , and . This helps reduce overall code footprint and improves efficiency in executing functional patterns.
"Anonymous functions provide a compact and efficient way to define simple behaviors without the overhead of traditional function definitions."
Use Cases in Python
Anonymous functions can be leveraged in a variety of scenarios within Python programming:
- Sorting Data: When using the method or function, lambda can specify complex sort orders without formal function definitions.
sorted_data = sorted(data, key=lambda x: x[1])# Sort by the second element
- Mapping Values: The function uses lambda to apply a function to all items in an iterable, providing a clean approach for transformations.
squares = list(map(lambda x: x ** 2, numbers))# Squares of numbers
Resolving these errors usually involves a careful review of the code to ensure everything is correctly formatted. Utilizing an Integrated Development Environment (IDE) or code editor with syntax highlighting can help prevent these errors by making the structure of the code more visible.
Type Errors
Type errors arise when an operation or function is applied to an object of an inappropriate type. For example, trying to concatenate a string with an integer will cause a type error. This type of error can be more challenging to diagnose, as it may not surface until the line of code is executed. Some frequent examples include:
- Passing incorrect types of arguments to functions.
- Performing operations between incompatible types, such as multiplying a string by a list.
For instance, executing the following code:
will result in an error:
To handle type errors, it is essential to be aware of the expected types of function parameters. Using function and explicit type casting can help ensure that the right types are used in operations. Utilizing exception handling can also safeguard against type errors, allowing for a smoother user experience when errors do occur.
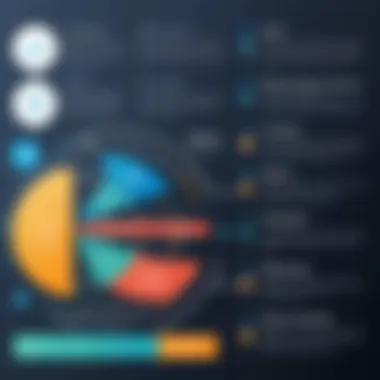
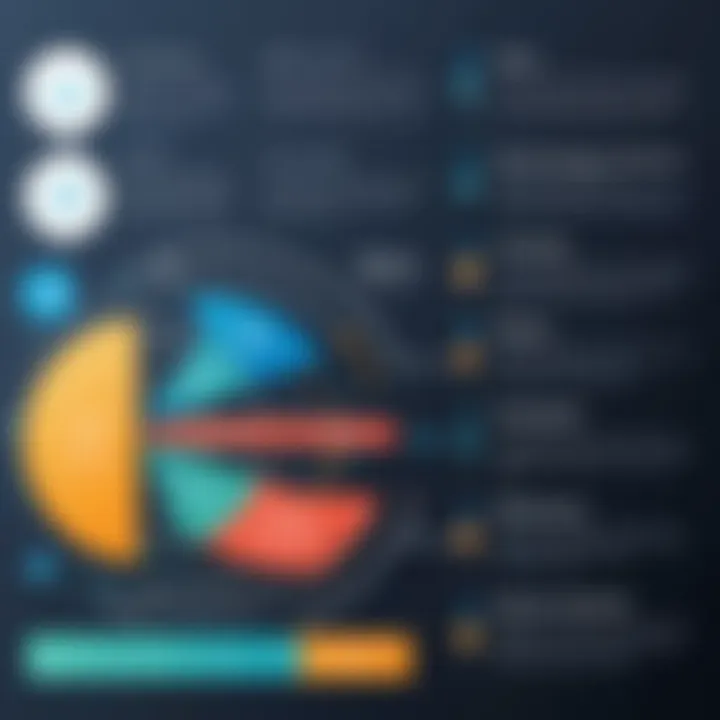
"Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it." - Brian Kernighan
By familiarizing yourself with these errors—syntax and type—you can enhance your ability to write functional Python code efficiently. Recognizing potential pitfalls early on can save extensive troubleshooting later. Understanding how to resolve these errors fosters a more productive coding experience.
Debugging Function Calls
Debugging function calls in Python is an essential aspect of programming. When working with functions, it is easy to encounter errors or unexpected behaviors. Understanding how to effectively debug these calls can significantly enhance coding proficiency.
Debugging helps identify and rectify issues early in the development process. It not only saves time but also improves the quality of your code. A clear understanding of how to debug function calls means you can maintain a robust codebase. Moreover, debugging encourages learning and comprehension of the code's structure and flow. This clarity can lead to more efficient coding practices while minimizing future errors.
Using Print Statements
Print statements serve as a fundamental tool for debugging in Python. They allow programmers to observe the flow of execution and examine variable values at various stages. When you insert print statements before and after function calls, you gain visibility into the program's operations. This can reveal whether a function is being called correctly and what data is being passed.
For instance, if you have a function that add two numbers, simply printing the inputs can clarify whether they are what you expect:
This example will show you the values being added, making it easier to troubleshoot any issues. However, while print statements are instructive, they can clutter your code if used excessively. Thus, they should be applied judiciously.
Utilizing Debugging Tools
Beyond print statements, Python offers several debugging tools that can enhance your debugging process. One of the most popular is the built-in (Python Debugger). This tool allows for an interactive debugging experience. You can set breakpoints, step through code, and inspect variable states at any time.
Using can look like this:
Upon hitting , execution will pause, allowing you to enter commands to explore your program's state. This can be particularly beneficial for complex functions where the flow of execution may not be immediately clear. Besides , there are other tools, like PyCharm’s built-in debugger or Visual Studio Code, which provide graphical interfaces for debugging functions.
Overall, employing debugging tools is about systematically testing and verifying your code. This practice can catch bugs early and clarify the functionality of your functions, leading to improved program quality.
Unit Testing Functions
Unit testing is a crucial process in software development, especially in Python programming. By systematically checking that individual units of code—such as functions—perform as expected, developers can identify and rectify errors early in the development cycle. This section covers the significance of unit testing and provides guidance on implementing tests in Python.
Importance of Unit Testing
Unit testing enhances the reliability of code and ensures that it behaves as intended. This practice offers several specific benefits:
- Early Detection of Bugs: Bugs can disrupt the functionality of an application. By testing individual functions, developers can catch errors before they are widely integrated into the system.
- Code Refactoring with Confidence: When you change an existing function, unit tests provide assurance that alterations do not introduce new issues. This is particularly useful in larger codebases where understanding the effects of changes can be complicated.
- Documentation of Functionality: Unit tests serve as a form of documentation. They clarify the purpose and intended behavior of functions, which can be beneficial for future developers or even for yourself after a time away from the code.
- Improved Design of Functions: Writing tests encourages programmers to create functions that are modular and easier to understand. Good tests often lead to better design practices.
"Effective unit testing is not about finding bugs, but about preventing them from ever reaching production."
Implementing Tests in Python
Python provides several frameworks that simplify unit testing, with being one of the most popular. Implementing unit tests in Python starts with structuring your tests properly.
- Import the Required Module: The first step is to import the module.
- Create a Test Case Class: A test case is a class that inherits from . This class will contain your test methods.
- Define Test Methods: Each method that starts with the word is treated as a separate test. In the test methods, you can call the function you want to test and use assertions to check if the outcomes meet expectations. For instance:
- Run the Tests:
Finally, to execute the tests, you can run:
By following these steps, you can set up effective unit tests that contribute significantly to your coding process. The common conventions and structure provided by Python's framework encourage best practices in software development.
End
The conclusion serves as a pivotal segment of this article. It encapsulates the core concepts explored throughout the text. By summarizing the key points, it reinforces the reader's understanding of function calls in Python. This section is crucial for several reasons.
First, it consolidates learning by highlighting the most important aspects. Readers can easily remember and apply these concepts in their programming practices. Recap of the essential elements further promotes retention, making it easier to reference them during actual coding tasks.
Second, this part of the article can inspire further exploration of Python programming. As readers see how function calls enhance their coding efficiency, they may be motivated to delve deeper into more complex programming topics.
Lastly, it allows the discussion of practical applications and potential pitfalls in function calls, preparing readers to approach their coding projects with caution and awareness. This holistic approach can prepare beginners and intermediate programmers for real-world programming challenges.
Recap of Key Points
- Functions are essential in Python: They provide structure and reusable components in code, making programming more efficient.
- Different types of arguments can be used: Understanding positional and keyword arguments improves function usability.
- Return values enable functions to output data for further use. Knowing how to correctly employ return statements is critical for effective coding.
- Scope of variables is important: It dictates where variables can be accessed within a program, which affects function behavior.
- Higher-order functions and lambda expressions offer advanced capabilities, enhancing the flexibility and power of Python code.
Future Directions in Function Usage
As programming evolves, so will the practices surrounding function calls. Here are some possible future directions:
- Integration with AI and Machine Learning: Functions will likely adapt to accommodate the specific needs of machine learning algorithms and data processing tasks. Programmers will need to learn how to structure functions for efficient data manipulation.
- Improved libraries: The Python ecosystem is constantly growing. New libraries and frameworks will emerge, allowing for more sophisticated function usage in various applications.
- Emphasis on functional programming: While Python supports multiple paradigms, there is a trend towards functional programming. This can drive a deeper understanding of function calls and their role in creating more maintainable and scalable code.
- Focus on code efficiency: As projects increase in scale, the need for faster and more efficient function calls will become critical. Developers will need to optimize their functions for performance.
Through ongoing education and practice, programmers will continue to refine their skills in using functions effectively, ensuring their relevance in the rapidly changing tech landscape.