Mastering the Art of the For Loop in Programming
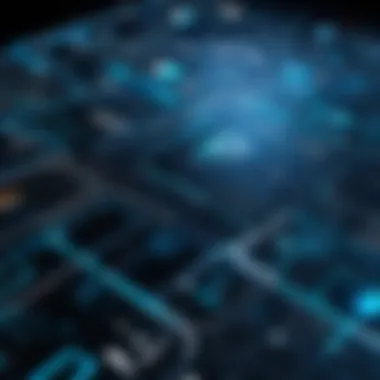
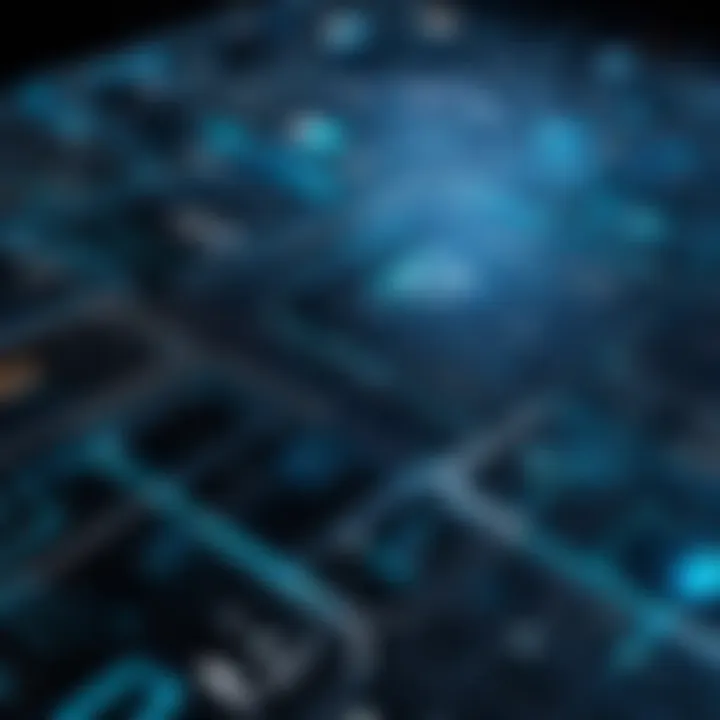
Introduction to Programming Language
Diving into the intricate realm of programming, one encounters the foundational concept of the for loop. In understanding the for loop, one must first grasp the core principles of programming languages. These languages serve as the vehicle through which instructions are communicated to computers. From the early days of assembly language to the modern high-level languages like Python and Java, the evolution of programming languages has shaped the digital landscape we navigate today.
From the rudimentary punch cards of the 1950s to the sophisticated compilers of the present day, the history and background of programming languages are a testament to human ingenuity and technological advancement. Features such as variables, arrays, and functions enable programmers to write efficient code, while the vast scope of applications ranging from web development to artificial intelligence underscores the omnipresence of programming languages in contemporary society.
Basic Syntax and Concepts
To comprehend the for loop, one must familiarize themselves with key concepts in programming such as variables, data types, operators, and expressions. Variables act as placeholders for storing data, while data types define the kind of values that variables can hold - be it integers, strings, or booleans. Operators, including arithmetic, logical, and relational, manipulate data within expressions, forming the building blocks of code execution.
Control structures like loops and conditional statements govern the flow of a program, determining the order in which instructions are executed. The for loop, a fundamental control structure, iterates over a specified range of values, executing a block of code multiple times. Understanding these foundational concepts is crucial in mastering the nuances of programming logic.
Advanced Topics
As one delves deeper into the realm of programming, advanced topics such as functions, object-oriented programming (OOP), and exception handling come into play. Functions encapsulate a set of instructions within a reusable block, promoting code modularity and reusability. OOP, a paradigm centered around objects and classes, enables programmers to model real-world entities in their code, fostering scalability and maintainability.
Exception handling facilitates graceful error management, allowing developers to anticipate and manage unexpected program behavior. The incorporation of these advanced topics enriches one's programming repertoire, enabling the creation of sophisticated and robust applications.
Hands-On Examples
To solidify understanding and proficiency in using the for loop, hands-on examples serve as invaluable learning tools. Simple programs demonstrating the iterative nature of the for loop, intermediate projects showcasing its versatility in real-world applications, and code snippets offering succinct implementations present learners with a spectrum of practical insights.
Crafting a for loop to iterate through a list of numbers or manipulating strings in various ways exemplifies the utility and power of this control structure. Building upon these examples, learners can enhance their problem-solving skills and logical thinking, honing their capabilities as adept programmers.
Resources and Further Learning
For individuals embarking on their programming journey, a plethora of resources and avenues for further learning await. Recommended books and tutorials provide in-depth explanations and exercises to consolidate one's understanding of the for loop and programming fundamentals. Online courses and platforms offer interactive learning experiences, allowing individuals to engage with coding challenges and projects in a supportive virtual environment.
Community forums and groups foster collaboration and knowledge sharing among peers, creating a vibrant space for individuals to seek guidance, exchange ideas, and cultivate a sense of camaraderie within the programming community. Leveraging these resources empowers learners to augment their skills, stay abreast of industry trends, and embark on a fulfilling programming odyssey.
Introduction to For Loop
The Introduction to For Loop serves as a cornerstone in understanding programming concepts. For beginners and intermediate learners, grasping the essence of looping mechanisms is crucial for building robust coding skills. This section sets the stage for a detailed exploration, shedding light on the fundamental role the for loop plays in iterative processes.
Definition of For Loop
Basic overview
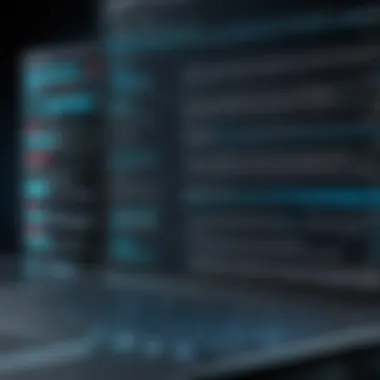
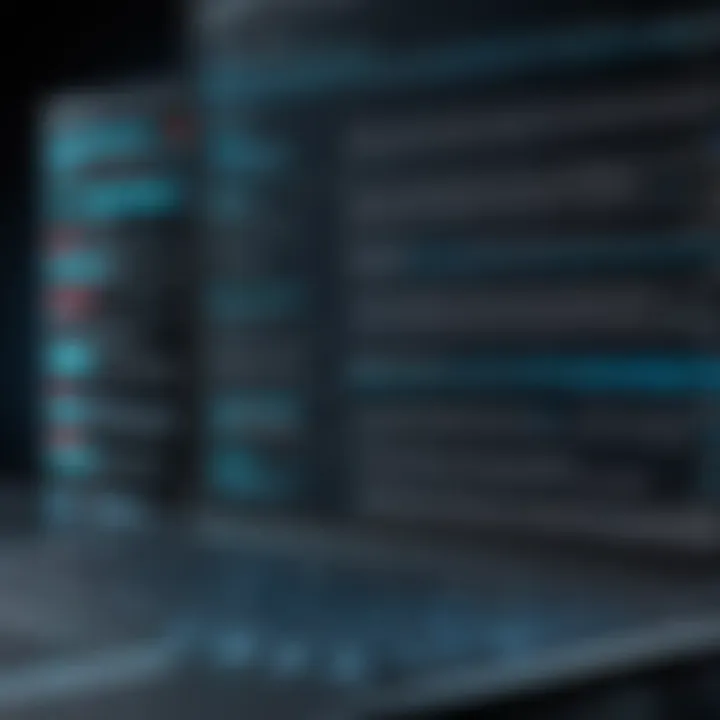
The Basic overview of the for loop unveils its foundational structure within programming paradigms. It acts as a pivotal command that directs the repetition of specific code blocks. Its simplicity in syntax and powerful functionality make it a go-to choice for developers looking to streamline repetitive tasks efficiently. The elegance of the for loop lies in its concise nature, allowing iterative operations to be executed with precision.
Purpose in programming
The Purpose in programming section elucidates the overarching goal of incorporating for loops in coding endeavors. By facilitating controlled repetition, for loops enhance code readability and maintainability. Their ability to iterate over a predefined range of values optimizes the execution of looping constructs, marking them as indispensable tools in the programming arsenal. Despite potential complexities in nested scenarios, the for loop's clarity and structured approach make it a favored method among coders.
Comparison with Other Loop Types
For loop vs. while loop
The comparison between for loops and while loops delves into contrasting mechanisms of iteration. Unlike while loops that predicate operation continuation on a specific condition, for loops offer a more compact and defined approach to iteration control. Their explicit initialization, condition, and iteration components provide a structured framework that can sometimes outperform while loops in clarity and efficiency. Understanding the nuanced distinctions between these loop types is vital for effective coding strategies.
For loop vs. do-while loop
When juxtaposing for loops with do-while loops, the iterative nuances become even more pronounced. While both constructs support repetitive execution, the do-while loop guarantees at least one iteration before evaluating the termination condition. This inherent difference impacts the flow and logic of code execution, influencing choices between the two loop variants based on specific programming requirements.
Importance of For Loop
Efficient iteration
Efficient iteration embodies the core strength of for loops, characterized by their precise control over looping sequences. By iteratively adjusting loop parameters, developers can finely tune execution paths and optimize performance. The for loop's ability to traverse data structures and perform repetitive tasks with minimal overhead underscores its value in accelerating program execution.
Simplified code structure
Simplified code structure represents a key benefit offered by for loops, promoting code elegance and coherence. Through encapsulating iterative logic within a concise block, for loops enhance code clarity and maintainability. This streamlined approach not only aids in debugging and troubleshooting but also cultivates a programming style that is both efficient and readable, aligning with best practices in software development.
Syntax and Structure
When delving into the intricate world of programming, the syntax and structure of a for loop stand as the backbone of repetitive code execution. Understanding the syntax and structure not only provides a foundational understanding but also lays the groundwork for efficient coding practices. Paying attention to the initialization, condition, and incrementdecrement aspects is crucial for creating robust for loops that perform seamlessly across various programming languages. For novice and intermediate programmers, grasping the nuances of syntax and structure can be a turning point in their coding journey, enhancing their ability to write intricate programs with ease.
Initialization
Initial Value Assignment
The process of initializing variables in the for loop carries significant weight in the realm of programming. Specifying the initial value sets the starting point for iterative processes, dictating how the loop will function. This assignment plays a pivotal role in determining the loop's behavior and its output. Clear and precise initializations are essential to ensure the correct flow of the loop and prevent potential errors. Understanding the nuances of assigning initial values empowers programmers to build efficient and error-free loops, thereby enhancing the reliability of their code.
Condition
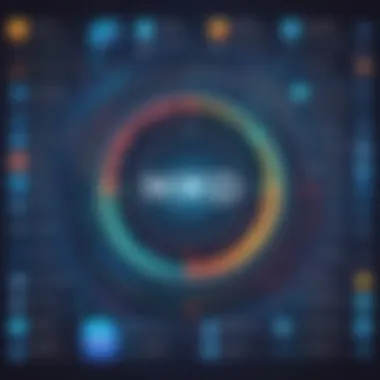
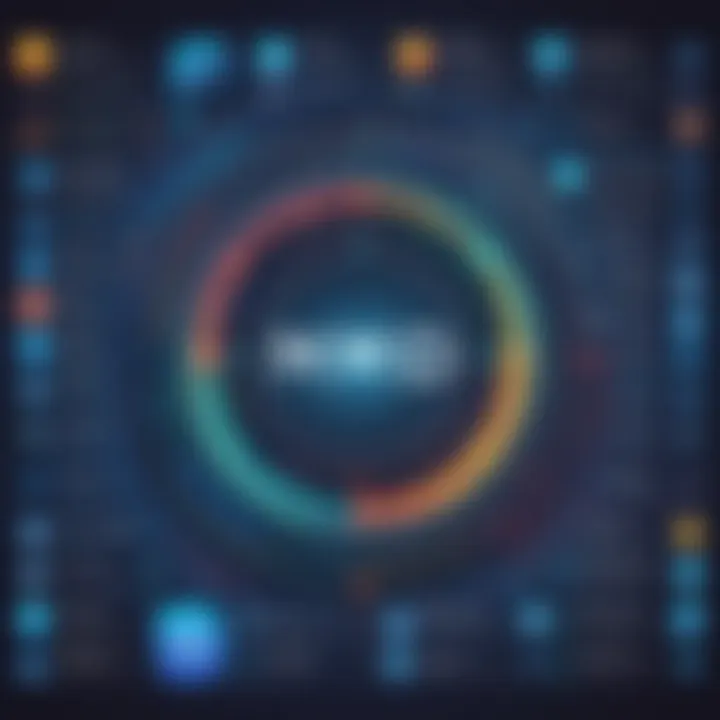
Loop Continuation Criteria
Among the crucial elements of a for loop is the condition that dictates the continuation of the loop. This criteria acts as a gatekeeper, regulating the loop's execution and determining when the loop should terminate. Crafting a robust condition is vital for ensuring that the loop iterates through the desired range without any hiccups. By defining clear and concise continuation criteria, programmers can control the flow of their loops effectively, leading to streamlined code execution and optimal performance. However, overlooking the importance of this aspect can result in infinite loops or premature loop exits, hindering the intended functionality of the program.
IncrementDecrement
Controlled Variable Modification
The concept of incrementing or decrementing variables within a for loop is a fundamental aspect that influences the loop's behavior. This modification lies at the heart of iterative processes, allowing for dynamic changes to the loop's controlled variable. By carefully managing variable increments or decrements, programmers can fine-tune the loop's operation, ensuring precise iteration over specified data sets. Leveraging controlled variable modification efficiently enhances the flexibility and scalability of for loops, granting programmers the ability to tailor their code to meet diverse requirements. Nevertheless, improper handling of variable modifications can lead to unexpected results or loop malfunctions, underscoring the importance of meticulous variable management in programming.
Working Mechanism
In elucidating the expansive realm of the for loop within the programming domain, it becomes imperative to delve into its working mechanism, a cornerstone of its functionality. Understanding the intricacies of the working mechanism provides a profound insight into how this fundamental construct enables the repetition of code blocks efficiently. At its crux, the working mechanism of the for loop revolves around orchestrating a seamless flow of iterations, each iteration progressing through predetermined steps with precision and control. By comprehending the working mechanism, programmers can harness its power to streamline code execution and enhance productivity in diverse programming endeavors.
Execution Flow
Step-by-step process
Unraveling the nuanced layers of the step-by-step process inherent in the for loop's execution flow unveils a meticulous sequence of actions guiding code iteration. This iterative process entails initializing the loop, validating the defined condition, executing the code block, and incrementing or decrementing the loop control variable. Each step in this process holds significance, contributing to the cohesive execution of the for loop and ensuring the logical progression of the code segments. The step-by-step process encapsulates a systematic approach to code repetition, fostering organized and structured programming practices for optimal efficiency in handling repetitive tasks. Embracing this detailed process empowers programmers to infuse precision and orderliness into their code, thereby fortifying the foundation of sound programming practices within the realm of the for loop.
Iteration Control
Loop termination conditions
Diving into the realm of iteration control, the spotlight falls on loop termination conditions as the linchpin of regulating the iterative cycle within the for loop paradigm. These conditions dictate when the loop ceases its execution, based on predefined criteria established within the code structure. Understanding the significance of loop termination conditions is vital for ensuring the controlled and purposeful execution of code blocks within programming loops. By defining clear and concise termination conditions, programmers can avert infinite loops and precisely control the number of iterations executed, preventing runtime errors and optimizing code performance. Grasping the nuances of loop termination conditions equips programmers with the tools to govern the termination of loops judiciously, fostering robust and efficient code execution practices within the programming landscape.
Common Applications
The Common Applications of the for loop in programming play a pivotal role in enhancing code efficiency and structure. By allowing developers to iterate over arrays, generate sequential output, and perform data processing operations, the for loop proves to be a versatile tool in various programming tasks. Understanding these applications is essential for harnessing the full potential of the for loop in programming languages. With its emphasis on efficient iteration and simplified code structure, the for loop stands out as a fundamental construct that significantly impacts development processes.
Iterating Over Arrays
Array Traversal Techniques
In the realm of programming, Array Traversal Techniques within the context of the for loop hold paramount importance. These techniques enable systematic traversal of array elements, facilitating streamlined data processing and manipulation. The key characteristic of Array Traversal Techniques lies in their ability to methodically cycle through array elements, ensuring comprehensive coverage and handling of data. This approach proves to be exceptionally beneficial in scenarios requiring sequential data access or modification, making it a popular choice for optimizing array handling routines within programming. The unique feature of Array Traversal Techniques is their inherent efficiency in processing large datasets, enabling swift and accurate operations. While advantageous in many aspects, these techniques may have limitations in highly complex array structures that demand specialized traversal methods.
Generating Sequential Output
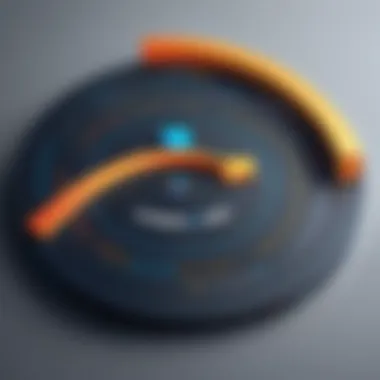
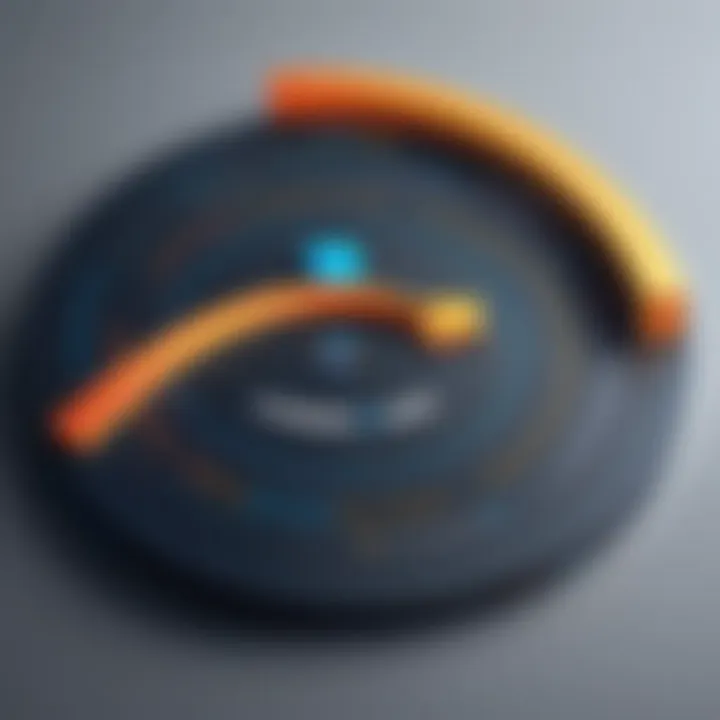
Number Series Creation
A crucial aspect of the for loop's Common Applications is Number Series Creation. This functionality allows for the systematic generation of numerical sequences, which is particularly useful in algorithmic development and mathematical computations. The hallmark characteristic of Number Series Creation is its ability to produce structured and ordered output, making it an essential tool in generating patterned data sets or iterating over numerical ranges. This feature's popularity stems from its versatility in applications that necessitate iterative numerical progression, contributing significantly to code logic and procedural design within programming contexts. Despite its advantages in controlled data generation, Number Series Creation may exhibit limitations in scenarios requiring non-linear or irregular data sequences.
Data Processing Operations
Database Queries
The integration of Database Queries with the for loop in programming underscores its relevance in data-centric applications. Database Queries facilitate seamless interaction with databases, enabling efficient retrieval and manipulation of information within programming environments. A defining characteristic of Database Queries is their ability to execute structured database operations iteratively, ensuring precise data handling and management. This attribute positions Database Queries as a valuable choice for tasks requiring synchronized data processing and database management, aligning seamlessly with the for loop's iteration control capabilities. The unique feature of Database Queries lies in their capacity to streamline data access and modification processes, enhancing the overall efficiency of data-driven applications. While advantageous in optimizing database interactions, Database Queries may present challenges in complex query formulations or resource-intensive database operations.
File Handling
File Handling operations within the for loop paradigm represent a crucial component of data management and processing workflows. By facilitating file access, manipulation, and storage operations, File Handling functionalities contribute significantly to data handling tasks within programming scenarios. The key characteristic of File Handling operations is their role in managing file resources systematically, ensuring secure and efficient file interactions during program execution. This attribute makes File Handling an invaluable choice for applications involving file readwrite operations, data backup procedures, and external resource management. The unique feature of File Handling lies in its versatility across different file formats, supporting various file types and structures for seamless data processing. While advantageous in maintaining file system integrity, File Handling may pose challenges related to file security concerns or complex file manipulation requirements.
Best Practices and Tips
In the realm of programming, adhering to best practices and tips is paramount to efficient and effective coding. This section aims to delve deep into the significance of incorporating best practices and tips into the utilization of for loops. By abiding by these principles, programmers can streamline their code, enhance performance, and foster maintainability. One key aspect to consider is the optimization of loop performance, which plays a pivotal role in the overall success of a program. Equally important is maintaining code readability, achieved through clear variable naming and consistent indentation. These practices not only improve the legibility of code but also contribute to the scalability and longevity of software projects.
Optimizing Loop Performance
When it comes to optimizing loop performance, a critical focus lies on reducing unnecessary iterations, a practice that can significantly impact the efficiency of a program. By minimizing redundant executions, developers can enhance the speed and resource utilization of their code. This strategy emphasizes the importance of writing concise and purpose-driven loops, thereby avoiding excessive computation and latency. By implementing this practice, programmers can achieve streamlined and high-performance code without compromising on functionality or accuracy.
Maintaining Code Readability
Within the domain of programming, maintaining code readability is a fundamental principle that greatly influences the comprehensibility and maintainability of software. Clear variable naming is one such practice that fosters understanding and clarity within a codebase. By using descriptive and meaningful identifiers, developers can easily grasp the purpose and function of variables, enhancing the overall cognitive load of the code. Another vital aspect is consistent indentation, which promotes visual organization and structure in code. This practice ensures that code blocks are visually distinguished, aiding in the identification of logical structures and facilitating code navigation. By upholding these practices, programmers can cultivate codebases that are not only functional but also user-friendly and conducive to collaborative development.
Conclusion
The conclusion section of this article serves as a pivotal component in wrapping up the exhaustive exploration of the for loop in programming. Understanding the depth and significance of the for loop empowers programmers with a fundamental tool for repetitive code execution. By meticulously dissecting the nuances of the for loop, individuals gain insights into how this construct streamlines code functionality and enhances programming efficiency. This section crystallizes the essence of the for loop's role in programming, underlining its indispensable nature in tackling iterative tasks with precision and clarity.
Summarizing Key Points
Versatility of for loop
Delving further into the versatility of the for loop reveals its robust nature in handling repetitive tasks across diverse programming paradigms. The key characteristic lies in the for loop's precise control over iteration, ensuring efficient data processing and manipulation. This feature underscores why the for loop stands out as a favored choice among programmers, offering a structured approach to repetitive code execution. The inherent flexibility of the for loop allows for tailored iteration control, enabling seamless adaptation to various programming challenges and scenarios. While the advantages of the for loop are abundant, there may be limitations in more complex algorithmic implementations where intricate iteration logic is required.
Essential role in programming
Unveiling the essential role of the for loop in programming showcases its pivotal contribution to code functionality and logic flow. The defining characteristic of the for loop lies in its ability to streamline iterative processes, promoting code efficiency and maintainability. This feature positions the for loop as an indispensable element in programming, driving the seamless execution of repetitive tasks with precision and finesse. The unique feature of the for loop lies in its structured approach to iteration control, ensuring code readability and logical sequence maintenance. While the advantages of the for loop are significant, complex computational tasks may necessitate alternative loop structures to accommodate specific algorithmic requirements.
Encouragement for Practice
Hands-on coding exercises
Embarking on hands-on coding exercises immerses learners in practical application, reinforcing comprehension of the for loop's functionality and versatility. The key characteristic of hands-on exercises is their efficacy in consolidating theoretical knowledge into practical skills, fostering a deeper understanding of programming concepts and logic. This approach serves as a beneficial choice for readers engaging with this article, as it cultivates a proactive learning environment that nurtures code proficiency. The unique feature of hands-on coding exercises lies in their interactive nature, enabling learners to experiment with code implementation and refine their problem-solving capabilities. While the advantages of hands-on exercises are immense, sustained practice and exploration are essential to master the complexities of the for loop and enhance overall programming proficiency.