Mastering the Enumerate Function: A Deep Dive into Python's Counter Utility
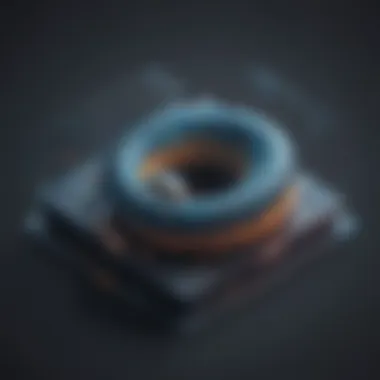
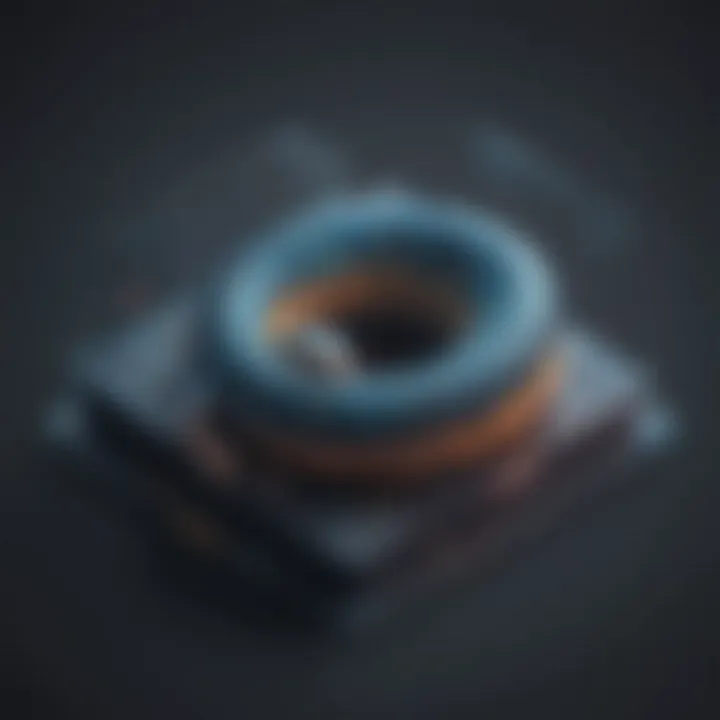
Introduction to Programming Language
Python is a versatile and widely-used programming language known for its readability and simplicity. Originally developed in the late 1980s by Guido van Rossum, Python has since gained immense popularity across various industries due to its extensive standard libraries and compatibility with different platforms. The language's clean syntax and support for multiple programming paradigms make it a go-to choice for beginners and seasoned developers alike.
Basic Syntax and Concepts
When diving into Python programming, one encounters fundamental concepts such as variables, data types, operators, and control structures. Variables are used to store data values, including integers, strings, and Boolean values. Data types specify the type of data that a variable can hold, such as integers for whole numbers, floats for decimal numbers, and strings for text. Operators are symbols used to perform operations on variables and values, while control structures like loops and conditional statements govern the flow of a program.
Advanced Topics
Beyond the basics lie advanced topics that elevate one's Python skills. Functions and methods allow developers to encapsulate and reuse code, promoting code modularity and organization. Object-oriented programming (OOP) enables the creation of classes and objects, fostering code reusability and scalability. Exception handling helps in managing and responding to errors gracefully, enhancing the robustness of Python programs.
Hands-On Examples
Practical application enhances understanding, and Python offers a myriad of hands-on examples to reinforce learning. From simple programs like calculating factorial numbers to intermediate projects like building a basic web scraper, coding snippets provide real-world context to theoretical concepts. By experimenting with code and participating in coding challenges, individuals can solidify their grasp of Python programming.
Resources and Further Learning
To embark on a comprehensive Python learning journey, aspiring programmers can leverage various resources. Recommended books such as 'Automate the Boring Stuff with Python' by Al Sweigart and 'Python Crash Course' by Eric Matthes offer in-depth insights and practical exercises. Online courses on platforms like Coursera and Udemy provide structured learning paths, covering Python from beginner to advanced levels. Engaging with community forums like Stack Overflow and joining Python user groups enable networking, knowledge sharing, and access to valuable insights from experienced Python developers.
Introduction to Enumerate Function
In the realm of Python programming, the Introduction to Enumerate Function serves as a foundational element that empowers developers to enhance their code efficiency and readability. Understanding how to utilize the enumerate function is crucial for iterating through data structures while keeping track of element indices. By grasping the nuances of this function, programmers can streamline their coding processes and optimize algorithmic logic. The Introduction to Enumerate Function sets the stage for exploring practical applications and advanced techniques within the Python environment.
Definition of Enumerate
Enumeration Purpose
The Enumeration Purpose within the context of Python's enumerate function is paramount for providing iterable objects with a numerical index. This feature significantly simplifies the process of iterating through collections by attaching a counter to each element. The primary benefit of Enumeration Purpose lies in its ability to offer a straightforward mechanism for accessing both the index and the value of items in a sequence simultaneously. This concise functionality enhances code clarity and simplifies complex iteration tasks.
Syntax Explanation
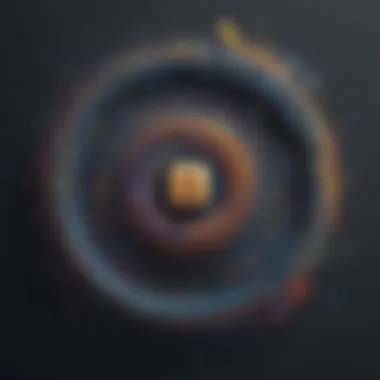
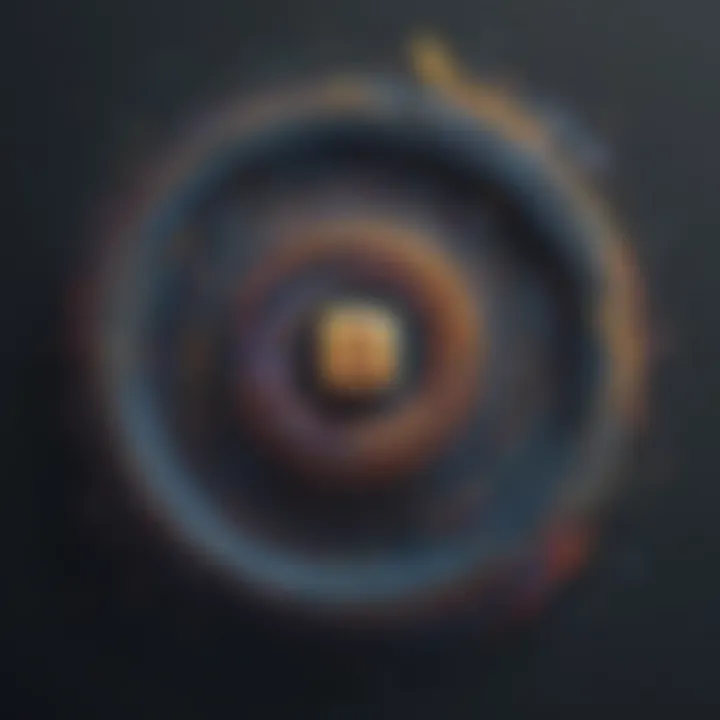
The Syntax Explanation aspect of the enumerate function details the precise structure required to implement this feature effectively. The syntax encapsulates the enumerate() method followed by the target iterable object, ensuring a seamless integration of the enumeration functionality into the codebase. By delineating the specific syntax involved, developers can effortlessly incorporate the enumerate function into their programming tasks, facilitating efficient data traversal and manipulation.
Working Principle
Upon delving into the Working Principle of the enumerate function, two fundamental aspects come to light: Iterating with Index and Index and Value Pairing. These components elucidate how the enumerate function operates under the hood, providing valuable insights into its functionality.
Iterating with Index
Iterating with Index underscores the core mechanism of the enumerate function, allowing programmers to traverse through a sequence while retaining access to the current index of each element. This feature streamlines the process of referencing element positions, enabling developers to execute tasks that necessitate index-based operations with ease. By leveraging Iterating with Index, programmers can optimize their code structure and enhance the efficiency of their algorithms.
Index and Value Pairing
Index and Value Pairing within the enumerate function emphasizes the seamless combination of index information with corresponding element values during iteration. This pairing facilitates a cohesive approach to working with data, ensuring that each element's index is directly associated with its respective value. Such synchronization simplifies data processing and empowers developers to manipulate elements systematically within a sequence.
Advantages of Enumerate
In unpacking the Advantages of Enumerate, two key factors come into focus: Simplifying Iteration and Enhancing Readability. These advantages underscore the inherent benefits of incorporating the enumerate function into Python programming endeavors.
Simplifying Iteration
The Simplifying Iteration aspect of the enumerate function drastically reduces the complexities associated with traversing through data structures. By providing a structured approach to accessing elements alongside their indices, Simplifying Iteration fosters a more intuitive coding experience. This streamlined process not only enhances code comprehension but also accelerates development cycles by minimizing the effort required for iterating through sequences.
Enhancing Readability
Enhancing Readability stands out as a hallmark advantage of using the enumerate function, as it elevates the clarity of code implementation. By capturing both the index and value of elements within a sequence, Enhancing Readability enables developers to craft more transparent and self-explanatory code. This enhancement promotes code maintainability and fosters collaborative development environments where code logic is easily decipherable.
Implementation of Enumerate Function
In this segment, we delve into the practical application of the Enumerate function in Python. Understanding the implementation of Enumerate is crucial as it equips programmers with the ability to add a counter to iterable objects, streamlining the process of iterating and accessing elements within data structures. By exploring the nuances of Enumerate's functionality, individuals can enhance their code readability and efficiency. This section will elaborate on specific elements such as its benefits and considerations, offering a comprehensive understanding of how Enumerate can elevate Python programming.
Basic Usage
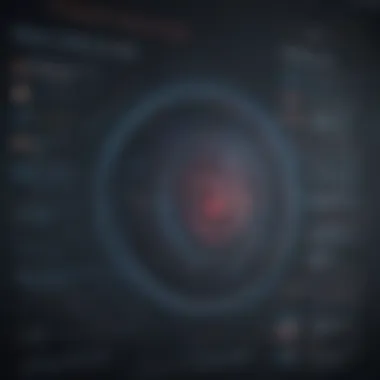
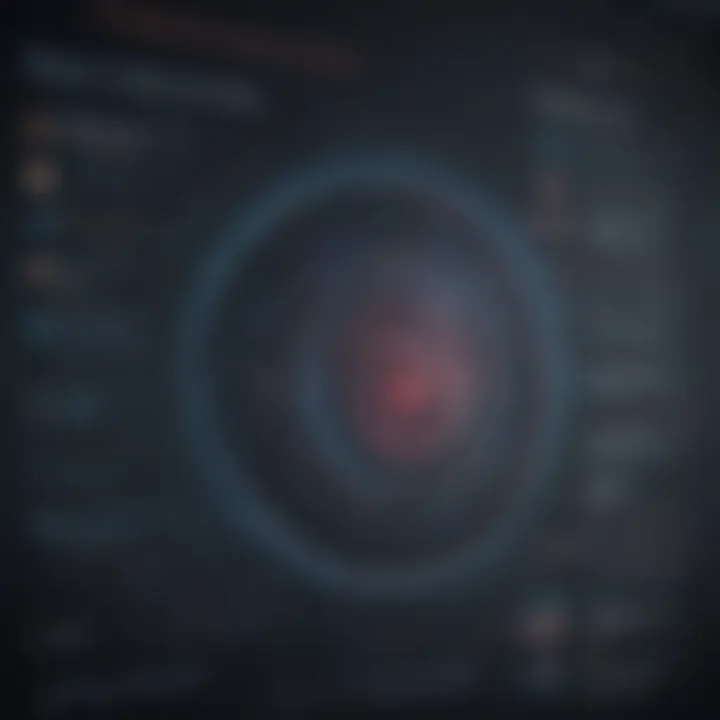
Using Enumerate with Lists
The utilization of Enumerate with lists is a fundamental aspect that simplifies the process of iterating through elements while simultaneously keeping track of their indices. This feature proves to be highly advantageous in scenarios where developers need to manipulate both the index and value of items within a list. By incorporating Enumerate with lists, programmers can enhance the overall functionality of their code, making it easier to implement logic that requires sequential data processing.
Applying Enumerate to Strings
Applying Enumerate to strings introduces a unique perspective to the Enumerate function, allowing programmers to traverse through strings character by character while enumerating them. This method provides a structured approach to analyze textual data efficiently. The ability to enumerate strings enables developers to perform operations based on individual characters, offering a granular level of control over string manipulation. Despite its versatility, it is essential to consider the performance implications of applying Enumerate to strings in certain scenarios.
Customizing Output
Specifying Start Value
The specification of a start value in Enumerate allows developers to define a custom initial index for enumeration, thereby introducing flexibility in data processing. By setting a specific starting point, programmers can tailor the enumeration process to align with specific requirements or constraints within their code. This capability facilitates a more personalized approach to data iteration, ensuring that the enumeration begins at a designated position, optimizing the handling of iterable objects.
Utilizing Enumerate in Loops
The integration of Enumerate in loops provides a practical way to leverage enumeration functionalities within iterative structures. This approach streamlines the process of iterating through data collections, offering a concise method to access both the index and value of elements simultaneously. By utilizing Enumerate in loops, programmers can enhance the efficiency of their code, enabling a seamless transition between enumeration and loop iterations. However, it is essential to evaluate the trade-offs between performance and usability when incorporating Enumerate in loop constructs.
Handling Enumerate Output
Accessing Index and Value
Accessing the index and value produced by Enumerate output empowers developers to extract pertinent information from the enumeration process efficiently. This feature allows for precise data manipulation based on the indexed position and corresponding values of elements. By understanding how to access and leverage Enumerate output effectively, programmers can implement targeted operations on enumerated data, enhancing the functionality and logic of their Python programs.
Converting Enumerate to List
Converting Enumerate output to a list format facilitates the transformation of enumerated data into a structured and accessible container. This conversion enables developers to manipulate Enumerate output as a list object, opening up a plethora of possibilities for data organization and processing. By converting Enumerate to a list, programmers can easily apply list-specific operations and functionalities to the enumerated data, further refining the data handling process and optimizing program performance.
Enhancing Code Clarity
Improving Code Understandability
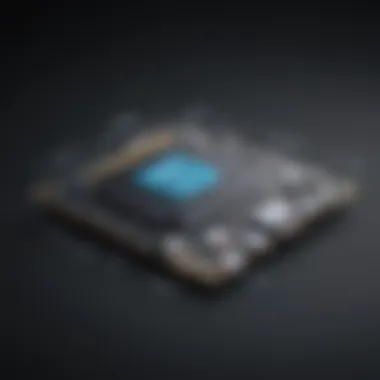
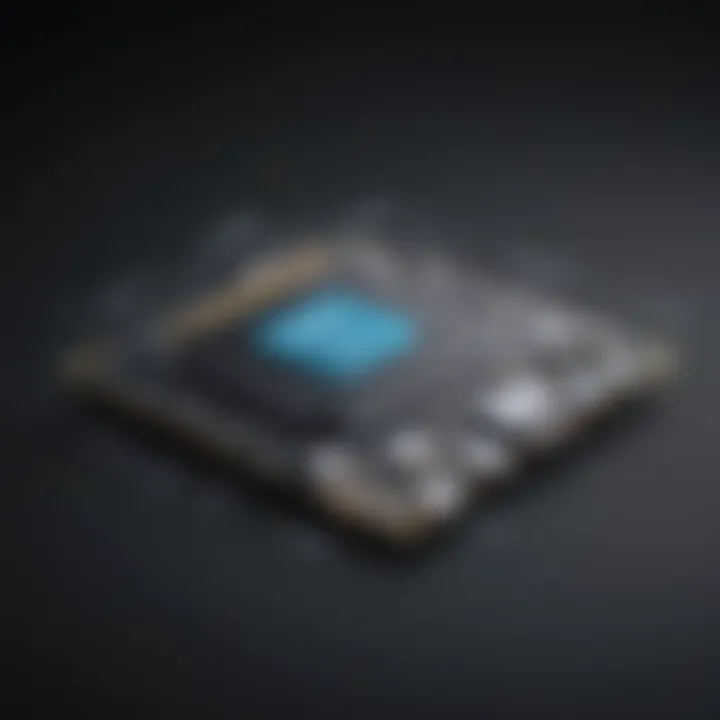
Improving Code Understandability is a paramount objective when integrating the enumerate function into Python code. By utilizing enumerate, programmers can improve the readability and comprehensibility of their codebase by providing explicit index-value associations within loops. This practice enhances code transparency and simplifies the process of understanding the logic behind data manipulations. Enhanced code understandability through the enumerate function promotes code maintainability and collaboration, fostering a conducive programming environment for individuals and teams.
Streamlining Algorithm Logic
Streamlining Algorithm Logic is a critical aspect of optimizing code efficiency using the enumerate function. By incorporating enumerate into algorithmic structures, programmers can streamline the logical flow of operations and enhance the overall performance of their code. This methodology enables algorithmic processes to be executed more efficiently, reducing redundant operations and improving the computational speed of Python programs. Streamlining algorithm logic through the enumerate function facilitates the development of efficient and scalable code solutions in Python programming.
Advanced Techniques and Best Practices
Advanced Techniques and Best Practices are crucial aspects covered in this article. Understanding advanced techniques can significantly enhance one's skills in utilizing the enumerate function in Python. By delving into nested enumerate functionality, programmers can gain insights into handling more complex data structures and iterations. This section emphasizes the importance of optimizing performance through efficient coding practices. Exploring alternative iteration methods such as itertools alongside enumerate allows for a comprehensive understanding of diverse programming approaches.
Nested Enumerate Functionality
Nested Iterations
Nested Iterations play a key role in the overall functionality of utilizing enumerate in Python. By allowing for iterations within iterations, nested iterations facilitate the processing of intricate data structures. The inherent characteristic of Nested Iterations lies in their capability to navigate through multi-layered data sets seamlessly. This feature proves beneficial in scenarios where deeply nested data requires systematic traversal, offering programmers a structured approach for data manipulation within this article.
Multi-dimensional Data Handling
Multi-dimensional Data Handling forms a vital component in leveraging enumerate effectively. This aspect focuses on managing complex data sets with multiple dimensions, providing programmers with the tools to navigate through arrays, matrices, or other multidimensional data structures. The key characteristic of Multi-dimensional Data Handling lies in its ability to organize and access data with enhanced clarity and efficiency within this article. Despite its advantages, dealing with multi-dimensional data can sometimes pose challenges, requiring careful consideration of memory usage and performance implications in this context.
Performance Considerations
Efficiency Tips
Efficiency Tips are essential considerations when working with the enumerate function. By implementing efficient coding practices, programmers can optimize the performance of their code, leading to faster execution times and reduced resource consumption. The key characteristic of Efficiency Tips is their ability to streamline processes and enhance the overall efficiency of code execution within this article.
Impact on Program Speed
Understanding the Impact on Program Speed is crucial for achieving optimal performance when utilizing enumerate in Python. By analyzing how different coding techniques and structures impact program speed, programmers can make informed decisions to enhance the responsiveness of their applications. The unique feature of Impact on Program Speed lies in its direct correlation to code optimization strategies, highlighting the significance of efficient coding practices in minimizing execution times within this article.
Alternative Iteration Methods
Comparison with Zip Function
Comparing Enumerate with the Zip Function provides programmers with insights into selecting the most suitable iteration method for a given scenario. By contrasting the functionalities and applications of Enumerate and Zip, programmers can determine the ideal approach for specific programming tasks. The unique feature of Comparison with Zip Function is its versatility in handling iterable objects and simplifying the process of merging data structures or creating tuples while iterating within this article.
Itertools and Enumerate
Exploring Itertools in conjunction with Enumerate presents programmers with advanced tools for handling iterations in Python. By utilizing the functionalities offered by the Itertools module alongside Enumerate, programmers can enhance the flexibility and efficiency of their code. The key characteristic of Itertools and Enumerate lies in their ability to extend the capabilities of traditional iteration methods, offering diverse functionalities for manipulating data structures effectively within this article.
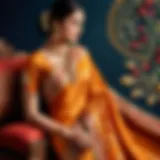
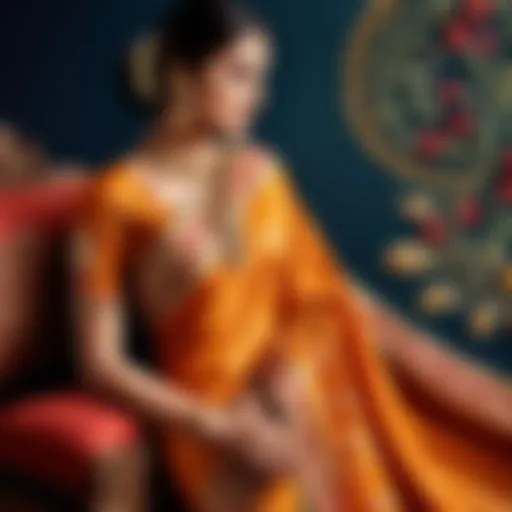