Understanding Call Back Functions: A Comprehensive Guide
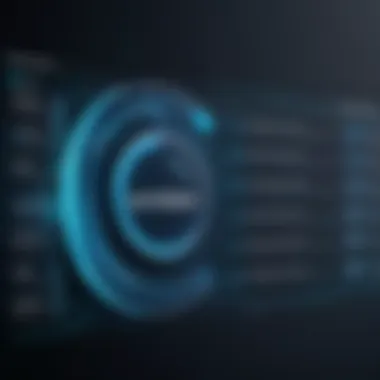
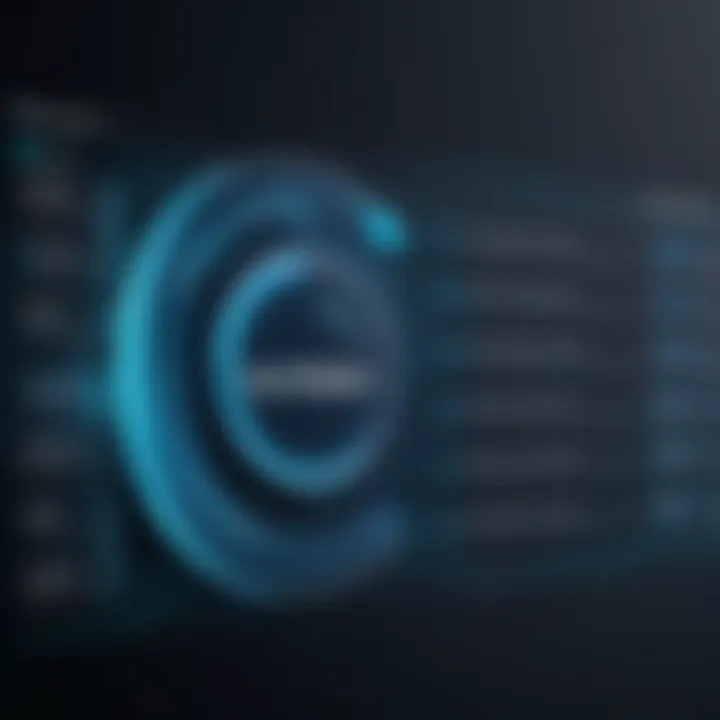
Intro
In programming, the concept of callback functions has become vital for creating responsive and functional code. This section aims to provide a foundational understanding of callback functions, their definitions, and their significance in various programming languages. The relevance of callback functions is evident in both JavaScript, known for its asynchronous operations, and C/C++, where they streamline control flow.
By exploring this topic, we can reveal how closely callback functions relate to event-driven programming and how they contribute to cleaner, more modular code. In this guide, the objective is to delve deep into the nuances of callback functions, unpacking their structure and utility.
"Understanding callback functions is crucial for navigating modern programming challenges."
The exploration begins with the basics, leading to advanced concepts, hands-on examples, and resources for further learning. Following this structured approach enhances the understanding of how callbacks operate and their applications in real-world scenarios.
Preface to Call Back Functions
Call back functions constitute a fundamental concept in programming that enables developers to manage and control code execution flow effectively. Their importance lies in their ability to allow functions to be passed as arguments, fostering greater flexibility and asynchronous behavior in applications. This flexibility is particularly vital in environments like JavaScript, where non-blocking operations are increasingly prevalent. Mastery of call back functions enhances a programmer's toolkit, empowering them to create more responsive and efficient applications.
Definition of Call Back Functions
Call back functions, in essence, are functions designed to be executed at a later point in time, often as a direct response to a specific event or condition. The name "call back" is derived from their ability to be "called back" by the program after the completion of an asynchronous operation or when a certain task concludes. In programming, they serve to react to changes or events, allowing the flow of execution to continue while waiting for some other task to finish. For instance, in web development, a call back might be triggered by a user action, such as clicking a button or receiving data from a web server. This allows developers to maintain responsiveness and keeps the user experience smooth.
Historical Context and Evolution
The concept of call back functions can be traced back to the early days of software development when the need for asynchronous programming began to surface. Initially, programming languages primarily supported synchronous operations, which could lead to inefficient execution, especially in I/O operations. As systems grew in complexity, the need for more manageable execution sequences became apparent. Over time, languages evolved to incorporate call back mechanisms.
JavaScript, in particular, embraced this concept due to its event-driven nature. The emergence of call back functions in JavaScript coincided with the growth of web applications, where interactions often depend on the asynchronous processing of user inputs and external resources. Technologies like AJAX further proliferated the use of call backs, changing how developers approached client-server communication. The evolution of programming paradigms continues to shape the usage of call backs, making them relevant even as other structures like Promises and async/await emerge. Their significance in handling asynchronous operations has remained constant.
Types of Call Back Functions
The role of call back functions in programming is pivotal. Understanding their types enhances one’s ability to write effective code. The two primary categories, synchronous and asynchronous call back functions, each have unique characteristics and applications, making it essential for programmers to grasp their distinctions and use cases.
Synchronous Call Back Functions
Synchronous call back functions are executed at the point in the code where they are invoked. The main thread waits for the call back function to complete its execution before it continues with the next line of code. This can lead to a straightforward flow of operations, simplifying the control and structure of the program.
Characteristics of Synchronous Call Backs
- Blocking behavior: The main thread does not proceed until the call back is resolved.
- Immediate execution: There is no delay between the function call and the execution of the call back.
- Suitable for short tasks: Ideal for smaller, simpler operations that do not require extensive processing time.
Synchronous call backs can enhance readability. However, using them excessively, especially for time-consuming operations, can lead to performance issues. The code can become less responsive. Asynchronous tasks can benefit from their execution, ensuring functional integrity without freezing the main thread.
Asynchronous Call Back Functions
Asynchronous call back functions, on the other hand, offer a different paradigm. They allow a program to continue executing while waiting for a call back to resolve. This is critical in scenarios where tasks take longer to complete, such as network requests or file operations.
Characteristics of Asynchronous Call Backs
- Non-blocking behavior: The main thread continues executing other code without waiting for the call back to finish.
- Deferred execution: The call back may run after other operations, often triggered by events, timers, or completion of external processes.
- Best for longer tasks: Particularly useful when dealing with operations that can take an indeterminate amount of time to complete.
Using asynchronous call backs can significantly improve application performance and user experience. However, managing asynchronous code can be challenging. Care must be taken to avoid complications such as callback hell, where nested callbacks become difficult to maintain and understand.
In summary, understanding the types of call back functions is crucial for software development. Synchronous call backs simplify flow for quick tasks, while asynchronous call backs enhance performance for longer operations.
Functionality in JavaScript
In programming, JavaScript is a language known for its dynamic capabilities. Central to its design are call back functions. These functions allow for greater flexibility and control when dealing with asynchronous operations. Understanding how these call back functions operate within JavaScript is crucial for developers, especially as web applications become more complex.
Benefits of Call Back Functions in JavaScript:
- Asynchronous Operation: Call back functions enable JavaScript to perform non-blocking operations. This means tasks can run in the background while the main thread continues executing, improving the user experience.
- Event Management: They help manage events effectively. When a user interacts with a web page, call backs can be triggered to respond to user input, such as clicks or key presses.
- Simplified Code Structure: Using call back functions can lead to cleaner and more manageable code. They provide a structured way to execute functions in response to specific events or conditions.
- Error Handling: Call backs can also be designed to handle errors during asynchronous operations. By including error handling within call backs, developers can ensure that the application behaves reliably.
Considerations About Call Back Functions in JavaScript:
While call back functions are useful, they also come with challenges. Developers must consider their scope and context to avoid unintended consequences.
- Scope Issues: If a call back function is defined in the wrong scope, it may not have access to the necessary variables. This can lead to bugs that are difficult to track down.
- Performance Overhead: Excessive use of nested call backs can lead to reduced performance and readability, a phenomenon known as "callback hell."
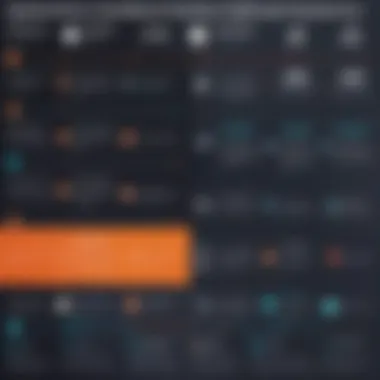
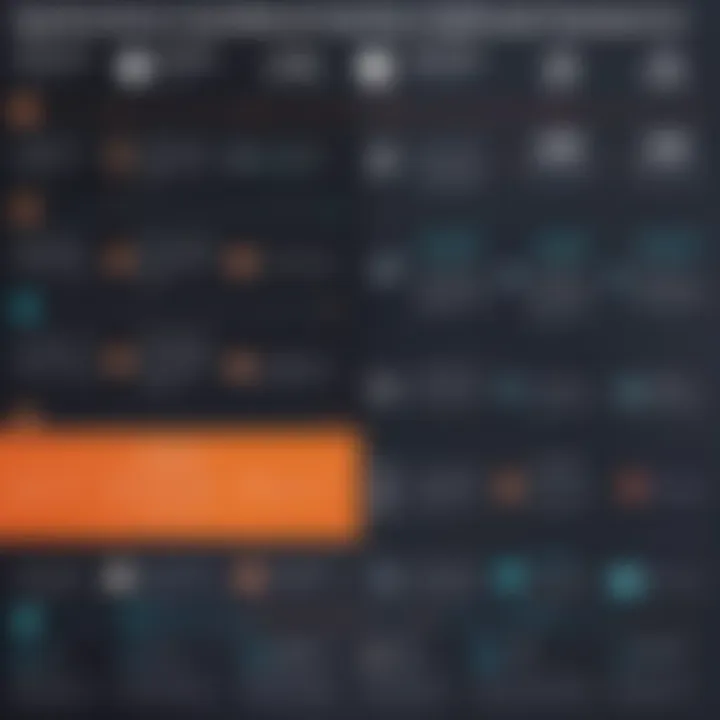
Overall, call back functions form an integral part of JavaScript. They facilitate a variety of functionalities that are essential for modern web development.
Using Call Back Functions in JavaScript
Using call back functions in JavaScript involves defining a function and passing it as an argument to another function. This allows the first function to execute once certain conditions are met or when an operation completes. A simple example can illustrate this:
In this code block, the function takes two parameters: a name and a call back function. After greeting the user, it calls the function. This demonstrates the simplicity of using call back functions for sequential operations.
Event Handling and Call Back Functions
In JavaScript, event handling is one of the key use cases for call back functions. When a user interacts with the web interface, such as clicking a button or submitting a form, event listeners are often employed to manage these interactions. Call back functions are essential for executing code in response to these events.
For instance, consider a button that a user can click. Here’s how you might attach an event listener to it:
In this example, the anonymous function acts as a call back. It gets executed when the button is clicked, demonstrating how smoothly JavaScript handles events. This capability is foundational for developing interactive web applications.
"Call back functions are the backbone of asynchronous event handling in JavaScript."
Utilizing call back functions for event handling not only enhances the interactivity of web pages but also leads to a more efficient, responsive user experience. As developers learn to harness these functions, they unlock the potential for sophisticated web applications.
Call Back Functions in and ++
Call back functions play a vital role in the C and C++ programming languages. They provide programmers with a way to create powerful and flexible code structures. A call back function is an integral part of many programming paradigms. Understanding how these functions work in C and C++ helps learners grasp the underlying principles of function behavior and control flow.
In C, call back functions are typically implemented through function pointers. This indicates a direct link between the function and its calling context. The unique aspect of C is its procedural nature, where the flow of execution can be altered by passing these function pointers. This functionality not only enhances the modularity of the code but also allows for a more dynamic execution of tasks.
In C++, call backs expand in utility with object-oriented features. Here, call back functions can be members of classes or stand-alone functions. Using templates and lambdas, C++ offers even more flexibility. Call backs are not just about defining functions; they also provide a framework for managing events and responses in a clean manner.
The following segments will provide an in-depth focusing on defining call back functions in C and using function pointers correctly in C++ to maximize the potential of your code.
Defining Call Back Functions in
Defining a call back function in C requires a clear understanding of function pointers. A call back function is simply a function that is passed as an argument to another function. This enables the execution of the passed function at a later point.
Here’s a simple illustration of defining a call back function in C:
In this code, is defined. It is passed as an argument to , demonstrating how call back functions allow for flexibility and code reuse.
Using Function Pointers for Call Backs in ++
Using function pointers in C++ extends the possibilities of call back functions beyond C's capabilities. In C++, functions can be methods of classes, and they can also utilize templates and lambdas. This brings both complexity and power to your program.
Here’s how you can utilize function pointers in a C++ context:
In this example, member functions are passed via function pointers. This highlights how C++ allows for more sophisticated call back structures. The instances where class methods serve as call backs illustrate a deeper engagement with object-oriented programming principles.
Practical Applications of Call Back Functions
Call back functions hold an essential role in modern software development. They enable developers to create flexible and responsive code. This section focuses on practical applications of call back functions, examining their significance in real-world scenarios and how they are integrated into various frameworks and libraries.
Real-World Scenarios
Call back functions are widespread in real-world applications. In web development, for example, they are utilized for event handling. When a user clicks a button, the associated call back function can execute specific logic. This makes the application responsive and interactive. Here are some common scenarios:
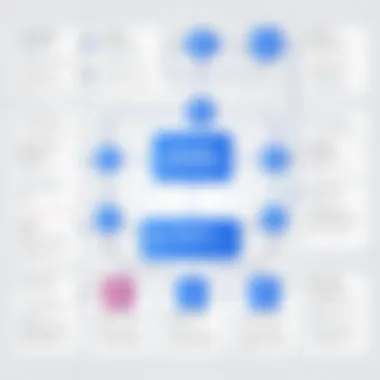
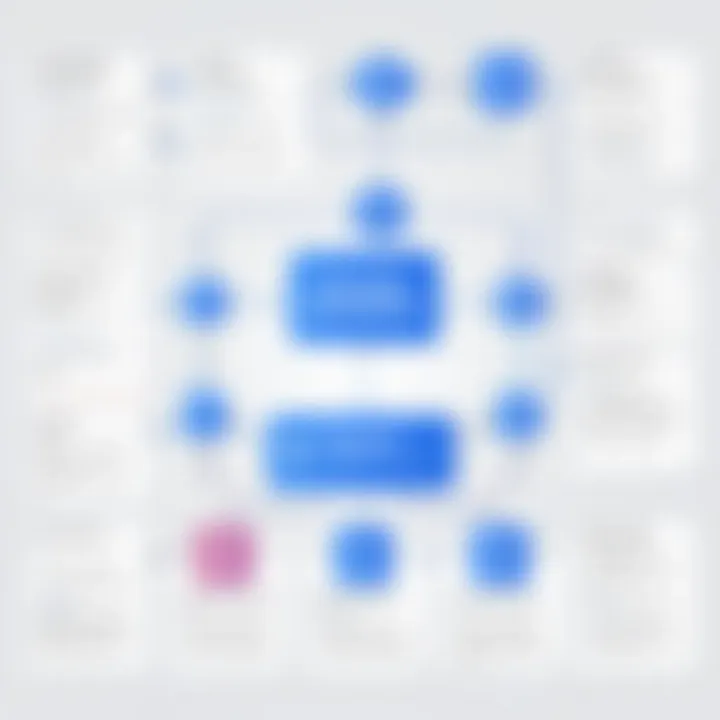
- User Interface (UI) Updates: Call backs are used to update UI components based on user interactions. For instance, when a user submits a form, a call back function can validate the inputs and provide immediate feedback.
- Network Requests: When requesting data from an API, asynchronous call backs manage the responses. This prevents the application from freezing while waiting for network responses. For example, libraries like Axios use call backs to dynamically update the UI after retrieving data.
- Timers and Animations: In JavaScript, functions like and accept call backs to execute code after a specific delay or at regular intervals. This application is crucial for animations and user experience enhancements.
Overall, call back functions help in managing asynchronous operations effectively, improving the responsiveness of applications.
Frameworks and Libraries Utilizing Call Backs
Many programming frameworks and libraries rely heavily on call back functions. This reliance underscores their importance in software development. Some notable instances include:
- jQuery: This popular JavaScript library uses call backs for handling events. Functions such as , , and take call backs to define the actions to take when events occur or when requests complete.
- React: In React, components often use call backs for state management. When a user interacts with a component, a call back can trigger state updates, leading to re-rendering of the component with updated data.
- Node.js: Call backs are foundational in Node.js for handling asynchronous operations. Functions such as reading files or handling HTTP requests frequently utilize call backs to manage completion states effectively.
"Call back functions are not just a technical construct; they embody the asynchronous nature of modern programming"
Embracing this concept is essential for any developer aiming to build robust applications.
Common Challenges and Issues
Understanding call back functions goes beyond their definitions and applications. It is essential to recognize the common challenges and issues that developers face when dealing with these functions. This topic is crucial because, despite the many advantages call backs provide, they can also lead to complex and hard-to-manage code. Developers often encounter specific pitfalls that can complicate both development and debugging processes. Focusing on common challenges ensures programmers can write more efficient and cleaner code, enhancing both performance and readability.
Callback Hell and Its Implications
Callback hell, sometimes described as the "Pyramid of Doom," refers to a situation where multiple nested call back functions are used. This nesting can make the code hard to read and maintain. When callbacks are chained together, especially in asynchronous operations, the indentation increases, resulting in additional complexity.
The implications of callback hell are significant. It can lead to:
- Reduced readability
- Increased difficulty in debugging
- Higher chances of introducing bugs
A typical scenario involves an asynchronous operation followed by another operation. Consider the following example:
In the above code, you see how quickly callbacks can stack up. Each new function adds another layer of indentation, complicating the structure. To address this issue, developers often utilize promises or async/await syntax, which provides a more manageable way to handle asynchronous operations.
Debugging Call Back Functions
Debugging call back functions can be particularly challenging. When errors occur inside a callback, pinpointing the source can take time, mainly due to the asynchrony in operations. If a callback fails or acts unpredictably, tracking down the error requires careful examination of both the primary function and the callback itself.
Some strategies to simplify debugging include:
- Utilizing console logs to trace data flow between callbacks.
- Implementing error handling inside callbacks to catch and respond to errors as they occur.
- Utilizing tools such as debuggers to step through code and observe the call stack.
Developers can effectively reduce difficulties by adopting best practices when implementing call back functions. Recognizing these challenges early can prepare programmers to write cleaner, more efficient code, thus improving their programming experience.
Best Practices for Implementing Call Backs
Implementing call back functions is essential for maintaining the efficiency and clarity of your code. Following best practices can enhance the maintainability, readability, and performance of your code, especially when dealing with asynchronous operations. It is crucial to understand how to effectively utilize call backs in various programming environments. Here, we discuss some guiding principles for implementing call backs effectively.
Ensuring Proper Context and Scope
Properly managing context is vital when implementing call back functions. Call backs often hold references to surrounding lexical scopes, which can lead to unexpected behavior if they are not handled properly.
- Use Arrow Functions: In JavaScript, arrow functions do not create their own context. They inherit it from the parent scope, which can prevent context-related issues. For instance:
- Bind Method: If you must use a regular function, consider using the method. This explicitly sets the context for the call back.
Managing context correctly reduces confusion and potential bugs in your applications.
Avoiding Common Pitfalls
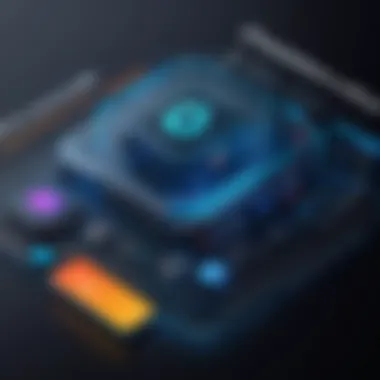
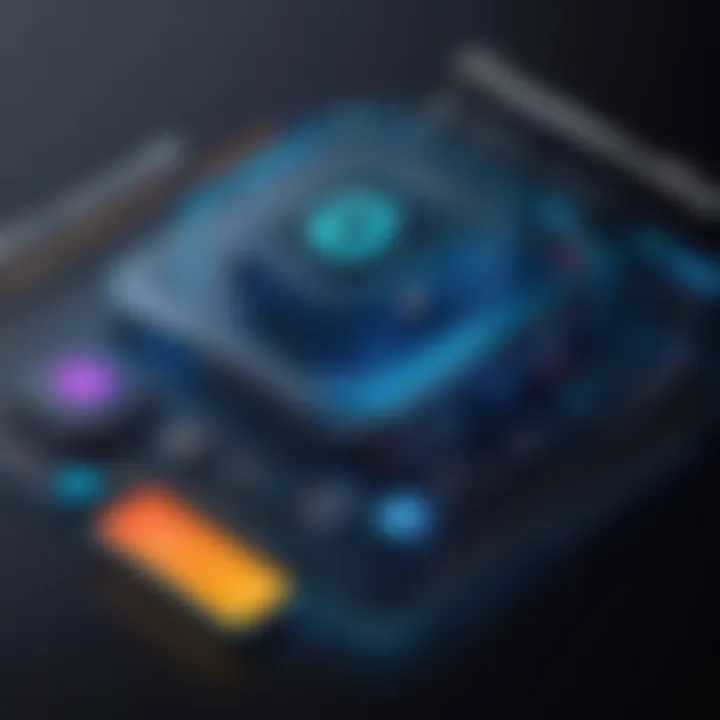
Even experienced developers can fall into traps when working with call back functions. Awareness of these pitfalls can prevent many common errors.
- Callback Hell: This term describes excessive nesting of call backs, leading to hard-to-read and difficult-to-maintain code. To combat this, you can use modular functions or consider Promises as an alternative.
- Not Handling Errors: Always include error handling in your call backs. Omitting error management can lead to unhandled exceptions that crash your application.
- Ignoring Performance: Call backs can introduce performance issues, especially when they lead to multiple subsequent calls. Always measure the performance impact of your call backs and consider optimizing with techniques like throttling and debouncing in UI applications.
In summary, implementing call back functions requires attentiveness to context and common pitfalls. By adhering to best practices, you can create efficient and maintainable code, fostering a smoother development process.
Comparing Call Back Mechanisms
When analyzing call back functions, it becomes crucial to compare different mechanisms. Understanding the distinctions between synchronous and asynchronous call backs can help clarify their usage and impact on programming efficiency. This section will uncover the fundamentals of these mechanisms and their significance in various programming contexts.
Synchronous vs Asynchronous Call Backs
Synchronous call backs execute in sequence within the same thread, leading to a straightforward flow of control. This means that the code execution must complete the current function before moving on to the next one. For instance, in the JavaScript example below:
Here, you see that the console logs "This runs first." immediately. Synchronous call backs can be more manageable in simple tasks, but they often cause delays in coding execution due to blocking behavior.
On the opposite side, asynchronous call backs allow the execution to continue while waiting for a task to complete. They do not block the thread, enabling programs to handle other tasks. In JavaScript, this is commonly used in API calls or event listeners. Consider this example:
In this case, "This runs after the API call." will log after a delay, while other code can continue running. This feature of asynchronous call backs is particularly valuable in web development, enhancing application responsiveness and user experience.
Performance Considerations
The choice between synchronous and asynchronous call backs has significant performance implications. Synchronous call backs may be easier to implement for small tasks. However, they can lead to performance bottlenecks in larger applications. For instance, during blocking tasks like file reading, the entire process halts, creating a less efficient user experience.
Conversely, asynchronous call backs improve overall performance, particularly in I/O-bound operations. They allow for better utilization of system resources, maintaining fluidity in applications. However, managing multiple asynchronous calls can introduce complexity, such as the aften-discussed callback hell scenario.
"Choosing the right call back mechanism is essential for application efficiency. While synchronous calls simplify control flow, asynchronous calls enhance performance in a multi-tasking environment."
Future Perspectives on Call Back Functions
In the evolving landscape of programming, understanding the future perspectives of call back functions is essential for both learners and professionals. Call backs are not just temporary solutions; they shape how we think about asynchronous actions within code. The significance of exploring future trends can lead to better programming practices, more efficient code structures, and greater adaptability to newer frameworks.
Important elements to consider include how call backs can influence the design of programming languages and the development of more efficient coding techniques. As the demand for responsive applications grows, ensuring that call backs remain relevant and effective is crucial.
Evolution of Programming Languages and Call Backs
The evolution of programming languages has brought significant changes to how call back functions are employed. In the early days, languages like C mostly relied on function pointers for creating call backs. The introduction of languages such as JavaScript and Python demonstrated a shift towards simplified call back handling, emphasizing ease of use without losing functionality.
The transition from synchronous to asynchronous call backs is another major development. With the rise of web applications and user interface demands, asynchronous programming is critical. Languages are adapting, integrating call back mechanisms directly into their syntax. This evolution allows developers to write cleaner code, reducing the likelihood of “callback hell,” a term describing the complexity arising from nested call backs. As programming languages continue to evolve, we can expect improved support for asynchronous patterns and perhaps even more intuitive ways to handle call backs.
Call Back Alternatives: Promises and Async/Await
While call back functions have been foundational, new alternatives are gaining traction. Promises and async/await patterns represent major advancements. Promises provide a cleaner alternative to traditional call backs by enhancing readability and reducing the likelihood of nested structures. They allow developers to chain operations, making coding more intuitive and manageable.
Async/await builds on the promise concept. It allows writing asynchronous code in a style that looks synchronous, improving clarity. This approach essentially flattens the code structure, making it easier to follow.
"Using promises and async/await is not only about syntax; it's about embracing a more straightforward way to handle asynchronous tasks."
Developers will benefit from learning these alternatives, as they are becoming more widely adopted in modern programming environments. Their integration into mainstream libraries will likely further influence the way call backs are used in the future, ensuring they remain a relevant concept in an ever-changing technological landscape.
End
The conclusion section of any article is crucial for reinforcing the importance of the topic discussed. In this case, the exploration of call back functions, their significance, and practical applications enriches the understanding of programming paradigms for students and developers alike. Call back functions serve as a foundational concept in programming, particularly when handling asynchronous operations. They allow flexibility and modularity in code design, leading to clearer, more maintainable programs.
Summary of Key Takeaways
- Definition and Types: Call back functions are functions passed into another function as an argument and can be synchronous or asynchronous. Understanding the distinction is vital for programming efficiency.
- Practical Insights: In languages like JavaScript and C, call back functions enable event-driven programming, contributing to user responsiveness and system performance.
- Common Challenges: Developers face issues like callback hell, which can complicate code readability and maintainability. Awareness of these challenges helps in effectively managing call back functions.
- Best Practices: Adhering to best practices, such as ensuring proper context and avoiding common pitfalls, enhances the effectiveness of call back functions.
- Future Perspectives: The evolution of programming languages has introduced alternatives to traditional call back functions, like Promises and async/await, improving code clarity and flow.
Final Thoughts on Call Back Functions
In summary, call back functions represent an essential component of modern programming. Their versatility in handling operations, especially in asynchronous contexts, cannot be overstated. Understanding how to implement and debug these functions is critical for anyone looking to excel in software development. As programming continues to evolve, staying informed about new methodologies related to call back functions, such as Promises, will be beneficial. The study of call back functions not only aids in improving individual coding skills but also fosters a deeper comprehension of software behavior and design patterns.