Mastering Tokenization in Python for Text Processing
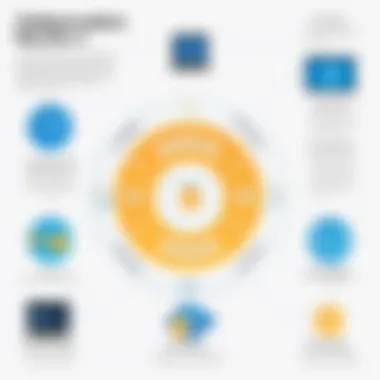
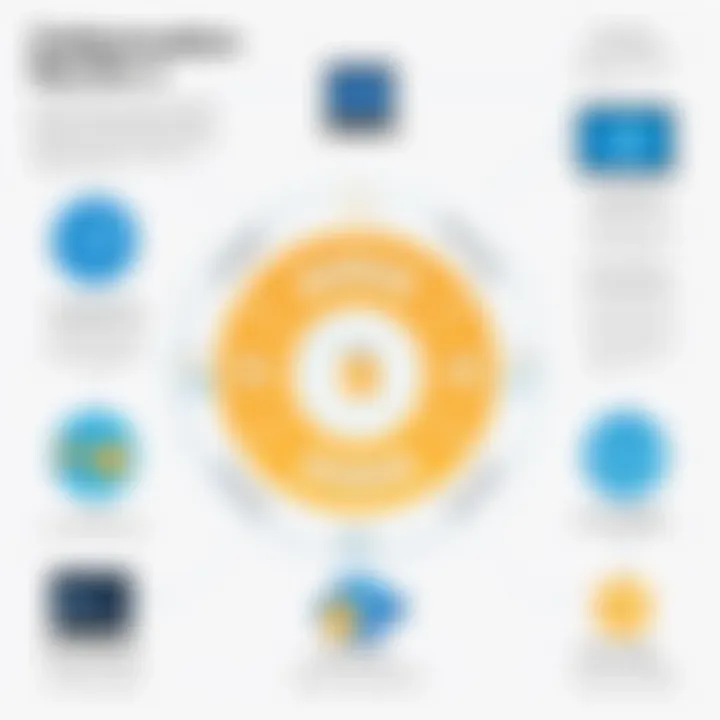
Intro
Tokenization plays a crucial role in the realm of natural language processing (NLP) and data pre-processing. At its core, tokenization involves breaking down a stream of text into smaller, manageable piecesâknown as tokens. These tokens can represent words, phrases, or even sentences, depending on the application. As Python has emerged as a leading programming language in this field, understanding its approach to tokenization is essential for anyone venturing into text processing.
The significance of tokenization canât be overstated. It's the first step in many NLP tasks, such as text classification, sentiment analysis, or even translation. When dealing with any form of textual data, you often need to preprocess it to machine-readable formats. This is where tokenization shines, making it a fundamental skill for developers and data scientists alike.
In this guide, we'll discuss various methodologies, tools, and libraries that help implement tokenization effectively in Python. Letâs explore why tokenization matters, along with some practical examples and best practices to enhance your coding skills in text processing.
Understanding Tokenization
To hammer home the idea, letâs clarify what we mean by tokenization in Python. Typically, this process involves dividing text into individual words or other types of tokens. For instance, the sentence "Hello world! How are you?" could get tokenized into the following tokens:
- Hello
- world
- How
- are
- you
You can see here how these tokens simplify later processing steps.
The Importance of Tokenization
Tokenization serves as the backbone for advanced techniques in natural language processing, such as named entity recognition and part-of-speech tagging.
For beginners and intermediate learners, grasping tokenization isnât just about writing code; itâs about understanding the nature of the data youâre working with. Text can be messy, full of inconsistencies, and tokenization helps make sense of this chaos. Letâs dive deeper into how you can leverage Python libraries for this task.
Libraries and Tools
Python boasts a rich ecosystem of libraries that facilitate smooth tokenization processes. Below are some of the most well-known libraries, which have become staples for developers.
- NLTK (Natural Language Toolkit): This library is like a Swiss army knife for NLP tasks. Its tokenization functions allow for straightforward sentence and word splitting. The flexibility and plethora of resources make it a go-to for many.
- spaCy: This is another powerful library that's user-friendly and efficient. It offers advanced tokenization methods that can handle complex linguistic features, making it particularly handy for large datasets.
- TextBlob: For those looking for simplicity, TextBlob could be perfect. It simplifies many processes, including tokenizationâideal for quick tasks and prototypes.
Practical Examples
Practical exposure often solidifies theoretical knowledge. Hereâs how tokenization can be applied using these libraries.
Example 1: Using NLTK
Hereâs a simple example of how to tokenize text with NLTK:
Example 2: Using spaCy
In this example, weâll employ spaCy for a more sophisticated tokenization:
These snippets show how easily you can tokenize strings with Python. Whether you stick to NLTK or opt for spaCy depends on your needs and preferences. Each library has its merits depending on the complexity of the text data.
Epilogue
Understanding tokenization is an essential first step toward mastering natural language processing with Python. By breaking down text into easily manageable pieces, you set the stage for more complex analyses and applications in NLP. As we move forward, itâs vital to practice these methodologies and keep building on the foundational knowledge of how tokens operate within your scripts.
Understanding Tokenization
Tokenization serves as a cornerstone in the realm of text processing. It might seem overly simplistic at first glance, yet its impact extends deeply into various domains, especially natural language processing (NLP). The primary function of tokenization is to decompose textual data into manageable units known as tokens, facilitating more complex analyses and manipulations that are fundamental in programming.
To really appreciate the importance of tokenization, it helps to think of it as the first step in a journey. Without this initial division of text, delving into deeper analyses like sentiment analysis or machine learning becomes akin to navigating a maze blindfolded. Each token acts like a breadcrumb that guides the algorithm, aiding it to comprehend sentence structure, meaning, and context.
Moreover, understanding tokenization is particularly crucial for learners in programming languages such as Python. It opens doors to myriad applications and frameworks that rely heavily on processing linguistic data. Grasping the essence of tokenization not only strengthens foundational skills but also enhances the ability to utilize various libraries and tools in Python that can refine text analysis.
"Tokenization is not just a technical necessity; it's the language of understanding in natural language processing."
Definition of Tokenization
At its core, tokenization is the process of splitting text into individual elements, or tokens. These tokens can be as short as a single character or may consist of entire words or phrases. For instance, taking the sentence "The quick brown fox jumps over the lazy dog," tokenization would yield the following tokens: "The," "quick," "brown," "fox," "jumps," "over," "the," "lazy," and "dog."
This division can be achieved using various methods such as whitespace tokenization, where text is split based solely on spaces. Alternatively, regex-based methods use regular expressions to define tokens, enabling more sophisticated handling of punctuation and special characters.
Importance in Natural Language Processing
Tokenization plays a vital role in many NLP tasks. Without it, further processing such as stemming, lemmatization, or even sentiment analysis would be challenging if not impossible. By converting text into tokens, algorithms are better equipped to parse the linguistic data, understand relationships, and comprehend contextual nuances.
Notably, one must consider the implications of maintaining the correct order and sequence when tokenizing. If tokens are mismanaged or incorrectly categorized, the meaning derived from the text can be severely altered. Hence, effective tokenization is paramount in building robust NLP applications.
Application Areas Beyond NLP
Tokenization isnât limited to just the confines of natural language processing. Its applications ripple out into several other areas. For example:
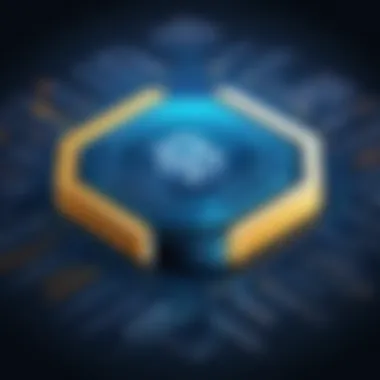
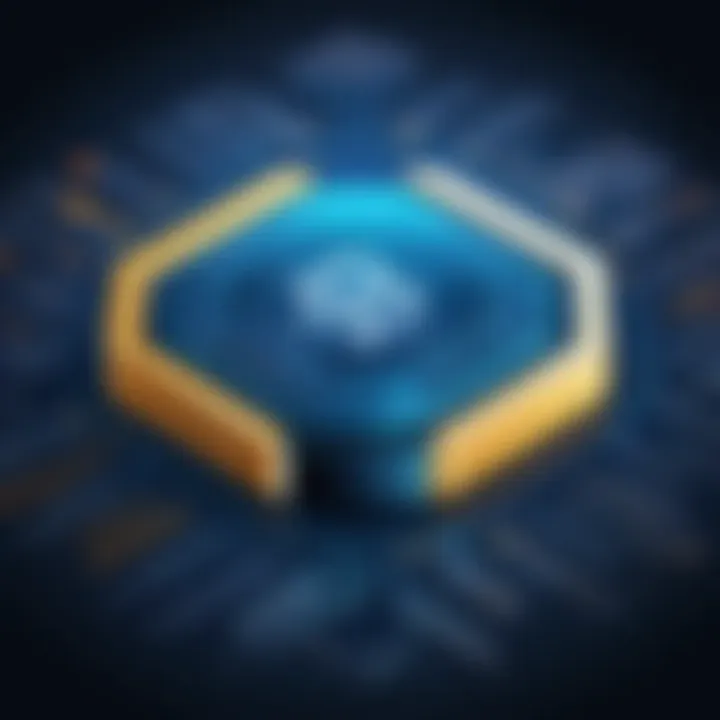
- Data Preprocessing: In data science, cleaning and organizing text data often starts with tokenization.
- Search Engine Optimization: Tokenizing content helps search algorithms match queries with relevant web content.
- Information Retrieval: Systems that fetch data often rely on tokenized data to improve accuracy.
In each of these scenarios, the ability to break text into manageable parts enhances understanding and facilitates better interactions with data. Thus, whether it's in machine learning, web development, or data analytics, mastering tokenization is an essential skill for programmers.
Tokenization Methods
Understanding different tokenization methods is crucial for mastering the art of text processing in Python. The choice of method can greatly affect the outcome of later analyses, such as sentiment analysis or machine learning tasks. Each approach has its own characteristics, benefits, and considerations to keep in mind, which can ultimately streamline your workflow.
Whitespace Tokenization
Whitespace tokenization is one of the simplest methods available. At its core, it divides text into tokens based on white spacesâthe gaps between words. This method proves functional for straightforward texts, where punctuation and other nuances don't necessitate specialized handling.
For instance, the string would be tokenized into using whitespace as the delimiter. While this technique is quick and easy to implement, it may not always produce desirable results in more complex texts that include contractions or punctuation. Some punctuation marks might become attached to words, leading to minor alterations in their intended meanings.
Regex-Based Tokenization
Regex-based tokenization takes things up a notch by allowing users to define custom delimiters. This method uses regular expressionsâpowerful tools for matching patterns in textâto create tokens that satisfy specific criteria. This flexibility enables the handling of complex scenarios, such as differentiating between various forms of punctuation and special characters.
For example, if you're interested in separating words but ignoring punctuation, you might employ the following regex pattern: . Such sophistication is useful when developing applications that need precise context from the source material, especially when nuances matter.
Library-Based Tokenization Techniques
Delving into libraries point us toward a plethora of powerful functionalities that can take our tokenization efforts to the next level. These libraries house pre-built functions that handle tokenization, saving significant time and effort, particularly for larger projects. Hereâs a brief look at three popular libraries in Python.
Using NLTK
The Natural Language Toolkit (NLTK) is a robust library designed to support various tasks in NLP. Its tokenization capabilities are no exception. NLTK offers easy-to-use functions like and that can break text into individual words and sentences, respectively. This key feature allows users to maintain a structured format of the data for easier analysis.
Moreover, NLTK comes with a rich assortment of corpora and linguistic data which can be invaluable for various language processing tasks. However, one of its downsides could be the library's size; beginners may find it daunting to integrate fully into their projects, given its extensive functionality.
Using SpaCy
Another powerhouse for NLP tasks is SpaCy, well-regarded for its efficiency and speed. SpaCyâs tokenization method is quick and convenient, with the added benefit of being able to recognize various aspects of the text, such as lemma forms and parts of speech.
A notable advantage of SpaCy is its easy-to-understand syntactic structure and powerful features, including entity recognition and dependency parsing. Itâs particularly beneficial for someone looking to dive deeper into NLP applications. However, its installation may be tricky for some users, given the need for compatibility with various operating systems.
Using Gensim
Gensim is a library specially tailored for topic modeling tasks and large text corpora. Though mainly recognized for its vectorization capabilities, Gensim also provides tokenization options, particularly useful for preprocessing data before subjecting it to more complex analyses.
The unique feature of Gensim lies in its ability to deal with massive corpuses effectively. It supports not just tokenizing text but also transforming it into various mathematical forms that can be beneficial for deeper analyses. Still, Gensim may be less intuitive for complete beginners who might find its focus on large datasets limiting their understanding of other tokenization practices.
Tokenization in Python: Libraries and Tools
Tokenization is not merely a foundational step in natural language processing; it sets the stage for many other processes critical to text analysis and understanding. When dealing with large volumes of data, simple and effective tools make all the difference. In Python, several libraries and tools stand at the forefront of tokenization techniques.
Preamble to NLTK
NLTK, or the Natural Language Toolkit, is a powerful library that offers a suite of tools for working with human language data. It caters to those just starting with natural language processing and seasoned pros alike. The library's comprehensive offerings are vital for anyone looking to perform tokenization effectively.
Installation
To get NLTK up and running, the installation is straightforward. While you can install it via the command line using , the real power comes when you set it up with its datasets. Afterward, it's necessary to download the corpora for optimal functionality. This process contributes significantly to starting your tokenization journey with ample resources.
One unique feature of NLTKâs installation is its ability to provide a wealth of built-in datasets right from the get-go. However, some users might find it overwhelming due to its sheer size and numerous options. Still, its robust nature and active community make it a top choice for education and experimentation in the realm of tokenization.
Basic Usage
Once installed, using NLTK for tokenization is quite intuitive. The function allows users to break down text into individual words easily. This functionâs flexibility stands out, making it suitable for various applications, from simple projects to complex analysis.
However, while NLTKâs basic usage is user-friendly, it does take some time to fully grasp the toolkit's full capabilities. This learning curve can be a drawback for those who want quick results. Nevertheless, the rich documentation and active discussions around it provide a supportive learning environment.
Exploring SpaCy
Unlike other libraries, SpaCy prioritizes efficiency and speed, making it a favorite among professionals who need fast processing of large texts. SpaCy's design focuses on practical usability. Its ability to integrate various models enhances its functionality immensely.
Installation
Installing SpaCy is a breeze and usually completed with a single command: . However, after that, youâd need to download a specific language model with commands like . This gives the library the context it needs to work wonders in text processing. The simplicity of this process is one of SpaCy's many draws; it allows users to hop into their projects swiftly.
What sets SpaCy apart during installation is its efficient memory management. This can be crucial for large datasets, as it avoids slowdowns that often accompany other libraries. However, new users may initially feel lost due to a lack of extensive tutorials.
Features Overview
SpaCy offers an impressive array of capabilitiesâit doesn't just do tokenization. This library also provides parts of speech tagging, named entity recognition, and much more. Its comprehensive feature set positions SpaCy as an all-in-one solution for many real-world tasks.
The most distinguishing aspect of SpaCy is its focus on production. Unlike other libraries that focus more on educational aspects, SpaCy is built with efficiency in mind, making it suitable for application in commercial environments. However, its production-ready design sometimes sacrifices depth in linguistic processing, which might not suit every researcherâs needs.
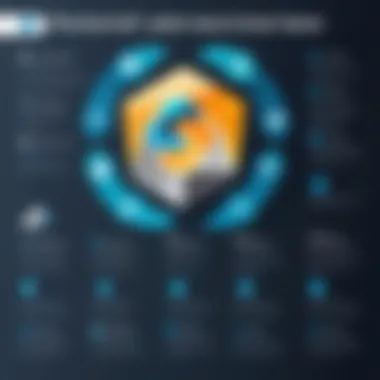
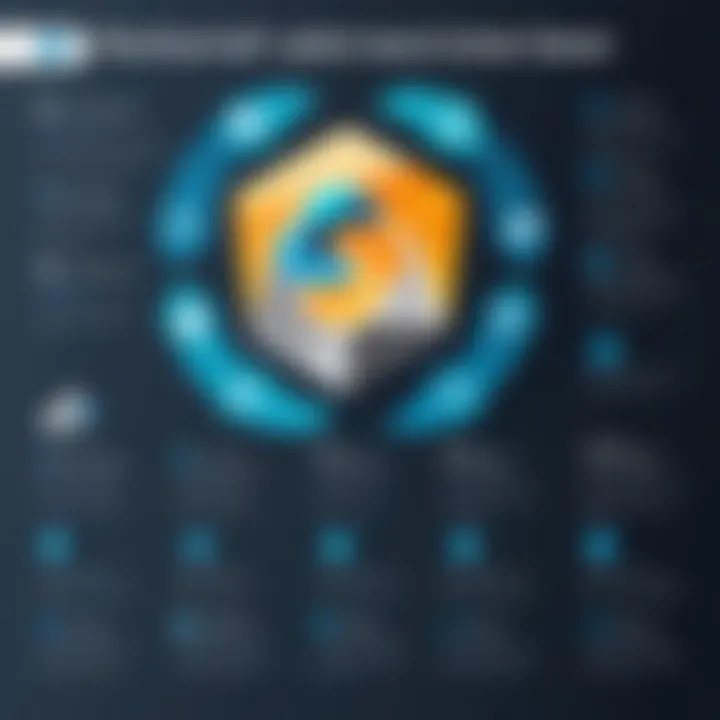
Gensim: Its Role in Tokenization
Gensim, primarily used for topic modeling and document similarity analysis, has a specific place when it comes to tokenization as well. It focuses on understanding semantic relationships in text, which is essential for more complex analyses.
Installation
To install Gensim, the process mimics that of earlier libraries: . While relatively simple, the libraryâs niche in vector space modeling might require further understanding of its dependencies. Installing Gensim opens doors to multiple advanced functionalities that other libraries lack.
A unique quirk about Gensim's installation is its optimization for compatibility with other libraries like NumPy and SciPy. This capability enables seamless integration for users who want to delve deeper into machine learning after tokenization. On the downside, users must ensure that they have a solid grasp of the mathematical concepts involved, or the benefits might just fly over their heads.
Practical Examples
Gensim shines in practical examples, especially concerning its ability to process large text corpuses. For instance, using the function, users can efficiently tokenize and preprocess text data for modeling. This capability highlights Gensim's strength in dealing with real-world datasets.
The unique feature of Gensim is its ability to manage large datasets dynamically. This means users can start working with extremely large sets of documents without running into performance hits. However, many new users might find the API and the advanced functionalities somewhat perplexing, requiring them to dig deeper into Gensimâs documentation for full mastery.
In summary, the landscape of tokenization libraries in Python offers various options that serve distinct needs. From NLTK's broad scope to SpaCy's speed and Gensim's focus on topic modeling, each library has unique characteristics that motivate their use in different contexts.
Practical Applications of Tokenization
Tokenization, while often acknowledged as a foundational step in the realm of natural language processing, finds its practical applications spanning numerous domains. Its significant role cannot be overstated, especially in the context of preparing data for various analytical tasks. When we talk about practical applications of tokenization, we are looking not only at the algorithms and libraries that facilitate the process but also at how these elements come together to solve real-world problems. Each application harnesses the power of tokenization to extract meaning from unstructured text in a structured manner. This structured approach enhances the efficiency of subsequent processing stages, be it machine learning or simple data analysis.
Text Preprocessing for Machine Learning
Before a machine learning model can make sense of the data it's fed, proper preprocessing must occur. In this context, tokenization acts as the first gatekeeper. By breaking down the text into manageable pieces, it transforms raw data into a form that's ready for analysis. For instance, in a scenario where raw chat logs from customer service interactions need to be analyzed for patterns, tokenization allows the extraction of individual words and phrases, facilitating easier pattern recognition. In more technical terms, by segmenting text, tokenization enables models to understand features which are essential for training.
Here's a simplified outline of how this preprocessing can unfold:
- Cleaning the Data: Strip out unwanted characters, such as punctuation or excess whitespace.
- Tokenization: Convert sentences into tokens (words, phrases).
- Normalization: Apply processes like stemming or lemmatization to ensure tokens are compared uniformly.
- Vectorization: Transform tokens into numerical representations for machine learning algorithms.
This systematic approach illustrates how tokenization lays the groundwork for successful model development, making it a critical element in many machine learning workflows.
Sentiment Analysis
In sentiment analysis, tokenization plays a pivotal role by enabling the breakdown of text data into components that can be analyzed for emotional tone. By distilling user-generated content, such as social media posts or customer reviews, into fundamental bitsâtokensâanalysts can gauge opinions more effectively. For instance, in an analysis of product reviews, tokenization helps identify keywords like "love" or "hate", which can significantly influence the sentiment score assigned to a product.
Sentiment analysis typically follows these steps:
- Input Collection: Gather text data from platforms like Reddit or social media.
- Tokenization: Break down the text to extract individual tokens.
- Feature Extraction: Determine which tokens carry sentiment, often emphasizing adjectives and verbs.
- Classification: Utilize machine learning or rule-based engines to categorize sentiment as positive, negative, or neutral.
The ability to process sentiments quickly and accurately can provide companies with a wealth of information, guiding product development, marketing strategies, and customer engagement efforts.
Text Classification
Tokenization is equally essential in text classification tasks where categorizing text documents into predefined classes is necessary. By providing a structured format to the unstructured data, it improves the efficacy of classification algorithms. For example, when working with news articles, tokenization splits the text into relevant segments, making it easier for algorithms to identify themes or topics.
Key steps involved in text classification include:
- Data Collection: Accumulate the texts to be classified.
- Tokenization: Segment text into tokens, ensuring critical phrases are captured.
- Labeling: Assign categories to the data based on the intended outcome.
- Algorithm Training: Utilize supervised or unsupervised learning techniques to train a model on labeled data.
- Evaluation: Test the model on new texts to measure its classification accuracy.
Tokenization, in this context, serves as the backbone for turning lengthy articles into digestible segments that classification algorithms can work with effectively.
In summary, whether itâs preparing data for machine learning, reading sentiments, or classifying text, tokenization paves the way for deeper insights and smarter data interaction. Its ability to simplify complex text into manageable units ensures that these sophisticated tasks can be performed with greater ease and accuracy.
Challenges in Tokenization
In the realm of text processing, tokenization is the cornerstone, but like most foundational techniques, it carries its own set of challenges. Understanding these challenges is crucial, especially for those learning programming or diving deeper into natural language processing (NLP). Tokenization isn't just about splitting text; it involves intricate decisions regarding context, language, and intended application. The ability to navigate these challenges can significantly enhance the performance of any NLP model.
Ambiguity and Context
Tokenization thrives in clarity. Yet, language is laden with ambiguity, making it a slippery slope for tokenizers. Consider a phrase like "I saw her duck". Here, one might interpret "duck" as a verb or a noun, and the role of "her" adds another layer of complexity. Different contexts yield different meanings. When a tokenizer splits this sentence, how should it handle such cases?
This ambiguity is a serious consideration. A naive approach that divides solely based on spaces might misinterpret the text. If we donât adapt our techniques to account for contextual cues, we risk distorting the true meaning behind the words.
"Context is not just king, it's the entire kingdom when it comes to human language."
Therefore, sophisticated tokenizers employ strategies that assess context, allowing for more intelligent decisions regarding token boundaries. Advanced implementations include supervised learning models that can better detect and preserve meaning across various scenarios.
Handling Special Characters and Punctuations
Another significant hurdle in tokenization is managing special characters and punctuation. While some characters like periods or commas can act as natural boundaries, take a look at examples like "let's", "mother-in-law", or "C++ programming". Here, we see a need to balance between breaking words down and maintaining their integrity.
Ignoring punctuation can lead to confusion; for instance, treating "I'm" as two separate tokens ('I' and 'm') fails to recognize the contraction. Proper handling can preserve the essence and readability of the text.
Utilizing regex expressions and whitespace tokenization can help curate a more effective segmentation. A layered approach often yields better results, allowing tokenizers to adapt to varying sentence structures and punctuation styles.
Language-Specific Intricacies
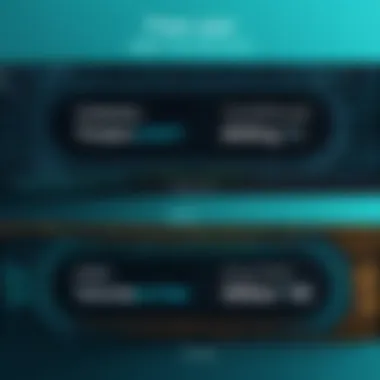
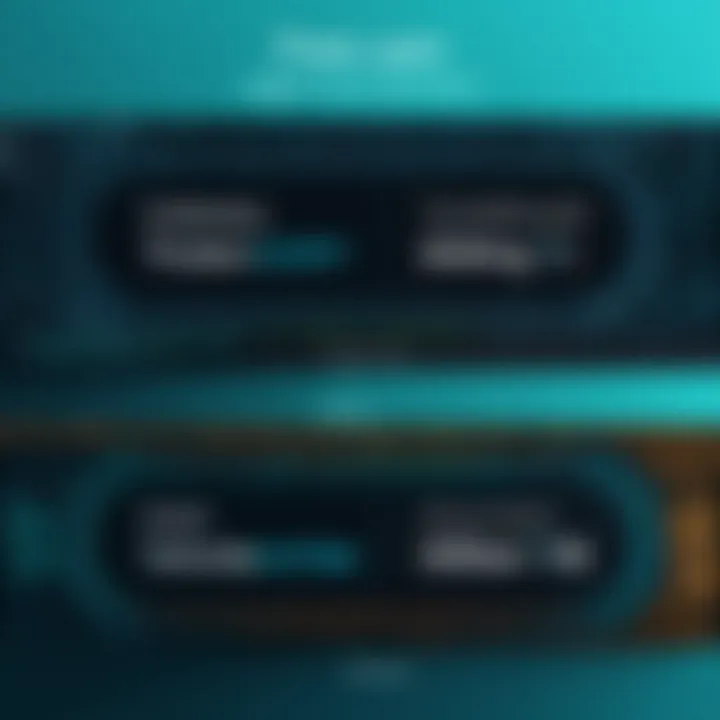
When it comes to tokenization, one size definitely does not fit all. Different languages exhibit unique characteristics. For instance, Mandarin Chinese doesn't space its words, creating a fundamental challenge for straightforward tokenization techniques. In such cases, tokenizers may rely on machine learning-based segmentation to identify word boundaries.
Furthermore, languages that employ inflections or agglutination possess their intricacies. In German, for example, compound nouns can merge multiple concepts into a single word, posing challenges for naive splitters. Here, understanding the language's structure becomes critical to creating an effective tokenizer.
To develop robust tokenization approaches, it is vital to consider these language-specific challenges. Building tokenizers that adapt to diverse languages while respecting their unique characteristics can significantly improve the quality of results for multilingual applications.
In summary, the challenges in tokenization require careful thought and a nuanced understanding. Addressing ambiguity, managing punctuation, and recognizing language-specific intricacies are crucial steps in achieving effective text processing in Python.
Advanced Tokenization Techniques
Tokenization is more than just breaking down text; it influences how data is processed in natural language processing (NLP). Advanced tokenization techniques serve as the backbone of nuanced and effective text analysis, allowing developers and data scientists to extract meaningful insights from large volumes of text. These methods tackle complexities associated with language, ensuring that the final token output is useful for machine learning models and other applications. In this section, we will discuss three major advanced tokenization techniques: subword tokenization, wordpiece tokenization, and byte pair encoding, shedding light on their importance, application, and impact.
Subword Tokenization
Subword tokenization assists in managing rare or new words that might not be present in a language model's vocabulary. By segmenting words into smaller units, it creates a more flexible mechanism for understanding and generating text. For example, rather than treating "bicycling" as a whole, it can be broken down into "bicycle" and "ing." This breakdown allows models to learn from familiar components regardless of the specific combination. Moreover, subword tokenization minimizes the risk of encountering out-of-vocabulary terms, making it exceptionally valuable in domains like machine translation and text generation.
By adopting subword techniques, models maintain a richer understanding of language nuances and improve their fluency across various applications.
In practice, developers often leverage libraries like SentencePiece and BPE (Byte Pair Encoding) that facilitate this advanced form of tokenization, ensuring their models remain robust even when encountering nonstandard text.
Wordpiece Tokenization
Wordpiece tokenization is akin to subword tokenization but specifically used within frameworks like BERT. It enhances performance by training on larger datasets, allowing it to break down words into meaningful pieces based on their frequency. The premise here is that common prefixes, suffixes, or roots (like "un," "ing," and "able") can be utilized to form new words while still retaining their meanings.
This approach has a double benefit: it improves the model's accuracy and compresses the vocabulary size, which helps in reducing the computational burden during training and inference. As a result, NLP tasks become more efficient and effective, addressing the common challenge of high-dimensional data. For instance, the word "unhappiness" may be split into "un," "happy," and âness" to present various word forms to the model, enhancing its response accuracy.
Byte Pair Encoding
Byte Pair Encoding (BPE) is an efficient algorithm used to tokenize text by iteratively replacing the most common pair of characters with a new single character. Initially, the text is represented as individual characters. Then, BPE builds a vocabulary based on the frequency of adjacent characters, effectively creating new tokens for commonly recurring patterns. This technique proves incredibly useful for languages with rich morphology and can handle uncommon phrases efficiently.
For instance, if the phrase "most common" appears frequently in a dataset, BPE may combine it into a single token over time. This not only optimizes space but also enhances the model's capability to recognize frequently occurring expressions. Developers can implement BPE through libraries like Hugging Face's Tokenizers, which provide easy-to-use interfaces for custom tokenization strategies.
In summary, advanced tokenization techniques play a pivotal role in processing data effectively. By breaking down language components into manageable, meaningful units, these methods enable more sophisticated analysis and application in machine learning models, ensuring better performance in various NLP tasks.
Best Practices in Tokenization
When delving into the realm of tokenization in Python, there are several best practices to bear in mind. These practices guide us in achieving the highest quality results, which is especially crucial when dealing with tasks such as Natural Language Processing (NLP). Adhering to these principles not only aids accuracy but also improves overall system efficiency.
Choosing the Right Method
Selecting an appropriate tokenization method is a pivotal step in the preprocessing phase. Each method can yield different results based on the nature of the text and the intended use of the tokens. Consider the following:
- Nature of the Data: For instance, if youâre processing straightforward texts like news articles, whitespace tokenization might suffice. However, for complex data with diverse symbols or even slang, regex-based tokenization may be the better choice.
- Language Considerations: Different languages have unique syntactical rules. Methods like subword tokenization can be particularly effective for languages with rich morphology, such as German or Turkish.
- Technology Stack: The choice can also depend on the libraries you plan to use. Libraries like NLTK and SpaCy offer pre-defined methods tailored to varied datasets, thus streamlining the selection process.
Ultimately, testing a few methods on a subset of your data may reveal which works best for your specific needs, setting a solid foundation for your project to flourish.
Preprocessing Steps to Enhance Tokenization
Before proceeding with tokenization, preprocessing of the text can greatly enhance the accuracy and efficiency of the process. Below are suggested steps:
- Lowercasing: Normalize the text by converting it to lowercase, which can help eliminate duplicates of the same word in different casings.
- Removing Noise: Special characters, digits, or irrelevant symbols might confuse the tokenization process. It's generally useful to strip out all unwanted characters.
- Handling Contractions: Expand contractions (like "donât" into "do not") to avoid tokenization issues in certain contexts.
- Stemming and Lemmatization: While this applies more broadly to NLP, understanding the base form of words can help in later stages, especially in classification tasks.
By applying these preprocessing steps, you set yourself up for a seamless tokenization process. Itâs like cleaning your workspace before an important project. A tidy message yields clearer tokens.
Testing and Validating Tokenization Outputs
Once tokenization is performed, it's crucial to assess the outputs to ensure they meet the intended criteria. Here are recommended practices:
- Manual Inspection: Start with a quick overview of a sample output. Are the tokens behaving as expected? If you see anomalies, it might warrant revisiting earlier steps.
- Statistical Checks: Use metrics like token counts or frequency distributions to evaluate if the tokenization aligns with expectations. Unexpected results can often signal an issue in the preprocessing phase.
- Unit Testing: Integrate tests into your workflow that verify the integrity of the tokens. This could involve ensuring that certain phrases or expected entities remain intact after tokenization.
- Feedback Loop: Adjusting your methods based on feedback from these tests can lead to incremental improvements. Always be ready to revisit your initial choices based on outcomes observed.
Implementing these practices will not only polish your tokenization process but will also reinforce your overall text processing model. Keep an eye on emerging tools and techniques in tokenization, as the field continues to evolve.
Closure
In wrapping up this exploration of tokenization in Python, it's essential to appreciate its pivotal role in processing textual data. Throughout the article, we have delved into the fundamentals of tokenizationâwhat it is and why it matters, especially in the realm of natural language processing. From various methods like whitespace tokenization to more complex techniques involving libraries such as NLTK and SpaCy, we have laid a comprehensive groundwork. The practical applications of tokenization were also highlighted, showing its necessity in fields like sentiment analysis and text classification.
The importance of understanding the intricacies of tokenization, especially for those learning programming languages, can't be overstated. It not only enhances the efficiency of data preprocessing but also serves as a stepping stone into more advanced concepts in natural language processing.
"The ability to break down text into meaningful parts can transform raw data into invaluable insights."
As you move forward, keep in mind that selecting the right tokenization method often depends on the specific use case and the characteristics of the text. Considerations such as handling ambiguity and language-specific nuances are crucial. Moreover, by adhering to best practices, including diligent testing and validation, you can ensure more accurate and reliable outcomes in your applications.
Recap of Key Points
- Understanding Tokenization: Tokenization is the process of dividing text into smaller parts, or tokens, which can be words, phrases, or even characters.
- Methods of Tokenization: We explored methods like whitespace tokenization, regex-based approaches, and library-based techniques.
- Libraries and Tools: NLTK, SpaCy, and Gensim are noteworthy tools that offer diverse functionalities for effective tokenization.
- Practical Applications: Tokenization plays a crucial role in various tasks including preparing text data for machine learning models and performing sentiment analysis.
- Challenges: Tokenization can be tricky due to ambiguity in language, special characters, and specific language characteristics.
- Best Practices: Itâs important to choose the right method, enhance preprocessing steps, and validate outputs for optimal results.
Future Prospects in Tokenization Research
Looking ahead, the field of tokenization is ripe for growth and innovation. As natural language processing continues to evolve, new methods are likely to emerge, focusing on the increasing complexity of language data. Emerging research could lead to the development of hybrid models that intelligently combine various tokenization strategies to handle different languages and contexts more effectively.
Advancements in neural network architectures may also influence tokenization techniques. For instance, the integration of contextual embeddings like BERT may drive tokenization models towards embracing a more holistic view of language, promoting the understanding of relationships between words based on their surrounding context.
Moreover, as the data landscape grows exponentially, techniques like subword tokenization and adaptive tokenization will become invaluable for accommodating rare words and mitigating vocabulary constraints.