Mastering Timeouts: Enhancing Your Code Strategy
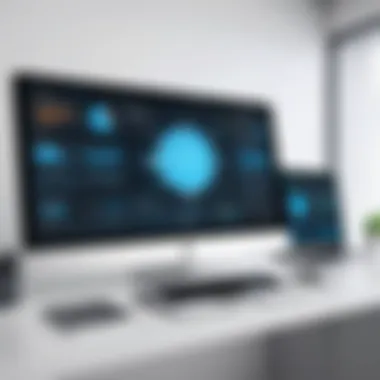
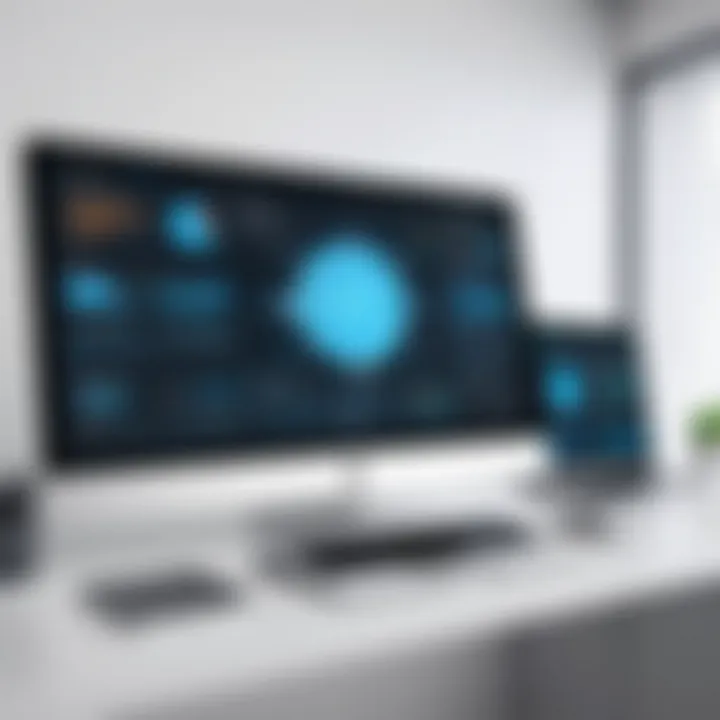
Intro
Timeouts play an significant role in programming, especially in scenarios involving asynchronous operations or managing resources effectively. Their importance touches on performance and user experience, silently impacting both.
We will cover their implementation in popular languages such as JavaScript, Java, and C++. Understanding how these timeouts function is crucial for developers at any level. In a hands-on guide, we will explore various aspects of managing time and resources within your code.
Prelude to Programming Languages
History and Background
Programming languages have evolved dramatically, reflecting the needs of developers and the technology landscape around them. From early languages like Assembly to modern multifaceted languages, enhancements emphasized clearer structure, robust functionality, and increased efficiency. Recognizing the history of these languages can enrich a programmer's approach to solving complex issues, such as the application of timeouts.
Features and Uses
Programming languages today emphasize several features, including readability, efficiency, and community support. With timeouts becoming a staple in resource management, they assist in preventing applications from getting stalled, mismanaging memory, or crashing due to unresponsive operations. They allow developers to create responsive interfaces that are vital for enriching user experience.
Popularity and Scope
Languages like JavaScript, Java, and C++ are incredibly popular in this domain due to their versatility. Each language offers unique features for managing tasks and implementing timeouts.
Basic Syntax and Concepts
Considering all the languages that deal with timeouts, it’s essential to revisit essential programming concepts first.
Variables and Data Types
Start with understanding basic variable concept. Variables store data that can change, while data types like integers,ly floats, and strings hold specific kinds of information. Timeouts also hinge on these factors, as improper types can lead to unexpected results.
Operators and Expressions
Knowing how to handle operators helps in forming expressions that aid functionality. Variables paired with logic create behavior conducive to timeouts.
Control Structures
Control structures such as loops and conditionals are critical for ensuring your program flow effectively handles timeouts and responds to events or conditions changing states.
Advanced Topics
Understanding advanced topics can leverage your programming effectiveness.
Functions and Methods
Defining reusable functions suits the requirement of implementing timeouts. For instance, you might create a function handling asynchronous calls with a specific timeout duration.
Object-Oriented Programming
Languages like Java and C++ use classes which can encapsulate behaviors and attributes. At this level, setting timeouts in class methods can enhance their reliability by ensuring timings are correct through instantiation.
Exception Handling
Defensive programming elevates application resilience. It includes properly managing exceptions triggered by timeouts or computation overruns.
Hands-On Examples
Real coding experience is beneficial for internalizing concepts.
Simple Programs
Creating simple applications like a basic timer application can offer insights into properly managing and implementing timeouts from scratch.
Intermediate Projects
Explore working methods of timeouts in asynchronous programming by developing projects that fetch remote data while handling inevitable latency issues.
Code Snippets
Some basic JavaScript code for implementing a timeout would look like this:
Resources and Further Learning
Exploring available resources can boost your knowledge and skills further.
Recommended Books and Tutorials
Look into titles like "You Don’t Know JS" and "Effective Java". Both focus on deepening your understanding in nuanced programming concepts.
Online Courses and Platforms
Websites like Codecademy and Coursera provide structured learning paths in various programming languages.
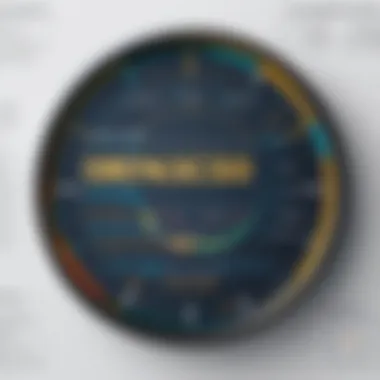
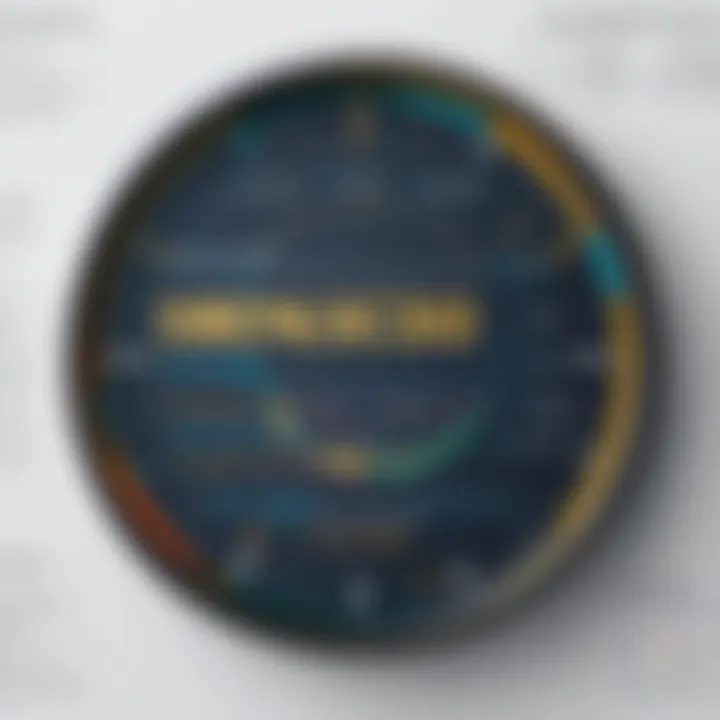
Community Forums and Groups
Engaging in development communities like Reddit or directly connecting through platforms like Facebook can offer support from peers in your journey.
Timed operations enhance our applications, a vital component for inevitable interactions in programming.
Understanding Timeouts
Timeouts play a significant role in computer programming, serving as a crucial tool for managing processes and ensuring the efficiency of applications. In the context of unpredictable network conditions and user interactions, implementing timeouts becomes essential. Without them, a program may hang indefinitely, waiting for a response that may never come. This can lead to a poor user experience and eventual application failure. Therefore, understanding how to work with timeouts can enhance reliability and responsiveness.
Definition of Timeouts
In programming, a timeout defines a predetermined duration during which a program waits for a specific task to complete before aborting the attempt. When the task exceeds this duration, the program can either handle the situation gracefully or alert the user of the failure. Writers often refer to timeouts in different contexts, like I/O operations, network requests, or function execution. Each case retains similar principles but differs in implementation specifics. Essentially, a timeout helps to avoid getting stuck and gives a chance to proceed with alternative actions
It is also crucial to distinguish between hard and soft timeouts. A hard timeout forcibly kills a task after the maximum duration; a soft timeout may signal the process to end gracefully before forcefully terminating it.
Importance in Programming
The importance of timeouts cannot be overstated. They are vital for maintaining the performance and integrity of applications. Here are some key benefits:
- Managed Execution: With timeouts, long-running processes can be controlled. Without them, performance issues or resource starvation may occur.
- Improved User Experience: Users encounter fewer frustrations when programs respond predictably, rather than freezing unexpectedly due to locked processes.
- Degraded Performance Handling: In production environments, implementing timeouts can help in running diagnostics or recovery routines when performance degrades.
- Testing and Debugging Simplification: Timeouts aid in highlighting where in the code delays or hanging occurs, making it easier to identify problematic areas.
Set Timeout in JavaScript
JavaScript offers a valuable tool in the form of , which plays a pivotal role for developers aiming to control timing within their applications. Understanding this method allows one to manage execution order efficiently, particularly in asynchronous programming workflows. For instance, developers can prevent performance bottlenecks that arise when multiple processes run simultaneously, or provide a smooth user experience by executing tasks after a specific delay.
Syntax and Basic Usage
The syntax for is quite straightforward:
- function: This is the function to execute after the timeout expires.
- delay: This value is the time to wait before executing the function, measured in milliseconds.
- params: Optional. Values to be passed to the function when it is invoked.
Here is an example demonstrating basic usage:
In this example, the message will appear in the console after a 2-second delay. This capability significantly enhances the fluidity of narratives within user interfaces.
Common Use Cases
Implementing timeouts creates many practical scenarios:
- Delaying UI Updates: When developing a web page, it can be beneficial to display a message temporarily or perform updates at specific times. This action helps manage user attention effectively.
- Game Timing: Games often utilize to control events. For example, introducing a temporary pause before reloading the game state contributes to user experience.
- Form Validation Effects: If a user makes an error while filling a form, you can highlight the error message after a brief timeout, allowing their action to process clearly before displaying feedback.
Handling Callbacks
Effective handling of callbacks is essential to harness the full power of . JavaScript uses event-driven programming, meaning certain functions should run once events occur. Managing these asynchronous calls allows developers to avoid inconsistent states.
Utilizing within callbacks can introduce complexities, especially if nested. A common pitfall is neglecting to clear timeouts when they are no longer necessary. For example:
Properly removing scheduled executions helps free resources and avoid unnecessary executions. Understanding these elements reinforces how critical the right implementation of timeouts can be in JavaScript applications.
Set Timeout in Java
In the realm of programming, timeouts hold significant value, particularly in Java. Implementing timeouts can greatly enhance the efficiency and resilience of applications. A timeout signifies a predetermined duration that a process is allowed to execute before it gets halted, thus preventing hangs or delays in functionality.
Setting timeouts is invaluable in managing resources. For developers, incorporating timeout mechanisms translates to better performance and improved user experiences. Applications can gracefully handle instances where operations exceed expected durations, fostering responsive applications. Below are key elements enthusiasts need to know when working with timeouts in Java.
Key considerations for using timeouts in Java:
- Code Efficiency: Timeouts help reduce waste of computational resources, improving efficiency.
- User Interaction: Instant feedback to the user becomes achievable. Users do not wait indefinitely for processes to finish.
- Error Management: Incorporating timeouts makes it simpler to manage unexpected faults within processes.
- Usability Enhancements: Controlled execution leads to elevated end-user satisfaction.
Timely intervention through the right timeout strategy becomes crucial for developers aiming to optimize performance and reliability.
Java Timer Class
The Timer class in Java is a powerful tool for scheduling tasks that require execution after a specified delay or at fixed intervals. This class provides a way to run background tasks seamlessly without blocking the main program execution. When tasks need to be executed in the future, developers often consider using the Timer class.
Features of the Java Timer Class:
- Single and Repeated Tasks: Flexibility to define one-off tasks or a series of automated actions at regular intervals.
- Thread Management: Runs tasks in a separate thread, ensuring that the main thread continues functioning smoothly.
- Granular Control: Enables the user to define task execution policies, optimizing flows without locking resources excessively.
The class makes it hassle-free for developers to focus on the business logic instead of managing thread states directly, leaving room for enhanced development efficiency.
In the example above, a task simply prints a message after a 2-second delay, highlighting the Timer class’s functionality for timed method execution.
Implementing Delayed Tasks
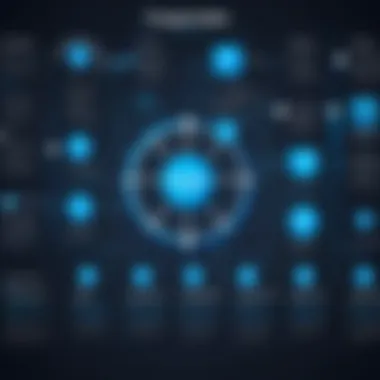
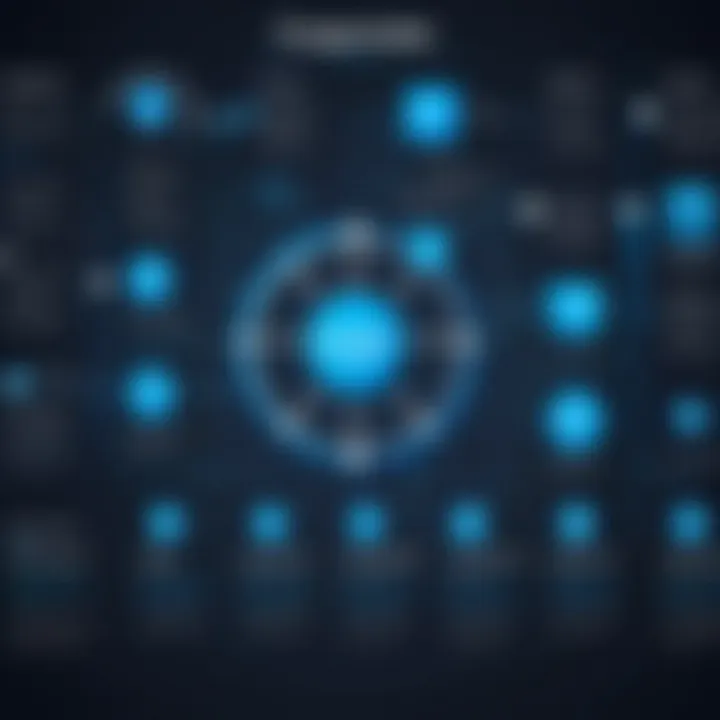
Delayed task implementation is crucial in Java programming for scenarios where actions must occur after specified durations. This process typically utilizes various techniques depending on the context and requirements. Many applications need precise execution time, whether it is about managing user sessions, orchestration of background jobs, or merely delaying tasks in relative time.
Common strategies include:
- Using ScheduledExecutorService: This service enhances thread control and scheduling.
- Combining CountDownLatch: Helpful for waiting until a specific condition is met after timeout expiration.
- Executors. DelayQueue*: Useful for designing priority-based delays within concurrent environments.
Balancing between necessary task delays and the required responsiveness often depends on user expectations and application performance goals. Establishing a clear understanding of delayed task mechanics through formulations ensures efficient execution of background processes and improved manageability of application flow.
“Implementing timeouts effectively can transform the way applications respond to users. Non-blocking behaviors become a key asset in modern applications.”.
Set Timeout in ++
Setting timeouts in C++ is an essential aspect of control flow management, especially in applications that rely on asynchronous execution. The use of timeouts allows programmers to ensure that their system can respond promptly to unexpected events or, conversely, wait for a task to complete over a limited duration. This is particularly useful in networking, user input handling, or time-sensitive computations.
Standard Library Options
C++ provides several standard library options for implementing timeouts. Two commonly used components include and . These libraries offer precise timing functionalities that can simplify the implementation of timeouts.
- std::thread: This allows the creation of threads that can execute tasks concurrently. You may manage threads alongside timing requirements by controlling their lifetimes.
- std::chrono: This library enables precise timekeeping. You can specify durations in terms of seconds, milliseconds, and even microseconds, thus offering flexibility in programming.
A common approach is to launch a new thread for a task and allow it to run for a defined time period. Here’s a simple demonstration:
This snippet demonstrates a simple timeout. However, it's key to note how to handle cases when tasks do not logically conclude within expected timeframes. One solution involves using conditions or flags checked alongside time measurements to handle unexpected execution durations.
Thread Management and Delays
Handling threads in conjunction with timeouts can present challenges. Proper management is critical to prevent resource leaks or deadlocks. Normally, when using timeouts, threads that execute long-running tasks should be carefully monitored.
Currently, there are strategies for effectively managing delays in threads:
- Timeout with termination: Check for task completion within a timeout and terminate the process if needed. Determining whether it is suitable to use forceful termination requires content understanding of ramifications to the state of the principal program.
- Polling strategy: Use a polling loop that checks the state of the main condition of the task at defined intervals.
When implementing delays, here is a brief code example:
In this case, the stalled operation (the long-running task) is compatible with a polling strategy. Depending on the design of programs, the execution context should keep running even while waiting for conditions to be met.
Timeouts in Asynchronous Programming
Asynchronous programming relies heavily on managing time and concurrent processes. Implementation of timeouts is a crucial element that helps prevent tasks from running indefinitely. Not only this improves the overall efficiency of applications, but it also leads to a better user experience.
Understanding timeouts allows developers to have more control over how functions behave, especially when they interact with external services or perform heavy computations. Attach a timeout to an operation, and you create a safety net. If the operation doesn't complete within the designated time frame, the associated action can be triggered without the need for manual termination. This feature handles unexpected delays and maintains application responsiveness.
Timeout not only conserves resources but also enhances error detection in asynchronous workflows. The proper management of timeouts can avoid resource contention, leading to better performance across server-client interactions.
Event Loop and Scheduling
In environments like JavaScript, the event loop concept is foundational. The event loop orchestrates the execution of code, collects and processes events, and schedules tasks. The inclusion of timeouts directly impacts how the event loop prioritizes operations. When you set a timeout, the function does not block subsequent code; instead, it places the function in the callback queue after the delay expires.
Understanding this interplay helps developers predict how their program will react in different scenarios. In JavaScript, for instance, once a timeout duration passes, the designated function will execute whenever the call stack is clear, significantly influencing the scheduling of tasks. The efficient utilization of timeouts enhances the handling of tasks and their scheduling in response to events.
Here is an example of how to use a timeout with the event loop:
Error Handling and Timeouts
Implementing timeouts not only serves a systematic scheduling purpose but also significantly enhances error handling mechanisms. When working with external APIs or operations dependent on remote resources, the chances of unpredictable failures or slow responses increase.
By placing timeouts in such cases, developers can create fallback procedures. This allows for graceful degradation in the application functionality. If an API call does not return data in time, you can initiate a timeout callback that triggers an error notification. This contributes to an overall more robust application stack.
Things to keep in mind when implementing timeouts include:
- The maximum time period set for operations, based on the average response rates of services used.
- Logging timeout events to diagnose issues or improve performance in the long run.
- Incorporating retries as part of your strategy to manage timeouts efficiently locates the balance between user experiences and resource management.
Remember, well-considered timeouts not only improve user engagement but simplify detected lead times and lower environmental strain on your systems.
Best Practices for Implementing Timeouts
Implementing timeouts effectively is essential in programming, particularly in enhancing performance and user experience. Appropriate timeout settings can prevent applications from hanging or freezing, especially in network-bound operations or query executions. Understanding the context and requirements of your application will determine your approach to implementing timeouts.
Choosing the Right Timeout Duration
Selecting an appropriate timeout duration is crucial for balancing responsiveness and operational reliability. Too short of a timeout can lead to premature termination of essential processes. Conversely, overly long timeout durations may result in users experiencing significant delays if something goes wrong.
When deciding on the timeout duration, consider these factors:
- Nature of the Task: Different operations have different time expectations. Small tasks, such as retrieving a value from a local database, generally require a shorter timeout than a data fetch from a third-party API or a remote server.
- User Expectations: Understanding your audience benefits both performance and usability. Analyze feedback and user behavior to gauge the expected timing for operations.
- Error Handling Procedures: Implement resilience strategies that expose users to informative messages or recovery options when timeouts occur.
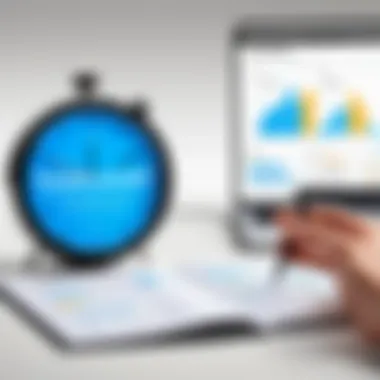
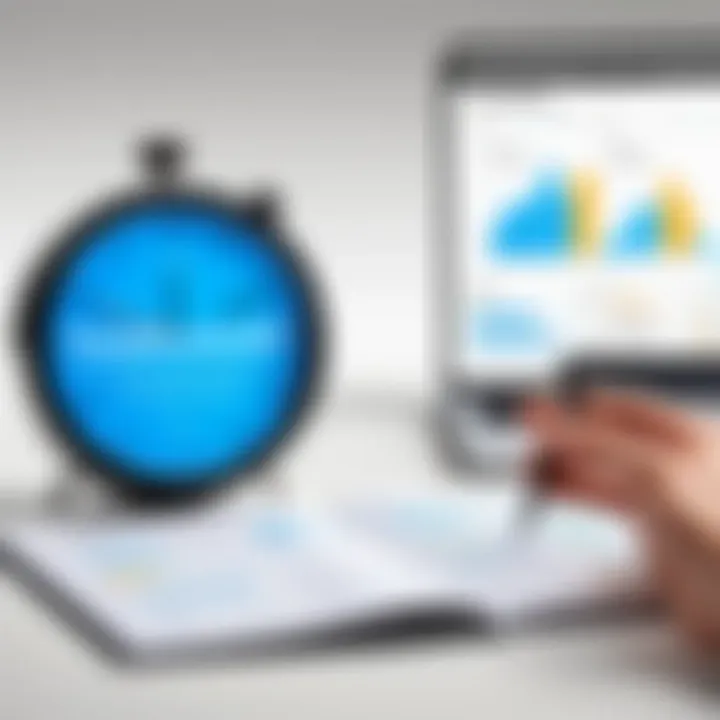
In code, an example JavaScript timeout implementation could look like this:
This method allows developers to execute a function after a set time. However, the time set can be adjusted as per current requirements and predictive user activity.
Managing Long-Running Processes
Long-running processes often present unique challenges. They can exceed acceptable runtimes and lead to inefficiencies in resource utilization. To deal with this effectively, a comprehensive management strategy must be exercised.
Consideration points include:
- Checkpointing and Resumability: Implement checkpoint strategies for long tasks which can pause the process and later resume, minimizing resource waste and providing improved reliability.
- Thread Management: Utilize proper mechanisms, such as thread pools in Java, to manage the occurrence of tasks. This helps prevent hitting resource bottlenecks and allows smoother task transition or cancellation.
- Progress Indicators: Implementing mechanisms to inform users about how long a task might take can reassure them during long waits. While this does not apply directly to timeouts, it adds a layer of user satisfaction.
Using timeouts effectively is tthe key to productive user experiences. It ensures that applications do not freeze and provides a responsive feel while maintaining functional stability.
Working with timeouts in these manners cultivates a more reliable programming environment, thereby ensuring efficient performance across multiple platforms and applications.
Real-World Applications of Set Timeout
Implementing timeouts is vital in many real-world programming scenarios. This section explores how the 'set timeout' feature enhances interaction, user experience, and performance in real applications. Given that frameworks and languages can present unique contexts, understanding these specific applications helps in making better design choices.
Web Development Scenarios
In web development, set timeouts play a critical role in managing asynchronous behavior effectively. For example, when a web application sends a request to a server, using timeouts ensures that the application doesn't wait indefinitely for a response. This strategy is crucial for:
- Improving user experience: By implementing a timeout, developers can manage how applications respond when external services experience latency.
- Error handling: Users can be informed with error messages or alternate plans, if a server does not respond in a given timeframe.
Example: In JavaScript, particularly when working with the fetch API, a simple timeout can be implemented to cancel requests that exceed the desired duration:
This code snippet allows developers to gracefully handle fetch requests that may take too long, signaling a timeout and giving options for how to proceed next.
Game Development Use Cases
In game development, timeouts are critical for enhancing overall performance and responsiveness. Games often rely on certain actions being completed within precise time windows, thus timeouts ensure smooth operation and player satisfaction.
Key objectives include:
- Managing game states: Developers can use timeouts to transition between game states consistently, like moving from a loading screen to active gameplay after a predetermined duration.
- Enhancing responsiveness: Timeouts can optimize how often the game polls input from the user. This helps to prevent degrading performance during high-intensity periods.
For instance, a multiplayer gaming experience might declare a timeout for player actions in order to synchronize all the participants effectively:
Timer integration like this keeps the game in sync, preventing situation where one player appears to act while others are left waiting.
In summary, understanding the real-world applications of timeouts offers great benefits across different sectors. In web and game development, not only do they optimize performance, but they actively engage users and mitigate potential frustration with unresponsive design.
Troubleshooting Timeout Issues
Timeouts play a crucial role in programming, ensuring that applications run smoothly and respond to user actions in a timely manner. When dealing with timeouts, issues may arise that hinder application performance or functionality. Troubleshooting these timeout issues is not just beneficial; it is essential. A minor delay, or an unresponsive application can lead to negative user experiences. Therefore, solving these issues promptly can improve the overall quality of the software.
Effective troubleshooting involves recognizing the problems that commonly appear when timeouts are implemented. Identifying these common complications can save valuable time and resources in software development. Furthermore, understanding debugging techniques related to timeouts facilitates better management of future problems. Consequently, all programmers—beginner and seasoned alike—must become adept at these practices to maintain the integrity of their applications.
Identifying Common Problems
Timeout issues can manifest in various ways, and recognizing the symptoms early is key to resolving them. Some of the frequent problems include:
- Long Blocking Operations: If the timeout value is set lower than the execution time of the operations that may block a process, failure or unexpected behaviors may occurr.
- Misconfigured Timeouts: Incorrectly setting the duration of a timeout can cause unnecessary interruptions or fails to react to real situations.
- Network Latency: In web applications, timeouts may trigger unexpectedly during data transmission due to fluctuations in network speed.
- Error Handling Issues: If an error handling mechanism is not robust enough, it might trigger timeouts improperly leading to further complexity.
Confirming these problems early allows for swift resolutions, avoiding deeper complications that could affect the entire system's functionality. Solutions often involve closely examining the configuration and quality of the code, along with understanding the environmental factors in play.
Debugging Techniques
Once issues are identified, applying effective debugging techniques is necessary for resolution. Some prominent techniques include:
- Verbose Logging: Implementing detailed logging can help Developers understand the execution flow and identify where the timeout occurs.
- Step-through Debugging: By sequentially executing code, one can determine which process was interrupted unexpectedly, leading to further analysis of each component's timing.
- Simulate Load: Replicating high-load situations will help evaluate how the application performs under stress and troubleshoot lag issues, creating tailored fixes as needed.
- Review Configurations: Closely examine timeout settings and other related configurations in the codebase to ensure they align with performance requirements.
Remember: Always reevaluate your configurations. Many complications are the result of improper settings and assumptions about network behavior or resource availability.
By employing these debugging strategies systematically, programmers can better manage timeout issues. This leads not only to quicker resolution but also more resilient application design as future problems can be anticipated and mitigated effectively.
Epilogue
The exploration of timeouts in programming is a critical aspect of developing robust applications. By mastering the implementation of timeouts, programmers can enhance the user experience, streamline resource management, and avoid issues related to long-processing tasks. Importance lies in how effectively these timeouts are applied across tasks and operations, ultimately ensuring smoother code execution. Good timeout management prevents freezing applications and provides opportunities for efficient resource allocation.
Summary of Key Points
- Timeouts play a vital role in usability and performance of applications.
- Understanding how to effectively implement timeouts can lead to better application responsiveness.
- Different programming languages like Java, JavaScript, and C++ have their specific methods and practices for timeouts.
- Incorporating best practices aids in managing operations that would otherwise consume critical resources.
- Proper error handling in conjunction with timeouts minimizes application failures.
Future Trends in Timeout Management
In the agile world of programming, the ways of managing timeouts are likely to evolve. New methodologies may emerge, aimed at optimizing application performance. For instance, innovations in asynchronous programming could lead to more refined timeout mechanisms coupled with enhanced responsiveness and reduced latency.
Advancements in machine learning and AI could also facilitate more intelligent timeout management. Codes may analyze patterns and automatically adjust timeout settings based on past performance analytics, allowing for highly personalized user experiences. It's essential for today’s programmers to stay abreast of these advancements, understanding how integrated timeout management can further improve applications.
In summary, effective timeout implementation is not just a technical necessity. It is increasingly a factor in enhancing user satisfaction and ensuring resource efficiency in a fast-paced programming environment.