Testing Spring Boot Applications: An In-Depth Guide
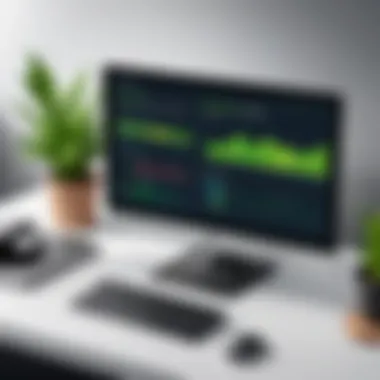
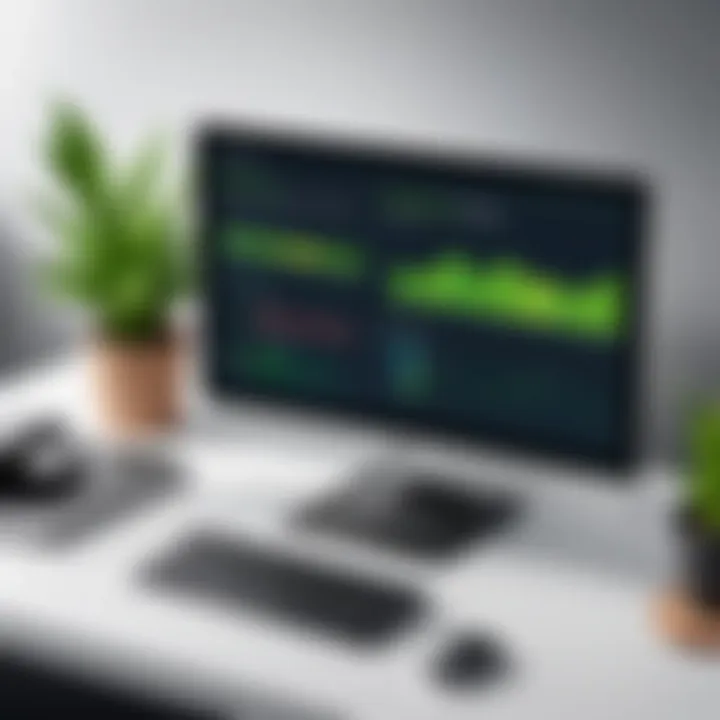
Intro
Testing is an essential facet of software development, particularly when it comes to frameworks like Spring Boot. It allows developers to ensure that their applications not only function as intended but also handle edge cases gracefully. Understanding the nuances of testing can boost the reliability and maintainability of code, offering peace of mind as applications evolve.
The importance of testing becomes clear when you consider the complexities involved in modern web applications. These applications often integrate with databases, third-party services, and various APIs, creating a web of dependencies. The likelihood of bugs increases, making robust testing methodologies indispensable. In this guide, we will journey through testing strategies, best practices, and the tools available in the Spring ecosystem to fortify your Spring Boot applications.
Prelude to Testing in Spring Boot
Understanding how to effectively test applications built on the Spring Boot framework is essential for developers who aim to deliver robust, high-quality software. Testing isn’t merely a phase that you can check off the list; it’s an ongoing practice that ensures every piece of your application functions as intended. In this article, we will dissect various testing methodologies and tools within the Spring ecosystem that can aid in developing applications that are not only efficient but also reliable.
Importance of Testing in Software Development
Testing serves as the backbone of solid software development. It’s the safety net that allows developers to catch errors before they escalate into larger issues. Here’s why it matters:
- Quality Assurance: It ensures the software works as expected without critical bugs. This quality leads to better user satisfaction.
- Cost Efficiency: Detecting issues early can save a project from extensive corrections later. The more comprehensive the tests, the less time spent on fixing post-release bugs.
- Maintainability: Well-tested code is easier to modify, as developers can use tests to assure that changes haven’t unintentinally broken previous functionality.
- Collaboration: With testing as part of the workflow, teams can work together more seamlessly, knowing that changes are protected by a suite of tests.
"A program is only as good as its tests."
This saying rings true, as it emphasizes that good testing practices are fundamental to great software.
Overview of Spring Boot Framework
Spring Boot is designed to simplify the process of developing new applications by offering a production-ready environment. Below are a few highlights of what makes Spring Boot attractive:
- Convention Over Configuration: Spring Boot takes away much of the boilerplate configuration needed in traditional Spring applications. It automatically configures components based on the dependencies present in the project.
- Dependency Management: By using Spring Boot’s dependency management, developers can easily manage libraries, avoiding version conflicts and streamlining updates.
- Embedded Servers: Spring Boot applications can run standalone and come with embedded servers like Tomcat or Jetty, which streamlines deployment.
- Actuator Endpoints: It provides built-in endpoints for monitoring and managing your application, such as health checks and metrics.
By tying together the ease of use and robust testing capabilities, Spring Boot stands out as a powerful tool for developers aiming to build reliable applications quickly. With a clear understanding of the importance of testing and the Spring Boot framework, we move forward to explore the different types of testing approaches applicable in this environment.
Types of Testing in Spring Boot
Understanding the various types of testing in Spring Boot is paramount. Each testing method serves a specific purpose and collectively ensures the application performs as intended. This section will delve into unit testing, integration testing, and end-to-end testing, emphasizing their unique benefits and best practices. With diverse techniques at your disposal, you can achieve a robust system that stands the test of time.
Unit Testing
Definition and Purpose
Unit testing focuses on verifying the smallest parts of the code, such as individual functions or methods. This method aims to catch bugs early in the development cycle, reducing costs associated with later fixes. It allows developers to quickly identify and fix issues, promoting more reliable and maintainable code. Its primary benefit lies in its ability to decouple tests from other system elements, providing isolation that simplifies identifying errors.
Tools for Unit Testing
Several tools exist for effective unit testing in Spring Boot, with JUnit and Mockito being the most popular. JUnit provides a solid framework for running tests, while Mockito enables the creation of mock objects, simplifying the testing of interactions between components. Each tool possesses distinct features that enhance the unit testing experience, making it crucial for developers to familiarize themselves with these options. The challenge can be the learning curve associated with mastering every tool's offerings.
Writing Effective Unit Tests
Writing effective unit tests involves understanding what to test and how to structure the tests for maximum coverage. It’s not just about having tests; it’s about ensuring those tests provide value and pinpoint any issues accurately. Effective tests should be concise, with a clear purpose and outcome. The more rigorous and thoughtful the tests are, the more they contribute to the robustness of the Spring Boot application. A key principle is to ensure tests remain relevant and up-to-date as the application evolves, which can be a common pitfall.
Integration Testing
Understanding Integration Testing
Integration testing involves checking the interactions between integrated components or systems to ensure they behave as expected together. Unlike unit testing, which isolates components, integration testing verifies that collaborating parts function seamlessly. This type of testing is essential for uncovering issues that may not surface during unit tests, particularly around data flow and interface connections. The need for integration testing arises from the complexity of modern applications, where different modules must cooperate for the overall system to function.
Setting Up Integration Tests
To set up integration tests in Spring Boot, developers often use tools like Spring Test and Testcontainers. These frameworks allow for the staging of realistic testing environments that mimic production settings. With lenses to various parts of the system, it’s much easier to spot any discrepancies that would affect overall performance. Getting the integration testing setup done right can be tricky, but it's essential for the long-term health of the application being built. Well-executed integration tests can save considerable time and resources.
Best Practices
Best practices for integration testing include:
- Keep tests as independent as possible to avoid cascading failures.
- Use fixture data that represents real-world scenarios for better insights.
- Continuously run integration tests as part of the development process to catch issues early.
By adhering to these practices, you can ensure the reliability of your integrated components and the overall application. Ignoring these can lead to major headaches further down the development line.
End-to-End Testing
Concept and Techniques
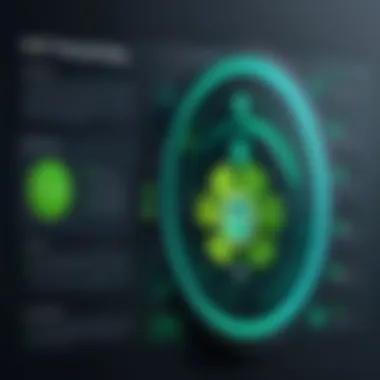
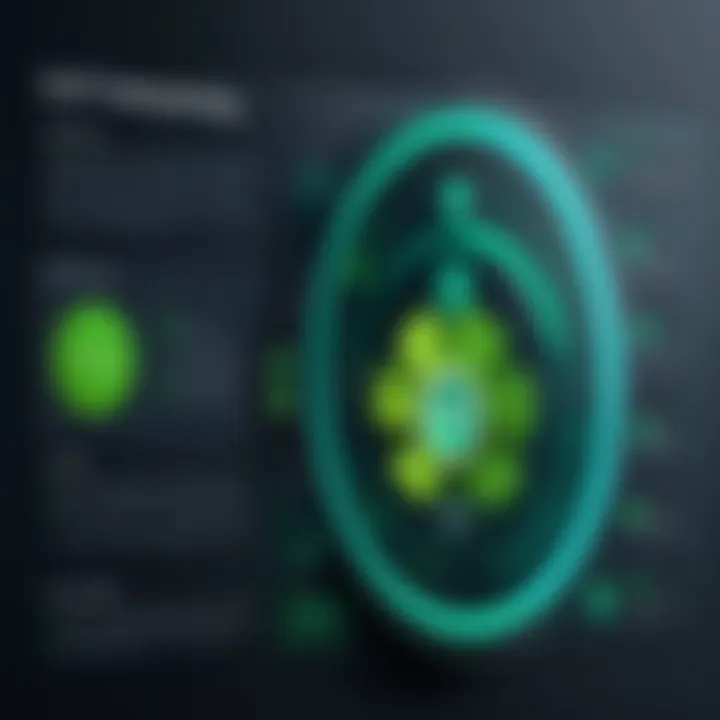
End-to-end testing evaluates the entire application workflow, simulating real user scenarios to ensure that all components work together as expected. This testing type is fundamental for validating that the integrated components function as anticipated in the real world. The key characteristic of end-to-end testing is its holistic approach, covering everything from user interfaces to database interactions. Its wider scope can be a double-edged sword; while it provides comprehensive assurance, it can also be time-consuming to set up and run.
Tools for End-to-End Testing
Tools like Selenium and Cypress are commonly used for end-to-end tests, enabling automated browser tests that mimic user interactions. These tools allow teams to validate the application's functionality from the user’s perspective, catching problems that unit or integration tests might miss. However, setting up these tools can be complicated, especially for beginners.
Case Studies
Examining successful case studies of end-to-end testing reveals significant gains in quality and performance for many applications. For example, a particularly vibrant case involved a large e-commerce platform that experienced high conversion rates after implementing rigorous end-to-end tests. With detailed testing strategies, they managed to uncover usability issues that directly impacted user experience. Demonstrating how end-to-end testing contributed to enhanced customer satisfaction can inspire teams to adopt these methodologies and invest in quality assurance.
“In the world of software, quality isn’t a luxury; it’s a necessity.”
By leveraging various testing methods effectively, developers can ensure their applications not only meet but exceed expectations.
Test-Driven Development in Spring Boot
Test-Driven Development (TDD) has emerged as a cornerstone practice for developers seeking to create reliable software within the Spring Boot framework. TDD is a methodology where tests are written before the actual code is implemented, enhancing the development process significantly. This approach fosters a mindset that prioritizes quality and functionality from the get-go, ultimately leading to cleaner, more maintainable code. The whole idea hinges on an iterative loop of writing a test, seeing it fail, writing the minimal code necessary to pass the test, and then refactoring to improve the structure of the code without changing its behavior.
Principles of TDD
The core principles of TDD revolve around the mantra of test first. This philosophy drives developers to think critically about their requirements and expected outcomes before proceeding with implementation. Understanding the expected behavior of a piece of code leads to better design decisions and helps uncover potential issues early in the development cycle.
Furthermore, TDD promotes simpler code structures, leading to better readability and maintainability. By adhering to this principle, developers often find themselves creating more modular components that can be easily tested in isolation, resulting in fewer bugs and increased confidence when making changes or enhancements.
Implementing TDD in Spring Boot
Implementing TDD within the Spring Boot ecosystem can be straightforward, but it requires discipline and a good grasp of both the framework and testing tools available.
Writing Tests Before Code
Writing tests before the actual code is not merely a tick on a to-do list; it’s a proactive step that shapes the development process. This method requires developers to articulate their expectations and goals clearly. When you write tests first, it is like drafting a blueprint before the construction begins. It clarifies what exactly is needed from the code, subsequently driving the development of features that serve real needs.
The beauty of this approach lies in its power to identify discrepancies early on. When new tests fail, they provide immediate feedback, highlighting any misunderstandings about the requirements. One notable benefit of shifting left, which encapsulates this practice, is the reduction of bugs in production, as developers are guided back to the requirements regularly.
However, writing tests first may also present challenges. It may feel like a slow start, especially for newcomers who are eager to dive into coding. The discipline required to adhere to this practice can be difficult to maintain. Nevertheless, the long-term benefits often outweigh these initial discomforts.
Refactoring and Iteration
Refactoring is where TDD truly shines. After the tests pass, developers must assess how to make the code clearer, more efficient, or more maintainable without altering its functionality. This step is crucial because it allows developers to optimize their code incrementally, ensuring that changes can be made without fear of introducing new bugs.
The iterative nature of TDD means that developers can continuously evolve their codebase. When they implement a new feature or fix a bug, they repeat the process — write a test, see it fail, write just enough code to pass, and refactor again. Such a cycle helps to solidify understanding and embed good practices deeply within the development culture.
In summary, TDD is not just about writing tests; it’s a holistic approach that refines both thought processes and code. It encourages clarity, reduces bugs, and facilitates adaptability, all while nurturing a mindset of quality assurance that is invaluable in any software development endeavor.
Testing Frameworks for Spring Boot
Testing frameworks are indispensable in the realm of software development, especially when dealing with robust frameworks like Spring Boot. These frameworks provide a structured approach to writing tests, fostering a culture where reliability and maintainability thrive. Utilizing a testing framework ensures that your application not only meets its specifications but also behaves as intended under various conditions. This section delves into two primary testing frameworks commonly used in Spring Boot: JUnit and Mockito, highlighting their unique features and how they complement each other.
JUnit Overview
JUnit stands at the forefront of testing frameworks, particularly in the Java ecosystem. Its simplicity and ease of use resonate well with developers. One of the compelling aspects of JUnit is its capability to facilitate both unit and integration tests. By empowering developers to write repeatable tests, it effectively reduces the likelihood of bugs slipping through the cracks, which is crucial when striving for software excellence.
Using JUnit for Tests
Using JUnit for tests offers a structured methodology to test Java code. One key characteristic is its straightforward assertion mechanism which allows developers to validate expected outcomes easily. A prevalent practice is to write a test case for each method, which ensures that as methods evolve, the tests can adapt, catching any unintended changes early on.
A unique feature of JUnit is its support for various assertions, including , , and , which provide a wealth of options for verifying your code's behavior. The advantage here is that it encourages developers to think critically about their code's expected behavior. However, having too many asserts in a single test can muddy the waters and make them harder to read, which is definitely a downside to watch out for.
Annotations and Assertions
Annotations play a pivotal role in JUnit, streamlining the testing process by designating test classes and methods. The annotation, for example, marks a method as a test case, while and annotations help manage setup and teardown processes before and after each test. This aids in keeping tests clean and focused.
The unique feature here is how annotations optimize test organization and execution. By categorically defining which methods are tests and their execution order, it maintains coherence in your test suite, enhancing readability. However, an overreliance on annotations could lead to complex test setups that might confuse newcomers.
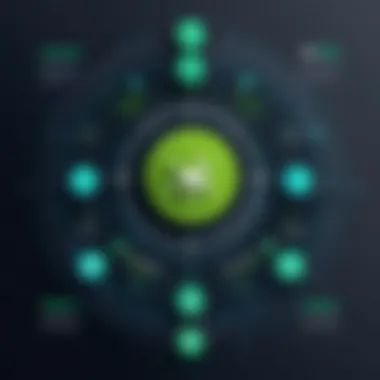
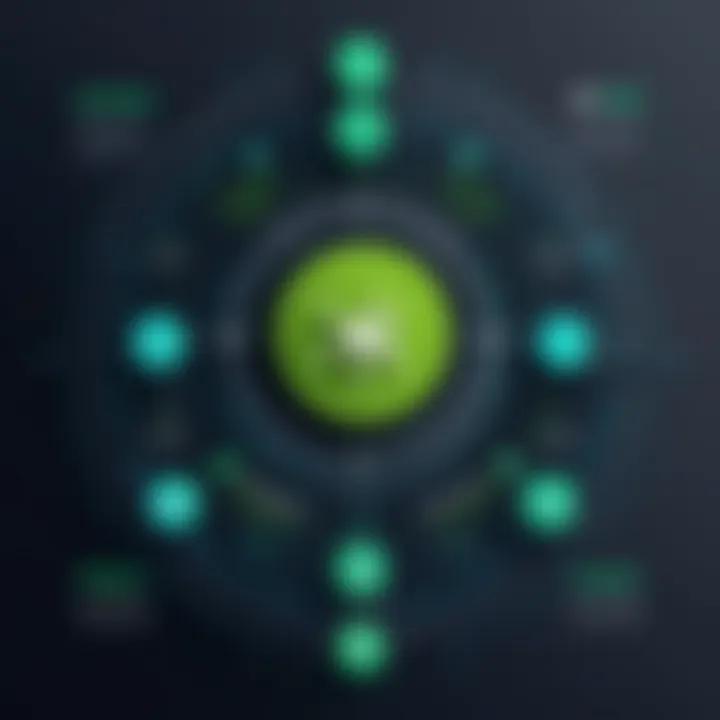
Mockito for Mocking
Mockito has carved its niche in the domain of mocking frameworks. Mocking is a vital concept in testing, aiding developers in isolating the behavior of a class by simulating its dependencies. Understanding mock objects is crucial for crafting effective tests, especially when dealing with complex systems.
Understanding Mock Objects
Mock objects allow developers to define expected behavior without relying on actual implementations of dependencies, which can be unmanageable in certain tests. This characteristic allows for precise unit testing, where one can evaluate a class in isolation. It's a beneficial practice, particularly in Spring Boot applications where layers of abstraction may complicate direct testing.
A significant advantage of using mock objects lies in their flexibility; developers can simulate various scenarios without needing extensive setup. That being said, overzealous mocking can lead to a disconnect between tests and real-life usage, potentially resulting in misleading results.
Creating Mocks in Tests
When creating mocks in tests, Mockito shines through its intuitive API. The method provides a clear, concise way to create mock objects and specify their behavior. This capability enhances productivity, allowing developers to focus on writing meaningful tests rather than getting bogged down in boilerplate code.
The unique feature of creating mocks is the ease with which developers can configure responses. A common approach is to use the syntax to set up expected interactions. Still, while this enables a streamlined testing process, it requires careful consideration to ensure that mocks do not diverge from actual implementations — that could potentially lead to false sense of security in your testing.
"Effective testing frameworks not only identify bugs but also promote better design through the practice of coding defensively."
In summary, embracing robust testing frameworks like JUnit and Mockito can significantly uplift the quality of your Spring Boot applications. By understanding their features and considerations, developers are better equipped to write reliable, maintainable tests that drive their software towards success.
Configuring the Testing Environment
When it comes to testing Spring Boot applications, properly configuring the testing environment is crucial. The environment encompasses a wide range of elements that contribute to how your tests will perform and how effectively they will mimic real-world scenarios. A well-structured testing environment allows developers to identify issues earlier in the development cycle, ensuring that the application runs smoothly when it reaches production.
Being meticulous about configuring the test environment can save a lot of headaches down the road. From managing external dependencies to ensuring consistent application behavior across various test scenarios, each choice can significantly impact test reliability and performance. It’s not just a box to tick off; it’s a critical step that informs every other aspect of your testing strategy.
Setting Up Application Properties
In Spring Boot, application properties play a pivotal role in determining how your application behaves in different contexts—be it development, testing, or production. Setting up application properties specifically for testing allows developers to isolate tests from the live environment, protecting vital data and configurations.
Using the file, you can configure various settings that apply solely when running tests. For example:
Establishing your testing properties like this ensures that the tests run against a lightweight, in-memory database ( in this case), which is fast and eliminates potential conflicts with other environments. Furthermore, using different properties for different environments aligns your testing strategy with the principles of isolated environments, making sure that your tests remain consistent across various setups.
Using Test Profiles
Spring Boot's profile feature offers an elegant solution to manage different application configurations. When it comes to testing, creating a dedicated test profile can significantly streamline how your tests are conducted. Profiles enable you to define a set of configurations that only apply during the execution of tests, allowing for a clean separation from production settings.
For instance, you could define a profile within the file like this:
This way, whenever you run tests, Spring will consider configurations under the profile, ensuring that no live data gets altered, and providing a tailored environment that encapsulates all necessary testing settings.
Moreover, utilizing test profiles keeps your tests restrained and focused. This is essential, as it allows developers to easily toggle profiles without restructuring code, promoting a smoother workflow when writing and executing tests. In brief, correctly setting up profiles can help mimic diverse operating conditions your application may encounter in the field, thus providing a solid base for reliable testing.
Executing Tests and Analyzing Results
Executing tests and analyzing results form the crux of validating the behavior of Spring Boot applications. It’s not merely about writing code and pushing it to production. This stage provides crucial feedback on whether your application performs as expected in various scenarios, helping to catch errors early and ensuring reliability in production. By methodically running and analyzing tests, developers gain insights into code quality, potential bottlenecks, and areas requiring improvement. Ultimately, this thorough scrutiny can save time and resources while enhancing overall project quality.
Running Tests in IDE
Integrating the testing process within your Integrated Development Environment (IDE) streamlines the workflow for developers. Most modern IDEs, like IntelliJ IDEA or Eclipse, come equipped with built-in test runners that allow you to execute tests with just a few clicks. This facility not only saves time but also enhances user experience by providing immediate feedback.
Here are some key points regarding running tests in an IDE:
- Easy Setup: It usually involves minimal configuration. Just ensure your test classes are placed correctly, and your IDE will take care of the rest.
- Immediate Feedback: As developers run tests, the IDE displays the results instantly. This real-time feedback loop is very beneficial as it encourages frequent testing.
- Debugging Support: Running tests directly within the IDE also allows for debugging. You can set breakpoints and examine variable states to understand where issues lie.
"Integrating testing into your development lifecycle is like having a safety net while walking a tightrope. It instills confidence in your code."
Continuous Integration and Testing
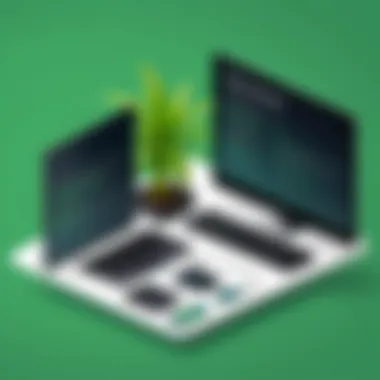
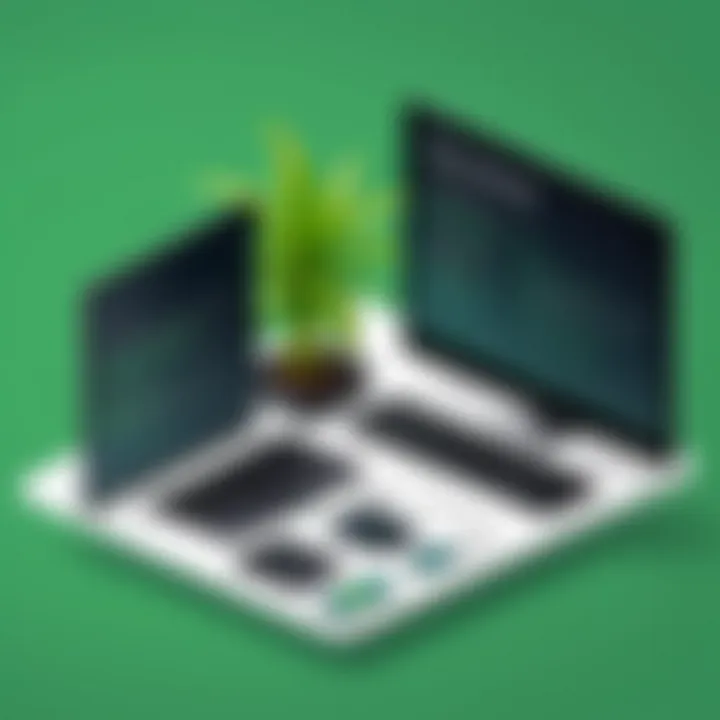
Implementing continuous integration (CI) in Spring Boot projects creates a robust framework for regularly executing tests, thereby accelerating the development process. CI emphasizes frequent code changes, which are automatically tested to catch bugs as they are introduced. This practice brings several advantages.
Integrating with Tools
Tools such as Jenkins, GitLab CI, and Travis CI serve as critical enablers in the CI process. These tools automate the testing and deployment pipeline, offering a seamless transition from code changes to executed tests. The significant characteristic of CI tools is their ability to enforce consistency across environments, ensuring that tests behave the same way in local development as they do in production.
- Key Benefits:
- Increased code quality through continuous testing.
- Early detection of failures reducing the cost of fixing issues later.
- Streamlining deployment processes.
However, a potential drawback lies in the initial setup and maintenance of these CI systems. The learning curve can be steep if teams are unfamiliar with CI/CD practices, requiring investment in time and effort.
Automating Test Runs
Automation of test runs is another cornerstone of modern software development. This entails having tests run automatically every time code is pushed to the version control system. This feature enhances code reliability and maintains consistency in testing.
- Benefits of Automation:
- Saves time and effort by eliminating the need for manual test execution.
- Increases coverage, leading to more thorough testing.
- Allows for immediate feedback, helping to identify issues quickly.
On the flip side, automated tests may lead to false positives or negatives if not written carefully, emphasizing the need for detailed attention during the test-writing phase. Also, maintaining automated tests over time requires diligence, as software changes can lead to tests becoming outdated or irrelevant.
In the grand scheme of testing Spring Boot applications, executing tests effectively and analyzing results enables developers to innovate confidently, knowing that they have proactively identified and addressed potential issues.
Common Challenges in Testing Spring Boot Applications
Testing Spring Boot applications isn’t just about writing tests and running them. It's like navigating a winding road—there are bumps, turns, and unexpected delays. If you want your tests to be effective, you need to understand the common challenges you'll encounter and figure out how to handle them. Here, we explore the two hiccups that can really trip you up: dealing with dependencies and performance issues in your tests. By tackling these challenges head-on, you can pave the way for smoother testing and a more robust application.
Dealing with Dependencies
Dependencies in Spring Boot applications can sometimes feel like a double-edged sword. On one hand, they enhance functionality and facilitate development, but on the other, they complicate testing. When your application relies on multiple components or services that interact with each other, it becomes crucial to isolate each test to ensure that they do not interfere with one another.
To manage such complexities, here are some strategies:
- Use Mocking Frameworks: Tools like Mockito can be lifesavers. They allow you to create mock objects that simulate the behavior of real dependencies. This way, when you're testing a service, you don't have to worry about the underlying database calls or external APIs, which can slow down your tests and lead to inconsistent results.
- Spring’s @MockBean Annotation: This annotation helps replace a bean in the application context with a mock version during tests. It’s handy when you want to test the server behavior without dealing with real components.
- Focus on Unit Tests: Aim to write unit tests that target individual components. This reduces inter-component dependencies and makes it easier to write faster-running tests.
In summary, while dependencies can add complexity, with the right tools and techniques, you can manage them effectively. Remember, the goal is to make your tests independent so that they give you reliable results without depending on the state of other parts of your application.
Performance Issues in Tests
Performance is another significant hurdle in testing Spring Boot applications. The speed of your test suite can range from lightning-fast to glacial, depending on how your tests are set up. It’s important to be mindful of this, not just for your sanity but also for efficient development cycles.
Here are several considerations to keep performance in check:
- Choose the Right Test Type: Always think about what type of test you are running. Unit tests should be swift and quick to provide feedback, while integration tests may naturally take longer due to their complexity. Knowing when to run each type becomes pivotal in maintaining a brisk development pace.
- Optimize Database Interactions: Database operations can drastically slow down tests. Consider using an in-memory database like for tests that require database interactions. This minimizes I/O overhead and enhances overall test performance.
- Avoid Overlapping Tests: If your tests share state or perform overlapping actions, they can slow each other down. Make sure every test resets its state and performs its actions independently.
A well-configured test suite can go a long way in reducing bottlenecks and keeping developers happy. Ultimately, performance issues shouldn't deter you but rather encourage you to strategize and refine your approach.
"In testing, as in life, it’s about learning from challenges and continually improving your processes."
Closure
In concluding our exploration on testing Spring Boot applications, it’s essential to highlight that the insights offered throughout this article reinforce the foundational role of testing in software development. It’s not merely a safety net but a crucial driver for enhancing application quality and reliability. By systematically implementing various testing strategies, developers can catch bugs early in the development cycle, ultimately saving time and resources.
Recap of Key Points
To summarize, we have delved into several pivotal aspects, including:
- Importance of Testing: Recognizing how testing solidifies software quality.
- Types of Testing: We discussed unit, integration, and end-to-end testing, laying out how each serves a distinct purpose.
- Test-Driven Development: Emphasizing the practice of writing tests before code, which fosters a tighter development cycle.
- Frameworks for Testing: An overview of JUnit and Mockito germinated a familiar ground on testing tools.
- Configuration: Setting up testing environments including application properties and utilizing test profiles.
- Common Challenges: Discussed hurdles like dependencies and performance issues that testers might face.
These elements form a robust framework for understanding testing in the Spring Boot environment, offering a roadmap for developers aiming to improve their technical competency.
Future of Testing in Spring Boot
Looking ahead, the landscape of testing in Spring Boot is poised for exciting developments. As software complexity grows, advancements in artificial intelligence and machine learning are likely to reshape testing methodologies. The integration of automated solutions can handle repetitive tasks, thus allowing testers to focus on more complex scenarios and edge cases.
Additionally, the rise of cloud computing is facilitating more collaborative environments, making it easier for teams to execute tests in real-time. The adoption of containerization technologies, such as Docker, also makes it simpler to replicate testing environments swiftly and efficiently, ensuring that tests reflect current application states accurately.
The dynamic world of programming continuously evolves, and with it, the tools and strategies for testing. It’s imperative for developers to stay abreast of these changes and adapt their practices accordingly.
By integrating advanced frameworks and embracing new technologies, the future of testing in Spring Boot can lead to even more resilient and high-quality applications. Thus, committing to ongoing learning and adaptation is essential for developers in this vibrant ecosystem.