Mastering Swift Coding Questions: A Complete Guide
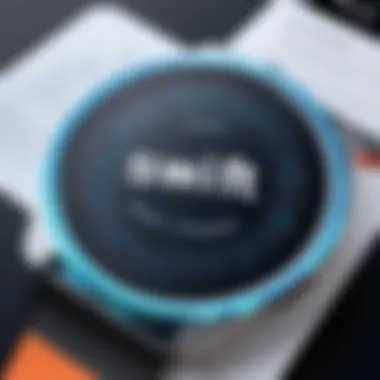
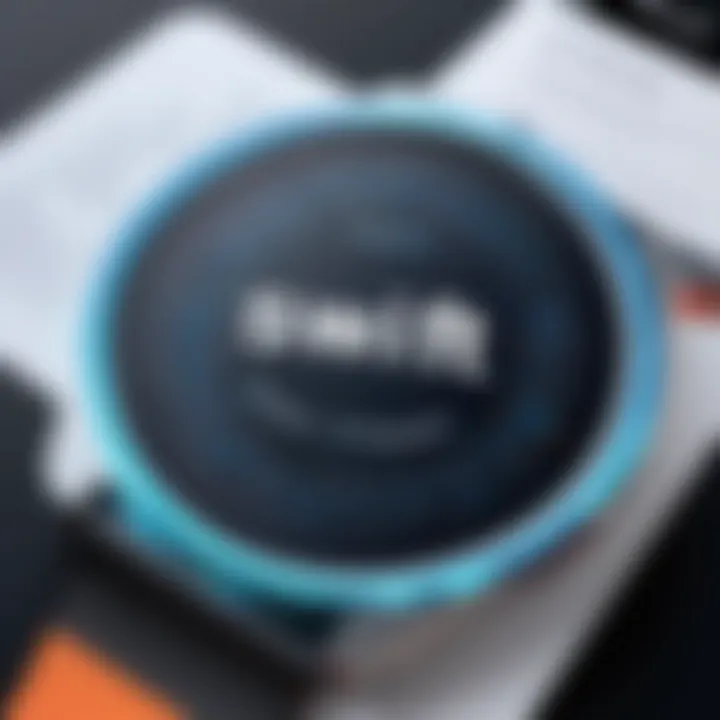
Preamble to Programming Language
When discussing modern software development, Swift is frequently mentioned as a robust and elegant choice. This programming language, developed by Apple, is like a fresh breeze in a world crowded with cumbersome code languages. Understanding Swift requires a grasp of its history, its design features, and the widespread applications across various domains.
History and Background
Swift was unveiled to the public in 2014, a product of Apple's long-term desire to provide a better programming language for its platforms. Rooted in the pleasant familiarity with Objective-C, Swift was designed to overcome some of the limitations found in C-based languages. By blending the simplicity of scripting languages with the power of low-level programming, Apple aimed to create a piece of software that would streamline app development for macOS, iOS, watchOS, and tvOS.
Features and Uses
Swift boasts a multitude of features that make it appealing to both novices and experienced developers alike. Its syntax is clear, expressive, and less prone to mistakes when compared to other languages. Notable features include:
- Type Safety: Swift's strong typing system ensures that errors are identified at compile time, which saves developers from headaches down the line.
- Performance: Swift is designed for performance, offering speeds comparable to those of C and Objective-C.
- Interactivity: Swift Playgrounds allow beginners to experiment with code in a playful, interactive way, a real boon for learning.
These features make Swift particularly well-suited for mobile app development, especially on Apple's renowned platforms.
Popularity and Scope
In recent years, Swift's popularity has surged, becoming a favorite among startups and big tech companies. According to a survey, a significant percentage of developers are now working with Swift for mobile applications. From e-commerce platforms to social media applications, Swift is increasingly being recognized as the go-to language for creating high-performance, user-friendly apps.
Furthermore, the language is expanding beyond mobile development. The introduction of server-side Swift has opened doors for web applications, allowing developers to utilize Swift in full-stack development. In short, Swift encompasses a vast territory of programming possibilities.
"Swift is not just a language, but a tool that makes coding more enjoyable and efficient."
As we leap into the next sections, we’ll explore the fundamental syntax and concepts of Swift, transitioning from the warmth of its historical roots to practical usage in the coding arena.
Understanding Swift Programming Language
Swift is not just another programming language; it's a significant advancement in how we think about coding for Apple’s platforms. Understanding Swift is essential for anyone eager to immerse themselves in the world of iOS and macOS development. This modern programming language was designed to be powerful yet easy to use, making it accessible to newbies while still satisfying the needs of seasoned developers. By grasping Swift, you not only learn a skill that is in high demand but also open the door to a myriad of applications ranging from mobile apps to complex back-end systems.
History of Swift
Swift made its debut in 2014, introduced by Apple as a replacement for Objective-C, which had been the go-to language for Apple platforms since the early days. This transition wasn’t just about refreshing the syntax; Apple aimed for a language that encouraged safer and more expressive code. During its early iterations, Swift went through numerous updates, each refining its capabilities. The community quickly embraced the language for its performance and safety features, which led to enhanced productivity in development. In an era where fast-paced technological advancements are the norm, Swift allowed developers to keep pace, ultimately transforming how apps are created for Apple devices.
Key Features of Swift
Swift is packed with features that set it apart. These benefits are not just for show; they are designed to make the programming experience smoother and more efficient:
- Safety: Swift eliminates entire classes of unsafe code. Optional types ensure that variables are initialized with a value or explicitly marked as missing.
- Performance: Swift is optimized for speed. It compiles to native code which can run incredibly fast compared to interpreted languages.
- Interoperability: Swift is designed to work alongside existing Objective-C code, making the transition smoother and allowing developers to leverage old projects while embracing new ones.
- Expressiveness: The syntax of Swift is clear and concise, allowing developers to express their ideas in a way that's easy to read and maintain.
By incorporating these features, Swift not only makes coding more enjoyable but also pioneers a new standard in app development. Developers are able to focus on what they are building rather than getting bogged down by the intricacies of the language itself.
Swift vs. Objective-C
When comparing Swift and Objective-C, it isn’t just a matter of preference; it’s about functionality and the future of development. Objective-C had been the stalwart for many years, but it’s worth recognizing its shortcomings:
- Syntax: Objective-C’s syntax can feel cumbersome and less-readable than Swift’s clean, modern look.
- Memory Management: Objective-C relies heavily on manual reference counting. In contrast, Swift uses automatic reference counting, significantly simplifying memory management.
- Ease of Learning: For beginners, Swift is easier to grasp. Objective-C's steep learning curve can be daunting for those just starting out.
In short, while both are powerful tools in their own right, Swift has emerged as the more desirable option for new projects, positioning itself as the future of Apple development.
"Swift revolutionizes the way developers can build applications, combining simplicity and power in a single language."
Types of Coding Questions in Swift
Understanding the types of coding questions is fundamental when it comes to mastering Swift programming. Each type serves as a building block, addressing different core concepts that students and budding developers will encounter frequently. Diving into various categories such as algorithm challenges, data structure inquiries, and basic syntax questions not only prepares you for real-world application of Swift but also sharpens your problem-solving skills.
Algorithm Challenges
Algorithm challenges are often at the heart of coding interviews and assessments. They test your ability to devise efficient solutions to problems that may seem complex at first glance. These questions promote critical thinking and help to enhance your understanding of Swift's capabilities in problem-solving contexts.
When approaching algorithm challenges, consider these aspects:
- Understand the problem clearly: Before jumping into coding, take your time to dissect the problem statement. What are the inputs? What do you need to achieve?
- Identify patterns: Many algorithmic problems share underlying patterns. Being able to recognize these can significantly speed up your solution development.
- Optimize: Always think about the efficiency of your solution. Is the algorithm running in linear time or quadratic time? Swift's language features can help minimize time complexity.
Common examples of algorithm challenges in Swift include:
- Sorting algorithms (like quicksort and mergesort)
- Searching algorithms (like binary search)
- Recursion problems (factorial calculations, Fibonacci numbers)
"Algorithm challenges not only test your coding skills but also your logical reasoning."
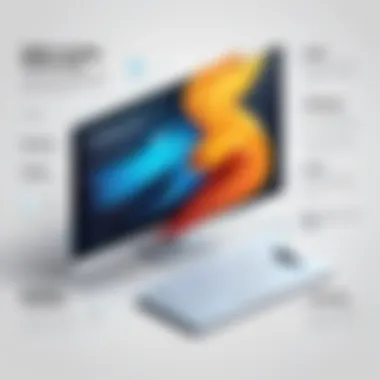
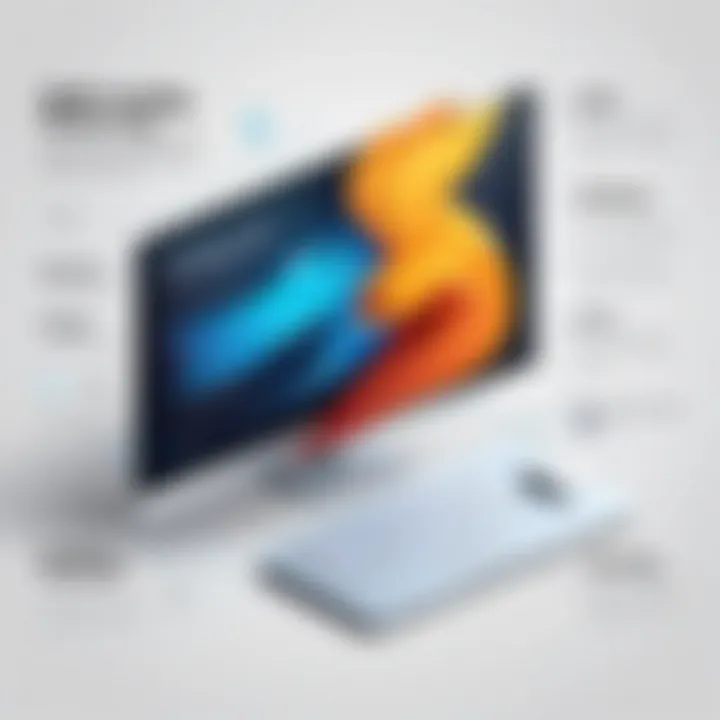
Data Structure Questions
Data structures are a crucial part of programming, as they dictate how data is organized, accessed, and manipulated. Questions related to data structures in Swift often focus on practical knowledge of collections such as arrays, dictionaries, sets, and custom structs or classes.
Here are some fundamental points to consider:
- Know your data types: Each data structure has its strengths and weaknesses. For example, dictionaries provide fast lookups, while arrays can store multiple items in a sequential manner.
- Operations: Be familiar with basic operations you can perform on these structures like insertion, deletion, and iteration. Understanding these operations deeply can often help in problem-solving tasks.
- Use cases: Familiarize yourself with when to use one structure over another. For instance, if order matters, an array might be the best bet; if unique values are required, consider a set.
Data structure questions that you might encounter include:
- Implementation of common data structures (like stacks or queues)
- Manipulating arrays or dictionaries
- Understanding linked lists or trees in Swift
Basic Syntax Questions
The foundation of any programming language is its syntax. Basic syntax questions serve as a primer for understanding the language's structure. These questions often lead to greater proficiency in coding by emphasizing Swift's unique features and capabilities.
These questions can cover:
- Fundamental language constructs: Understanding how to properly declare variables, define functions, and control flow statements such as , , and .
- Error handling techniques: Swift has robust error handling options. Knowing how to implement blocks is essential.
- Best practices: Writing clean and idiomatic Swift code is a skill honed over time. Learn the conventions, such as naming conventions and code organization, to make your code readable.
While often overlooked, mastery of basic syntax empowers learners to tackle more complex problems with confidence. Ensuring fluency in syntax can lead to faster debugging and implementation of more complex Swift concepts.
Common Swift Coding Problems
In the realm of Swift programming, understanding common coding problems is pivotal for both budding developers and seasoned professionals. Tackling these frequent challenges can not only reinforce foundational skills but also build confidence when approaching more complex tasks. Knowing how to navigate these problems enhances one’s ability to write effective code, pinpoint errors, and ultimately produce reliable applications. Furthermore, addressing these issues provides a significant opportunity to engage with the Swift community, fostering discussions and solutions that benefit all.
String Manipulation
String manipulation is a cornerstone of programming, especially in Swift where it is often employed for tasks ranging from user input to data processing. A thorough grasp of how to work with strings can empower developers to craft robust applications that handle textual data efficiently. Common tasks include:
- Concatenation: Joining two or more strings together to form a single string. For instance:
- Substrings: Extracting portions of a string. Using Swift’s methods allows for easy manipulation of text. This can be particularly useful in scenarios like parsing user information.
- String Interpolation: Swift offers a clean syntax to incorporate variables directly into strings, enhancing readability and reducing potential errors. For example:
By mastering string manipulation, learners can not only perform essential operations but also optimize their programs for better performance. Handling strings efficiently is key, especially considering the high frequency of such tasks in everyday coding scenarios.
Array Operations
Arrays in Swift serve as fundamental structures for storing ordered collections of elements. Whether it’s a list of scores, user inputs, or a collection of images, knowing how to manipulate arrays is crucial for any programmer. Key operations include:
- Appending Elements: Adding new items to an existing array can be done effortlessly, thus allowing for dynamic data storage. For example:
- Iterating Over Arrays: Utilizing loops to perform operations on each element in an array is common. This aids in processing data on an individual basis.
- Filtering and Mapping: Swift provides powerful methods to manipulate arrays, such as and . For instance:
Mastering these array operations ensures that programmers can handle collections of data smoothly, leading to more efficient and clean code.
Control Flow Issues
Control flow in Swift directs the execution of code based on conditions and loops, making it essential for building interactive applications. Understanding how to manage these flows allows developers to handle varying situations adeptly. Some common aspects include:
- Conditional Statements: The use of , , and forms the backbone of decision-making in code. For example:
- Loops: Swift supports both and loops, which allow repetitive task execution. This is vital for scenarios such as processing multiple entries in data sets.
- Early Exit: Utilizing and can streamline loop execution by allowing programmers to skip iterations or exit a loop altogether when certain conditions are met.
Control flow not only structures the logic of an application but also enhances its functionality and performance. Effectively managing these elements ensures the resulting application is both responsive and user-friendly.
Mastering common coding problems like string manipulation, array operations, and control flow not only builds a solid foundation in Swift but also prepares learners to tackle more complex scenarios with confidence.
Mastering Swift Concepts
Mastering the concepts of Swift programming is like having a sturdy compass while navigating through a dense forest. It equips learners with the necessary tools to tackle complex coding challenges, enabling them to write efficient, maintainable, and robust code. Understanding certain key areas—such as optionals, closures, and protocols—forms the backbone of effective Swift programming. Each of these components not only enhances code clarity and functionality but also empowers programmers to adopt more clever and innovative problem-solving techniques.
Understanding Optionals
Optionals are a fundamental aspect of Swift that allows developers to express the possibility of absence. The significance of optionals lies in their ability to prevent runtime crashes, a frequent issue when dealing with variables that might not hold values. They introduce a safer programming environment by mandating the developer to handle the absence of values explicitly. Let's delve into the two types of optionals:
Implicitly Unwrapped Optionals
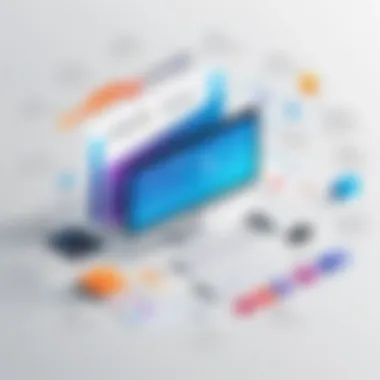
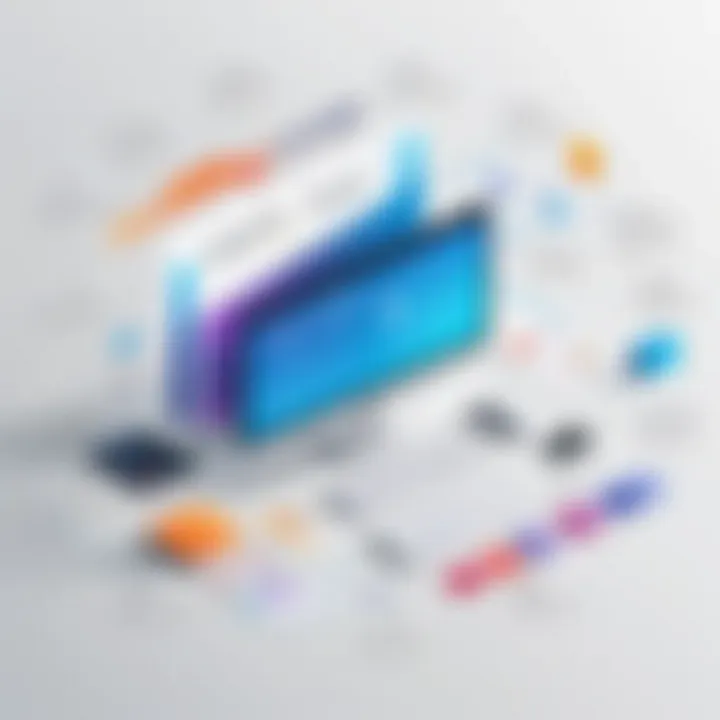
Implicitly unwrapped optionals, denoted with an exclamation mark (), offer a way to work with optionals that are expected to have a value after being set initially. They are a convenient choice when one is certain that the optional will not be nil after being assigned. This contributes positively by eliminating repetitive checks for nil, easing the burden of conditional statements in regards to optional binding.
A key characteristic of implicitly unwrapped optionals is their suitability for properties in classes where it is known that they will be instantiated at some later point in time. For example, using it in view controllers where UI elements are guaranteed to be present helps in avoiding unnecessary checks. However, caution is needed, as accessing an implicitly unwrapped optional when it is nil can lead to a crash. This duality of convenience and risk makes it a valuable, if sometimes perilous, tool in your programming arsenal.
Optional Chaining
Optional chaining allows for smooth navigation through multiple optional values, making it easier to call properties and methods on optional which might currently be nil. This capability to safely access nested optionals is beneficial, as it condenses what could otherwise be verbose code into a more streamlined format.
The primary advantage of optional chaining is that it removes the need for lengthy nested checks. Instead of writing multiple if-statements to safely access properties, developers can streamline their access with a simple question mark (). If at any point the optional is nil, the entire chain returns nil, avoiding potential crashes. This feature highlights Swift's emphasis on safety and clarity, although it necessitates a solid understanding of when and how to use optionals within a coding context.
Closures in Swift
Closures are self-contained blocks of functionality that can be passed around and used in your code. They are similar to functions but have an important distinction: closures capture and store references to any constants and variables from the surrounding context. This characteristic allows for powerful functional programming techniques in Swift.
Trailing Closures
Trailing closures are a nifty syntax feature in Swift, enabling you to write cleaner, more readable code. When the last parameter of a function is a closure, Swift permits placing it outside the parentheses. This is especially useful when dealing with functions that take closure as a parameter since it enhances readability.
A key aspect of trailing closures is that they can make the usage of functions more intuitive, particularly when working with UI development, such as animations or event handling. It helps keep code organized, yet it comes with a trade-off. The untrained eye may not recognize where a function call ends and the closure begins, potentially leading to misunderstandings in larger blocks of code.
Closure Expressions
Closure expressions provide a lighter syntax to create closures. They can be utilized when you need to write quick, succinct inline closures without creating a named function. This feature contributes to maintaining brevity and focus in the code.
The strength of closure expressions lies in their clarity and efficiency. For example, when working with collection methods like or , writing inline closures can significantly reduce the volume of code. That said, for complex operations, opting for named functions may aid readability. Knowing when to use closure expressions versus named functions is essential for maintaining clarity while coding.
Protocols and Extensions
Protocols in Swift define a blueprint of methods, properties, and other requirements that suit a particular task or functionality. They are a cornerstone of Swift’s emphasis on protocol-oriented programming, allowing for incredibly modular and reusable code. Extensions complement protocols by enabling you to add functionality to an existing class, structure, or enumeration.
Using protocols and extensions makes code adherent to the DRY (Don't Repeat Yourself) principle, enhancing file organization by grouping related functionalities. Swift emphasizes the integration of protocols with types, allowing for versatile and adaptable coding solutions. Moreover, as you dive deeper into Swift, understanding how to effectively leverage protocols and extensions will offer immense value in structuring your applications efficiently, leading to scalable and testable code.
Tips for Answering Swift Coding Questions
When it comes to tackling Swift coding questions, having a solid strategy is essential. This section focuses on practical tips that can bolster your problem-solving abilities and improve your confidence. Programming isn’t just about knowing syntax or libraries; it’s also about thinking critically and approaching problems methodically. Here, we'll dive into the art of reading, breaking down, and testing to offer a comprehensive framework for a successful coding interview or development task.
Reading the Problem Statement Carefully
Before jumping into the code, you need to grasp a complete understanding of what the question is asking. It might seem trivial, but overlooking details can lead you down a rabbit hole of errors. Here’s how to improve your reading:
- Identify the main request: Determine if the question is asking for a function, a class, or something else entirely.
- Recognize important constraints: Pay attention to any limits mentioned. For example, does the input size affect how you approach the solution?
- Locate examples: If provided, examples can illuminate the intended use of your code.
An essential point is to avoid jumping the gun. Take your time to analyze what’s in front of you. Understanding the nuances can often reveal solutions that are not apparent at first glance.
Breaking the Problem Down
Once you have a firm handle on the problem, it’s time to dissect it into manageable parts. This technique helps prevent feeling overwhelmed. Here’s how to go about it:
- Divide into smaller tasks: Break the problem into logical sections. For instance, if asked to manipulate an array, consider operations like filtering, sorting, or aggregating values separately.
- Outline your approach: Jot down the steps needed to resolve each component. It could be as simple as listing functions or sketching a flowchart.
- Consider edge cases: Think about possible inputs that could cause issues, such as empty arrays or nil values. This helps in refining your solution before you even dive into coding.
Breaking the problem into components not only clarifies your path but also reveals potential pitfalls that might trip you up later on.
Testing Your Solution
Finally, after you’ve crafted your code, relentless testing is what will affirm your solution's robustness. Here's how to approach this:
- Start with basic tests: Use straightforward cases that reflect what the problem described to ensure the main features work properly.
- Explore edge cases: Don’t shy away from testing inputs that lie outside the normal expectations, such as maximum values or unexpected types.
- Refactor as needed: If your initial solution isn’t performing optimally or as expected, don't hesitate to refactor it. Swift offers plenty of features that might streamline or simplify your approach.
"Testing isn’t just about finding bugs; it's about ensuring your code actually does what it’s supposed to."
By adhering to these outlined tips: reading diligenty, breaking down problems, and thorough testing, you set yourself up for success in Swift coding challenges. As with any skill, practice enhances these techniques. With time, they’ll become second nature, improving your agility and effectiveness as a programmer.
Resources for Fluent Swift Programming
Understanding the vast landscape of Swift programming and its practical applications is crucial for anyone looking to become proficient. This section focuses on Resources for Fluent Swift Programming, which encompass the tools, materials, and communities that can propel your learning journey in Swift.
Accessing the right resources can make a world of difference. When diving into programming languages like Swift, having diverse options becomes a protective shield against stagnation. Each resource type offers its unique angle, whether it's visual, theoretical, or hands-on. The core idea is to harness these tools effectively for a more comprehensive learning experience.
Online Courses and Tutorials
Online courses and tutorials serve as an excellent starting point for both beginners and seasoned developers alike. Platforms such as Udemy, Coursera, and edX provide a rich tapestry of learning experiences tailored to varying levels of expertise. Here are key benefits of utilizing these resources:
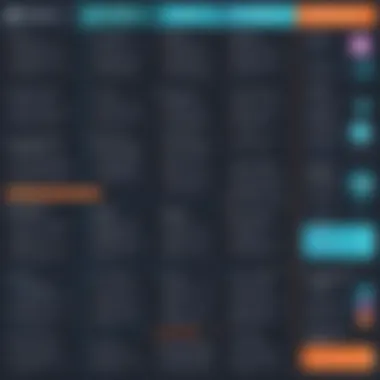
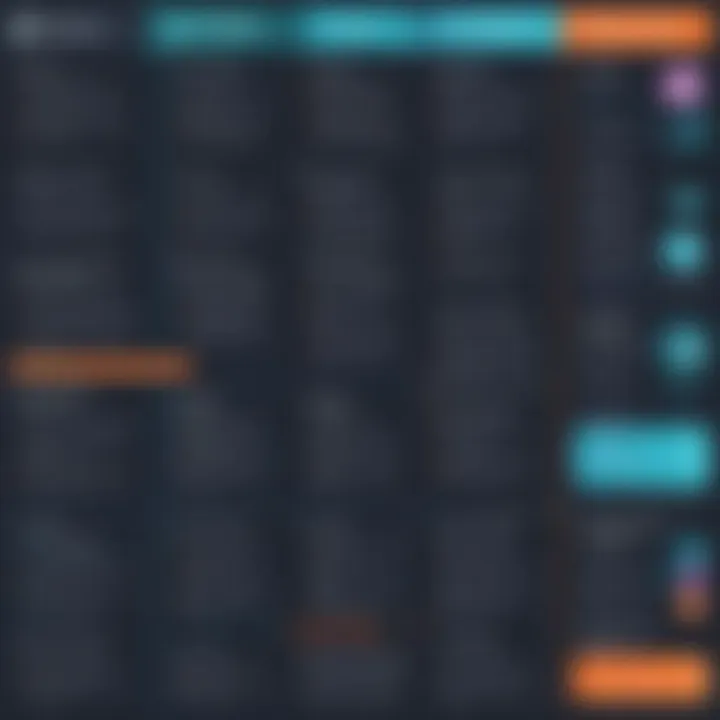
- Structured Learning: Courses often follow a systematic approach, breaking down complex concepts into bite-sized pieces.
- Interactive Components: Many online tutorials include quizzes and exercises that reinforce learning.
- Accessibility: You can learn from anywhere, whether it's on a lunch break or cozying up on your couch at home.
For example, a course like "iOS App Development with Swift" could help learners build a foundational understanding while creating real-world applications. These courses not only teach coding but also project management skills—essential in today's collaborative work environments.
Books and Literature
Books remain timeless resources for those venturing into Swift programming. Titles like The Swift Programming Language by Apple Books and SwiftUI by Tutorials from raywenderlich.com provide in-depth coverage of concepts from the ground up. Here are a few considerations:
- In-Depth Knowledge: Books often delve into subtleties and complexities that online tutorials might gloss over.
- Reference Material: Having a physical book allows one to highlight, annotate, and revisit complex topics easily.
- A Variety of Perspectives: Different authors bring unique insights, enhancing your understanding of Swift.
Investing in literature expands your toolkit, allowing for self-paced exploration of topics like closures or protocols, making you adaptable in various coding scenarios.
Community Forums and Support
Perhaps one of the most underrated resources are community forums. Websites like Reddit and Stack Overflow offer invaluable peer support where you can ask questions, share challenges, and receive constructive feedback. Here’s why engaging with the community is a form of educational gold:
- Real-Time Help: Instantaneous answers to your coding dilemmas can save you hours of frustration.
- Networking Opportunities: Connecting with other developers opens doors to collaboration and mentorship.
- Learning Through Questions: Observing how others tackle problems teaches you before you even face similar issues.
Engaging in forums isn’t just about seeking answers; it’s also about contributing your knowledge. Giving back to the community enhances your understanding and positions you as a resource for others.
"The way to gain a good reputation is to endeavor to be what you desire to appear." - Socrates
In summary, leveraging a variety of resources—be it courses, books, or community forums—can enrich one’s understanding of Swift programming. By cultivating a multi-faceted learning environment, learners can enhance both their technical skills and confidence as they embark on this programming journey.
Practical Applications of Swift
Swift, since its unveiling, has become a powerhouse in the programming realm, showcasing its prowess across diverse applications. This section delves into the practical uses of Swift, illuminating not just its features, but the profound impact it has on development practices today. With its relatable syntax and performance efficiency, Swift has positioned itself as an indispensable tool for developers across various sectors.
Building iOS Applications
When it comes to crafting iOS applications, Swift is the cream of the crop. Developed by Apple, it has been designed specifically for iOS, which is crucial for performance. With Swift, developers can leverage a clean and modern syntax while adhering to Apple's stringent performance standards.
Benefits of Using Swift for iOS Development
- Safety: Swift's type system and error handling mechanisms help in building safer applications.
- Speed: As Swift compiles to native code, it runs faster than its predecessors, which often translates to quicker loading times and better performance.
- Interoperability: Swift can be easily integrated with existing Objective-C code, which offers a smooth transition for teams migrating to a modern environment.
Moreover, Swift's support for dynamic libraries means that developers can include more features without adversely affecting the performance of their apps. It has become the go-to choice for many iOS developers wanting to build robust applications that shine in the app store.
Server-Side Development with Swift
The expansion of Swift beyond the confines of mobile app development has given rise to its application in server-side programming. This shift has been particularly evident with frameworks like Vapor and Kitura, which allow developers to use Swift on the server.
Key Considerations for Server-Side Development
- Performance: Swift is known for its speed, and this is vital for server applications where efficiency is the name of the game.
- Swift’s Type Safety: By ensuring stronger type definitions, errors can be caught during compile time, reducing runtime failures.
- Ecosystem Growth: The vibrant ecosystem surrounding server-side Swift is growing, enabling developers to build full-stack applications using a single language.
This trend towards server-side Swift development is significant, as it allows for cohesive development ecosystems where teams can maintain a uniformity in languages throughout the development pipeline. It efficiently merges client-side and server-side programming, streamlining the workflow considerably.
Using Swift with Machine Learning
With the rising importance of machine learning across industries, Swift's role has expanded into this exciting field. Swift for TensorFlow, a prominent tool, illustrates this intersection between Swift and machine learning, enabling efficient performance with easy-to-read code.
Benefits of Swift in Machine Learning
- Conciseness and Clarity: Swift’s syntax aids in making complex algorithms more understandable, which is crucial for data analysis and modeling phases.
- Integration with Core ML: Apple’s Core ML framework enables developers to integrate trained machine learning models directly into iOS applications, enhancing interactivity and responsiveness for end-users.
- Real-time Processing: Swift's speed allows for real-time data analysis, an essential feature for applications dealing with high-velocity data inputs.
Swift is an excellent choice for developers delving into machine learning, as it seamlessly blends high-performance with an accessible coding style. This combination not only promotes rapid prototyping but also supports long-term project scalability.
In summary, Swift's applications span from mobile development to server-side solutions and machine learning, making it a versatile language in today’s tech landscape. Its emphasis on performance, safety, and clarity contributes to its rising popularity across diverse domains.
The Future of Swift Programming
The landscape of programming is always shifting, and with it comes the evolution of languages that shape our digital world. Swift has carved out a significant niche since its inception, and understanding its future is not merely an academic exercise—it’s crucial for developers, companies, and educators alike. This section will delve into the future of Swift, exploring emerging trends and how this language is positioned within the broader tech ecosystem. The insights gathered here will not only inform your strategies as a programmer but also inspire confidence as you navigate Swift coding challenges.
Emerging Trends and Technologies
The tech field is buzzing with new ideas, and Swift is at the forefront of several of these trends. Here are some developments to keep an eye on:
- Cross-Platform Development: As the demand for apps on multiple platforms increases, Swift’s interoperability is gaining more traction. The SwiftUI framework, for example, allows developers to create applications for iOS, macOS, watchOS, and tvOS using a single codebase.
- Server-Side Swift: Companies are increasingly adopting Swift for server-side applications. This trend is not just a fling; Swift’s robust features are drawing businesses that need reliable and efficient solutions in their backend. Frameworks like Vapor and Kitura are leading this charge.
- Integration with Machine Learning: Swift is starting to blend seamlessly with AI technologies. With integration capabilities like Core ML, developers can easily incorporate machine learning models into their Swift applications. This synergy is essential as AI continues to transform the tech landscape.
"Swift is not just a language for mobile; it’s evolving into a multi-purpose tool that can tackle a range of challenges in the tech realm."
Swift in the Evolving Tech Landscape
As technology transforms over time, Swift finds itself adapting to meet new demands while keeping its essence intact. Some key considerations include:
- Open Source Development: Swift's transition to open source has broadened its scope. Developers from all corners can contribute to its improvement, leading to innovation that aligns with current tech trends. This opens up a door for collaborative growth and community-driven developments.
- Increased Adoption in Academia: Educational institutions are incorporating Swift into their curriculum as a preferred choice for teaching programming. This shift signifies the language's relevance in future software development landscapes.
- Growing Community: With a steadily increasing pool of Swift developers, the community is thriving. Forums like Reddit offer valuable platforms for sharing knowledge and troubleshooting common issues. This communal spirit boosts learning, making it easier for new developers to engage and grow.
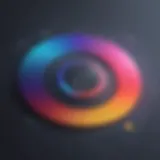
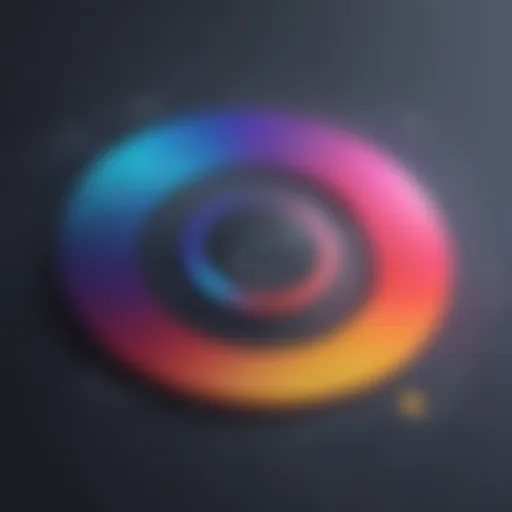