Effective Strategies for Web Application Development
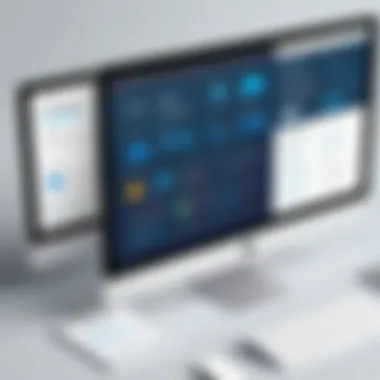
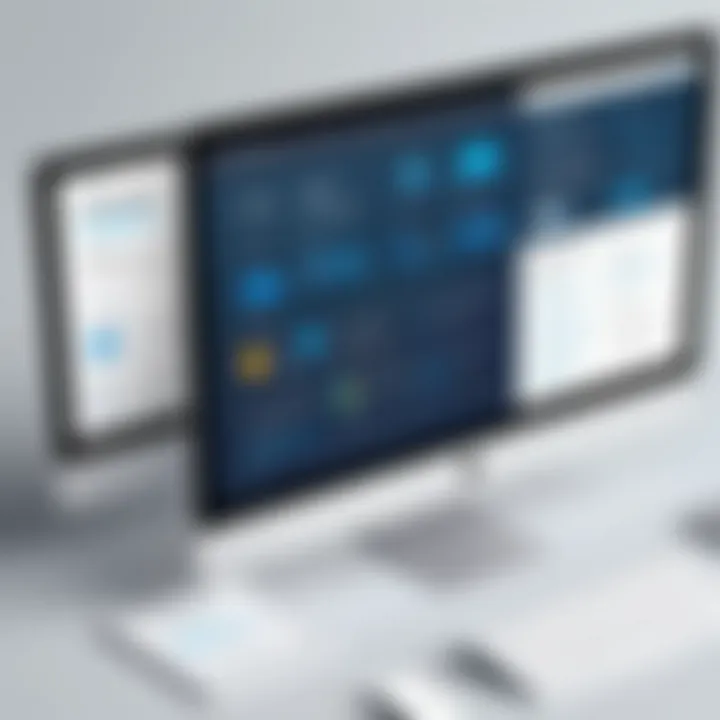
Intro
When embarking on the journey of building a web application, foundational knowledge is key. The choice of programming language plays a significant role in shaping the development process and outcome. Understanding how various languages evolved, their features, and where they fit within the larger tech landscape offers invaluable insight for both newcomers and those looking to deepen their understanding.
Foreword to Programming Language
History and Background
Programming languages have a rich history that dates back to the early days of computing. The first high-level languages, such as Fortran and COBOL, laid the groundwork for what we have today. Over the years, as technology evolved, so did the languages, leading to modern staples like JavaScript, Python, and Ruby. Each language carries its unique tale of development and purpose, reflecting the needs and creativity of its time.
Features and Uses
Different programming languages offer a variety of features that cater to specific needs. For instance:
- JavaScript, a staple for web development, is renowned for its ability to create interactive and dynamic user interfaces.
- Python is celebrated for its simplicity and readability, making it a favorite among beginners and data scientists.
- Ruby, with its elegant syntax and powerful Rails framework, excels in building web applications quickly and effectively.
With these features, developers can select a language that aligns with their project objectives, ensuring that the application meets both functionality and user experience standards.
Popularity and Scope
The landscape of programming languages is always shifting. Languages rise and fall in popularity, influenced by trends, community support, and industry demand. According to the TIOBE index and Stack Overflow surveys, JavaScript continues to dominate web development roles, while Python’s growth in data science and machine learning has positioned it as a versatile powerhouse.
"Choosing the right programming language is like selecting the right tool for the job—the right fit can make all the difference in efficiency and outcome."
Basic Syntax and Concepts
Variables and Data Types
Understanding variables and data types is crucial for any developer. Variables serve as storage for data, and knowing how to manipulate them is essential for programming. Common data types include integers, strings, and booleans, each serving different purposes within the application logic.
Operators and Expressions
Operators are the bread and butter of programming, enabling the manipulation of variables. They can be classified into:
- Arithmetic operators (e.g., +, -, *, /)
- Comparison operators (e.g., ==, !=, >, )
- Logical operators (e.g., &&, ||, !)
These operators allow developers to craft expressions that drive the functionality of the application.
Control Structures
Control structures, such as loops and conditionals, guide the flow of the program. They allow developers to make decisions and repeat actions based on specific conditions, essential for building interactive and responsive web applications.
Advanced Topics
Functions and Methods
Functions are blocks of reusable code designed to perform specific tasks. They not only streamline the process but also enhance code readability and maintainability. Understanding how to create and utilize functions is vital for structuring complex applications.
Object-Oriented Programming
Object-Oriented Programming (OOP) facilitates a modular approach, enabling developers to create objects that encapsulate data and behaviors. Concepts like inheritance, encapsulation, and polymorphism allow for building intricate and scalable applications.
Exception Handling
Error handling, or exception handling, is crucial in making an application robust and user-friendly. Understanding how to anticipate and gracefully handle errors can significantly enhance user experience and application reliability.
Hands-On Examples
Simple Programs
Practicing with simple programs helps solidify concepts. A basic "Hello, World!" application can provide a solid foundation in syntax and language structure. Here's a quick JavaScript example:
Intermediate Projects
Once comfortable with the basics, diving into intermediate projects helps bridge the gap between theory and application. Building a simple to-do list application can provide practical experience with CRUD operations and user interactions.
Code Snippets
Integrating code snippets in learning can illustrate how concepts come together in real-life scenarios. Whether it's a function for calculating the sum of an array or a method for fetching data from an API, these snippets serve as useful references.
Resources and Further Learning
Recommended Books and Tutorials
Books such as "Eloquent JavaScript" and "You Don’t Know JS" provide great insights into web development. Online platforms like Codecademy and freeCodeCamp also offer structured pathways for learners.
Online Courses and Platforms
Consider platforms like Coursera and Udacity for in-depth courses tailored to varying skill levels, including specializations in web development.
Community Forums and Groups
Engaging with communities on platforms like Reddit and Stack Overflow can offer support and guidance as challenges arise during the development process.
Building a web application can seem daunting at first, but understanding the right methodologies, languages, and tools can simplify the journey. Armed with knowledge, developers can create functional and appealing web applications that cater to modern user needs.
Understanding Web Applications
Understanding web applications is fundamental in today’s digital landscape. They serve as essential tools that facilitate tasks, interaction, and access to information for users across the globe. As technology continues to evolve, the relevance and complexity of web applications have grown exponentially. Thus, grasping what they are, how they differ from regular websites, and their functional essence is crucial for anyone diving into web development.
Web applications are not merely static pages; they are dynamic platforms that allow users to perform specific tasks like managing accounts, editing content, or even shopping online. They require a solid grasp of both frontend and backend technologies, making the learning curve steep yet rewarding for budding programmers.
Definition of a Web Application
A web application is, at its core, a software program that runs on a web server. Unlike traditional desktop applications, which are installed on individual computers, web applications are accessed through web browsers. This means that platform independence is built-in. Users can interact with the application using devices like smartphones, laptops, or desktops, irrespective of the operating system.
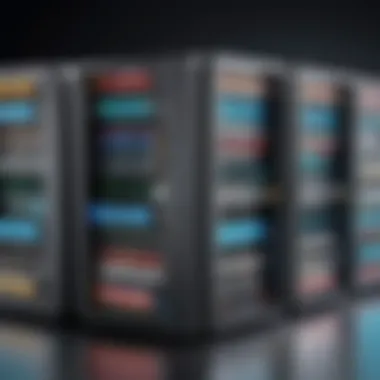
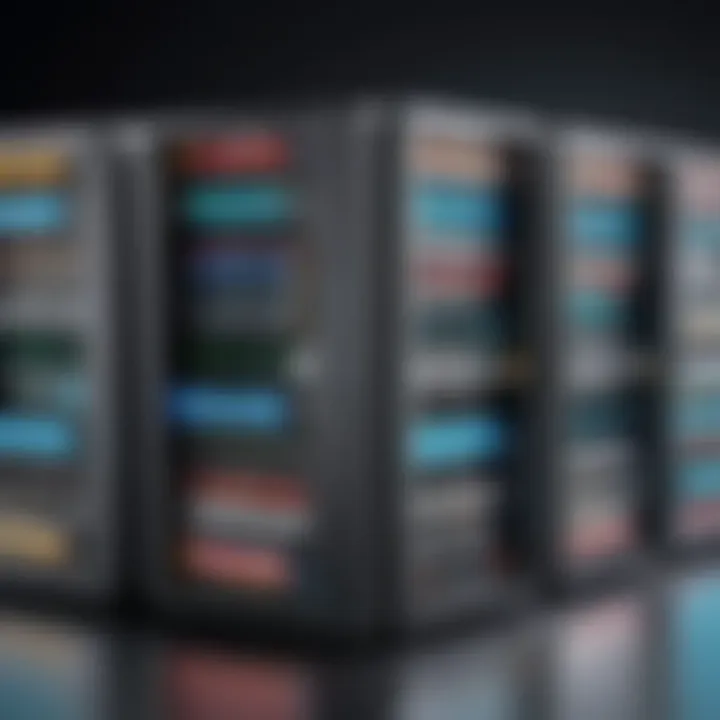
In practice, web applications require a blend of technologies to function effectively. For example, they use client-side languages like HTML, CSS, and JavaScript to create the user interface and experience. Further down the line, server-side languages such as Python, Ruby, or PHP integrate with databases, allowing the web app to handle, store, and manage user data.
Distinction Between Web Apps and Websites
Sometimes, the line between web apps and websites can get a bit blurry. It’s like comparing apples to oranges but let’s break it down. While both are accessible through browsers, the primary difference lies in their functionality and purpose.
- Websites: Typically, these are informational and static, intended to provide content to visitors. Think of an online newspaper. Users can read articles but cannot modify or contribute directly to the content. Websites rely heavily on HTML and might incorporate CSS and some JavaScript.
- Web Applications: These are designed to be interactive, providing functionality for users to do more than just view content. This could include editing profiles, making transactions, or even submitting forms. They often rely on more complex coding and frameworks than a standard website does.
"A true web application allows users to interact dynamically, where actions lead to immediate responses, greatly enhancing the user experience."
In essence, understanding these distinctions and the underlying technologies of web applications is critical for developers. It lays the groundwork for creating robust applications tailored to user needs, emphasizing usability and accessibility, aspects that should never be overlooked.
Key Components of Web App Development
Building a web application is much like constructing a house; it requires a solid foundation and the right materials. The key components of web app development encompass various facets, ranging from user interface design to backend functionality. Understanding these elements is crucial for creating a successful application that meets user needs and performs efficiently.
Frontend Development
Overview of Client-Side Technologies
In web application development, frontend development refers to everything a user interacts with directly. Client-side technologies are the tools that shape the user interface and create an engaging experience. These technologies typically include HTML, CSS, and JavaScript. They allow developers to build visually appealing and responsive applications that work across multiple devices and platforms.
The primary characteristic of client-side technologies is their ability to execute in the user's browser, reducing the load on the server and enhancing performance. This is particularly beneficial because it can lead to a smoother user experience; the application becomes more interactive, with less lag. Additionally, as these technologies are widely supported and well-documented, they form a common foundation that beginner programmers can easily grasp.
However, there’s a trade-off. While client-side processing can improve performance, it can also lead to security vulnerabilities, as sensitive data may be exposed in the browser. Developers must balance usability and security when choosing their approach.
HTML, CSS, and JavaScript
HTML, CSS, and JavaScript are the backbone of web applications, each playing a distinct role. HTML structures the content, CSS styles it, and JavaScript brings it to life through interactivity. Together, they form a powerful trio that empowers developers to create dynamic and engaging web applications.
The standout feature of this combination lies in its simplicity and ubiquity. HTML is easy to learn, making it ideal for newcomers, while CSS allows for extensive customization of appearances. JavaScript enables advanced functionalities, allowing developers to implement features like form validation, animations, and real-time updates.
The downsides include potential performance issues. If not optimized, JavaScript-heavy applications can suffer from lag, leading to a frustrating user experience. Furthermore, as a web app scales, managing code complexity can become challenging.
Backend Development
Server-Side Languages
Shifting gears to backend development, server-side languages are crucial for handling the logic behind web applications. These languages, which include PHP, Python, Ruby, and Java, are responsible for managing database interactions, user sessions, and server communications.
The key characteristic of server-side languages is their ability to process data securely on the server before sending the results to the client. This means sensitive operations, such as user authentication and database queries, can be conducted away from the user's view, enhancing overall security. The popularity of these languages is also based on their extensive libraries and frameworks that help streamline the development process.
Yet, with great power comes great responsibility. Server-side operations can become a bottleneck if not managed properly, leading to slower response times. The right choice of language and framework is vital to ensure that the application scales effectively as user demand increases.
Frameworks and Architecture
When it comes to backend frameworks and architecture, they act as blueprints that streamline the development process. Frameworks like Django for Python, Laravel for PHP, and Ruby on Rails for Ruby provide pre-organized code structures and components that significantly reduce development time.
The main benefit of using these frameworks is their focus on best practices and efficient code reuse. By following a structured approach, developers can avoid common pitfalls and build more maintainable and secure applications.
On the downside, the initial learning curve can be steep for beginners unfamiliar with specific frameworks. Moreover, reliance on a framework without understanding its underlying principles can lead to difficulties in troubleshooting or customizing solutions to unique problems.
In summary, the key components of web application development encompass frontend and backend elements, each with its own unique features. As a prospective developer, grasping these concepts is vital for building applications that not only serve their purpose but do so in an efficient and user-friendly manner.
Choosing the Right Technology Stack
Selecting the right technology stack is like choosing the right tools for a craftsman. Boxing yourself into a corner with an inadequate set can lead to frustrating outcomes, delays, and a whole heap of redesign or rework down the line. A sound technology stack serves as the backbone of your web application, guiding you through user demands and project requirements. It’s crucial for the longevity of the app and its capacity to adapt to future needs.
When weighing your options, consider several elements:
- Project Requirements: What are the specific functionalities you want your app to have?
- Team Expertise: Are your developers comfortable using the selected technologies?
- Community Support: Is there a large community backing the technology for troubleshooting and resources?
- Budget Constraints: Will the technology fit within your budget, including licensing?
A robust technology stack not only facilitates efficiency but can also make a world of difference in scalability, performance, and maintenance. In short, investing time to choose wisely now pays dividends later.
Popular Technology Stacks
MEAN Stack
The MEAN stack, comprising MongoDB, Express.js, Angular, and Node.js, presents itself as a complete package for JavaScript developers. One key characteristic of the MEAN stack is its ability to run JavaScript on both the frontend and backend—unifying your development under one language. This consistency paves a smoother path for collaboration among teams, decreasing friction and streamlining code.
A unique feature of MEAN stack is its non-relational database, MongoDB. This gives developers flexibility in how they structure data, which suits applications that might see rapid evolution in their data needs. The downside? It might not be the best fit for apps requiring complex transactions typical in financial sectors, as traditional SQL databases handle that more gracefully.
MERN Stack
Similar to the MEAN stack, the MERN stack swaps Angular for React, providing a more dynamic approach to building user interfaces. The key characteristic here is React’s efficiency and potential for high performance in rendering complex UI components. This key trait makes MERN a notable choice for those who value a rich user experience.
A unique aspect is its component-based architecture, which allows for the reusability of code and easier management of UI states. The downside could be React's steeper learning curve, especially for newcomers who may find the ecosystem around it slightly overwhelming.
LAMP Stack
Dating back a while now, LAMP stands for Linux, Apache, MySQL, and PHP. This stack is a classic vote of confidence in tried-and-true technologies. The fundamental characteristic of LAMP is its strong foundation built on open-source components, standing as an affordable option for startups.
Notably, LAMP has wide-ranging community support and vast resources available, which can come in handy when challenges arise. However, it may not be the right choice for projects seeking fast, real-time performance as its reliance on PHP could become a bottleneck compared to more modern stacks dealing with non-blocking operations, like the MEAN or MERN stacks.
Importance of Scalability and Performance
When developing a web application, keeping scalability and performance in mind is often what separates a successful launch from a crashing flop. Scalability ensures that your app can handle growth in user traffic or data volume without a hitch. If your application is a runaway success, you don’t want to be scrambling to keep it afloat.
Performance, on the other hand, is all about how efficiently your application runs. No one likes dealing with slow-loading pages or laggy interfaces, and in a competitive market, users may jump ship to a competitor with snappier performance.
By selecting the right technology stack, you'll have the ingredients for both scalability and performance out of the gate. This will help you build a web application that not only functions well upon launch but evolves alongside your users and market trends.
"A technology stack is more than just software; it’s the lifeblood of your application."
In essence, focusing on your technology stack can make or break your ambitions in app development. Thoroughly assessing each of these elements can empower your project and pave the way for future successes.
Development Methodologies
In the realm of web application development, the choice of methodology is not just an afterthought; it shapes the entire project lifecycle. Whether you’re a student dabbling in your first coding project or a seasoned developer tackling a complex enterprise application, understanding various development methodologies is crucial.
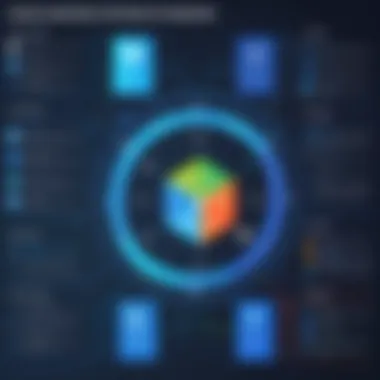
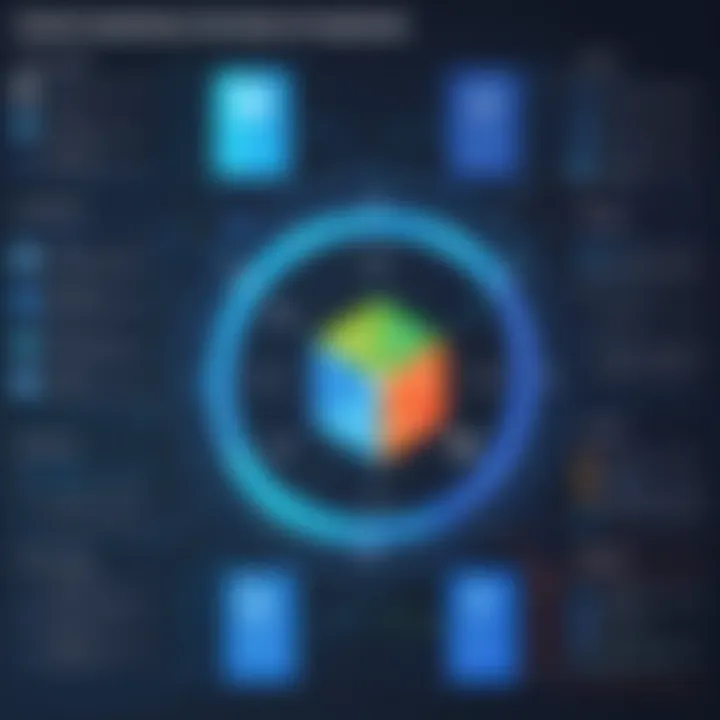
Using the appropriate methodology isn't only about ensuring that the app is functional; it also involves fostering communication, collaboration, and adaptability within the team. This becomes especially apparent in fast-paced environments where requirements are likely to shift, and teams must remain nimble to meet user needs and market demands.
Here, we’ll examine two of the most prominent methodologies: Agile and Waterfall, each with its unique approach to tackling development processes.
Agile Development Process
Agile development stands as one of the most effective processes in today's dynamic tech landscape. Emphasizing flexibility, collaboration, and early delivery, Agile encourages teams to break down projects into smaller, manageable units called iterations or sprints. This allows for constant feedback and improvement over time.
In essence, the Agile methodology is not just a set of practices; it's a mindset. The team continually evaluates progress through regular check-ins, fostering a sense of ownership and accountability. This is a distinguishing feature of Agile, setting it apart from traditional approaches.
Key Elements of Agile Development:
- Iterative Progression: Incremental improvements ensure that changes can be integrated smoothly based on user feedback.
- Collaboration: Stakeholder involvement is paramount, ensuring that the end product aligns closely with user expectations.
- Adaptability: Agile teams are not tied down by rigid planning and can pivot as needed when requirements change.
This approach results in faster delivery times, increased user satisfaction, and overall better product quality. While Agile may come with challenges, like the need for constant communication, its benefits far outweigh the drawbacks.
Waterfall vs. Agile
When comparing Waterfall to Agile, it’s like contrasting apples and oranges. Waterfall follows a more linear and sequential approach. The process flows through distinct phases like requirement analysis, design, implementation, and maintenance. However, this rigidity can lead to complications when changes arise mid-project.
Considerations When Choosing Between Waterfall and Agile:
- Project Nature: For projects where requirements are unlikely to change, like construction or manufacturing, Waterfall might be more fitting.
- Flexibility Needed: If user feedback is crucial and the project demands frequent iterations based on evolving requirements, Agile shines.
- Team Structure: Agile often works best with smaller, cross-functional teams capable of self-organization. Waterfall may suit larger teams with defined roles and responsibilities.
"Agile methodologies foster environments where change is embraced, providing opportunities to refine and enhance the user experience."
While both methodologies can achieve successful outcomes in different contexts, it’s essential to weigh your project's specific needs carefully. Ultimately, the right approach hinges on understanding your goals, team dynamics, and the product's intended functionality.
Adopting an informed stance leads to more effective web applications, better team performance, and ultimately a more satisfying experience for end users.
User Experience and Design Considerations
Creating a web application is not merely about lines of code or complex functionalities; it’s also about how users interact and feel with the platform. The significance of user experience (UX) and design can’t be overstated. A well-designed application is like a smooth road for drivers; it improves navigation, enhances satisfaction, and keeps users coming back for more. Without these elements, even the most sophisticated web app risks failing to capture its audience.
Importance of UI/UX Design
UI (User Interface) and UX design play a critical role in how users engage with a web application. Imagine walking into a beautifully arranged store, where every item is easy to find and the layout invites you to explore. That’s what effective UI/UX design does for web applications.
- First Impressions Matter: The initial look and feel of a web app can make or break user engagement. Studies suggest that users form opinions about a website in less than 50 milliseconds. Think of UI as the first handshake; if it's strong, users are more likely to stick around.
- Ease of Use: A web app should be intuitive and straightforward. Users shouldn’t have to pull their hair out trying to navigate or figure out how to complete a task. Clear calls to action, engaging visuals, and logical flow will ensure users can achieve their goals without unnecessary frustration.
- Consistency Builds Trust: Just like a well-furnished home, consistency within a web app creates a pleasant atmosphere. Users expect to see familiar buttons, layout structures, and fonts, which can build trust in the application. This trust nurtures user loyalty, making them likely to return.
A properly considered UI/UX design leads to more satisfied users, which in turn can translate to higher conversion rates.
Responsive Web Design Principles
Responsive web design is no longer an option but a necessity. With a multitude of devices available – from smartphones to tablets to laptops – ensuring that your web application provides a seamless experience across all platforms is crucial. Here are some core principles to consider:
- Fluid Grids: Layouts should adapt fluidly to the screen size. Instead of using fixed pixel widths, use percentages to make elements proportionate to their container.
- Flexible Images: Images should resize correctly according to the screen size or container. Using CSS techniques like keeps them from overflowing their containing elements.
- Media Queries: CSS media queries allow the application to change styles depending on the characteristics of the device viewing it. This tailored approach ensures optimal viewing regardless of screen dimensions.
Responsive design is about creating an experience that fits from the smallest to the largest screens.
- Navigation Simplification: On mobile devices, prioritize essential navigation options. A hamburger menu or a simple bottom navigation bar can keep the interface clean and user-friendly.
In summary, placing great emphasis on UI/UX design and adhering to responsive web design principles are paramount in crafting a successful web application. Neglecting these areas could mean missing out on potential users and revenue.
Integration with Databases
In the realm of web application development, integration with databases holds a vital role. This is not simply about storing data; it’s about ensuring that the application runs smoothly and efficiently. From user data to app settings, databases are the backbone that allows web developers to transport, store, and manage data effectively. Integrating a robust database solution provides several benefits, including increased performance, better data accessibility, and improved data security. Proper database integration ensures that a web application remains responsive while handling multiple requests, which is fundamental for user satisfaction.
Types of Databases
Choosing the right type of database is a critical decision in the web development process. Knowing the difference between relational databases and NoSQL databases can greatly influence how you design your application.
Relational Databases
Relational databases, such as MySQL and PostgreSQL, utilize a structured format to store data. They use tables to manage relationships among various entities. One prominent characteristic of relational databases is their use of Structured Query Language (SQL) for defining and manipulating data. This offers clear advantages, primarily in data integrity and accuracy due to the enforceable schema.
Nevertheless, they also have downsides. For instances where scalability or high availability is paramount, these databases might become less efficient. Despite that, their robustness makes them a popular choice for applications requiring complex queries or transactions.
"A relational database allows for a strong degree of data integrity, ensuring that what you store is validated and structured effectively."
NoSQL Databases
On the flip side, NoSQL databases like MongoDB and Cassandra cater to a different set of needs. They are known for their flexibility, allowing for unstructured data storage. This key feature makes them an excellent choice for applications that require rapid scaling or when the data model isn't well-defined.
While NoSQL databases shine in performance for large volumes of data and high throughput operations, they can lack the robust transactional support that relational databases offer. Consequently, this can lead to challenges in data integrity during simultaneous updates or high-traffic scenarios. Thus, knowing when to use a NoSQL database can position your application to handle today's demands efficiently.
Data Handling Techniques
Once the decision on the database type is made, handling the data properly is next in line. Effective techniques for managing data can determine the success or failure of an application. Understanding CRUD (Create, Read, Update, Delete) operations is fundamental for any developer. These operations integrate directly into handling users' requests efficiently. Moreover, ensuring that data is normalized helps in avoiding redundancy, thus enhancing the overall performance of the application.
Testing and Quality Assurance
Testing and quality assurance are absolutely vital in the realm of web application development. Without proper testing, your application could be riddled with bugs, inconsistencies, and performance issues that could drive users away faster than you can blink. Think of testing as the safety net that ensures your web app operates smoothly.
The principal goal of testing is to guarantee that the software functions as intended. This might appear straightforward, yet the intricacies involved can become quite complex. The benefits of diligent testing include enhanced user satisfaction, lower maintenance costs in the long run, and improved code quality. Regular quality assurance checks lead to reliability, which is fundamental for user retention.
Types of Testing
Unit Testing
Unit testing focuses on verifying the smallest parts of your application in isolation. These units, often individual functions or methods, undergo scrutiny to ensure each operates correctly. The beauty of unit testing lies in its ability to catch bugs early in the development cycle, which is money saved in the long run.
A defining feature of unit tests is their modular nature—they allow developers to test specific pieces of code without running the entire application. This approach not only fosters a faster development cycle but also increases confidence in code changes. However, unit testing can be time-consuming initially, requiring well-structured tests to cover various scenarios. The trade-off is worth it, as it leads to cleaner, more maintainable codebases.
Integration Testing
Once the individual units are tested, integration testing comes into play to ensure that these units work together as intended. Integration testing is crucial because it helps identify issues that may not surface during unit testing. Interactions between components can cause unexpected behavior that could derail the user experience.
A key characteristic of integration testing is its broader scope compared to unit tests. It incorporates the interaction vectors between various parts of the system—like how the database interacts with the backend API. The downside? Integration tests can be tricky to set up and may require a more elaborate environment. However, the resulting insights into your application's functionality are indispensable, marking it as a popular choice for ensuring cohesive application performance.
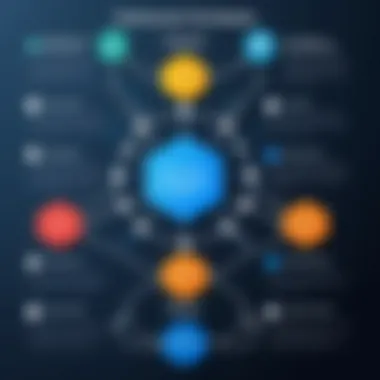
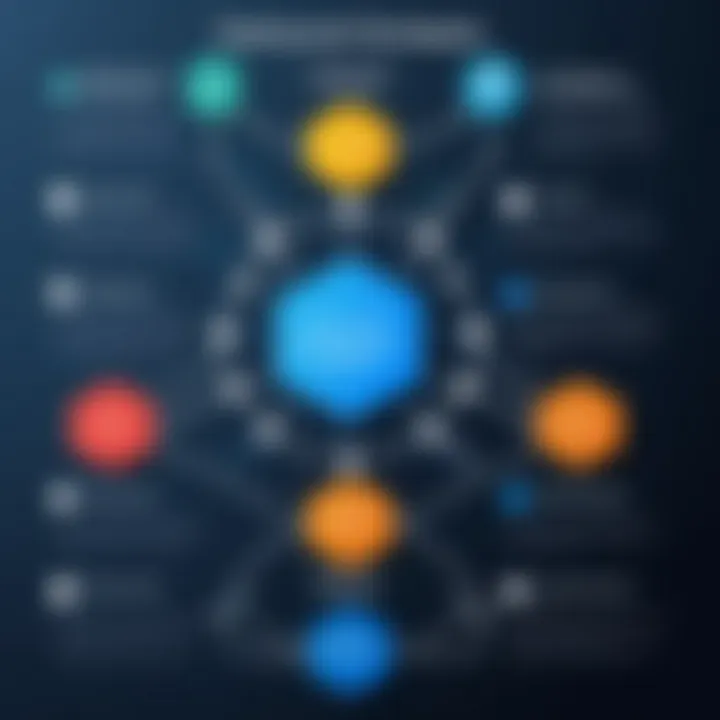
User Acceptance Testing
User acceptance testing (UAT) is where the rubber meets the road. This stage involves real users operating the application to validate its capabilities against their expectations and requirements. UAT is significant as it focuses not on the technical operations of the software but rather on its usability.
The hallmark of UAT is its user-centric approach, engaging actual users to assess whether the application meets business needs. While UAT can surface critical usability issues, gathering user feedback can often become a double-edged sword; it can lead to scope creep if not managed carefully. Nonetheless, it provides invaluable insights that developers often overlook, solidifying its role as a cornerstone in the development process.
Automated vs. Manual Testing
The mechanics of testing can generally be categorized into automated and manual testing. Automated testing utilizes software tools to perform tests that would be time-intensive to complete by hand, which is particularly useful for regression tests or for repetitive tasks. This efficiency helps teams focus more on writing new features rather than sifting through past code.
On the flip side, manual testing relies on human insight. This more subjective process is useful for exploratory testing where intuition and creativity play key roles. Manual testing can be invaluable for UI/UX analysis since humans are more adept at discerning experience nuances that tools simply can't recognize.
Ultimately, choosing between automated and manual testing often depends on the specific project requirements, team expertise, and budget constraints. A well-balanced strategy typically involves an interplay of both methods to tailor a comprehensive testing framework that addresses all necessary facets of application quality.
Deployment Strategies
When it comes to building a web application, deployment strategies tinker at the heart of ensuring a seamless transition from development to production. It may seem like a mere step in the process, but how and where you deploy your application can greatly affect its performance, reliability, and user experience. Therefore, understanding various deployment strategies is fundamental for any developer keen on crafting robust web applications.
Choosing a Hosting Provider
The choice of a hosting provider plays an instrumental role in the overall success of your web application. Typically, there are several options available: shared hosting, virtual private servers (VPS), dedicated servers, and cloud hosting. Each platform comes with its own set of pros and cons, depending on your application's requirements and projected traffic.
- Shared Hosting: Generally the most cost-effective, but it can limit performance and scalability as resources are shared among multiple users. This might work well for small applications or those still in the testing phase.
- VPS Hosting: This offers a middle ground by providing dedicated resources while still keeping costs reasonable. It strikes a balance, giving you more control and flexibility over your server environment.
- Dedicated Servers: If your app demands high performance and security, dedicated servers may be the solution, though they come at a premium.
- Cloud Hosting: This option scales automatically according to traffic and usage, which is particularly useful for applications that experience unpredictable loads.
Choosing a provider isn’t purely about cost. You also need to consider uptime guarantees, customer support, and whether they support the technologies you plan to use. The right hosting provider not only ensures that your application is accessible but also enhances its resilience and reduces the chances of downtime.
Continuous Integration and Deployment
The realms of Continuous Integration (CI) and Continuous Deployment (CD) are game-changers in modern web application development. By establishing a CI/CD pipeline, developers can automate the process of integrating code changes from multiple contributors into a shared repository. This helps in identifying issues earlier in the development cycle and improves the overall quality of the software.
- Continuous Integration allows code from multiple developers to be merged frequently, reducing integration challenges and leading to fewer bugs. Each commit triggers an automated build, allowing for immediate feedback.
- Continuous Deployment, on the other hand, extends this automation further. Once code passes automated tests, it can be deployed directly to production without manual intervention. This ensures that new features or fixes reach users quickly and efficiently.
A well-implemented CI/CD strategy can enhance collaboration among team members, improve deployment speed, and reduce the risk of human error in deployment processes.
In fact, organizations that adopt CI/CD practices often report significant efficiency gains and faster time-to-market for their applications.
In summary, effective deployment strategies can greatly influence the functionality and success of your web application. Choosing the right hosting provider, combined with a rigorous CI/CD pipeline, lays the foundation for a scalable, efficient, and resilient application, ensuring that it stands out in a competitive landscape.
Maintenance and Updates
Maintaining and updating a web application is like tending to a garden; neglect can lead to weeds that choke the life out of what you've worked hard to grow. In the realm of web development, regular maintenance ensures that your app remains functional, secure, and user-friendly over time. As technology evolves, so do user expectations and security threats. Thus, understanding the significance of maintenance isn’t just advisable—it’s essential for longevity in the digital landscape.
Importance of Regular Maintenance
The importance of regular maintenance can't be overstated, especially for developers and businesses aiming to provide a seamless user experience. Regular maintenance involves fixing bugs, updating software, and ensuring compatibility with new technology. This ensures that your application not only runs smoothly but also continues to meet the needs of users.
- User Satisfaction: Frequent updates enhance user experience. Regular performance tweaks and feature refreshes keep users engaged and happy with the product.
- Security: Cyber threats are rampant. Each piece of updated software comes with enhanced protections against vulnerabilities. Regular patching can prevent security breaches that may lead to data loss.
- Improved Performance: Just like a car needs regular check-ups to perform at its best, web applications benefit from hardware and software tuning. This optimization can lead to faster load times and a smoother experience for users.
- Adaptation to New Technologies: With rapid advancements in technology, apps need to integrate with new system updates, frameworks, and tools to remain relevant. Regular maintenance allows you to keep up with these trends and introduce new features.
Regular maintenance may require an investment of time and resources, but the dividends it pays in user retention and satisfaction are invaluable.
Monitoring Performance and User Feedback
Continuous monitoring of performance and gathering user feedback should be at the forefront of maintenance efforts. After all, what good is an app if it doesn’t align with user expectations or faces downtime? Monitoring tools play an integral role, allowing developers to keep a keen eye on how well the application performs.
- Performance Metrics: Utilize tools like Google Analytics or New Relic to keep track of application performance. Identifying slow loading times or excessive downtime can lead to immediate fixes, enhancing overall user experience.
- User Feedback: Incorporate channels for users to provide feedback. This could be through surveys or direct user interactions. Taking this seriously not only helps identify issues but also fosters a sense of community and value around your app.
- Iteration Based on Feedback: Use the feedback you receive as a springboard for new features or alterations. The landscape of user needs is constantly shifting, and being open to change can give your application a robust foothold in the market.
By actively engaging in monitoring and embracing user feedback, web applications can evolve in a way that prepares them for future challenges and ensures they remain pivotal in users' lives. > "An app that adapts and listens is an app that survives."
Investing in ongoing maintenance and being proactive about changes not only shields against potential pitfalls but also lays the groundwork for a thriving web application. With a commitment to continuous improvement, developers can unlock the true potential of their platforms.
Future Trends in Web App Development
As we look ahead to the landscape of web development, it's clear that staying informed about future trends is crucial. The field continually evolves, driven by technological advancements and changing user expectations. Understanding these trends allows developers and businesses to future-proof their projects and harness new capabilities for improved user satisfaction and engagement.
Emerging Technologies
Artificial Intelligence
With the rise of Artificial Intelligence (AI), many aspects of web application development have transformed significantly. A primary characteristic of AI in web apps is its ability to analyze vast amounts of data fast, enabling smarter decision-making processes. For instance, AI can enhance user personalization by predicting user preferences based on their interaction patterns.
This technology stands out because of its versatility; it can automate processes such as customer service through chatbots, or enhance user experiences by incorporating intelligent recommendation systems.
Advantages of AI:
- Increased efficiency: Automates tasks that would otherwise take much longer for humans to complete.
- Enhanced user experience: Offers customized experiences based on user behavior.
Disadvantages of AI:
- Implementation complexity: More intricate to set up than traditional programming methods.
- Potential bias: If not properly managed, AI systems can reproduce existing biases in their training data.
Progressive Web Apps
Progressive Web Apps (PWAs) serve as a bridge between web and native applications, leveraging modern web capabilities to deliver an app-like experience. One of their key traits is offline functionality; while traditional web apps require constant internet access, PWAs can operate without a connection, offering a more flexible user experience.
The unique feature of PWAs lies in their accessibility. Users can install them directly from the browser and access them like native apps. This simplifies the user journey significantly, allowing for instant access without the hassle of navigating app stores.
Advantages of PWAs:
- Cross-platform compatibility: Works seamlessly across devices, enhancing reach.
- Improved performance: Faster loading times lead to better user retention.
Disadvantages of PWAs:
- Limited browser support: Although growing, some functionalities may still not work on all browsers.
- Device capabilities: Not all native functions can be accessed, thus limiting features.
Impact of Mobile Development
Mobile development continues to dominate the digital landscape, with more users relying on their smartphones for web access. This shift emphasizes the need for responsive and mobile-first designs. Developers must ensure that web applications are optimized for smaller screens and touch interactions. A focus on mobile development isn't merely a trend; it's become a prerequisite for any successful web app strategy.
To adapt, consider this advice:
- Empathize with your users: Understand their mobile behavior to create a more intuitive experience.
- Test extensively: Different devices and screen sizes can affect how users engage with your app. Regular testing can help catch issues early.
"In the world of web applications, adapting to new trends isn't optional; it's an imperative for future success."
With this understanding of future trends, developers can strategically align their projects with emerging technologies and user demands, creating web applications that are not only relevant but also poised for longevity in a competitive market.