Mastering Email Automation with Python3: A Complete Guide
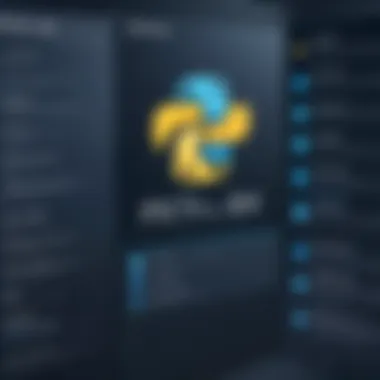
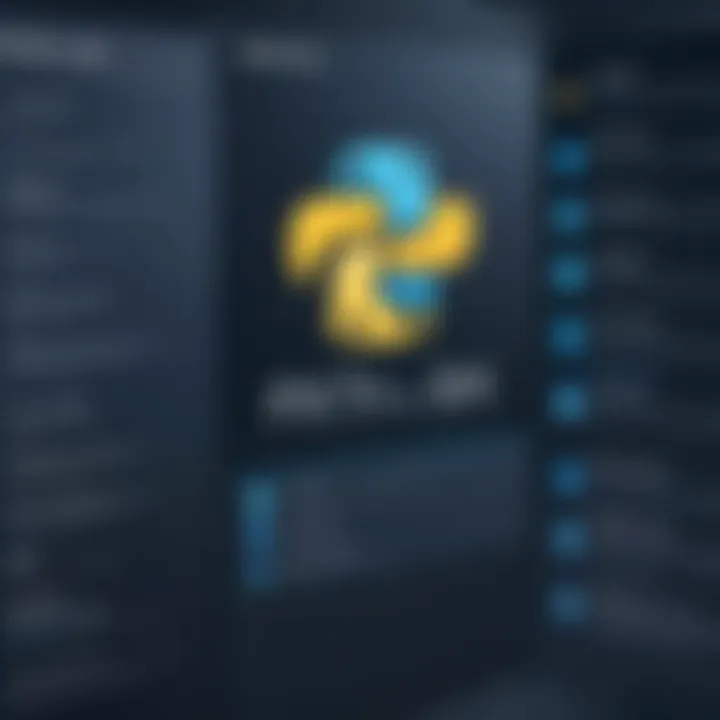
Intro to Programming Language
Programming has become an essential skill in today’s digital world. Among multiple languages, Python stands out because of its simplicity and flexibility. The goal of this article is to explore how Python can be used for sending emails. By focusing on practical aspects, we aim to enhance your understanding and skills in automating email communication.
History and Background
Python was created by Guido van Rossum and first released in 1991. It was designed to be easy to read and write, making it accessible to both beginners and experienced programmers. Over the years, Python has evolved significantly. Its extensive libraries and frameworks have enabled it to be used in web development, data analysis, artificial intelligence, and more.
Features and Uses
Python's key features include:
- Easy Syntax: Its clear syntax makes it straightforward for new developers.
- Versatile Libraries: Libraries such as and simplify email operations.
- Cross-Platform: Python runs on various operating systems like Windows, macOS, and Linux.
These features make Python the preferred choice across many industries for tasks that include automating email processes.
Popularity and Scope
Python’s popularity is profound and growing. As per the latest surveys, it ranks among the top programming languages. This is due to its strong community support and a wide range of applications. The scope of Python in programming continues to widen, especially in the area of email automation.
Basic Syntax and Concepts
Understanding the basic syntax is crucial when working with Python for sending emails. Here are some essential concepts:
Variables and Data Types
In Python, variables are used to store data. Common data types include:
- Strings: Text data, e.g.,
- Integers: Whole numbers, e.g.,
- Floats: Decimal numbers, e.g.,
- Booleans: True or false values, e.g.,
Operators and Expressions
Python supports various operators, such as addition (), subtraction (), multiplication (), and division (). These operators allow you to perform mathematical operations easily.
Control Structures
Control structures like , , and help manage the flow of the program. For instance, you can use an statement to check if an email is sent successfully:
Advanced Topics
To send emails effectively, one must delve into advanced topics. Understanding functions, object-oriented programming, and exception handling is vital.
Functions and Methods
Functions are reusable blocks of code that perform a specific task. This is helpful, for instance, in crafting email messages. You can create functions to set recipients and content, enhancing code efficiency.
Object-Oriented Programming
Python supports object-oriented programming (OOP) which allows for better organization of code. By using classes, you can represent email attributes and behaviors, making code more intuitive.
Exception Handling
When working with email protocols, issues may arise. Utilizing exception handling with and blocks can help manage errors gracefully, ensuring the program runs smoothly even when problems occur.
Hands-On Examples
Simple Programs
To begin, a straightforward script can send a basic email. Using the , you can create a minimal email sender:
Intermediate Projects
As you progress, you might want to schedule email sending. This project might involve using a task scheduler or libraries like to automate the task.
Code Snippets
Here is a helpful snippet for handling attachments:
Resources and Further Learning
To deepen your knowledge, the following resources are valuable:
- Recommended books include "Python Crash Course" by Eric Matthes.
- Online courses on platforms such as Coursera and Udemy cover Python comprehensively.
- Join community forums like Reddit's r/learnpython and Facebook groups to connect with other learners and experts.
Remember, regular practice is key to mastering email automation in Python.
This guide provided a robust starting point for learning how to send emails with Python3. By understanding each concept and practicing examples, you are well on your way to becoming proficient in this essential skill.
Preamble to Email Automation in Python3
Email automation in Python3 has become increasingly relevant in today's digital communication landscape. With rapid advancements in technology, the need for efficient methods to send and manage emails has never been greater. Email automation can save time, reduce errors, and enhance communication effectiveness. As Python3 is a powerful programming language, it simplifies the process of automating email tasks.
One important aspect of email automation is the ability to programmatically send messages at scale. This is crucial for businesses and developers looking to engage with their audience swiftly and consistently. Email marketing campaigns, notifications, and reminders are only a few examples of tasks that can be automated using Python3. Such automation provides the flexibility to customize messages based on user data, thereby enhancing the relevance of communications.
Benefits of automating email tasks include increased productivity and the ability to reach larger audiences without manual intervention. Furthermore, automated emails can be scheduled and personalized, ensuring they reach recipients at the right time and with tailored content, improving overall user engagement.
When considering email automation, it is essential to understand not only its advantages but also potential challenges. Ensuring the delivery of emails to the inbox instead of spam folders, maintaining compliance with regulations, and ensuring data security should be prioritized. These factors are critical to ensure successful implementation of automated email functions in any project.
"Email automation not only streamlines communication but also transforms how organizations connect with their audiences."
Overall, mastering email automation with Python3 equips programmers and organizations with tools that improve efficiency and facilitate seamless communication.
Importance of Email Automation
Email automation serves as a cornerstone for effective digital communication strategies. The ability to automate sending emails plays a vital role in not only delivering information but also in managing customer relationships. Automation reduces human error, ensuring that messages are consistently sent and formatted correctly. This efficiency saves valuable time for programmers and organizations alike.
For instance, automated responses to inquiries can be set up using Python3, allowing businesses to maintain engagement without manual oversight. This not only helps in providing timely responses but also assists in maintaining a professional image. Automation leads to consistent branding across all communications, critical for reputation management.
As organizations grow, the volume of emails typically increases. Automation facilitates the handling of this volume without overwhelming employees. This scalability is particularly important for businesses that rely on email marketing or customer outreach initiatives.
Use Cases for Sending Emails with Python
Python’s versatility opens the door to numerous use cases for sending emails. Here are a few key applications:
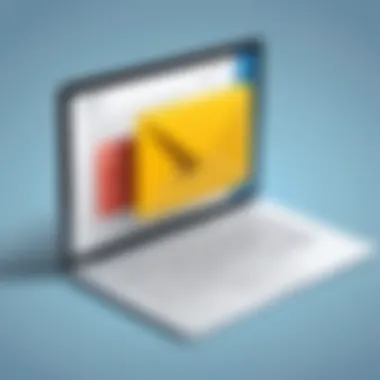
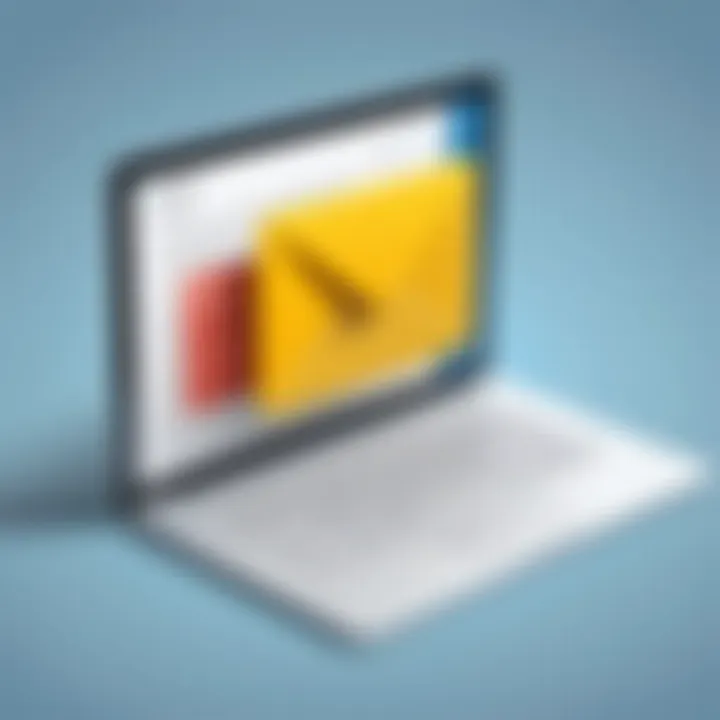
- Marketing Campaigns: Automating promotional emails to target specific audience segments can optimize engagement and conversion rates.
- Notification Systems: Developers can use Python to create systems that send alerts for events such as account registration, password changes, or updates on services.
- Automated Reminders: Sending reminders for appointments or deadlines can help users manage their time more effectively.
- Surveys and Feedback: Collecting user feedback through automated email surveys can provide valuable insights for improving services or products.
By integrating email functionality into various projects, Python3 allows for effective communication strategies that facilitate interaction with users in a personalized and timely manner.
Understanding SMTP Protocol
The Simple Mail Transfer Protocol (SMTP) forms a core part of email technology. Understanding SMTP is crucial for anyone interested in automating email communication through programming, particularly with Python. SMTP is the standard protocol for sending emails across the Internet. It plays a pivotal role by defining the rules and procedures for email transmission, ensuring messages are sent and received effectively. By mastering SMTP, programmers can enhance their applications, creating systems that efficiently manage email notifications, alerts, or communications.
SMTP simplifies the process of email sending by acting as a middleman between the email client and the server. This protocol allows clients to communicate with the server, facilitating the successful transfer of messages. Hence, a solid grounding in SMTP not only streamlines communication but also leads to fewer errors and improved user experiences.
What is SMTP?
SMTP stands for Simple Mail Transfer Protocol. It is a protocol used for sending emails from one server to another. SMTP is an application layer protocol that operates over the Transmission Control Protocol (TCP). Essentially, it defines the commands and responses supported by the SMTP server, allowing clients to transmit data effectively.
SMTP primarily works by establishing a connection with an email server, sending email data, and then completing the transaction.
Here are several key features of SMTP:
- Text-Based: SMTP operates through a text-based interaction between clients and servers.
- Push Protocol: SMTP is considered a push protocol since it pushes emails from the sender's system to the recipient’s.
- Reliability: It ensures messages are transferred reliably, handling outgoing emails efficiently.
How SMTP Works
The functioning of SMTP involves several important steps. When an email is sent, the process unfolds as follows:
- Client Initiation: The email client establishes a connection to the SMTP server using a specific IP address and port number, usually port 25 or port 587 for secured connections.
- HELO Command: Upon connection, the client sends a HELO command, introducing itself to the server. The server acknowledges the request, indicating it's ready to receive emails.
- Mail From Command: The client sends the sender's email address using the MAIL FROM command. This informs the server who the sender is.
- Recipient's Address: The client specifies the recipient using the RCPT TO command. The server checks if the address exists.
- Data Command: Following the recipient acknowledgment, the client sends the DATA command, signaling that it will send the email content next.
- Content Transmission: The client transmits the full email content, including the subject and body.
- Completion: The client sends a termination command, and the server acknowledges the successful receipt of the message.
SMTP’s structure ensures that emails are sent efficiently and correctly. The use of a command-response format allows developers to implement error handling and debugging processes more effectively, contributing to a more resilient email communication system.
Remember, understanding SMTP is fundamental for creating any email automation application in Python. Without grasping how this protocol works, it is challenging to send emails successfully.
Setting Up Your Environment
Setting up your environment is a crucial step when beginning to send emails using Python3. It serves as the foundation on which all your email automation tasks will be built. A properly set environment ensures that all necessary libraries function seamlessly, and minimizes common issues that may arise during development.
Having the right tools can greatly speed up the development process and improve overall code performance. Moreover, understanding how to configure your environment correctly helps in troubleshooting errors later on. Without this preparation, one may find themselves tangled in problems that could have been avoided.
Required Libraries for Email Functionality
To send emails using Python, you need specific libraries that provide the necessary functionality. The two primary libraries you should focus on are smtplib and email.mime. Each of these libraries offers unique capabilities that are essential for composing and sending emails.
smtplib
smtplib is a core library in Python that allows you to connect to an SMTP server, which is essential for sending emails. The primary characteristic of smtplib is its simplicity and ease of use. It facilitates the process of establishing a connection with an email server, authenticating with it, and sending messages through the server.
One unique feature of smtplib is its support for both secure and unsecure connections, allowing for flexibility based on different server requirements. Additionally, it’s popular among developers for its straightforward interface. The advantages of using smtplib include a seamless integration with Python applications, which makes sending emails straightforward. However, its major disadvantage is that it does not handle complex email formatting directly. For those needs, it is often used in conjunction with the email.mime library.
email.mime
The library email.mime is part of the larger email package in Python, specifically designed for creating and handling email messages. A key characteristic of email.mime is its capability to build messages that can include attachments, rich content, and various media types. This makes it a preferred choice when dealing with multi-part emails.
One unique feature of email.mime is its ability to create MIME (Multipurpose Internet Mail Extensions) types, which are pivotal in distinguishing between plain text emails and those with HTML format. Its advantages include greater flexibility in email content presentation and support for attachments. However, its complexity can be a drawback for novices who might find it overwhelming initially.
Installing Necessary Packages
To start using these libraries, ensure that you have Python already installed in your system. Most Python distributions come with smtplib and email.mime included. However, in case these packages are missing for any reason, using pip to install or update them may be necessary. This is done using the following command in your terminal or command prompt:
Always verify the version of Python in use, as compatibility may vary with different library versions. This proactive approach can prevent inconsistencies and runtime errors during email sending procedures.
Creating a Simple Email Sender
Creating a simple email sender using Python serves as a foundational skill for those interested in automating communication processes. This section emphasizes the significance of constructing an effective email sending script. It allows developers to initiate email transmission for notifications, alerts, and updates with ease. Learning how to send basic emails prepares you for more complex functionalities, including sending HTML emails and handling attachments.
The process can be straightforward, yet it provides critical insight into setting up communication through code. Understanding how to send emails is not just about writing scripts; it's about empowering potential applications in your project. Here’s how to go about it:
Basic Code Structure for Sending Emails
The basic code structure for sending emails can be simplified into several steps. You start by importing the necessary libraries, which will handle the email protocols. The following code snippet outlines the essential components needed for basic email sending:
This structure encompasses the importing of libraries, preparing the email content, and actually sending the email. Ensuring that each step is correctly formatted and executed is vital for successful email transmission.
Sending Text Emails
Text emails are the simplest form of electronic communication. They include basic content without advanced formatting. With Python, sending text emails can be accomplished using the and classes as illustrated earlier. The body of the email can be created as plain text, making it straightforward and suitable for many general use cases. Here’s how you accomplish this:
- Define the sender and receiver email addresses.
- Add a subject line that summarizes the content.
- Write the plain text email body.
This approach stresses clarity in communication, as the recipient gets straightforward information without distraction.
Sending HTML Emails
HTML emails enable richer formatting and the ability to include images, linking, and more complex layouts. Sending HTML emails in Python is also simple but requires a shift in how the email body is defined. Here’s how to achieve this:
- Prepare the HTML content using standard HTML tags, ensuring all necessary elements are included.
- Modify the to indicate the content as HTML instead of plain text.
Here’s an example snippet
Utilizing HTML in emails allows for a visually appealing presentation, making the communication more engaging for the recipient.
Handling Attachments
Handling attachments in emails is a crucial aspect of email communication, especially when using Python3 for automation. This feature allows users to send files such as documents, images, or presentations directly. In many professional settings, attachments are necessary for conveying information effectively. They add a layer of detail that plain text cannot achieve. Understanding how to manage email attachments can greatly enhance the functionality of your email application.
Basics of Email Attachments
To begin, it is important to know what email attachments are. An email attachment is a file sent along with email messages. These attachments can be of various formats, from PDFs to images. They provide additional context or information, often essential for the email’s purpose. However, handling attachments requires careful consideration of several factors:
- Size Limits: Most email services impose size limits on attachments. Knowing these limits ensures that your emails do not fail to send due to oversized files.
- File Type Restrictions: Some email providers restrict certain file types for security reasons. It is essential to know what types are allowed.
- Security Risks: Sending sensitive information as an attachment can pose security risks. Ensuring the attachment's safety through encryption or secure channels is advisable.
"Attachments enrich your communication, but they must be handled with caution due to security and compatibility concerns."
Including Files in Emails
Once you understand the basics, the next step is including files in your emails using Python. The library in Python3 facilitates this process. The following code snippet illustrates how to add an attachment to an email:
In this code, we create a multipart email message and attach a PDF file to it. It is important to ensure that the file is opened in binary mode to prevent data corruption.
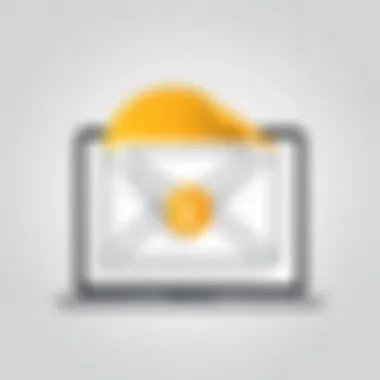
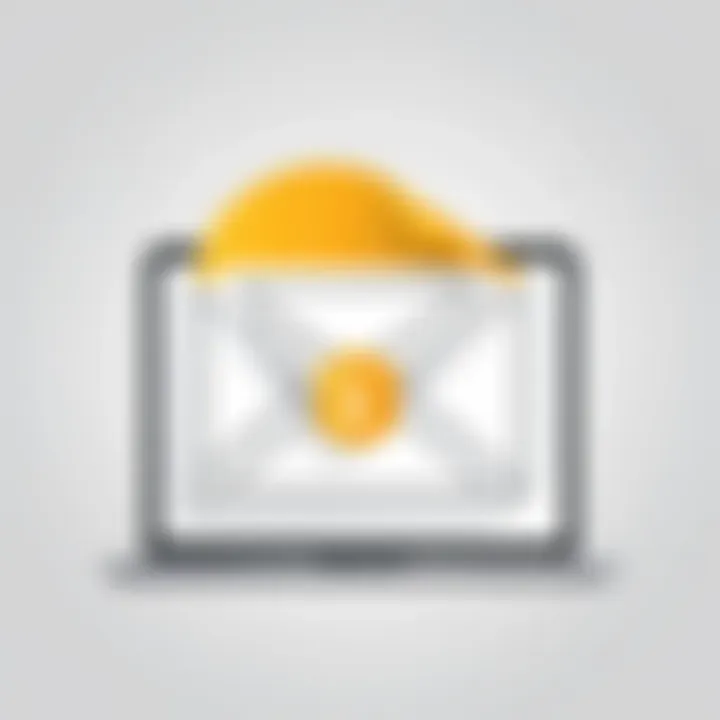
Handling attachments correctly allows you to send numerous formats, enhancing communication efficiency. In the following sections, we will delve deeper into more advanced techniques and strategies for optimizing email sending in Python.
Configuring SMTP Servers
Configuring SMTP servers is a fundamental aspect of email automation in Python. It enables your Python scripts to effectively send out emails using the Simple Mail Transfer Protocol (SMTP), which standardizes email sending over the internet. The importance of proper configuration cannot be overstated, as it directly impacts the reliability and security of your email communication.
Several elements must be considered when configuring SMTP servers. First, the choice of SMTP server plays a crucial role in how effectively your emails are sent and received. Each server may have different limitations regarding the number of emails that can be sent per hour, the types of authentication required, and the protocols supported. This requires thorough research and possibly testing multiple options before deciding on the best server for your needs.
Additionally, you must consider the security features of the SMTP server you select. An insecure SMTP configuration can expose your email content and expose sensitive information. This is why many programmers prefer reputable providers that offer secure transmission methods, such as Transport Layer Security (TLS).
Choosing the Right SMTP Server
Choosing the right SMTP server is critical for achieving successful email delivery. The choice can depend on several factors, including your specific requirements for sending emails, pricing, and ease of integration with Python libraries.
Some popular options for SMTP servers include Gmail, SendGrid, and Amazon SES. Each has unique features and limitations. For instance:
- Gmail: Good for personal use and integration with Google services. However, it has sending limits that might not suit larger applications.
- SendGrid: Offers high volumes and analytics, making it great for marketing emails but may incur costs.
- Amazon SES: Highly scalable and cost-effective for those already using Amazon Web Services.
When selecting an SMTP provider, consider these questions:
- What is the expected volume of emails?
- Do you need features like tracking or analytics?
- What is your budget for email sending services?
Authentication Methods
Authentication is a vital part of configuring your SMTP server. It ensures that only authorized users can send emails through the server, helping to prevent spam and other security issues. Different servers offer various authentication methods that you should be aware of.
The most common methods include:
- Basic Authentication: Involves sending a username and password in the email header. Though simple, it is not the most secure method unless used with SSL/TLS.
- OAut: A more secure method where users grant permission through a token system, commonly used by platforms like Google and Microsoft.
- API-based Authentication: Some services offer APIs for sending emails, which may use tokens instead of conventional username/password methods.
When configuring your SMTP settings, make sure you follow the documentation provided by your SMTP server. Each method has specific requirements that must be followed to ensure successful email delivery. When done right, proper configuration will enhance the functionality of your email-sending applications.
Implementing Security Measures
When automating email communication with Python, addressing security becomes essential. In a landscape fraught with cyber threats, ensuring that emails are sent securely protects sensitive information and maintains the integrity of the communication. This section covers why email security matters and how one can implement various security measures.
Importance of Email Security
Email security is crucial for several reasons. First, email remains a common vector for cyber attacks, making it a target for cybercriminals. Malware, phishing attacks, and data breaches can arise from insecure email transmission. Once an attacker gains access to a user's email account, they can misuse personal data and confidential information. Therefore, investing in email security safeguards not only personal data but also builds trust with recipients.
Secondly, regulatory requirements often mandate strict compliance with data protection standards. For instance, laws like General Data Protection Regulation (GDPR) require organizations to implement security measures to protect personal information. Failure to comply can lead to severe penalties or reputational damage.
Lastly, a secure email system enhances the credibility of communications. Recipients are more likely to engage with emails that they trust. If emails are consistently sent with security measures in place, it fosters confidence and improves overall response rates.
Using TLS for Secure Email Transmission
Transport Layer Security (TLS) is a cryptographic protocol that enhances the security of data sent over networks, including emails. Using TLS in email transmission ensures that data is encrypted, protecting it as it travels from sender to recipient.
Implementing TLS is often straightforward with libraries like smtplib in Python. When configuring the SMTP server, establish a secure connection by enabling TLS. This is done by calling the method on the SMTP object before sending an email. Here is an example code snippet:
Employing TLS ensures that the connection between the server and client is secure. This means that even if the data is intercepted, it will be unreadable to unauthorized parties. Additionally, TLS can help avoid man-in-the-middle attacks, where an attacker could potentially hijack communication between the sender and receiver.
In sum, understanding the significance of security measures in email sending using Python is vital. By implementing TLS and being aware of email security best practices, individuals can protect sensitive information and communicate with greater confidence.
Advanced Email Features
Advanced email features enhance the basic functionality of email communication by introducing capabilities that improve user experience and efficiency. In the context of sending emails through Python3, these features not only enable robust communication strategies but also save time and increase productivity for users. Understanding these elements is essential for anyone looking to build sophisticated email solutions.
Sending Bulk Emails
Sending bulk emails involves distributing a single message to a large group of recipients. This practice is widely used in marketing campaigns, newsletters, and announcements. By utilizing Python and the right libraries, one can automate the process, which greatly facilitates outreach efforts.
Benefits of Bulk Email Sending
- Efficiency: Sending one email to many recipients is significantly faster than composing individual messages.
- Cost-Effective: Reduces the costs associated with traditional mailing systems.
- Scalability: Easily adjust the size of your email list without changing your method of communication.
Key Considerations
- Compliance: Ensure your bulk emails comply with regulations like the CAN-SPAM Act. This includes providing unsubscribe options.
- Spam Filters: Craft email content and structure thoughtfully to minimize the risk of being flagged as spam.
- Personalization: Personalizing elements in bulk emails increases engagement rates.
Personalizing Email Content
Personalizing email content involves tailoring messages to suit individual recipients. This strategy can significantly enhance engagement and responses from readers.
Importance of Personalization
- Higher Engagement Rates: Personalized emails tend to have higher open and click-through rates, leading to better outcomes.
- Improved Relationships: Addressing recipients by name and acknowledging their preferences fosters a sense of connection.
- Targeted Messaging: Specific content based on user behavior or interests improves relevancy, making the communication feel more relevant to the recipient's needs.
Tips for Personalizing Emails
- Use Merge Tags: Employ placeholders for names and other personal details.
- Segment Your Audience: Group your contacts based on attributes like location, purchase history, or interests.
- Dynamic Content: Utilize dynamic fields to customize portions of your email based on recipient data.
By incorporating advanced features such as sending bulk emails and personalizing content, users can significantly elevate their email communication strategies. These practices promote better engagement and enhance overall effectiveness in any email campaign.
Common Errors and Troubleshooting
Understanding common errors and troubleshooting methods is crucial when working with email sending in Python. Problems may arise due to various factors like incorrect configurations, connectivity issues, or authentication failures. Knowing how to pinpoint these errors saves time and enhances the overall email automation process. This section offers insights into identifying and resolving typical SMTP errors as well as general troubleshooting techniques that programmers can rely on to streamline their work.
Identifying Common SMTP Errors
When sending emails using Python, several errors can emerge. Identifying these SMTP errors is the first step towards resolving them. Here are some common SMTP errors programmers might encounter:
- SMTP Authentication Errors: These occur when the email server rejects the login credentials provided. Ensure that the username and password are correct and that specified settings like two-factor authentication are correctly configured.
- Connection Timeout: This happens when your script cannot connect to the SMTP server within the expected time period. It could be due to network issues or incorrect SMTP server details.
- Relay Denied: If the server does not allow the sending of emails to recipients that are not affiliated with it, a relay error will occur. This often requires that you either authenticate your session or use the correct sender address.
- SMTP Server Not Found: This points to a misconfiguration in server settings or the server being down. Double-check the server address for typographical errors.
To assist in identifying these errors, examining the output of the Python script, particularly error messages, can provide clues. Implement try-except blocks in your code to catch and analyze exceptions effectively.
Debugging Email Sending Issues
Debugging email sending issues is a skill that can significantly improve your efficiency. When emails fail to send, follow a methodical approach to troubleshoot. Here are several steps to consider:
- Examine Your Code: Review your Python code for any syntax errors or logical mistakes. Ensure that your SMTP setup and message creation are correct.
- Check SMTP Server Status: Visit the SMTP provider’s website or service status page to confirm if there are any outages.
- Verify Network Connectivity: Ensure that your local machine has access to the internet and is not being blocked by a firewall. Sometimes corporate networks impose restrictions on email sending.
- Test with Basic Scripts: Simplify your code to the basics required to send an email. This eliminates complexities that may hide the source of the problem. A basic script could look like this:
- Enable Debugging for the SMTP Object: Use the method on the SMTP object to see real-time connection information and errors during the sending process. Example:
Following these steps can help resolve most issues with email sending in Python. Remember that methodical diagnosis often leads to swift resolutions.
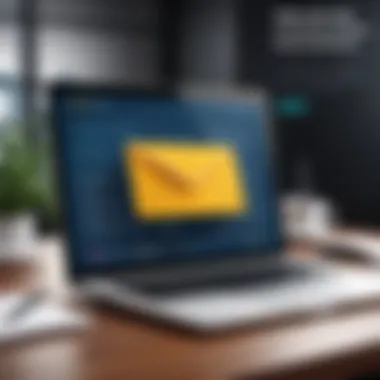
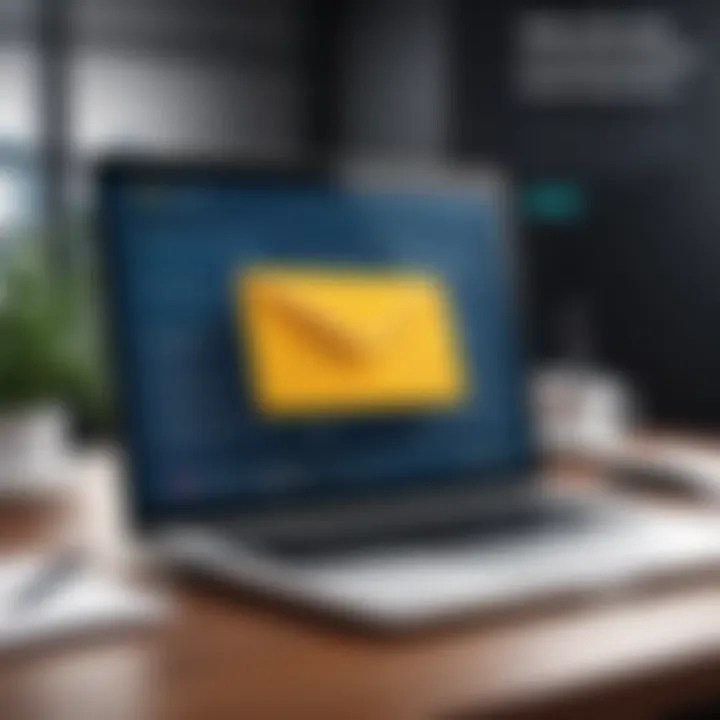
Best Practices for Email Communication
In the realm of email communication, especially when utilizing Python for automation, adhering to best practices is crucial. Effective email communication can enhance clarity, foster engagement, and help to achieve the desired response from recipients. Emphasizing the importance of this practice ensures your messages are not just received but also acted upon.
Best practices encompass a variety of elements, from writing to formatting and timing. Each of these components contributes to how your message is perceived. Focusing on clear and concise communication can significantly reduce misunderstandings. Ensuring your emails are tailored to their audience can drive better interactions.
Writing Effective Subject Lines
The subject line plays an integral role in email communication. It serves as the first impression and the gateway to engagement. A well-crafted subject line can improve open rates and encourage readers to take action.
- Be Brief: Aim for subject lines that summarize the content in a few words. Typically, a length of 6 to 10 words is effective.
- Use Action Words: Start with action-oriented verbs. This can stir curiosity and encourage immediate action. For instance, "Join our upcoming Python workshop!" clearly conveys what to expect.
- Avoid Spam Triggers: Certain phrases like "Free" or excessive punctuation can categorize your email as spam. General awareness of these triggers can improve deliverability.
- Personalize When Possible: Personalization enhances engagement. Inserting the recipient's name or relevant interests can create a more inviting tone.
- Ensure Relevance: Ensure that the subject line is relevant to the content of the email. Misleading subject lines can lead to increased unsubscribe rates.
"A great subject line invites intrigue and sets the tone for the entire communication."
Crafting Clear Email Body Content
After the subject line, the body of the email carries the bulk of the message’s weight. Clarity in this section is essential for effective communication.
- Use Short Paragraphs: Break down information into digestible parts. Short paragraphs can prevent reader fatigue and ensure your points are impactful.
- Utilize Bulleted or Numbered Lists: Lists can enhance readability. They allow for quick scanning, making it easier for recipients to grasp important information.
- Be Direct: State your main point early. Readers often skim emails, so presenting crucial information upfront ensures it isn’t missed.
- Proofread for Errors: Spelling or grammatical errors can undermine your professionalism. A quick review can help maintain credibility.
- Include a Call to Action: End with a clear next step. Whether you seek a reply, a click, or another action, clearly stating your request can lead to favorable outcomes.
Incorporating these principles into your Python email automation project will not only enhance the communication experience but also serve to build trust and foster relationships. Maximizing email effectiveness through these best practices can lead to higher engagement levels and more efficient communication.
Real-World Applications of Python Email Sending
In today's digital landscape, the ability to send emails programmatically is vital for countless applications. Using Python for email automation offers significant advantages that are essential for various professional fields. From startups to large enterprises, emails are an integral part of communication, making the Python email sending capability not just useful but sometimes necessary.
One primary benefit of using Python for email sending is efficiency. Automating the process of sending emails can save time and reduce human error. For example, instead of manually informing a list of subscribers about updates, one can write a program that automatically sends out personalized emails. This not only frees up time but also ensures that the information reaches the intended audience promptly.
Moreover, Python's versatile libraries facilitate integration with existing systems, allowing businesses to streamline their operations. The possibilities stretch from simple personal use to complex corporate solutions, enabling tailored responses or mass communications according to specific criteria. Leveraging this capability effectively can enhance customer relationships, boost engagement, and ultimately lead to better outcomes for businesses.
Integration with Other Python Applications
Integrating email functionality into other Python applications can significantly amplify their usability. By connecting Python's email capabilities with tools or frameworks, developers can create more comprehensive solutions that deliver high value.
For instance, a web application that collects user feedback can employ Python to send out thank-you emails automatically. The process could look like this:
- User submits feedback on a web form.
- Python application captures this feedback and triggers an email function.
- The system sends a personalized thank-you email to the user, acknowledging their input.
This seamless integration not only improves the user experience but also fosters a positive relationship. Furthermore, developers can build applications that monitor specific events and notify users via email as soon as those events occur. For instance, an e-commerce platform may notify customers when their orders are shipped, enhancing customer satisfaction.
Automated Notification Systems
Automated notification systems are withouth a doubt a significant application for Python email sending. Organizations often need to deliver timely information to various stakeholders, whether it’s about project updates, alerts, or reminders. Such notifications ensure that everyone remains informed and can act promptly when necessary.
By establishing an automated email notification system, businesses benefit from consistent communication. For example, in a project management tool, users can receive automatic notifications about task deadlines or changes in project status. This setup can be implemented easily using Python with libraries like smtplib and email.mime.
- Step 1: Define the events that require notification.
- Step 2: Use Python to create scripts that monitor these events.
- Step 3: Configure the scripts to send out email updates, using predefined templates for consistency.
Such systems not only save time but also reduce the cognitive load on teams tasked with updating stakeholders. Ensuring that everyone is in the loop cultivates better teamwork and supports project success.
Future Trends in Email Automation with Python
The landscape of email automation is evolving rapidly, with Python emerging as a key player in this domain. Understanding the future trends in email automation with Python is crucial for developers and organizations looking to enhance their digital communication strategies. The integration of innovative technologies not only improves efficiency but also addresses the increasing demands for personalization and security in email communication.
As we look forward, several specific elements warrant attention. First, there is a growing emphasis on integrating automation with other technologies. This trend facilitates the development of more robust email solutions, allowing for seamless connections between email applications and databases or CRMs.
Furthermore, the rise of APIs enables developers to create custom applications that enhance the functionality of existing email services. By leveraging these APIs, Python can automate processes that were previously manual, drastically reducing the time and effort involved.
Another important consideration is the increasing awareness of data privacy. With regulations like GDPR in place, email automation must prioritize user consent and data protection, influencing how developers approach automation solutions.
Emerging Technologies Shaping Email Automation
Email automation is significantly impacted by various emerging technologies. One of the most notable is the integration of cloud computing. This technology allows organizations to manage infrastructure and storage needs efficiently. By using cloud platforms, developers can offer scalable email solutions that adapt to the volume of email traffic.
Additionally, blockchain technology has started making its mark in securing email transactions. By utilizing a decentralized approach, blockchain ensures that only authorized entities can access sensitive information within emails, thus enhancing security.
Machine learning is also acquiring relevance in the realm of email automation. Through algorithms and predictive analytics, systems can analyze user behavior to optimize email targeting, sending times, and content personalization.
The Role of Machine Learning in Email Management
Machine learning plays a critical role in revolutionizing email management systems. It enables systems to analyze vast amounts of data to improve email deliverability and engagement rates. For instance, algorithms can predict which subject lines yield higher open rates based on historical data.
Moreover, machine learning can help identify spam patterns, enhancing filters that protect users from unwanted emails. This not only improves user experience but also contributes to maintaining a clean inbox.
The personalization aspect is also enhanced through machine learning. Systems can learn from user interactions, suggesting tailored content that aligns with user preferences. This leads to higher engagement rates and more successful campaigns.
Ending
In this article, we have explored various facets involved in sending emails using Python3. Understanding how to automate email communication is crucial for anyone looking to enhance their software development skills. Automation streamlines many tasks, saving time and reducing human error. This guide serves multiple purposes, from providing foundational knowledge to offering insights into advanced features.
One of the most significant aspects of this tutorial is the emphasis on security. As email becomes a primary communication tool, ensuring secure transmission is a vital consideration. By implementing practices such as using TLS, you not only protect your data but also build trust with the users you communicate with. Moreover, understanding SMTP protocols deepens your comprehension of how email travels across networks, enriching your programming skills.
The discussion on best practices for email communication highlights the need for clarity and effectiveness in message delivery. The methods shared are not just technical; they encourage thoughtful crafting of emails that respect the recipient’s time and attention. This is invaluable in both personal and professional settings.
By integrating these guidelines into your email-sending practices, you make sure that you are not only technologically equipped but also strategically positioned to communicate efficiently. This balance of technical skill and effective communication is what sets apart great programmers from good ones.
"In the realm of programming, mastering not just the code but the communication that follows it distinguishes a true professional."
Thus, as you continue your journey with Python, keep these best practices and insights in mind. The world of email automation holds endless possibilities for enhancing productivity and connection.
Recap of Key Points
- Email Automation Importance: Streamlines tasks and improves efficiency.
- Security Measures: Essential for protecting sensitive information during email transmission.
- SMTP Understanding: Provides insight into how emails are sent and received.
- Best Practices: Ensures clarity and effectiveness in communication, respecting your audience.
- Continuous Learning: Always be open to absorb new trends in email automation and adapt accordingly.
Further Reading and Resources
In the realm of programming, continual learning is essential. This guide introduces various methods to send emails using Python3, but to truly master the concepts, further reading is vital. Engaging with additional literature and resources allows individuals to build a deeper understanding and tune their skills effectively.
Recommended Books and Guides
Engaging with books dedicated to Python programming can greatly improve one’s grasp of sending emails through the language. Here are a few notable recommendations:
- "Automate the Boring Stuff with Python" by Al Sweigart: This book covers practical aspects of Python programming, including automating tasks like email sending. It’s particularly helpful for beginners eager to implement the knowledge immediately.
- "Python for Data Analysis" by Wes McKinney: While focused on data analysis, this book discusses library usage that can also apply to email operations - especially useful in automating the report generation and emailing process.
- "Learning Python" by Mark Lutz: An essential guide for comprehensive understanding, it lays a robust foundation in Python, which is necessary for grasping email automation.
Books are thorough and structured resources that serve as references as readers apply concepts learned in practical scenarios.
Online Courses and Tutorials
Online courses offer interactive learning experiences. They often include practical exercises, discussions, and community support. Here’s a list of recommended online platforms:
- Coursera: Offers courses like “Python for Everybody” which covers the use of libraries for email automation and other practical usages.
- Udemy: This platform features several Python courses focusing specifically on automating tasks, including sending emails.
- Codecademy: Provides hands-on exercises that may include email functionalities. While not exclusively focused on email, it helps build foundational skills needed for effective programming.
These resources give learners the opportunity to engage with content at their own pace, deepening their understanding and application of email automation techniques. Furthermore, many tutorials available on platforms like Reddit and various blog sites amplify learning with community discussions and real-life examples.
Remember, systematic practice and ongoing learning are key components for success in Python programming, especially when dealing with applications like email automation.
By leveraging these resources, readers can enhance their skills and navigate the complexities of email communication effectively.