Securing REST APIs with Spring Boot: A Practical Guide
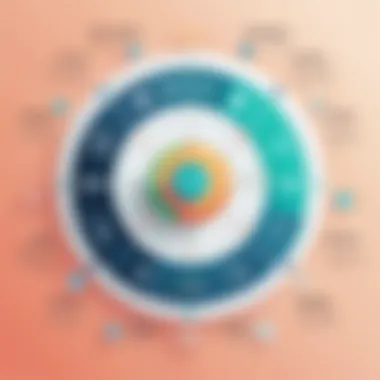
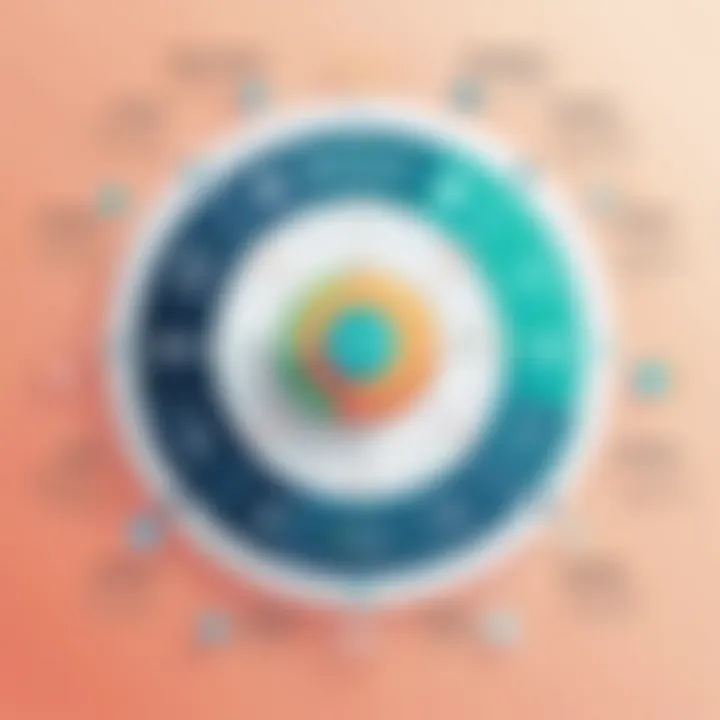
Intro
Securing REST APIs in Spring Boot is crucial in today’s digital landscape. With increasing reliance on web services, developers must safeguard their applications from various threats. This article aims to provide a detailed examination of effective methods for securing REST APIs built with Spring Boot. The focus will be on practical implementations, best practices, and actionable examples that can enhance your skill set in API security.
Importance of API Security
APIs serve as the backbone of modern software architecture. They facilitate communication between different applications and services. However, if not secured properly, they can be vulnerable to attacks like data breaches and unauthorized access. By securing your APIs, you protect sensitive data and maintain the integrity of your applications. In this article, we will delve into essential concepts, such as authentication and authorization, and explore techniques to mitigate common vulnerabilities.
Foreword to Spring Boot REST API Security
As we plunge into the realm of modern application development, the emphasis on security is more pronounced than ever. Spring Boot offers a robust framework popular for building RESTful APIs. However, with popularity comes a surge in security threats. Understanding how to secure these APIs is essential not only for developers but also for organizations that rely on them.
Securing a REST API involves protecting it from various vulnerabilities. The rise in cyber attacks underscores the importance of having strong security measures in place. When data breaches occur, the consequences can be detrimental. Beyond the immediate financial impact, there is reputational damage and loss of customer trust.
Spring Boot provides tools to facilitate API security through comprehensive features. These include built-in support for authentication and authorization. With these features, developers can implement security measures that are both effective and efficient.
In this article, we will discuss the fundamental concepts of Spring Boot REST API security. We will delve into authentication mechanisms, role-based access control, and the importance of protecting sensitive data. Moreover, we will highlight practical implementations of these concepts using real-world scenarios.
Key Benefits of Securing REST APIs in Spring Boot
- Data Protection: Guarding data from unauthorized access protects the integrity of the information.
- Compliance: Many industries are governed by strict regulations regarding the handling of data. Adhering to these regulations is crucial for avoiding penalties.
- Trust Building: Users are more likely to engage with a platform they perceive as secure.
- Scalability: A secure application can grow without compromising user data or system integrity.
Understanding REST APIs
Understanding REST APIs is crucial when delving into security measures within a Spring Boot application. REST, which stands for Representational State Transfer, places stringent guidelines on how resources should be defined and addressed. It is an architectural style that allows different software agents, often including mobile applications and web browsers, to communicate via the HTTP protocol.
Definition of REST
REST is fundamentally about stateless interactions. Each request from a client must contain all the necessary information for the server to understand and process it, ensuring sessions are not maintained by the server. This approach promotes scalability and decentralization.
Clients interact with REST APIs primarily over HTTP, and they make use of various HTTP methods like GET, POST, PUT, and DELETE to perform different operations on resources. The resources in a REST API are typically represented in JSON or XML format.
If you're looking to understand REST better, Wikipedia provides a good overview of its principles at Wikipedia REST.
Principles of RESTful APIs
RESTful APIs are built on several guiding principles. Here are some key ones to consider:
- Statelessness: Each API call is independent. The server does not store any client context from previous requests.
- Client-Server Architecture: This principle separates the user interface from data storage, allowing for a more flexible development cycle.
- Cacheability: Responses should specify whether they can be cached. Correct use of caching can reduce server load and improve performance.
- Layered System: An API can be composed of various layers, each fulfilling different roles. A client may not know if it's connected directly to the end server or an intermediary.
- Uniform Interface: This constraint simplifies and decouples the architecture. It allows different clients to communicate with various services in a structured way.
Understanding these principles not only aids in developing RESTful APIs but also lays a foundation for implementing sound security practices. As REST APIs are often exposed to the internet, understanding their structure helps identify potential security threats.
In summary, mastering these concepts facilitates a greater understanding of how to secure REST APIs effectively when securing a Spring Boot application.
Overview of Spring Boot
Spring Boot has become a popular choice for developers when creating web applications with Java, especially RESTful APIs. This section will explore the significance of Spring Boot regarding REST API security, emphasizing its features, advantages, and practical implications. Understanding Spring Boot is essential as it streamlines application development, allowing developers to focus on security measures without getting bogged down in configuration details.
With Spring Boot, developers can utilize a variety of built-in functionalities that enhance the security of their applications. The framework simplifies security configurations, making it easier to integrate important practices like authentication and authorization. By using Spring Boot, developers can set up a secure environment quickly while maintaining best practices in API design. This helps in protecting sensitive data and improving the overall robustness of the application.
Key Features of Spring Boot
- Auto Configuration: Spring Boot automatically configures applications based on the libraries found on the classpath. This capability enables developers to reduce the amount of boilerplate code and configure security frameworks more efficiently.
- Standalone Applications: Spring Boot allows developers to create stand-alone applications that can run without external web servers. This increases flexibility and eases deployment since there is no need for complex server installation processes.
- Embedded Servers: By offering embedded servers like Tomcat or Jetty, Spring Boot allows developers to run applications directly within the development environment. This feature simplifies testing and enhances security since the development and production environment can remain consistent.
- Production Ready: Spring Boot comes with a set of tools that provide metrics, health checks, and various monitoring capabilities. This ensures that applications remain robust and can be managed effectively in production settings.
- Spring Security Integration: Spring Boot seamlessly integrates with Spring Security, providing developers with comprehensive options for securing REST APIs. This integration forms the backbone for implementing authentication and authorization mechanisms.
Setting Up a Spring Boot Project
Setting up a Spring Boot project is straightforward, making it accessible even for novice developers. Here’s a simple step-by-step process to kickstart your Spring Boot application:
- Use Spring Initializr: Start by visiting the Spring Initializr website. This tool helps generate a basic Spring Boot project configuration. You can select project metadata like the project name, packaging type, and preferred Java version.
- Add Dependencies: In the Initializr, you can include necessary dependencies such as Spring Web, Spring Security, and any database connectors that you might need for your application. Choosing the right dependencies is crucial for setting up security features.
- Download the Project: After configuring your project, download the zip file. Extract it to your working directory where you can begin making changes to the application.
- Import into Integrated Development Environment (IDE): Use tools like IntelliJ IDEA or Eclipse to import your project. This IDE integration will ease your coding experience and provide syntax highlighting and autocompletion features.
- Run the Application: With the project set up, you can now start the application. Spring Boot simplifies this with the command if you are using Maven or for Gradle projects. This command will launch the embedded server, and your REST API will be ready for testing.
Following these steps, developers can create a solid foundation for their RESTful API while ensuring adequate security configurations are implemented from the beginning.
Security Challenges in API Development
In the increasingly interconnected digital landscape, securing APIs becomes paramount. Developers face a myriad of challenges that can impact the safety and performance of their applications. This section delves into the critical security challenges in API development, emphasizing the need for a robust security framework.
One key aspect of API security is the potential exposure to various vulnerabilities. Threats range from unauthorized access to data breaches, which can severely undermine user trust. APIs, serving as gateways to sensitive data and functionalities, are prime targets for attackers. A breach in an API can lead to unauthorized access to backend systems and sensitive information. Hence, understanding these vulnerabilities is crucial for developers.
Furthermore, the complexity of APIs and their interactions with multiple systems adds another layer of difficulty in securing them. Each endpoint within an API can become a point of vulnerability if not properly managed. Consequently, a comprehensive security strategy must encompass not only authentication and authorization but also continuous monitoring and updating of the security measures.
By recognizing these security challenges, developers can prioritize their efforts to establish strong defenses against potential threats. This proactive approach not only protects their applications but also fosters user confidence in their products.
Common Threats to APIs
API security threats come in various forms, each requiring distinct defense strategies. Some of the most common threats include:
- Injection Attacks: Attackers inject malicious code through vulnerable input fields, often exploiting SQL or XML parsers. This can lead to unauthorized data access or modification.
- Cross-Site Scripting (XSS): This attack allows hackers to inject malicious scripts into web applications, potentially compromising user data.
- Distributed Denial of Service (DDoS): Attackers overwhelm an API with excessive requests, causing it to crash or perform poorly. This disruption can render services unavailable.
- Broken Authentication: Poor implementation of authentication mechanisms can lead to user impersonation and unauthorized access.
- Insecure Direct Object References: If proper access controls are not enforced, users might access sensitive data or functionalities they shouldn't have access to.
Each of these threats underscores the need for rigorous API security practices. Effective measures can mitigate these risks, ensuring data integrity and user safety.
Importance of Securing APIs
Securing APIs is not just a technical consideration; it is essential for maintaining trust and reputation in an organization. With the increasing reliance on APIs for third-party integrations and mobile applications, safeguarding these interfaces has become critical. Here are several reasons why API security is vital:
- Protecting Sensitive Data: APIs often handle confidential information. Securing them prevents data leaks and unauthorized access, enhancing customer trust.
- Regulatory Compliance: Many regulations, such as GDPR or HIPAA, require organizations to protect user data. Failure to secure APIs can lead to significant legal penalties and reputational damage.
- Maintaining Service Availability: An unsecured API risks being targeted for attacks, potentially leading to downtime. Ensuring robustness through security can prevent service disruptions.
- Enabling Secure Integrations: As businesses adopt cloud services and partner with third-party providers, secure APIs allow seamless and safe integrations.
Foundational Security Concepts
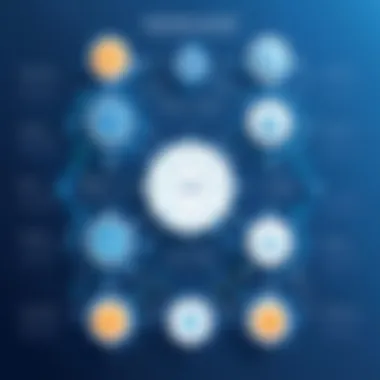
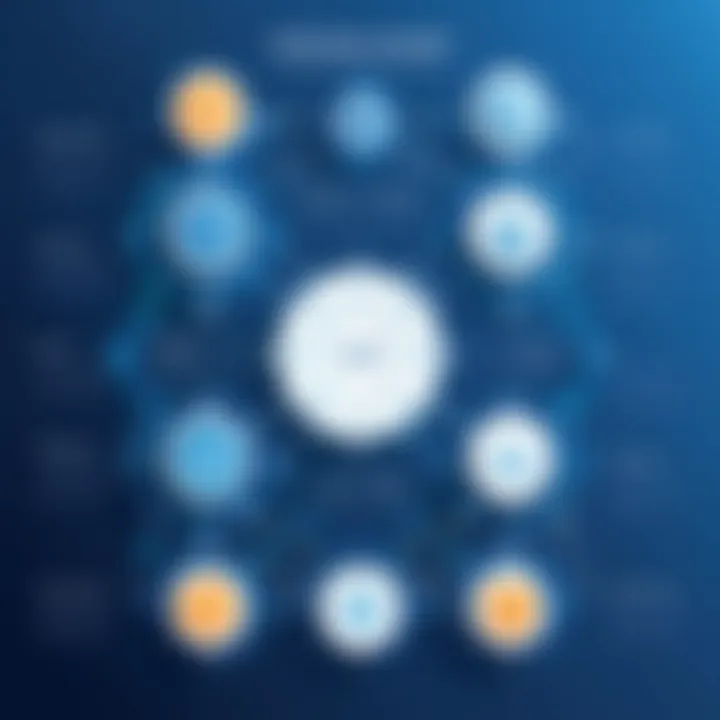
Understanding foundational security concepts is crucial for anyone involved in developing secure REST APIs. These concepts serve as the bedrock for building a security framework for your application. A robust security framework ensures sensitive data remains protected while also defining how users interact with the application.
Authentication vs Authorization
Authentication and authorization are two critical components that must not be conflated. They play distinct roles in the security landscape.
- Authentication is the process of verifying the identity of a user. This is typically done through username and password combinations but can also involve more advanced mechanisms like biometric data or multi-factor authentication. In essence, it answers the question: "Who are you?"
- Authorization, on the other hand, occurs after authentication. This step determines which resources a user can access and what actions they are permitted to take. It addresses the question: "What are you allowed to do?"
When developing secure APIs, it is vital to handle both concepts correctly. An API might authenticate a user successfully but still allow them access to unauthorized data if not managed well. Therefore, understanding these concepts deeply allows developers to implement tailored security policies that protect API resources effectively.
Role-Based Access Control (RBAC)
Role-Based Access Control is a powerful strategy for managing user permissions. With RBAC, users are assigned roles that dictate their access levels within the system. This simplifies security management significantly by aggregating permissions into roles rather than administering them individually for each user.
The benefits of RBAC include:
- Simplicity: Managing user rights becomes easier as administrators can adjust permissions by changing the roles rather than updating each user individually.
- Scalability: As organizations grow, their API security needs become more complex. RBAC allows for smooth scaling without overwhelming administrative procedures.
- Compliance: Many regulations demand that access to sensitive data is strictly controlled. RBAC enables companies to demonstrate adherence to these compliance requirements by maintaining clear access controls.
Implementing RBAC in a Spring Boot application can be done through integration with Spring Security, which makes it easier to manage user roles and permissions seamlessly.
Understanding foundational concepts sets the groundwork for a secure API. Prioritizing both authentication and authorization is critical in reducing vulnerabilities and protecting sensitive data.
Implementing Security in Spring Boot
Implementing security in a Spring Boot application is a critical aspect of API development. As REST APIs serve as the backbone of modern web and mobile applications, ensuring their security cannot be understated. Security is not merely an addition; it is a fundamental necessity. It protects sensitive data, safeguards user information, and prevents unauthorized access, thus maintaining the integrity of the application.
Using Spring Security
Spring Security is a powerful and customizable authentication and access control framework for Java applications, particularly those built using Spring Boot. By leveraging this framework, developers can easily secure their applications with minimal effort. The integration of Spring Security provides several benefits:
- Robust Authentication Options: Whether you prefer basic authentication, form-based login, or token-based systems like JSON Web Tokens (JWT), Spring Security has options that can fulfill these needs.
- Access Control: You can authorize users based on roles and permissions, ensuring that only authenticated users can access protected resources. This is crucial for controlling what users can do within the application.
- Comprehensive Documentation: Extensive resources and community support make it easier for developers to implement security configurations effectively.
To implement Spring Security, you need to add the relevant dependencies in your or files. This can typically be done with:
After adding the dependencies, Spring Security automatically configures default security settings. However, for finer control, custom configurations are often necessary.
Configuring Security in your Application
Configuring security in a Spring Boot application involves creating a security configuration class that extends . This allows developers to override default behavior and define custom security rules for their applications. Here is a simplified example of how to configure basic HTTP security:
This configuration ensures that any request to the path is accessible without authentication, while all other requests require the user to be authenticated. Developers can customize these configurations further according to their specific needs.
Overall, security configuration is essential in keeping APIs secure and preventing unauthorized access. Properly implemented security measures help in building trust and reliability in web applications.
Authentication Mechanisms
Understanding authentication mechanisms is pivotal for safeguarding REST APIs in Spring Boot. Authentication serves as the first line of defense against unauthorized access. Failing to implement effective authentication can lead to data breaches, misuse of services, and loss of sensitive information. Therefore, a robust authentication mechanism is essential not only for securing the API but also for building trust with users.
Two prominent authentication mechanisms are Basic Authentication and JSON Web Tokens (JWT). Each method has unique characteristics that suit different scenarios and requirements. It is crucial to assess your application's needs before choosing the appropriate mechanism.
Basic Authentication
Basic Authentication is one of the simplest forms of authentication. It involves sending user credentials (username and password) as part of the HTTP headers. This method is easy to implement, requiring minimal configuration in Spring Boot. However, Basic Authentication has notable security concerns.
- Credentials in Plain Text: When using Basic Authentication, credentials are encoded with Base64 but are not encrypted. This means that anyone intercepting the data can decode it.
- Over HTTPS: It is critical to use Basic Authentication over HTTPS to secure the transmission of credentials. Without HTTPS, data can easily be exposed to attackers.
- Session Management: Each request must include the credentials, otherwise, access is denied. This may not be practical for applications requiring extensive sessions.
Basic Authentication can be suitable for internal applications or scenarios where security requirements are minimal. Keep in mind, its simplicity does not come without risks, urging the necessity for using it thoughtfully.
JSON Web Tokens (JWT)
JWT is an advanced and flexible authentication mechanism that offers several benefits over Basic Authentication. A JSON Web Token is a compact, URL-safe means of representing claims to be transferred between two parties. The structure typically includes a header, a payload, and a signature, which collectively serves to ensure data integrity and authenticity.
- Stateless: JWT allows for stateless authentication since the server does not need to store session information. This can enhance performance, especially in distributed systems.
- Bearer Tokens: JWTs are often used as bearer tokens. This means that the receiver can grant access simply by presenting the token without needing to send credentials repeatedly.
- Laid Out Claims: JWT can contain claims that provide additional information about the user, such as roles and permissions, which can facilitate Authorization.
- Expiration: The token can include expiration information, reducing the potential for unauthorized access if a token is compromised. It’s crucial to implement token refresh strategies to maintain user experience while ensuring security.
JWT can be used in various applications, especially those that require a more advanced level of security and flexibility. Understanding the differences and implications of Basic Authentication and JWT is fundamental in choosing the right authentication mechanism for your Spring Boot REST API.
Defining Security Configuration
In the realm of securing REST APIs with Spring Boot, defining a proper security configuration is foundational. Effective security measures not only protect sensitive data but also ensure that the system remains robust against unauthorized access. Defining security configuration involves creating rules and policies that dictate how authentication and authorization are handled within an application. By establishing a clear security structure, developers can implement effective measures that reduce vulnerabilities and strengthen overall system integrity.
The process of defining security configurations allows developers to tailor the security measures according to the needs of their application. Important considerations include:
- Granularity of Access Control: This refers to how detailed the access policies are. Having fine-grained access control enables the application to specify permissions for various user roles, limiting what each user can do based on their role.
- Flexibility: Security configurations should be adaptable. As business needs evolve, the ability to modify security settings without extensive refactoring is valuable.
- Maintainability: A well-defined security configuration facilitates easier maintenance. When security policies are clear, identifying and resolving potential issues becomes more straightforward.
- Scalability: Anticipate growth and traffic increases. Configurations should be capable of supporting more users or expanded functionality without compromising security.
Overall, defining security configurations sets the stage for effective security management within a Spring Boot application.
Creating a Security Configuration Class
To create a security configuration class in Spring Boot, one typically extends the class. This approach provides the foundation on which security features can be defined effectively. The class allows us to override methods that let us customize authentication and authorization processes. Here’s a simple example of a basic security configuration class:
In this example, we define a class named that restricts access to certain endpoints, allowing unauthenticated access to the endpoint while requiring authentication for all others. The configuration also sets up basic authentication using an in-memory user store.
Customizing Defaults in Spring Security
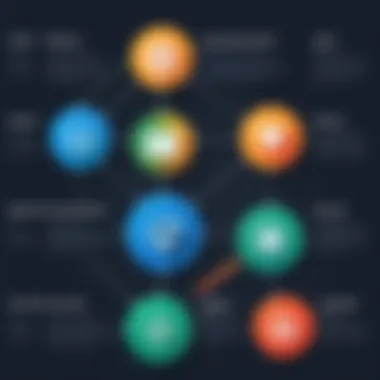
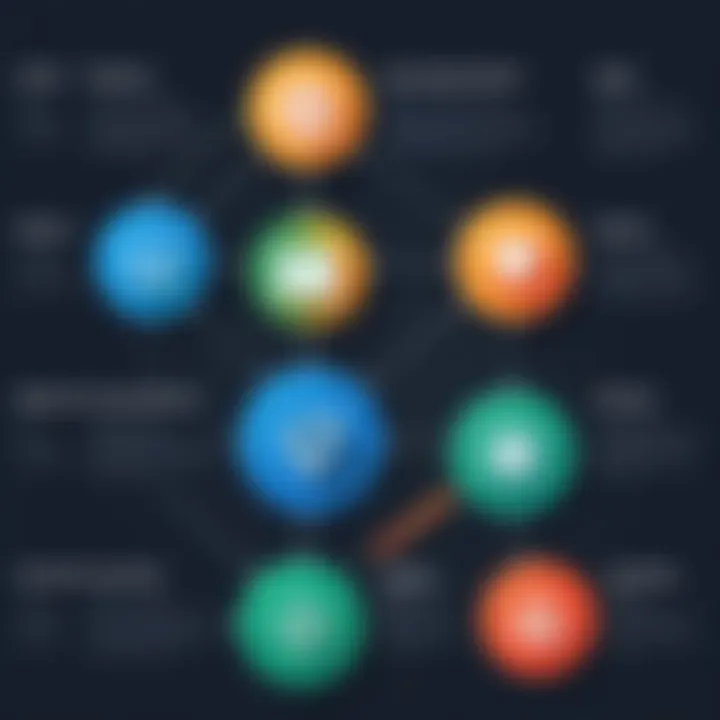
Spring Security provides sensible defaults, but it is often necessary to customize them based on specific requirements. This can involve defining custom user details services, setting password encoders, or modifying session management behavior.
Customizations may include:
- User Details Service: This allows for integration with a database or another data source to manage users instead of using an in-memory store.
- Password Encoding: For security reasons, it's important to ensure that passwords are stored securely. Spring Security allows the use of various encoders to hash passwords.
- Session Management: Customizing session management policies can help improve security against session fixation attacks. Setting session timeouts or restricting concurrent sessions are common practices.
Here’s an example of customizing the password encoder:
By ensuring proper configurations and customizations, developers can create a secure and efficient environment for their Spring Boot applications, making security an integral part of the development process.
Securing Endpoints with Annotations
Securing your REST API endpoints is crucial to protect sensitive data and maintain system integrity. The increasing number of cyber threats makes it imperative for developers to adopt secure coding practices. Annotations in Spring Boot provide a powerful mechanism for implementing security at various levels. They simplify the code and make it more maintainable while ensuring that only authorized users can access specific endpoints. Properly utilizing annotations can significantly enhance both your application's security posture and your development workflow.
Using @PreAuthorize
The annotation enables method-level security in Spring applications. It allows you to specify pre-conditions that must be satisfied before a particular method is executed. This is particularly useful when dealing with complex authorization requirements that depend on user roles or certain conditions.
Example usage:
In this example, only users with the role can access the endpoint. By using , developers can enforce security rules directly in their code while keeping business logic separate. This leads to cleaner and more manageable code.
When using , it is important to ensure that the authorization expressions are well-defined. A poorly defined expression can lead to unauthorized access or blocks that impact user experience. Additionally, the performance overhead should be considered, especially if the security checks are complex or if they involve external service calls.
Defining Roles with @Secured
The annotation offers a straightforward way to specify security roles on methods or class levels. It checks if a user has a specific role before allowing access to annotated methods. This is a simpler alternative to but does not support complex SpEL expressions.
Example usage:
Here, the endpoint will be accessible only to users who have the . This annotation is particularly beneficial for applications with a clear-cut role-based access control mechanism. It simplifies the security configuration by allowing for easy recognition and management of roles.
One consideration when using is that it does not support multiple roles directly. Developers may need to define separate methods or nested annotations to handle multiple roles efficiently. Also, it is crucial to keep roles updated across the application to avoid inconsistencies in access control.
"Securing APIs using annotations provides a clear and concise approach to access management. Make sure to balance simplicity with security needs."
In summary, both and are effective tools for securing endpoints in Spring Boot applications. By leveraging these annotations wisely, developers can enforce robust security measures while keeping the codebase clean and comprehensible. The choice between these two often depends on the complexity and requirements of your application's security model.
Testing Security Measures
Testing security measures is a critical component of developing a robust and resilient Spring Boot REST API. The landscape of security threats is ever-evolving, and developers must ensure that their applications are not only functional but also secure against potential attacks. This section delves into the importance of testing security measures, focusing on how it enhances system reliability and user trust.
Effective testing of security configurations helps identify vulnerabilities before they can be exploited. It ensures that the authentication and authorization mechanisms function as intended. Additionally, it validates that sensitive data is properly protected and that any security protocols are correctly implemented. Through thorough testing, developers can address weaknesses, improving the overall security posture of their APIs.
Key benefits of security testing include:
- Proactive risk management: Identifying vulnerabilities early reduces the chances of real-world breaches.
- Enhanced compliance: Regular testing can help meet different regulatory standards in security requirements.
- Increased confidence: Consistent testing builds trust among users and stakeholders, ensuring them of the safety of their data.
- Streamlined development: Uncovering and solving security issues during development prevents costly fixes after deployment.
Incorporating a comprehensive strategy that includes both unit testing and integration testing reinforces the importance of maintaining a secure application environment.
Best Practices for API Security
Securing APIs is critically important in today's digital landscape. As APIs become a central part of many applications, ensuring their security becomes paramount. Best practices for API security are not merely suggestions; they are essential steps that developers should integrate into their workflow to protect both the application and its users.
The significance of best practices lies in their ability to mitigate risks associated with API vulnerabilities. By following established guidelines, developers can minimize the chances of exploitation by malicious actors. Furthermore, adhering to best practices helps build trust with users, as they can have confidence that their data is well-protected.
Implementing Rate Limiting
Rate limiting is an effective strategy to control the number of requests a client can make to an API within a specified timeframe. Its implementation prevents abuse and helps safeguard against denial-of-service attacks, ensuring fair usage of resources.
Here are some reasons why rate limiting is essential:
- Preventing Abuse: By limiting the number of requests, you can protect your API from being overwhelmed by excessive usage.
- Enhancing Security: Rate limiting helps defend against various attacks, such as brute force attempts.
- Improving Performance: It ensures that all users enjoy a responsive service by preventing a surge of requests from a single source.
To implement rate limiting in a Spring Boot application, consider the following steps:
- Use Spring Cloud Gateway or Zuul to encapsulate request management.
- Define the maximum allowed requests per user within a certain period.
- Store request data in a database or cache for tracking usage patterns.
Here's an example of a rate limiter using Spring:
Using HTTPS
Using HTTPS is a non-negotiable aspect of securing APIs. HTTPS encrypts the data exchanged between clients and servers, protecting sensitive information from eavesdropping and man-in-the-middle attacks.
The following points illustrate the benefit of moving from HTTP to HTTPS:
- Data Protection: All data transferred is encrypted, providing a secure channel for communication.
- Integrity Assurance: HTTPS ensures that the data has not been altered in transit, maintaining its integrity.
- User Trust: Users are more likely to interact with APIs they perceive as secure, as signified by the HTTPS protocol.
To implement HTTPS in a Spring Boot application, obtain an SSL certificate from a trusted certificate authority. Configure the application to use the certificate by adding the following to your application.properties file:
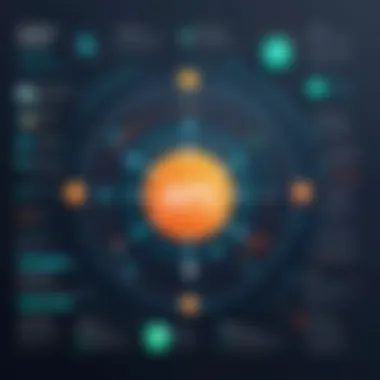
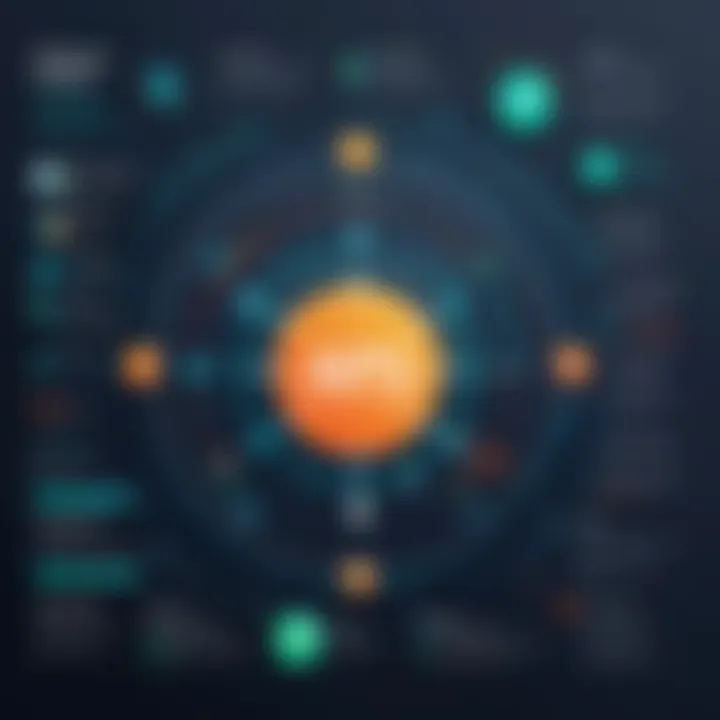
Ensuring that APIs use HTTPS is not just a best practice; it is foundational for secure communications in today's internet-driven ecosystem.
In summary, implementing best practices for API security, including rate limiting and using HTTPS, significantly enhances the security posture of an application. These practices not only protect against potential threats but also foster user trust and satisfaction. As applications continue to evolve, developers must prioritize security to create resilient solutions.
Addressing Common Security Vulnerabilities
In the rapidly evolving landscape of web applications, security remains a pivotal concern. When it comes to Spring Boot REST APIs, understanding and addressing common security vulnerabilities is essential. These vulnerabilities can have severe implications, such as data breaches and unauthorized access. By effectively mitigating these risks, developers not only protect sensitive data but also enhance the overall integrity and trustworthiness of their applications.
High-profile security breaches often exploit vulnerable points in an application. Thus, by prioritizing security, developers are taking proactive measures to safeguard their work against potential threats. In this section, we will explore two significant vulnerabilities: Cross-Site Scripting (XSS) and SQL Injection. Each presents unique challenges but can be managed with the right approach and best practices.
Cross-Site Scripting (XSS)
Cross-Site Scripting, commonly known as XSS, poses a significant threat to web applications. Essentially, XSS allows attackers to inject malicious scripts into web pages viewed by unsuspecting users. This can lead to the theft of cookies, session tokens, or other sensitive information. Understanding how to defend against XSS is crucial for maintaining user trust and protecting applications.
To prevent XSS attacks, developers should employ several strategies:
- Input Validation: Always validate and sanitize user inputs to ensure that malicious scripts cannot be executed. Use libraries that offer output encoding features to ensure proper encoding of data before rendering it on webpages.
- Content Security Policy (CSP): Implement a CSP to add an extra layer of security. This helps restrict where resources can be loaded from, significantly lowering the risk of XSS.
- Use of Frameworks: Frameworks like Spring provide built-in mechanisms for escaping output. Leveraging these tools can help reduce the risk of XSS attacks.
By focusing on these preventive measures, developers not only address the risks associated with XSS but also foster secure coding practices throughout the development cycle.
SQL Injection Prevention
SQL Injection remains one of the most prevalent vulnerabilities affecting databases. In this scenario, attackers exploit weaknesses in an application’s interaction with a database, allowing them to execute arbitrary SQL code. This can lead to unauthorized data access, data manipulation, and even data destruction.
Preventing SQL Injection requires diligent coding practices. Here are several effective strategies:
- Parameterized Queries: Always use parameterized queries or prepared statements. This method separates SQL code from data input, greatly reducing the risk of SQL injection. For instance, using Spring's JdbcTemplate can help implement parameterized queries easily.
- Input Sanitization: Just like with XSS, user input must be validated and sanitized. Disallowing certain characters and patterns can prevent malicious input from being processed.
- Least Privilege Principle: Configure database users with limited privileges. This minimizes damage if an injection attack is successful.
"Protecting against SQL injection is not just a technical challenge but a foundational aspect of building secure applications."
By implementing these strategies, developers can significantly reduce the chances of falling victim to SQL injection attacks. Awareness and methodical prevention are key components of an effective security posture in any application.
Each of these vulnerabilities highlights the critical need to integrate security into the development process from the very outset. As such, addressing common security vulnerabilities is not merely an afterthought; it is an integral aspect of creating resilient applications.
Logging and Monitoring in Spring Boot
Logging and monitoring are vital components of securing Spring Boot REST APIs. They serve as an early warning system for potential security issues and offer insights into application behavior. Effective logging ensures that all critical events in the application lifecycle are captured. This aids in diagnosing problems quickly. Additionally, good monitoring practices help developers keep track of performance and detect unusual patterns that may signify unauthorized access.
Setting Up a Logging Framework
To implement logging in Spring Boot, it is essential to choose the right logging framework. Spring Boot supports various logging libraries out of the box, such as Logback, Log4j2, and Java Util Logging. Using Logback is often recommended due to its performance and flexibility.
You can set up logging in your application by including the necessary dependencies in the file if using Maven:
Once the dependency is in place, you can configure logging levels in the file. For example:
The above snippet configures the default logging level to . This means all INFO level and higher messages will be logged. The log file will be named , and the log format is specified as well. This helps in creating structured log outputs that can easily be parsed later.
Understanding Audit Trails
Audit trails are systematic record-keeping of events that occur within an application. In Spring Boot, enabling audit trails allows you to track critical information related to user actions and system changes. This can be crucial when investigating security incidents or meeting compliance requirements.
To create audit trails, you can utilize Spring Data JPA, which allows you to implement event listeners to log actions like create, update, and delete operations. An example is shown below:
In this example, the entity tracks creation and last modification timestamps. This data proves beneficial for auditing purposes. Ensuring the existence of an audit trail can help rectify issues stemming from unauthorized access or data corruption.
Proper logging and monitoring open the door to better security and improved application resilience. They help maintain a secure environment, building user confidence in your Spring Boot REST API.
Ending
One significant aspect discussed is the importance of authentication and authorization. These processes ensure that users are verified and have appropriate privileges. Without these measures, APIs become vulnerable to various attacks, often leading to data breaches.
Additionally, we emphasized the need for preventing common vulnerabilities. Measures against Cross-Site Scripting (XSS) and SQL injection are vital. Implementing these defenses can significantly reduce the risk of exploitation, ensuring that your API remains resilient against attempts to compromise its security.
Another key takeaway is the emphasis on best practices, including rate limiting and using HTTPS. These steps provide an extra layer of security, safeguarding against denial-of-service attacks and ensuring that data in transit remains encrypted and secure.
Overall, the knowledge shared throughout this article is designed to equip you with the necessary skills to foster a secure API environment. By applying the principles and practices discussed, developers can better protect their applications, gaining user trust and ensuring data integrity.
Remember, security is a continuous process that requires constant evaluation and enhancement. It is best to stay updated with the latest security trends and technologies.
Further Reading and Resources
In the context of securing Spring Boot REST APIs, a thorough understanding goes beyond initial concepts and implementations. Further reading and resources plays a pivotal role in equipping developers with deeper insights and advanced skills. This section outlines why this aspect is crucial for both novices and seasoned professionals alike.
Firstly, the ever-evolving nature of security means that staying updated with the latest practices is essential. Technologies and threats progress rapidly; hence, continuous learning helps developers to adapt their knowledge to contemporary challenges. Incorporating latest frameworks or methodologies may involve complex decisions. Resources can guide these choices effectively.
Benefits of Further Reading
- Enhanced Knowledge: Delving into books, articles, and online courses can provide a stronger grounding in security principles and techniques.
- Access to Expert Insights: Various forums and community discussions offer perspectives from experts who have faced similar challenges. This can provide pragmatic solutions.
- Learning from Case Studies: Real-world applications often highlight mistakes or successes in security implementations. Analyzing such scenarios offers valuable lessons.
- Updates on Security Trends: Following relevant blogs and subscribing to security newsletters can help in understanding the latest vulnerabilities and mitigations that impact API security.
Considerations for Selecting Resources
- Reputation of Source: Choose established authors, organizations, or platforms that are known for their credibility in software security.
- Current Relevance: Ensure that the resources address contemporary issues and not outdated practices.
- Practical Focus: Look for materials that emphasize hands-on examples and real-life applications, which are valuable for skills enhancement.
Suggested Resources
- Wikipedia on API Security for foundational knowledge.
- Online forums like Reddit can foster community learning and discussion.
- Scholarly articles on security trends can be found through platforms like Britannica.
"Continuous education is the key to unlocking security potential in your applications."