Running Python Programs: A Comprehensive Step-by-Step Guide
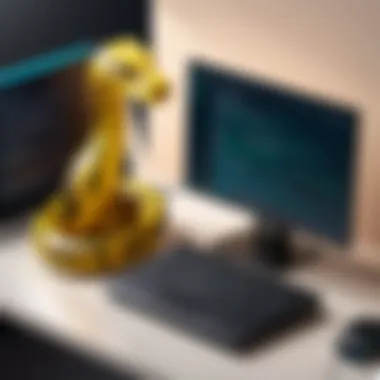
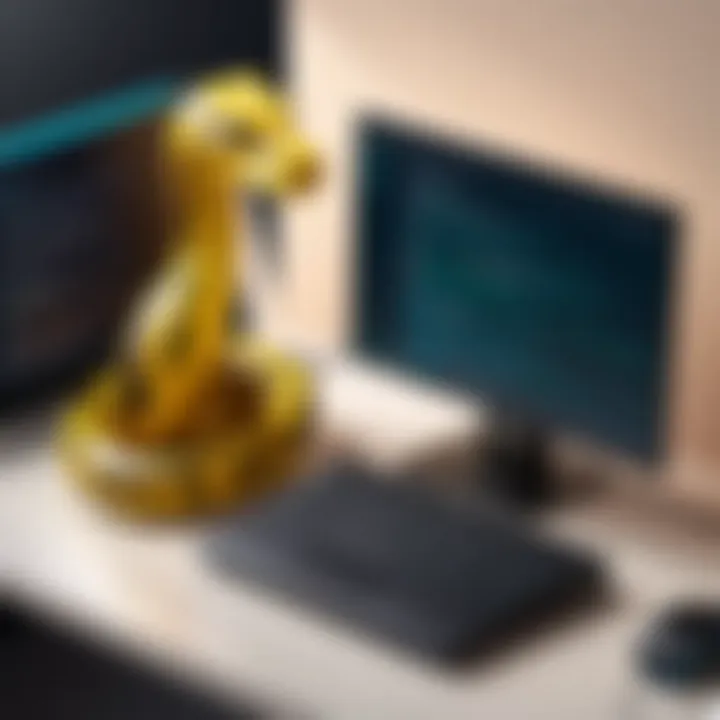
Intro
Foreword to Programming Language
Programming languages serve as the foundation for developing a diverse array of software applications. Python, a high-level language, stands out for its versatility and straightforward syntax. This language enables beginners to quickly grasp core concepts while providing advanced features for skilled programmers to leverage complex functionalities. Learning Python opens doors to various fields, from web development to data analysis.
History and Background
Python was conceived in the late 1980s by Guido van Rossum. Named after the British comedy television show, Monty Python's Flying Circus, the intent was to create a language that was fun and easy to use. Its first official release came in 1991. Over the years, Python has evolved through numerous revisions, significantly increasing its functionality and user base. By version 3.0, released in 2008, there was an emphasis on improving the programming experience and making better use of modern programming practices.
Features and Uses
Python is known for its simplicity and elegance. Here are some features:
- Easy-to-learn syntax: Makes it accessible for beginners.
- Extensive libraries: Offers numerous modules and packages for various tasks.
- Cross-platform compatibility: Runs on various operating systems with ease.
- Strong communities: A large user base provides support and resources.
Python's applications range widely. It is frequently used in web development with frameworks like Django, in data science with libraries such as Pandas and NumPy, and in automation, among others.
Popularity and Scope
Python's reputation has skyrocketed over the past few years, often ranking in the top programming languages around the world. Job market demand for Python skills has also risen. Its blend of application, community support, and straightforward learning curve positions it as a top choice for both new and seasoned developers.
Basic Syntax and Concepts
Understanding Python’s syntax and fundamental concepts is crucial for anyone starting their programming journey.
Variables and Data Types
In Python, variables store data values. They do not require explicitly declared data types, which promotes ease of use. Common types used in Python include integers, floats, strings, and booleans.
For example:
Operators and Expressions
Operators in Python are used to perform operations on variables and values. This includes arithmetic, assignment, comparison, and logic operators such as +, -, , >, and ==.
Python allows combining these operators in expressions, enabling a straightforward calculation or comparison.
Control Structures
Flow control signatures such as if-statements, loops like for and while establish how the code runs. Proper mastery of this would improve coding efficiency and accuracy in executing specific tasks.
Advanced Topics
As programmers advance, they encounter more complex concepts that can enhance their coding abilities.
Functions and Methods
Functions are reusable pieces of code. Defined with the keyword, they take inputs, perform actions, and produce outputs. "Methods" can be understood as functions tied to object types, showcasing the object-oriented nature of Python.
Object-Oriented Programming
Python supports object-oriented programming, which aims to utilize 'objects' to model real-world data. This paradigm encourages clear modularization and encapsulation, making code easier to manage and scale.
Exception Handling
Using try and except blocks can prevent program crashes due to unpredictable errors. This adds robustness to the code and enhances the overall user experience.
Hands-On Examples
To solidify understanding, engaging with hands-on examples is effective.
Simple Programs
Starting with basic console printouts or variable manipulations helps learners gain confidence in using Python syntax and getting used to running scripts.
Intermediate Projects
Building projects like calculators or small text games can encourage a mix of fundamental and advanced skills. Implementing functions or following object-oriented practices can be explored.
Code Snippets
Reusable code snippets serve as an essential resource as learners expand their projects. They are often shared across forums such as Reddit and GitHub.
Resources and Further Learning
A wealth of resources exists to enhance Python learning.
Recommended Books and Tutorials
Books like Automate the Boring Stuff with Python by Al Sweigart are great for beginners. Extensive tutorials can be easily found online to supplement learning.
Online Courses and Platforms
Platforms like Coursera, edX, and freeCodeCamp offer structured courses from reputable institutions.
Community Forums and Groups
Active discussions and collaborations can be found on sites like Reddit's /r/learnpython and active Facebook groups for problem-solving and tips.
In summary, mastering Python involves understanding its syntax, code execution methods, and best practices through practical engagement.
Prelude to Python Programming
In this digital era, Python has emerged as a critical language for various realms of technology and software development. It offers a blend of simplicity and powerful features that cater to novices and seasoned programmers alike. Understanding Python programming is indispensable for realizing its full potential in real-world applications.
Overview of Python
Python, created in the late 1980s by Guido van Rossum, places great emphasis on code readability and developer productivity. The syntax of Python is designed to be clear and straightforward, making it an ideal choice as students explore programming languages for the first time.
The extensive standard library and the vast ecosystem of third-party packages make Python versatile. This enables developers to tackle web applications, data analysis, artificial intelligence, and more with remarkable efficiency. With its evolving nature and continual updates, Python retains its status as a popular tool in various technology sectors.
Key features include:
- Interpreted language: This feature allows developers to run code without excessive pre-compilation, fostering rapid development cycles.
- Dynamically typed: This results in less code to write and simplifies many processes.
- Multi-paradigm: Support for procedural, object-oriented, and functional programming offers the programmer numerous ways to approach a problem.
- Extensive libraries: Access to a massive repository of libraries minimizes the need to reinvent the wheel in coding projects.
Importance of Python in Modern Development
The importance of Python in modern development cannot be understated. In contemporary tasks, it not only serves as a foundational language, but also enhances productivity across various domains. Should you be engaging with data science projects, machine learning applications, or conventional web development, Python provides consistency which often solves complexities inherent in coding with other languages.
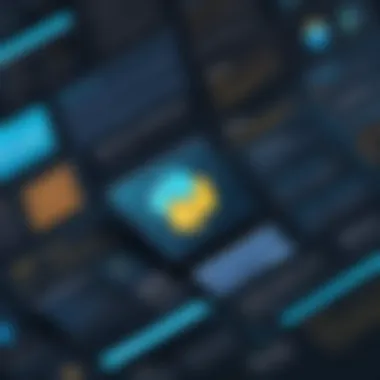
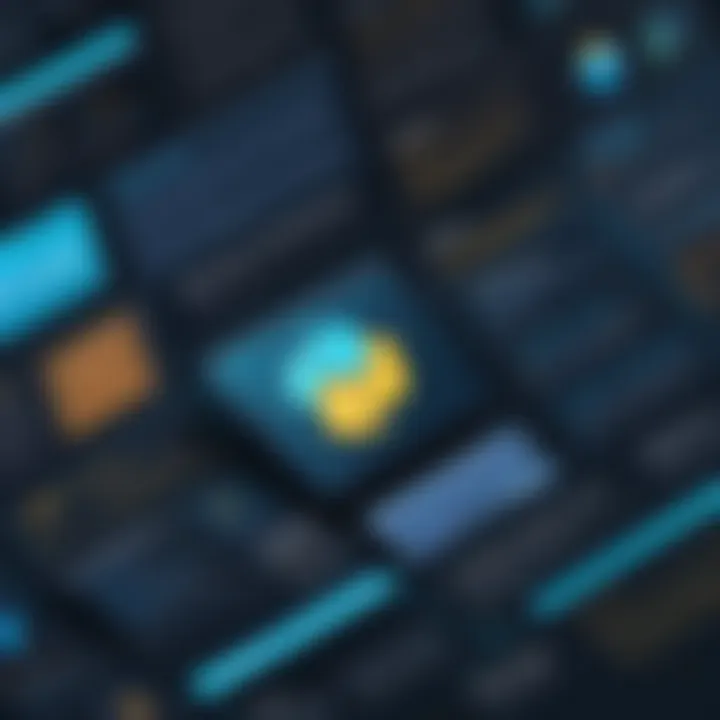
Several factors contribute to Python's significance in the tech landscape today:
- Community and Resources: A robust community contributes to an extensive set of documentation, tutorials, and support, facilitating trouble resolution.
- Adaptability: Python integrates easily with other languages and technologies, providing agility and versatility in your programming toolkit.
- Employment Opportunities: As many firms recongnize Python's growing presence in crucial projects, career opportunities in data analysis, web development, and machine learning are growing.
- Education Sector: Python is widely adopted in academic curriculums as a primary programming language for Computer Science studies. Its uncomplicated syntax makes it excellent for gradually building programming skills.
In an ever-evolving technological landscape, embracing Python remains critical for learners and professionals alike.
Understanding the foundations of Python programming sets the groundwork for delving deeper throughout this guide, allowing learners to maximize their coding efforts efficiently.
Setting Up the Python Environment
Setting up the Python environment is a fundamental step for anyone who wants to run Python programs effectively. This configuration ensures that the Python interpreter operates correctly while providing access to the necessary libraries and tools for development. Without a proper setup, one may experience issues ranging from execution errors to compatibility problems with packages or dependencies.
Establishing a well-defined environment allows for a smoother coding experience. It allows developers to focus on building applications instead of troubleshooting problematic configurations. Best practices in setting up Python environments might involve creating isolated spaces for projects, using virtual environments, which help to prevent version conflicts of modules and packages. Consequently, this enables cleaner development and fewer headaches down the line, which is essential, especially for students or novice programmers.
Selecting a Python Version
Choosing the right version of Python is crucial, as different projects may have specific requirements. Python is continually evolving, with variant releases regularly. Each version can introduce enhancements, debugging features, or changes in behavior that can affect existing code. Thus, understanding the distinctions among versions—primarily between Python 2 and Python 3, as well as maintaining awareness of the latest updates—will directly influence development choices and compatibility with libraries. For beginners, aligning with the latest stable release is usually a prudent option, as it includes the most current features and security patches.
Installing Python on Different Operating Systems
Installing Python varies across operating systems. The underlying operating system often influences the methods and packages available for developers. Here's a brief overview of how Python is set up on popular platforms:
Windows
For many users, installing Python on Windows is straightforward due to an installer that guides the process through user-friendly interface. Windows is a dominant operating system in many environments, making it a popular choice among developers. The installation wizard simplifies the process significantly, even for less experienced users.
One unique feature for Windows is its use of the PATH variable. Users must remember to add Python to the PATH variable to run scripts from the command prompt successfully. A key element is ease of integration with IDEs like PyCharm or Visual Studio Code; they offer extensive support and generally lead to efficient programming experience. However, certain challenges include inconsistent package commands when utilizing system-based installations compared to others like homebrew on macOS or apt for Linux.
macOS
The macOS operating system comes with Python pre-installed, which arguably makes it simpler for macOS users to start programming. This characteristic ensures that users can begin executing simple scripts without additional installation steps. Many developers on this operating system prefer tools like Terminal for their rich Unix capabilities, allowing them to harness powerful scripting functionalities.
Installing third-party versions via Homebrew becomes convenient in macOS, as users can easily manage Python installations without affecting system components. One drawback, however, can be the frequent updates of the macOS itself, which sometimes affect Python installations negatively, as libraries might break compatibility after upgrades. Developers should keep abreast of such changes.
Linux
When it comes to Linux, Python's integration is deeper, as most distributions include Python as part of the core system. Many experienced developers enjoy using Linux because of its robust package management systems like apt, yum, or pacman to manage Python packages effectively. Working in Linux encourages familiarity with command-line operations, vital for developers proficient in systems programming.
The overwhelming variety of distributions can be a challenge. Users may encounter discrepancies in Python versions across Linux OS flavors, thus complicating projects reliant on specific dependencies. Every version brings unique implications that might initially appear bewildering to learners; however, it becomes quintessential knowledge for more advanced programming tasks.
In summary, selecting and setting up the Python environment requires careful consideration of the operating system and user needs. Each system provides unique features and hurdles. However, understanding the installation techniques allows one to create functioning and efficient Python environments, leading to a successful coding journey.
Using Integrated Development Environments (IDEs)
Integrated Development Environments, or IDEs, are essential tools for Python programmers. They provide a unified environment that integrates code writing, debugging, and execution. Using an IDE can greatly elevate the coding experience. Many IDEs offer features such as syntax highlighting, code auto-completion, and debugging capabilities. These streamline the development process and enhance productivity, making coding more efficient. Different IDEs cater to diverse user needs, making familiarity with them crucial for effective learning and development.
Popular IDEs for Python Development
PyCharm
PyCharm is a widely used IDE developed by JetBrains. It stands out because of its powerful code navigation and refactoring functionalities. PyCharm supports various frameworks, such as Django. The strong integration with version control systems makes it a favorable choice for collaborative projects. One unique feature of PyCharm is its intelligent coding assistance, which offers code suggestions as you type, reducing development time significantly. While it provides robust tools, its community version doesn't include all features present in the Professional version, which may discourage very beginner users who require simplicity over advanced tools.
Visual Studio Code
Visual Studio Code, often abbreviated as VS Code, is a free, open-source IDE favored by many developers. It's known for its flexibility, allowing extensive customization through extensions. This IDE is lightweight and offers exceptional performance, which makes coding smooth and efficient. A unique feature of VS Code is its integrated terminal, which allows users to run command line tools directly within the IDE. This means you can execute scripts without switching between different windows. Although full functionality depends on the installation of extensions, some beginners may find their configuration possibilities overwhelming.
Anaconda
Anaconda is an open-source distribution focused on data science and machine learning. Its appeal lies in its inclusive nature, featuring pre-installed libraries and packages vital for scientific computing. Anaconda’s package manager, conda, simplifies the installation of various libraries and dependencies, which is particularly beneficial for users working with data analysis. Furthermore, Anaconda includes Jupyter Notebooks, enhancing its functionality for users interested in data visualization and exploratory analysis. While it’s robust, Anaconda might be considered excessive for general Python development tasks that do not involve heavy data tasks, leading some to prefer lighter IDEs for simpler projects.
Setting Up an IDE
To start using an IDE for Python programming, one must follow specific steps. First, choose an IDE that fits your personal or project requirements. Download and install the desired IDE from its official website. Upon installation, familiarize yourself with the layout and available tools. It's advisable to take advantage of available documentation and tutorials provided by the IDE’s creator to optimize your setup. After installation, configure settings like terminal paths or environment configurations which aids in better version control of coding projects in your workspace.
Running Python Programs from the Command Line
Running Python programs from the command line is a fundamental skill for any aspiring developer or programmer. It allows users to execute scripts without the need for graphical interfaces, making it particularly useful in environments where graphical support may be limited. Understanding how to navigate the command line and execute Python programs from there is particularly valuable for automating tasks and working in cloud infrastructure. Moreover, efficiency often occurs in the hands of those who command their environments with proficiency.
Opening the Command Line Interface
Windows Command Prompt
The Windows Command Prompt (CMD) serves as a direct interface between the user and the underlying system. One of its basic yet profound features is its simplicity and universal accessibility across Windows systems. It allows for executing commands by typing them directly, which can streamline multiple processes. CMD is highly beneficial as many Python scripts, especially those planned for deployment or automation, may run more seamlessly from this solid platform.
- Advantageously, CMD gives users access to various command-line tools which increase productivity. However, it may lack some advanced features found in third-party terminals or shells.
To open Command Prompt, press , then type and hit enter. Your initial interaction with Python within this interface can commence from there.
macOS Terminal
The macOS Terminal is a powerful tool that serves as the gateway to the Unix-like shell of macOS. What stands out about Terminal is its integration with a robust and versatile UNIX subsystem, which allows users to use Linux tools natively within macOS. This makes it a powerful choice for Python developers who want to leverage Python's capabilities alongside standard fabric of the operating system.
- It's seen as beneficial because of its supportive community; users can easily find resources and support online based on Unix/GNU/Linux standards. Nevertheless, for someone unfamiliar with Unix commands, the learning curve might be steeper in the beginning.
Accessing the Terminal can be done by searching for it in Spotlight or through your Applications folder. Once opened, entering Python commands is straightforward and direct.
Linux Terminal
Linux Terminal stands as an advanced option for managing system interactions thanks to its scriptability and extensive command set. This terminal provides a robust interface that integrates well with Python programming through its command execution processes. The Linux environment is renowned for supporting developers across different platforms, making this terminal a natural fit for Python tutorials or collaborative open-source projects.
- Learning to interact with the Linux Terminal can be a rich and rewarding experience as it often provides many scripting possibilities, but it can also be bewildering for newcomers. Essential navigation and proper use of commands is key when running Python programs since mistakes could cause unintended outcomes.
The Terminal can be accessed through various distributions of Linux, often through shortcuts in menus or using keyboard shortcuts Alt + F2, followed by or equivalent for your particular environment. Once accessed, executing scripts is prompt and effective.
Executing Python Scripts
Basic Syntax
The basic syntax of running Python scripts from a command line may seem intuitive, but clarity in this step is crucial. Standard command formatting is essential: one simply needs to prefix their Python script name with the , or command, depending on their version of Python.
This command must be executed from your working directory where the script is located. Ignoring path requirements can lead to significant errors. This direct execution method fast-tracks many processes and minimizes error rates when launching Python scripts.
File Navigation
File navigation stands as a crucial skill within command line interfaces when executing Python programs. It enables the user to directly locate the Python file they wish to execute, giving them autonomous management over their coding environment. Using commands like (change directory) is key. For example:
This highlights the nature of how to easily switch your current directory within these interfaces. Here, knowing your file structure is paramount to increasing coding efficiency. This process can seem cumbersome for those used to clicking but becomes second nature over time. Each successful execution reinforces the Learning and problem-solving that underpins Python programming.
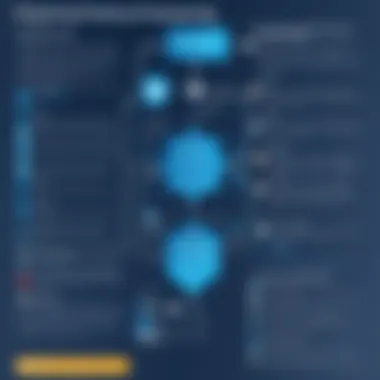
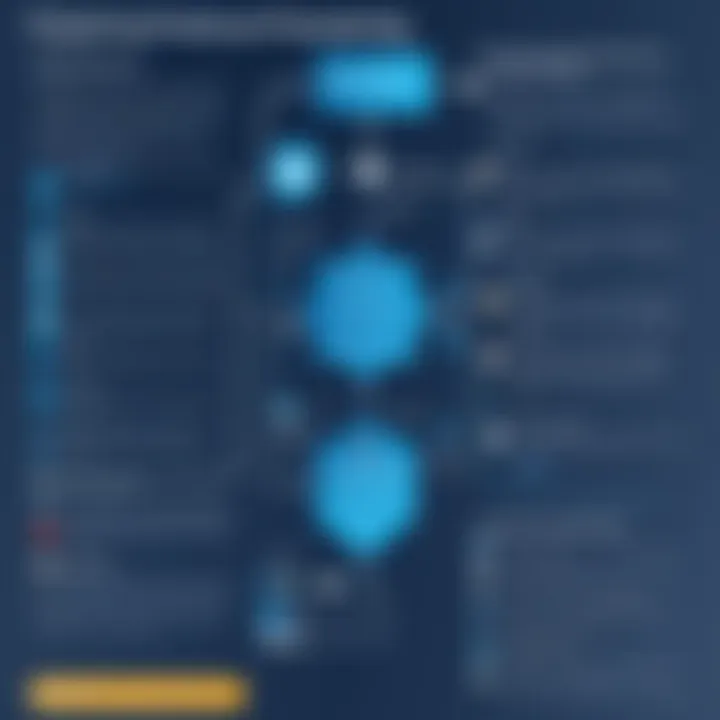
Using Jupyter Notebooks
Jupyter Notebooks are a vital tool in the Python programming landscape. They offer an interactive computing environment that blends code execution, visualization, and rich narrative text. Students and aspiring developers can utilize Jupyter Notebooks for various purposes, such as data analysis, data visualization, and machine learning experiments. The ease of use and flexibility makes it an essential aspect of running Python programs efficiently.
Prologue to Jupyter Notebooks
Jupyter Notebooks originated from the IPython project and have quickly become a favorite among programmers and data scientists. One key advantage of using Jupyter is its ability to combine executable code, formatted text, equations, and visualizations in a single document. This feature heightens understanding and allows for easier sharing of insights.
Moreover, notebooks can be run in varios ways, such as locally installed on one's machine, on cloud services, or even within frameworks like Apache Spark. Interest in Jupyter Notebooks has increased, especially in educational settings where demonstrative and interactive elements enhance teaching of programming concepts. Several resources are available to start with Jupyter, including relevant documentation which one can find here.
Creating and Running Cells
- Insert a New Cell: Use the button or press when a cell is selected to add a new cell below.
- An example Code Cell: Here is a small snippet that adds two numbers:
The flexibility provided by running individual cells allows users to go back for adjustments without needing to rerun the entire program. This proves invaluable for debugging and refining analysis over time. Learning how to manage cells proficiently is fundamental for an efficient computing workflow.
A well-managed Jupyter Notebook is a living document, illustrating both the process and result of your coding journey.
Mastering Jupyter Notebooks effectively boosts the coding skills of students and brings structure to their programming projects.
Running Python Scripts in the Cloud
Running Python scripts in the cloud introduces flexibility and accessibility for programmers at all levels. The availability of cloud computing resources enables users to execute their code without the need for powerful hardware on their local machines. This section will break down the benefits provided by cloud execution while outlining popular platforms that offer this service.
Benefits of Cloud Execution
Cloud execution delivers several advantages to programmers. First and foremost, it can reduce the dependency on personal hardware. Users can leverage the cloud's computation power, bypassing the limitations of their local environments. Additionally, running Python scripts in the cloud promotes collaboration. As scripts can be accessed from any device, sharing projects become easier among colleagues or sharing insights in community forums.
There are several considerations to keep in mind when working in the cloud. Idenitfying your specific needs, you can easily find the appropriate cloud platform for your work. Assessments for connectivity and necessary resources are essential to ensure an integrated and smooth development experience.
Popular Cloud Platforms
Google Colab
Google Colab stands out as a versatile platform designed for data scientists and machine learning enthusiasts. Its integration with Google Drive facilitates effortless storage and sharing of notebooks. One key characteristic is its support for libraries such as TensorFlow and PyTorch, delivered straight from the cloud without initial installations on personal systems.
Google Colab offers a significant advantage: GPU support. Users can easily access GPU resources for demanding tasks without high costs associated with traditional setups. However, there are limitations regarding session times, and should take precaution if working on long-execution tasks.
Replit
Replit introduces an assortment of programming languages, prominently featuring Python. This user-friendly platform enables real-time collaboration, with an easily navigable interface. A unique feature of Replit is its environment where multiple contributors can write code simultaneously. This fosters a productive and pleasant coding atmosphere.
Together with collaboration tools, users can run scripts instantly without intense configurations. However, the optimizing of more intense, resources during execution can be less effective based on free-tier resources.
Azure Notebooks
Azure Notebooks is another robust cloud training and deployment option, which supports Jupyter notebooks. It allows users a comprehensive workspace designed for machine learning students and educators alike. A notable quality is the integration with Microsoft's extensive suite of services, building powerful workflows.
Azure Notebooks exhibits great versatility. Creating environments allowing specific projects to utilize particular versions or libraries can assist developers with specifics like matching corporate requirements. However, complexity and Azure's multiple tools-forward structure may contribute some challenges for those new to cloud programming.
Understanding Python Package Management
Python package management is essential for developers working in various computational fields. Managing packages effectively ensures a smooth coding experience, enabling one to leverage external libraries and tools that simplify or enhance productivity. Package management involves the installation, updating, and removal of libraries as needed, making it a crucial part of working with Python.
Contemporary development often relies on numerous packages to extend functionality. Using a well-provided package allows programmers to focus on their unique problem-solving aspects instead of re-creating existing solutions. The pros of utilizing solid package management practices include:
- Efficiency: Quickly install and utilize various libraries, avoiding redundant coding.
- Version Control: Keeps programs updated with the latest features while handling compatibility issues when using different library versions.
- Framework Support: Many web and data science frameworks rely closely on various packages for optimal operation, enabling developers to tap into existing communities and resources of shared knowledge.
Developers must appreciate the dynamics involved in package management because they can lead to faster and better programming. Knowing how and when to utilize package management enhances the coding workflow, often yielding significant advantages in application performance and speed.
Using pip for Package Installation
Pip, an essential tool in Python, simplifies package installation for users. It serves as the de facto package manager for Python, allowing easy downloading of third-party libraries from the Python Package Index (PyPI). With its extensive repository, users can access an almost limitless pool of extensions.
Basic Usage
To get started with pip, follow these simple commands in the command line:
Replace with the actual name of the desired package. Users should familiarize themselves with basic pip concepts to harness its full potential:
- Installing a package:
- Updating a package:
- Uninstalling a package:
- Listing installed packages:
Familiarity with these commands will position users favorably during their programming endeavors.
Creating Virtual Environments
Isolating project environments is a best practice that developers should consider for robust application development. Virtual environments create separate spaces for dependencies related to different projects, mitigating compatibility and dependency management issues.
To establish a virtual environment, one can use the module included with standard Python installations. The main steps include:
- Create a new virtual environment:
Navigate to your project directory and run:This command produces a new folder named , containing the isolated environment. - Activate the virtual environment:
- Install packages within the environment:
Use pip as demonstrated: - Deactivate the virtual environment:
When done, exit with:
- On Windows:
- On macOS / Linux:
Maintaining isolated environments increases the reliability of application performance across different computational tasks.
Creating virtual environments focuses on clarity within development workflows. The method is not just clean; it safeguards against eerie conflicts arising from mixed libraries in the system. Combining pip and virtual environment management optimizes programming capabilities significantly.
Debugging Python Programs
Debugging is an essential skill for any programmer, and this holds especially true for those using Python. It involves identifying and removing bugs or errors from Python programs. A rigorous debugging strategy enhances not only the efficiency of the code but also the programmer’s understanding of Python’s intricacies. As learners develop their skills, effective debugging leads to greater quality in their applications.
Understanding the importance of debugging cannot be understated. It allows developers to ensure their code behaves as expected. The practice has significant benefits, including:
- Improved code quality
- Reduced time spent on repeated troubleshooting
- A more profound understanding of program logic
- Enhanced parallel knowledge that can be applied across different languages
Debugging is also crucial for learning. Beginners will often encounter common hurdles that can obstruct their progress. Recognizing and addressing these issues can prevent the loss of motivation and keep learning on track.
Debugging is not just about fixing errors; it's a journey toward understanding the code better.
When approaching the debugging process, one must keep feasibility in mind. Anecdotes of frustration are common, particularly among beginners faced with formidable error messages. Thus, understanding the context of an error can provide insight into its origin, leading to more effective solutions in the long run.
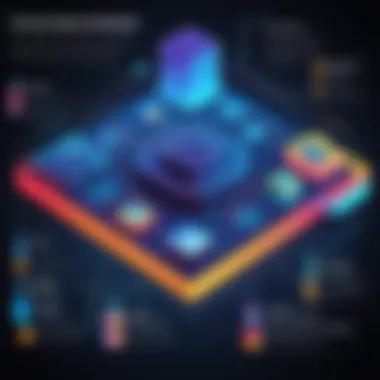
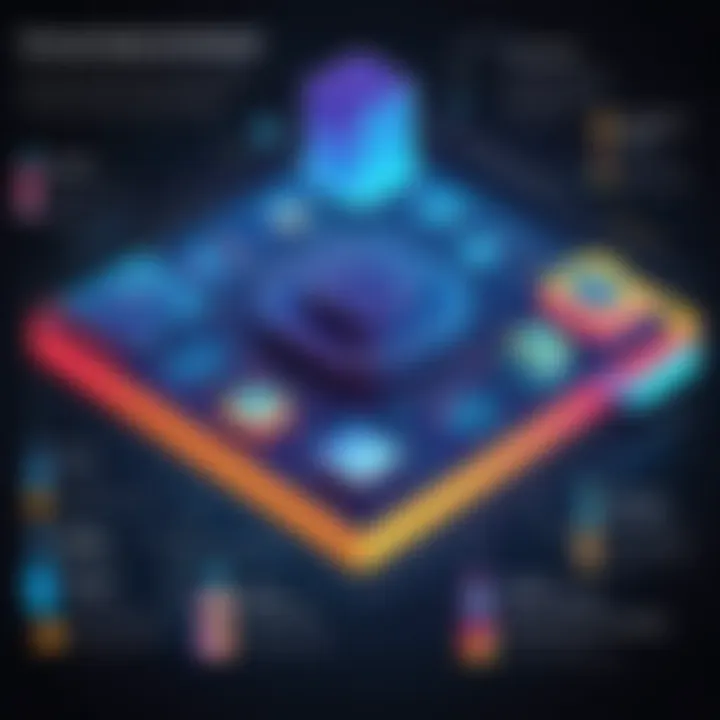
Common Errors in Python
Numerous pitfalls await Python programmers at various learning stages. Knowing these common errors is vital. Some frequent mistakes include:
- Syntax errors: Typos or incorrect punctuation can break the script. For instance, forgetting a colon at the end of a statement will result in an error.
- Indentation errors: Python's use of indentation means this mistake is particularly tricky. Unable to correctly indent blocks of code can confuse the interpreter.
- Name errors: Using variables that have not been declared can confuse code execution. This leads often to the infamous ‘NameError’ exception.
- Type errors: Mismatching data types when performing operations also causes the script to abort unexpectedly.
Each of these errors provides learning moment. Identifying and fixing them builds familiarity with the subtleties of Python programming, encouraging more efficient coding practices. Additionally, adhering to a set of practices helps to identify coding issues swiftly: maintaining clear comments, following naming conventions, and understanding standard libraries are just a few essential strategies developers should adopt.
Using Python Debugger (pdb)
The Python Debugger, or pdb, is a powerful tool that plays an essential role in simplifying the debugging process. It provides interactive debugging capabilities, allowing programmers to pause execution, examine variables, and explore the program state step by step. Utilizing pdb can demystify complex code behavior, enhancing both learning and function.
Key features of pdb include the ability to:
- Set breakpoints: This feature allows stopping the program at specified lines, granting time to investigate partial execution.
- Step through code: Developers can execute code one line at a time while observing how variables evolve. This helps to pinpoint precisely where an error arises.
- Examine stack frames: Observing function calls can provide insights into how the program reached its current state.
Starting with pdb is straightforward. Here is a minimal setup example:
Running this code will allow users to interact with the terminal directly when the error occurs, presenting immediate feedback that is vital for correcting mistakes swiftly. Familiarizing oneself with pdb will not eliminate all challenges but can significantly ease the study of Python runtime behavior.
Testing Python Programs
Testing Python programs is a crucial part of the development process. It ensures that our code behaves as expected. By implementing tests, developers can detect errors early, leading to fewer defects in production. This section explores why testing is important, focusing on frameworks and how to write effective tests.
Prolusion to Testing Frameworks
Testing frameworks provide structured methods to test Python code efficiently. They organize tests, run them, and report outcomes in a clear manner. Familiarization with these tools like pytest, unittest, and nose is vital. Each framework has unique features and suited best for specific testing tasks.
pytest is widely used for its simple syntax and rich feature set. It supports fixtures and plugins, enhancing the testing experience. unittest, on the other hand, is part of the standard library, offering a reliable foundation for both unit and functional tests. This makes it easy for programmers to get started without needing external dependencies. Knowing the strengths of each framework helps in choosing the right tool for testing needs.
Key features of popular frameworks include:
- Ability to group tests for organization
- Clear reporting of passed and failed tests
- Extensive support for assertions and error detail
Overall, using these frameworks allows developers to maintain and enhance code quality, making software development more efficient.
Writing Unit Tests
Unit tests form the first line of defense in testing. These are small, focused tests that target individual components or functions in a program. Writing unit tests ensures each piece works correctly before they are integrated into larger systems.
A good practice is to follow the Arrange-Act-Assert pattern. This helps structure the tests and makes them easier to understand. Here’s a simple example of a unit test using the unittest framework:
This code sets up a basic test case. It verifies that the addition of 2 and 2 equals 4. Clear organization of tests helps others (or future you) grasp the intent quickly.
Testing is a discipline, it creates trust in our code, and trust makes collaboration easier.
The benefits of writing unit tests include:
- Improved code reliability.
- Flexibility to make changes with assurance.
- Better documentation of coding behavior.
Investing time in creating thorough tests pays off as it reduces debugging time and enhances productivity. Clear and effective unit tests are invaluable, especially in larger projects, where understanding interactions between different elements can be challenging.
Common Challenges in Running Python Programs
Running Python programs can present a range of challenges, especially for those who are new to programming or only at an intermediate level. As with any skill, understanding these challenges is critical for developing proficiency in Python. An awareness of the typical hurdles assists learners in rapidly navigating issues that might otherwise hinder their progress. This knowledge lays a solid foundation for effective problem-solving in future programming tasks.
Identifying Common Issues
Successfully identifying common issues is the first step towards resolving them. Here are several frequent challenges that new and even experienced Python developers may encounter:
- Syntax Errors: These mistakes often occur due to typos, omitted punctuation, or incorrect indentation. Python is sensitive to syntax details, making this the most prevalent issue.
- Type Errors: When an operation or function receives an argument of an inappropriate type, it results in a type error. Such issues usually stem from discrepancies in expectations about data types.
- Import Errors: When attempting to import a module, errors may arise if the module is not installed or cannot be located. This is particularly prevalent in larger projects with many dependencies.
- Logical Errors: These errors can be the most challenging to find as they occur when the program runs without issue, but the outcome is incorrect. Proper testing can help in identifying the source.
Identifying these issues allows developers to act quickly and minimize disruption to their coding efforts. Being receptive to the signs of such defects can facilitate a smoother programming experience.
Troubleshooting Techniques
Once you have identified an issue, employing effective troubleshooting techniques is paramount. Here are some strategies to consider:
- Read Error Messages: Python often provides clear error messages, including line numbers and specific descriptors. Take the time to analyze these messages before moving forward. They usually point directly to the problem area.
- Use Print Statements: Inserting print statements helps in tracking the flow of your program. This method is effective for understanding what parts of your code are executing. You can display the values of variables at critical points to see how they change.
- Leverage the Debugger: Python’s built-in debugger () can step through the program execution and allow for interactive inspections of variable states. Understanding where things go wrong at runtime becomes easier with this tool.
- Check Dependencies: Ensure that your libraries and modules are correctly installed. Use pip to confirm installation and resolve any version inconsistencies.
- Seek Help Online: Communities like Stack Overflow and subreddit r/learnPython are invaluable resources. Engaging with other programmers can provide fresh insights, additional assistance, and alternative solutions.
Utilizing a combination of identifying issues and employing reliable troubleshooting techniques significantly decreases the time spent on debugging, allowing for a more productive programming experience.
Embracing these methodologies is not just about finding solutions but also about enhancing your overall coding skills, leading to a more confident engagement with Python programming.
Best Practices for Running Python Programs
Learning how to run Python programs effectively encompasses more than just executing scripts. It involves understanding the best practices that can significantly enhance productivity and code maintainability. By adhering to these practices, you reduce the risk of errors, create more readable scripts, and make collaboration simpler when working in teams. In this section, we explore essential practices for organizing your code, structuring your scripts, and maintaining good documentation.
Code Organization and Structure
Organizing code is a critical aspect of writing Python programs (or any programming languages). A well-structured codebase enhances clarity, decreases the chances of errors, and improves overall maintainability. Here are significant elements to consider when organizing your Python code:
- Project Structure: Create a clear directory structure. Following the convention of separating source files, tests, and documentation can guide users through your project effectively. Utilizing a folder for tests makes it easier to locate and run unit tests and ensures clarity.
- File Naming: Use descriptive names for your scripts and packages. Opting for names that reflect functionality provides immediate insight into the script's role.
- Modularity: Break up large scripts into smaller modules. Functions should have single responsibilities. This makes code easier to read, maintain, and test.
- Consistent Style: Follow a consistent code style with PEP 8 as an example. This established standard encompasses spacing, indentation, and naming conventions, contributing to improved readability.
Maintaining such practices results in fewer errors and an improved experience for anyone who interacts with your code, enhancing both personal and collective productivity.
Documentation and Comments
Documentation plays an indispensable role in coding. Whether for future reference or for others working on the same code, clarity in documentation and thoughtful comments can be a project’s lifeline.
- Docstrings: Each module, class, and function should begin with a docstring explaining its purpose and basic usage. Docstrings make it easier to understand what a piece of code does without diving deep into its mechanics.
- Comments: Include inline comments judiciously to explain complex logic. Clarity is essential; while some comments state the obvious and are unnecessary, meaningful insights will help users understand your thought process during development.
- Changelog: Maintain a changelog to document modifications over time. This helps collaborators (or yourself) grasp what has changed and why, which contributes to effective version control.
Effective documentation and insightful comments build a foundation for professionals and enthusiasts alike, simplifying both current development and comprehension in the future.
The End and Future Directions
In the exciting world of Python programming, understanding how to run your programs effectively is just the starting point. Having gone thrugh various environments and methods to execute Python programs, it is evident that mastery of this skill set lays the groundwork for more advanced undertakes. This article's conclusion brings an opportunity alias forward looking considerations, further elevating your programming journey.
Summary of Key Takeaways
Throughout this comprehensive guide, several vital aspects have been underscored:
- Understanding different environments where Python code executes hosts valuable learnings.
- Awareness of everyday challenges and the common bugs you may encounter.
- The significance of debugging and testing frameworks that underscore clean, efficient code.
- Practical approaches towards efficient code organization and documentation reduce future headaches.
It is crucial for one to remember, running Python within various contexts affects how an application behaves.
The insights shared here will help learners face future programming challenges with a more equipped mindset. Practical experience in each of the discussed categories fortifies your readiness for Python's intricate capabilities.
Exploring Advanced Python Concepts
As you reflect on the content covered so far, what lies beneath these fundamentals is an expansive field of advanced Python concepts eagerly await your attention. Venture into topics like asynchronous programming, decorators, and iterators to enrich your understanding further. Each concept enables different programming paradigms that offer flexibility and efficiency.
Exploration could include focused studies on:
- The unique features of modules like .
- Advantages of using decorators for optimizing repeated coding tasks.
- Mastery of comprehension expressions for succinct and readable data lists.'
All of these advanced territories are integral slices of Python’s ecosystem. From here, the future beckons with possibilities that often leads toward robust and scalable projects, enhanced artificial intelligence capabilities, and more outstanding web development tasks. Engaging with resources like Wikipedia), Britannica, relevant subreddits on Reddit, and industry updates could be variables than nurture your intelligent progression.
Continuous improvement and indeed lifelong learning engages with the broader developer community, help accelerate your evolution as a proficient Python programmer.
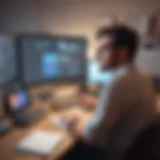
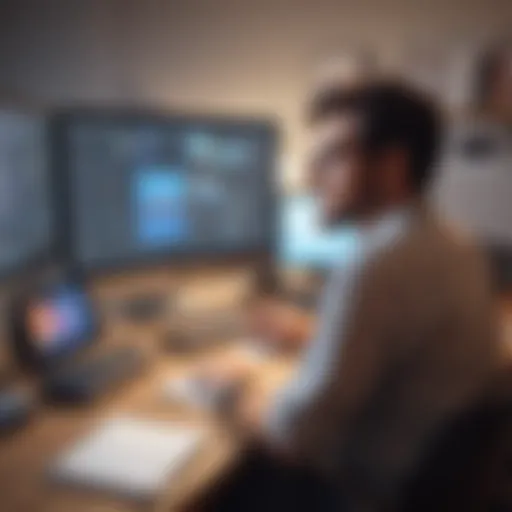