Ultimate Guide to Building a React Native App
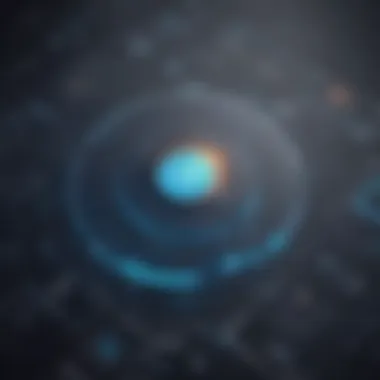
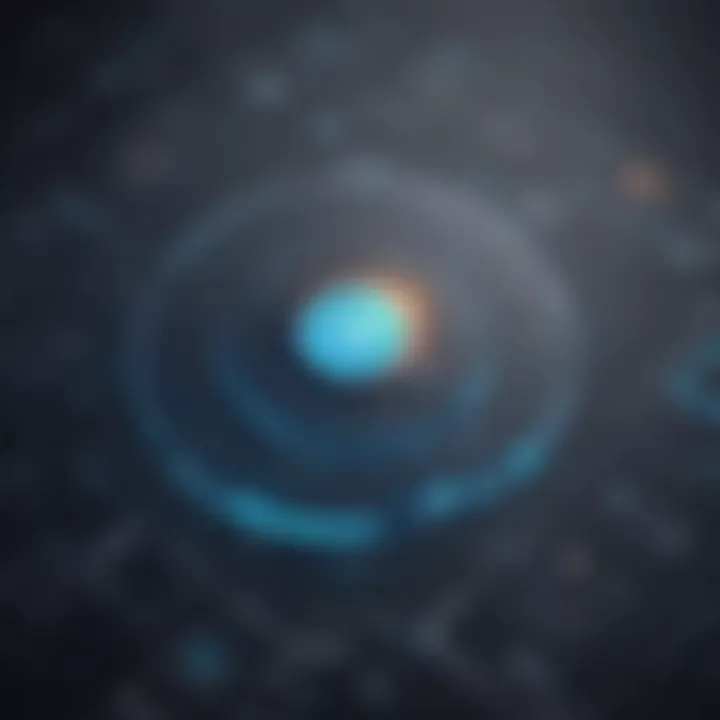
Introduction to React Native Development
In delving into the realm of creating a React Native app, one must first grasp the primary concepts surrounding this versatile framework. Developed by Facebook, React Native enables the building of mobile applications using JavaScript and React. Its cross-platform nature allows developers to craft applications that work seamlessly on both Android and iOS platforms, optimizing efficiency and reducing the need for separate codebases. This introduction serves as a foundational stepping stone to comprehend the intricacies and advantages of utilizing React Native in app development.
History and Background of React Native
React Native was first introduced by Facebook in 2015, revolutionizing the approach to mobile app development. Its inception aimed to provide a solution for building cross-platform applications without compromising performance or user experience. By leveraging the power of JavaScript and React, React Native empowers developers to create sophisticated apps with a focus on code reusability and time efficiency.
Features and Uses
The key features of React Native lie in its ability to streamline the app development process by offering a single codebase for multiple platforms. This not only simplifies maintenance but also enhances consistency across different devices. Additionally, React Native supports third-party plugins, granting developers access to an extensive range of functionalities to enrich their applications. Its flexibility and responsiveness make it a preferred choice for organizations seeking rapid app development without compromising on quality.
Popularity and Scope
Over the years, React Native has garnered substantial popularity within the developer community due to its scalability and performance advantages. Its robust framework accommodates a wide range of applications, from social media platforms to e-commerce ventures. With tech giants like Instagram, Tesla, and Airbnb incorporating React Native into their app development strategies, its prominence continues to soar, solidifying its position as a leading framework for cross-platform app development.
Introduction
Creating a React Native app is a significant milestone in mobile app development. It revolutionizes the way applications are built by offering a single codebase for both iOS and Android platforms. This section lays the groundwork for understanding the intricacies of React Native, from its core concepts to the advantages it brings to developers of all levels.
Understanding React Native
Exploring the fundamentals of React Native
React Native's core foundation lies in its ability to create native-like experiences using JavaScript. By leveraging native components, React Native bridges the gap between web and mobile development. Its efficiency in rendering UI components and native modules make it a compelling choice for app development. Despite some minor performance gaps compared to fully native apps, React Native's rapid development capabilities and extensive community support outweigh these limitations.
Benefits of using React Native for app development
The primary allure of React Native is its cross-platform compatibility, enabling developers to write code once and deploy it on multiple platforms. This significantly reduces development time and costs. Additionally, React Native allows for hot reloading, facilitating real-time code changes without recompilation. Its modular architecture promotes code reusability and ease of maintenance, making it a preferred framework for app development.
Setting Up the Development Environment
Installing Node.js and npm
Node.js and npm serve as the foundation for React Native development. Node.js provides a runtime environment for executing JavaScript code outside a web browser, while npm manages project dependencies. Installing these tools is crucial for setting up the development environment and ensuring seamless integration with React Native.
Setting up React Native
The React Native Command Line Interface (CLI) is instrumental in creating, building, and deploying React Native projects. It provides developers with essential commands to initialize new projects, run emulators, and bundle assets efficiently. Configuring the CLI streamlines the development process and enhances project organization.
Emulator configuration
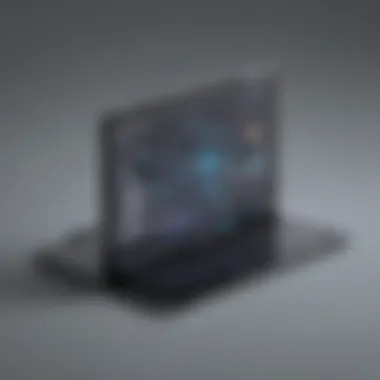
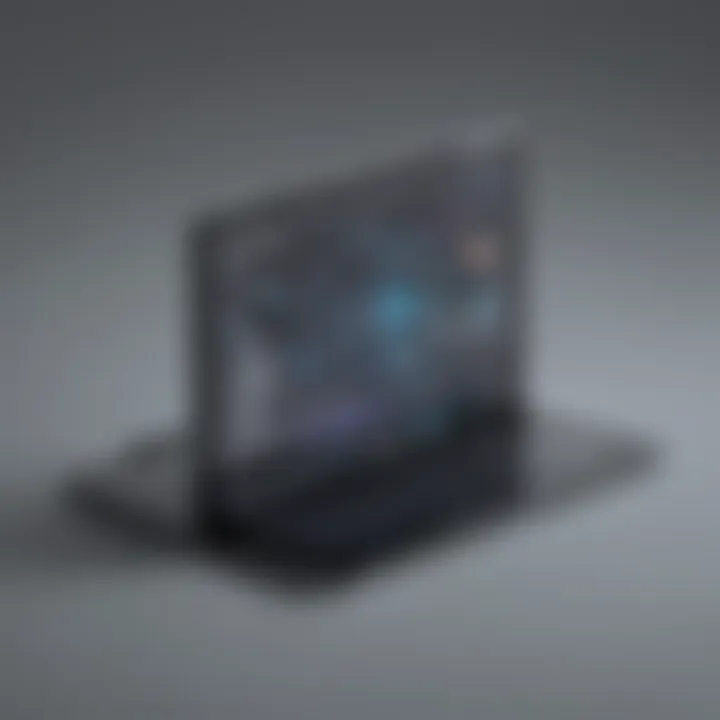
Emulators simulate mobile devices on desktop computers, allowing developers to test and debug their applications before deployment. Configuring emulators for both iOS and Android platforms is essential for cross-platform app development. By setting up emulators correctly, developers can ensure consistent performance across different devices.
Creating a New React Native Project
Initializing a new project
Creating a new React Native project initiates the app development process. This involves setting up the project structure, configuring dependencies, and establishing the foundational components. The initialization phase sets the groundwork for coding functionalities and designing the app interface.
Project structure overview
Understanding the project structure is crucial for efficient development and collaboration. React Native projects typically consist of directories for source code, assets, and configurations. By examining the project structure, developers gain insights into where different elements of the app are located and how they interact.
Building the App
In the process of developing a React Native app, the section on Building the App holds paramount significance. This segment serves as the fundamental core where various elements come together to shape the app. Building the App involves the meticulous crafting of components, implementing navigation for smooth user experience, and integrating data fetching functionalities. Each step plays a crucial role in ensuring the app's functionality and usability are optimized. By focusing on this essential aspect, developers can create a robust and user-friendly application that meets the desired objectives and user expectations.
Writing Components
Creating functional components
A pivotal aspect of app development, creating functional components forms the cornerstone of the app's structure. Functional components offer a streamlined approach to building user interface elements, promoting reusability and modularity within the app. By utilizing functional components, developers can efficiently manage and update different parts of the app without affecting the overall functionality. The key characteristic of creating functional components lies in their simplicity and flexibility, allowing developers to easily customize and adapt them to various requirements. This approach is a preferred choice in this article due to its scalability and maintainability, enabling developers to swiftly iterate and enhance the app's design and functionality.
Styling components with CSS
Styling components with CSS plays a significant role in enhancing the app's visual appeal and user experience. By applying CSS styles to components, developers can achieve a consistent and visually pleasing layout across the app. The key characteristic of styling components with CSS lies in its ability to separate the presentation layer from the app's logic, ensuring a clear distinction between design and functionality. This approach is favored in this article for its efficiency in creating responsive and aesthetically pleasing interfaces. However, a potential disadvantage of using CSS for styling is the complexity it may introduce when managing styles for multiple components, potentially leading to styling conflicts or performance issues within the app.
Implementing Navigation
Setting up navigation stack
The implementation of a navigation stack is crucial in defining the app's flow and structure, allowing users to navigate seamlessly between different screens and sections. Setting up a navigation stack involves organizing screens hierarchically, creating a logical flow for users to follow. The key characteristic of this approach is its ability to provide a well-defined navigation structure that enhances the overall user experience. This method is widely acclaimed for its simplicity and effectiveness in managing app navigation, making it an optimal choice for ensuring a cohesive and intuitive app design. However, one potential disadvantage of using a navigation stack is the added complexity it introduces, especially when dealing with nested navigation and complex app hierarchies.
Adding screens to the navigation
Expanding the navigation capabilities by adding screens to the navigation further enriches the app's usability and functionality. By incorporating additional screens, developers can create a more comprehensive user journey, providing access to distinct features and content within the app. The key characteristic of this approach is its versatility in accommodating diverse user interactions and scenarios. Adding screens to the navigation is a fundamental step in creating a seamless and engaging user experience, allowing users to explore different app sections easily. However, a potential disadvantage of this practice is the risk of overwhelming users with too many screen options, potentially leading to navigation confusion and reduced user engagement.
Fetching Data
Using APIs to fetch data
Integrating APIs to fetch data is essential for enabling real-time information retrieval and content updates within the app. By fetching data from external sources through APIs, developers can dynamically populate app content, ensuring fresh and relevant information for users. The key characteristic of using APIs for data fetching lies in its ability to establish a connection between the app and external databases or services, facilitating seamless data transmission. This practice is widely favored for its efficiency and scalability, allowing developers to access a wide range of data resources to enrich the app's content. However, a potential disadvantage of relying on APIs for data fetching is the dependency on external services, which may introduce latency issues or data inconsistencies based on API performance.
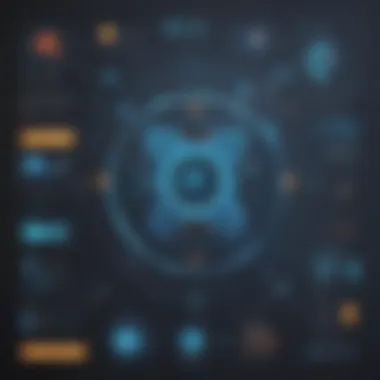
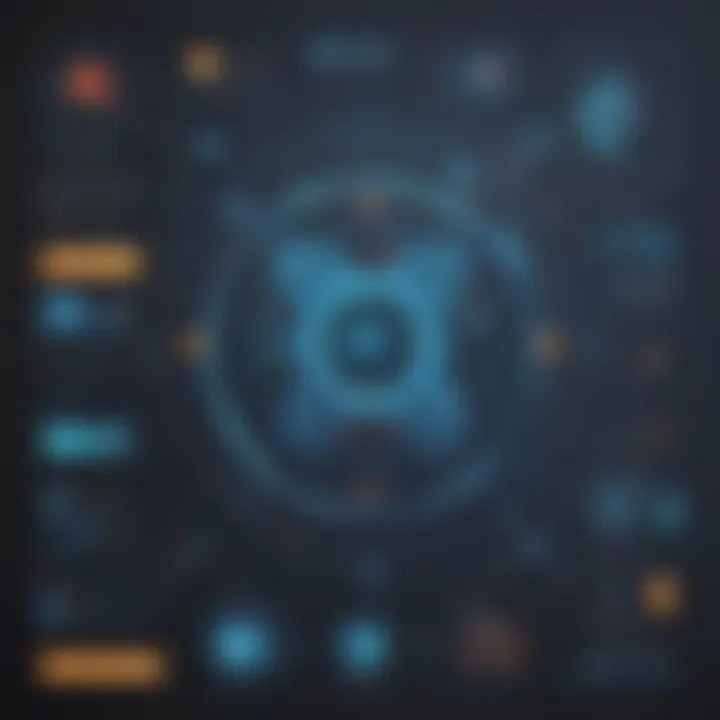
State management with React hooks
Effective state management is crucial for maintaining the app's state and ensuring data consistency throughout different app components. By utilizing React hooks for state management, developers can seamlessly handle component states and data updates, improving app responsiveness and overall performance. The key characteristic of state management with React hooks is its simplicity and flexibility, enabling developers to manage varying states efficiently. This approach is highly beneficial in this article for its ability to streamline state handling and data flow within the app, leading to a more coherent and organized development process. However, one potential disadvantage of state management with React hooks is the learning curve associated with understanding and implementing hooks effectively, which may require additional time and effort for developers to master.
Testing and Debugging
Testing and debugging are crucial aspects in the process of creating a React Native app as they ensure the functionality and quality of the application. In this article, we emphasize the significance of thorough testing and effective debugging techniques to streamline the development process and deliver a reliable end product.
Testing the App
Unit testing with Jest
Unit testing with Jest plays a pivotal role in ensuring the individual components of the React Native app function as intended. Jest, a popular testing framework, simplifies the testing process by providing a comprehensive suite of testing utilities. Its ability to run tests in parallel enhances efficiency, enabling developers to identify and rectify bugs or issues promptly.
Integration testing with Detox
Integration testing with Detox adds another layer of testing, focusing on the interaction between different components of the app. Detox's unique capability to simulate user interactions and behaviors aids in validating the seamless flow and integration of various modules. This robust testing methodology helps in identifying integration bugs early in the development cycle, ensuring a more stable and functional application.
Debugging Techniques
Using React Native Debugger
Employing React Native Debugger simplifies the debugging process by providing a centralized platform to inspect and debug React Native apps. Its user-friendly interface offers real-time analysis of app state, network requests, and component hierarchies, facilitating efficient bug identification and resolution. The integration of Redux DevTools further enhances the debugging experience, enabling developers to trace state changes and enhance overall app stability.
Troubleshooting common issues
Troubleshooting common issues involves a systematic approach to identifying and resolving prevalent issues encountered during app development. By leveraging best practices and diagnostic tools, developers can efficiently troubleshoot issues related to performance, compatibility, or functionality. Addressing common issues proactively ensures a smoother development process and minimizes unforeseen challenges during app deployment.
Optimizing Performance
Optimizing performance is a critical aspect when developing a React Native app. By focusing on enhancing efficiency and speed, developers can ensure a seamless user experience. In this section, we will delve into various key elements to consider for optimizing performance in the context of this comprehensive guide. Through optimizing performance, developers can significantly improve app responsiveness and overall user satisfaction.
Improving App Performance
Optimizing images and assets
Optimizing images and assets plays a pivotal role in boosting app performance. By compressing images, choosing the right file formats, and scaling assets appropriately, developers can reduce loading times and enhance overall app speed. This approach not only enhances user experience but also optimizes storage space and decreases bandwidth consumption. The strategic optimization of images and assets is essential for creating a high-performing React Native app, ensuring swift rendering and seamless interactions.
Key characteristics of optimizing images and assets:
- Efficient utilization of image compression techniques
- Selection of suitable file formats like WebP for better performance
- Proper scaling of assets for various screen resolutions
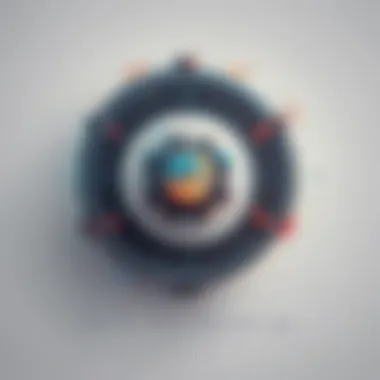
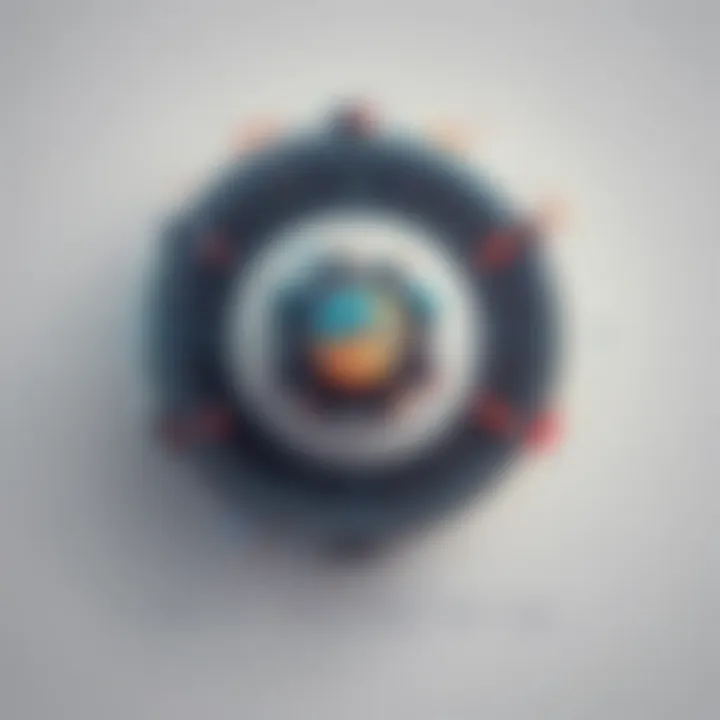
While optimizing images and assets offers notable benefits in terms of performance improvement, it requires careful consideration of the balance between image quality and file size. Developers must also ensure compatibility across different devices and screen sizes to maintain a consistent user experience.
Implementing code splitting
Implementing code splitting is a valuable strategy for improving app performance. By breaking down the application code into smaller chunks and loading only the necessary components on demand, developers can reduce initial loading times and enhance overall responsiveness. This approach helps in optimizing bundle size, improving app loading speed, and prioritizing critical functionalities for better user engagement. Implementing code splitting allows for more efficient resource utilization and can significantly enhance the overall performance of a React Native app.
Key characteristics of implementing code splitting:
- Efficient division of code into smaller modules for targeted loading
- Dynamic loading of code segments based on user interactions
- Better resource allocation for enhanced app performance
While implementing code splitting offers benefits in terms of performance optimization, it requires careful planning and implementation to ensure smooth navigation and seamless resource loading. Developers need to consider the app structure and user flow to effectively implement code splitting for maximum performance gains.
Deployment
In the realm of React Native app development, the deployment stage holds paramount importance. This section ensures that all the hard work put into creating and refining the app translates seamlessly into the hands of users worldwide. Deployment is the final step in the app development lifecycle, where the app is made available for download and usage. Understanding the intricacies of deployment is crucial for app creators as it dictates how users interact with their product. Without a smooth deployment process, even the most well-crafted app may fail to reach its intended audience, highlighting the significance of this phase in the development journey.
Preparing for Deployment
Generating APKIPA Files
Generating APKIPA files is a critical aspect of preparing an app for deployment. These files serve as the installation packages for Android and iOS devices, respectively. The process involves packaging the app code along with all necessary resources into a single file that can be easily installed on devices. One key characteristic of generating these files is the ability to create platform-specific packages, ensuring compatibility and optimal functioning on different operating systems. This choice is particularly valuable for this article because it streamlines the deployment process, enabling developers to reach a broader audience efficiently. The unique feature of generating APKIPA files lies in their self-contained nature, containing everything needed for the app to run independently, which can expedite the deployment process. However, a potential disadvantage may arise from the increased file size of these packages, which may impact download and installation times.
Configuring App Permissions
Configuring app permissions is another crucial aspect that developers must address when preparing for deployment. App permissions dictate what actions and data the app can access on the user's device, safeguarding user privacy and security. Understanding the key characteristic of app permissions involves balancing between providing necessary functionality and respecting user rights. This choice is essential for this article as it establishes a secure environment for the app to operate within, fostering trust with users. The unique feature of configuring app permissions is the granular control it offers over different aspects of the device, enhancing user experience and data protection. However, a drawback could be the potential user resistance if permissions are perceived as excessive or intrusive, highlighting the delicate balance developers must strike.
App Store Submission
Submitting the app to app stores marks the final phase of deployment, making the app accessible to a vast user base. This process involves adhering to the guidelines set by platforms such as the Apple App Store and Google Play Store to ensure the app meets quality and security standards. Creating an Apple Developer account is an essential aspect of app store submission for iOS apps, granting developers access to Apple's developer resources and enabling app distribution. This choice is beneficial for this article as it paves the way for app visibility and monetization on Apple's popular platform. The unique feature of creating an Apple Developer account lies in the personalized support and tools available, simplifying the app development and submission process. However, a disadvantage could be the associated costs of maintaining the developer account, especially for individual developers or small teams.
Submitting the App to Google Play Store
Submitting an app to Google Play Store is a critical step for Android app deployment, reaching millions of Android users worldwide. This process involves preparing the app listing, uploading the APK file, and complying with Google's policies and guidelines. The key characteristic of submitting an app to Google Play Store is the access to a vast global audience and the potential for app discovery and downloads. This choice is valuable for this article as it broadens the app's reach and facilitates user acquisition. The unique feature of submitting to Google Play Store is the robust analytics and marketing tools available, empowering developers to track app performance and optimize user engagement. However, a downside could be the stringent review process and competition on the platform, requiring developers to meet high standards for app approval.
Conclusion
In the realm of app development, the Conclusion serves as the pivotal point where all efforts and components merge into a coherent outcome. It plays a crucial role in the continuity and closure of the app-building process detailed in this comprehensive guide. By highlighting the significance of summarizing key steps, the Conclusion ensures that developers have a clear overview and understanding of their React Native app creation journey. It encapsulates the core essence of the entire developmental process and allows developers to reflect on their achievements and learning throughout.
Summary
Recap of key steps in creating a React Native app
Delving into the concept of Recap of key steps in creating a React Native app unveils a crucial aspect of this comprehensive guide. It acts as the linchpin that ties together various stages of app creation, offering developers a consolidated view of their progress and tasks accomplished. The Recap succinctly encapsulates the essential milestones in the app development journey, providing a concise yet comprehensive overview for developers to navigate through effortlessly.
A paramount characteristic of Recap of key steps in creating a React Native app is its ability to streamline the app development process, ensuring a systematic approach to coding, testing, and deployment. It serves as a roadmap that guides developers through intricate technical details, ensuring the smooth transition from ideation to a fully functional React Native application.
Moreover, the unique feature of Recap of key steps in creating a React Native app lies in its ability to condense complex development procedures into manageable and digestible segments. By breaking down the app creation process into key steps, developers can focus on individual tasks with precision, leading to an efficient and structured development workflow.
This approach not only enhances productivity but also facilitates better coordination among team members, fostering collaboration and optimized performance in app development projects. With its strategic guidance and actionable insights, Recap of key steps in creating a React Native app stands as a quintessential tool for developers embarking on their app development endeavors, equipping them with the necessary framework and knowledge to succeed in the competitive app market.