Mastering React JS: A Quick Learning Path
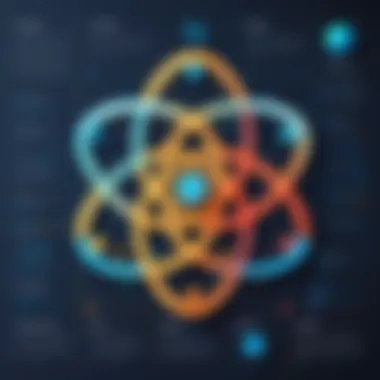
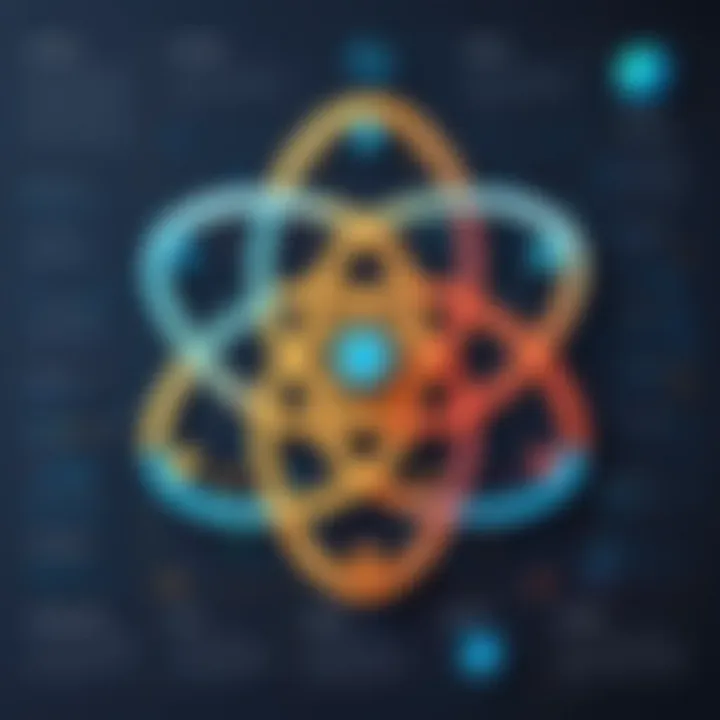
Intro
As the web development landscape continues to evolve, React JS has firmly established itself as a go-to library for building user interfaces, particularly single-page applications. It allows developers to create dynamic and responsive web applications with remarkable efficiency.
Why Learn React?
Learning React is not just a checkbox for developers; it is a chance to elevate their skill set significantly. This library, maintained by Facebook, promotes the concept of reusable components, enabling developers to create complex interfaces more easily. That’s like building with LEGO blocks—each piece fits together seamlessly, allowing for quicker adjustments and updates.
In this guide, we'll embark on a journey that delves into effective strategies and resources for mastering React JS swiftly. We'll discuss foundational concepts, essential tools, and practical applications, laying the groundwork for a fruitful learning experience.
What to Expect
This article will cover:
- Key Concepts: Fundamental principles that lay the groundwork for understanding React.
- Resources: Recommended tools and courses to enhance your learning.
- Hands-On Practices: Simple programs and projects to solidify your knowledge.
- Community Support: The importance of engaging with fellow learners and experienced developers.
By synthesizing the information throughout this guide, you will gain valuable insight into not only the mechanics of React JS but also how it integrates into the broader ecosystem of web development.
Understanding React JS
Understanding React JS is essential for anyone looking to delve into the world of web development. It’s not just a library; it’s like the backbone for building user interfaces that are efficient and scalable. As you read through this article, grasping the nuances of React can unlock doors to dynamic web applications that provide smooth user experiences.
React has gained traction and recognition among developers due to its simplicity and power. Its focus on component-based architecture allows programmers to build large-scale applications efficiently. These components can be reused, which narrows down redundancy and saves time in development. Add to that its prowess in managing state and rendering updates seamlessly, and you begin to see why mastering React is pivotal.
What is React JS?
At its core, React JS is an open-source JavaScript library created by Facebook for building user interfaces. It allows developers to build web applications that can change data, without needing to reload the page, hence delivering a smooth experience for users. One distinguishing factor of React is its ability to operate in a declarative way, meaning it updates the user interface as needed rather than requiring the developer to manually manipulate the DOM.
The Evolution of React
React was introduced to the public in 2013. While its foundation is rooted in JavaScript, its evolution has spurred additional tools and libraries that work in harmony with it. For instance, the advent of React Native allows developers to create mobile applications utilizing the same principles of React. Each evolution builds on the strength of community feedback, driving the development of features and improvements that make React even more appealing.
Core Principles of React
Understanding the core principles of React is critical. These principles not only define how React operates but also influence how you, as a developer, will interact with it.
Component-Based Architecture
Component-Based Architecture is arguably the heart of React. It encourages developers to break down the user interface into smaller, manageable parts known as components. By doing so, each piece becomes self-contained with its logic and rendering capabilities. One key characteristic of this architecture is reusability; components can be utilized in various parts of the application without reinventing the wheel.
This leads to cleaner code and easier maintenance. However, managing dependencies and the communication between these components can pose challenges, especially in larger applications.
Virtual DOM Concept
The Virtual DOM is a lightweight representation of the actual DOM. Whenever there is a change in state, React updates this Virtual DOM first. After the updates, it determines which changes need to be reflected in the actual DOM and applies only those necessary changes. This selective update process boosts performance significantly since the costly process of directly updating the DOM is minimized. It allows for a more efficient rendering process, impacting user experience positively. However, understanding how it operates might require a bit of a learning curve for newcomers.
Declarative Syntax
Declarative Syntax in React allows developers to describe what the UI should look like for any given state. This is different than imperatively instructing the application on how to update the UI. With declarative syntax, you code in a more intuitive manner where you focus on what the UI should render, not how to do it step by step. This approach enhances readability and maintainability of code.
"Declarative programming allows more time to focus on the logic, rather than the mechanics."
In summary, grasping the fundamentals of React JS empowers you to build interactive and efficiently managed applications. As we progress through this guide, these principles will serve as the foundation upon which your React knowledge will grow.
Setting Up Your Development Environment
When embarking on your journey to master React JS, you might’ve heard the saying, "A craftsman is only as good as his tools." This couldn’t ring truer in the realm of web development. The right development environment is vital for setting the stage for an efficient and effective learning experience. Your development environment encompasses all the tools and technologies that help you write, test, and debug your code. Setting it up correctly can save you a heap of time and frustration, letting you focus on mastering the intricacies of React JS.
Choosing the Right Tools
Setting the right tools in place is half the battle won. Here, we’ll delve into the essential components of a development environment, where key tools make a significant difference.
Code Editors
Code editors serve as your primary workspace, and choosing the right one can really enhance your coding experience. A popular choice for many React developers is Visual Studio Code. One of its main features is its robust extension marketplace that allows you to add functionality tailored to your needs, such as linting and code formatting.
The standout characteristic of Visual Studio Code is its intuitive user interface, which is approachable whether you’re new or have some experience. It’s packed with features like auto-completion and debugging support. However, it can sometimes feel a bit overwhelming due to its extensive settings and features, which might not be fully utilized by beginners.
Package Managers
When it comes to package managers, npm (Node Package Manager) stands out as an indispensable tool in the React ecosystem. npm helps you install, share, and manage dependencies in your projects. The great part is that it automatically tracks version updates and handles them deftly, reducing the headache of manual management.
One of npm’s most compelling features is its vast library of packages, providing countless options that can speed up your development process. However, new users might find the command line interface daunting, leading to some initial confusion.
Browser DevTools
The Developer Tools integrated into modern web browsers, like Chrome or Firefox, are crucial for debugging your React applications. With features like the Elements panel for inspecting HTML and the Console for testing JavaScript code, these tools offer invaluable insights.
A key advantage of Browser DevTools is its live editing capability, allowing you to tweak your code and see results in real-time, which can greatly accelerate your learning. However, the wealth of features can be overwhelming, especially if you’re just starting out.
Installing Node.js and npm
Before you can dive into the world of React, you need to have Node.js and npm installed on your machine. Node.js is a JavaScript runtime that lets you execute JS code outside of the browser, while npm is the package manager that comes with Node.js. Together, they form the backbone of the React ecosystem, enabling you to manage libraries and run your applications with serious ease.
Creating Your First React App
Once your environment is set up, it’s time for the fun part: creating your first React application. This process can be both exhilarating and a tad intimidating, especially for newcomers.
Using Create React App
Create React App is a CLI tool that allows you to set up a new React project with just a single command. It handles configuration for you, enabling you to hit the ground running. The critical advantage of using Create React App is that it reduces boilerplate, letting you focus on writing React components.
What makes it particularly popular is its built-in best practices — from simple testing setups to optimizations for production builds, you get a polished foundation right away. The initial setup simplicity comes at the cost of customization, which sometimes can be a bit restrictive later on if your project requires specialized configurations.
Understanding Project Structure
After you create a React app, it's essential to understand its file structure. The folders and files are organized in a manner that promotes modular coding practices. You will typically find a folder where your JavaScript files reside and a folder holding static assets.
A key feature of the project structure is component separation. By organizing your code files based on components, not only do you maintain clarity, but it also enhances reusability. However, newcomers may feel lost in navigating this structure at first, as it actively promotes a slightly different way of thinking about web development.
"A good development environment lays the groundwork for efficient learning. Choose wisely to make your journey smoother!"
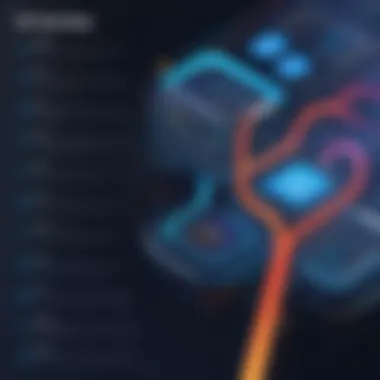
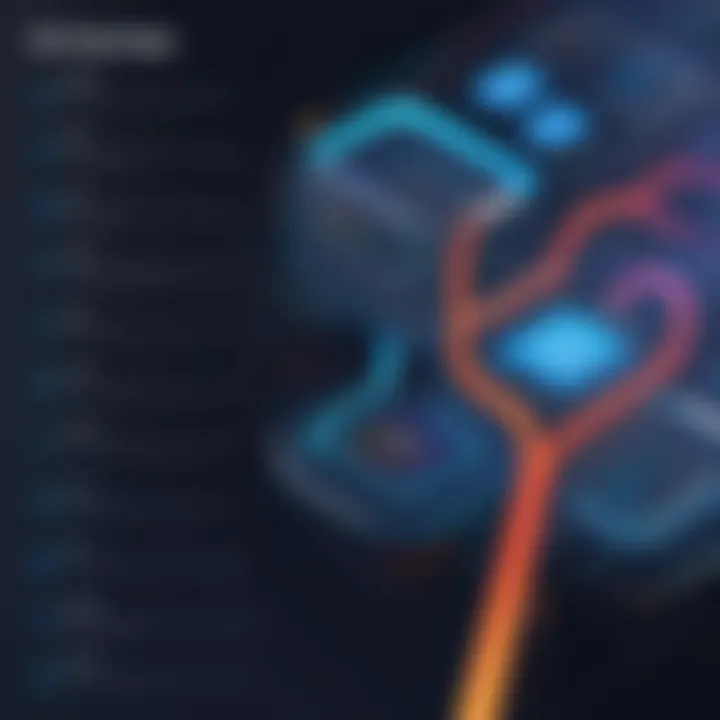
Your development environment is more than just a setup; it’s your foundation for all that you will create. With the right tools, you’re primed to elevate your React skills rapidly and effectively.
Learning Modern JavaScript
In the realm of web development, proficient understanding of Modern JavaScript is crucial for mastering React JS. As React is built on top of JavaScript, grasping its modern syntax and features ensures that learners can dive into the framework without feeling swamped. Getting a grasp on these specifics not only elevates coding efficiency but also enhances the readability and maintainability of code. Here, we will explore key elements of modern JavaScript, breaking them down to provide clarity and insights that are beneficial for both new and seasoned developers.
ES6 Syntax and Features
Arrow Functions
Arrow functions represent a succinct way of writing function expressions without the need for the traditional function keyword. One of the standout characteristics of arrow functions is their non-binding nature of . This means they inherit from the enclosing scope, allowing for more predictable outcomes in certain contexts, such as when a function is passed as an argument.
Additionally, arrow functions reduce the amount of boilerplate code, making them a popular choice for succinctness in React components or event handlers. For example:
While this concise syntax is great, there are specific scenarios where arrow functions may not always fit perfectly. For instance, when dealing with object methods that rely on their own , using traditional function expressions might be necessary instead.
Destructuring
Destructuring allows developers to unpack values from arrays or properties from objects into distinct variables. This is particularly useful when working with props in React, as it simplifies code and reduces redundancy. A key feature of destructuring is its ability to handle default values, which can streamline error handling and ensure that variables are initialized properly. Here's a quick example:
This approach not only enhances readability but also minimizes the chances of typos that could arise from repeatedly typing out object names. However, projects that involve deep nesting of objects may find destructuring to be slightly cumbersome at times.
Modules and Imports
In Modern JavaScript, the module system allows for organizing code into reusable pieces, promoting better structuring of applications. The syntax makes it easy to export functionalities from one file and import them into another. A prominent characteristic of this system is its support for both named and default exports, giving developers flexibility based on specific needs.
For example:
By leveraging modules, developers can keep their codebase clean and manageable. Yet, managing imports can sometimes become tedious, especially in larger applications where the structure might be complex, requiring careful attention to paths to avoid unresolved module imports.
Asynchronous Programming
Promises
Promises provide a modern solution to handling asynchronous operations, which are quite common in JavaScript. A promise represents a completed or failed asynchronous operation and its result, thus allowing for cleaner error handling and chaining of operations. What makes promises especially valuable in the context of React is their ability to work seamlessly with APIs, ensuring that applications can fetch data without blocking the UI.
However, creating promises can introduce additional complexity if not managed correctly. Errors in the promise chain can lead to silent failures if not handled properly, making it imperative for developers to include to address potential issues.
Async/Await
Async/await is a syntactical sugar on top of promises, allowing for more readable asynchronous code that resembles synchronous flow. This feature became a game-changer for many developers as it eliminates the cascading structure often seen with promises. Using async/await enhances the maintainability of the codebase, particularly when handling multiple asynchronous calls. Here's an example of its use:
Though async/await is generally user-friendly, it can sometimes be limiting, particularly if you're handling multiple asynchronous operations that need their results concurrently. In such cases, it can be beneficial to mix async with traditional promise methods for better control over execution flow.
Understanding Modern JavaScript is not just about learning new syntax; it’s about mastering the tools available to write efficient, maintainable, and scalable code.
Core React Concepts
The foundation of React JS lies in its core concepts, which are essential for mastering this powerful library. Understanding these principles allows developers to create efficient, maintainable, and scalable web applications. Core concepts such as components, state, and props are the building blocks that empower developers to effectively manage the flow of data and user interactions. Delving into these concepts provides clarity on how different aspects of React work together, ultimately enhancing the developer's expertise and productivity.
Components and Props
Functional vs Class Components
Functional components and class components are two fundamental ways to create React components. Functional components, as the name implies, are JavaScript functions that return JSX. They tend to be simpler and require less boilerplate code, making them a popular choice among developers, especially with the introduction of hooks. The key characteristic of functional components is their stateless nature; however, this has changed with hooks that enable local state management and side-effects. On the other hand, class components are more complex, involving ES6 classes and lifecycle methods. They allow for greater control over component behavior but come with a steeper learning curve. A noteworthy aspect is that functional components often lead to more readable and maintainable code, which is crucial for this article's emphasis on rapid learning.
Passing Data with Props
Props, or properties, are the primary means of passing data from one component to another in React. This is especially vital in maintaining a unidirectional flow of data. When discussing Props, a critical aspect is that they are read-only; thus, a component cannot alter its own props. This characteristic supports predictable component behavior. Passing data using props enhances reusability, allowing components to render dynamic content based on received data. The downside, however, is that deeply nested components may lead to excessive prop drilling—an issue where data must pass through multiple layers of components. Addressing this problem often falls under the scope of state management solutions.
State Management
Using State within Components
State represents the parts of the application that can change over time, thus reflecting that change on the UI. In this article, state management is vital as it influences how components interact dynamically. A key characteristic of using state is that it can only be managed within class components or functional components that utilize hooks. This capability lays the groundwork for responsive user interfaces. However, managing local state can become cumbersome in larger applications, making it crucial to grasp the benefits and limitations associated with state usage.
Lifting State Up
Lifting state up refers to the practice of moving shared state up to the nearest common ancestor of components that need to access that state. This approach ensures that the state is maintained in a single location, fostering easier data management. A notable aspect is that it unifies component behavior, reducing the potential for inconsistency across the UI. While lifting state can simplify state management, the challenge lies in determining the right level at which to lift state. Moreover, it may introduce additional complexity, since changes to the state must be communicated to multiple components.
Handling Events
Event handling in React is straightforward, yet it plays a crucial role in user interactivity. In fact, how components respond to user actions can significantly affect the overall user experience. React normalizes events as synthetic events, which means they conduct a consistent API across different browsers. This guarantees that developers can focus on functionality regardless of the underlying complexities of event handling in web browsers. Furthermore, the declarative nature of React allows developers to write clearer and more predictable event handling code.
"Mastering core React concepts is like learning the ABCs of web development with React; without them, reaching fluency in more advanced topics is nearly impossible."
In summary, honing in on these core React concepts empowers learners to create optimized and efficient applications, making their journey toward mastery more effective.
Working with React Hooks
Understanding React Hooks has turned into an essential part of mastering React JS. Hooks provide a way to use state and other React features without writing a class. This is a game changer for many developers, especially those looking to simplify their components and improve functionality without getting bogged down by component lifecycle methods. As a result, developers can focus more on how data flows through their applications rather than getting tangled up in class-based components.
React's introduction of hooks allows for a cleaner, more functional programming style, which many find more intuitive. Utilizing hooks can lead to cleaner code with less duplication, making maintenance and readability a breeze. There's a lot of buzz around using hooks, and as you dive deeper into this world, understanding their importance will undoubtedly heighten your coding efficiency.
Preamble to Hooks
Hooks were introduced in React 16.8 and have drastically changed how developers create components. Before hooks, handling state and side effects often resulted in cumbersome class components. React Hooks empower functional components, enabling them to manage state, lifecycle events, and side effects smoothly and effectively.
This transition to functional components is crucial because it allows for better code separation and a more modular design. Furthermore, hooks are called inside React component functions, which means they can seamlessly integrate with any React logic.
Commonly Used Hooks
A trio of hooks stand out for most React developers. Each serves a specific purpose yet works in harmony with the others to enhance application functionality. Let’s break down these hooks:
useState
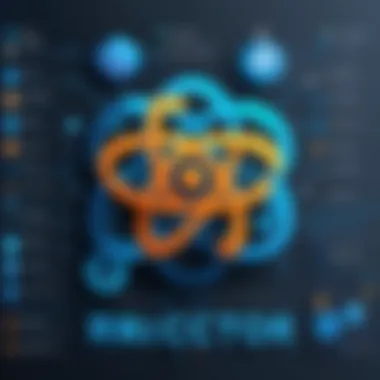
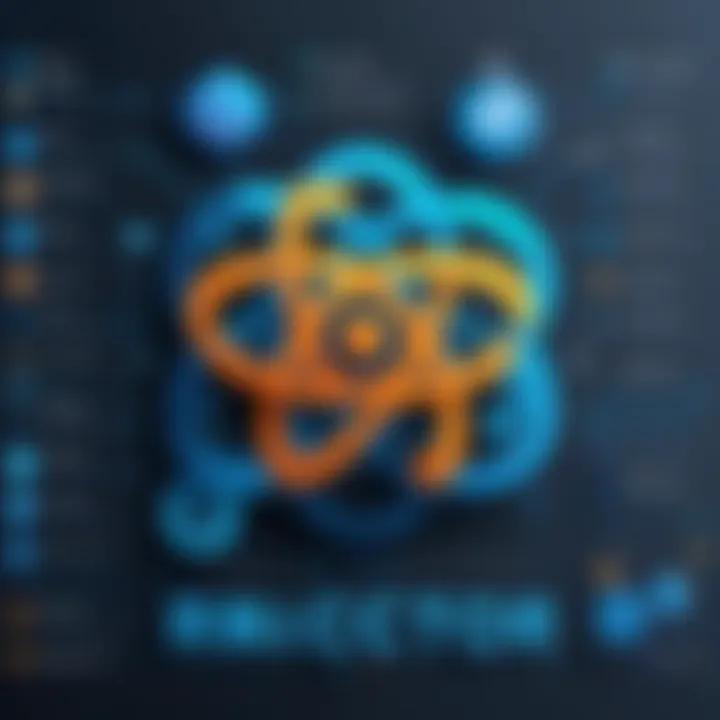
The hook is a foundational element for managing state in functional components. It allows you to add state to your component by calling it as a function. The key characteristic of is its simplicity; you can define state variables and update them within your components with ease. This aspect makes it a popular choice among developers willing to have a smoother, more concise coding experience.
Here’s how it looks in action:
This line initializes a state variable called to zero. You can easily change this state by calling the function.
A unique feature of is its ability to maintain the state across re-renders. This ensures that you’re not just working with stale values, a common pain point in class-based components.
However, a drawback is that managing complex state logic can make this hook less effective, requiring alternative solutions or additional hooks like .
useEffect
The hook is essential for handling side effects in your components. This might include data fetching, subscriptions, or manual DOM manipulations that don’t fit neatly into the component's main logic. A vital feature of is its dependency array, allowing you to specify when the effect should run, which can optimize performance significantly.
For instance:
This will execute the effect after the first render and each time the dependencies change.
On the flip side, a common mistake is mismanaging its dependency array, leading to inefficiencies and unnecessary re-renders.
useContext
The hook offers a way to access the context directly without having to wrap your components in the higher-order component. Its main advantage is that it simplifies accessing global data and passing state between components without prop drilling. This is particularly helpful in larger applications where managing state through props can become cumbersome.
Here’s how you can use it:
This single line can make your life much easier by accessing what you’ve set in the context without any hassle.
While can streamline state management, it has a caveat: if the context value changes, all components that consume the context will re-render. This can lead to performance issues if not managed properly.
Building Custom Hooks
Building custom hooks can be one of the satisfying parts of using React. They allow you to abstract away common logic that may be used across multiple components, promoting code reuse and cleaner architecture. If you find yourself writing similar logic in different components, turning that into a custom hook can save time and reduce complexity.
For example, if you had code to handle fetching data that appears in multiple places, you could create a hook. This makes your components leaner and focusses them on rendering, rather than managing data fetching logic directly.
The early adoption of hooks in your React projects not only gets you ahead of the curve but also aligns your skill set with modern trends in web development. Embracing hooks might just be that secret sauce you need to take your React applications from good to outstanding!
Advanced Concepts
As learners delve further into React JS, encountering advanced concepts is unavoidable. These elements hold significance since they expand the utility and versatility of React applications. Understanding how to utilize advanced methodologies effectively can lead to more efficient coding practices and a deeper appreciation of the framework's capabilities. In essence, these concepts equip developers not only with practical skills but also with a strategic mindset to tackle complex challenges in web development.
Context API
The Context API serves a crucial role in state management for React applications. It allows data to be passed through the component tree without having to pass props down manually at every level. This becomes particularly useful in larger applications where prop drilling—the process of passing data through many layers—can lead to messy and hard-to-maintain code.
By utilizing the Context API, developers can seamlessly share values like user information or theme preferences across the application. One major advantage is that it avoids the headache of managing state in multiple locations. However, developers must be cautious. Overusing Context can lead to excessive re-renders, thereby harming performance.
React Router
Rounding out the advanced toolkit, React Router is the go-to library for handling routing in React applications. The ability to manage navigation and rendering of different components based on user interactions is essential, especially in single-page applications, where users expect instant transitions.
Implementing React Router allows developers to create routes that are easy to read and crawl, making installations of dynamic content straightforward. It enhances user experience by keeping the interactions smooth. Just bear in mind that React Router does introduce complexity that requires a solid grasp of both React fundamentals and the routing library itself.
Performance Optimization
Code Splitting
Code splitting is a performance optimization technique that enables developers to load only what is necessary for a specific part of their application. Instead of bundling the entire JavaScript code into a single file, code splitting helps break the code into smaller chunks. Each chunk can then load independently, which dramatically reduces initial loading times.
This is popular because it leads to a faster user experience and lower data usage, particularly on mobile devices. With tools like Webpack, achieving this is relatively straightforward, and it integrates well with the overall build process in React applications. However, developers must manage loading states effectively to prevent users from experiencing blank screens as chunks load.
Memoization Strategies
Memoization strategies are vital for enhancing performance, specifically in how components re-render in response to state changes. By storing the results of function calls and caching them based on input parameters, developers can avoid unnecessary recalculations. This can be particularly beneficial when working with heavy computations or rendering large lists.
Using hooks like and , React offers built-in ways to memoize values and callbacks easily. One of its attractive features is that it helps in maintaining clean and efficient functional components. Nonetheless, it's crucial to apply memoization wisely since excessive use can lead to added overhead, nullifying performance benefits.
"Embrace advanced concepts in React with an open mind. They provide the keys to unlock your project’s full potential."
In summation, exploring advanced concepts in React JS, such as the Context API, React Router, and Performance Optimization strategies like Code Splitting and Memoization, provides learners with the tools they need to build sophisticated applications. Understanding these principles can distinguish a novice developer from an expert one, all while making the coding process much more manageable and enjoyable.
Integrating External Libraries
Integrating external libraries into a React application can significantly elevate its functionality and maintainability. With a robust ecosystem, React allows developers to pick and choose libraries that best suit their project needs. This flexibility can lead to faster development cycles and more efficient code, ultimately resulting in better performance.
Using State Management Libraries
State management is a critical aspect of building scalable applications. When your app grows in size, managing states directly within components can become a tangled web that's hard to manage. This is where state management libraries shine, providing clear methods to centralize and manage application state.
Redux
Redux is a predictable state container that's become one of the cornerstones of state management in the React community. Its main characteristic is the store, which holds the entire state of the application in a single immutable object. This structure allows for very clear and centralized state management.
The key advantage of Redux lies in its middleware capabilities, which can handle asynchronous actions gracefully. This means if your app needs to fetch data from an API, Redux can process that flow seamlessly. However, one downside to Redux is its boilerplate code — it often requires a fair amount of setup that might seem overwhelming for newcomers. This can lead to what some users might experience as "Redux fatigue." Despite its challenges, the clarity and predictability that Redux brings makes it a prevalent choice.
MobX
MobX offers an alternative approach to state management, focusing primarily on observability. The unique feature of MobX comes from its reactivity: state updates are automatically propagated, minimizing the need for manual updates to the UI when state changes.
MobX is less verbose compared to Redux, making it easier for beginners to grasp. Its intuitive and low-boilerplate nature can be incredibly beneficial for small to medium-sized applications. However, with very large applications, some developers find MobX's implicit nature can lead to complex and less predictable state changes, making debugging a bit more difficult. Each library offers its own set of pros and cons, and the choice between Redux and MobX largely depends on the specific requirements of your applications.
Styling Your Application
Styling a React application can sometimes feel like herding cats. Different approaches can be applied to make your UI both appealing and manageable. Each has its own gusto, allowing versatility in design and development.
CSS Modules
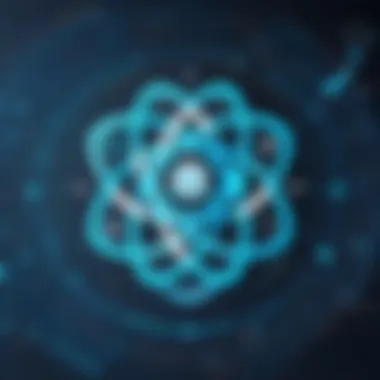
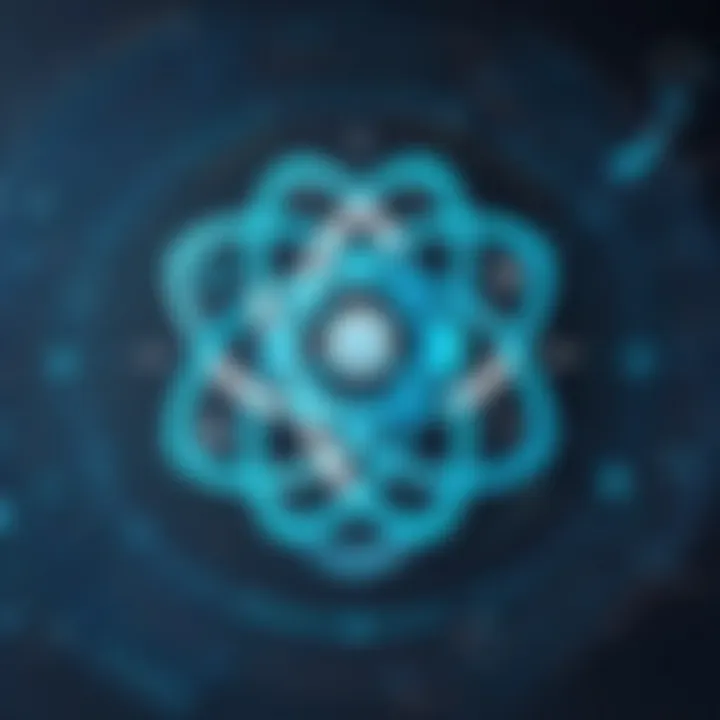
CSS Modules provide a way to scope styles locally without any global namespace conflicts. This means that styles defined in a CSS module are only relevant to the component it was imported into, drastically reducing the chances of interference from other styles. This is particularly beneficial in large applications where many developers might be working on various components simultaneously.
One of the unique strengths of CSS Modules is that it utilizes regular CSS syntax, helping those who are familiar with CSS to adapt quickly. However, the downside might present itself in terms of flexibility and ease of use of advanced features compared to other methods like Styled Components. They can also require a separate build step, which can complicate setup for less experienced developers.
Styled Components
Styled Components introduce a modern approach to styling by utilizing tagged template literals in JavaScript. This method allows you to style your components from within the same file where you define your component logic. It encapsulates the styles at the component level, so styles won't bleed into other components, which helps maintain clean code.
The key feature of Styled Components is its dynamic nature: you can pass props to the styled components and change the styles according to the component's state. This creates a seamless blend between UI and logic. However, for developers who prefer traditional CSS, the switch might seem jarring, as the syntax can take some getting used to. Furthermore, as your application grows, managing many styled components could lead to bloating, which is something a developer should keep an eye on.
Choosing the right libraries and styling techniques is crucial for maintaining clarity and performance in a React application. Each library and approach comes with its own set of strengths and trade-offs that developers must weigh according to the needs of their projects.
Building and Deploying Applications
Creating robust applications is not just about developing them efficiently; it’s equally about how you bring them to life in the real world. This section emphasizes the significance of building and deploying applications in the realm of React JS, exploring key aspects that every budding developer should grasp.
Building and deploying an application encompasses more than the mere completion of coding tasks. It requires a solid understanding of many varying processes, from effective testing to suitable hosting solutions and integration workflows. When handled accurately, these processes can mean the difference between an application that’s functional and one that’s truly user-ready. Moreover, robust deployment practices can pave the way for a smoother user experience, minimize downtime, and ultimately lead to greater user satisfaction.
Testing React Applications
Unit Testing with Jest
Unit testing serves as the first line of defense against bugs in your application. Choosing Jest for unit testing offers several advantages. One of its standout traits is its simplicity. Jest is designed specifically for testing JavaScript applications and integrates seamlessly with React, making it a go-to option for many developers. Its built-in assertion library allows for clear and concise tests, enhancing overall productivity.
A unique feature of Jest is its ability to run tests in parallel, significantly reducing test time. While that’s a great plus, it's also crucial to remember that the isolation of unit tests can sometimes lead to overlooked integration issues. Still, the benefit it brings in catching errors early in the development cycle cannot be overstated, ultimately leading to a more stable application.
Integration Testing with Testing Library
Integration testing takes the process a step further, ensuring that different modules of an application work together as expected. The Testing Library shines in this area, primarily due to its focus on testing user interactions rather than implementation details. This key characteristic makes it a popular choice for React developers aiming to simulate how a user would actually interact with the application.
The Testing Library’s unique feature is its ability to prioritize the user's perspective in tests. In this way, it helps ensure that the application behaves in a way that is intuitive and realistic. One disadvantage to consider, however, is that integration tests can often be more time-consuming to write and maintain. Nonetheless, their value in validating user workflows is indispensable in building reliable applications.
Deployment Strategies
Hosting Options
When it comes to deployment, selecting the right hosting service could make or break your application’s performance. The options are vast; services like Netlify, Vercel, or even cloud providers like AWS and Heroku offer unique features tailored for React applications. Choosing the right one depends on your specific needs and the traffic you expect.
One of the primary advantages of using platforms like Netlify is their streamlined deployment process—often as simple as pushing to a Git repository. However, this convenience comes with its own set of limitations in terms of customization. Therefore, contemplating your application’s scale and growth is paramount, as it could influence your choice of hosting long-term.
Continuous Integration Techniques
In the world of software development, Continuous Integration (CI) is essential for maintaining a high standard of code quality. Implementing CI techniques means automatically testing your code every time a change is made. This helps catch issues early, simplifying debugging and ensuring that every integration works seamlessly.
A pivotal characteristic of CI is its ability to streamline collaboration among team members. Developers can work in parallel without fear of stepping on each other's toes, as automated testing provides immediate feedback. Tools like CircleCI and GitHub Actions are often favored in this space due to their straightforward setup and integration capabilities. The downside? Setting up a good CI pipeline can sometimes feel like drinking from a fire hose, especially for newcomers. But once established, the benefits far outweigh the initial complexity, leading to a smooth deployment process and heightened application reliability.
Ongoing Learning and Resources
In the fast-paced world of web development, the journey does not end once you grasp the basics of React JS. The landscape of technology continues to evolve, and new practices, tools, and methodologies emerge regularly. This reality underscores the significance of ongoing learning and maintaining resources to stay abreast of trends and updates. Engaging with the right materials and communities allows developers to enhance their skills continually, adapt to changes, and become more proficient in building robust applications.
When considering ongoing learning, one must take a multi-faceted approach that combines structured education, community engagement, and real-world application. Grabbing hold of varied resources helps in developing a well-rounded skill set, and the benefits are vast:
- Enhanced problem-solving skills through exposure to diverse coding styles and methodologies.
- An increased network of like-minded individuals who can offer support, share experiences, and foster collaboration.
- Access to updated knowledge and practices that keep your skills relevant in a competitive market.
In this section, we will discuss essential avenues for ongoing learning that can provide you with continuous growth opportunities in the realm of React JS.
Recommended Books and Online Courses
Books and online courses serve as foundational resources for anyone serious about mastering React JS. They not only introduce you to core principles but also dive deep into advanced topics, providing a comprehensive understanding.
Some remarkable books include:
- "Learning React: Functional Web Development with React and Redux" by Alex Banks and Eve Porcello encompasses both concepts and practical exercises that reinforce learning.
- "React Up & Running: Building Web Applications" by Stoyan Stefanov offers a solid blend of theory with hands-on examples.
As for online courses, platforms like Udemy, Coursera, and freeCodeCamp provide structured learning paths, often created by industry professionals. These courses can range from beginner-friendly introductions to in-depth explorations of advanced topics. The choice of a book or course often depends on your personal learning style and preferred pacing.
Community and Networking
Joining Online Forums
Online forums play an important role in the learning ecosystem. They provide a space where learners can ask questions, share knowledge, and resolve doubts collaboratively. The key characteristic of online forums, such as reddit.com, is the communal support mechanism that empowers users to help each other. This community-driven aspect makes it a beneficial choice for those looking to overcome hurdles or deepen their understanding of React JS concepts.
Forums like these offer unique opportunities:
- Immediate answers to technical queries, often from experienced developers.
- Real-world perspectives on common issues encountered during development.
- A sense of belonging to a community with shared interests, which can ultimately enhance your learning experience.
However, it's essential to engage critically with the information shared. Not every piece of advice might suit your particular situation.
Participating in Meetups
Participating in meetups adds a personal touch to the learning experience. Face-to-face interactions with peers and experts can significantly enhance your understanding. The characterizing aspect of meetups is the opportunity to learn from each other's experiences. They are often beneficial for building networks that could lead to collaborations and job opportunities.
Meetups often feature:
- Workshops that provide hands-on experiences guided by knowledgeable hosts.
- Panel discussions where experts share insights and answer questions in real-time.
- The chance to forge connections that can extend beyond just learning, potentially leading to mentorship.
That said, attending meetups can sometimes require time management considerations, especially if you’re juggling work or other commitments.
Staying Updated with Trends
In technology, being up-to-date with trends and new features is vital. Continuous learning isn't just about understanding what you already know, but also keeping pace with what’s new. Two effective strategies here are:
Following Influencers
Following influencers in the React JS community can be an excellent way to stay informed about the latest trends. Influencers share insights, tips, and updates about new features or best practices via blogs and social media platforms. A standout feature of this approach is the accessibility to expert opinions, which continually shapes your knowledge.
However, it’s crucial to ensure the influencers you follow are credible and knowledgeable in their field, as misinformation can mistakenly lead you astray.
Subscribing to Newsletters
Subscribing to newsletters is another straightforward yet powerful method of receiving curated content. Newsletters often aggregate information from multiple sources and deliver it straight to your inbox, making it easier to digest. A notable characteristic of this approach is the varied perspectives on contemporary issues.
Some advantages of newsletters include:
- Convenience of having tailored content delivered, eliminating the need to scour multiple sites.
- The potential for discovering new tools and resources through recommendations in the newsletter.
Yet, it’s essential to avoid overwhelm by selecting newsletters that align closely with your interests and expertise level.
Ultimately, the goal of ongoing learning and leveraging resources is to cultivate a mindset that values knowledge as a continual journey rather than a destination. This approach empowers you to evolve alongside the rapidly changing technological landscape.