Coding the Snake Game in Python: A Comprehensive Guide
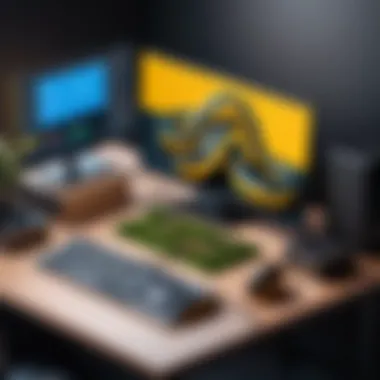
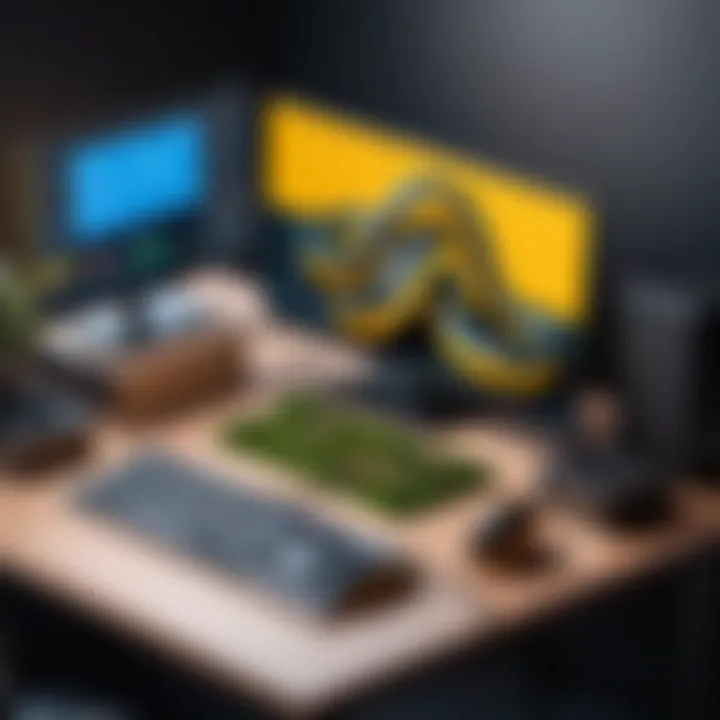
Intro
The journey into the world of programming often begins with practical projects that reinforce fundamental concepts. One such classic project is the Snake game, which offers more than just entertainment; it provides valuable insights into game development using Python. This article aims to guide you through the entire process of coding this timeless game, enabling both beginners and those with moderate experience to enhance their coding skills and understand core programming principles.
Intro to Programming Language
Python’s evolution is noteworthy and has made it a favorite for many. Born in the late 1980s, it was created by Guido van Rossum, with the intention of providing a language that emphasizes code readability. This design philosophy making Python a robust choice for various applications, ranging from web development to data science.
Features of Python:
- Easy Syntax: Python's syntax is clear and intuitive, making it accessible for novices.
- Versatile Libraries: A plethora of libraries support various functionalities, enhancing development efficiency.
- Strong Community Support: An active community ensures assistance and resources are readily available.
Given these attributes, Python’s popularity continues to surge. Currently, it stands as one of the most widely used programming languages worldwide, particularly favored in educational settings.
Basic Syntax and Concepts
Understanding basic syntax and concepts is crucial before diving into game development. Here are some essential topics you should familiarize yourself with:
Variables and Data Types
Variables are containers for storing data. In Python, you can define variables without specifying the type:
Understanding data types like integers, strings, and lists is important, as they influence how data is stored and processed.
Operators and Expressions
Python offers various operators—arithmetic, relational, and logical—allowing you to perform calculations and make decisions:
- Arithmetic Operators: , , ,
- Relational Operators: , , ``,
Control Structures
Control structures guide the program flow. Conditional statements (, ) and loops (, ) allow decision-making and repetitive actions, key components in game mechanics.
Advanced Topics
To create a more sophisticated game, you may encounter several advanced concepts:
Functions and Methods
Functions organize code into reusable blocks. For instance, creating a function to handle score updates keeps your code efficient and clean:
Object-Oriented Programming
Understanding classes and objects can greatly aid in game design. Through OOP, you can design complex entities like the snake or fruit as objects with specific properties and behaviors.
Exception Handling
When coding games, errors are inevitable. Using exception handling (, ) allows your game to manage errors gracefully, instead of crashing unexpectedly.
Hands-On Examples
To solidify your understanding, consider the following examples:
Simple Programs
A simple program that counts numbers can show basic loops and control structures. This lays the groundwork for understanding game loops.
Intermediate Projects
Building a small graphical program with Pygame can offer hands-on experience with libraries used in game development.
Code Snippets
Reviewing well-commented code snippets can illuminate how to implement specific features within your game.
Resources and Further Learning
For those eager to explore more:
- Recommended Books:
- Online Courses:
- Community Forums:
- "Automate the Boring Stuff with Python" by Al Sweigart
- Consider platforms like Coursera or edX for structured learning.
- Engage with fellow developers on Reddit or various Facebook groups dedicated to Python programming.
In the landscape of technology, the ability to program is becoming increasingly essential, with applications across industries.
By examining these topics, you will not only learn how to code the Snake game in Python but also derive skills that apply broadly in the programming field. As you progress, keep refining your techniques through practice and exploration, as this hands-on approach will solidify your understanding.
Prelude to the Snake Game
The Snake game holds a significant place in gaming history. While simple in design, it encapsulates essential programming and game development principles. This section will guide you through the fundamental aspects of the Snake game, its relevance, and the benefits of learning the mechanics involved in its creation.
History and Evolution
The origins of the Snake game trace back to the 1970s. Initially appearing in arcade formats, its now-familiar grid layout caught the attention of many players. Over the decades, various versions emerged on different platforms, from the early Nokia mobile phones to modern iterations on computers and gaming consoles. Each adaptation introduced new features, but the core gameplay remained unchanged: guiding the snake to consume items while avoiding self-collision and the game world boundaries.
Understanding this evolution not only offers insight into programming but also highlights how game mechanics can be translated across platforms and technologies. One of the earliest digital renditions of the game introduced players to the concept of collision detection and scorekeeping, two vital components in game programming. Today, the game's simple yet compelling concept serves as an ideal project for beginners looking to enhance their coding skills.
Why Learn Game Development?
Learning game development presents numerous advantages, especially for those with a basic understanding of programming. Firstly, it provides a practical application that reinforces programming concepts. For example, understanding loops and conditionals is crucial when developing a game.
Additionally, game development encourages problem-solving skills. As you create a game like Snake, you will encounter various challenges, which require innovative thinking to resolve.
Benefits of learning game development include:
- Hands-on experience: You engage directly with coding, making abstract concepts tangible.
- Creativity: Game development allows for personal expression through design, storytelling, and gameplay mechanics.
- Community engagement: The gaming community is vast and supportive, offering resources and feedback opportunities. Platforms like Reddit and dedicated forums can be excellent places to connect with other developers.
- Career opportunities: With the growing gaming industry, skills in game development can lead to exciting career paths.
"Game development fosters creativity and encourages innovative problem-solving skills that apply beyond just coding."
This foundational knowledge in game programming, particularly through a project like the Snake game, lays the groundwork for tackling more intricate game designs. Learning to code a game is not merely an exercise in programming; it is a way to cultivate a range of skills that are beneficial in multiple career paths.
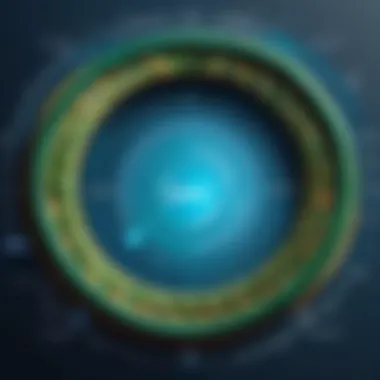
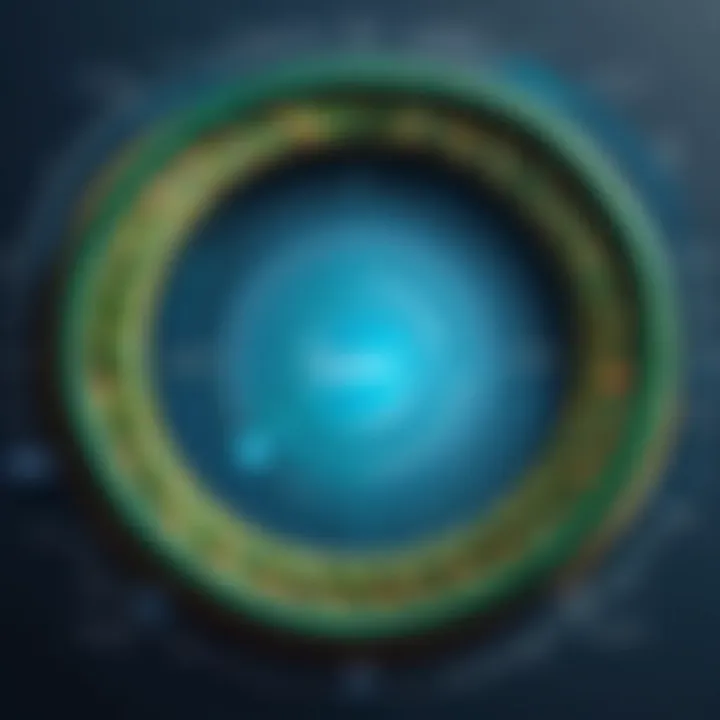
Setting Up Your Environment
Setting up your environment is a crucial first step in coding the Snake game in Python. This not only involves installing Python but also selecting an suitable Integrated Development Environment (IDE) for your coding needs. A well-prepared environment can significantly enhance your efficiency and productivity.
Having Python correctly installed ensures that you have the right tools to execute your code effectively. Moreover, a capable IDE can provide features like syntax highlighting, debugging tools, and project management capabilities. All these factors contribute to creating a seamless coding experience. Without these elements in place, you may run into unnecessary issues that can hinder your learning and development.
Installing Python
To begin coding, you must install Python on your computer. This is generally straightforward. Python is available for different operating systems, including Windows, MacOS, and Linux.
- Download Python: Go to the official Python website at python.org. Here, you will find the latest version available for download. Ensure you choose the version that fits your operating system.
- Run the Installer: After downloading, run the installer executable. If you're on Windows, make sure to check the box that says "Add Python to PATH" before you click install. This is vital to ensure that you can run Python from the command line.
- Verify Installation: After the installation is complete, open a command line or terminal window. Type to ensure Python has been installed correctly. You should see the version number displayed.
Choosing an Integrated Development Environment (IDE)
Selecting an appropriate IDE can make a significant difference in your coding experience. The right IDE will cater to your needs as you work on the Snake game and other projects in Python.
Popular IDEs for Python
Several IDEs have gained traction among Python developers for their capabilities. Some of the noteworthy options include:
- PyCharm: Known for its powerful features tailored specifically for Python development, including code analysis and integrated debugging.
- Visual Studio Code: A flexible and lightweight editor that supports multiple programming languages, making it a good choice for developers who may work outside Python.
- Jupyter Notebook: Ideal for data-centric applications, it allows for interactive code execution and data visualization in a single document.
Each of these IDEs has its distinct features, so consider your project needs when choosing one.
Configuring Your IDE
Once you have chosen an IDE, configuring it properly is next. A well-configured IDE will streamline your workflow and make coding more efficient.
- Set Up the Python Interpreter: Make sure your IDE is pointing to the version of Python you installed. Most IDEs have options in settings where you can specify the Python interpreter.
- Install Necessary Plugins: Depending on the IDE, consider installing plugins that enhance functionality, such as linters or version control systems.
- Customize Your Workspace: Adjust the interface to meet your preferences—this could involve changing themes, fonts, and layout to promote your coding comfort.
Configuring your IDE will help create an environment that is not only functional but also tailored to your personal working style. Proper configuration minimizes distractions and helps you focus on your code, enhancing your overall learning experience.
"A prepared environment is the foundation upon which great projects are built."
Understanding Game Logic
Understanding game logic is crucial in the context of game development. It establishes the rules and operations that govern how the game behaves. For Python's Snake game, mastering game logic enables developers to create a seamless experience for players. The logic involves the behavior of the game objects, interactions among them, and how the game state changes based on user inputs and events.
Game Loop Structure
The game loop is a fundamental part of any game. It is the cycle that keeps the game running. In essence, the loop continuously checks for user input, updates game state, and renders graphics. A well-structured game loop is vital for maintaining fluid gameplay.
A basic game loop for the Snake game can follow these steps:
- Initialization: Start by initializing all game parameters and loading assets such as graphics and sounds.
- Process Input: Capture player inputs, such as keyboard presses. This involves checking whether the control keys are activated.
- Update Game State: Here, the program updates positions of the snake and food. Collision detection, scoring, and state changes occur during this step.
- Render Graphics: After updating, the graphics are drawn on the screen, reflecting the current game state. This ensures the player sees the latest changes.
- Repeat: The loop continues until a game-over condition is met.
Implementing an effective game loop requires careful consideration of performance. An example of a simple loop in Python might look like this:
This structure allows for organized code, making it easier to manage and expand in the future if needed.
Managing Game State
Managing game state is about tracking the various conditions within the game. This includes the current score, the position of the snake, and whether the game has ended. It is essential for providing feedback to the player and determining actions like spawning food or growing the snake.
One common approach is to utilize a dictionary or class to maintain game properties. For example:
Tracking game state helps in sustaining player engagement. Players receive meaningful information about their performance and the challenges ahead. Without effective state management, a game can become confusing and frustrating, leading players to lose interest.
Creating Basic Game Components
Creating basic game components is fundamental in the development of the Snake game. This aspect focuses on essential elements such as the snake itself and the food it consumes. Understanding these components not only builds a strong foundation for the game but also enhances the player's experience. The snake is the player's main character while the food serves as a goal to achieve. Together, they create the core interaction of the game that keeps players engaged.
Drawing the Snake
The first critical step is drawing the snake on the screen. This requires an understanding of how to represent the snake visually. In Python, Pygame is often used for graphics, making tasks like this simpler. To initialize a snake, you can represent it as a collection of segments. Each segment can be a simple rectangle. When drawing the snake, the game's state dictates how long it is, which can change as it consumes food.
Important considerations in this process include color selection, size, and smoothness of movement. Consistency in these elements can create a more appealing and playable experience. To draw the snake, you can use the following code snippet in Pygame:
This will depict each section of the snake in a defined color. The coordinates and size of each rectangle depend on the current position of the segments. Careful management of these properties ensures the snake moves fluidly, enhancing gameplay.
Implementing Food Generation
After successfully drawing the snake, the next step is implementing food generation. Food is key in achieving higher scores and lengthening the snake. The food can be represented diversely, often as a colored square or circle. The placement of the food must be random but remain within the boundaries of the game area. This ensures the player has the opportunity to reach the food without it appearing outside the game screen.
When generating food, consider the following points:
- Random placement: Use the random module to ensure the food appears in various locations.
- Collision with the snake: The food location should check if it overlaps with the snake's position. If so, it should regenerate until it's in a free area.
- Significance of the score: Each food item consumed boosts the player's score. This can motivate players to keep playing.
Here’s a simple example to generate the food:
Incorporating these components seamlessly into gameplay is essential. The snake and food are not only visual elements but driving forces of interaction that lead to an engaging game. By mastering these components, the player experiences effective and responsive game mechanics.
Creating a game is about balance—functionality and aesthetics must work together for best results.
User Input and Controls
User input and controls are essential components in game development. They determine how players interact with the game and can greatly influence the gameplay experience. In a Snake game, the player's ability to control the snake's movement directly affects the game's strategy and outcome, making this topic critical for creating an engaging and responsive game.
Understanding user input ensures that the controls are intuitive. When players can easily navigate using the keyboard, they can focus more on the strategy rather than struggling with the mechanics. Properly implementing controls enhances user engagement, making the game more enjoyable and challenging. This section emphasizes the significance of user input and controls in the Snake game development process.
Handling Keyboard Input
Detecting Key Presses
Detecting key presses is a fundamental aspect of handling keyboard input in games. This process involves monitoring which keys are pressed by the user and reacting accordingly within the game. In the context of our Snake game, detecting key presses enables smooth control of the snake's movement. This responsiveness is what keeps players immersed in the gameplay, adding to the overall excitement.
A key characteristic of detecting key presses is its real-time capability. The game must constantly check for key inputs, allowing instantaneous reactions to player commands. This feature makes keyboard detection a popular choice for many game developers, including those creating the Snake game.
However, there can be challenges associated with detecting key presses. For instance, some key events might get missed if the game's frame rate is too low, leading to a frustrating experience for the user. Thus, it is important to ensure the game's loop runs efficiently to maximize responsiveness.
Mapping Controls for Movement
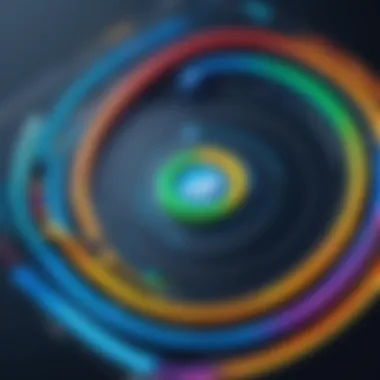
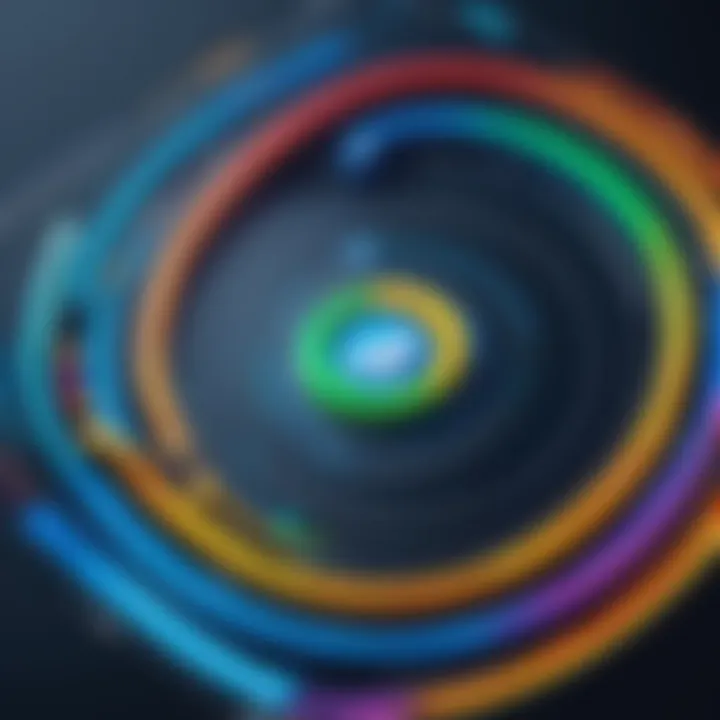
Mapping controls for movement defines how the detected key presses translate into actual movements within the game. In our Snake game, specific keys are assigned to control the snake's directions: up, down, left, and right. This mapping is vital for offering a clear and precise control scheme. It helps players quickly adapt and understand how to play, contributing to a more accessible gaming experience.
The mapping of controls is another critical aspect of user interface design. It ensures that commands are logical and consistent. For example, using the arrow keys to move the snake aligns with common expectations and provides a user-friendly approach.
One unique feature of mapping controls is the potential for customizability. Players might have different preferences for how they wish to control the snake. Allowing them to change control schemes can enhance their engagement. However, not providing default settings may lead to confusion for new players. Striking a balance between customizability and simplicity is important in design.
Responsive Gameplay
Responsive gameplay is the culmination of effective user input and controls. When implemented well, it ensures that players feel in control of their actions and can respond to the game's challenges seamlessly. This responsiveness is crucial in a fast-paced game like Snake, where split-second decisions can determine success or failure.
To achieve responsive gameplay, developers must consider the accuracy and timing of user inputs. The game should have minimal latency between the player's key press and the snake's movement on the screen. If players experience delays, it can lead to frustration and diminish enjoyment.
A well-designed user input system paves the way for responsive gameplay, creating a rewarding feedback loop where players feel as though their skills directly influence the game. Thus, mastering this topic allows developers to create a memorable gaming experience.
Rendering Graphics
Rendering graphics is a vital component in game development, especially when creating games like the Snake game. It is the part that gives life to the game, transforming mere code into a visually engaging experience for the player. Not only does it contribute to the aesthetics, but effective graphics also play a crucial role in gameplay. This section will delve into the importance of rendering graphics, illustrating its various elements, benefits, and considerations.
When players interact with a game, their experience is influenced heavily by the graphical representation of the game elements. The snake, the food, and the game environment must be clear and responsive. If the graphics are poorly rendered, players may feel frustrated, leading to a subpar gaming experience. The right visual appeal keeps the players engaged. It also helps in communicating vital information, like the position of the snake or how much food has been eaten, in a clear and concise manner.
Using Pygame for Graphics
Pygame is a timeless library in the Python ecosystem that simplifies the process of working with graphics. Its popularity stems from its ease of use and efficiency in handling 2D graphics. For anyone learning to code games, Pygame provides a straightforward and accessible entry point.
Setting up Pygame involves importing the library and initializing its modules. Below is an example of initializing Pygame:
Once initialized, Pygame allows you to create a screen, load images, and manipulate graphics. With it, developers can draw shapes, display images, and render text easily. The blitting feature in Pygame refers to copying the pixels of one surface to another, making it incredibly useful for rendering graphics efficiently in real-time.
You can create different game elements like the snake and food by loading images or drawing rectangles using Pygame's built-in functions. This library also handles the game loop seamlessly, making it easier to refresh the screen and update the graphics consistently.
Enhancing Visuals
While basic graphics might suffice to create a functional Snake game, enhancing visuals can significantly improve the overall experience.
Here are several strategies to elevate your game's graphics:
- Using Colors Wisely: Color schemes impact user engagement. A bright and contrasting color palette can help the player identify important elements instantly.
- Smooth Animations: Adding animations for the snake's movements enriches the visual experience. Simple transitions can make the gameplay feel more fluid.
- Background Design: Instead of a plain background, consider a thematic design that aligns with the game’s feel. An attractive background can set the mood for the gameplay.
- Iconography: Use symbols or icons to represent different features in the game. For example, you could design unique icons for different levels of difficulty which can enhance understanding and accessibility.
When enhancing visuals, it's essential to maintain a balance. Overly complicated graphics can detract from gameplay if it distracts players. Therefore, improvements should always focus on clarity and engagement without overwhelming the user's interface.
"Simplicity is the ultimate sophistication." - Leonardo da Vinci
Overall, rendering graphics in Pygame not just adds to the aesthetic appeal but also improves gameplay dynamics. By implementing effective visual strategies, developers can create an immersive and enjoyable gaming experience.
Implementing Collision Detection
Collision detection is a fundamental aspect of game design, especially in games like the Snake game, where it determines the outcome of player actions. Understanding how to implement collision detection effectively can greatly enhance the gameplay experience. It impacts not just how snakes interact with their environment, but also how scores are calculated and how players respond to game events. Proper collision handling creates realistic and engaging interactions within the game, thus transforming abstract movement into a vivid gaming experience.
Understanding Hitboxes
A hitbox is an invisible shape that defines the area of a game entity, such as the snake and the food. In simple terms, it is used to determine if two objects in the game have collided. For the Snake game, the hitbox can be considered a rectangular area that encloses both the snake and the food.
When designing a hitbox system, consider the following:
- Shape: Often a simple rectangle suffices. This allows for straightforward calculations when checking for intersects. However, if the snake has a more complex route, adjusting the hitbox shape can add nuance to the collision.
- Position: The hitbox must be accurately positioned relative to the graphical representation of the object it represents. Mismatched positions can lead to frustrating gameplay experiences as players might feel they are colliding even when visually they are not.
- Size: The dimensions of the hitbox should be proportional to the size of the game elements. A larger hitbox may lead to more forgiving collisions, while a smaller one requires precise movements.
- Implementation: This typically involves calculating whether two hitboxes intersect. Most programming environments, including Python with Pygame, provide functions for this task.
Understanding this mechanics is crucial. By setting appropriate hitboxes, you can ensure interactions feel natural and rewarding. As you refine these elements, the overall playability of your game will improve significantly.
Game Over Conditions
In any game, establishing clear game-over conditions is essential. For the Snake game, certain criteria must be present that determine when the game ends.
Typical game-over conditions in the Snake game include:
- Collision with walls: If the snake hits the boundary of the game area, it could signify the end of the game. You can implement this by checking the snake’s position coordinates against the defined boundaries.
- Collision with itself: This means if the snake's head intersects any part of its body, the game will end. This requires careful management of the segments of the snake, as each movement updates their positions.
- Lack of food: While less conventional, you might consider a condition where no food is available for an extended period as a game-over scenario.
Game over conditions add tension and challenge to the gameplay, compelling players to make quick decisions for survival.
When a game-over condition is met, it’s vital to handle it gracefully. This might involve displaying a message, recording the score, or giving players an option to restart. Clear feedback ensures players understand why the game has ended and encourages them to improve their future performance.
Scoring System and High Scores
The scoring system is a crucial element in any game, including the Snake game. It serves as a measure of player performance and a way to enhance engagement. A well-planned scoring system provides players with a tangible objective; they can see the results of their efforts. As they progress, their scores reflect their skills and strategies, which can motivate them to improve.
Calculating Scores
The basic premise for calculating scores in the Snake game revolves around the collection of food items. Each time the snake eats food, the score should increase. The score can be managed as a simple integer that adds a value each time food is consumed. For example, if the snake eats one unit of food, the score can increase by ten points. This scoring mechanism should be implemented within the game loop so that it updates the score in real-time without hindering gameplay.
Here's a simplified concept of how to calculate the score in Python:
This concise approach establishes the scoring framework. Low complexity in the calculation helps maintain a smooth gaming experience while keeping the main focus on the gameplay itself.
Storing and Displaying High Scores
Storing high scores is essential for encouraging player competition. Players often enjoy the challenge of beating their previous scores and competing against others. High scores can be stored in a file or database, enabling persistence across game sessions.
When displaying high scores, a simple sorting algorithm can help arrange scores from highest to lowest. This feedback loop encourages players to engage more deeply with the game. For displaying these scores, consider creating a dedicated screen that shows the top scores along with player names if applicable.
An example of managing high scores might look like this:
- Store Scores: Use a list or file to keep track of scores.
- Display Scores: Present the high scores when the game ends. This can be done using a simple text output or a graphical user interface that lists the top ten scores.
- When the current score exceeds the existing high scores, replace the lowest score accordingly.
"Effective scoring and high score management motivate players to hone their skills while enjoying the game experience."
To sum up, a robust scoring system and efficient high score management transform a simple game into an engaging challenge. They add depth and replayability, making players want to return and improve their performance.
Finalizing Game Features
Finalizing game features is a critical stage in game development. At this point, developers take what they have built and refine it to create a more engaging and polished gaming experience. This stage typically involves adding elements that enhance gameplay, such as levels, difficulty settings, and user interface components. These features serve to improve player retention, encourage engagement, and provide opportunities for skill development as the players progress.
Specific Elements
When finalizing features in your Snake game, consider implementing various levels and difficulty settings. Adding complexity through levels can keep players interested, as each stage can introduce new challenges or mechanics. Difficulty settings allow players to choose the level of challenge they are comfortable with, catering to both beginners and seasoned players. This customization of difficulty can lead to more profound enjoyment of the game.
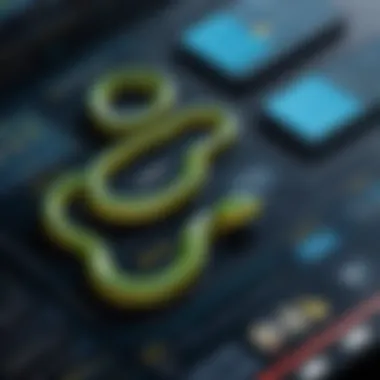
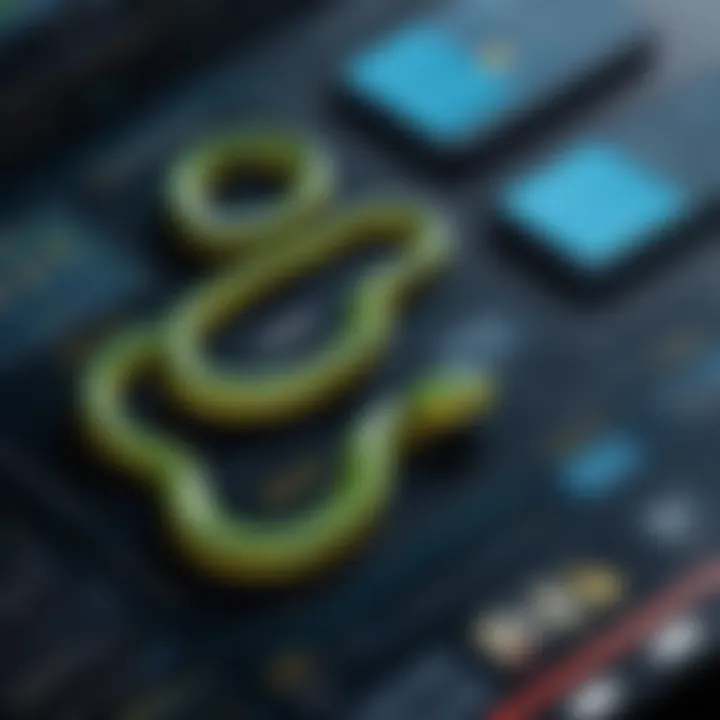
Benefits
The ability to adjust difficulty and features means that players will likely have a more personalized experience. If they are able to find enjoyment at their skill level, they may spend more time playing the game. Furthermore, adding levels can significantly impact the game's replayability. Levels that progressively become more difficult encourage players to improve their skills and push their limits.
Considerations about Finalizing Game Features
While finalizing these features, it’s essential to ensure that the scaling of difficulty feels natural. A sudden increase in difficulty can frustrate players rather than challenge them. A good approach is to gather feedback from testers at various points in development. This can provide insights on how players are experiencing the game, allowing for better adjustments.
Adding Levels and Difficulty
Adding levels and difficulty to your Snake game enriches the gameplay by creating a structured progression. As you develop each level, consider different mechanics or obstacles unique to that level to keep the game fresh. For instance, you could introduce faster food generation, or blocks that impede the snake's path as levels increase.
One common method of scaling difficulty is to increase the snake's speed after certain levels. This helps maintain player interest as the game becomes more fast-paced and challenging. Furthermore, utilize rewarding mechanisms, such as bonus points for completing a level, which also encourages players to keep trying until they master each stage.
User Interface Elements
A well-designed user interface enhances the player's interaction with the game. It presents crucial information clearly and succinctly. For your Snake game, consider integrating elements like a scoreboard, a timer, and a pause button.
- Scoreboard: Update the scoreboard dynamically to show current points in real-time. Informing players of their score motivates them to improve.
- Timer: A timer can add a sense of urgency, especially in timed levels, increasing the excitement.
- Pause Button: Allow players to pause the game, which is essential for those needing breaks or those who might be interrupted.
The layout should be intuitive. Use contrasting colors so that important information stands out. Avoid clutter to ensure that critical gameplay elements remain easily accessible. A clean user interface facilitates a smoother gaming experience, allowing players to focus on gameplay rather than navigating through confusing menus or screens.
Testing and Debugging
Testing and debugging are critical components in game development. They ensure that your Snake game functions correctly and provides a good user experience. Testing allows you to identify issues early in the development process, while debugging helps you fix those issues. The relationship between these two tasks is fundamental; without proper testing, bugs can go unnoticed, leading to potential crashes or gameplay issues.
In a game like Snake, several factors can influence performance and functionality. Ensuring that the snake moves smoothly, the collisions are detected accurately, and the food spawns correctly are just a few elements that require thorough testing. An organized testing strategy helps maintain a high quality of the game.
Common Issues and Solutions
While developing your Snake game, you may encounter various problems. Here are a few common issues and their solutions:
- Snake Movement: Sometimes, the snake might not move correctly. This could be due to misconfigured key bindings. Ensure that the key presses are being recognized correctly in your event loop.
- Food Spawning: If the food does not appear or spawns in the wrong location, check the logic that generates the coordinates for food. Ensure that they do not overlap with the snake's current position.
- Game Over Trigger: If the game over condition does not activate as expected, verify that the hitbox detection logic is accurate. You may need to recheck the boundaries where the snake collides with itself or the walls.
"Debugging is like being the detective in a crime movie where you are also the murderer."
— Unknown
Testing these scenarios thoroughly can help you catch issues before they reach the end-user.
Improving Performance
Improving the performance of your Snake game can significantly enhance user experience. Here are some ways to achieve better performance:
- Optimize the Game Loop: Ensure that your game loop runs efficiently. Avoid unnecessary calculations inside the loop. This will minimize frame drops and provide smoother gameplay.
- Limit Redraws: Only redraw the game screen when necessary. For instance, instead of redrawing all game elements each frame, consider only updating what changes, like the snake's position or the food.
- Efficient Resource Loading: Minimize loading times by ensuring that resources, such as images or sounds, are pre-loaded and managed efficiently.
By addressing common issues and focusing on performance improvements, you make your Snake game more enjoyable and reliable for the players. Through careful testing and debugging, you set a strong foundation for your completed game.
Publishing the Game
Publishing your Snake game is an essential step that takes your project from a personal endeavor to sharing it with a broader audience. This phase is not just about making the game available for others to play. It involves several decision-making processes and technical considerations that can significantly impact player engagement and reach.
For many, the learning journey culminates in the satisfaction of seeing others enjoy the fruits of your labor. Additionally, this experience can provide valuable feedback for future projects, enhancing your skills and understanding of game dynamics. To achieve this effectively, you must navigate through two main aspects: exporting your game and choosing the right platforms for sharing.
Exporting Your Game
When you reach this stage, it is crucial to package your game in a format that can be easily shared and run on other systems. Python, with its various libraries, allows you to create executable files that can run independently of the source code. Tools such as PyInstaller or cx_Freeze facilitate this process. These tools convert your scripts into stand-alone executables, simplifying distribution.
Here is a basic process of exporting your game:
- Choose a Packaging Tool: Select PyInstaller or cx_Freeze based on your project needs.
- Prepare Your Code: Ensure your code is free from errors and well-structured. This will help avoid complications during the packaging process.
- Follow Tool Instructions: Each tool has its syntax and commands; consult the official documentation for guidance.
- Test the Executable: After creating the executable, test it on different systems to ensure compatibility.
The export process can sometimes involve dependencies, so list these clearly. This makes it easier for others to install and play your game.
Sharing on Platforms
Identifying the right platforms for sharing your game significantly influences its visibility and reception. Several options are available, each with its strengths and weaknesses. Share your game through these popular avenues:
- Game Distribution Sites: Platforms like itch.io and Game Jolt specialize in indie games and provide a straightforward interface for uploading your game.
- Social Media: Utilize social media networks such as Facebook and Twitter to announce your game. They offer a direct way to engage with potential players and gather feedback.
- Forums and Communities: Engage with communities on Reddit or other forums dedicated to game development. Participants are often eager to play and provide constructive criticism.
“Sharing your work can be nerve-wracking, but it is also an opportunity for growth and connection.”
Remember to promote the distinctive features of your game when sharing it online. This will help attract an audience that appreciates what you've created. Also, consider incorporating feedback mechanisms. This approach helps foster community and allows you to refine your game based on player experiences.
Ultimately, publishing your Snake game goes beyond just making it available; it is about creating a platform for interaction and learning, both for you as a developer and for your players.
Further Learning Resources
In the realm of game development, the path to mastery is often bolstered significantly by consistent and enriching learning resources. As you venture into the world of coding the Snake game in Python, utilizing further learning resources is crucial. They provide new insights, optimize your skill development, and expand your understanding of both Python and game development principles.
With the foundational skills established through this guide, exploring supplemental materials can deepen your knowledge and offer new perspectives. Textbooks and online courses provide structured learning, while communities and forums present a platform for real-time interaction, fostering collaboration and aiding in problem-solving.
Books and Online Courses
Delving into books and online courses creates a rich substrate for skill enhancement. Books like Invent Your Own Computer Games with Python by Al Sweigart are particularly helpful. This title not only covers Python programming but also presents engaging projects that cultivate a practical understanding of game mechanics.
Online platforms such as Coursera and edX offer courses tailored to Python game development. For instance, courses focused on Pygame can enhance your understanding of rendering graphics and handling user input effectively. Consider the following elements as you seek further knowledge:
- Diversity of Topics: Engage with resources covering various aspects of game development, from coding principles to game design.
- Hands-On Projects: Look for courses that include practical projects. This approach helps solidify knowledge through application.
- Accessibility: Choose materials that cater to your level of expertise. Whether you’re a beginner or more advanced, there are resources that suit your needs.
Communities and Forums
Engaging with communities and forums plays an essential role in skill development and networking. Reddit, particularly the r/learnpython and r/gamedev subreddits, is an excellent place to ask questions, share experiences, and obtain feedback on your projects. The sense of community often encourages sharing knowledge and collaborative learning.
Another significant platform is Stack Overflow, where users can query specific programming challenges. Here, you can find tailored advice and solutions from experienced developers. Participation in forums offers numerous benefits:
- Problem Solving: You can seek immediate help on coding issues or game mechanics you face.
- Networking Opportunities: Interacting with fellow developers opens doors to collaborations and mentorship.
- Learning from Asks: Reading questions and answers from others can expose you to new concepts and coding strategies.
Investing time in further learning resources can significantly elevate your development skills and enrich your programming journey.
By consistently engaging with diverse educational materials and communities, you will cultivate a robust understanding of Python and game development, ultimately enriching your coding experience.
Closure
In this article, we have explored the entire process of coding the Snake game using Python. The importance of this topic is significant for those who seek to enhance their programming skills through practical application. By engaging with this project, learners not only grasp essential programming concepts but also experience the satisfaction of seeing their code come to life as a playable game.
Recap of Key Points
Throughout our journey, we covered several essential elements:
- Setting Up: We began with the proper installation of Python and ideal IDE choice. This foundation is crucial for smooth coding.
- Game Logic: Understanding the structure of a game loop and how to manage game state is fundamental to creating any game.
- Game Components: Learning how to draw the snake and implement food generation laid the groundwork for gameplay.
- User Input: We highlighted how to handle keyboard input effectively, ensuring responsive gameplay that keeps players engaged.
- Graphics Rendering: Utilizing Pygame for game graphics enhanced the visual aspects of our creation, making it more attractive.
- Collision Detection: Implementing hitboxes and stating game-over conditions are pivotal for a functionally sound game.
- Scoring System: Scoring systems and high scores motivate players, adding another layer to game engagement.
- Final Features: Adding levels, difficulty, and user interface elements improved player experience.
- Testing and Debugging: Addressing common issues ensured the game ran smoothly, which is vital for user satisfaction.
- Publishing: Finally, we delved into exporting and sharing the game on various platforms, allowing for wider reach and usability.
These components collectively illustrate how detailed and comprehensive game development can be. Each section is not just merely a step; it is an essential part of the puzzle that contributes to the overall gaming experience.
Encouragement for Future Projects
As you conclude this Snake game project, I encourage you to build upon what you have learned. The knowledge gained through coding and testing this game provides a robust framework to tackle more advanced projects in the future. Consider exploring different game genres or even expanding on this game with new features, such as multiplayer options or enhanced graphics.
Engaging with programming challenges, joining online forums like Reddit or Facebook to discuss, and seeking out additional resources can further fuel your development journey. Exploring books or online courses can offer new insights, and remaining curious will open doors to innovative programming solutions.
The world of game development is vast and full of opportunities. Keep practicing and applying your skills, and you will discover a rewarding path ahead.