Essential Python Selenium Interview Questions Explained
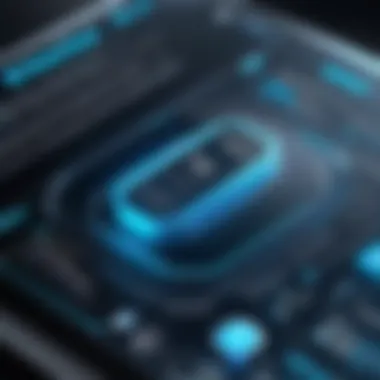
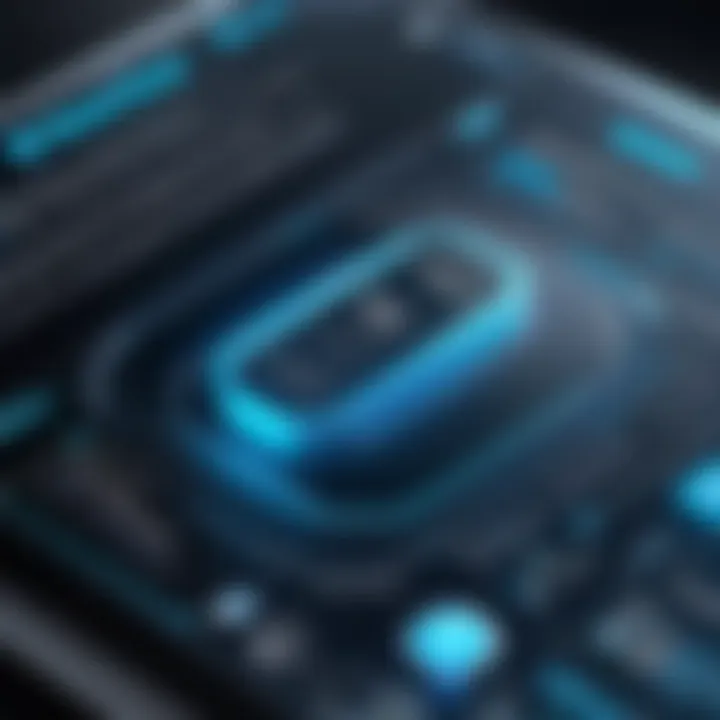
Prolusion to Programming Language
When it comes to automation testing and scraping data from the web, Python stands tall as a preferred programming language. Its clear syntax and robust libraries make it a wonderful choice for both seasoned developers and beginners. In this section, weāll delve into the interesting history, distinctive features, and the growing scope that Python brings to the table.
History and Background
Python was conceived in the late 1980s by Guido van Rossum and was first released in 1991. The idea was to create a language that emphasized code readability and simplicity. What began as a hobby project has burgeoned into one of the most popular programming languages in the world. Python's lineage traces back to the ABC language, influenced by a variety of other languages like C and Modula-3, to craft a versatile tool that has found its niche in many areas, including web development, scientific computing, and, notably, automated testing with Selenium.
Features and Uses
Python is renowned for its elegant syntax, which is not only beginner-friendly but also powerful enough for complex applications. Some standout features include:
- Dynamic Typing: Variables do not need an explicit declaration to reserve memory space, allowing for more flexibility in coding.
- Extensive Libraries: Python boasts a rich ecosystem of libraries and frameworks, like Selenium, Pandas, and NumPy, making it well-suited for various tasks.
- Cross-Platform Compatibility: You can run Python on different operating systems, which enhances its usability in diverse environments.
Its uses are far-reachingāranging from web applications with frameworks like Django to data analysis and machine learning with libraries like TensorFlow. For our focus, Pythonās role in automation testing with Selenium not only simplifies test scripts but also aids in the maintenance and scalability of testing frameworks.
Popularity and Scope
Pythonās growth is no mere flash in the pan. A peek into the statistics reveals a consistent upward trajectory in popularity across various industries. Itās a favored choice among developers, with a survey by Stack Overflow showing that over 40% of developers use Python, particularly for data science and web scraping. Additionally, its community contributes to an extensive pool of resources, ensuring learners always have help at hand.
The demand for Python skills continues to surge as more organizations adopt DevOps practices, integrating testing within their workflow. As such, understanding Selenium with Python is more crucial than ever for anyone seeking to make an impression during job interviews in software development.
āLanguage is the dress of thought.ā This reminds us that a clear, effective framework like Python, paired with tools such as Selenium, can significantly streamline the process of testing and automation.ā
As we venture deeper, we will explore the basic syntax and core concepts that form the foundation of Python programming, specifically tailored for utilizing Selenium effectively in various testing scenarios.
Understanding Python Selenium Basics
In the realm of automation testing and web scraping, grasping the fundamentals of Selenium with Python is crucial for any developer or aspiring software tester. This section lays the groundwork for understanding how these two powerful tools work in tandem. By diving into the basics, you trigger a chain reaction of knowledge that extends into deeper technical discussions and practical applications later in this guide.
When you're looking to write effective test scripts or automate web interactions, having a solid foundation in the principles and concepts of Selenium with Python is non-negotiable. Not only does this understanding empower you to troubleshoot issues effectively, but it also equips you with the skills to devise better solutions when faced with complex testing scenarios.
Prologue to Selenium
Selenium is an open-source framework designed primarily for web application testing. It allows developers to automate browser actions, simulating human behavior for the purpose of testing and validating web applications. The ease of automation with Selenium can save time and reduce error rates in manual testing. Its lightweight architecture supports various browsers and platforms, making it a go-to choice among testers.
Selenium is composed of several components which together create a versatile testing environment:
- Selenium WebDriver: This is a tool that allows you to programmatically control a web browser using various programming languages. It communicates directly with the browser, driving it through a series of commands.
- Selenium IDE: This is a Chrome and Firefox add-on that allows testers to record their interactions with the browser and convert them into reusable test scripts.
- Selenium Grid: This component is pivotal for running tests on multiple machines using different browsers concurrently, drastically speeding up the testing process.
With these tools at your disposal, automating testing becomes less of a chore and more of a strategic advantage.
Python and Selenium Integration
Pythonās integration with Selenium takes full advantage of Pythonās simplicity and ease-of-use. Python is renowned for its clear syntax, which makes writing and reading test scripts straightforward, even for those who may not have a deep programming background. This seamless collaboration introduces you to powerful libraries and frameworks that can expand Selenium's capabilities.
When you use Python with Selenium, you can leverage libraries like or to manage tests efficiently. This combination nurtures a thriving ecosystem where automated testing can flourish. Additionally, Python's strong community support provides ample resources, tutorials, and examples, making it easier to find assistance when needed.
Key Features of Selenium
Understanding the key features of Selenium is paramount for anyone aiming to work efficiently with this testing framework. Here are some standout characteristics:
- Cross-Browser Testing: Selenium supports multiple browsers, including Chrome, Firefox, and Safari, ensuring a wide range of compatibility across different platforms.
- Multi-Language Support: Although primarily used with Python, Selenium can also be utilized with Java, C#, Ruby, and more, thus catering to diverse programming environments.
- Dynamic Web Elements Handling: Selenium can interact effectively with elements that load dynamically, thanks to its locating techniques and waiting strategies.
- WebDriverās Robustness: The WebDriver API interacts directly with the browser, ensuring a more realistic user experience during testing, as opposed to older versions that relied on JavaScript.
By mastering these features, you position yourself on solid ground when entering the world of automation testing, setting a strong precedent for more advanced explorations.
"Understanding the basics of any tool is essential for leveraging its full potential."
Key Concepts in Selenium With Python
Understanding the key concepts in Selenium with Python is essential for anyone looking to master web automation and testing. These concepts form the backbone of your ability to effectively navigate various web elements and interact with applications. Youāll find that a solid grasp of these elements not only boosts your confidence during interviews but also empowers you to handle real-time challenges in the field.
WebDriver Architecture
WebDriver is the cornerstone of Selenium, allowing the automation of web applications for testing purposes. Its architecture is essentially about how Selenium interacts with different web browsers. The WebDriver acts like a client-server communication mechanism.
The WebDriver follows a protocol that connects the script to the browser, issuing commands that the browser executes. The architecture consists of a client, which is the automation script, and a driver specific to each browser, like ChromeDriver for Google Chrome or GeckoDriver for Firefox. One of the primary benefits of WebDriver is its ability to control various browsers based on the same script. This cross-browser functionality is crucial for conducting comprehensive tests across environments.
To illustrate:
- Client: Your Python script.
- Browser Driver: Interacts with the browser.
- Browser: Executes the commands.
This layered setup ensures that commands made in the script get translated correctly into actions performed on a web page.
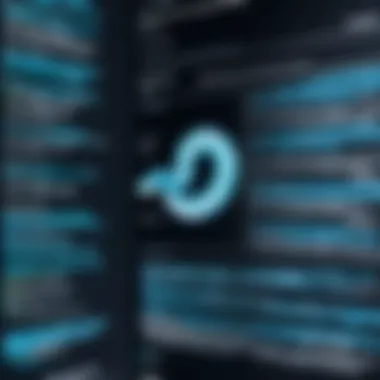
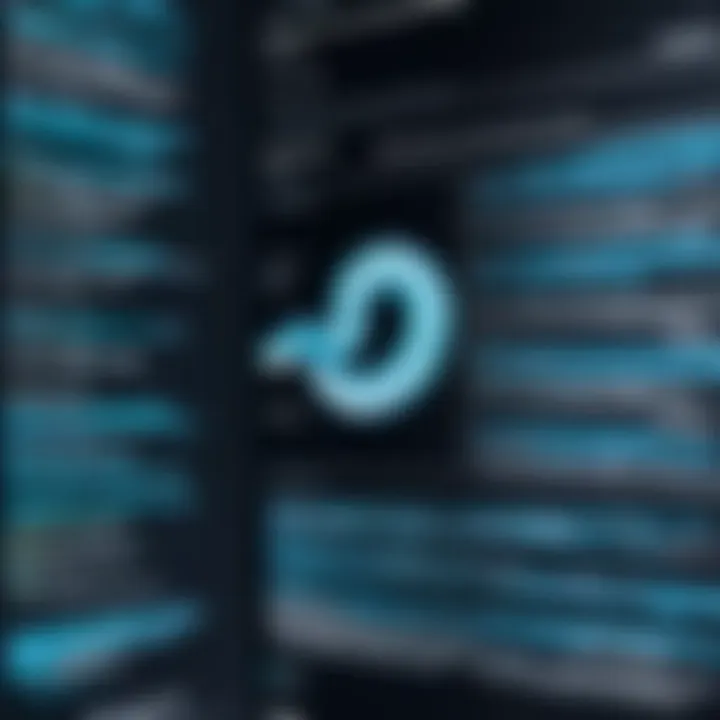
Locators in Selenium
Locators are essential in Selenium for identifying elements on a webpage. Without them, automating interaction with web components would be nearly impossible. Selenium provides various types of locators, each suited for different use cases
- ID Locator: By far the quickest way to find an element.
- Name Locator: Useful when ID is not available.
- Class Name Locator: Can be used for elements with specific classes.
- XPath Locator: Extremely powerful, allows complex queries based on the document structure.
- CSS Selector: A popular choice for its speed and flexibility.
Mastering these locators equips you with the skill to interact with almost any web element effectively, making your automation tasks smoother.
Actions and User Interactions
Selenium is not just about clicking buttons and retrieving text; itās about simulating real user interactions. This section covers how to create realistic scenarios where users can drag, drop, and navigate web pages as they would in reality.
Selenium's library is your trusty toolkit for these tasks. Here are some fundamental actions you might implement:
- Clicking: Triggering events just as a user would.
- Double-clicking: Often overlooked, but certain UI elements only respond to this type of click.
- Dragging and Dropping: Useful for applications that require moving elements from one place to another.
- Mouse Hover: It can reveal dropdowns or tooltips that require attention.
Understanding how to implement and manipulate these actions vastly enhances the control you have over automated interactions.
Synchronization in Selenium
In the world of web automation, timing is everything. Web applications donāt always behave predictably. Elements might take a moment to load or display, leading to potential failures if your automation doesn't account for that. Therefore, synchronization strategies become vital.
There are two primary types of waits in Selenium:
- Implicit Waits: Set once and apply to all elements; it tells WebDriver to poll the DOM periodically when searching for elements.
- Explicit Waits: More sophisticated, allows you to wait for specific conditions to be met before proceeding. For instance, waiting for an element to become clickable.
Understanding these synchronization techniques helps ensure that your scripts run smoothly, reducing the odds of unexpected errors during tests.
Remember: Proper synchronization leads to fewer false negatives.
By mastering the key concepts in Selenium with Python, you bolster your credibility as an automation testing candidate. Grasping the underlying mechanics not only prepares you for interviews but also equips you with the knowledge to tackle complex testing challenges.
Common Interview Questions
In the realm of automation testing, particularly with Selenium and Python, common interview questions play a crucial role in preparing candidates. These questions are not just about assessing knowledge; they serve as a window into the candidate's understanding of fundamental principles and practical applications. By familiarizing oneself with these queries, one gets a clearer picture of the expectations in the tech industry. Moreover, mastering these questions can streamline the preparation process, making it easier to build confidence before stepping into the interview arena.
What is Selenium?
Selenium is a widely used tool for automating web browsers, allowing developers and testers to write scripts that automate interactions with a web application. Its significance in testing cannot be overstated. Selenium supports various programming languages, but when paired with Python, it becomes an exceptionally powerful combination due to Python's concise syntax and readability.
More than just a simple automation tool, Selenium allows for complex testing scenarios. For instance, in a user login flow, while testing if the button clicks and input fields work as intended, itās practical to ensure that negative scenarios, such as incorrect passwords or empty username fields, are handled gracefully by the application. This breadth of testing capability is what makes Selenium an essential tool in the automation framework.
What are the different types of locators in Selenium?
Locators are integral to identifying web elements within the HTML structure. Here are the primary types of locators used in Selenium:
- ID: This is one of the fastest locators since IDs are unique within a webpage. It's definitely the go-to choice if available.
- Name: Similar to ID, name attributes can be used to find elements, albeit not as commonly as IDs.
- Class Name: This allows you to pick elements based on their classes, but be cautiousāmultiple elements can share the same class.
- Tag Name: This selects elements based on their HTML tag. It's useful in some specific scenarios, like identifying all elements on a form.
- Link Text: This locator is particularly handy when working with links; it retrieves links by their visible text.
- XPath: This locator is very versatile and enables the selection of elements using their hierarchical structure but can be slow compared to others.
- CSS Selector: These are similar to XPath but may run faster and offer more concise access to elements.
Understanding these locators and when to use each of them is indispensable for effective test automation in Selenium.
Explain the Page Object Model
The Page Object Model (POM) is a design pattern widely adopted in Selenium automation that enhances the maintainability and readability of test code. By separating the representation of the web pages from the tests, it streamlines the structure of automation scripts.
When using POM, you would create a class for each page of your application. For example, a class named could encapsulate all interactions related to the login page, such as entering a username, password, and clicking the login button.
This abstraction leads to:
- Reusability: Once an element or function is modeled, it can be reused across different test scripts without duplication.
- Readability: The test scripts become cleaner, as they reference high-level methods instead of deep HTML navigation.
- Maintainability: Changes to the UI only require updates in the page classes, not the entire suite of tests.
This makes the Page Object Model a best practice in Selenium test design.
How does Selenium handle browser windows and tabs?
Browser window and tab management is a crucial element of automated testing, as many applications open multiple windows or tabs for various functionalities. Selenium provides methods to handle these effectively.
When a new window or tab is opened, Selenium can switch between them using the concept of window handles. Each window has a unique identifier, or handle, and you can access these through the method. To switch to a particular window, you can use the command, where is the identifier for the desired window.
Here's a quick code snippet:
It's essential to remember to switch back to the original window when your testing is done, to avoid confusion and maintain the right context for subsequent actions.
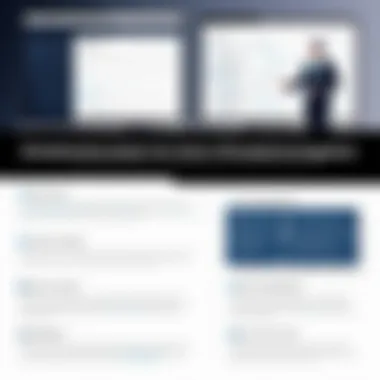
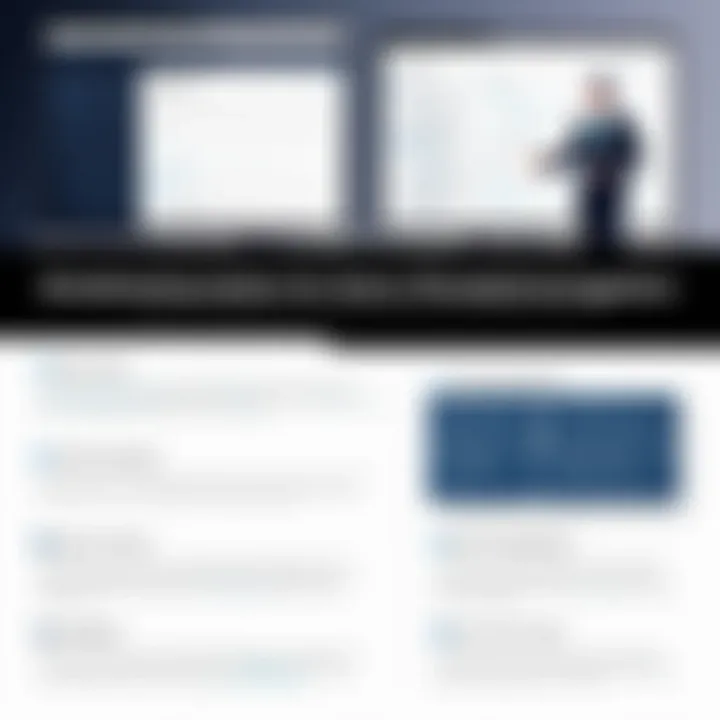
What are implicit and explicit waits?
In Selenium, managing element visibility is paramount for robust tests. This is where waits come into play. Specifically, there are implicit and explicit waits.
- Implicit Wait: This sets a maximum wait time for the entire session, meaning the WebDriver will retry finding elements for the specified duration before throwing an exception. For example, setting this to 10 seconds means Selenium will look for an element for 10 seconds before moving on.
- Explicit Wait: This is more flexible as it can be applied to specific elements. You define conditions to wait for, enhancing the specificity of your tests. For instance, you can wait for an element to be clickable before performing an action on it.
Example of explicit wait:
Mastering the use of both implicit and explicit waits can significantly improve test reliability and performance, making sure that elements are interactable before actions are attempted.
Technical Deep-Dive Questions
In the realm of Python Selenium interviews, Technical Deep-Dive Questions aim to sift through surface-level knowledge. These inquiries dig deep into a candidate's understanding of Selenium, requiring not just familiarity, but a tangible grasp of technical implementations and decision-making processes. When you're confronted with such questions, your answers can unveil your hands-on experience. Detailed responses can exhibit your critical thinking capabilities and how you tackle real-world challenges. Addressing these aspects can distinguish you as a candidate who not only knows the tools but knows how to wield them effectively.
How to handle alerts and pop-ups?
Navigating alerts and pop-ups is a fundamental skill when working with Selenium. Alerts are often used in web applications to notify users of important information or to prompt them for confirmation. Handling these alerts can be straightforward if you understand the methods available in Selenium. Here's what is typically involved:
- Switch to the alert: You use the method to shift the focus from the current window to the alert box.
- Interact with the alert: Once you have switched the context, you can:
- Retrieve the alert text using .
- Accept the alert with .
- Dismiss it using .
Here's a brief snippet:
Handling pop-ups, like alerts, follows a similar methodāthough it may require additional strategies depending on whether the pop-up is modal or not. Donāt overlook the importance of doing this seamlessly in user flows; it can make or break a testing scenario.
What is the difference between headless and headed browsers?
When it comes to running tests, headless browsers often change the game. A headed browser displays the UI, meaning you can see everything thatās happening. Conversely, a headless browser operates without a graphical user interface. It executes tests faster, which can be particularly beneficial for continuous integration/continuous deployment (CI/CD) pipelines.
Here are some distinctions:
- Performance: Headless often delivers better performance because it reduces the overhead of rendering the UI.
- Environment: You might want to use headless browsers during automated tests on servers where an actual browser isnāt feasible.
- Debugging: Conversely, headed browsers can make it easier to debug problems since you visually see whatās happening.
Choosing between headless and headed configurations involves weighing speed against the need for visibility in your tests.
Describe the process of taking screenshots in Selenium.
Taking screenshots can be a valuable tool in a tester's arsenal, especially when you need to capture the state of a web page at a particular moment. Selenium provides a convenient method to accomplish this:
- Use the method to save a screenshot directly to your file system.
- Alternatively, can be used to retrieve the image in Base64 format, handy for embedding in reports.
A basic code example:
Having screenshots at your disposal can be a game changer, especially when responding to issues raised during testing or illustrating a point during a review.
How can you execute JavaScript using Selenium?
JavaScript execution via Selenium can be a powerful tool for directly interacting with web pages. You might want to manipulate the DOM, access properties, or even trigger events. Seleniumās method streamlines this process.
Hereās a compact overview of what you might do:
- Inject logic to alter webpage elements or styles.
- Trigger JavaScript functions that arenāt directly callable through Selenium commands.
Example code:
Executing JavaScript can offer unique test solutions that might not be achievable using standard Selenium commands, making it a vital skill for aspirant candidates.
Remember, demonstrating a deep understanding of these technical aspects reveals your readiness for the job to prospective interviewers.
Best Practices in Selenium Automation
When it comes to automation testing with Selenium, applying best practices is like having a sturdy map while navigating through a dense forest. It keeps your project on the right path, prevents potential pitfalls, and ultimately ensures that your testing efforts yield reliable and maintainable outcomes. Since Selenium is a powerful tool for browser automation, employing these best practices can significantly enhance your testing efficiency and effectiveness.
Writing Maintainable Test Scripts
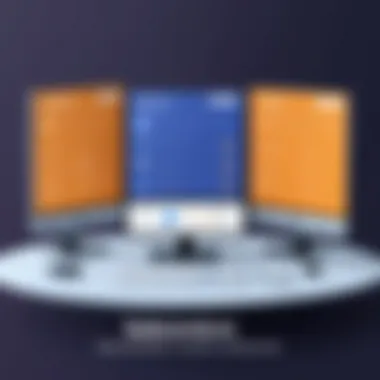
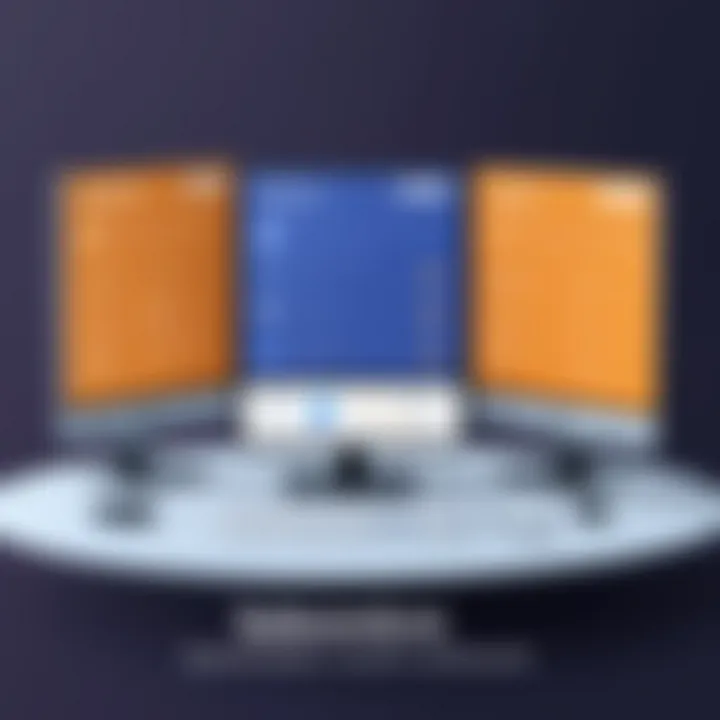
Among the myriad of practices, writing maintainable test scripts stands out as a cornerstone for successful automation. Scripts that are hard to read and understand can become a burden down the line, especially when modifications are necessary. Here are a few vital considerations to keep in mind:
- Clear Naming Conventions: Choose intuitive names for your methods and classes. For instance, a function named is far clearer than . This small detail can save hours of rummaging through code later.
- Modular Approach: Structure tests in a modular way. Break the test scripts into smaller functions or classes that handle specific tasks. This modularity makes it easier to troubleshoot and update code, as you can zero in on specific areas without digging through unrelated logic.
- Commenting and Documentation: Donāt underestimate the value of comments. Effective documentation makes it simpler for someone new to the projectāor even for your future selfāto grasp the purpose and functionality of certain parts of the code.
- Consistent Style: Stick to a coding style guide. Consistency in indentation, spacing, and structure reduces cognitive load and makes it less likely for errors to creep in.
"Good code is its own best documentation." ā Steve McConnell
By following these principles, your scripts will be more navigable than a well-marked hiking trail.
Using Version Control with Selenium Projects
Utilizing version control, like Git, is crucial in any software development, but especially in automation testing. It provides a safety net that allows you to track changes, collaborate with others, and roll back if you encounter a few bumps along the way. Hereās why itās essential:
- Track Changes: Version control makes it easy to review changes over time. If a test suddenly fails, you can pinpoint what was altered.
- Collaboration: If your team is working together on tests, version control allows different team members to make changes without overwriting one another's work. Branching strategies can help in managing multiple features or fixes simultaneously.
- Documenting Progress: Commit messages serve as a log of what changes were made and why. This documentation can assist in understanding the evolution of a project.
- Backup and Recovery: In case your project encounters an unfortunate mishap, version control gives you a way to recover your scripts. Having a backup is like having a safety cushion; it cushions fall when things go awry.
Integrating with / Pipelines
The integration of Selenium tests into Continuous Integration / Continuous Deployment (CI/CD) pipelines is another important aspect. It adds a level of automation to your testing efforts that can save valuable time and resources. Here are some factors to consider:
- Automated Testing: By connecting your Selenium scripts to a CI/CD tool, you ensure that tests run automatically every time there is a code change. This catches issues before they reach production.
- Immediate Feedback: As soon as a developer commits code, tests execute, allowing quick feedback. This prompt response can prevent bugs from sneaking into later stages.
- Environment Consistency: CI/CD allows you to run tests in consistent environments, reducing issues caused by environment mismatches. You can set your tests to run on various configurations to ensure robustness.
- Scalability: As your project grows, a CI/CD pipeline makes it easy to scale your testing efforts. You can continue to add more tests without worrying about how they fit into the workflow.
By embracing these best practices in Selenium automation, you can not only strengthen the reliability and maintainability of your tests but also empower your team to tackle challenges more effectively. Automation should enhance your processes, not complicate themākeep these principles in mind to ensure you're steering your efforts in the right direction.
Emerging Trends and Technologies
In the evolving field of automation testing, it's crucial to stay ahead of the curve. The trends and technologies surrounding Selenium and Python are constantly reshaping the landscape, offering new possibilities for efficiency, effectiveness, and innovation. For aspiring automation testers, understanding these developments can provide a competitive edge during interviews and in actual job scenarios. This section delves into emerging trends and technologies, discussing their importance and implications for Selenium testing.
Future of Selenium with Python
The journey of Selenium with Python continues to keep its trajectory strongly towards integration and automation advancements. As industries push for more rapid deployment cycles, there's an increasing demand for robust testing frameworks. Deep integration capabilities of Selenium with Python make it a prime candidate for addressing this need.
Pythonās versatility plays a significant role here. Developers can utilize its simple syntax to write complex testing scripts. The asyncio library, for example, enables asynchronous programming ā resulting in faster test execution.
Furthermore, as web applications become more sophisticated, emerging libraries and frameworks are appearing to simplify interaction with Selenium. For instance, frameworks like pytest add additional features that significantly enhance testing capabilities. The rise of cloud-based Selenium services also means testers can execute test cases remotely, providing greater flexibility and reducing local setup time.
In summary, the future of Selenium with Python is closely tied to its adaptability. Test automation will continue to evolve, enabling a more streamlined approach that matches the pace of web development.
AI and Machine Learning in Testing
Artificial Intelligence (AI) and Machine Learning (ML) are setting a new bar in automation testing, with the potential to transform traditional methods. Integrating Python with AI and ML opens new avenues for smarter and more efficient testing.
- Predictive Analytics: AI tools can predict the likelihood of failures in specific areas of applications, allowing testers to prioritize their efforts. This is hugely beneficial in managing large test suites and improving workflow efficiency.
- Test Automation: Machine learning can enhance script generation through pattern recognition. For instance, instead of creating scripts manually, ML algorithms can learn the behavior of an application over time, automating test case generation accordingly.
In addition, the capacity to carry out visual testing can be notably improved by implementing AI techniques. Modern tools can detect visual discrepancies with uncanny accuracy, ensuring UI consistency across various platforms and devices.
The important takeaway here is that integrating AI and ML with Selenium testing is not just a trendāit represents a significant resource for addressing the increasingly complex challenges in automated testing today.
While itās evident that AI and ML will reshape automation, adapting to these technologies requires continuous learning and a willingness to experiment. The future promises advanced strategies that go beyond traditional testing paradigms.
Preparing for the Interview
When stepping into the realm of technical interviews, especially for positions emphasizing Python and Selenium, preparation can make the difference between a job offer and a missed opportunity. It's crucial to focus on integrating both theory and practical knowledge. Familiarity with Selenium's capabilities and how they mesh with Python will help you answer questions confidently and walk through practical scenarios effortlessly.
Preparing for the interview isnāt just about learning the right answers. It requires an understanding of the concepts, so you can tackle questions from different angles and even ask insightful questions of your own. Here, we take a look at the essential aspects that must not be overlooked in your preparation.
Structuring Your Responses
One of the most effective ways to impress interviewers is through the way you structure your responses. When faced with a question, always take a moment to gather your thoughts. Aim for a clear structure; this generally includes an introduction to the topic, a detailed explanation, and a conclusion summarizing your key points. For instance, if asked about the advantages of using Selenium with Python, start by stating why Selenium is preferred for automation testing, then dive into the specific benefits offered by Python, like the rich libraries and ease of writing scripts.
Beyond merely answering the question, it pays to weave in relevant examples. A good practice is to narrate an experience you've had using Selenium in a project. Something like:
"In my previous project, I utilized the Page Object Model to improve the maintainability of our test scripts, which ultimately led to a significant reduction in time spent on test maintenance."
Breaking your answer into bite-sized, digestible pieces makes it easier for interviewers to follow your logic. Additionally, avoid using jargon excessively, as it may come off as attempting to showcase knowledge rather than genuinely sharing information.
Common Pitfalls to Avoid
Understanding what not to do can be just as essential as knowing what to do. Here are some frequent missteps when preparing for an interview:
- Over-Complicating Answers: Simplicity often trumps complexity. It's easy to get lost in technical details that may overwhelm the interviewer.
- Failing to Listen: When a question is posed, ensure to listen intently. Sometimes, interviewers might provide hints or context that can aid in answering more accurately.
- Neglecting to Prepare for Practical Questions: Itās one thing to discuss Selenium in theory, but having hands-on experience where complexities can arise is invaluable.
- Rushing Through Explanations: Take your time in explaining; a rushed response can denote nervousness or lack of knowledge.
Avoid these traps as you prepare, and it will place you in a far better position to showcase your capabilities.
Resources for Further Study
There are numerous materials that can bolster your understanding of Selenium and aid you in preparing for your interview:
- Books: Look for updated literature focusing on Selenium with practical examples and case studies. Books like "Selenium Testing Tools Cookbook" provide hands-on projects.
- Online Courses: Websites like Coursera or Udemy offer targeted courses that cover Selenium from beginner to advanced levels, reinforcing practical skills.
- Documentation: Review Seleniumās official documentation. It's thorough and regularly updated, making it a reliable source.
- Forums and Communities: Engage with others in communities like Reddit and Stack Overflow. Asking and answering questions can provide deeper insights and different approaches.
- Practice Sites: Platforms like LeetCode and HackerRank can offer coding challenges where you can apply your Selenium knowledge practically.
By diligently focusing on how to articulate your thoughts, avoiding common errors, and immersing yourself in studying from the right resources, you position yourself for success in the competitive landscape of Python Selenium interviews.
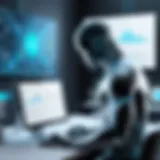
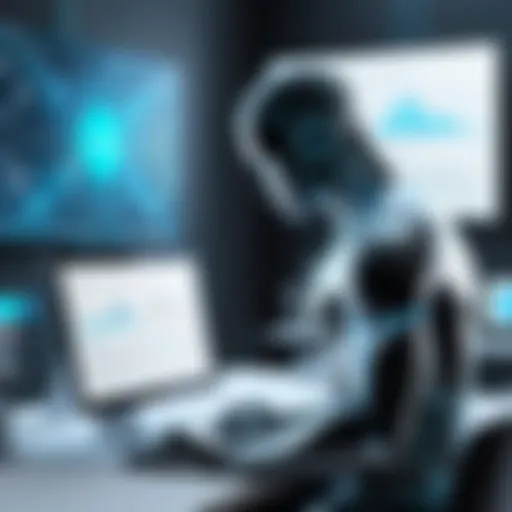