Mastering Python Modules: Key Concepts and Practices
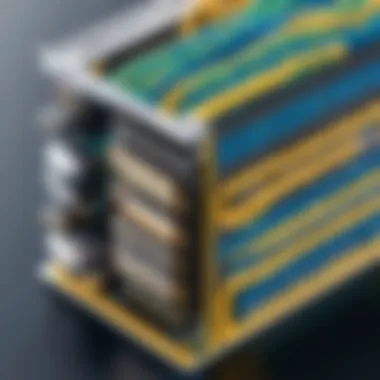
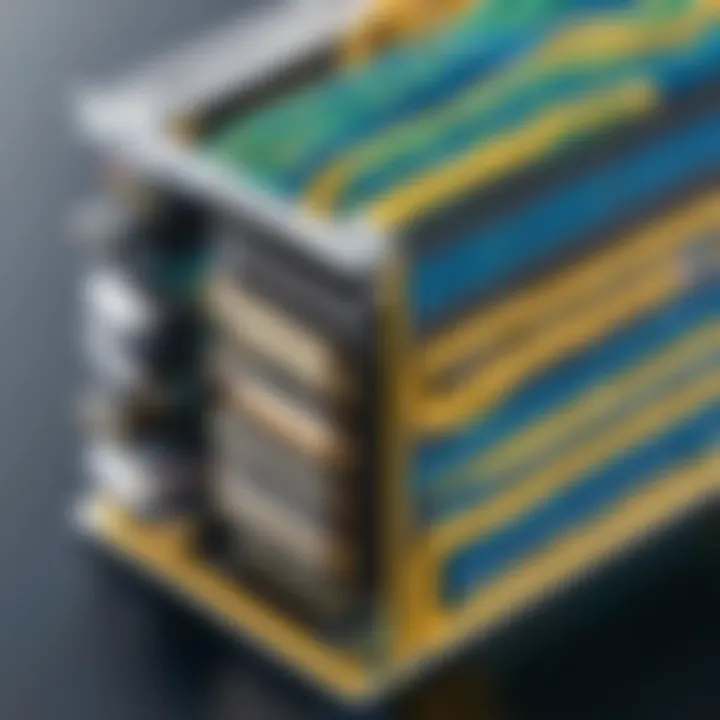
Intro
Python has evolved into a key player in the world of programming, making it indispensable for newcomers and seasoned coders alike. Understanding how to manage and utilize modules is essential not just for writing clean code, but also for promoting modular programming, a practice that enhances reusability and readability.
Preface to Programming Language
History and Background
Emerging from the humble beginnings in the late 1980s, Python was designed by Guido van Rossum with a fitting philosophy: simplicity and readability. Aiming to create a language that was user-friendly yet powerful, it has grown into a predominant force across various domains, including web development, data analysis, and artificial intelligence.
Features and Uses
One standout feature of Python is its extensive standard library, which comes packed with pre-built modules, meaning less time writing code from scratch. This is where modules shine! They help keep things tidy by breaking down coding tasks into manageable pieces. For instance, if you want to interact with the internet, you could leverage the module instead of crafting HTTP requests manually.
- Readable Syntax: Pythonās syntax often resembles plain English, making it easier to learn and recall.
- Versatile Applications: From scripting simple tasks to building complex systems, the uses of Python modules are limitless.
- Strong Community Support: Python has a vibrant community that continually contributes to libraries, frameworks, and resources.
Popularity and Scope
Python's popularity is no fluke. It's a language that gets things done, whether you're delving into data science with NumPy or creating web applications using Flask. According to recent surveys, it consistently ranks among the top programming languages worldwide. Its broad applicability attracts a diverse user base.
"Python is like a Swiss Army knife in programming ā versatile and handy for almost any task."
Basic Syntax and Concepts
Modules are built on some fundamental principles of Python, so itās worth revisiting basic syntax concepts that serve as a foundation for using them effectively.
Variables and Data Types
In Python, handling data is straightforward. Variables are dynamically typed, meaning you don't need to specify their data type explicitly.
Example:
Operators and Expressions
Combining values and variables often involves operators. Mathematical operations are quite clear. So a simple expression might look like this:
Control Structures
Control flow statements such as , , and guide the execution of code based on conditions. This is crucial for creating more dynamic and responsive programs.
Advanced Topics
As one delves deeper into Python, mastering certain advanced concepts can enhance your approach to modules significantly.
Functions and Methods
Functions encapsulate specific tasks, making it convenient to reuse code. When working with modules, understanding how to define and invoke functions is important. Modules often expose functions to be utilized externally.
Object-Oriented Programming
Modules can be designed to contain classes that encapsulate data and behaviors. Object-oriented principles allow you to produce code that mimics real-world scenarios, fostering reusability and organization.
Exception Handling
Not every program goes as planned. Learning how to handle exceptions will enable graceful handling of errors. Using the and blocks can prevent your program from crashing unexpectedly.
Hands-On Examples
Now that we've covered some essentials, it's time to look at practical applications of Python modules.
Simple Programs
Let's say you want to write a program that calculates the square of numbers in a list. By utilizing a module, like , you can achieve this simply:
Intermediate Projects
Consider a small project where you create a script that fetches weather data using an API. You could use the module to simplify the HTTP requests needed for this task.
Code Snippets
Including code snippets in discussions or documentation can make understanding easier. For example, consider a simple module creation:
Afterward, you can import and utilize it in your main code.
Resources and Further Learning
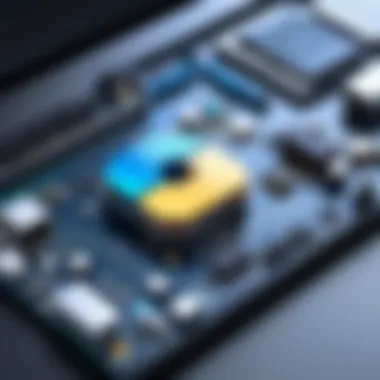
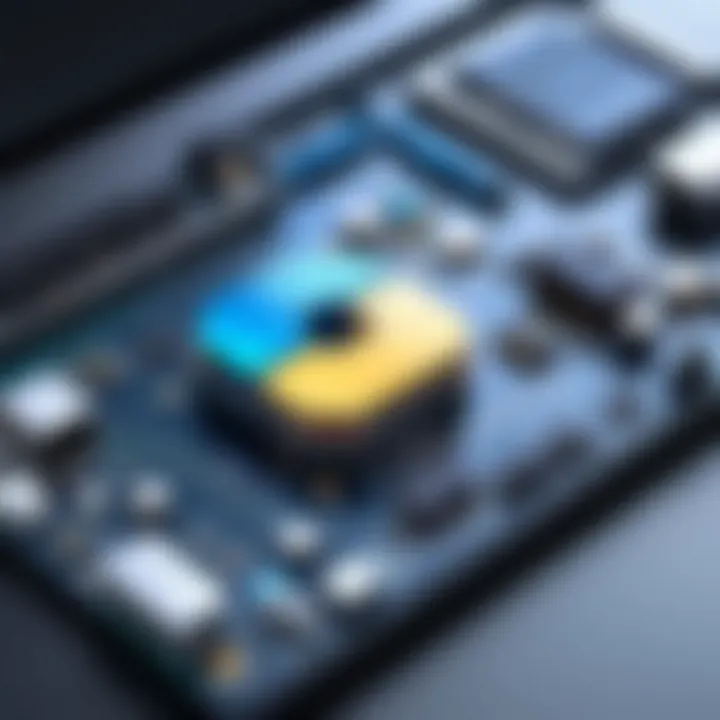
As you traverse through the world of Python modules, you may want to arm yourself with additional resources to bolster your understanding.
Recommended Books and Tutorials
- "Automate the Boring Stuff with Python" by Al Sweigart: A great resource for practical Python.
- "Python Crash Course" by Eric Matthes: Focuses on project-based learning.
Online Courses and Platforms
- Coursera: Offers several courses focused on Python modules and libraries.
- edX: A variety of Python courses from different universities.
Community Forums and Groups
- reddit.com/r/Python: An active discussion board with plenty of insights.
- facebook.com/groups/python: Engage with fellow learners and experts.
Prelims to Python Modules
Understanding Python modules marks a pivotal step in mastering the language and elevating oneās programming skills. Modules alleviate the complexities of software development by promoting organized code, enabling developers to break down their programs into manageable, reusable pieces. By understanding modules, programmers can not only streamline their workflow but also enhance collaboration within teams, as well-structured modules allow for quicker comprehension and adaptation of existing code.
The significance of modules resonates deeply across all levels, from beginners trying to grasp the basics to seasoned professionals looking to refine their work. They serve as the building blocks of Python's architecture, allowing for scalability and efficiency in code management. When one thinks of the modular approach, itās like assembling pieces of a puzzle where each module holds its unique purpose, fitting perfectly into the wider picture that constitutes a program.
Definition of a Module
A module in Python is essentially a file containing Python code. This code can define functions, classes, and variables, and can also include executable code. For instance, if you create a file named , it can house various mathematical functions that you can call from other Python files or modules.
By creating modules, you encapsulate functionality that can be reused across different projects or within a larger application without duplicating code. Each module can be imported into other modules or scripts, promoting the principle of DRY (Don't Repeat Yourself).
Importance of Modules in Python
The importance of modules lies not only in code organization but also in enhancing code quality and maintainability. Here are some of the key benefits:
- Code Reusability: Once a module is created, it can be reused across multiple programs. This significantly reduces development time and effort.
- Namespace Management: Using modules helps avoid name conflicts in larger applications. It ensures that identifiers declared in one module won't clash with those in another.
- Easier Collaboration: Teams can work on individual modules without stepping on each other's toes. Each member can build, test, and refine their slice of the project before integrating it with others.
- Testing and Debugging: Modules facilitate separate testing. By isolating functionality, developers can troubleshoot more effectively and ensure quality control before merging code.
- Standardization: Following best practices around module structure leads to better documentation and a more consistent coding environment, making it easier for team members to onboard.
"Modules let you keep your workspace tidy and your brain clear. Think of them as the organized drawers in your toolbox; everything has a place, and itās all readily accessible."
In summary, introducing yourself to modules can truly reshape how you approach Python programming. Their role goes beyond mere code, influencing workflow, collaboration, and most importantly, the efficiency of your coding practice.
Types of Modules in Python
Understanding the various types of modules available in Python is crucial for anyone venturing into this programming language. Modules serve as containers for code, allowing for organization, reuse, and clarity. By categorizing them, you can choose the right module depending on your needs, thereby enhancing your coding efficiency. This section discusses three main types of modules you will encounter while working with Python: built-in modules, user-defined modules, and third-party modules.
Built-in Modules
Built-in modules are the gems provided by Python itself. These modules are part of the standard library and come pre-installed with Python, which means you donāt need to install anything extra to use them. They cover a wide array of functionalities, from mathematical operations to file handling.
Some noteworthy built-in modules include:
- : Provides mathematical functions, such as trigonometric functions and constants like Ļ.
- : Enables interaction with the operating system, allowing you to manipulate files and directories easily.
- : Gives access to some variables used or maintained by the interpreter and to functions that interact with the interpreter.
These modules save a lot of time and effort, letting you focus on higher-level programming without reinventing the wheel.
User-defined Modules
User-defined modules are created by programmers for their specific needs. They allow you to encapsulate code that performs certain functions, making it easy to reuse in different projects. Crafting a user-defined module is essentially packaging a few functions into a .py file. For example, if you regularly perform calculations in a script, you could create a module called that contains all those functions.
To create your own module, follow these steps:
- Write the functions you want in a Python file.
- Save the file with a extension.
- Use the statement to bring your module into another script.
This practice not only enhances code readability but also improves collaboration when working in teams. team members can easily understand and use your modules.
Third-Party Modules
Third-party modules are libraries created by other developers to extend Python's functionality. Unlike built-in modules, these need to be installed separately, often using a package manager like . They offer a treasure trove of functionalities that are not found in standard Python library.
Some popular third-party modules include:
- : Essential for scientific computing, it provides support for large, multi-dimensional arrays and matrices, along with a suite of mathematical functions.
- : Ideal for data manipulation and analysis, it offers data structures and operations for manipulating numerical tables.
- : A favorite for making HTTP requests, simplifying the process of handling web data.
Using these modules can often exponentially boost your productivity, making your life as a programmer more manageable.
In summary, knowing about these three types of modulesābuilt-in, user-defined, and third-partyāenables you to leverage them effectively, propelling your programming skills forward.
Creating Your Own Module
Creating your own module is a fundamental step in mastering Python programming. Modules enhance code organization, promote reusability, and boost collaborative efforts among developers. By crafting a module, programmers can encapsulate functionalities that can be easily imported into various projects, saving time and maintaining consistency across codebases. This section aims to break down the process of module creation into digestible parts, covering everything from the necessary structure to the actual coding practices.
Module Structure and Naming Conventions
When working on a module, following a proper structure and naming convention is crucial. A well-structured module can significantly simplify the development process and make it easier for others to understand and utilize your code. Here are some key considerations:
- Naming: The name of the module should be descriptive enough to indicate its purpose. Avoid using special characters and spaces. Instead, opt for underscores or camel case (e.g., or ). This helps with readability and maintainability.
- Organization: Each module is generally placed in its own file. The file name should match the module name. For instance, a module named should be found in .
- Directory Structure: If you have several related modules, consider grouping them into a package. A package is simply a directory containing a special file called , which can be empty or contain initialization code.
Following these convention ensures that your module stands a good chance at being used by other programmers, whether you share it publicly or within a team.
Writing Code in a New Module
Writing code in your module is where the real creativity happens. Start simple; donāt attempt to overcomplicate things at the beginning. Here are some practical steps:
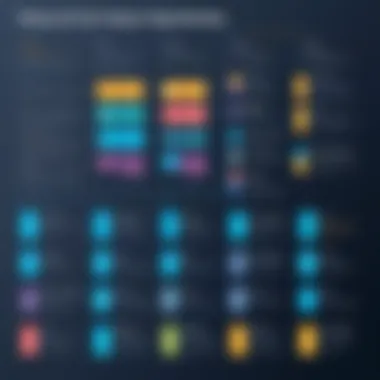
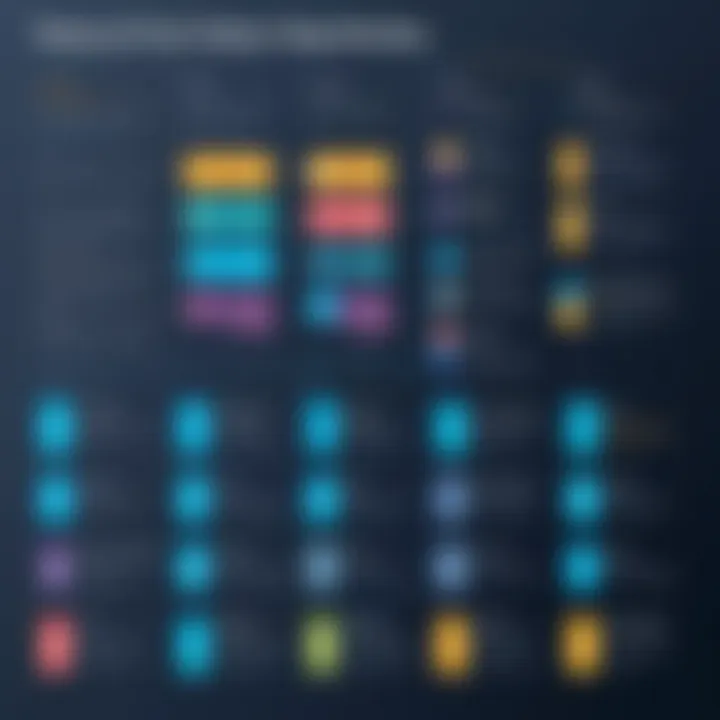
- Define Functions: Clearly define the functions your module will provide. Consider making them flexible and well-commented. A well-commented function is like a well-marked trail in a forest; it guides others (and your future self) through the logic.
- Use Variables and Constants: Keep your variables and constants organized. Group related items logically and avoid using overly complex data structures unless necessary. This helps reduce confusion.
- Implement Error Handling: Incorporate basic error handling to make your functions robust. Use try-except blocks to catch potential errors and handle them gracefully.
This is a simple example of what a module named might look like:
This basic structure shows how to define functions and gives a glimpse of how to use them in testing.
Saving and Importing the Module
Once you've written your module, the next step is saving it properly and importing it into other Python scripts. The process is straightforward:
- Saving the Module: Save your file with the extension, ensuring it fits the naming conventions discussed earlier. Place it in the directory where your main script will run or in a location included in the Python path.
- Importing the Module: In your main script, use the import statement to bring your module into the current namespace. Here are different ways to perform the import:
- Using imports the entire module.
- If you just need specific functions, use .
- If you want to rename during import, you can use . This allows you to call it easier, like .
By mastering how to create, organize, and import your own modules, you're taking a significant step towards writing cleaner and more maintainable code. Engage in practicing this skill, as it contributes to a deeper understanding of Python programming.
Importing Modules in Python
When you venture into the realm of Python programming, understanding how to import modules becomes a cornerstone of efficient code writing. Modules serve as repositories of functions, classes, and variables, which can save you heaps of time and effort. This section delves into the importance of importing modules and how it enhances code reusability and organization. Moreover, it will guide you through the various methods of importing modules, from basic syntax to more specific importing techniques.
Basic Import Syntax
The basic import syntax in Python is straightforward yet powerful. To pull a module into your script, one can simply use the statement, like this:
Once this line is executed, all the functionalities contained in become accessible in your current namespace. It's as if you are taking a treasure chest of tools and placing it right on your desk for instant use. However, keep in mind that the name of the module should adhere to Python's naming conventionsāthink simple, lowercase, and no spaces.
Using the Import Statement
Beyond just the basic import, diving deeper into using the import statement reveals more nuanced capabilities. For example, the statement can be expanded to include a specific component of the module. Here's how it looks:
This tactic is especially useful when a module has multiple functions, but you only need one or two of them. It also helps to keep your code clean and prevents namespace clutter. After executing this line, you can directly call the function without prefixing it with the module name. This saves typing time and makes the code easier to read. Just like a well-organized toolbox, it allows you to grab what you need swiftly without rummaging through everything.
Importing Specific Functions
When it comes to importing specific functions, one can carry on with the approach highlighted above but with a more focused view. Frequently used functions can be imported on an individual basis to simplify access further. Take a look:
Here, you import the function and the constant from the module. This means you can use them directly in your code without extra notation.
This selective importing enhances your script's performance by keeping its footprint smaller. Each function you donāt import helps to lighten the load, just like traveling light on a long journey.
Epilogue
Importing modules in Python is not merely about lifting code from other files; itās about leveraging existing tools to build bigger, better applications. It streamlines your workflow, reduces redundancy, and keeps your codebase manageable. Understanding and applying these import techniques will become second nature as you grow in your Python journey, turning your coding skills from novice to nimble.
Exploring Standard Libraries
In the realm of Python programming, standard libraries are akin to a treasure chest filled with indispensable tools that enable developers to accomplish a wide range of tasks with relative ease. These libraries offer ready-to-use modules that can significantly boost productivity and reduce the amount of code one needs to write from scratch. Understanding the functionality and importance of Python's standard library can elevate a programmerās skill set from basic to advanced, allowing for efficient and effective code development.
Overview of Python Standard Library
The Python Standard Library is a vast collection of pre-written code that comes bundled with every Python installation. It functions as a rich repository, offering modules and packages that handle everything from mathematical computations to data manipulation and network protocols. Knowing what these libraries offer is like having a Swiss army knife on handāeach tool is designed to solve a specific problem, streamlining the coding process.
For example, modules like help manage dates and times, interfaces with the operating system, and provides support for regular expressions. The beauty of the standard library lies in its versatility: it allows programmers to focus on solving unique problems rather than reinventing the wheel.
Key Libraries to Know
Familiarity with specific libraries within the Python Standard Library can make a world of difference in a programmer's efficiency. Some key libraries that every programmer should know include:
- : This module provides mathematical functions such as trigonometric functions, logarithms, and constants like pi. It's handy when you need to perform complex calculations without manually coding them.
- : For anyone working with web APIs or needing data exchange in a structured format, the library is essential. It allows for easy parsing and generating of JSON strings.
- : Handling dates and times is crucial in many applications. This module provides classes for manipulating dates and times, facilitating everything from simple formatting to complex scheduling solutions.
- : Interacting with the operating system environment becomes seamless with this library, whether you're accessing file systems, creating directories, or fetching environment variables.
- : Though not a built-in standard library, itās worth mentioning for its frequent use. This library simplifies HTTP requests, making it a favorite among developers when consuming web services.
"Using standard libraries effectively means being able to shorten your development time without compromising the quality of your code."
In sum, the Python Standard Library is a powerful ally for any programmer. Its extensive collection of modules not only saves time but also enhances the code's robustness, allowing for cleaner and more maintainable solutions. As you dive into each of these libraries, youāll find countless examples and community resources that make mastering them easier. Websites like wikipedia.com), reddit.com, and facebook.com serve as valuable platforms for further exploration and discussion.
Best Practices in Using Modules
Utilizing modules wisely can dramatically enhance the quality of Python code. Best practices in this area help promote a clean, efficient, and maintainable codebase. It's akin to wearing a well-fitted suit; it not only improves your appearance but also boosts your confidence. By adhering to these practices, developers can elevate their programming game while reaping the numerous benefits of module usage.
Code Reusability and Clarity
Maximizing code reusability is a cornerstone of effective programming, particularly when dealing with modules. When code is designed to be reused, it saves time and reduces the potential for errors. Rather than rewriting the same functionalities across various projects, developers can import existing modules, allowing them to focus on more significant challenges.
Consider a scenario where you create a function to calculate the area of a circle. Instead of recoding this function whenever needed, you can build it into a module. Import that module when you require it, thus ensuring consistency and reliability across your codebase.
Another essential aspect is clarity. If a module is well-organized and properly documented, it becomes a breeze for others (or your future self) to understand it. Keeping functions small and descriptive can help in this regard. Each function should ideally accomplish one specific task, which not only boosts clarity but also aids testing and debugging. Here's a simple example of a module:
By breaking down functionalities into modules, you create a system that is both organized and easy to read, which is essential when you're diving back into code months later.
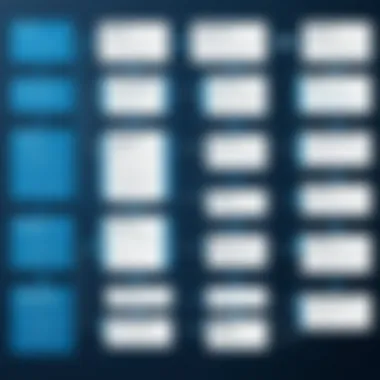
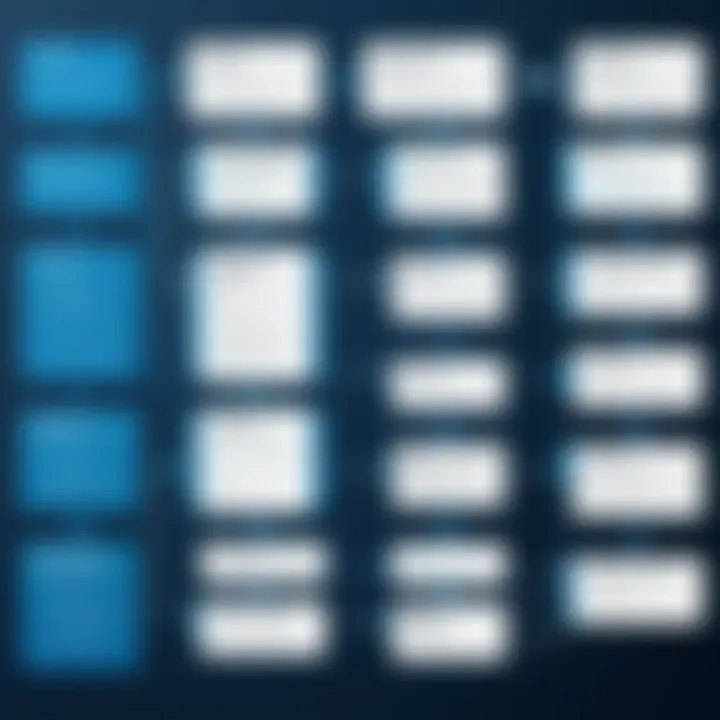
Organizing Code with Modules
Proper organization of code through modules is more than just aesthetics; it's critical for maintenance and scalability. As projects grow, cluttered code can become overwhelming. Modules provide a framework for breaking down complex systems into manageable chunks. You might visualize it as sorting tools in a toolbox; when each tool has its own spot, finding what you need in the heat of the moment is much simpler.
Start by grouping related functionalities into a single module. For example, if you're working on a web application, you might have separate modules for database handling, user authentication, and data validation. This separation not only cleans up your workspace but also allows for collaborative work without stepping on each other's toes.
There are also naming conventions worth adhering to. Module names should be descriptive and preferably lowercase. This will not only clarify the contents of the module but also follow Python's style guidelines, making your code more palatable for other developers. A good practice is to prefix utility modules with , indicating their purpose, like for string utilities.
Ultimately, proper organization and clarity in module design enhance collaboration, promote error tracking, and foster a better understanding of the overall code structure.
By taking these best practices to heart, you set the stage for less hectic coding sessions and pave the way for scalable and maintainable software solutions.
Common Issues with Modules
Modules are the lifeblood of Python programming, enabling code organization and reuse. While they come with a plethora of advantages, developers often encounter common issues that can create roadblocks in the coding journey. Understanding these challenges is crucial for navigating Python effectively. By recognizing potential pitfalls, programmers can troubleshoot more efficiently, saving time and frustration in the long run.
Module Not Found Error
The Module Not Found Error is a misplaced landmine every coder dreads stepping on. Itās that sinking feeling when you know youāve got a module ready to go, yet Python insists on playing hard to get. This error typically means that Python cannot find the module youāre attempting to import. Causes can range from typos in your import statements, the module not being installed properly, or simply the module living in a different directory.
Hereās a breakdown of common reasons for this error:
- Spelling Mistakes: Double-check your module name. A simple typo can lead the interpreter on a wild goose chase.
- Module Installation: Ensure that the module is actually installed. You can use pip to check this by running .
- Incorrect Python Environment: If youāre working in a virtual environment, make sure it is activated, otherwise Python might not find your module.
- Path Issues: Sometimes the module is there but Python doesnāt know where to look. Adjust your or move the module to the appropriate folder.
A good practice would be to catch this error in your code like so:
This way, instead of crashing your entire program, it will give helpful feedback.
Circular Import Issues
Next on the list is Circular Import Issues, which can feel like being stuck in a loop with no way out. This occurs when two or more modules try to import each other directly or indirectly. Imagine Module A trying to import Module B while Module B is simultaneously attempting to import Module A. Python doesnāt know where to start, resulting in an import error.
To get a clearer picture, consider these ideas:
- Redesign Your Modules: If possible, rethink the architecture of your code. Perhaps blending some functions into one module would help keep things neat.
- Use Import Statement Wisely: Instead of a top-level import, consider placing the import statement within a function to delay the import until necessary.
- Decoupling: If youāre operating with multiple classes or functions, separating them into a different module can help. This avoids having modules depend on each other directly.
Hereās a simple illustration:
In the example here, trying to run either file will raise an error due to the circular dependency. Rethinking module structure may resolve this problem.
Circulatory imports can become a coding nightmare. Since the system can't resolve the imports, your implementation comes to a screeching halt. It's best to try and plan your modules with minimal dependencies to avoid these traps.
Tackling common issues with modules not only refines the development process but also makes coding in Python less cumbersome. š Understanding these errors means fewer headaches, allowing for a more focused approach to programming.
Module Documentation and Resources
Understanding modules in Python is not just about knowing how to create and use them; documentation plays a crucial role in maximizing their utility. Well-documented modules can serve as a roadmap for developers, guiding them through the intricacies of functions and classes within. When you hit a bump in your coding journey, having thorough documentation can be like having a trusty compass in the wilderness.
Using Docstrings for Documentation
Docstrings are a pivotal part of creating effective documentation for your modules. These strings, which inhabit the very first line inside your module or function, allow you to detail the purpose, parameters, and return values clearly. But they go beyond mere explanations; they're a form of communication, creating a bridge between your code and its users.
Here's a quick look at why you should harness this powerful tool:
- Clarity and Transparency: When someone (including your future self) reads your docstrings, they get an immediate understanding of what a function or module does without combing through the code.
- Error Prevention: By detailing expected inputs and outputs, you reduce the risk of users coding errors that stem from misunderstanding how to use the module.
- Integration with Tools: Many IDEs and documentation generators pick up on docstrings, making it easier to create external documentation or onboard new team members.
Example of a simple docstring:
In this example, the docstring captures essential information concisely, making it easy to comprehend at a glance.
Resources for Learning More
With technologies evolving at breakneck speed, continuously enhancing your understanding of modules and accompanying tools is non-negotiable. Several resources can aid you on this journey:
- Official Python Documentation: The Python documentation is an invaluable starting point. It covers everything from basic usage to advanced details on modules.
- Online Communities: Platforms like Reddit house vibrant communities where users share their insights, answer questions, and provide guidance on best practices.
- Books and Tutorials: Books like "Fluent Python" by Luciano Ramalho offer deeper insights into Python programming, including practical examples of module usage. Online platforms like Coursera, Udacity, or even Facebook groups can also provide structured learning paths.
- Forums for Troubleshooting: Engaging with communities such as Stack Overflow can be a goldmine for solving specific issues you might encounter while working with modules.
Remember, a well-rounded toolbox is essential for any developer. Whether it's through docstrings, online forums, or comprehensive documentation, being informed and prepared is key to effective coding.
In sum, the importance of documentation and resources in Python modules cannot be overstated. They empower users to leverage the full potential of their code, ensuring that each team member can get on with contributing efficiently. As you grow in your programming journey, remember to cultivate not just your ability to write code, but also the skill to communicate its purpose and functionality effectively.
Ending
When delving into the realm of Python modules, it becomes evident how crucial they are in sharpening one's programming skills and bolstering code management. The ability to structure code through modules not only enhances readability but also facilitates maintenance across larger projects. This article has provided a comprehensive overview of various facets of Python modules, from understanding their definition and types to exploring best practices and troubleshooting. Every aspect covered contributes to a clearer grasp of how to utilize modules effectively, allowing individualsāwhether they are novices or seasoned programmersāto leverage these building blocks of Python.
Recap of Key Points
To encapsulate the essential elements:
- Definition and Importance: Modules serve to encapsulate functionalities, fostering code reuse and organization.
- Types of Modules: Built-in, user-defined, and third-party modules each offer unique capabilities, tailored to diverse programming needs.
- Creating Modules: Understanding the conventions surrounding module structure simplifies the process of writing, saving, and importing your own code.
- Importing Modules: Grasping the syntax and methods for importing can streamline workflows and enhance the efficiency of your coding practices.
- Common Issues: Being aware of potential pitfalls, such as circular imports, empowers programmers to troubleshoot effectively.
- Documentation and Resources: Utilizing docstrings enriches your code, while external resources provide avenues for continual learning.
Final Thoughts on Modules
In the grand scheme of programming, mastering modules can feel like acquiring the keys to a vast library. They empower you to build more complex applications with a methodical approach to code organization. Many successful developers advocate for the prudent use of modules to facilitate not only code clarity but also to encourage collaboration in teams. As learners journey through programming, understanding how to leverage modules will yield benefits in efficiency and functionality.
Moreover, as Python continues to grow and evolve, so do its modules. Keeping abreast with evolving libraries or contributing to open-source modules might also position you as a proactive member of the programming community. This is not just a hobby; it becomes a constant learning curve wherein one always finds room to improve.
"In programming, every line of code tells a story; make sure your modules are clear and well-organized to narrate the tale of your creativity and logic."
Ultimately, embracing the various aspects of Python modules fosters a deeper understanding of the language and enhances coding prowess. With the right approach, your programming could take on new dimensions, one module at a time.