Unveiling Python's Lambda Functions: A Complete Exploration
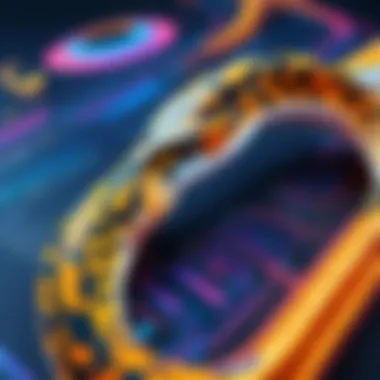
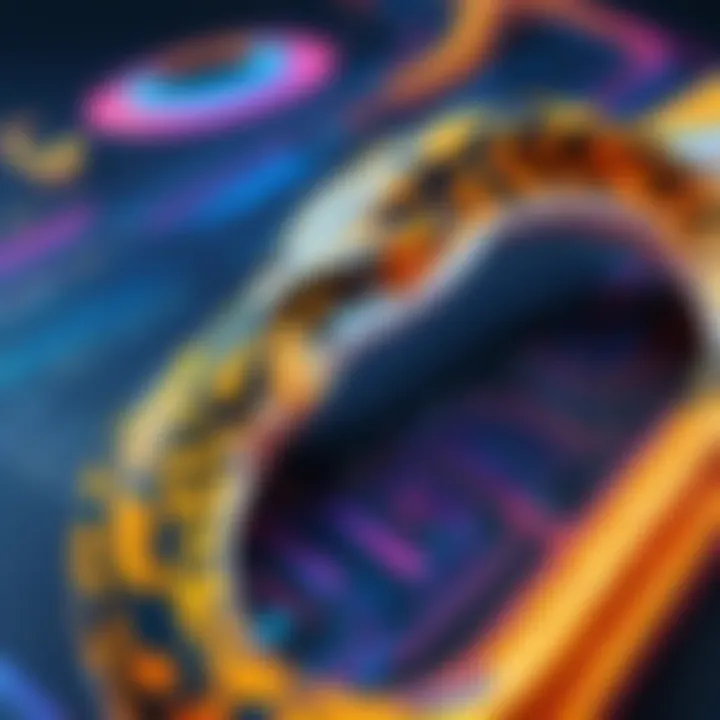
Introduction to Programming Language
Python, a versatile and dynamic programming language, has ushered in a new era in software development due to its user-friendly syntax and powerful capabilities. With a rich history and widespread adoption across various domains, Python continues to solidify its position as one of the most popular programming languages in the world. Its elegant features and expansive scope make it an ideal choice for both beginners and seasoned developers alike.
Basic Syntax and Concepts
When delving into Python programming, one must first grasp the fundamental building blocks that form the language's syntax and concepts. From variables and different data types such as integers, strings, and lists, to operators and expressions that manipulate these variables, Python offers a robust foundation for developing efficient code. Understanding control structures like loops and conditional statements further enhances one's ability to navigate and manipulate data within Python programs.
Advanced Topics
Beyond the basics lie advanced topics that elevate one's Python proficiency to greater heights. Exploring functions and methods allows programmers to modularize their code, promoting reusability and organization. Object-oriented programming introduces concepts like classes and inheritance, enabling developers to create complex, scalable applications. Additionally, mastering exception handling ensures graceful error management, enhancing the robustness of Python programs in various scenarios.
Hands-On Examples
To solidify theoretical concepts and apply newfound knowledge, engaging in hands-on examples becomes paramount. Simple programs serve as building blocks, allowing learners to implement basic algorithms and understand Python's execution flow. Intermediate projects present challenges that test one's problem-solving skills and creativity, pushing boundaries and fostering innovation. Code snippets offer concise yet powerful solutions to common programming dilemmas, providing valuable insights into efficient coding practices.
Resources and Further Learning
For individuals seeking to deepen their understanding and proficiency in Python, a plethora of resources and avenues for further learning exist. Recommended books and tutorials cater to diverse learning styles, offering comprehensive insights into advanced Python concepts. Online courses and platforms provide interactive learning experiences, allowing learners to practice in realistic environments. Community forums and groups foster collaboration and knowledge-sharing, creating a supportive ecosystem for Python enthusiasts to engage with like-minded individuals and experts in the field.
Introduction to Lambda Functions
In the realm of Python programming, lambda functions hold a significant role as they offer a concise way to create small anonymous functions. These functions, also known as lambda functions, are pivotal in functional programming paradigms due to their agility and succinctness. As we embark on exploring the intricacies of lambda functions in this comprehensive guide, we will unravel the distinctive characteristics and virtues that make them a valuable tool in a programmer's arsenal.
Defining Lambda Functions
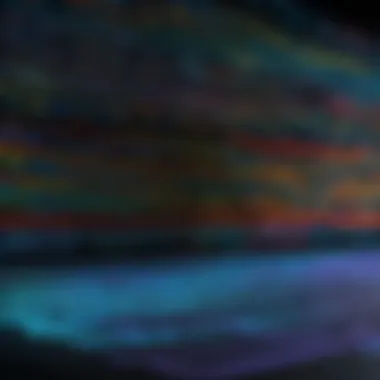
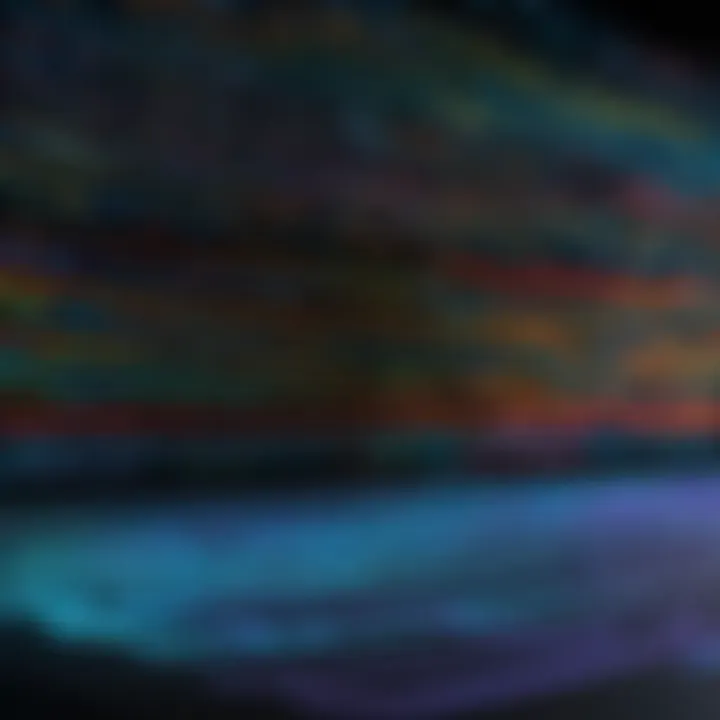
Lambda functions, characterized by their simplicity and compactness, introduce a paradigm shift in how functions are defined in Python. The beauty of lambda functions lies in their ability to be defined in a single line of code, foregoing the need for a formal function definition ceremony. Let's delve into the specifics of
Syntax of Lambda Functions
The syntax of lambda functions is strikingly minimalistic, with the lambda keyword followed by parameters and a single expression. This brevity facilitates the creation of quick throwaway functions, aiding in enhancing code readability and conciseness. Despite its simplicity, the syntax underscores the essence of functional programming by promoting a more declarative style of coding.
Anonymous Function Creation
Anonymous function creation, a hallmark of lambda functions, allows for the dynamic generation of functions without the constraints of naming conventions. This attribute grants programmers the flexibility to craft functions on the fly, where the emphasis is more on the function's purpose rather than its identity. The ability to create functions without assigning them to a variable opens up avenues for streamlined code structures and swift implementation.
Key Characteristics
Within the realm of lambda functions, two key characteristics merit attention for their profound impact on coding efficiency and style.
One-Line Functionality
The one-line functionality of lambda functions embodies brevity without compromising functionality. This succinct nature fosters a coding environment where complex operations can be encapsulated in a single line, promoting a more elegant and compact codebase. However, this brevity comes at a trade-off with readability in more intricate scenarios, necessitating a judicious balance between concise writing and clarity of expression.
Absence of Name Binding
Lambda functions operate without the conventional concept of name binding, meaning they are not tethered to a specific identifier. This feature enables the creation of throwaway functions that can be used directly in line, offering a more immersive and agile coding experience. Despite its benefits in certain contexts, the absence of name binding can pose challenges in debugging and code maintenance, requiring thoughtful consideration when integrating lambda functions into larger codebases.
Implementing Lambda Functions
Implementing Lambda Functions is a crucial aspect of this comprehensive guide on Python lambda functions. Understanding how to effectively utilize lambda functions in Python can greatly enhance a programmer's ability to write cleaner and more concise code. By delving into the implementation details, readers will grasp the significance of incorporating lambda functions into their programming arsenal. This section will explore the key elements, benefits, and considerations that come with integrating lambda functions in practical scenarios.
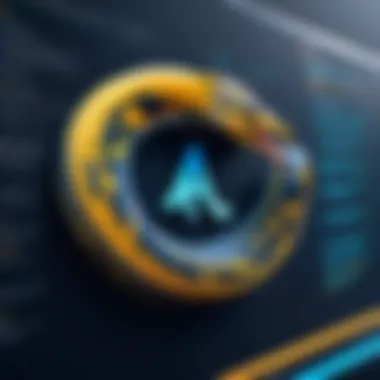
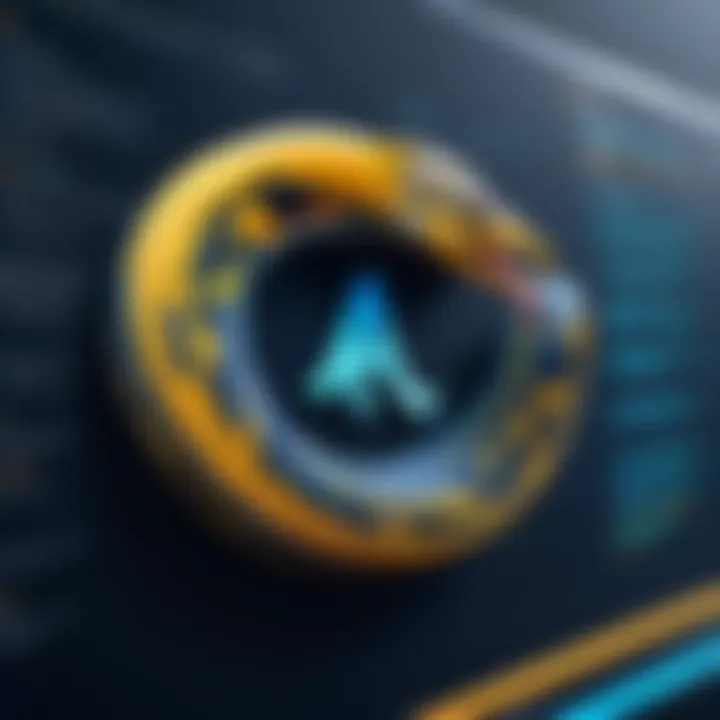
Common Use Cases
Sorting Lists
Sorting Lists is a fundamental operation in programming that plays a vital role in various applications. When it comes to lambda functions, sorting lists is particularly intriguing due to its ability to offer a streamlined approach to arranging data. The inherent simplicity of lambda functions allows programmers to implement sorting logic efficiently without the need for elaborate functions. This makes sorting lists a popular choice for showcasing the power of lambda functions, emphasizing their versatility and effectiveness in handling data manipulation tasks.
Filtering Data
Filtering Data is another essential function that lambda expressions excel at. By leveraging lambda functions for data filtering, programmers can swiftly sift through massive datasets to extract specific information based on defined criteria. The beauty of using lambda functions for filtering lies in their concise yet powerful nature, enabling users to succinctly define complex filtering conditions. While this method is advantageous for its brevity and clarity, it may require careful consideration to ensure optimal performance in data-intensive operations.
Integration with Built-In Functions
Map() Function
The Map() Function holds a pivotal role in demonstrating the seamless integration of lambda functions with built-in Python functions. By harnessing the Map() Function in conjunction with lambda expressions, programmers can perform element-wise transformations on iterables with remarkable ease. This integration not only showcases the expressive nature of lambda functions but also highlights their compatibility with existing Python functionalities. Understanding how to effectively leverage the Map() Function alongside lambda expressions enhances a programmer's ability to manipulate data structures efficiently.
Filter() Function
The Filter() Function complements lambda functions by providing a mechanism for selectively extracting elements from iterables based on specified criteria. When combined with lambda expressions, the Filter() Function empowers programmers to isolate elements that meet particular conditions, creating tailored subsets of data effortlessly. This synergy between lambda functions and the Filter() Function underscores the utility of functional programming paradigms in simplifying data processing tasks. By exploring the nuances of integrating lambda functions with the Filter() Function, readers can gain deeper insights into the art of data filtration and extraction.
Advantages of Lambda Functions
Debatably one of the pivotal sections of this article focuses on the advantages that Python lambda functions bring to the programming table. From succinct code expression to functional programming advantages, lambda functions offer a diverse set of benefits. Owing to their ability to condense functions into compact one-liners, lambda functions present a compelling case for developers looking to streamline their code. Moreover, the absence of name binding grants them a sense of flexibility and adaptability, allowing for agile function creation without the constraints of traditional function definition. Understanding these advantages is fundamental to harnessing the full potential of lambda functions in Python.
Concise Code Expression
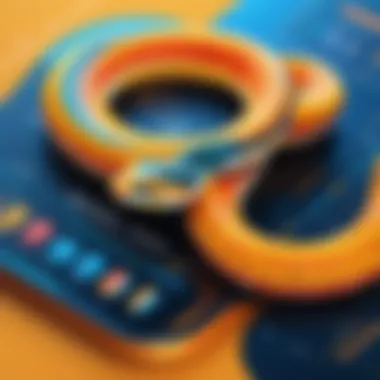
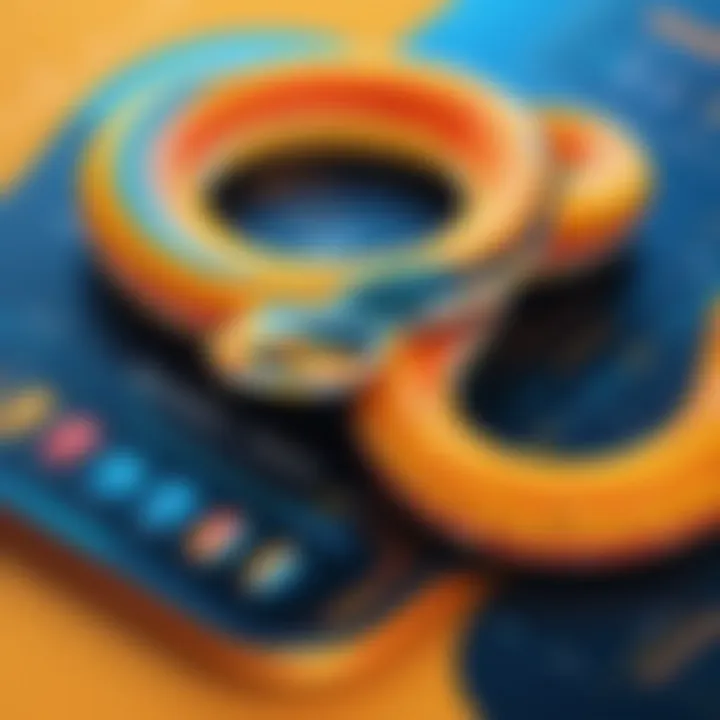
Simplicity and Readability
In the realm of lambda functions, simplicity and readability hold paramount importance. The intrinsic nature of lambda functions to condense complex functions into concise expressions plays a vital role in enhancing code clarity and maintainability. By encapsulating functionality into minimal lines of code, lambda functions promote a more efficient coding style, enabling developers to grasp the logic behind the functions swiftly. The elegance of lambda expressions lies in their simplicity, which not only reduces unnecessary verbosity but also fosters a more streamlined approach to coding. While simplicity and readability are indeed the cornerstone of lambda functions, it is crucial to strike a balance between brevity and comprehensibility to ensure code remains accessible and comprehensible to collaborators or future maintainers.
Functional Programming Paradigm
Higher-Order Functions
Diving into the functional programming paradigm, higher-order functions stand out as a defining feature of lambda functions in Python. The concept of higher-order functions underscores the capacity of functions to operate on other functions, treating them as first-class citizens in the language. This characteristic empowers developers to manipulate functions as data, passing them as arguments or returning them from other functions. By leveraging higher-order functions, lambda functions enable a more modular and flexible programming approach, facilitating the implementation of advanced coding patterns such as map-reduce or filter operations with ease. While higher-order functions open up a myriad of possibilities for functional programming, they also require a deep understanding of function manipulation and abstraction, emphasizing the importance of mastering this aspect for proficient use of lambda functions in Python.
Practical Tips and Best Practices
As we navigate the realm of Python Lambda Functions, understanding the importance of practical tips and best practices becomes paramount. These guidelines serve as the compass guiding developers towards efficient implementation and maintenance of code. By adhering to specific elements such as avoiding complex expressions and applying lambda functions judiciously, programmers can enhance code readability and simplify troubleshooting processes. Embracing practical tips and best practices not only streamlines the coding experience but also fosters a structured approach to utilizing lambda functions effectively.
Optimizing Lambda Usage
Avoiding Complex Expressions
Delving into the intricacies of lambda functions, the notion of avoiding complex expressions emerges as a fundamental consideration for maintaining code clarity and simplicity. By steering clear of convoluted lambda expressions, developers uphold the core principle of lambda functions' concise nature. Simplifying code logic through straightforward lambda expressions minimizes the risk of errors and facilitates seamless comprehension for fellow programmers. Emphasizing simplicity over complexity in lambda functions not only accelerates development processes but also cultivates a more cohesive coding environment.
Applying Lambda in Moderation
In the landscape of Python Lambda Functions, the strategy of applying lambda in moderation underscores the importance of balanced utilization. While lambda functions offer a compact alternative for defining small, one-time-use functions, an overreliance on lambdas can lead to code obfuscation and reduced maintainability. By employing lambda functions judiciously, developers strike a harmonious balance between succinctness and readability in their codebase. Moderation in lambda usage ensures that the functional elegance of lambda functions harmonizes with the overall code structure, promoting code efficiency and manageability.
Testing and Debugging
Unit Testing Lambda Functions
When exploring the domain of testing lambda functions, the practice of unit testing emerges as a critical aspect in ensuring code reliability and functionality accuracy. Conducting rigorous unit tests on lambda functions allows developers to validate the behavior of these succinct functions under various input conditions. By testing individual lambda functions in isolation, programmers can pinpoint and rectify errors efficiently, fostering code robustness and stability. Integrating unit testing as a core practice not only fortifies the quality of lambda functions but also instills confidence in the reliability of the overall application.
Identifying Error Sources
Unraveling the intricacies of lambda functions entails adeptly identifying error sources that may arise during the development process. By honing the skill of pinpointing error origins within lambda functions, developers streamline the debugging process and expedite issue resolution. Detecting and addressing errors promptly elevates code quality and minimizes potential disruptions to application functionality. Equipping oneself with the ability to swiftly identify error sources in lambda functions empowers developers to cultivate resilient and efficient codebases, propelling software development initiatives towards success.