In-Depth Exploration of Python Interview Problems for Success
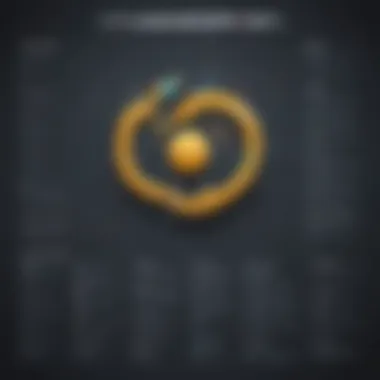
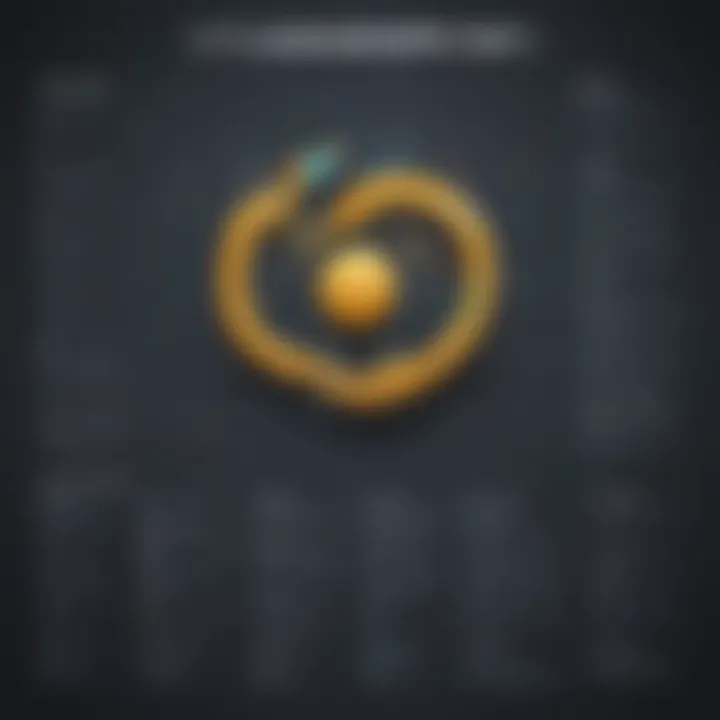
Introduction to Python Programming
Python, a versatile and high-level programming language, originated in the late 1980s and was officially released in 1991 by its creator, Guido van Rossum. Known for its readability and simplicity, Python gained popularity rapidly due to its emphasis on code clarity and ease of use. It has a wide range of applications, including web development, data science, artificial intelligence, and automation. The language's flexibility and dynamic typing make it a go-to choice for developers worldwide.
Basic Principles and Fundamentals
In Python, variables serve as containers for storing data, with each variable assigned a data type based on the value it holds. The language supports various data types, such as integers, floats, strings, lists, tuples, and dictionaries. Operators enable the manipulation of variables and values through arithmetic, comparison, and logical operations. Control structures like loops and conditional statements govern the flow of program execution, allowing for efficient decision-making and iteration.
Advanced Concepts and Techniques
In the realm of more advanced Python programming, functions and methods play a pivotal role in breaking down complex tasks into manageable units of code. Object-Oriented Programming (OOP) is a powerful paradigm in Python, facilitating the creation of reusable and organized code structures through classes and objects. Exception handling ensures that programs gracefully manage errors and unexpected events, enhancing reliability and fault tolerance.
Practical Application and Demonstration
To solidify understanding, engaging in hands-on examples proves invaluable. Starting with simple programs to grasp fundamental concepts, moving on to intermediate projects to apply acquired knowledge, and utilizing code snippets to enhance coding efficiency are essential steps in the learning process. Practical experience not only reinforces theoretical learning but also fosters creativity and problem-solving skills.
Learning Resources and Continued Growth
For individuals seeking to expand their Python proficiency, various resources are available. Recommended books and tutorials offer comprehensive insights and guidance, while online courses and platforms provide interactive learning experiences. Community forums and groups serve as valuable spaces for collaboration, knowledge sharing, and networking within the Python community, fostering continual growth and exploration of the language.
Introduction
Understanding the Significance of Python in Interviews
When it comes to technical interviews, Python holds significant importance due to its versatility, readability, and widespread adoption in the industry. Employers often use Python coding problems to evaluate a candidate's programming proficiency, logical reasoning, and problem-solving abilities. Therefore, being well-versed in Python interview questions and solutions is indispensable for individuals seeking roles in fields such as software development, data science, and machine learning. To prepare effectively for Python interviews, candidates must not only have a strong grasp of Python syntax and concepts but also be adept at applying these skills to solve real-world problems efficiently.
Preparing for Python Interview Questions
Preparing for Python interview questions requires a strategic approach that involves familiarizing oneself with a diverse range of topics, including data structures, algorithms, object-oriented programming, and problem-solving techniques. Candidates should practice solving different types of Python problems, ranging from basic to advanced complexity levels, to build their confidence and proficiency. Additionally, being able to explain the rationale behind their code solutions and demonstrate good coding practices is essential during technical interviews. Effective preparation for Python interview questions involves regular practice, continuous learning, and staying updated on the latest industry trends and best practices. By investing time and effort in mastering Python problems, candidates can significantly enhance their chances of success in technical interviews and secure rewarding career opportunities in the tech industry.
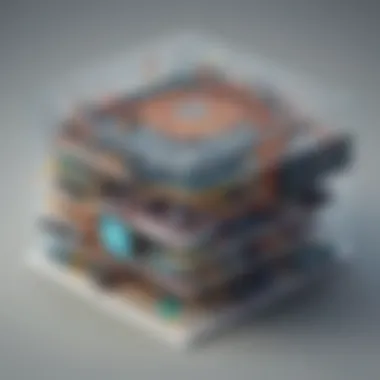
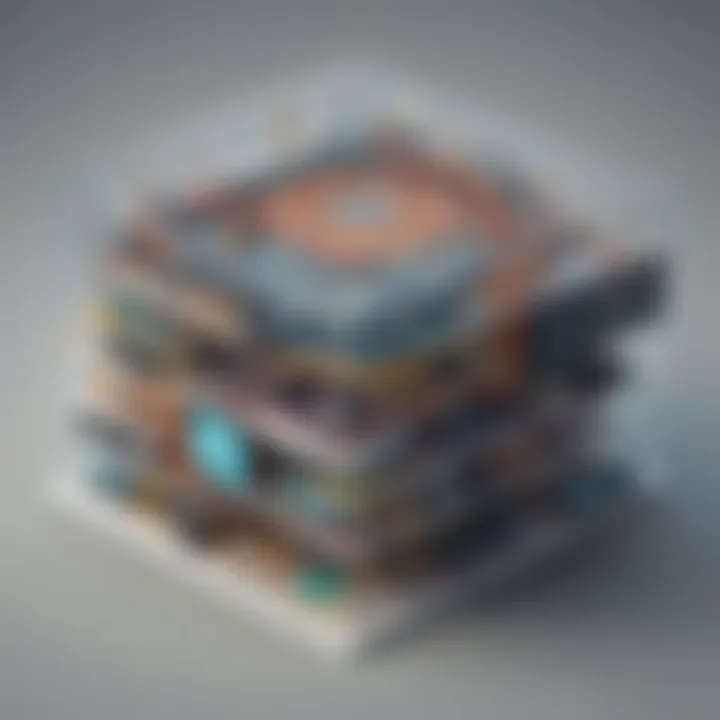
Data Structures and Algorithms
In the realm of technical interviews, mastering Data Structures and Algorithms is the cornerstone of success. These concepts form the bedrock of problem-solving abilities, crucial in evaluating a candidate's proficiency. Data Structures aid in organizing and storing data efficiently, while Algorithms provide the blueprint for solving complex computational problems. Understanding these concepts not only enhances coding skills but also refines logical and analytical thinking.
Arrays and Strings
Reversing a String
Reversing a String entails flipping the elements within a string, altering their order. This operation is fundamental in programming challenges involving data manipulation and pattern recognition. By reversing a string, programmers can unravel hidden patterns or verify symmetrical properties within the input data. Despite its simplicity, this exercise sharpens one's array processing skills and fosters a deeper understanding of string manipulation techniques.
Finding the Duplicate Element in an Array
The process of identifying duplicate elements within an array is instrumental in efficient data handling and error prevention. This operation prevents redundancy and ensures data integrity, particularly in scenarios where data accuracy is paramount. By detecting duplicates, programmers can streamline data operations and optimize memory usage. However, intensive duplicate checks may impact performance in large datasets, necessitating efficient algorithms to balance accuracy and speed.
Linked Lists
Detecting a Cycle in a Linked List
Detecting a cycle in a linked list entails identifying repetitive patterns in the list structure, critical for maintaining data integrity and preventing infinite loops. This functionality is vital in ensuring the security and efficiency of linked list operations, particularly in traversal and data retrieval. By spotting cycles, developers can institute safeguards to enhance algorithmic efficiency and prevent system crashes. However, intricate cycle detection mechanisms may introduce complexities that require meticulous handling.
Reversing a Linked List
Reversing a linked list involves flipping the pointer direction of nodes, altering the list's traversal order. This operation is pivotal for optimizing data access patterns and streamlining list manipulation operations. By reversing a linked list, programmers can enhance search efficiency and improve memory utilization. Nevertheless, improper handling of reversals may lead to memory leaks or unintended side effects, underscoring the importance of meticulous implementation and testing.
Stacks and Queues
Implementing a Stack using Queues
Implementing a stack using queues involves simulating stack behavior through queue operations, leveraging first-in, first-out principles. This emulation facilitates stack functionalities like push and pop while maintaining queue integrity. Such implementations offer a hybrid approach to data structure design, combining the benefits of both stacks and queues. However, intricacies in implementing stack operations using queues may introduce complexities in managing data flow and access patterns.
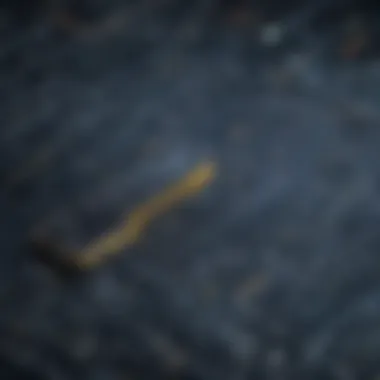
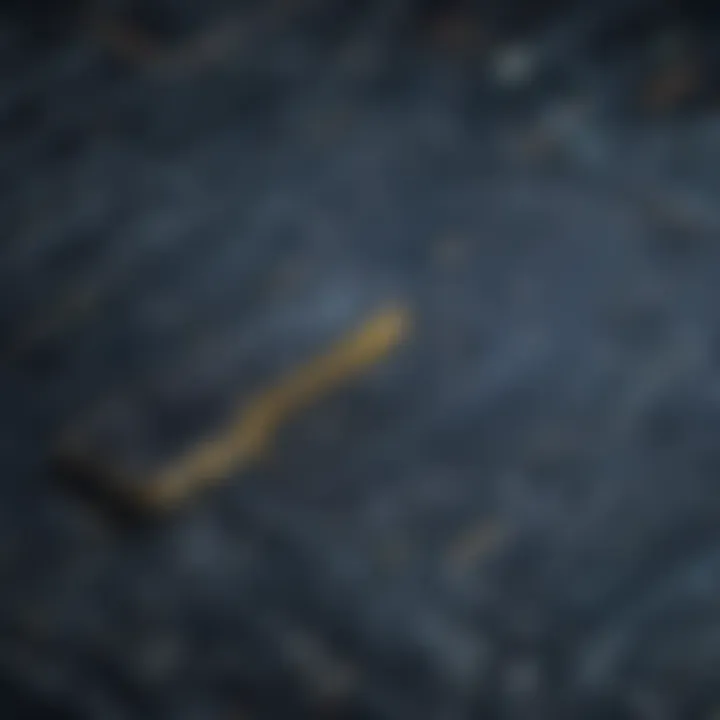
Checking for Balanced Parentheses
Verifying balanced parentheses involves ensuring the symmetrical arrangement of opening and closing brackets, imperative in expression evaluation and syntax validation. This process mitigates parsing errors and enhances code accuracy, particularly in arithmetic or logical expressions. By confirming balanced parentheses, programmers can preempt runtime exceptions and logic distortions. Nonetheless, stringent validation criteria for parentheses balance may impose restrictions on expression complexity, necessitating adaptable algorithms for diverse use cases.
Trees and Graphs
Traversing a Binary Tree
Traversing a binary tree entails navigating tree nodes in a specific order, crucial for exploring tree structures and data retrieval. This traversal method enables efficient data access and search within tree hierarchies, optimizing algorithm performance. By mastering binary tree traversal, programmers can implement sophisticated data processing algorithms and enhance computational efficiency. Despite its benefits, traversal algorithms may vary in complexity based on tree shape and size, demanding tailored solutions for optimal traversal outcomes.
Detecting a Cycle in a Graph
Identifying cycles in a graph involves uncovering repetitive paths within graph structures, significant for route optimization and network analysis. This capability aids in identifying circuitous routes and streamlining data flow, essential for graph traversal algorithms. By detecting cycles, developers can refine graph connectivity analysis and avert recursive data processing pitfalls. However, intricate cycle detection algorithms may introduce computational overhead, influencing algorithm scalability and performance optimization.
Dynamic Programming
Dynamic programming plays a pivotal role in mastering Python problems for interviews. This advanced problem-solving technique involves breaking down complex problems into simpler subproblems to optimize efficiency and reduce redundancy in computations. By storing the results of subproblems in a table and utilizing them when needed, dynamic programming enhances algorithm performance, making it essential for tackling challenging coding tasks effectively. Understanding dynamic programming allows developers to devise elegant and efficient solutions for a wide range of problems, greatly benefiting those aiming to excel in technical interviews.
Fibonacci Series
The Fibonacci series is a classic example frequently used to illustrate the application of dynamic programming. By calculating the Fibonacci numbers iteratively or recursively, programmers can demonstrate the concept of overlapping subproblems and optimal substructure, key principles underpinning dynamic programming. Solving the Fibonacci series efficiently involves storing intermediate results in an array or table, enabling quick access and minimizing redundant calculations. Mastering the Fibonacci series not only sharpens problem-solving skills but also fosters a deeper understanding of dynamic programming's mechanics and applications.
Longest Common Subsequence
In the realm of dynamic programming, the longest common subsequence problem presents a significant challenge for developers. This problem seeks to find the longest subsequence shared between two sequences, requiring a dynamic programming approach for optimal solution. By identifying repeated subproblems and leveraging memoization or tabulation techniques, programmers can efficiently determine the longest common subsequence, showcasing the power and versatility of dynamic programming. Mastering this problem not only hones algorithmic thinking but also equips individuals with a valuable skill set crucial for excelling in Python-related technical interviews.
Sorting and Searching
In the realm of technical interviews, understanding the significance of sorting and searching algorithms holds immense value. Employers often gauge a candidate's problem-solving abilities and algorithmic thinking skills through these topics. Sorting algorithms like Merge Sort play a vital role in organizing data efficiently, enhancing the performance of applications handling large datasets. On the other hand, searching algorithms, such as Binary Search, are instrumental in quickly finding specific elements within a sorted collection, optimizing search processes. Candidates must grasp the intricacies of these algorithms, including their time and space complexities, to effectively tackle interview challenges related to data organization and retrieval.
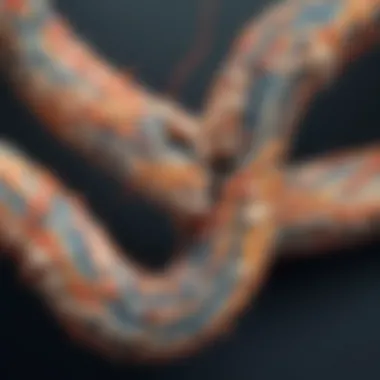
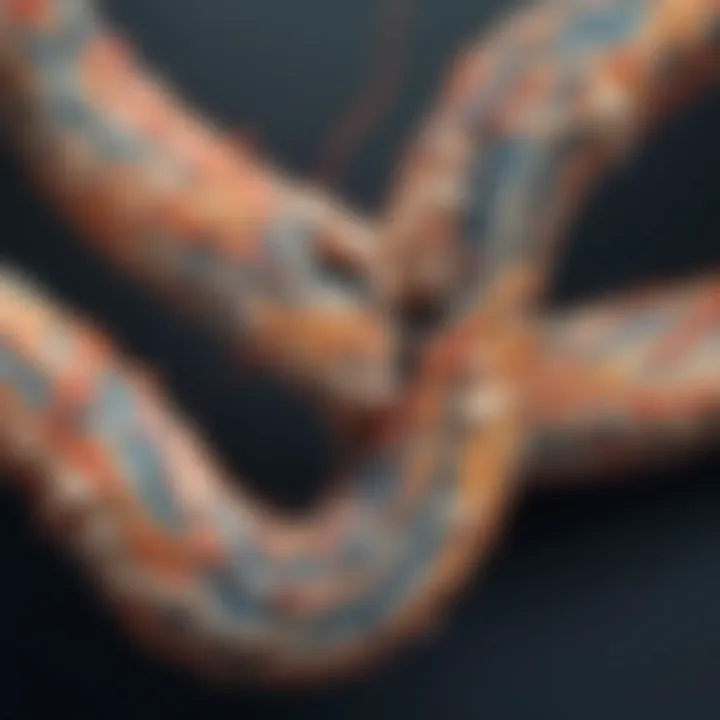
Merge Sort
When delving into the intricacies of sorting algorithms, Merge Sort emerges as a powerful and efficient technique for sorting arrays. Its divide-and-conquer approach involves breaking down the array into smaller sub-arrays, sorting them individually, and merging them back in a sorted manner. Merge Sort guarantees a time complexity of O(n log n), making it suitable for handling large datasets with optimal performance. By comprehensively explaining the implementation and working principles of Merge Sort, aspiring Python developers can enhance their problem-solving skills and be better prepared to tackle sorting-related interview questions.
Binary Search
Binary Search stands out as a fundamental searching algorithm, renowned for its effectiveness in locating elements within a sorted collection. Its divide-and-conquer strategy enables rapid searches by continuously halving the search space until the target element is found. With a time complexity of O(log n), Binary Search offers significant advantages in scenarios requiring efficient search operations. Mastering the intricacies of Binary Search equips candidates with the ability to swiftly locate elements, demonstrating their proficiency in algorithmic thinking during technical interviews. By emphasizing the importance of understanding and implementing Binary Search, individuals can boost their problem-solving capabilities and enhance their performance in Python interview assessments.
Additional Python Concepts
In the realm of Python programming, delving into additional concepts beyond the basics is imperative to enhance one's proficiency and versatility. This section serves as a crucial component in our comprehensive guide to mastering Python interview challenges. By exploring advanced topics like Object-Oriented Programming and Exception Handling, aspiring Python developers can elevate their coding skills to tackle complex problems with finesse. Understanding these concepts is not merely advantageous but rather essential for individuals aiming to excel in technical assessments and interviews.
Object-Oriented Programming
Inheritance and Polymorphism:
A fundamental concept within Object-Oriented Programming is Inheritance and Polymorphism. These principles enable code reusability and promote a modular approach to programming. Inheritance allows classes to inherit attributes and methods from other classes, facilitating the creation of a hierarchical structure that saves time and enhances readability. On the other hand, Polymorphism permits objects of different classes to be treated as instances of a common superclass, fostering flexibility and simplifying complex implementations. The versatility and efficiency offered by Inheritance and Polymorphism make them indispensable tools for solving intricate coding challenges encountered in interviews.
Class Methods and Instance Methods:
Another pivotal aspect of Object-Oriented Programming is understanding Class Methods and Instance Methods. Class Methods are functions that operate on the class itself while Instance Methods are functions that interact with specific class instances. Class Methods provide functionality that is not tied to a specific instance, offering a broader scope of operations, whereas Instance Methods deal with unique object attributes, enhancing the object-oriented nature of Python programming. The distinction between Class Methods and Instance Methods is crucial in designing robust and scalable Python applications, amplifying code organization and facilitating seamless maintenance.
Exception Handling
Exception Handling plays a vital role in ensuring the reliability and robustness of Python code. By anticipating and dealing with errors and exceptions, developers can prevent program crashes and bolster user experience. Exception Handling involves using try-except blocks to gracefully manage unforeseen circumstances and maintain program integrity. Through strategic error handling, Python developers can diagnose and troubleshoot issues effectively, enhancing the overall reliability and user satisfaction of their applications. Embracing proper Exception Handling practices is paramount for aspiring Python programmers to exhibit competency and diligence in addressing potential pitfalls during technical assessments and interviews.
Conclusion
In the realm of mastering Python problems for interviews, the conclusion serves as the culmination of a candidate's proficiency displayed throughout the technical assessment process. This final section encapsulates the significance of adeptly navigating through the intricacies of Python coding challenges and demonstrating a robust understanding of fundamental concepts. The conclusion acts as a summary of the article, reinforcing the idea that success in Python interviews hinges on not just problem-solving skills but also on a holistic comprehension of data structures, algorithms, and Python syntax. It emphasizes the importance of refining one's coding abilities continually to stay competitive in the job market, marking the conclusion as a critical takeaway for aspiring Python developers. By comprehensively integrating the key learnings unearthed in this article, individuals can equip themselves with the prowess needed to ace technical interviews and advance their careers.
Mastering Python Problems for Success
In the pursuit of mastering Python problems for success, candidates are prompted to delve deep into a wide array of coding challenges that stimulate analytical thinking and problem-solving skills. This section emphasizes the necessity of honing not only technical competencies but also the ability to strategize efficiently under pressure. Aspirants are encouraged to practice coding consistently, using a variety of platforms and resources to broaden their understanding of Python concepts. By tackling diverse problem sets and delving into complex algorithms, individuals can fortify their coding acumen and build a robust portfolio that showcases their proficiency. Mastering Python problems lays a solid foundation for excelling in technical interviews, enabling candidates to approach challenges with confidence and precision.
Continuous Learning and Practice
Continuous learning and practice play a pivotal role in the journey of aspiring Python developers aiming to excel in interviews. This section underscores the significance of consistent engagement with coding exercises, challenges, and real-world projects to sharpen skills and deepen understanding. By committing to a regimen of regular practice, individuals can cultivate problem-solving abilities, enhance their fluency in Python syntax, and develop a strategic approach to tackling complex coding scenarios. Furthermore, embracing a growth mindset and staying abreast of new developments in the Python landscape is essential for staying competitive in a dynamic tech industry. Continuous learning and practice not only solidify existing knowledge but also foster adaptability and innovation, equipping candidates with the agility needed to thrive in a rapidly evolving job market.