Exploring Python in Data Structures and Algorithms
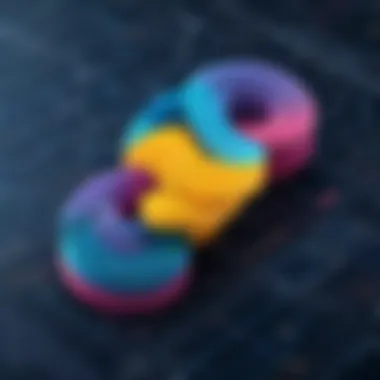
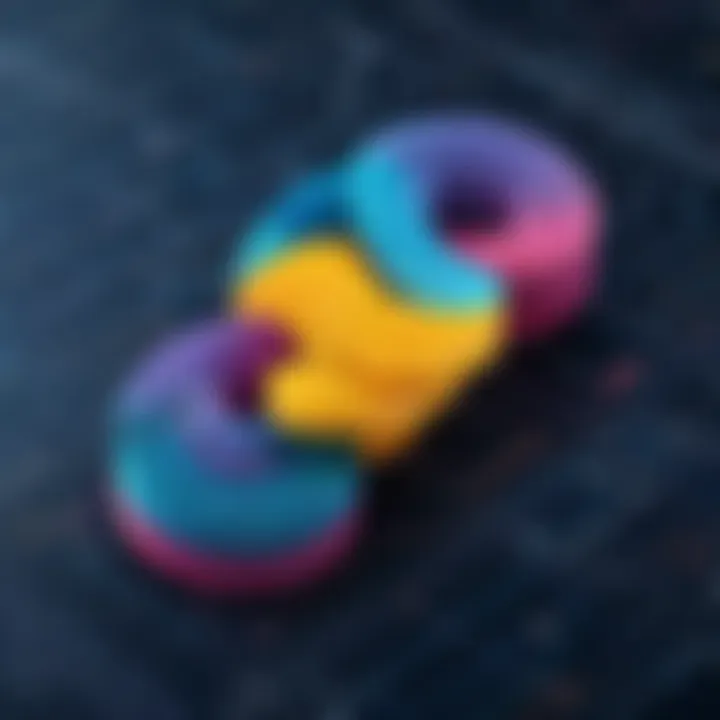
Prelims to Programming Language
History and Background
Python was created by Guido van Rossum and first released in 1991. The language has grown significantly over the years. It has gained favor among programmers due to its simplicity and readability. Python drew inspiration from earlier languages like ABC, combining ease of use with powerful features. Today, it stands as a versatile tool for a variety of applications, from web development to scientific computing. Its foundational philosophy is that code should be easy to read and write, which is especially important in the context of learning data structures and algorithms.
Features and Uses
Python is known for several key features:
- Simplicity: The clean syntax makes it accessible for beginners.
- Versatility: It can handle different tasks, making it suitable for everything from data analysis to machine learning.
- Comprehensive Libraries: Python boasts numerous libraries, including NumPy for numerical calculations and Pandas for data manipulation.
These qualities make Python an appealing choice for learning about data structures and algorithms, as they allow learners to focus on concepts without getting bogged down by complex syntax.
Popularity and Scope
The popularity of Python has surged in recent years. It ranks high on the TIOBE index and is widely used in industry. The language's community contributes a wealth of resources and libraries. Its usage spans fields like web development, data science, artificial intelligence, and more. As such, understanding Python becomes crucial for both aspiring developers and those looking to enhance their algorithmic proficiency.
Basic Syntax and Concepts
Variables and Data Types
In Python, variables are fundamental. They store information that you can use later. The main data types include integers, floats, strings, and booleans. For example, a simple variable assignment looks like this:
Operators and Expressions
Python supports various operators, such as arithmetic, relational, and logical operators. Each type facilitates different operations on variables. For instance, you can add integers or check if two values are equal. Using operators allows you to construct expressions for calculations and comparisons that are crucial when designing algorithms.
Control Structures
Control structures, such as loops and conditionals, direct the flow of a program. The statement lets you execute code based on conditions. Loops, like and , allow repetitive execution of code segments. Effective use of these structures is essential for implementing algorithms efficiently.
Advanced Topics
Functions and Methods
Functions are reusable code blocks that perform a specific task. In Python, you define functions using the keyword. For example:
This function greets a user by name. Functions encapsulate functionality, promoting cleaner code in algorithm implementation.
Object-Oriented Programming
Python supports object-oriented programming, where concepts like inheritance and encapsulation foster code organization. Creating classes to model data structures lays a strong foundation for more complex algorithms. For instance, defining a class for a stack can simplify the implementation of stack-related algorithms.
Exception Handling
Errors are inevitable in programming. Python uses try-except blocks to handle exceptions. This approach allows you to write robust code that can manage unexpected situations gracefully. For instance:
Effective error handling is vital in algorithm implementation to ensure programs run smoothly.
Hands-On Examples
Simple Programs
Start with basic examples like creating a list and performing operations on it. Such tasks provide practical experience with Python’s syntax and data structures. A code snippet for a simple list might look like this:
Intermediate Projects
As you progress, try building more complex projects, like a to-do list application or a simple game. Intertwining data structures into these projects enhances your understanding of algorithms' practical side. You will apply your knowledge as you troubleshoot and refine your code.
Code Snippets
Various code snippets can illustrate implementation of algorithms. For instance, here’s a simple bubble sort algorithm:
Resources and Further Learning
Recommended Books and Tutorials
- "Automate the Boring Stuff with Python" by Al Sweigart
- "Python Crash Course" by Eric Matthes
- Online platforms like Codecademy provide a structured path for learning Python.
Online Courses and Platforms
Websites like Coursera and edX offer courses specifically focused on data structures and algorithms in Python. Such educational resources provide guided instruction tailored to various proficiency levels.
Community Forums and Groups
Engaging with communities can enhance your learning experience. Joining forums like Reddit's r/learnpython or Facebook groups can help you connect with other learners. These platforms provide a space for discussions, queries, and sharing resources.
Engagement with the community can be invaluable. It connects learners and mentors, fostering growth and understanding in programming.
Exploring these resources will augment your knowledge and enrich your understanding of using Python for data structures and algorithms.
Prelude to Data Structures and Algorithms
Data structures and algorithms form the backbone of computer science. They are essential for processing data effectively and solving complex problems. In this article, we explore how Python, a widely used programming language, is suited for understanding and implementing these concepts. This section serves as an introduction, laying the foundation for the detailed discussions that follow.
Understanding Data Structures
Data structures refer to the organized ways of storing and managing data. They enable efficient access and modification of data. Python supports various built-in data structures, such as lists, sets, and dictionaries, which provide flexibility and ease of use. Each type is designed for specific applications, making it crucial for developers to understand their properties.
- Lists: Dynamic arrays that hold ordered collections of items. Easy to add or remove elements.
- Dictionaries: Key-value pairs ideal for fast lookups based on unique keys, allowing quick data retrieval.
- Sets: Unordered collections of unique elements. Useful for operations like union and intersection.
These structures are fundamental because they influence the efficiency of algorithms performed on them. A poor choice in data structure can lead to slower execution times and increased complexity.
Importance of Algorithms
Algorithms are step-by-step procedures or formulas for solving problems. They dictate how efficiently data is processed and analyzed. In programming, selecting the right algorithm is crucial. It ensures optimal use of resources such as time and memory. A few key points about algorithms are:
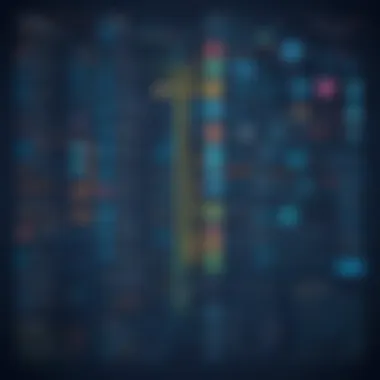
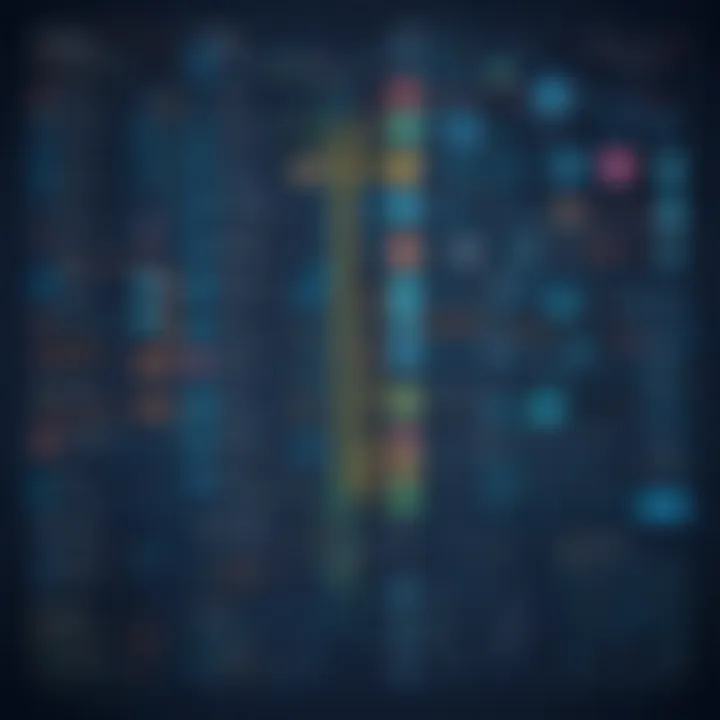
- Efficiency: The speed at which an algorithm executes, often analyzed in terms of time complexity.
- Scalability: An algorithm’s ability to adapt and perform well as the data size grows.
- Versatility: The solution strategies that can be applied to various problems.
Understanding algorithms helps programmers choose the best method for a given problem, optimizing performance. Sorting and searching algorithms, for example, can significantly impact how quickly data is retrieved or organized.
"Choosing the right algorithm is as crucial as using the right tools in construction. It can determine the success of a project."
In summary, a solid grasp of data structures and algorithms is essential for anyone looking to excel in programming. They are not just abstract concepts but practical tools that can lead to efficient, effective solutions in real-world applications.
Why Choose Python?
Python has emerged as a dominant programming language that resonates well with both beginners and experienced coders. The suitability of Python for data structures and algorithms can be dissected into two critical components: its simplicity and readability, alongside its rich library support. Understanding these elements helps to appreciate why Python is often the preferred choice for many programming tasks.
Simplicity and Readability
One of the strongest advantages of Python is its simplicity. The language is designed to be straightforward, allowing developers to express concepts in fewer lines of code compared to languages such as Java or C++. This efficient use of syntax reduces cognitive load, making it easier to understand and maintain the codebase.
Readability is another cornerstone of Python. The clean and visually uncluttered code makes it intuitive. For example:
In this snippet, you can quickly grasp the intention of each line. A beginner does not need extensive training to understand basic operations. This promotes better learning for novice programmers, as they can focus on algorithms and data structures without wrestling with complex syntax. The emphasis on readability also facilitates collaboration, as teams can easily comprehend each other’s code.
Additionally, Python enforces best practices through its design. For instance, it uses indentation to denote code blocks, which encourages clean coding habits. This promotes not only better organization but also a deeper understanding of flow control within the code.
Rich Library Support
The strength of Python also lies in its extensive libraries and frameworks. The Python Standard Library provides modules that implement many useful functionalities, making it easier to handle data structures and algorithms right out of the box. For instance, the module offers several specialized data structures, like named tuples and deque, that can be utilized instead of creating custom implementations.
Beyond the standard library, numerous third-party libraries exist to enhance Python’s capabilities. Libraries such as NumPy and Pandas streamline operations on numerical and tabular data, thereby facilitating high-level data manipulation and analysis. In addition:
- SciPy leverages Python for scientific computing with numerous available functions.
- NetworkX aids with graph algorithms, enhancing the exploration of graphs in an intuitive manner.
- Matplotlib helps visualize data, making it easier to analyze performance or results of algorithms.
These libraries significantly reduce the amount of code you need to write, allowing programmers to focus on solving complex problems without getting bogged down by low-level details.
Python's library ecosystem is designed to help programmers avoid reinventing the wheel, promoting productivity while ensuring efficiency in solving problems.
Leveraging these resources, Python programmers can implement complex algorithms and data structures more efficiently than many other programming languages support, which, in turn, accelerates the development process.
In summary, the combination of simplicity, readability, and extensive library support makes Python a noteworthy contender in the field of programming, especially when delving into data structures and algorithms. These attributes collectively foster a conducive environment for learning and applying programming principles.
Fundamental Data Structures in Python
Understanding fundamental data structures is essential in programming. These structures form the building blocks for efficient data manipulation and storage. In Python, they facilitate various operations while maintaining clarity and efficiency. Choosing the right data structure can lead to optimized performance in algorithms. This section will delve into the core data structures such as lists, tuples, dictionaries, and sets.
Lists
Lists are one of the most widely used data structures in Python. They offer a flexible way to store an ordered collection of items. With lists, you can easily add, remove, or change elements. This makes them particularly useful in scenarios where data changes frequently. Lists are indexed, meaning you can access elements using their position. They can also hold items of different types, which adds to their versatility.
Considerations for lists include their performance. Operations such as insertion or deletion can be costly in terms of time if they occur in the middle of the list. However, accessing an element by index is very quick, typically operating in constant time.
Tuples
Tuples are similar to lists but are immutable. Once a tuple is created, its contents cannot be changed. This property makes tuples suitable for storing fixed sets of values. For example, you might use a tuple to hold coordinates. The immutability of tuples provides advantages regarding performance. They are usually faster than lists when it comes to iteration and access.
The use of tuples can help prevent accidental changes to data. This characteristic plays a crucial role in maintaining integrity in programs.
Dictionaries
Dictionaries are key-value pairs that allow for efficient data retrieval. When you need to store data and want to efficiently look it up, dictionaries are invaluable. You can think of them as a mapping of unique keys to corresponding values. This structure supports fast lookups, making it a great choice for implementing associative arrays or databases.
It is important to note that keys must be unique. Attempting to add a duplicate key will overwrite the existing value. This behavior can be useful but should be used with caution.
Sets
Sets are used to store unique items only. They are unordered collections, meaning that the items do not have a defined order. This is beneficial when you want to eliminate duplicates from a collection of data. Operations like union, intersection, and difference can also be performed on sets, making them very useful for mathematical purposes.
Sets provide efficient methods for membership testing and duplication removal, important for optimizing performance when managing large datasets.
The right choice of data structure can significantly enhance the performance of algorithms you implement.
Choosing the appropriate fundamental data structure in Python can lead to better performance and clearer, more maintainable code. Each structure has its unique features and benefits, making it critical to understand them for effective programming.
Complex Data Structures
Complex data structures are essential in the realm of programming, serving as foundational elements that allow for efficient data manipulation and representation. Their significance becomes particularly evident when dealing with large datasets or intricate relationships among data points. Understanding complex data structures not only enhances your ability to implement algorithms effectively but also improves the overall performance of your programs.
These structures go beyond simple variable types, enabling programmers to manage data in a more sophisticated way. By utilizing stacks, queues, linked lists, trees, and graphs, developers can solve problems that require dynamic memory management, hierarchical data representation, or connections between data points. With Python, these complex structures can be implemented effortlessly, owing to its rich set of built-in tools and libraries.
Stacks
Stacks are a fundamental data structure that operates on a Last In, First Out (LIFO) principle. They are best understood through visualization: consider a stack of plates. You can only add or remove the top plate, not any plate deeper in the stack. This behavior is particularly useful in various applications - such as reversing strings, parsing expressions, and backtracking algorithms. When implementing stacks in Python, the type is most often utilized due to its dynamic sizing and ease of use.
Key operations of stacks include:
- Push: Add an element to the top of the stack.
- Pop: Remove and return the top element.
- Peek: Return the top element without removing it.
Queues
Queues, following the First In, First Out (FIFO) principle, are similar to stacks but operate differently. Imagine a line of people waiting to enter a concert; the first person in line gets to enter first. Queues are favorable for managing tasks that require orderly processing, such as task scheduling or handling requests in web servers.
In Python, queues can be efficiently implemented using the class, which allows for quick appends and pops from both ends.
Core operations for queues comprise:
- Enqueue: Add an element to the end of the queue.
- Dequeue: Remove and return the front element.
- Front: View the front element without removing it.
Linked Lists
Linked lists offer a dynamic alternative to arrays, where elements are stored and linked using pointers. Each element, often referred to as a node, consists of data and a reference to the next node in the sequence. This structure allows for efficient insertion and deletion operations, particularly when manipulating large datasets.
Python does not have a built-in linked list data type, but it is straightforward to implement one. With linked lists, you can build more complex structures like stacks and queues.
Components of a linked list include:
- Node: Contains the data and a reference to the next node.
- Head: The first node in the list.
- Tail: The last node, typically pointing to .
Trees
Trees are hierarchical data structures that play a crucial role in representing relationships among data items. A binary tree is a common type, where each parent node can have at most two children. Trees are useful for operations like searching, sorting, and decision-making.
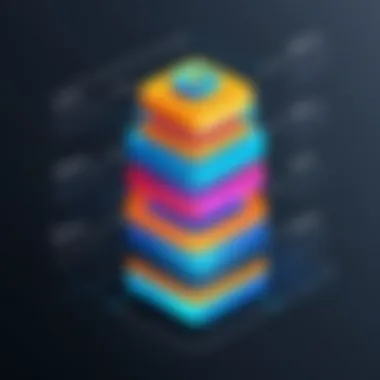
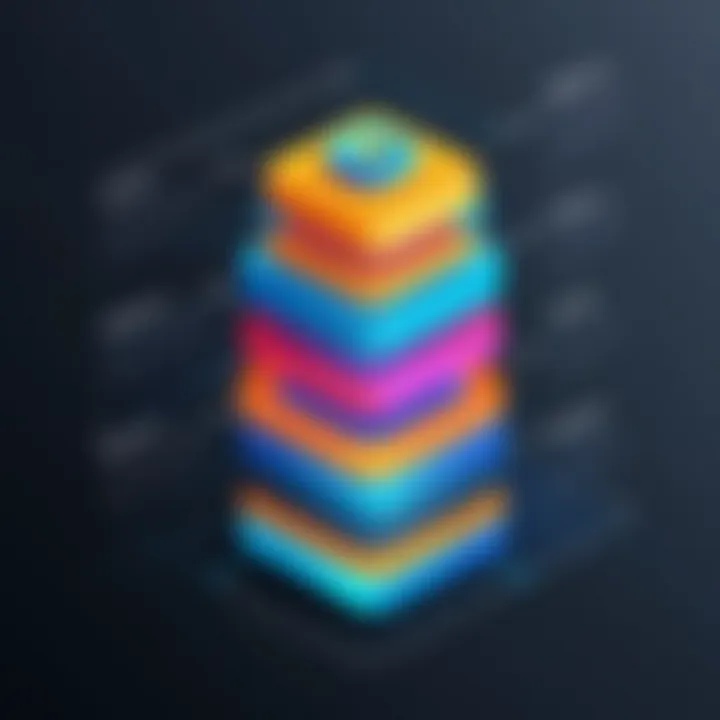
Python’s various libraries support tree structures, allowing for efficient data management in applications such as file systems, databases, and artificial intelligence.
Common tree types include:
- Binary Trees: Each node has a maximum of two children.
- Binary Search Trees: A binary tree where the left child has a lower value and the right child has a higher value.
- AVL Trees: A self-balancing binary search tree where the difference in heights of the left and right subtrees cannot be more than one.
Graphs
Graphs are perhaps the most complex of data structures, capable of representing a multitude of connections among a set of points (vertices). They can be directed, where edges have a direction, or undirected. Graphs are integral in numerous fields, such as social networks, transportation systems, and network topology.
Python provides libraries like NetworkX and Graph-tool to facilitate graph manipulation and analysis.
Key features of graphs include:
- Vertices: Points or nodes.
- Edges: Connections between vertices.
- Weights: Values assigned to edges, often representing costs, distances, etc.
Understanding and effectively implementing these complex data structures helps programmers tackle more advanced problems, ultimately leading to more efficient and optimized code.
Essential Algorithms
Understanding essential algorithms is a cornerstone of programming. In Python, these algorithms enable efficient data processing and problem-solving. This section describes fundamental algorithms that are useful in a variety of applications. It highlights why both sorting and searching algorithms are crucial for programmers.
Sorting algorithms arrange data in a particular order, facilitating easier data manipulation. They come in various flavors, each with unique features and efficiencies. Searching algorithms allow for finding specific data points within datasets. Knowing both types greatly enhances one's ability to write effective code.
Sorting Algorithms
Bubble Sort
Bubble Sort is a basic sorting algorithm. It repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. The process repeats until no swaps are needed, which means the list is sorted. The key characteristic of Bubble Sort is its simplicity, making it an accessible choice for beginners.
- Advantages: Easy to understand and implement; minimal code.
- Disadvantages: Its time complexity is O(n^2), which makes it inefficient for large datasets.
Bubble Sort's main functionality serves as a learning tool rather than a practical sorting solution in most applications.
Merge Sort
Merge Sort takes a different approach by utilizing a divide-and-conquer strategy. It splits the dataset into smaller sublists until these become trivial enough to sort. After sorting the sublists, it merges them back together in a sorted order. The key feature of Merge Sort is its O(n log n) time complexity, offering performance advantages for larger datasets.
- Advantages: Consistent O(n log n) performance; works well with large datasets.
- Disadvantages: Requires additional space for temporary arrays, which may increase memory usage.
For larger applications, Merge Sort is often a preferred choice due to its efficiency, especially when dealing with extensive data.
Quick Sort
Quick Sort is another highly efficient sorting algorithm. It selects a 'pivot' element and partitions the array into two sections: elements less than the pivot and those larger. It then recursively sorts the sections. The most distinguishing feature of Quick Sort is that it often runs faster than Merge Sort in practice, even though both have similar time complexities of O(n log n).
- Advantages: Typically faster than other O(n log n) algorithms; uses less memory compared to Merge Sort.
- Disadvantages: The worst-case time complexity can reach O(n^2) depending on pivot choice.
Quick Sort's efficiency and performance make it widely adopted in real-world applications, emphasizing its practicality in various scenarios.
Searching Algorithms
Linear Search
Linear Search is straightforward. It checks each element in a list until the target value is found or all elements have been checked. The key characteristic here is that this method does not require any sorting of the dataset beforehand.
- Advantages: Simple and easy to implement; works on unsorted data.
- Disadvantages: Inefficient for large datasets with a time complexity of O(n).
Despite its simplicity, Linear Search may not be suitable for cases with large amounts of data unless quick organization is not feasible.
Binary Search
Binary Search is a faster method compared to Linear Search. It only works on sorted datasets. By repeatedly dividing the search interval in half, the algorithm narrows down potential locations for the target value. The key feature is its O(log n) time complexity, making it vastly superior to Linear Search in large datasets.
- Advantages: Significantly faster for sorted data; efficient performance in large datasets.
- Disadvantages: Requires data to be sorted beforehand; not practical for small or unsorted datasets.
The efficiency of Binary Search makes it a critical tool for students and programmers looking for rapid data retrieval methods.
Understanding both sorting and searching algorithms is essential for any programmer looking to enhance their skills in data management and manipulation.
Implementing Data Structures in Python
Implementing data structures in Python is a crucial part of understanding how to efficiently manage and manipulate data. This section emphasizes the practical applications of theoretical concepts within the realm of data structures and algorithms. The ability to implement these structures directly influences the performance and scalability of applications, particularly in programming languages like Python.
In Python, developers benefit from both its inherent simplicity and the extensive library support available. Through practical examples, one can appreciate the utility of data structures while also grasping their underlying principles. This section will cover the implementations of lists as stacks and queues and a detailed look at designing a linked list.
Using Lists as Stacks and Queues
In Python, lists are versatile and can be easily manipulated to behave as stacks and queues. A stack follows a Last In First Out (LIFO) structure, where the last element added is the first one to be removed. Conversely, a queue follows a First In First Out (FIFO) structure, meaning the first element added is the first one to be removed.
To implement a stack with a list, you can use the following methods:
- append() to add an item to the stack
- pop() to remove the most recently added item
Here is an example of implementing a stack using a list:
On the other hand, a queue can be implemented by using the same list but with different methods:
- append() to add an item at the end of the list
- pop(0) to remove the item from the beginning of the list
Example of implementing a queue:
Despite their usefulness, it is important to consider that using lists as queues can lead to inefficiencies, particularly with larger datasets. This is because removing elements from the start of a list has a time complexity of O(n).
Designing a Linked List
A linked list is a more advanced data structure that offers dynamic memory allocation and efficient insertion and deletion of elements. Unlike lists, linked lists do not store data in contiguous memory locations. Instead, each element, known as a node, contains its data and a reference to the next node in the sequence.
To create a simple linked list in Python, one would typically define a Node class that holds the data and the reference to the next node. The linked list itself would manage these nodes.
Below is a basic implementation of a singly linked list:
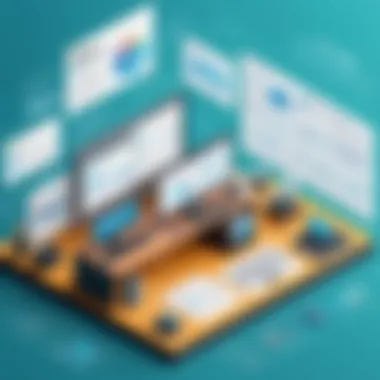
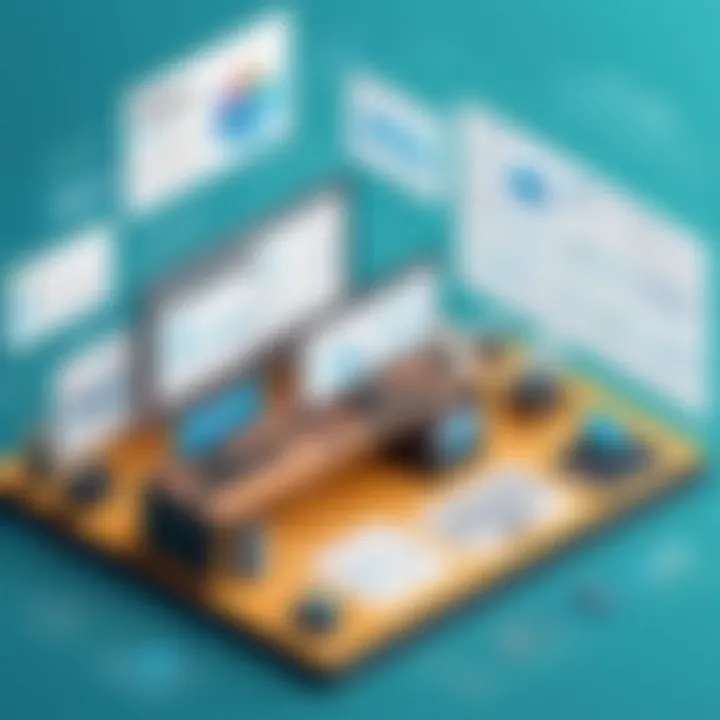
The linked list above starts with a head node and allows adding new elements to the end of the list. This structure is particularly useful when inserting or removing nodes frequently since it maintains a constant time complexity for these operations.
By understanding how to implement data structures in Python, developers can optimize their code and improve its efficiency. This knowledge is foundational for tackling more complex algorithms and data challenges.
Analyzing Algorithm Efficiency
In the realm of programming, particularly when working with data structures and algorithms, analyzing algorithm efficiency is a cardinal subject. Understanding how algorithms perform under varying conditions is vital for optimizing code and improving performance. This section breaks down the critical aspects of evaluating algorithms through time complexity and space complexity.
Time Complexity
Time complexity is a measure that expresses how the runtime of an algorithm increases as the input size increases. Generally, this is represented using Big O notation, which provides a high-level idea of the algorithm's efficiency. For instance, an algorithm with a time complexity of O(n) suggests its runtime will grow linearly with the input size.
Knowing the time complexity helps programmers identify which algorithm to use under certain constraints. For example, if a sorting algorithm like Quick Sort works on average in O(n log n) time but has a worst-case complexity of O(n²), the choice of algorithm becomes critical depending on input characteristics.
Benefits of Time Complexity Analysis
- Performance Prediction: Formulating expectations on how an algorithm behaves with larger data sets.
- Selecting Algorithms: Guiding the choice of data structures and algorithms based on performance needs.
- Identify Bottlenecks: Determining sections in code that require optimization to improve speed.
Space Complexity
Space complexity considers the amount of memory an algorithm needs relative to the input size. Like time complexity, it also utilizes Big O notation for expression. Understanding space complexity is crucial for environment limitations, particularly in lower-resource systems or constrained platforms.
For example, recursive algorithms often exhibit high space complexity due to function call stacks. An iterative solution might prove advantageous in these cases. Understanding the space required by your algorithms ensures you can make informed decisions, balancing between performance and memory usage.
Benefits of Space Complexity Analysis
- Memory Management: Understanding resource requirements helps in efficient allocation and release of memory resources.
- Resource-Constrained Environments: Useful in applications such as mobile development where memory is limited.
- Optimization Avenues: Identifying memory-heavy processes aids in possible optimization efforts to streamline code.
A comprehensive understanding of time and space complexity can lead to a more systematic and effective approach to coding.
Common Pitfalls in Python Programming
Understanding the common pitfalls in Python programming is crucial for both learners and seasoned developers. Python's simplicity can sometimes lead to complacency. As a result, programmers may overlook fundamental principles, leading to inefficient code and unnecessary errors. Recognizing these pitfalls not only aids in writing better code but also improves overall programming practices.
Inefficiencies in Algorithm Implementation
When implementing algorithms in Python, inefficiencies often arise from the choice of algorithm design or execution method. One common mistake is using algorithms that are not suited for the given problem. For example, opting for a bubble sort instead of a merge sort on a large dataset can lead to excessively long processing times. The time complexity of the chosen algorithms should always be considered against the size of the input data.
Moreover, misusing data structures can cause inefficiencies. Using lists for frequent insertions and deletions can severely hinder performance. In such cases, linked lists or deque may offer improved efficiency. Developers must analyze their specific scenarios to ensure they are selecting the right algorithm for the right problem.
"The choice of a suitable algorithm affects not only the performance but also the maintainability of the code."
Mistakes in Data Structure Choice
Selecting the appropriate data structure is pivotal in ensuring efficient data handling. A common error is assuming that the default structures provided by Python, like lists and dictionaries, will suit all scenarios. For instance, using lists for searching data can lead to inefficiencies. Searching through a list utilizes linear time complexity. In contrast, using a set enables faster average-time complexity, providing O(1) access times.
Another mistake is not accounting for mutability. Python lists are mutable, whereas tuples are immutable. Misusing these structures can lead to unintended side effects in programs, especially in multi-threaded applications.
Best Practices for Writing Efficient Code
Code Readability
Code readability refers to how easily a human can understand the logic and intent behind the code. High readability is crucial as it allows programmers to review and modify code without extensive re-familiarization with the codebase. Here are some points to consider:
- Use Meaningful Variable Names: Variables should have clear, descriptive names that indicate their purpose. Avoid ambiguous names that could lead to confusion.
- Consistent Formatting: Employ consistent indentation, spacing, and line breaks. This visual structure aids in understanding the code's flow.
- Commenting: Document important sections of the code with comments. Explain complex logic or why certain decisions were made.
By adopting these practices, novices will not only learn how to write code but how to write code that is easy to read and maintain.
Modular Programming
Modular programming involves breaking down large codebases into smaller, self-contained modules. This approach simplifies development and enhances code management. Key aspects include:
- Encapsulation: Each module should encapsulate its own functionality. This means that changes to one module should not adversely affect others, promoting ease of debugging and enhancement.
- Reuse of Code: Modules allow for reusability across different projects. Developers can leverage existing code, which saves time and minimizes redundancy.
- Testing Simplification: Smaller modules can be tested individually, making it easier to isolate and fix issues.
Modular programming not only promotes organization but also fosters a systematic approach to coding, making it easier for students and beginners to grasp complex concepts.
"Good code is its own best documentation."
In summary, employing best practices in coding is vital for anyone developing algorithms and data structures in Python. Code readability and modular programming are essential components that lead to efficient and manageable code. As students progress in their programming skills, these principles will aid in fostering a deeper understanding of creating scalable and effective solutions.
Real-world Applications of Python Data Structures
Understanding real-world applications of Python data structures is fundamental for anyone looking to become proficient in programming. Knowledge of data structures is not just an academic exercise; it is crucial in creating efficient and effective solutions to real-world problems. In this section, we will explore two main areas of application: web development and data analysis. Both fields heavily rely on various data structures to manage and process information in a way that can significantly enhance performance and usability.
Web Development
Web development encompasses a wide array of tasks, from designing user interfaces to managing backend databases. Python is a popular choice, largely due to its simplicity and versatility. When it comes to web development, various data structures play key roles:
- Lists: Lists are often used to store user data or website content. They are easy to use, allowing developers to append, modify, and delete items quickly. This dynamic nature makes lists suitable for situations where the amount of data is not fixed.
- Dictionaries: In web applications, dictionaries are essential for storing data in key-value pairs. This structure allows for quick access to user preferences, settings, or even session data based on unique identifiers. Their efficiency in lookups is an advantage in both performance and resource management.
- Sets: Sets help eliminate duplicate entries, which is particularly useful in managing user registrations or filtering results in search functionalities. They are optimized for operations concerning unique elements.
Overall, Python’s robust data structures facilitate the development of sophisticated web applications that can dynamically handle various user interactions and data-driven functionalities.
Data Analysis
In the realm of data analysis, Python is favored for its strong libraries, such as Pandas and NumPy, which are built around efficient data structures. Here, data structures are not simply containers for data; they can define the entire approach to how data analysis is executed. Consider the following components:
- DataFrames: DataFrames provided by Pandas are similar to dictionaries but are structured like tables. They allow for organized manipulation of data sets, making it easy to analyze large amounts of data quickly. Analysts frequently use DataFrames to perform operations like filtering, grouping, and aggregating data.
- Arrays: NumPy arrays are designed for numerical computations. These provide better performance for mathematical operations compared to standard Python lists. This efficiency is crucial when analyzing vast data sets, where processing time is often a limiting factor.
- Graphs: In data analysis, visualizing relationships among data points is important. Python’s capabilities in handling graph data structures enable analysts to represent data interactions effectively.
Data analysis not only benefits from these structures but also relies on them to derive insights from data efficiently. By leveraging Python data structures, data analysts can streamline processes, enhance output quality, and ultimately drive informed decision-making.
"Effective use of data structures can significantly reduce runtime and improve the performance of your applications."
Epilogue and Future Learning Paths
In this article, we have traversed through the essential landscape of Python in the context of data structures and algorithms. Understanding the fundamentals is crucial for students and aspiring programmers. As you progress in your learning journey, reflecting on what you have absorbed helps reinforce knowledge.
Summary of Concepts Learned
Throughout this exploration, several key concepts emerged:
- Data Structures: We examined fundamental structures like lists, stacks, and queues. Each serves its purpose in organizing data efficiently.
- Algorithms: Sorting and searching algorithms are foundational to programming. Knowing which algorithm to use in specific scenarios enhances problem-solving capabilities.
- Efficiency: The analysis of time and space complexity guides you in selecting the right approach for any given problem.
- Common Pitfalls: Awareness of inefficiencies and mistakes in data structure choice can significantly impact your programming performance.
- Best Practices: Writing readable code and adopting modular programming fosters better collaboration and maintainability.
By digesting these elements, you should now appreciate how Python serves both learning and practical applications in data structuring and algorithmic thinking.
Resources for Further Study
To delve deeper into these concepts, consider the following resources:
- Wikipedia provides an extensive overview of data structures and their applications.
- Britannica offers insightful articles on algorithms and their importance in computing.
- Engage with communities on Reddit to exchange knowledge and seek help from fellow learners.
- Explore Facebook groups dedicated to Python programming for networking and support.
Fostering a habit of continuous learning will serve you well. Dive into coding challenges and projects to practice applying these concepts. Learning Python is a stepping stone; use it effectively to build a solid foundation in programming.
"Learning is a treasure that will follow its owner everywhere."
As you move forward, equip yourself with curiosity and resilience. The landscape of programming is vast, and every challenge is an opportunity for growth.