Unlocking the Power of Python: A Comprehensive Guide to Basic Commands
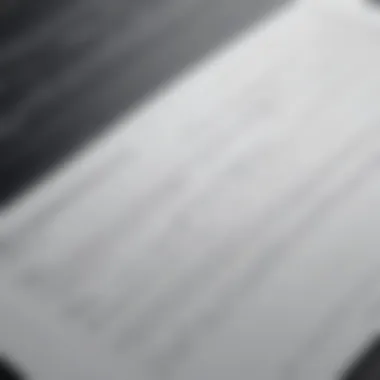
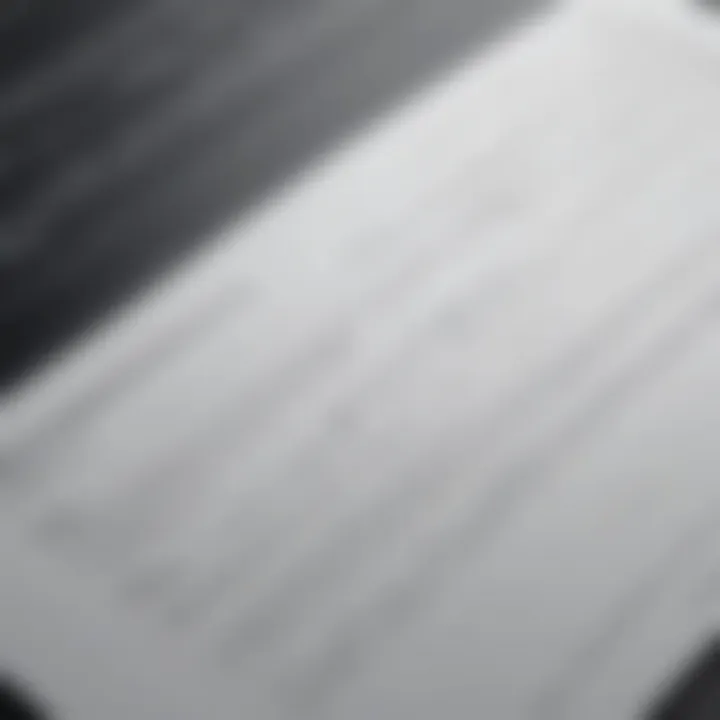
Introduction to Programming Language
Python, a versatile and high-level programming language, has gained immense popularity and adoption in various tech domains due to its readability and ease of use. Its roots can be traced back to the late 1980s when Guido van Rossum conceptualized it with a focus on code simplicity and readability over complex syntax structures.
Python offers a wide array of features and use cases, making it a top choice for tasks ranging from web development to data analysis. Its ability to support multiple programming paradigms, including procedural, object-oriented, and functional programming, further enhances its versatility and applicability in diverse projects and industries.
The language's simplicity in syntax and readability has contributed to its broader scope, attracting a vast community of developers, educators, and enthusiasts worldwide. Python's extensive standard library and compatibility with major operating systems make it an ideal choice for both beginners and seasoned programmers seeking to develop robust applications efficiently.
Basic Syntax and Concepts
When delving into Python programming, understanding key concepts such as variables, data types, operators, and expressions is essential for grasping fundamental operations. Variables serve as placeholders for storing data values, and Python supports various data types like integers, floats, strings, lists, tuples, and dictionaries to cater to different data requirements.
Utilizing operators and expressions enables programmers to manipulate values, perform arithmetic operations, and evaluate conditions within the code efficiently. Control structures, including loops and conditional statements, empower developers to alter program flow and make logical decisions based on specific conditions, enhancing code logic and functionality.
Advanced Topics
As proficiency in Python grows, exploring advanced topics like functions, methods, and object-oriented programming (OOP) becomes crucial for developing scalable and modular code. Functions encapsulate a set of statements for performing a specific task, offering code reusability and organization for complex programs.
Object-oriented programming in Python emphasizes the creation of classes and objects, promoting code encapsulation, inheritance, and polymorphism for building robust and extensible applications. Exception handling mechanisms allow developers to manage and recover from errors gracefully, ensuring program stability and enhancing user experience.
Hands-On Examples
Practical application of Python concepts through hands-on examples, simple programs, intermediate projects, and code snippets is key to reinforcing learning and honing programming skills. Writing and running simple programs help beginners grasp basic syntax and logic, while intermediate projects provide opportunities to tackle more complex challenges and enhance problem-solving abilities.
Incorporating code snippets from real-world scenarios or open-source projects enables programmers to analyze practical implementations, optimize code efficiency, and learn best practices from experienced developers. Engaging in hands-on practice cultivates a deeper understanding of Python fundamentals and equips learners with the proficiency to tackle diverse coding tasks confidently.
Resources and Further Learning
For further enrichment and continuous learning, exploring recommended books, tutorials, online courses, and joining community forums and groups dedicated to Python programming is highly beneficial. Quality resources offer in-depth insights, practical exercises, and valuable guidance for mastering Python concepts and advancing skill sets.
Online platforms such as Coursera, Udemy, and Codeacademy provide structured courses covering Python basics to advanced topics, offering interactive learning experiences and hands-on projects to consolidate knowledge. Engaging with Python communities through forums like Stack Overflow, Reddit, and Python.org enhances networking opportunities, facilitates knowledge sharing, and fosters a supportive learning environment for aspiring and experienced Python programmers.
Introduction to Python Commands
Python commands play a crucial role in the world of programming and are the foundational building blocks of Python language. Understanding the basics of Python commands is essential for beginners and intermediate learners to grasp the structure and functionality of Python. This section serves as a gateway to the realm of Python programming, shedding light on the significance and practical applications of Python commands within the context of this article.
What are Python Commands?
Overview of Python commands
Python commands encompass a vast array of functions and operations that enable programmers to execute tasks efficiently. The overview of Python commands delves into the fundamental syntax and structure of Python, highlighting its simplicity and readability. By exploring the intricacies of Python commands, readers can grasp the core principles of programming in Python, laying a robust foundation for further exploration.
Importance in programming
The importance of Python commands in programming cannot be overstated. They serve as the backbone of Python language, allowing users to perform a wide range of actions from simple arithmetic operations to complex algorithmic tasks. Understanding the significance of Python commands is vital for optimizing code efficiency and achieving desired outcomes. This section delves into the essential role that Python commands play in the programming landscape, emphasizing their practicality and versatility for developers.
Setting Up Python Environment
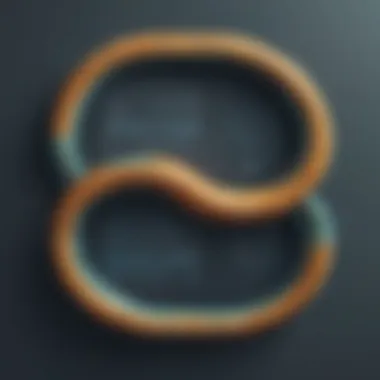
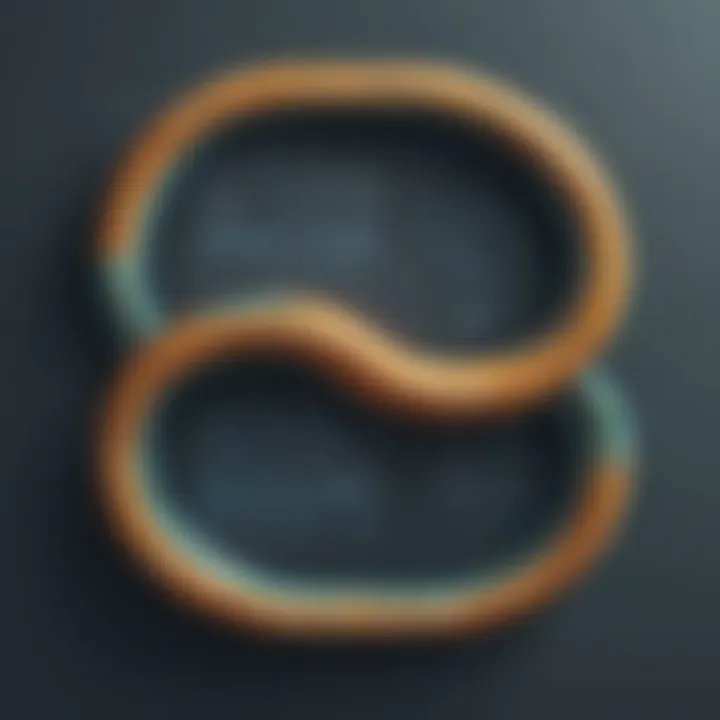
Installing Python on different platforms
Installing Python on various platforms is a critical step in setting up the programming environment. It enables users to access the Python interpreter and execute Python commands seamlessly. This section explores the installation process on different operating systems, providing step-by-step instructions and tips for a smooth installation experience.
Configuring Python interpreter
Configuring the Python interpreter is essential for customizing the programming environment to suit individual preferences and requirements. From setting environment variables to managing packages, configuring the Python interpreter allows users to enhance their coding experience. This section delves into the intricacies of configuring the Python interpreter, offering insights into optimizing the development environment for maximum efficiency.
Accessing Python Interpreter
Launching Python shell
Launching the Python shell is the initial step in accessing the Python interpreter and executing Python commands. The Python shell provides an interactive interface for testing code snippets and exploring Python functionalities in real-time. By understanding the process of launching the Python shell, users can harness the full power of Python's interactive mode for seamless code execution.
Interactive mode vs. Script mode
Differentiating between interactive mode and script mode is essential for optimizing coding workflows in Python. Interactive mode allows for immediate code execution and evaluation, making it ideal for quick prototyping and testing. In contrast, script mode enables users to save and execute Python code as scripts for larger projects. This section compares and contrasts interactive mode and script mode, highlighting their unique features and applications in Python programming.
Fundamental Python Commands
Fundamental Python commands are the building blocks of every Python program. Understanding these commands is crucial for mastering Python programming. In this article, we will delve into the core aspects of fundamental Python commands to provide readers with a solid foundation. By exploring variable declaration, printing output, and basic arithmetic operations, readers will gain a comprehensive understanding of how to manipulate data and control flow within Python programs.
Variable Declaration
Assigning values to variables
Assigning values to variables is a fundamental concept in programming, allowing developers to store and manipulate data efficiently. In Python, this process involves using the assignment operator (=) to bind a value to a variable. This fundamental operation is essential for performing calculations, saving user input, and controlling program logic. The ability to assign values to variables forms the basis of data manipulation in Python programs, making it a critical aspect of programming assignments and projects.
Data types in Python
Data types in Python define the attributes of variables and the operations that can be performed on them. Python offers a rich set of built-in data types, including integers, floating-point numbers, strings, lists, tuples, dictionaries, and more. Understanding data types is crucial for effectively handling data and ensuring the accuracy of computations in Python programs. By leveraging the correct data types, developers can optimize memory usage, enhance program performance, and write more robust and efficient code.
Printing Output
Using print() function
The print() function in Python is an essential tool for displaying output to the console. By passing arguments to the print() function, developers can showcase results, messages, and variables during program execution. This function is valuable for debugging code, providing feedback to users, and monitoring program progress. The ability to use the print() function effectively enables programmers to communicate information clearly and concisely, enhancing the readability and usability of their Python programs.
Formatting output
Formatting output in Python allows developers to control the appearance and structure of displayed information. By incorporating formatting options such as string concatenation, placeholders, and alignment specifiers, programmers can present data in a visually appealing and organized manner. Properly formatted output enhances the professional presentation of results, improves user experience, and underscores the attention to detail and quality of Python programs.
Basic Arithmetic Operations
Addition, subtraction, multiplication, division
Basic arithmetic operations, including addition, subtraction, multiplication, and division, are fundamental mathematical processes in Python. These operations enable developers to perform numerical calculations, manipulate variables, and implement algorithmic logic. By mastering basic arithmetic operations, programmers can conduct mathematical tasks, analyze data, and solve complex problems efficiently. Understanding the principles behind addition, subtraction, multiplication, and division is key to developing robust and functional Python applications.
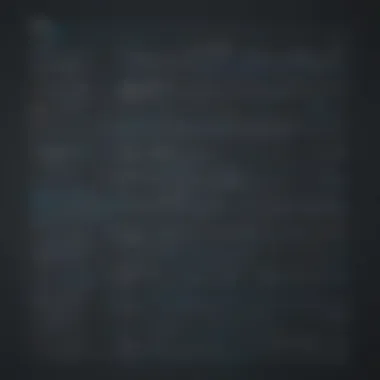
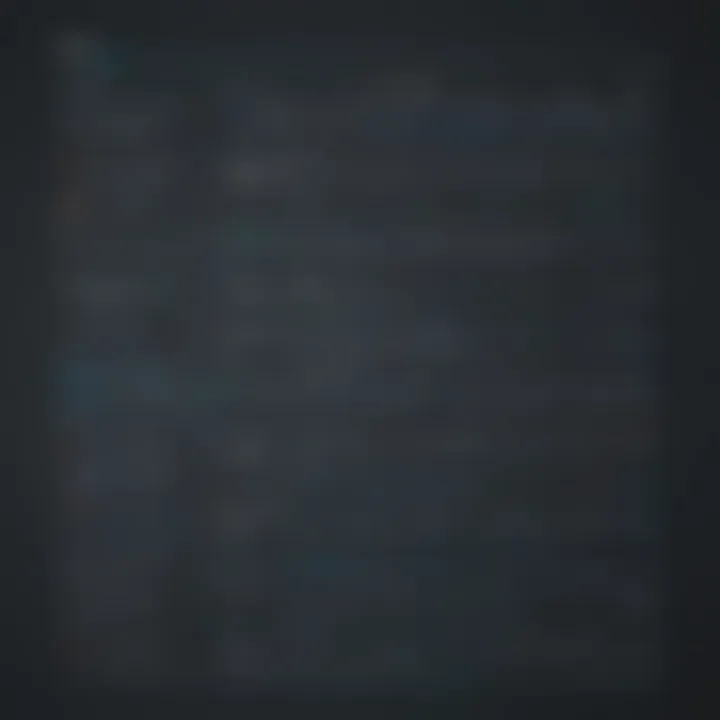
Modulus and exponentiation
In Python, the modulus (%) and exponentiation (**) operators provide additional mathematical functionalities for handling remainders and exponentiation operations. The modulus operator computes the remainder of a division calculation, while the exponentiation operator raises a value to a specified power. These operations are valuable for advanced mathematical computations, cyclic patterns, and exponent manipulations within Python programs. Integrating modulus and exponentiation operations enhances the mathematical capabilities and versatility of Python applications, allowing developers to implement sophisticated algorithms and processes effectively.
Conditional Statements and Loops
Conditional statements and loops are foundational elements in programming, especially in Python. These constructs allow for decision-making and repetition in code, enhancing the efficiency and logic of programs. Understanding conditional statements, like if-else statements, enables programmers to control the flow of their code based on specified conditions. This article delves into the significance of mastering conditional statements and loops for a comprehensive grasp of Python basics.
If-Else Statements
Syntax and Usage
If-else statements in Python provide a way to execute different blocks of code based on whether a certain condition is met. The syntax for these statements is straightforward, making them a valuable tool for implementing various decision-making scenarios in programming. Their flexible nature and clear structure make if-else statements a popular choice for handling multiple branching paths in code. Despite their simplicity, if-else statements offer a powerful mechanism for creating dynamic and responsive programs.
Nesting Conditions
Nesting conditions within if-else statements allow for greater complexity in decision structures. By nesting conditions, programmers can create intricate logic flows that cater to specific use cases. This depth of logic helps in building more sophisticated algorithms and handling intricate scenarios efficiently. While nesting conditions provide flexibility and precision in programming, overcomplicated nesting can lead to code that is challenging to debug and maintain. Striking a balance between nesting levels is crucial to ensure code readability and maintainability.
For and While Loops
Iterating through Sequences
For and while loops are essential for iterating through sequences of data in Python. These loop structures enable repetitive execution of code blocks, making them indispensable for tasks like traversing lists, strings, and other iterable objects. The ability to iterate through sequences efficiently is key to performing computations, data processing, and automation tasks effectively. Understanding how to utilize for and while loops optimally equips programmers with the ability to handle data manipulation and repetition with ease.
Control Flow in Loops
Control flow mechanisms within loops dictate how the loop operates and when it terminates. By managing control flow within loops effectively, programmers can regulate the iteration process and avoid infinite loops or premature exits. The control flow features in loops empower programmers to fine-tune the behavior of their code, ensuring precise and predictable outcomes. Mastering control flow in loops is crucial for developing robust algorithms and efficient iterative processes in Python.
Working with Functions
When delving into the realm of Python programming, one cannot overlook the pivotal role that functions play in streamlining code execution and enhancing reusability. Understanding how functions operate is crucial for any programmer, as they serve as building blocks for structuring code efficiently. Working with functions allows developers to encapsulate specific tasks, promoting modular design and code readability. In this section, we will explore the significance of functions within the context of Python programming, shedding light on their importance in simplifying complex operations and fostering code maintainability.
Defining Functions
Creating Reusable Code Blocks
A fundamental aspect of defining functions is the ability to create reusable code blocks that can be invoked multiple times within a program. This practice significantly reduces redundancy in code, promoting a more concise and organized programming approach. By encapsulating a set of instructions into a function, programmers can execute the same logic whenever necessary without duplicating code segments. The versatility offered by reusable code blocks enhances code efficiency and maintainability, making it a preferred choice for structuring programs effectively.
Function Parameters and Return Values
Another key aspect of function definition is the utilization of parameters and return values to facilitate dynamic input and output interactions. Parameters allow functions to accept external data for processing, enhancing flexibility and customization within function calls. Return values enable functions to produce outcomes or results based on the provided inputs, enabling seamless data flow within the program. The ability to define function behaviors using parameters and return values empowers developers to create versatile and adaptable functions suited to diverse programming requirements. While these features enhance the functionality of functions, careful consideration is required to optimize their usage for specific programming tasks.
Built-in Functions
Built-in functions in Python serve as a repository of pre-defined functionalities that expedite common programming tasks and enhance overall development efficiency. These functions encompass a wide range of operations, from mathematical calculations to string manipulations, catering to various programming needs. By leveraging built-in functions, programmers can expedite development cycles and focus on core logic implementation rather than redefining standard operations. In this section, we will delve into the significance of commonly used functions and the process of importing modules in Python programming.
Commonly Used Functions
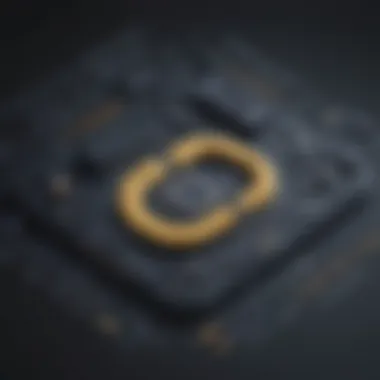
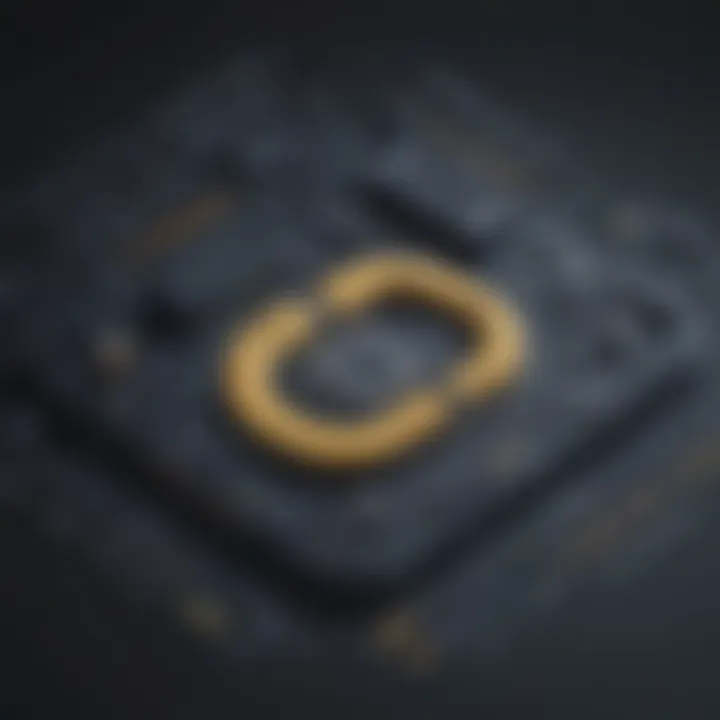
Commonly used functions in Python encapsulate essential operations that are frequently employed in programming tasks. These functions offer a standardized approach to executing routine tasks, promoting code consistency and simplicity. By incorporating commonly used functions in code frameworks, developers can expedite development processes and improve overall code maintainability. The versatility and reliability of these functions make them a preferred choice for handling repetitive tasks efficiently within Python programs.
Importing Modules
The process of importing modules in Python facilitates the integration of external functionalities and libraries into a program. Modules encapsulate reusable code components, enabling developers to extend the capabilities of their programs seamlessly. By importing modules, programmers can leverage existing libraries and utilities to enhance program functionality without reinventing the wheel. This modular approach promotes code reusability and fosters collaboration within the Python community by encouraging the sharing of code resources. While importing modules enriches program functionalities, it is essential to maintain a strategic approach to module management to optimize program performance and maintain code integrity.
File Handling in Python
File handling plays a crucial role in programming, particularly in Python. It allows developers to read from and write to external files, enabling data manipulation and storage. Understanding file handling is essential for programmers as it facilitates interaction with persistent data, creating practical applications. Python simplifies file operations, making it user-friendly for beginners and versatile for complex projects.
Reading and Writing Files
Opening and closing files
Opening and closing files is a fundamental aspect of file handling in Python. It involves establishing a connection to a file for reading or writing operations and closing it to release system resources. This process ensures data integrity and prevents memory leaks. The ability to open and close files seamlessly is critical for efficient file management in Python.
Read, write, append operations
Read, write, and append operations are core functionalities in Python file handling. Reading allows fetching data from a file, writing enables adding new information, while appending appends content to an existing file without overwriting. These operations provide flexibility in handling different file types and sizes. Understanding read, write, and append operations is essential for managing data effectively in Python.
Working with File Paths
Manipulating file paths
Manipulating file paths involves changing, extracting, or manipulating the location of files within the system. Python offers robust libraries for file path manipulation, aiding in tasks like locating files, organizing data, or accessing resources across directories. Proper file path handling enhances program portability and accessibility, streamlining file operations in Python.
File handling best practices
Implementing file handling best practices is crucial for efficient and secure data management in Python. Techniques like using context managers for file operations, validating input data, and handling exceptions gracefully enhance code reliability. Adhering to best practices ensures code maintainability, reduces errors, and promotes better collaboration in Python projects.
Error Handling and Debugging
Error handling and debugging are crucial aspects in programming, including Python. Efficient error handling ensures code reliability and enables developers to identify and rectify issues quickly. In this article, we will delve into the significance of error handling and debugging, emphasizing their role in maintaining code quality and enhancing overall program functionality.
Exceptions Handling
Try-except Blocks:
Try-except blocks are fundamental in managing exceptions within Python programs. By enclosing risky code within a try block and specifying fallback actions within an except block, developers can gracefully handle errors without terminating the program abruptly. The key characteristic of try-except blocks lies in their ability to facilitate controlled execution paths based on unforeseen conditions, making them a favored choice for this article. One of the unique features of try-except blocks is their robustness in preventing application crashes by providing alternate routes in scenarios of unexpected errors, highlighting their importance in maintaining program stability.
Raising Custom Exceptions:
Custom exceptions offer developers the flexibility to create specialized error classes tailored to specific program requirements. By raising custom exceptions at distinct program junctures, developers can convey detailed error information that aids in pinpointing issues swiftly. The key characteristic of raising custom exceptions is the precision they offer in error signaling, making them a valuable choice for this article. Moreover, the unique feature of custom exceptions lies in their capacity to enhance code readability and maintainability by defining explicit error scenarios, thereby streamlining the debugging process and contributing to overall program robustness.
Debugging Techniques
Using Print Statements:
Print statements serve as a fundamental tool in debugging Python code, allowing developers to display variable values and intermediate outputs for error investigation. The key characteristic of using print statements is their simplicity and versatility in providing real-time insights into program state, making them a popular choice for this article. A unique feature of print statements is their immediate feedback mechanism, enabling programmers to identify logic flaws and variable values efficiently, thereby expediting the debugging process and enhancing code clarity.
Debugging Tools in Python:
Python offers a suite of robust debugging tools that aid developers in identifying and resolving issues effectively. From integrated development environments (IDEs) to specialized debugging libraries, Python's debugging ecosystem caters to diverse debugging needs. The key characteristic of debugging tools in Python is their comprehensive feature set, encompassing breakpoints, watchpoints, and stack traces, rendering them a beneficial choice for this article. The unique feature of debugging tools lies in their ability to streamline error diagnosis by providing interactive debugging capabilities, empowering developers to isolate and rectify issues promptly, thereby improving code efficiency and reliability.