Innovative Programming Projects for Beginners
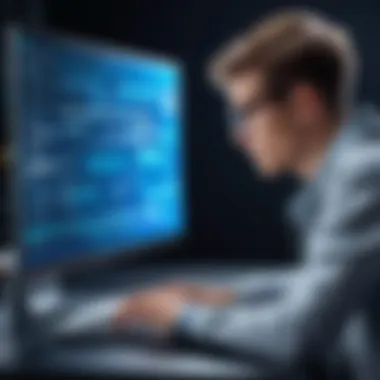
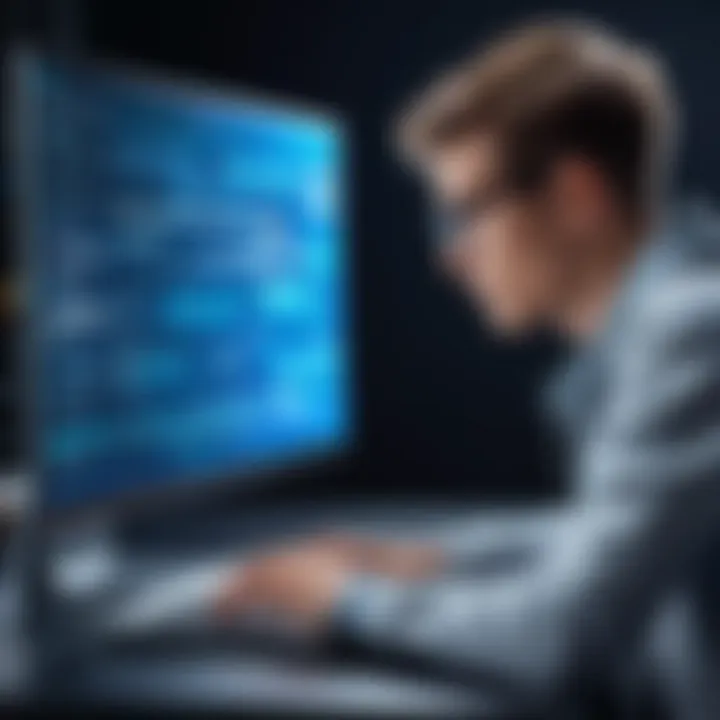
Foreword to Programming Language
Programming language serves as the backbone for developing software, enhancing digital experiences and automating tasks. Understanding its essence is crucial for novices as they embark on their coding journey. This section explores the historical background, core features, and the current landscape of programming languages.
History and Background
The evolution of programming languages began in the mid-20th century. Early languages like Fortran and Lisp laid the foundation by introducing abstract concepts for instructing machines. As demands grew, new languages emerged, evolving into lower-level assembly languages and higher-level languages aimed at enhancing productivity. This progression established a diverse array of languages, each catering to specific needs.
Features and Uses
Programming languages vary in features, catering to different aspects of software development. Some key features include:
- Syntax: Rules governing how code is written.
- Semantics: Meaning conveyed by the written code.
- Paradigms: Approaches to programming, such as procedural or declarative.
These features influence how languages are utilized. For instance, Python is known for its readability and ease of use, making it a popular choice for beginners, while C++ offers deeper control over system resources, making it suitable for performance-intensive applications.
Popularity and Scope
The popularity of programming languages fluctuates with trends and market demands. According to various surveys, languages like Python, JavaScript, and Java often rank highly among developers. Understanding this scope is vital for beginners. Choosing a language that aligns with their goals and the current job market can accelerate their learning and career prospects.
Basic Syntax and Concepts
For effective programming, understanding basic syntax and concepts is essential. Beginners should focus on foundational elements that build into complex programs.
Variables and Data Types
Variables serve as containers for storing data. Each variable has a data type, which defines the kind of data it can hold. Common data types include integers, floats, strings, and booleans. For example:
Operators and Expressions
Operators allow performing operations on variables and values. They include arithmetic, comparison, and logical operators. For instance, the expression evaluates to . Understanding how to manipulate data using these operators is integral for beginners.
Control Structures
Control structures dictate the flow of execution in a program. Basic structures include if-statements, loops, and switches. For example, an if-statement checks a condition before executing a block of code, guiding the program's logic.
Advanced Topics
Once the basics are grasped, advancing to more complex concepts can deepen understanding and versatility in programming.
Functions and Methods
Functions are reusable blocks of code designed to perform a specific task. They enhance code organization and readability. For instance, a function to add two numbers can be structured as:
Object-Oriented Programming
Object-Oriented Programming (OOP) introduces the concept of objects that encapsulate data and behaviors. It promotes code reuse and flexibility by allowing the creation of classes and instances.
Exception Handling
Managing errors is paramount in programming. Exception handling techniques allow developers to respond to unexpected situations gracefully, preventing crashes and providing user-friendly error messages.
Hands-On Examples
Practical experience reinforces theoretical knowledge. Engaging in simple projects helps beginners apply what they learn.
Simple Programs
Starting with uncomplicated programs, such as a calculator or a number guessing game, can build confidence. These projects teach problem-solving while making learning enjoyable.
Intermediate Projects
As skills improve, tackling intermediate projects like a personal to-do list app or a basic web page enhances understanding of application development.
Code Snippets
Sharing code snippets in community forums can provide guidance and inspire creativity. Platforms like Reddit offer a space for coders to discuss ideas and collaborate on projects.
Resources and Further Learning
A wealth of resources exist for those keen to learn more. These can support their development journey.
Recommended Books and Tutorials
Books such as "Automate the Boring Stuff with Python" and "Eloquent JavaScript" offer practical insights and exercises.
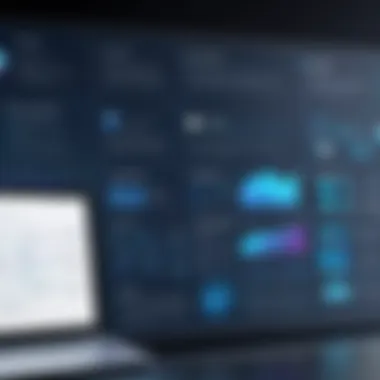
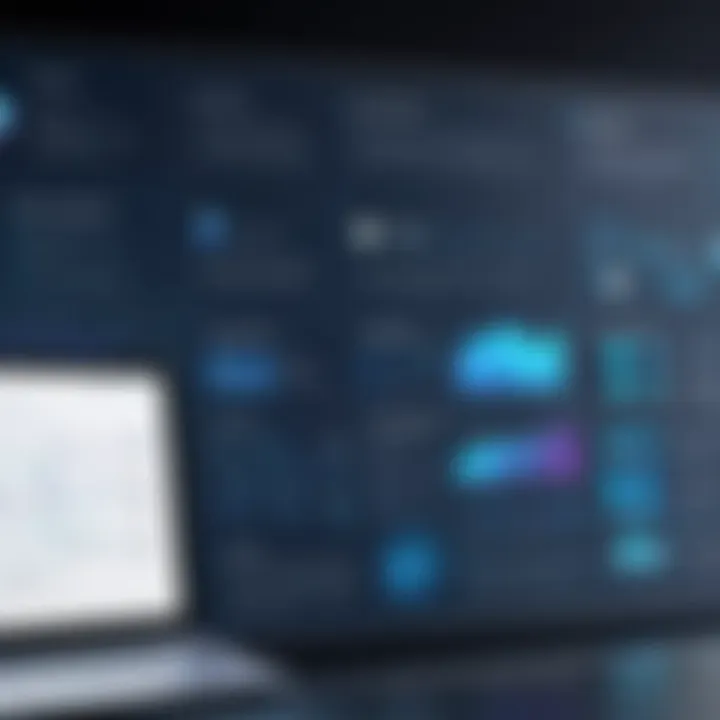
Online Courses and Platforms
Platforms like Codecademy, Coursera, and edX provide structured learning paths. They often feature interactive sessions that cater to different learning styles.
Community Forums and Groups
Joining forums like Stack Overflow and subreddits dedicated to specific languages provides support. Engaging with a community fosters growth and opens doors to collaboration.
Programming is not just about writing code; it's about solving problems and thinking critically.
Foreword to Programming Projects
Programming projects are crucial for anyone starting their journey in coding. They serve as practical examples of how programming concepts come together in real-world applications. By engaging in projects, beginners not only learn syntax and rules of a programming language but also develop logical reasoning, problem-solving skills, and creativity. This hands-on experience helps solidify theory and transforms abstract knowledge into practical skills.
Importance of Project-Based Learning
Project-based learning is an effective method for grasping programming essentials and pushing beyond theoretical knowledge. It captures the active engagement of learners with the material. Some key points regarding the value of undertaking projects include:
- Real-World Application: Projects demonstrate how programming can resolve actual problems. This relevance motivates learners to progress.
- Skill Assessment: Engaging in projects allows beginners to assess their skills. It encourages them to confront challenges head-on and find solutions independently.
- Portfolio Creation: Each completed project can become part of a personal portfolio, showcasing the learner's skills to potential employers or educational institutions.
"Learning to code is not just about mastering specific languages but also about understanding how to think like a programmer."
How Projects Enhance Understanding
Projects are an effective way to deepen understanding. They enable learners to explore new concepts at their own pace. Some aspects of how projects promote understanding include:
- Hands-On Experimentation: Through projects, learners can experiment freely. They can modify code, fix bugs, and see how their changes affect program behavior.
- Interconnection of Concepts: Completing a project requires a combination of different programming skills. It helps learners see the relationships between various concepts and how they work in harmony.
- Critical Thinking Development: Working on projects necessitates critical thinking. It demands planning, execution, and troubleshooting, all of which sharpen the learner's analytical abilities.
As beginners embark on their programming journey, focusing on project-based learning can create a more enriching educational experience. This approach helps in transitioning from theoretical understanding to practical expertise.
Foundational Ideas for Beginners
Foundational ideas are crucial for anyone starting their journey in programming. These projects often serve as the cornerstone for understanding more complex concepts later. They offer a fundamental grasp of programming logic, syntax, and problem-solving techniques. Engaging with simple projects does not only build technical skills but also fosters a positive mindset towards programming. Beginners can see immediate results, increasing their motivation to dive deeper into the field.
Understanding the basic concepts in programming is vital. It helps in mitigating discouragement that often arises when facing challenges. Foundational projects lay the groundwork for advanced topics, like algorithms and data structures. This preparation is essential for future learning, making transitions to more complex tasks smoother.
Simple Calculator Application
Creating a simple calculator application is a practical first project for beginners. This project introduces users to fundamental programming concepts such as variables, conditionals, and functions. The process involves designing a user interface and implementing arithmetic operations like addition, subtraction, multiplication, and division.
To get started, one can use languages like Python or JavaScript as they offer straightforward syntax. For example, in Python, one can define functions for each of the operations. The user interface could be a command line or a simple web page, depending on the desired complexity.
Example Code Snippet
This project not only solidifies one’s understanding of basic operations but also serves as a gateway to exploring user input and error handling.
To-Do List and Task Manager
A to-do list application is another excellent project idea for beginners. It combines front-end and back-end components, providing insight into how applications work. Users will learn about creating, reading, updating, and deleting tasks, commonly referred to as CRUD operations.
This project can utilize various technologies such as HTML, CSS, and JavaScript for front end, while using storage options like local storage or a simple database for the back end. Furthermore, implementing features like due dates and task categories enhances the project’s complexity and utility.
Working on a to-do list application encourages beginners to think about user experience and interface design. This is not just about programming but also about constructing applications that are user-friendly and efficient.
Basic Countdown Timer
Building a basic countdown timer allows beginners to apply their knowledge in a more dynamic way. This project introduces concepts such as timing functions and user interfaces. Users can start, pause and reset the timer, which involves managing states and understanding asynchronous programming.
This project can be built using JavaScript within a web environment, allowing for real-time updates on the timer displayed to the user. It also teaches time management in programming, adding a layer of practicality to the learned concepts.
In summary, foundational ideas are not merely simple tasks. They allow learners to explore crucial programming skills that are essential for further explorations in the field. Practical projects such as a calculator, a to-do list, or a countdown timer enrich the programmer's toolkit and foster a deep understanding of programming fundamentals.
Interactive Applications
Interactive applications hold significant value in beginner programming projects. They not only engage users but also facilitate hands-on learning experiences. By implementing interactive applications, beginners learn to apply programming concepts in real-world scenarios. This means they practice coding, debug issues, and understand user experience designs. Furthermore, they learn about event-driven programming and how to manage user input effectively.
Creating interactive applications encourages creativity and problem-solving skills. It challenges students to think critically as they consider how users will interact with their projects. Moreover, these projects can be engaging. Students often find themselves more motivated when working on applications that have immediate users. Consequently, interactive applications present an excellent starting point for those new to programming.
Text-Based Adventure Game
A text-based adventure game is a compelling project for beginners. This type of game requires players to make choices that affect the story, presenting a unique way to learn about programming logic and structure.
To create such a game, beginners start by understanding the fundamental concepts of programming, like variables, data types, and control structures. They design a storyline and implement various pathways based on player decisions. This entails using conditional statements to determine outcomes based on the choices the player makes.
A basic structure of a text-based adventure game might involve:
- Setting up the narrative. This can include defining settings and characters.
- Creating decision points. Each decision the player makes leads to different branches in the story.
- Implementing a simple command parser. This allows the game to understand player inputs, such as “go north” or “take key.”
The simplicity of this project makes it ideal for newcomers. It involves basic programming skills while allowing room for creativity. Additionally, beginners gain experience with user input, which is a critical aspect of software development.
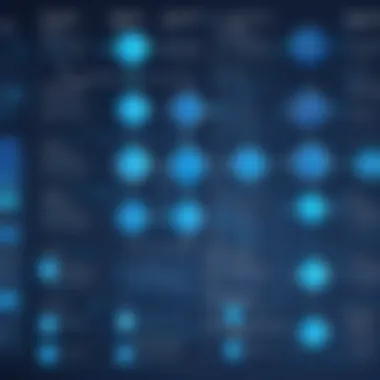
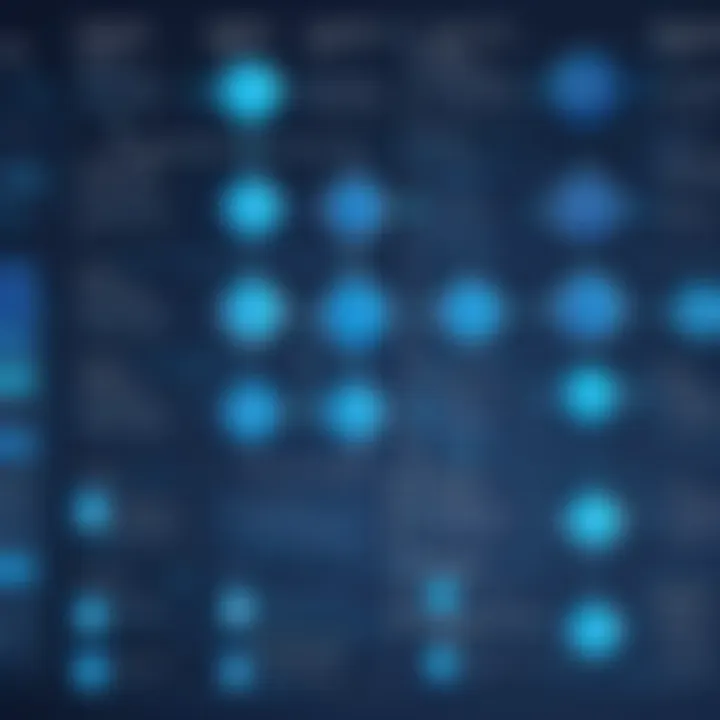
Quiz Application
Developing a quiz application is another excellent option for beginners. It combines various programming principles, such as user interfaces, data handling, and condition checks. Creating a quiz can help learners grasp essential concepts while building something functional.
To create a quiz application, beginners can follow a structured approach:
- Define the questions and answers. This can be hardcoded or sourced from an external file.
- Build the user interface. This can be done using simple HTML and CSS for web-based applications, or command line inputs for console applications.
- Handle user input. Beginners can learn to capture user answers and evaluate them against the correct answers.
- Provide feedback. After users complete the quiz, the application can display scores and correct answers, reinforcing learning.
A quiz application also provides an excellent opportunity to learn about arrays or other data structures for managing questions and answers. With time, one can add features like timers or score tracking that can enhance the application further.
In summary, both the text-based adventure game and the quiz application serve as valuable projects for beginners. They not only reinforce programming fundamentals but also cultivate a deeper understanding of how to create interactive and user-centric applications.
Web Development Projects
In the realm of programming, web development serves as a gateway. It allows beginners to understand the core concepts of how applications operate on the internet. Engaging in web development projects can enhance both practical skills and theoretical knowledge. The process involves learning HTML, CSS, and JavaScript, among other technologies. These foundational skills cultivate a sense of accomplishment and confidence in fledgling programmers.
Web development projects have several benefits:
- Hands-On Learning: The best way to learn web development is by doing it. Creating real projects cements knowledge.
- Portfolio Building: Completed projects can showcase abilities to potential employers or clients.
- Problem-Solving: Each project presents unique challenges that foster analytical skills and creative solutions.
- Responsive Design: Learning to build responsive sites prepares students for modern web standards.
Individuality in projects can also be important. Each student brings their perspective, leading to varied outcomes even when following the same guidelines. This diversity enriches learning experiences and helps develop unique coding styles.
Personal Portfolio Website
A personal portfolio website is a vital project for any aspiring web developer. It serves multiple purposes, primarily showcasing skills and completed work. Visitors to the site can view examples of your abilities and understanding.
Creating a personal portfolio involves:
- Content Development: Select which projects to highlight. Include descriptions for context.
- Design Choices: A visually pleasing layout can reflect personal style. It's essential to balance aesthetics and function.
- Technical Implementation: Use HTML, CSS, and perhaps JavaScript to create interactive elements.
Once finished, this project extends beyond learning. It acts as a digital resume. Sharing it through platforms like LinkedIn or other social media can broaden networking opportunities.
Blog Platform
Building a simple blog platform is a beneficial learning experience for beginners. This project exposes students to dynamic web applications where users can interact by creating, reading, updating, and deleting posts.
Key elements of a blog platform include:
- User Authentication: Implementing sign-up and login functionalities encourages understanding of security.
- Content Management: Learning to manage data effectively is crucial. Tools like databases can assist in this area.
- User Interface (UI): Ensuring an intuitive design improves user experience. It may include posting templates, comment sections, and more.
This project not only consolidates knowledge but also highlights the importance of user engagement.
Basic E-commerce Site
Creating a basic e-commerce site introduces students to the commercial aspects of web development. Such sites demand specific features that enhance understanding of both front-end and back-end functionalities.
Key components of a basic e-commerce site are:
- Product Listings: This involves displaying items for sale, including images, descriptions, and prices.
- Shopping Cart Functionality: Understanding state management is vital. Developers must manage how items are added or removed from the cart.
- Payment Integration: While a simplified version is acceptable, learning about payment gateways is beneficial for real-world applications.
Building an e-commerce platform provides an excellent insight into how businesses operate online, making it a valuable addition to a beginner's portfolio.
Data Handling Projects
Data handling projects play a significant role in programming education. They introduce learners to basic concepts of data management, which is a critical skill in software development. Working with data allows beginners to understand how to collect, store, and manipulate information effectively. These projects showcase the practical applications of coding skills while emphasizing logical reasoning and analytical thinking.
When working on data-related tasks, programmers must consider data structures, data types, and basic algorithms. These concepts are fundamental as they enhance the programmer's ability to manage and transform data efficiently. Moreover, data handling projects often involve file manipulation, which is an essential skill for various programming contexts. Understanding how to read from and write to files lays the groundwork for more advanced applications involving databases and web APIs.
Benefits of engaging in data handling projects include:
- Improved Problem-Solving Skills: Tackling data-related tasks requires identifying patterns and creating efficient solutions.
- Hands-On Experience: Practical exposure helps reinforce theoretical knowledge gained from learning programming languages.
- Versatility: Data handling is relevant in various fields like finance, healthcare, and technology, increasing a beginner's job prospects.
"Mastering the handling of data is crucial in today’s data-driven world."
CSV File Analyzer
The CSV file analyzer project is a great starting point for beginners. CSV stands for Comma-Separated Values, a simple file format widely used to store tabular data. This project teaches learners how to read, parse, and analyze data from these files, providing valuable insights into data manipulation.
To begin, the programmer must decide the functionalities this analyzer will have. Typically, basic functions could include:
- Reading a CSV file: The program should be able to accept a path to a CSV file and load its contents.
- Data Summary: Analyzing the data for key statistics, such as counts, means, or sums, depending on the content.
- Filtering Data: Implementing a feature to filter specific entries based on user-defined criteria.
- Data Export: Optionally, allowing users to export analyzed data in another CSV format.
Here’s a simplified example of how the code may look to read a CSV file:
This project not only reinforces core programming skills but also introduces important concepts about data storage and retrieval, which are applicable in many programming scenarios.
Simple Expense Tracker
Building a simple expense tracker is another insightful project for beginners. This application can help individuals monitor their spending habits and manage their finances. It can be designed to record income and expenses, categorize transactions, and generate simple reports based on this data.
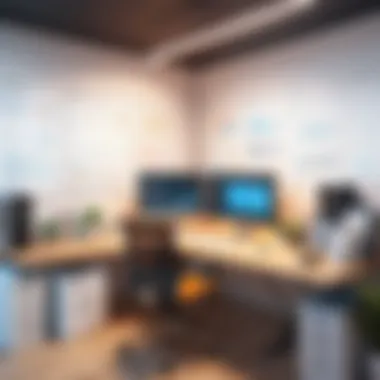
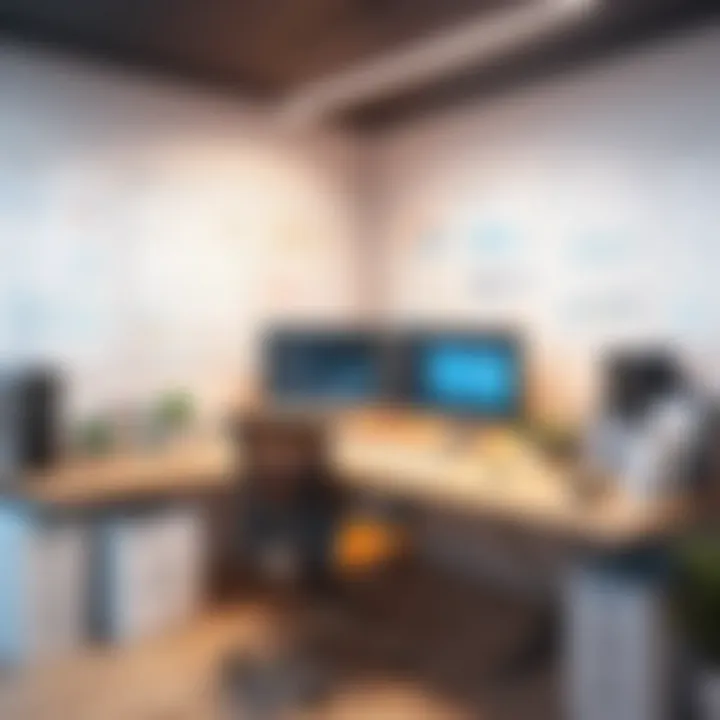
The key features of an expense tracker might include:
- Adding transactions: A function to input expenses with details such as amount, category, and date.
- Viewing transactions: A display function that presents a list of all recorded transactions in an easy-to-read format.
- Basic calculations: The ability to calculate total expenditure over a specific time frame and perhaps even budget comparisons.
- Data visualization: Displaying the spending habits visually through graphs could enhance user understanding and engagement.
By working on this project, beginners can learn how to manage user inputs and outputs effectively, which is critical in many software applications. This project also introduces users to important principles of software design, such as modularity and maintainability. By keeping functions clear and focused, they can simplify future updates and enhancements.
Specialized Programming Concepts
Specialized programming concepts are critical in the realm of programming as they introduce learners to more advanced topics and practical tools that are applicable in real-world scenarios. Learning about these concepts can enhance a beginner's skill set considerably. This section delves into two specialized areas: APIs and gaming. Both topics not only enrich a programmer’s toolkit but also build a deeper understanding of how programming interacts with the broader tech landscape.
The integration of APIs helps users to effectively communicate between different software systems. APIs, or Application Programming Interfaces, serve as a bridge that allows various applications to access and interact with each other. This makes it easier to enhance functionality without having to build everything from scratch. Similarly, creating simple games provides an engaging way to grasp programming logic, event handling, and other essential concepts. These areas encourage learners to experiment and think critically, which are essential skills in programming.
Prolusion to APIs with Weather Application
Working with APIs can initially seem daunting, yet it is an invaluable skill. Developing a weather application serves as a suitable project for beginners to learn how to fetch and display data from a public API. Such a project typically utilizes an API that offers weather information, allowing users to retrieve data based on location.
To start, learners need to:
- Select a public API: A good example is the OpenWeatherMap API, which provides weather data for various locations.
- Understand API requests: Familiarization with GET requests is vital, as this is how data is retrieved from the API.
- Handle responses: After sending a request, a response containing weather data is received in formats like JSON or XML.
To implement this, here is a simple skeleton code snippet to illustrate:
This snippet demonstrates how to create a function to get weather data for a specified city. Building upon this, learners can then focus on displaying this information in a user-friendly manner, practicing not only their programming skills but also developing an understanding of real-world applications of API knowledge.
Creating Simple Games
Creating simple games allows beginners to delve into programming concepts while keeping engagement levels high. Games often incorporate various programming principles like logic, control flows, and problem-solving. Moreover, they provide immediate feedback, which can be encouraging for new programmers.
One simple game idea is a text-based number guessing game. Here, the program generates a random number, and the player must guess the number within a limited number of attempts. The core programming tasks include:
- Generating a random number: This involves using libraries suited for random number generation, based on the language being used.
- Reading user input: Learners will practice getting input from players and processing that input accordingly.
- Implementing game logic: The game must provide feedback to the user, whether the guess is too high, too low, or correct.
By executing such projects, beginners can experience an invigorating mix of fun and learning that solidifies their understanding of basic concepts while honing their coding abilities.
"Success is not the key to happiness. Happiness is the key to success. If you love what you are doing, you will be successful."
Encouraging Good Practices
Encouraging good practices in programming is vital for anyone starting their coding journey. These practices lay down the foundation for sustainable development, efficient collaboration, and personal skill growth. As beginners engage in projects, understanding the nuances of these practices can help them avoid common pitfalls and enhance their productivity. In this section, we will focus on two essential areas: version control and code documentation. Both topics are fundamental in establishing not only technical competence but also a professional mindset.
Embracing Version Control
Version control is the backbone of modern software development. It allows developers to manage changes to their codebase efficiently. For beginners, mastering version control early on offers several benefits:
- Tracking Changes: You can track every modification in your code. This feature is essential when experimenting with new ideas or fixing bugs, as it provides a safety net.
- Collaboration: Most projects involve teamwork. Version control systems like Git facilitate collaboration by allowing multiple developers to work on the same codebase without overwriting each other's changes.
- Branching and Merging: You can create separate branches for different features or experiments. Once tested, changes can be merged back to the main branch, allowing for organized development.
For those starting, using platforms like GitHub can be incredibly beneficial. GitHub offers a user-friendly interface for managing repositories and provides a wealth of resources and community support. Understanding basic Git commands such as , , , and will create a strong foundation in version control.
"Using version control not only protects your code but also enhances your focus on coding rather than administration."
Code Documentation Essentials
Documentation is often seen as tedious, but effective code documentation is key to both personal understanding and teamwork. As a beginner, documenting your code can significantly improve your learning experience. Here are important aspects of code documentation:
- Clarity: Writing clear comments and documentation helps in recalling your thought process when you revisit the code later. This practice also aids others looking at your code, making it easier for them to understand your work.
- Guidance: Proper documentation serves as a guide for future modifications. When adding new features or debugging, well-documented code provides context, which is invaluable during the development process.
- Standards: Establishing a habit of writing documentation sets a standard for professional growth. Many organizations expect clear documentation as part of their coding standards.
For beginners, using tools like JSDoc for JavaScript or Sphinx for Python can streamline the documentation process. These tools allow you to generate documentation automatically from comments in your code, turning your explanations into structured documents.
Next Steps in Programming Development
This section of the article emphasizes the significance of advancing in programming after grasping the basic concepts through projects. As one transitions from beginner to intermediate programming, it becomes critical to adopt new challenges. Intermediate projects can showcase skills acquired and push the boundaries of one’s coding capacity.
When beginners complete initial projects, they often gain a sense of accomplishment. However, staying stagnant does not foster growth. Engaging with more complex concepts and frameworks is crucial. This is where the importance of intermediate projects comes in.
Transitioning to Intermediate Projects
Stepping into intermediate projects allows learners to refine their coding techniques. These projects often incorporate features such as user authentication and data manipulation. Working on these aspects enhances problem-solving abilities.
Some examples include:
- Web Applications: Building a full-stack application can help understand client-server architecture.
- Mobile Apps: Developing simple apps can introduce mobile development frameworks and practices.
- Data Science Projects: Exploring data visualization or machine learning can be beneficial in honing analytical skills.
By undertaking these projects, one will encounter more complex logic and require understanding of advanced programming concepts. Transitioning to intermediate projects is about resilience and eagerness to learn.
Continuous Learning and Resources
The learning process in programming is never complete. Continuous education is vital. Engaging with resources such as online courses, tutorials, and communities can enhance understanding. They provide updated knowledge on emerging technologies and best practices.
Useful resources include:
- Online Learning Platforms: Websites like Coursera and edX offer specialized courses.
- Programming Communities: Sites like Reddit provide forums where one can ask questions and share experiences.
- Documentation: Official documentation from languages and frameworks contains invaluable information.
Moreover, engaging with coding challenges on platforms like LeetCode can sharpen problem-solving skills while fostering a competitive spirit.
"Real learning comes from trying out ideas, making mistakes, and growing from the experience."