Programming in Assembly: An In-Depth Exploration
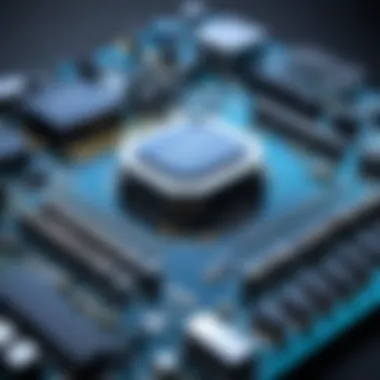
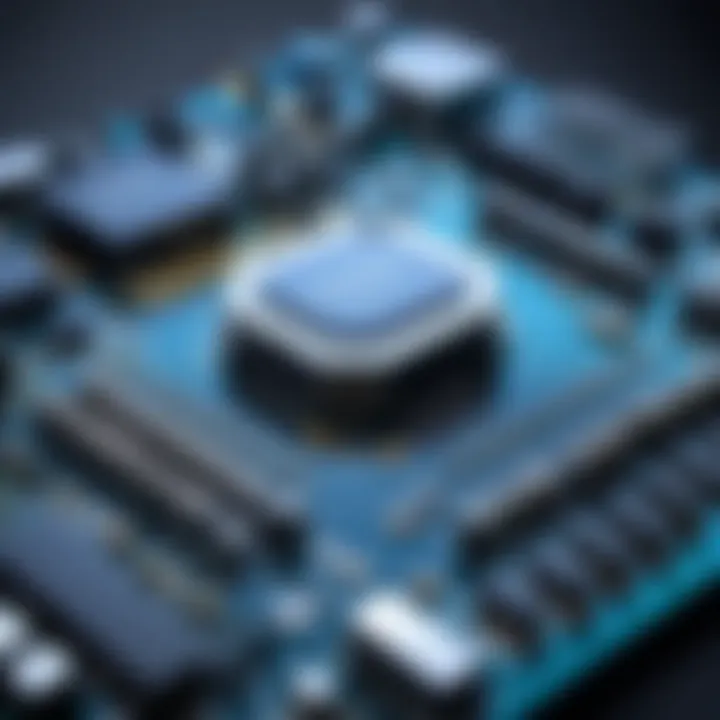
Intro
The study of programming languages is a crucial area in computer science. Assembly language stands out as a fundamental technology that bridges the gap between hardware and higher-level programming languages. Its design makes it a low-level language, which offers fine control over computer hardware. Understanding assembly is vital for grasping how computers execute instructions.
History and Background
Assembly language has roots in the early stages of computer programming, evolving in parallel with the development of computer architecture. It serves as a mnemonic representation of machine code. The advent of this language was necessary, as primitive machine code inputs posed significant challenges for humans to comprehend. By introducing symbolic representations, assembly language simplified coding for programmers.
Features and Uses
Assembly language is characterized by its low-level nature, allowing for direct manipulation of hardware. The main features include:
- Portability: While less portable than high-level languages, assembly code can run on various computer architectures with some adjustments.
- Efficiency: Assembly allows for efficient use of system resources, providing control over memory and processor instructions.
- Access to Hardware: Direct communication with hardware components is possible, making it essential for system level programming.
These features make it particularly useful in systems programming, embedded systems, and performance-critical applications.
Popularity and Scope
While assembly is not as popular as high-level languages, it retains significance in specific domains. Areas like operating systems, firmware, and real-time systems prominently feature assembly language programming. Its role remains indispensable, especially where performance optimization and hardware-level programming are necessary.
In the upcoming sections, we will explore basic syntax, advanced topics, practical examples, and resources that will aid learners in mastering assembly language programming.
Prelude to Assembly Language
Assembly language is a crucial component of computer science education and systems programming. Understanding assembly language provides insight into how software interacts with hardware at a fundamental level. Such knowledge is essential for optimizing performance, debugging complex applications, and developing low-level system software. Learning assembly language is not only about writing code but also about grasping the core principles of how computers function. It is an avenue for programmers to refine their skills and contribute to the development of sophisticated technology.
Definition and Purpose
Assembly language serves as a bridge between high-level programming languages and machine code, which is the language understood directly by the computer's CPU. Unlike high-level languages like Python or Java, assembly language is specific to a particular computer architecture. Each processor family has its unique assembly language, making it necessary for programmers to understand the architecture they are working with.
The primary purpose of assembly language is efficiency. Writing programs in assembly language allows developers to have precise control over hardware and system resources. This is particularly important in scenarios where performance is critical, such as in embedded systems or real-time applications. The use of assembly can lead to significant performance improvements compared to higher-level languages, though at the cost of increased complexity and reduced portability.
History of Assembly Language
The concept of assembly language dates back to the early days of computing in the 1940s and 1950s. Initially, programmers wrote programs in binary machine code, which was tedious and error-prone. To alleviate this issue, assembly language was introduced, allowing programmers to use symbolic representations for operations and memory locations. This made coding more approachable and less error-prone.
As computers evolved, so did assembly languages. Variations emerged corresponding to different architectures, such as x86 and ARM, adapting to the technological advancements of the time. Over the decades, as high-level languages gained popularity due to their ease of use, assembly language retained its importance in specific domains like systems programming and embedded systems development. Understanding its history is essential as it reveals the evolution of programming practices and the enduring relevance of low-level programming today.
Understanding Computer Architecture
Understanding computer architecture is a fundamental aspect when learning assembly language. It is essential because assembly language operates very close to the hardware. By grasping computer architecture, learners will see how assembly language interacts with the underlying physical components. This interaction is where the efficiency of a program is determined. Knowing the architecture surely enhances the programmer's skills in optimizing code and resolving problems effectively.
This section will focus on two critical areas. These are the role of assembly language in system architecture and the components of a computer system. Both are crucial for a deep understanding of programming at the assembly level. The insights gained here will aid in writing better assembly code and develop a clearer sense of how software commands translate into hardware actions.
The Role of Assembly in System Architecture
Assembly language plays a vital role in system architecture. It serves as a middle ground between high-level programming languages and machine code. When a programmer writes in assembly language, they have a more straightforward representation of the actual instructions executed by the computer. Each assembly instruction corresponds to a specific machine language instruction.
Using assembly language gives programmers direct control over the hardware. This level of control is particularly useful in operations requiring high performance, such as in embedded systems or real-time applications. A better understanding of this role enables programmers to leverage hardware capabilities fully.
Key benefits of knowing assembly language in this context include:
- Easy mapping from logical operations to hardware execution.
- The ability to optimize performance by working directly with memory and CPU registers.
- Enhanced debugging skills, as programmers can better trace errors at the instruction level.
Assembly language acts as a gateway for programmers to harness the full potential of the computer's architecture.
Components of a Computer System
A computer system comprises several interconnected components that work together to perform computations. Each component serves a unique function that is critical for overall system operation. Understanding these components is essential for anyone delving into assembly programming.
The major components include:
- Central Processing Unit (CPU): Executes instructions from programs. It interprets and processes data. A strong grasp of CPU architecture can impact how efficiently assembly programs run.
- Memory: Where data and instructions are stored temporarily. Concepts such as RAM, ROM, and cache memory are crucial in understanding how program data is accessed quickly.
- Input/Output (I/O) Devices: Facilitate interaction between the user and the computer. Knowing how to manage I/O operations in assembly can enhance program efficiency profoundly.
- Storage: Long-term data storage provides the capability to save data and programs beyond temporary sessions. Understanding storage management impacts programming practices in assembly.
These components together enable the execution of instructions written in assembly language. By comprehending how they interact, programmers can write code that is more optimized and efficient all while harnessing the true capabilities of the computer.
Assembly Language Syntax and Structure
Understanding assembly language syntax and structure is paramount for anyone venturing into low-level programming. This domain emphasizes efficiency and control of the hardware. Assembly language serves as a bridge between machine code and higher-level languages. The syntax provides rules that inform how operations are to be performed by the CPU, allowing programmers to write instructions that directly manipulate hardware.
Mastering the syntax allows developers to produce efficient and performant code. High-level languages abstract many hardware details, but assembly exposes these complexities. Knowing these fundamentals is essential for debugging, optimization, and systems programming.
Basic Syntax Rules
Basic syntax rules dictate how instructions and commands are structured in the assembly language. Each line of assembly usually corresponds to a single operation. In general, the syntax to write these instructions starts with the opcode, followed by the operands. The opcode specifies the operation, while operands specify data or addresses.
For example, in x86 assembly, an instruction may look like this:
This statement moves the content of register BX to register AX.
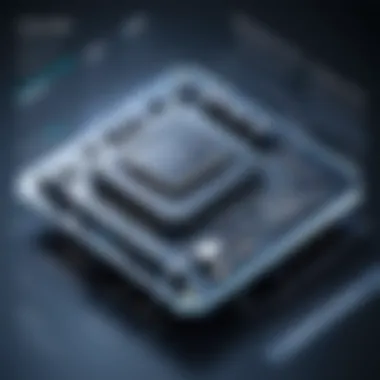
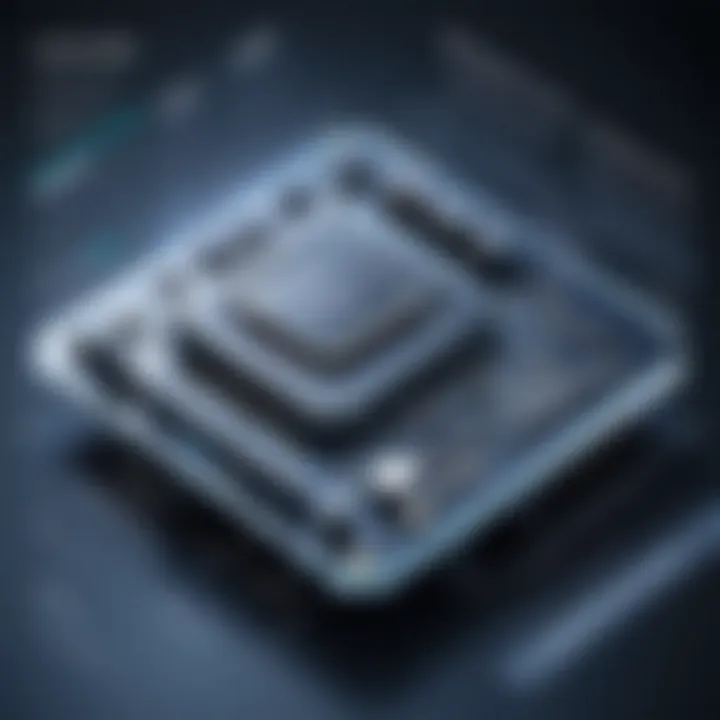
Key syntax rules include:
- Case Sensitivity: Assembly instructions are often case-sensitive.
- Comments: Use of comments is crucial for documentation. Comments can generally be added by using a semicolon ().
- Labels: Labels mark positions in the code. They help in navigating through the code, especially for jumps and loops.
Instruction Formats
Instruction formats define how instructions are encoded. Each format serves a purpose, depending on the architecture. Common formats include:
- Zero-operand instructions: Used for operations like (no operation).
- One-operand instructions: Often include operations like which increments the specified register or memory location.
- Two-operand instructions: The most common format, as seen in , where two operands are manipulated.
Each format has its constraints and allows for various types of data manipulation. Understanding these formats is critical when writing efficient assembly programs as they directly impact processor execution.
"The intricacies of assembly language syntax and structure are not just academic; they are critical for efficient, reliable software development."
In summary, grasping assembly language syntax and structure is foundational. It enables developers to write precise, low-level code, enhancing the functionality and performance of programs.
Different Assembly Language Dialects
Understanding different assembly language dialects is essential in grasping how various computer architectures function. Each dialect is tailored to a specific architecture and thus reflects its design philosophy and operational characteristics. This section will explore the significance of x86, ARM, and MIPS assembly languages. By learning these dialects, programmers gain insights into performance optimization, hardware interaction, and efficient resource management.
x86 Assembly Language
x86 assembly language is associated with Intel architecture, which has been the dominant platform for personal computers for decades. Its syntax is complex due to the rich set of instructions and addressing modes. For programmers, knowing x86 assembly is crucial for low-level system programming, operating systems, and performance-critical applications.
The x86 architecture includes both 32-bit (IA-32) and 64-bit mode (x86-64). A key benefit of learning this dialect is its widespread use in applications. The ability to write assembly code for x86 processors can lead to better optimization in performance-sensitive tasks like game development or software requiring direct hardware interaction.
ARM Assembly Language
ARM assembly language is linked to the ARM architecture, which is prevalent in mobile and embedded systems. ARM’s instruction set is simpler than x86’s, offering a reduced number of instructions. This makes it easier to understand but does not compromise power. The ARM architecture emphasizes energy efficiency, making it ideal for use in smartphones and tablets.
One notable feature of ARM assembly is its support for a wide range of devices. Almost any developer creates applications that run on ARM-based systems. Understanding ARM assembly is beneficial for developing applications in mobile computing and embedded systems, as it allows software developers to exploit advanced features and optimize for low power consumption.
MIPS Assembly Language
MIPS assembly language is designed for a specific architecture often used in educational settings and embedded systems. MIPS stands for Microprocessor without Interlocked Pipeline Stages. The clean and straightforward design of MIPS assembly makes it an excellent choice for teaching the principles of computer architecture and assembly programming.
MIPS architecture uses a fixed instruction length and a load/store model, simplifying the programming process. Its minimalism helps learners understand core concepts without being overwhelmed by complex instructions and modes of addressing. MIPS assembly is particularly useful in embedded systems and networking devices, where efficiency and performance are paramount.
In summary, familiarity with these assembly dialects—x86, ARM, and MIPS—provides programmers with the tools they need to interact with the hardware efficiently. Each dialect brings its own set of challenges and benefits, making the learning process both necessary and rewarding for those looking to work closely with computer architecture.
Tools and Environments
The realm of assembly language programming revolves around the use of specific tools and environments that facilitate the development process. Understanding these tools is crucial for both novice programmers and seasoned professionals. It allows them to write, compile, and debug assembly code efficiently. The choice of tools can significantly impact productivity and the effectiveness of the coding workflow.
Assemblers and Linkers
Assemblers convert assembly language code into machine code, which the computer's CPU can understand. This transformation is vital because CPU operates only on binary instructions. An assembler typically takes a human-readable assembly program and creates an object file with machine code.
In addition to assembling, linkers play a key role. They are responsible for combining multiple object files into a single executable program. This is particularly important in large projects where functionalities are divided into different modules. The linker resolves references between these modules, ensuring that the final executable runs smoothly.
Key benefits of using good assemblers and linkers include:
- Error Detection: Many modern assemblers provide error reporting that helps programmers identify syntax mistakes early in the development process.
- Optimization: Some assemblers have optimization options that can improve the execution efficiency of the resulting machine code.
- Modularity: Linkers allow programmers to reuse code by linking libraries, which can save time and effort.
Popular assemblers include NASM (Netwide Assembler) for x86 architecture, and ARM's assembler for ARM architecture. These tools cater to specific architectures, and each has its own advantages and settings.
Emulators and Debuggers
Emulators simulate hardware components, allowing programmers to test their assembly code in a controlled environment. They are essential for running code that is designed for different architectures without needing physical hardware. Emulators enable developers to replicate the behavior of a machine, ensuring that the code will function as intended when transferred to the target system.
Debuggers are integral to the development process, providing the means to inspect the state of a program while it runs. This can help in identifying issues such as incorrect data manipulations or logical errors in the code. **Tools like GDB (GNU Debugger) are commonly used with assembly code, allowing users to:
- Set breakpoints to pause execution.
- Inspect variables and memory contents.
- Step through code line by line, which aids in understanding program flow.
Both emulators and debuggers can greatly enhance the learning experience for new assembly language programmers. They provide a safe and effective way to explore programming concepts without the risk of affecting live systems.
"Understanding the tools of assembly language programming enhances productivity and effectiveness in grasping low-level computing concepts."
Assembly Language Programming Concepts
Assembly language programming is the bedrock of understanding low-level computing. This section highlights key concepts like data types, control flow, and the use of subroutines and procedures, underlining their significance in assembly programming.
Data Types and Variables
In assembly language, data types are crucial. Unlike high-level languages that offer abstract data types, assembly language exposes the programmer directly to memory. This requires understanding how various types of data are stored and manipulated. Common data types include bytes, words, and double words.
Variables in assembly are essentially labels that correspond to memory locations. They hold data values, and the process of defining variables is vital. By using proper naming conventions and data types, programmers can ensure that their code is not only functional but also comprehensible. Each variable in assembly has a defined size and must be carefully managed to minimize errors like overflow.
Programmers should keep in mind that each computer architecture may define its own set of data types, which can influence programming style and strategy. For instance, x86 architecture has its own specifications that must be considered when defining and using variables.
Control Flow Instructions
Control flow instructions dictate the execution order of the program. In assembly language, these instructions are fundamental for making decisions based on conditions. Common control flow instructions include jumps, branches, and loops. They enable programmers to implement logic such as statements and loops, all at a low level.
The significance of control flow cannot be overstated. In assembly, a simple branch can make the difference between a functioning program and one that fails. Effective use of control flow constructs allows for the creation of complex algorithms in assembly language.
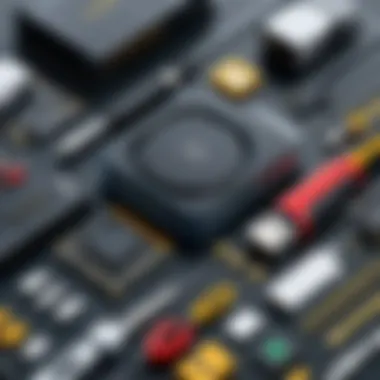
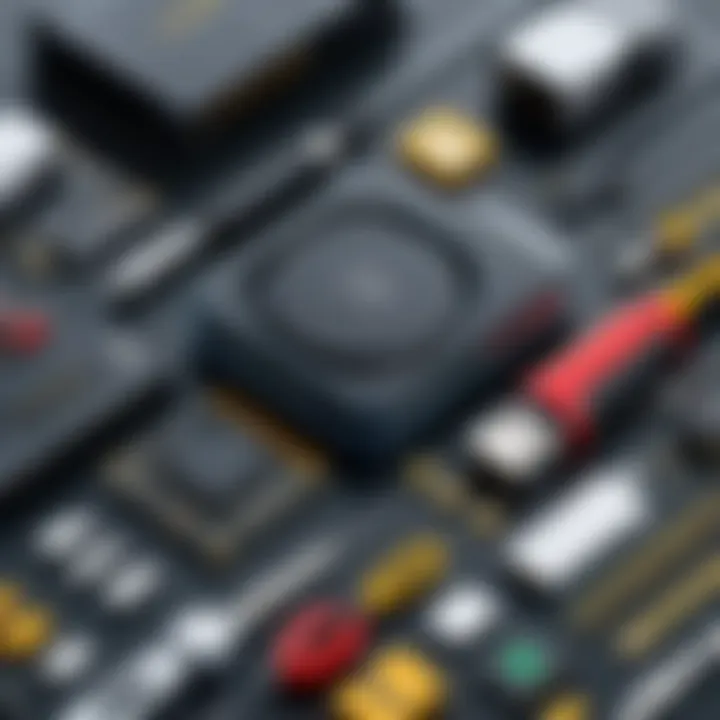
Programmers must also understand how the flow of control interacts with the processor's architecture. The way jumps are handled, for example, can vary significantly between different assembly dialects such as ARM or MIPS, adding another layer of complexity.
Control flow is the lifeblood of any program. It shapes how a program behaves based on conditions and user inputs.
Subroutines and Procedures
Subroutines and procedures enhance modularity in assembly programming. They allow portions of code to be reused without duplication, improving organization and readability. A subroutine can be thought of as a smaller program within a larger program, performing a specific task and returning a result.
The use of subroutines is particularly important when dealing with repetitive tasks. Instead of writing the same code multiple times, programmers can define a subroutine once and call it as necessary. This not only makes the code cleaner but also easier to maintain.
However, transitioning from high-level programming may lead to unique challenges. For instance, managing the stack for parameters and return values is crucial. Assembly requires a manual approach to parameters, often pushing them onto the stack before calling a subroutine.
In summary, mastering subroutines involves understanding the call stack, how to pass parameters, and how to clean up after the call.
Realizing the potential of assembly language programming involves grasping these foundational concepts. Through proper management of data types, control flow, and the use of subroutines, programmers can unlock powerful capabilities in assembly language.
Memory Management in Assembly
Memory management is a crucial topic when programming in Assembly language. Unlike high-level languages, where memory handling is often abstracted away, Assembly requires explicit management of memory allocation and deallocation. Understanding memory management allows programmers to utilize system resources efficiently, which is vital in systems programming and embedded development.
Effective memory management can improve the performance of an application. When one knows how to allocate memory correctly, it avoids unnecessary fragmentation and ensures that resources are optimally used. Furthermore, careful management can prevent common bugs, such as memory leaks and buffer overflows, which can lead to unpredictable behavior in programs.
Understanding Memory Addressing
Memory addressing is the method by which memory locations are accessed in a program. In assembly language, there are several addressing modes that dictate how data is retrieved or stored. Some common addressing modes include:
- Immediate addressing: The operand is a constant value. For example, in the instruction , the value 1 is stored directly in the AL register.
- Direct addressing: The operand specifies a memory location directly. For instance, where 1234H is the address of the data in memory.
- Indirect addressing: The operand contains a pointer to another memory location. An example is , where BX contains the address of the data.
- Indexed addressing: Combines two values to access the memory location. Like , which uses both BX and SI registers.
These modes allow developers to write efficient and flexible programs. Understanding how to effectively use each mode is essential for optimizing performance and resource management in assembly programming.
Stack vs. Heap Allocation
Memory allocation in assembly assumes a different approach compared to high-level languages. It can be categorized into two main types: stack and heap.
Stack Allocation:
- The stack is a section of memory that follows the Last In First Out (LIFO) principle.
- Memory management for stack is automatic. When a function is called, space is allocated on the stack, and it is freed when the function ends.
- It is generally used for local variables and function call management.
- Fast memory access is a key feature of stack allocation.
Heap Allocation:
- The heap is a large region of memory used for dynamic memory allocation.
- Unlike the stack, developers must manage the memory manually. When allocating memory on the heap, one must remember to free it when done.
- The heap provides flexibility for dynamic data structures but can lead to fragmentation if not managed carefully.
Both stack and heap offer unique benefits. Choosing between them depends on the requirements of the application being developed.
Efficient memory allocation and management are key components in optimizing assembly programs, both in performance and in resource handling.
Effective memory management is not only about choosing stack or heap but also about understanding the intricacies of how memory is laid out and accessed in an Assembly language context.
Practical Applications of Assembly Language
Assembly language is not merely a theoretical study; it has substantial real-world applications that highlight its importance in the field of computer science. Understanding these applications aids in appreciating the relevance of low-level programming. Here, we explore two significant domains where assembly language plays a crucial role: systems programming and embedded systems development.
Systems Programming
Systems programming refers to the creation of system software that provides a platform for applications to run. Programs like operating systems, device drivers, and system utilities are commonly developed using assembly language. Given that assembly provides direct access to hardware resources, it allows developers to write highly efficient code that utilizes the underlying hardware to its fullest potential.
- Efficiency: Assembly language allows for precise control over system resources, enabling developers to optimize performance. This is particularly crucial for components where speed and resource management are critical.
- Low-Level Access: By using assembly language, programmers can interact directly with hardware components, which is not feasible with high-level languages. This is essential when developing system-level applications that need to manipulate processor registers or memory directly.
- Resource-Constrained Environments: In situations where hardware resources are limited, such as embedded systems, assembly language proves more efficient than high-level programming languages. Assembly language helps to produce smaller and faster executables.
"Assembly programming fosters an understanding of how computers work at the most basic level, providing insight that is beneficial for all programmers."
This practicality makes assembly language invaluable for performance-critical applications such as game engines and real-time processing systems.
Embedded Systems Development
Embedded systems are computer systems designed for specific functions within larger systems. This can be found in appliances like microwaves, washing machines, and various consumer electronics. Developing software for embedded systems often requires assembly language due to the necessity for fine-tuned performance and minimal resource consumption.
- Customized Solutions: Embedded systems often require customized code that respects memory constraints and processing limitations. Assembly language allows developers to tailor solutions to meet specific design requirements.
- Real-Time Performance: Many embedded applications must meet real-time performance criteria. Assembly language enables precision timing and robust hardware interaction, which is essential for meeting time-sensitive tasks in applications such as automotive and medical devices.
- Power Efficiency: In battery-operated devices, conserving power is a priority. The ability to write lightweight assembly code ensures that the system consumes minimal energy while performing its tasks, prolonging battery life.
Learning Assembly Language
Understanding assembly language is crucial for anyone looking to delve deeper into computer science and programming. It serves as a fundamental layer in the programming spectrum, bridging the gap between high-level programming languages and machine code, which is directly executed by the computer's hardware. Learning assembly language equips programmers with insights into how their code interacts with the processor and memory, enabling them to write more efficient code and troubleshoot at a lower level.
Mastering assembly language also fosters critical skills such as debugging and optimizing code. The process encourages a deeper understanding of algorithms and system performance, allowing learners to appreciate the importance of each instruction executed by the processor. In addition, this knowledge can be invaluable when working in environments where performance is of utmost importance, such as systems programming and embedded systems.
However, the study of assembly language is not without challenges. Many learners find the syntax challenging and often feel overwhelmed when faced with concepts like pointers, registers, and memory management. Engaging with these topics does, however, create a more rounded programmer, able to reason about complex systems with a higher degree of clarity.
Recommended Resources and Tutorials
To effectively learn assembly language, various resources are available that cater to different learning styles.
- Books:
- Online Courses:
- Online Communities:
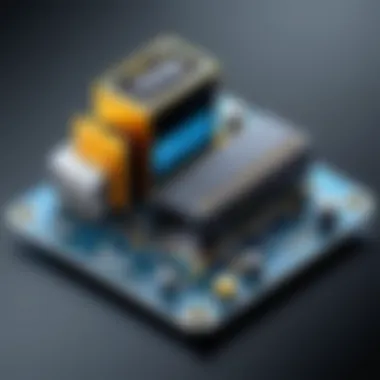
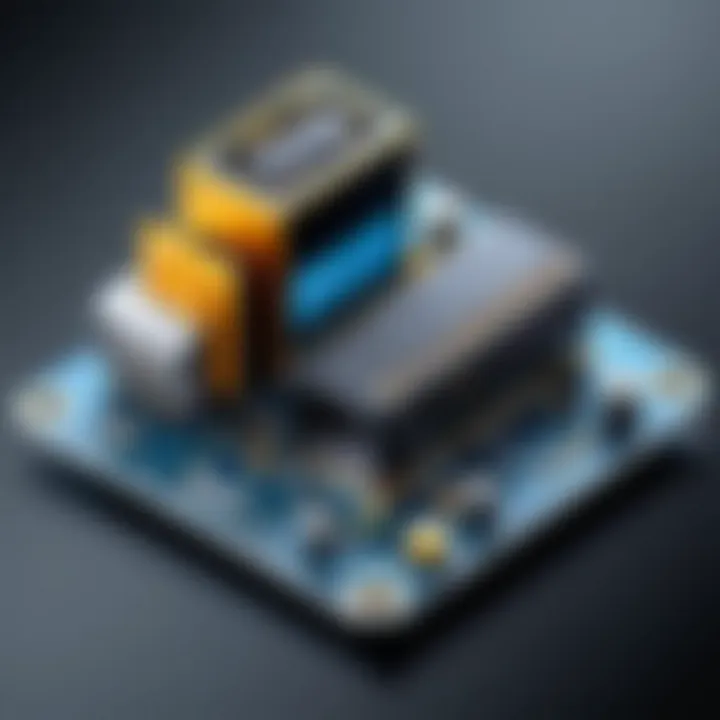
- Programming from the Ground Up by Jonathan Bartlett provides a practical approach to understanding assembly through real-world examples.
- Computer Systems: A Programmer's Perspective by Randal E. Bryant and David R. O'Hallaron emphasizes the connection between high-level programming and system-level coding.
- Courses on platforms such as Coursera and edX offer structured learning paths and can be beneficial for beginners. Topics typically include fundamentals of assembly language, systems programming, and architecture.
- YouTube channels like "The Cherno" often produce helpful video explanations and coding sessions that can complement text-based resources.
- Websites like Reddit have active forums where learners can engage in discussions about problems they face. Joining communities focused on programming can provide immediate support and foster collaboration.
Possessing a mix of these resources can greatly enhance the learning experience, allowing for different perspectives and methodologies to be applied.
Common Challenges Faced by Learners
While learning assembly language can be rewarding, students often encounter several hurdles:
- Complex Syntax:
Assembly language has a steeper learning curve compared to higher-level languages. The syntax can be cryptic and varies significantly between different architectures. This inconsistency may lead to confusion, especially for those who are accustomed to more readable languages like Python or Java. - Memory Management Understanding:
Memory concepts can be daunting. Understanding how the stack and heap are managed, as well as how to effectively allocate and deallocate memory, is crucial but often challenging for new learners. - Debugging:
Debugging assembly code can be more complex than debugging in high-level languages. When things go wrong, pinpointing the issue may require an intimate understanding of the inner workings of the code and the hardware it runs on. - Lack of Immediate Feedback:
Unlike high-level languages that often feature interactive environments, assembly language programming usually does not provide instant feedback, making it difficult to gauge correctness until the program is assembled and tested.
"Embracing the challenges of learning assembly language can lead to profound insights into programming and computer science."
Overall, while the path to learning assembly language may be complex, the benefits derived from mastering it far outweigh the hurdles. With the right mindset, resources, and community support, learners can navigate the intricacies of assembly language effectively.
Assembly Language vs. High-Level Languages
In the realm of programming, understanding the differences between Assembly language and high-level languages is essential. Assembly language serves as a bridge between raw machine code and high-level programming, making it crucial for anyone interested in low-level programming concepts. This section will explore key elements that distinguish Assembly language from higher-level alternatives, including their benefits, considerations, and real-world applications.
Performance Considerations
When it comes to performance, Assembly language often outshines high-level languages. This advantage arises primarily from the following factors:
- Direct Hardware Manipulation: Assembly language allows programmers to interact closely with hardware components. This means they can optimize code for specific hardware architectures, resulting in faster execution speeds.
- Minimal Overhead: High-level languages come with abstractions that may introduce overhead, such as automatic memory management or extensive libraries. Assembly language, in contrast, enables fine-tuned control over resources, often resulting in leaner code.
- Greater Efficiency: In scenarios where performance is critical, such as embedded systems or real-time applications, Assembly can provide the necessary capabilities to meet strict timing constraints.
Despite these advantages, one must also consider the following challenges when opting for Assembly language:
- Complexity: Writing in Assembly requires a deep understanding of the underlying architecture. This complexity can lead to longer development times compared to high-level languages.
- Maintainability: Assembly code tends to be less readable and more challenging to maintain. High-level languages often employ clear syntax and structure, aiding collaborative programming efforts.
In summary, while Assembly language offers superior performance, the implications for code complexity and maintainability cannot be ignored.
Use Cases for Each Language Type
Different programming languages offer distinct strengths that make them suitable for various contexts and applications. Here are some common use cases for both Assembly language and high-level languages:
Assembly Language Use Cases
- System Software Development: Writing operating systems or device drivers often requires the precision and performance that Assembly provides.
- Embedded Systems: Assembly is frequently used in microcontrollers found in appliances, automotive controls, and medical devices.
- Performance-Critical Applications: Real-time systems or applications requiring minimal lag often rely on Assembly for its efficiency.
High-Level Language Use Cases
- Web Development: Languages like JavaScript and Python are commonly used for building web applications due to their extensive libraries and frameworks.
- Data Analysis and Machine Learning: Python and R dominate the fields of data science and machine learning, offering robust libraries that simplify complex tasks.
- Rapid Prototyping: High-level languages allow for quicker development cycles, making them better suited for projects where speed is essential.
"Choosing the right programming language depends on the project requirements and the specific goals of the developer. Understanding the characteristics of each language is critical for making informed decisions."
Future of Assembly Language
The future of assembly language is not just a reflection of its past but rather an indication of how computing evolves. This programming language, often seen as archaic in comparison to modern high-level languages, remains relevant due to its fundamental connection to how computers operate. As technology advances, understanding assembly language will become increasingly important for certain niche areas of programming and computer science.
Evolving Technologies and Trends
As technology progresses, several trends are shaping the landscape of assembly language. First, there is a growing interest in performance optimization. As systems become more complex and demands for speed increase, programmers are turning to assembly language to achieve maximum efficiency. This is particularly true in systems programming, where accessing hardware directly can minimize latency and improve execution speed.
Moreover, the rise of embedded systems highlights the need for assembly language. Devices such as IoT sensors and automotive control systems often require low-level programming to operate effectively. These systems need to manage resources efficiently, making assembly a suitable choice for developers.
The development of more user-friendly assemblers is also noteworthy. As tools improve, more programmers may be encouraged to explore assembly language, which can in turn lead to innovation within low-level programming.
The Role of Assembly in Modern Computing
While high-level languages dominate the software development landscape, assembly language retains a vital role in various applications. It serves as the backbone for operating systems, device drivers, and performance-critical software. Understanding how these components interact at a low level is crucial for debugging and optimizing performance.
Additionally, assembly language helps demystify how computers execute high-level code. When a developer knows how their code translates into machine instructions, they can write more efficient programs. This knowledge is beneficial not only for academic purposes but also in the professional sphere where performance can make or break a product.
In education, introducing assembly language allows students and those new to programming to grasp essential concepts of computer architecture and operation. This foundation is invaluable, paving the way for advanced studies in computer science and software engineering.
"A strong grasp of assembly language can set apart skilled developers from their peers, enabling them to create optimized, high-performance applications."
Epilogue
The conclusion of this article serves as the final touchstone for understanding the significance of assembly language within the broader landscape of programming. As explored throughout the sections, assembly language provides a direct means of interacting with hardware. It is not just a historical artifact; its application remains relevant for several reasons.
Importance of Key Concepts: Understanding assembly language is essential for grasping how computers operate at a fundamental level. Knowledge of assembly can lead to better optimization of code when developing in higher-level languages. This comprehension translates to improved performance in critical applications, especially in systems programming and embedded systems development.
Summary of Key Points
- Core Understanding: Assembly language is the bridge between machine code and high-level programming languages. This knowledge is vital for debugging and optimizing applications.
- Applications: The use of assembly language spans across diverse fields such as system-level programming, operating systems, and embedded systems, demonstrating its continued relevance.
- Learning Pathways: Resources and hands-on practice remain crucial to mastering assembly language. Many tools are available to aid learners from assemblers to emulators, reinforcing practical learning.
- Future Trends: Despite the rise of high-level languages, assembly language evolves alongside technological advancements, remaining a cornerstone of computer science influence.
Final Thoughts on Learning Assembly
Learning assembly language is undoubtedly challenging, but the rewards are significant. It cultivates a deeper understanding of computing mechanisms, offering insights that high-level programming cannot fully provide. As students and aspiring programmers dive into this low-level language, they gain:
- Enhanced Problem-Solving Skills: Working with assembly language sharpens analytical skills, as it requires a precise approach to problem-solving.
- Real-World Application Knowledge: The principles learned have direct applications in cybersecurity, hardware design, and performance optimization.
- Community Learning Opportunities: Engaging with communities on platforms like Reddit can provide support and motivation as learners navigate through complexities.
Embracing assembly language opens pathways to a profound understanding of computer science, equipping learners with skills that are not only foundational but also transformative for their careers. Through patience and dedication, the struggles faced in learning assembly language can lead to a more robust programming capability.