Fundamental Principles of Software Engineering Explained
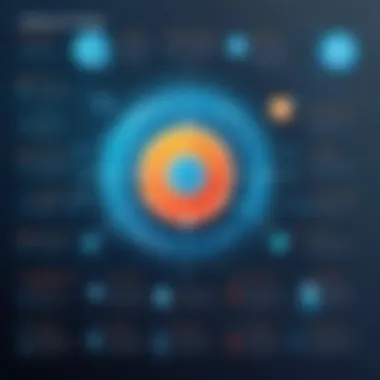
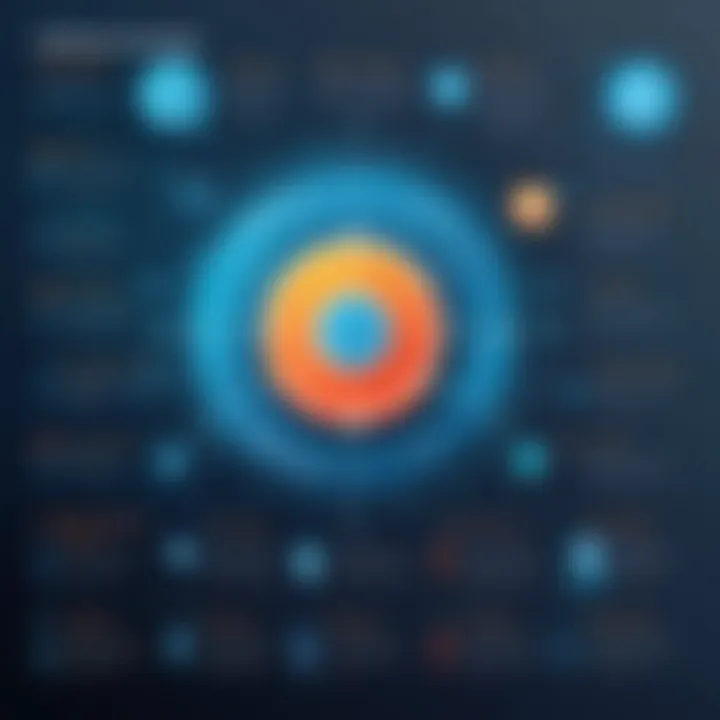
Prelude to Programming Language
Software engineering relies heavily on programming languages. Understanding how these languages work is essential for anyone delving into the field. Each language offers unique features which cater to different development needs.
History and Background
Programming languages have evolved significantly since their inception. Early languages, like Assembly, were cumbersome. They required a deep understanding of hardware. As computers advanced, higher-level languages like Fortran and C emerged. These allowed developers to write code that is more understandable and closer to human language. Today, languages like Python and JavaScript dominate the scene due to their versatility and ease of use.
Features and Uses
Modern programming languages come with various features that make them suitable for different applications. Some key features include:
- Syntax simplicity: Easier to learn and use.
- Built-in libraries: Pre-written code for common tasks.
- Cross-platform compatibility: Can run on different systems without modification.
These features enable languages to be used in web development, data analysis, artificial intelligence, and more.
Popularity and Scope
Every developer should be aware of the popularity of certain programming languages. JavaScript reigns supreme in web development. Its vast ecosystem supports frameworks like React and Angular. Python is known for data science. It provides libraries like Pandas and NumPy. Understanding the scope of these languages helps when choosing which to learn based on career goals.
Basic Syntax and Concepts
To fully grasp software engineering, one must first understand basic syntax and concepts of programming languages. These are the building blocks for writing effective and efficient code.
Variables and Data Types
Variables are used to store data. Each variable can hold different types of data, such as:
- Integers: Whole numbers.
- Floats: Numbers with decimal points.
- Strings: Text data.
- Booleans: True or false values.
Understanding data types is critical for correctly processing information in programs.
Operators and Expressions
Operators allow developers to perform operations on variables and values. Common operators include:
- Arithmetic operators: For basic math, like addition and subtraction.
- Comparison operators: To compare values, such as equal to or greater than.
- Logical operators: Used for combining multiple conditions.
Control Structures
Control structures dictate the flow of a program. Key control structures include:
- If statements: Execute code based on conditions.
- Loops: Repeat actions until certain conditions are met, such as and loops.
Advanced Topics
Advanced programming concepts build on basic knowledge. These topics enhance efficiency and code organization.
Functions and Methods
Functions encapsulate reusable code. They take inputs and return outputs. This promotes the DRY (Don't Repeat Yourself) principle. Methods are functions associated with objects in object-oriented programming. They help manage complex data structures effectively.
Object-Oriented Programming
Object-oriented programming (OOP) is fundamental in modern software engineering. It promotes:
- Encapsulation: Bundling data and methods.
- Inheritance: Allows new classes to adopt properties from existing ones.
- Polymorphism: Enables a single interface to represent different data types.
Exception Handling
Exception handling is crucial for managing errors gracefully. It prevents program crashes. Try-catch blocks are common patterns used for handling errors without halting the program. Implementing proper exception handling improves reliability.
Hands-On Examples
Practical examples cement theoretical knowledge. Here, we explore simple and intermediate coding practices.
Simple Programs
Starting with simple programs helps build confidence. A basic Hello World program is often the first written:
This example showcases basic syntax and output operation in Python.
Intermediate Projects
As skills improve, tackling intermediate projects becomes essential. Creating a todo list application introduces concepts like user input and data storage. Integrating with databases can be a valuable practice.
Code Snippets
Utilizing code snippets can save time and enhance productivity. Familiarity with reusable code, such as sorting algorithms or data validation functions, enables quicker development cycles.
Resources and Further Learning
For those seeking to deepen their understanding further, numerous resources are available.
Recommended Books and Tutorials
Books like "Clean Code" by Robert C. Martin provide essential insights into writing maintainable code. Online tutorials on platforms like Codecademy assist in practical learning.
Online Courses and Platforms
Websites like Coursera and Udacity offer comprehensive courses on various programming languages. These courses are ideal for structured learning.
Community Forums and Groups
Joining forums like Stack Overflow or subreddits on Reddit can connect you with fellow learners and professionals. Engaging with the community accelerates learning through shared knowledge and resources.
"A solid understanding of programming principles lays the foundation for success in software engineering."
By exploring these principles, software engineers enhance their capabilities. The journey of software engineering is ever-evolving; staying informed is vital for achieving quality and efficiency in this field.
Intro to Software Engineering
Software engineering represents a pivotal discipline in the realm of technology. It encapsulates a structured approach to the development of software, ensuring that applications not only meet user needs but also function efficiently and reliably. This initial section guides readers through essential considerations regarding software engineering, emphasizing its relevance, core tenets, and applications.
With this article, readers will uncover how these fundamentals guide the processes involved in software development. This includes an examination of requirements analysis, design strategies, user interface considerations, implementation protocols, testing methodologies, and essential maintenance. Each component plays a role in enhancing the overall quality and efficiency of software products.
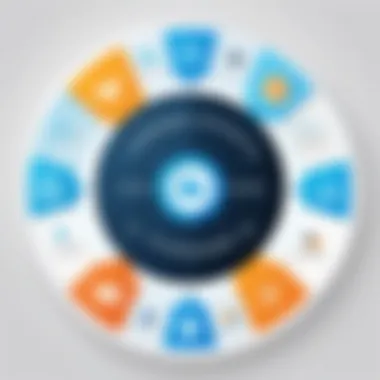
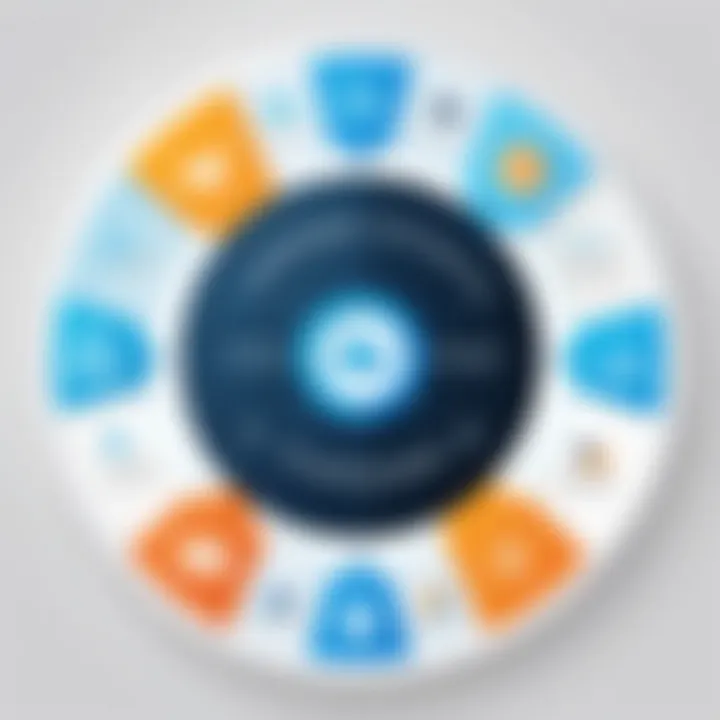
The benefits of mastering software engineering principles are manifold:
- Increased Quality: Software engineering practices aim to reduce defects and improve user satisfaction.
- Efficiency in Development: Methodologies streamline workflows, ensuring timely delivery.
- Scalability: Well-engineered software can adapt to changing demands without significant rework.
- Collaboration: Defined processes foster teamwork, making it easier for diverse skill sets to contribute.
In the upcoming sections, we will delve deeper into specific aspects of software engineering, shedding light on its definition, vital importance, and core principles. This exploration will furnish readers with a comprehensive understanding of how software engineering shapes our technological landscape.
Definition of Software Engineering
Software engineering refers to the systematic application of engineering approaches to software development. It involves utilizing methodologies, practices, and tools to cultivate high-quality software products that meet established requirements. The discipline encompasses various stages: from requirement analysis to maintenance, integrating best practices throughout.
Software engineering is not merely about coding. It involves understanding user needs, designing effective solutions, and ensuring reliability and maintainability in the long term. The convergence of technical skills with a structured approach is what defines good software engineering.
The Importance of Software Engineering
The importance of software engineering cannot be overstated. In an era where software permeates every facet of our lives—from business applications to social interactions—effective engineering practices become imperative.
Here are key points to highlight its significance:
- Risk Mitigation: Proper engineering practices help identify potential issues early in the process, reducing the likelihood of failure.
- Cost-Effectiveness: By adhering to established methodologies, organizations can avoid unnecessary rework, saving both time and resources.
- User Satisfaction: Well-engineered software is designed with the user in mind, leading to higher levels of satisfaction and engagement.
- Sustainability and Maintenance: Good software engineering considers long-term use and the need for updates, ensuring that software remains relevant and functional over time.
In summary, understanding software engineering is essential for anyone engaged in software creation. The principles laid out in this article serve as a guide to navigate complex software projects, enhancing the prospects for success.
Modularity
Modularity is a fundamental principle that involves breaking down a software system into smaller, manageable components or modules. Each module can be developed, tested, and maintained independently. This separation allows for easier debugging and enhances the flexibility of the software. Modularity promotes reusability; once a module is created, it can be reused in different projects, saving time and resources.
Benefits of modularity include:
- Improved maintainability: Isolating individual components simplifies changes and updates, reducing the potential for introducing new bugs.
- Ease of collaboration: Teams can work on different modules simultaneously, speeding up the development process.
- Focus on specific functionalities: Developers can concentrate on one aspect of the project at a time, which can lead to more thoughtful and quality code.
Abstraction
Abstraction is the process of simplifying complex systems by hiding unnecessary details and exposing only the essential features. This principle helps in managing complexity by allowing developers to focus on high-level functionality rather than low-level implementation. For example, when using a programming language, abstraction enables a programmer to utilize functions and methods without needing to understand the underlying code.
Common advantages of abstraction include:
- Reduced complexity: By hiding implementation details, developers can focus on what the software does rather than how it does it.
- Simplified interfaces: Abstraction allows for cleaner code and easier interaction between components, which aids in understanding and maintainability.
- Encouragement of modular design: As systems are designed with abstraction in mind, they tend to naturally align with modular structures.
Encapsulation
Encapsulation refers to bundling the data with its operations in a single unit and restricting access to some components. This principle not only protects the integrity of the data but also enforces a clear separation between the interface and implementation. By hiding the internal state of an object and requiring all interaction to occur through well-defined methods, encapsulation aids in building robust and maintainable code.
Important aspects of encapsulation include:
- Data hiding: By preventing external access to internal data, encapsulation protects the system from unintended interference and misuse.
- Controlled access: Only the methods provided allow interaction, which enforces a strict interface and improves design clarity.
- Ease of maintenance: Changes in the internals of a class can happen without affecting those who interact with it, leading to reduced side effects during updates.
Separation of Concerns
The principle of separation of concerns dictates that a software system should be divided into distinct sections, each addressing a separate concern or functionality. This practice reduces complexity by allowing developers to focus on isolated parts of the system without impacting others. It promotes a cleaner structure and enhances readability.
Key benefits of separation of concerns include:
- Enhanced organization: Clearly defined components lead to better project structure and prevent overlap that can complicate the code.
- Better collaboration: Teams can work on different concerns independently, allowing for parallel development and more efficient workflows.
- Easier debugging: Isolated concerns mean that when an issue arises, it can be easily traced back to a specific component, facilitating faster resolutions.
In summary, mastering these core principles not only elevates the standard of software engineering but also prepares practitioners for the complexities of real-world development challenges.
Software Development Life Cycle Models
Software Development Life Cycle (SDLC) models are essential frameworks that guide the software development process. Understanding these models is crucial for effective project management and successful software delivery. Each model provides a structured approach to planning, executing, and validating software development projects. The choice of model impacts timelines, resource allocation, and quality. Thus, software engineers must carefully consider their project needs when selecting an appropriate SDLC model.
The benefits of a well-defined SDLC model include enhanced process visibility, improved communication among stakeholders, and a systematic approach to managing risks and changes. By following a model, teams can adapt to challenges and ensure that project objectives align with client expectations.
"An effective SDLC model acts as a roadmap for project teams, providing direction and reducing uncertainty."
Waterfall Model
The Waterfall Model is one of the earliest SDLC approaches. It is characterized by a linear sequence of phases where each stage must be completed before the next one begins. Typically, these phases include requirements analysis, design, implementation, testing, deployment, and maintenance. This model is straightforward and easy to understand, making it particularly suitable for projects with clearly defined requirements.
However, the Waterfall Model has limitations. Changes in requirements after the project has commenced can lead to significant challenges, as one cannot go back to the previous phase easily. Hence, while it promotes discipline and documentation, it lacks flexibility. It is often most successful in projects where requirements are stable and predictable.
Agile Methodology
Agile Methodology represents a more flexible approach to software development. Its core principle revolves around iterative progress through small increments or sprints. Each sprint typically consists of planning, execution, testing, and review. Agile promotes constant collaboration between teams and stakeholders, which helps in accommodating changing requirements even late in the development process.
The benefits of Agile include increased adaptability and customer satisfaction. Projects can adjust direction based on feedback, ultimately leading to software that better meets user needs. Although Agile requires significant communication and can sometimes lack detailed planning, its focus on value delivery places it as a popular choice in today's dynamic development environment.
Scrum Framework
Scrum is a subset of Agile that provides a more structured approach to implementing Agile principles. It defines specific roles such as the Product Owner, Scrum Master, and Development Team, each with their responsibilities. Scrum utilizes short iterations, referred to as sprints, usually lasting two to four weeks. During each sprint, teams focus on delivering a potentially shippable product increment.
Scrum also emphasizes regular ceremonies like daily stand-ups, sprint reviews, and retrospectives. These activities foster accountability, enhance communication, and offer opportunities for process improvement. The Scrum framework is particularly effective for projects requiring rapid delivery and frequent user feedback, allowing teams to pivot and innovate continuously.
Spiral Model
The Spiral Model combines elements of both iterative and waterfall approaches, facilitating risk management along with early project prototyping. This model emphasizes a circular process where developers complete multiple iterations or spirals. Each spiral consists of four main phases: planning, risk assessment, engineering, and evaluation.
This model is particularly beneficial for large projects with extensive risk factors. It allows flexibility in investments and development, as stakeholders can evaluate development needs at every loop of the spiral. However, the Spiral Model can be complex and may require significant budgeting and resources, making it more suitable for large organizations with adequate resources.
In summary, choosing the right Software Development Life Cycle model is essential for successful project execution. Each model possesses unique strengths and challenges, influencing factors such as team dynamics, project scope, and stakeholder engagement. Thus, software engineers should assess their project's specific context and requirements carefully to determine the most appropriate SDLC framework.
Requirements Engineering
Requirements Engineering is a fundamental aspect of software engineering. It serves as the foundation for the development process. This phase focuses on understanding and documenting what the stakeholders need from the software product. Poor requirements can lead to project failure, delays, and increased costs. Therefore, a thorough approach to requirements is crucial. It ensures that the product meets user expectations and fulfills business objectives.
In this section, we will discuss several key areas of Requirements Engineering including gathering requirements, specification techniques, and validation and verification processes. By exploring these topics, software engineers can gain insight into effectively managing the needs of their stakeholders, leading to successful project outcomes.
Gathering Requirements
Gathering requirements is the initial step in the requirements engineering process. It involves collecting information about what users want and need from the software. This phase often employs various techniques such as interviews, surveys, workshops, and observation.
In addition, engaging with stakeholders is essential. It ensures that the team captures a wide range of perspectives. This collaboration avoids potential misinterpretations later in the project. Misunderstandings during this stage can significantly hinder the development process.
Effective communication is key. Clarifying goals and expectations can lead to a more accurate depiction of requirements. Utilize tools like user stories and use cases to express these requirements clearly. Establishing a clear understanding at this step is vital for the entire project's success.
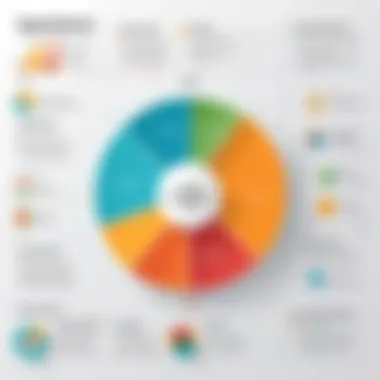
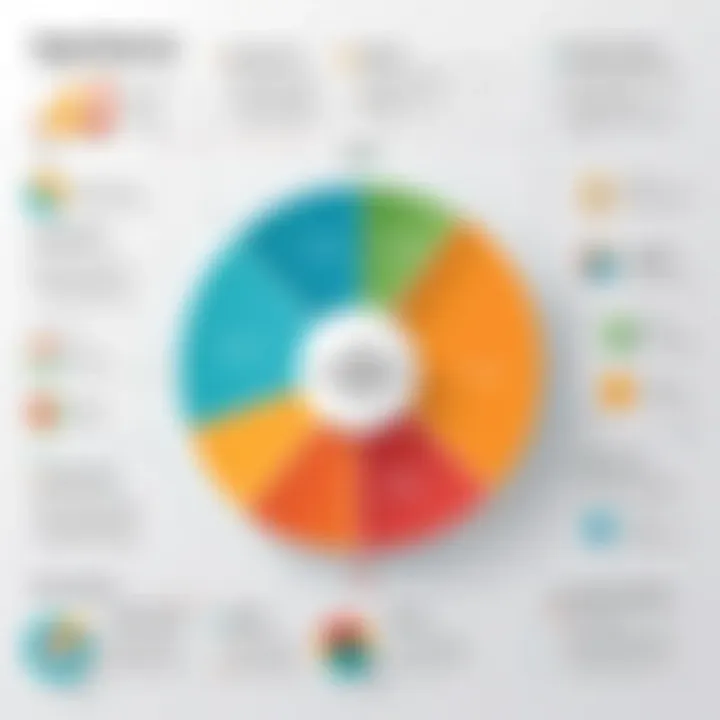
Specification Techniques
Once requirements are gathered, the next step is specifying them in a formalized way. Specification techniques help delineate the functionalities of the software system clearly. Common methods include:
- Natural Language: Writing detailed descriptions in everyday language. This approach is accessible but may introduce ambiguity.
- Modeling: Creating visual representations of requirements, such as UML diagrams. Diagrams provide clarity and can assist with understanding complex systems.
- Prototyping: Developing a preliminary version of the software to demonstrate functionalities. Prototyping allows stakeholders to provide feedback based on tangible examples.
Selecting the appropriate technique is essential. It depends on factors like the project size, complexity, and stakeholder familiarity with technical concepts. A right approach can facilitate effective communication and understanding of requirements.
Validation and Verification
Validation and verification are crucial to the requirements engineering process. Validation is about ensuring that the correct requirements were gathered, while verification checks that they are implemented correctly in the software.
Validation can occur through techniques like reviews and inspections. Bringing multiple stakeholders together to discuss requirements can uncover issues early. This proactive approach minimizes the chances of costly changes during later phases.
On the contrary, verification ensures that the software product meets the specified requirements once developed. This can involve testing against the specified requirements to confirm the system behaves as expected.
Software Design Principles
Software design principles are fundamental concepts that guide the structuring of software systems. They help ensure that software is not only functional but also maintainable, scalable, and efficient. These principles reduce the complexity of software development by establishing clear guidelines for design choices. Good design principles lead to improved communication among team members, easier debugging, and the potential for greater reuse of code components. Therefore, studying software design principles is essential for those involved in programming and software engineering.
Design Patterns
Design patterns are recognized solutions to common problems that occur in software design. They serve as templates to address recurring design challenges. By leveraging design patterns, developers can avoid reinventing the wheel, thus saving time and resources.
Some notable design patterns include:
- Singleton: Ensures a class has only one instance, providing a global point of access to it.
- Observer: Provides a subscription mechanism to allow multiple objects to listen and react to events or changes in another object.
- Factory Method: Defines an interface for creating an object, but lets subclasses alter the type of objects that will be created.
Understanding and applying these patterns can greatly enhance the quality of software solutions and promote best practices among developers.
User Interface Design
User interface design is a critical aspect of software engineering. It focuses on the layout and interaction elements of software to ensure an intuitive and efficient user experience. Good user interface design improves user satisfaction and engagement, leading to better retention rates.
Key considerations in user interface design include:
- Consistency: Uniform design across various screens helps users learn the application faster.
- Feedback: Providing users with feedback on actions taken fosters a sense of control.
- Accessibility: Ensuring that interfaces are accessible to all users, including those with disabilities, is crucial.
These principles not only improve usability but also contribute to the overall success of a product in a competitive market.
System Architecture
System architecture defines the structure and organization of a software application. It encompasses various components, standards, and interactions that shape the final product. A well-defined architecture can lead to significant benefits including:
- Scalability: Ability to handle growth efficiently without compromising performance.
- Maintainability: Easier updates and modification of the software over time.
- Resilience: Enhanced reliability by anticipating potential failures and designing systems that can withstand them.
Different architectural styles, such as microservices and monolithic architectures, offer various trade-offs for developers. Thus, understanding these choices is paramount in designing software that meets both current and future needs.
"Good software design is a vital part of successful software development. It influences how easy the software is to maintain and enhance over time, ultimately affecting the longevity of the product."
By incorporating robust software design principles, developers can create applications that not only function as intended but also evolve according to user needs while maintaining high-quality standards.
Implementation Strategies
In the realm of software engineering, implementation strategies serve as essential frameworks that guide developers through the process of translating design specifications into functional software. A well-considered implementation strategy ensures that the software is constructed efficiently and correctly, minimizing defects and facilitating easier maintenance. Various strategies exist, each with its unique strengths and challenges. By understanding these methodologies, software engineers can select the best approach for their specific project requirements, enhancing both workflow and product quality.
Coding Standards
Coding standards are a set of guidelines that dictate how code should be formatted and written. The importance of adhering to these standards cannot be overstated. Consistency in coding practices helps in enhancing readability, which is crucial for collaboration among developers. Code that follows a clear standard is easier to understand and modify. This leads to improved maintainability and lower chances of introducing bugs.
For practical application, many teams adopt well-known standards such as Google's Java Style Guide or Microsoft's C# Coding Conventions. Such standards often cover naming conventions, file structure, indentation, and more. By enforcing coding standards, teams can streamline code reviews and improve onboarding processes for new developers. The result is a more coherent codebase.
Version Control Systems
Version control systems (VCS) play a critical role in software development. They allow teams to track changes in source code over time. The benefits of employing a VCS are multifaceted. It provides a structured way to manage project files, enabling collaboration across different locations and among team members. Git, for instance, is a widely-used VCS that supports branching and merging, making it easier to experiment with new features without disrupting the main codebase.
Moreover, using version control systems helps in maintaining a chronological history of changes, which is invaluable for debugging and auditing purposes. In case of errors, reverting to a previous version can save time and effort. Understanding how to effectively use VCS becomes a valuable skill for software engineers.
Continuous Integration
Continuous integration (CI) is a development practice where developers integrate code into a shared repository multiple times a day. Each integration is verified by automated builds and tests. This practice greatly improves software quality and reduces integration issues. It ensures that errors are caught early, before they proliferate through the codebase.
The main advantages of CI include:
- Fast Feedback Loop: Developers receive immediate feedback on their code, allowing them to address issues right away.
- Reduced Integration Problems: By integrating frequently, teams minimize the chances of integration conflicts.
- Automated Testing: CI systems often run a suite of automated tests on new code to ensure functionality remains intact.
"Effective implementation strategies foster collaboration and reduce errors in software development."
Labels: en.wikipedia.org, reddit.com.
Software Testing Methods
Software testing methods are essential for ensuring the quality and reliability of software products. These methods allow developers to identify and correct defects before software is deployed. In the fast-paced world of software development, testing should not be an afterthought; rather, it must be an integral part of the development process. Proper testing increases customer satisfaction, reduces costs related to failures, and maintains the credibility of the software.
Given their importance, it is crucial to understand the various types of testing available. Each type has its specific focus and contributes to a comprehensive testing strategy.
Unit Testing
Unit testing involves testing individual components or modules of software. The main objective is to validate that each unit of the software performs as expected. Unit tests are generally automated and executed frequently. By running these tests, developers can catch issues early in the development cycle. This minimizes the time and cost associated with fixing problems later in the process.
Benefits of Unit Testing:
- Improves code quality
- Facilitates easier refactoring
- Enhances documentation of code functionality
Many popular frameworks exist to support unit testing, such as JUnit for Java and NUnit for C#. An important consideration in unit testing is the isolation of each component, ensuring that tests are independent of one another.
Integration Testing
Integration testing focuses on verifying the interactions between integrated components. After unit testing, components are combined, and integration tests ensure they work together correctly. The key goal here is to identify interface defects among modules.
"Integration testing helps to reveal issues that may not have surfaced during unit testing, particularly those related to data flow between modules."
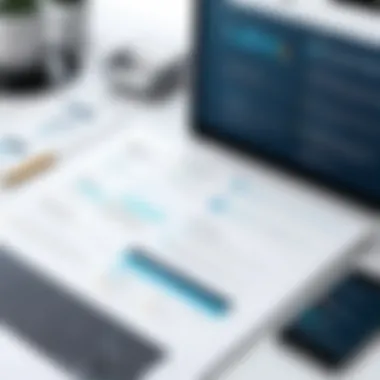
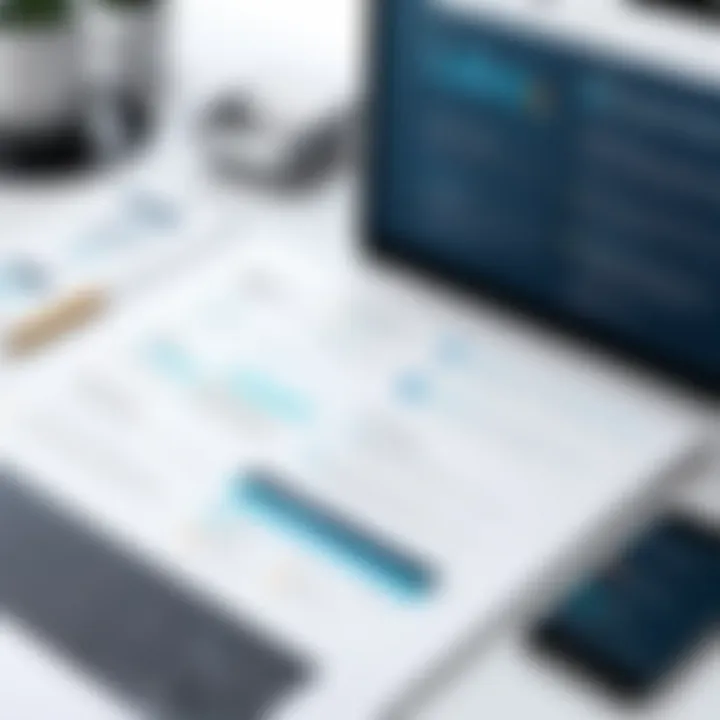
Key aspects to consider during Integration Testing:
- Check data transfer between modules
- Look for discrepancies in combined functionalities
Integration tests can be conducted using bottom-up, top-down, or sandwich methods, depending on the components involved and the specific testing requirements.
System Testing
System testing examines the entire software system as a whole. It takes into account the overall interactions and functionalities to ensure that the system meets its specified requirements. System testing is typically performed in an environment that closely mimics the production environment.
This level of testing is essential as it validates the software's compliance with functional and non-functional requirements. System testing also assesses aspects like performance, security, and usability.
Components of System Testing:
- Functional testing
- Performance testing
- Security testing
- Usability testing
Performing thorough system testing helps reduce the risks of software failures post-deployment.
Acceptance Testing
Acceptance testing is the final stage of testing before the software is released. This testing verifies whether the system meets the acceptance criteria and whether it is ready for deployment. It is often categorized as user acceptance testing (UAT), conducted by the end-users, or operational acceptance testing (OAT), which focuses on operational readiness.
Benefits of Acceptance Testing:
- Ensures that end-users are satisfied with the product
- Validates the software against business requirements
- Identifies discrepancies before the software goes live
Acceptance testing plays a vital role in confirming that the software functions as intended in real-world conditions. Often, any issues found during this phase can be critical for the success of the software product.
Maintenance and Evolution of Software
Software maintenance is a critical aspect in the lifecycle of any software system. It entails the necessary updates and modifications made after the software has been deployed. The importance of this topic cannot be overstated as it influences not just usability but also the longevity and reliability of the software. As technology evolves, the expectation from software systems also changes. Users demand enhanced features, security patches, and improved performance. Hence, maintenance and subsequent evolution are crucial to keeping software relevant and effective.
Types of Software Maintenance
There are several types of software maintenance that organizations must consider:
- Corrective Maintenance: This refers to fixing bugs discovered during the use of the software. It is often reactive and immediate, required to ensure that the software works as intended.
- Adaptive Maintenance: Over time, software may need updates to accommodate changes in the environment such as updates to operating systems, hardware changes, or even new business processes. This type of maintenance ensures that software can adapt to these changes without complete rewrites.
- Perfective Maintenance: This involves improving or enhancing existing features. Users may request new functionalities or better performance, leading to modifications that make the software more efficient and user-friendly.
- Preventive Maintenance: This type prevents potential issues before they happen. Regular updates and code reviews are included here, aiming to reduce future problems and extended downtime.
Effective maintenance strategies not only improve software performance but also prove essential in extending the software's life cycle.
Software Reliability
Software reliability pertains to the ability of the software to perform its intended functions consistently without failure. A reliable software product is crucial for maintaining user trust and satisfaction. Factors influencing software reliability include:
- Error Free Code: Ensuring that the base code is well-written and thoroughly tested before deployment contributes significantly to overall reliability.
- Regular Updates: Consistent updates, including patches for security vulnerabilities, enhance reliability by safeguarding against potential threats.
- User Feedback: Monitoring user interactions and applying suggestions can lead to improved performance and overall effectiveness of the software.
Achieving high reliability requires a proactive approach. It is far more beneficial to anticipate problems and act swiftly than to react after issues arise.
Refactoring
Refactoring is the process of restructuring existing computer code without changing its external behavior. This practice is essential for long-term software health. Benefits of refactoring include:
- Improved Code Quality: Regular refactoring helps enhance the readability and maintainability of the code. It makes the code easier for new developers to understand and modify.
- Easier Bug Fixing: A cleaner code structure reduces the chances of introducing new bugs when making changes, thus facilitating corrective maintenance.
- Increased Performance: By optimizing existing code, refactoring can lead to performance boosts, resulting in a more responsive application or system.
Refactoring should be an ongoing activity in software development. As the codebase grows, maintaining its quality through well-planned refactoring cycles becomes increasingly important.
Maintaining and evolving software is not optional; it is a requirement for the sustainability of software solutions in a dynamic environment.
Ethical Considerations in Software Engineering
In software engineering, ethical considerations are crucial. They serve as a framework for software engineers to navigate their responsibilities towards users, society, and the larger technological ecosystem. In this section, we will explore the importance of ethical considerations, diving into aspects that shape responsible engineering practices.
Developing software involves decision-making that can directly affect users’ lives. Be it through data privacy issues or software that impacts daily activities, engineers must ensure that their work adheres to high ethical standards. The incorporation of ethics in software development can lead to numerous benefits, such as increased user trust, compliance with regulations, and enhancement of professional reputation.
Professional Ethics
Professional ethics in software engineering concern the principles that guide behavior in a professional setting. This includes honesty, integrity, and transparency in work. When software engineers adhere to professional ethics, they ensure accuracy and reliability in their projects.
Some key considerations in professional ethics include:
- Confidentiality: Keeping user data and project details secure.
- Competence: Continuously updating skills to deliver quality work.
- Accountability: Taking responsibility for one’s work and its outcomes.
Inadequate attention to professional ethics can lead to software failures, security breaches, and loss of user trust. Thus, fostering an ethical culture within organizations is essential. This can involve ethical training, open discussions about ethical dilemmas, and, importantly, a clear set of guidelines.
Social Responsibility
Social responsibility is another vital ethical consideration in software engineering. It emphasizes the obligation of engineers to contribute positively to society and protect public interests. This goes beyond compliance with laws and regulations; it means actively thinking about how software affects users and society at large.
Key areas of focus for social responsibility include:
- User Well-being: Designing software that enhances quality of life and prioritizes user safety.
- Accessibility: Ensuring that software is usable by people of varying abilities.
- Sustainability: Developing solutions that consider environmental impacts.
As technology permeates every aspect of our lives, software engineers play a significant role in shaping culture and ethics within the tech community. They must recognize their influence and act with a commitment to the greater good.
"With great power comes great responsibility."
This quote is particularly relevant within software engineering. Engineers must recognize the potential impacts of their creations and take ethical considerations seriously.
In summary, addressing ethical considerations in software engineering is not just about compliance; it’s about creating a better future through responsible practices. Engineers must be proactive in integrating both professional ethics and social responsibility into their work to contribute positively to society and the technology landscape.
Future Trends in Software Engineering
The landscape of software engineering is constantly evolving. Keeping abreast of future trends is crucial for practitioners seeking to stay relevant. These trends not only shape development methodologies but also influence the tools and technologies utilized across the industry. Understanding emerging technologies and the impact of artificial intelligence and automation can significantly enhance a developer's skill set.
Emerging Technologies
Emerging technologies are integral to software engineering's progression. Familiarity with these technologies allows engineers to leverage new opportunities and adapt to evolving client needs. Notable trends include:
- Blockchain: This decentralized ledger technology offers transparent and secure transaction methods. Understanding blockchain enables developers to create applications in finance, supply chain, and healthcare.
- Internet of Things (IoT): The continued rise of IoT creates vast opportunities for software that connects devices. This requires knowledge of networking protocols and data processing strategies.
- 5G Technology: As 5G networks roll out, software engineering must account for improved speed and reliability in applications, enabling new services for users.
- Quantum Computing: Although still nascent, quantum computing promises to revolutionize problem-solving. Software developers will need to understand this paradigm shift as applications evolve.
AI and Automation in Software Development
Artificial Intelligence (AI) and automation are shaping the future of software development fundamentally. The benefits they bring are multifaceted, yet they also entail careful consideration of their implications.
AI facilitates smarter decision-making in software engineering. It can optimize code through recommendation systems and automated refactoring. Tools like GitHub Copilot utilize AI to aid developers in writing code, saving time and effort.
Automation enhances the efficiency of various development processes. Continuous integration and deployment, achieved through tools such as Jenkins or Travis CI, reduce manual workloads. This enables teams to focus on innovation rather than repetitive tasks.
However, reliance on AI and automation raises concerns. The fear of diminishing human oversight is a topic often debated. Balancing automation with human intelligence will determine the effectiveness of these technologies in practice.
"Embracing AI and automation while maintaining human insight will pave the way for future innovations in software development."