POI for Beginners: Mastering Plain Old Java Objects
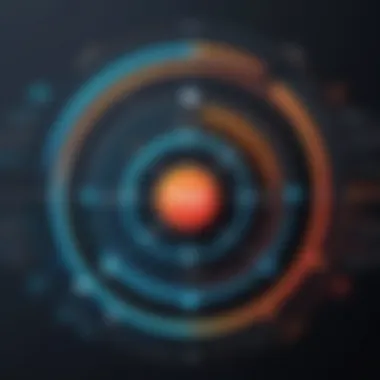
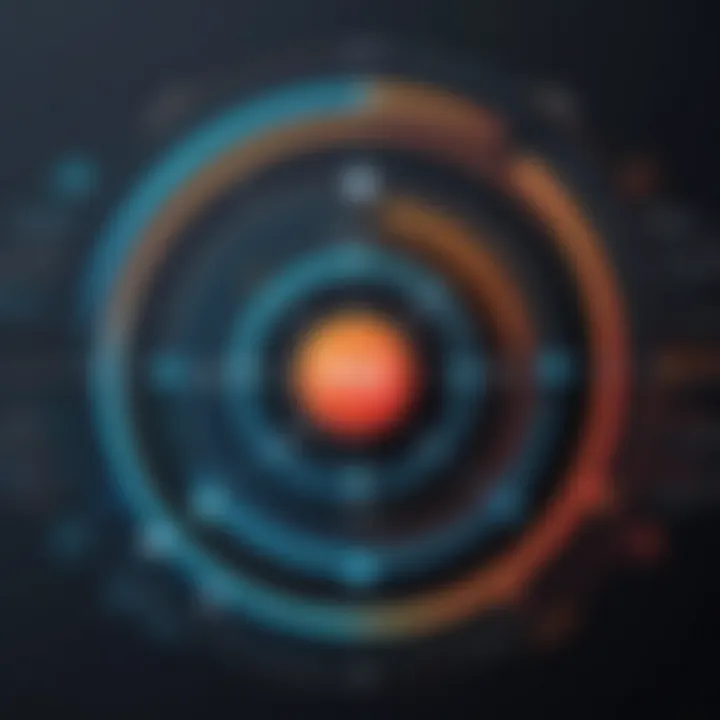
Intro
Understanding the fundamentals of programming languages can feel daunting at first, especially when navigating through concepts such as POI or Plain Old Java Objects. Learning to harness the power of POI can open up a world of opportunities in Java development by enhancing your programming proficiency.
History and Background
The concept of POI emerged within the Java ecosystem as a way to represent simple data structures. Its significance lies in the idea that objects encapsulate data and functionality. Knowing where it comes from enriches your comprehension of its use in Java applications.
POI was originally devised to facilitate easier manipulation of Java objects. Over the years, it evolved, becoming an essential aspect of Java programming. The power of POI is in its simplicity, emphasizing plain objects without any extravagant complexity which can at times cloud understanding.
Features and Uses
When we talk about features, we refer to the core characteristics that make POI practical in programming. Here are some of the main ones:
- Simplicity: POI favors straightforward structures, making it accessible for beginners.
- Flexibility: POI adapts well to various development needs, whether for building complex systems or simple applications.
- Interoperability: Being plain and standard, POI allows easy integration with other Java technologies.
POI is widely used in Java back-end development. You might find it in scenarios like data transfer objects, which help move data between software layers. These are just a couple of places where POI shines and aids developers in enhancing clarity and efficiency in their projects.
Popularity and Scope
In recent years, the trend in programming has shifted towards using objects to manage data more effectively. POI fits into this trend amazingly well. Its neatness and ease of use have drawn a crowd of developers, especially those new to the field. As more folks join the tech world, the demand for comprehensible tools like POI continues to rise.
"Simplicity is the ultimate sophistication." ā Leonardo da Vinci
Moreover, the popularity of Java as a programming language makes POI even more relevant. As Java continues to be pivotal in web development, mobile applications, and enterprise solutions, having a solid grasp of POI is beneficial for anyone looking to navigate the Java seas smoothly.
This comprehensive guide aims to take you from the basics to a more intricate understanding of POI, so you're not just another face in the crowd, but rather a well-informed and skillful practitioner ready to tackle your Java projects with confidence.
Preamble to POI
When diving into the realm of Java programming, one term often mentioned is POI, which stands for Plain Old Java Object. This concept is significant for both novices and seasoned developers, serving as a foundation for understanding how objects in Java can be structured and utilized. In modern programming, where frameworks and intricate architectures can sometimes muddle clarity, POI offers a breath of fresh air. It provides a straightforward approach to object-oriented programming by emphasizing simplicityāideal for those just entering the field.
The beauty of POI lies in its ease of use. By reducing complexities associated with more advanced concepts, beginners can wrap their heads around the core principles of Java quickly. This section will focus on unpacking the essentials of POI, including its definition and its substantial role in Java programming.
So, letās not beat around the bush. Understanding POI can also lead to improved code readability and maintainability. In todayās fast-paced tech environment, these qualities have become essential for building robust applications. By internalizing POI, developers can enhance their coding practices and subsequently streamline their project development phases.
What is POI?
At its core, POI refers to a design pattern that utilizes simple objects in Java. The phrase encapsulates the idea of creating Java objects that are not bound to any particular framework or complex programming constructs. Instead, POI leverages the Object-Oriented Programming principlesālike encapsulation and inheritanceāwhile maintaining a no-frills approach.
To better grasp what POI entails, consider this analogy: Think of POI as a blank canvas. Just as an artist can approach a blank canvas without constraints, a programmer can approach POI without the weight of additional frameworks. This freedom allows for creativity and flexibility in coding practices.
This kind of object-oriented programming facilitates easier troubleshooting and modification. When you work with a Plain Old Java Object, youāre generally dealing with straightforward instances and methods. This simplicity permits both experienced coders and newcomers to collaborate more effectively, bridging the knowledge gap that can hinder project development.
Importance of POI in Java Programming
The significance of POI in Java programming extends beyond its basic functionality. One of the primary benefits of using POI is its interoperability with numerous Java frameworks and libraries. Unlike more entangled object models, POI allows developers to seamlessly integrate different technologies without the usual overhead. This is paramount in today's development ecosystem, where interoperability is the name of the game.
Moreover, using POI sets a clear path toward best practices in software design. By adopting POI, developers often find themselves adhering to more standardized coding rules. This not only promotes better organization within code but also enhances team collaboration.
"Embracing POI can significantly streamline both development and maintenance phases of a project, allowing for easier adjustments in the long run."
In addition, with POI, the learning curve is minimized. For those who are just starting, understanding how to manipulate POI can lead to a quicker grasp of programming fundamentals. When programmatic intricacies are stripped down, itās much easier to grasp how Java works. Less clutter means clearer thinking.
All things considered, POI serves as a launchpad for both individual and organizational success in Java programming. Its straightforward approach not only benefits the early coders but similarly provides value to experienced developers looking to maintain clarity in their projects.
The Core Concepts of POI
Understanding the core concepts of POI is not just a matter of familiarity, but rather a gateway to effectively utilizing Plain Old Java Objects in a variety of applications. In the rapidly evolving world of Java programming, knowing how to leverage these concepts can yield significant benefitsābe it in efficient coding, better performance, or ease of integration with other Java frameworks.
Understanding Plain Old Java Objects
Plain Old Java Objects, abbreviated as POJOs, are simple objects that do not adhere to any special restriction other than those forced by the Java Language Specification. They are uncomplicated data structures, mainly used to represent data in a well-defined way. This simplicity is appealing because it enables clear separation between data and behavior, allowing developers to easily create and manipulate objects without worrying about additional complexities often associated with Java frameworks.
For instance, letās say you are developing an application to manage book information. A POJO might look something like this:
This structure provides clarity and precision in data handling, something that is particularly critical when building larger applications.
Differences Between POI and Other Java Objects
At a glance, POJOs might seem like just another Java class; however, they are different from other Java objects primarily in terms of their design philosophy. Unlike JavaBeans or Enterprise JavaBeans which have additional requirements, such as implementing specific interfaces or following particular naming conventions, POJOs embrace a straightforward model. No technical wizardry is neededāitās just plain old Java.
Consider this comparison:
- Complexity: POJOs are simple and require no special behavior, while other object types might enforce specific behaviors (like lifecycle methods).
- Framework Dependency: POJOs do not rely on frameworks, whereas many Java objects might be tightly coupled with an underlying framework, which can restrict usage.
- Serialization: Some Java object forms mandate serialization, while POJOs can be made serializable if desired, without that being an inherent requirement.
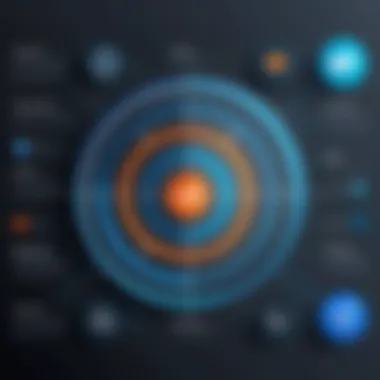
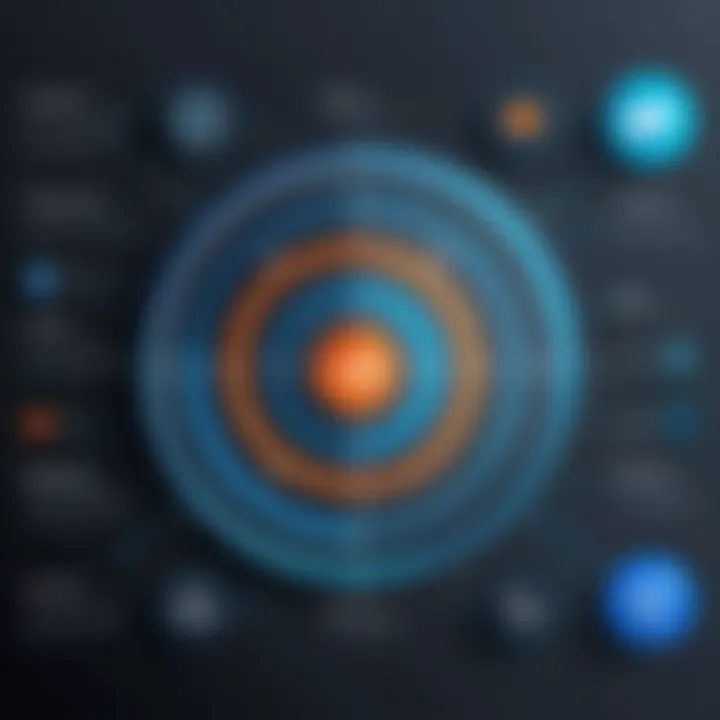
This ease of use makes POJOs particularly advantageous in various programming scenarios, especially when rapid application development is needed without the overhead of complex configurations.
POI Annotations and Their Importance
Annotations in Java provide a way to add metadata information to your code. In the context of POI, annotations can greatly enhance how Java objects interact within a system. They offer a way to provide instructions or enable features without modifying the underlying codebase significantly.
For example, with Java, you might use the annotation to mark a class as representing a table in the database, making it effortless for frameworks to recognize and handle that class appropriately. This not only streamlines the coding process but improves code readability.
Some key points about POI annotations include:
- Configuration: Annotations allow you to configure object behavior on the fly, making application maintenance smoother over time.
- Clarity: They provide self-documenting capability, meaning other developers can easily understand how to work with your objects just by looking at the annotations.
- Framework Collaboration: Annotations are widely used in various Java frameworks like Spring and Hibernate, which means adopting POI with annotations can ease the integration process.
"Understanding POI annotations opens a door to a more declarative style of programming, where intent can be inferred and learned from code without extensive documentation."
Setting Up Your Environment for POI
Setting up your environment for working with POI is crucial to ensure a smooth coding journey. Think of it as prepping your kitchen before cooking a delicious meal. If the tools and ingredients are in disarray, confusion and frustration could spoil the entire dish. In the world of programming, having the right software, tools, and libraries ready at hand makes the difference between an enjoyable experience and stumbling upon countless errors and roadblocks.
With POI, the initial setup not only allows for coding but also enhances understanding of how the objects interact within the Java framework. This foundational step equips you with the necessary tools and knowledge to explore and implement POI in various projects effectively. It genuinely sets the stage for any beginner looking to delve deeper into Java programming.
Required Software and Tools
To embark on this journey with POI, you need a few essential software tools at your disposal:
- Java Development Kit (JDK): The JDK is like the magic wand for any Java developer. Make sure you download the latest version to enjoy all its features. Itās available from Oracle's official site or you can check out OpenJDK for an open-source version.
- Apache POI Library: Of course, youāll need the POI library itself. You can fetch it either via a direct download from the Apache POI website or manage it with popular build tools like Maven or Gradle.
- Integrated Development Environment (IDE): An IDE makes coding a lot easier. Popular choices include IntelliJ IDEA, Eclipse, or NetBeans. Each of these has its strengths, but ultimately, it's about what feels most comfortable for you.
This combination lays the groundwork necessary for a successful POI experience. Ensure your version numbers are compatible for the best performance.
Installing POI Libraries in Java
Once you have the necessary tools in place, itās time to dive into installing the Apache POI libraries. The beauty of utilizing popular build systems makes this much easier.
- Using Maven:
- Using Gradle:
- Direct Download:
- If youāre working with Maven, add the following dependency to your :
- This ensures that all necessary files are downloaded automatically.
- If Gradle is your game, simply add the following lines to your file:
- After saving changes, run the command , and everything you need will be fetched.
- Alternatively, download the files straight from the Apache POI website and include them in your project's build path. This involves a bit more legwork but is valid for anyone not keen on using Maven or Gradle.
"Taking the time to ensure your environment is set up correctly is an investment for future success in your programming endeavors."
Walking through this setup process will familiarize you with the environment and prepare you well for the programming challenges ahead. A solid foundation ensures youāll spend less time troubleshooting and more time innovating.
Creating Your First POI Application
Creating your first POI application marks a significant milestone in your journey with Java and POI. Itās not merely about writing code; it's about understanding how to translate theoretical concepts into practical applications. With Java being a dominant programming language, blending it with POI for creating efficient applications assists in solidifying your grasp of the language itself. This segment sheds light on essential elements concerning the project structure and basic features you will want to implement, thus aligning theory with practice.
Setting Up the Project Structure
The backbone of any successful application is a well-organized project structure. It sets the stage for clarity and maintenance down the line. When creating your first POI application, consider the following key components:
- Source Folders: Have a dedicated folder for your Java source files. This helps keep your code organized.
- Libraries: Ensure you clearly define a place for external libraries, particularly the POI libraries. This is where you will add any jars necessary for functionalities.
- Resources: If your application requires other resources, such as images or configuration files, create a resources directory to store them.
Hereās a simple structure:
Having your project structure laid out is like having a map before venturing into uncharted territory. It enables you to navigate your development phase smoothly and aids in collaboration if you're working with others.
Implementing Basic POI Features
Once your project structure is in place, the next step is to begin implementing basic POI features. This step is vital because it allows you to interact with Plain Old Java Objects effectively. Hereās a breakdown:
- Creating a POI Object: Start by defining your class. In a real-world scenario, this might represent a user or a product.
- Initializing POI Features: Once you have your POI class set up, you can start using libraries like Apache POI to read and write Excel files. For this, include the necessary imports and write methods that will utilize these libraries effectively.
- Writing Simple Methods: Add methods to save or manipulate data within your application. It could be inserting a new product into an Excel sheet or retrieving one. This will empower your application, making it functional.
Implementing these features engages you with the syntax and operations of POI, giving practical exposure. As you get the hang of these fundamental elements, youāll find youāre increasingly confident not just in applying POI principles but also in their relevance to constructing effective Java applications.
Remember: Successful applications are built piece by piece. Each feature you implement adds to your understanding and sets a foundation for more complex functionalities.
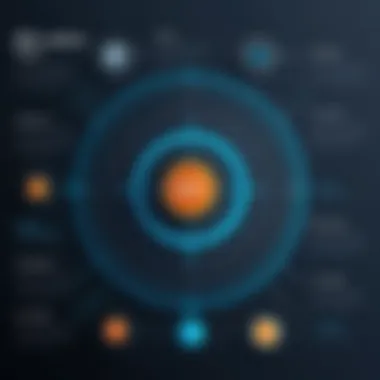
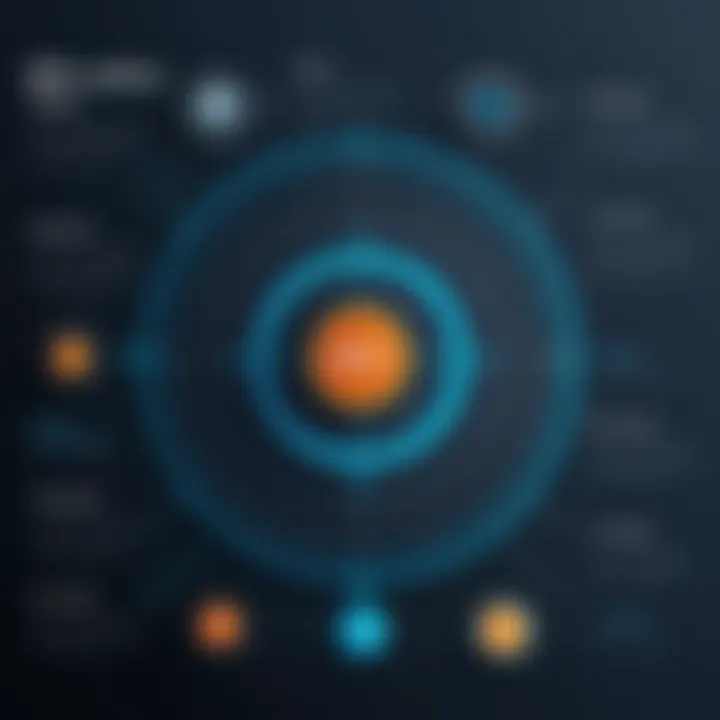
A strong start in your first POI application can pave the way for advanced capabilities later. Take your time, experiment, and let the code guide you.
Advanced Features of POI
Navigating through the realm of Plain Old Java Objects is much like venturing into a deep forest; the basics can get you started, but to grasp the true essence, one must explore the deeper paths. Advanced features of POI allow developers to harness the full potential of these objects. They bring efficiency, clarity, and robustness to Java applications. The importance of mastering these advanced aspects cannot be understated, as they significantly enhance the application's performance and maintainability.
Working with POI Collections
Collections in POI serve a critical function as they help manage groups of objects with ease. Imagine trying to manage a dozen kitchen utensils scattered all over the place without any organization. Using collections is like having a well-structured drawer where everything has its dedicated space.
- Efficiency: Utilizing collections means you can easily store and manipulate large sets of data, limiting redundancy and maximizing efficiency in your code.
- Flexibility: Collections offer various interfaces, such as List, Set, and Map, allowing developers to choose the most suitable according to their needs.
- Integration: POI Collections can beautifully integrate with other Java frameworks. For example, the ease of converting a collection into a Json object makes it very useful when working with web applications.
Implementing collections might seem daunting for newcomers, but understanding the basics can act as an anchor when delving deeper. Hereās a simple example of how to create a list in POI:
By leveraging collections, developers can devise solutions more adeptly and cleanly.
Implementing POI with Annotations
Annotations in Java act like little flags that provide metadata about the program elements they annotate. With POI, they become a magnet for succinctness and clarity.
- Clarity: Annotations improve code readability by reducing boilerplate code, which can sometimes resemble a tangled ball of yarn. By simply adding an annotation, you clarify the intent of the code without needing verbose explanations scattered through the codebase.
- Configurability: POI leverages annotations to allow configuration directly in the code. This means fewer XML files and more maintainable code structures.
- Framework Compatibility: Many popular Java frameworks recognize POI annotations, fostering a diverse ecosystem where developers can easily switch between libraries without facing a steep learning curve.
To illustrate, hereās how a simple annotation can be declared:
This example shows how annotations can encapsulate metadata about fields while keeping the code clean. Using annotations is not only time-saving but also a genuine nudge towards effective programming practices.
Mastering advanced features of POI, including collections and annotations, can dramatically elevate your Java development journey. They reduce complexity while increasing efficiency.
Handling Errors in POI Applications
Errors in programming are like potholes on the smoothest of roads; they can disrupt your journey and slow you down, but knowing how to navigate them can lead to a seamless traveling experience. When diving into Plain Old Java Objects (POI), understanding how to handle errors effectively becomes crucial. This section sheds light on common issues that may arise while working with POI and viable debugging techniques, which in turn will bolster your development skills and confidence.
Common POI Issues and Debugging Techniques
When youāre knee-deep in code, unexpected errors can creep up on you like an uninvited guest. Here are some prevalent issues you might encounter while working with POI:
- Incorrectly configured dependencies: Ensure your libraries are properly linked. Often, importing the wrong version can lead to runtime exceptions.
- Mismatch between POI versions and Java versions: Using an incompatible Java version with POI can result in various headaches, especially when you start facing class not found errors.
- Data format errors: This is a common pitfall, particularly when dealing with excel files. If the data format in the sheet doesn't match what your code is expecting, you'll quickly hit a wall.
To debug these hiccups effectively:
- Utilize logging: Incorporate logging frameworks like SLF4J or Log4J. They help trace back the errors by allowing you to see what your code was doing when things went awry.
- Step through your code: Use debugging tools built into IDEs such as Eclipse or IntelliJ. This can provide insights into variable states and where your code might fail.
- Rely on stack traces: When something fails, Java gives you a stack trace that provides invaluable information about what went wrong. Donāt ignore it; itās your best friend in troubleshooting!
A bit of resilience goes a long way in resolving coding errors, and remembering that every issue solved is a step toward mastery can motivate you through your challenges.
Best Practices for Error Handling
Navigating through your first POI application teaches many lessons, not just about coding, but about building resilience. Following some best practices will better prepare you for the challenges ahead:
- Anticipate potential errors: Always code with the mindset that errors will happen. Using blocks allows your application to handle unexpected situations gracefully. For example:
- Provide clear error messages: Make sure your application communicates what went wrong. This helps not just you, but others who may work with your code in the future.
- Log, log, log: Keep records of what went wrong. Use a consistent logging framework to audit errors and fix recurring issues.
Incorporating these strategies can save you time and frustration down the line, keeping your development process fluid.
Understanding not just what to do but how to do it well will give you a solid ground on which to build your POI skills. This will not only enhance your ability to write effective Java applications but also prepare you for the inevitable bugs that come along for the ride.
Integrating POI with Other Java Frameworks
Integrating POI with other Java frameworks can really elevate your programming endeavors. The interplay between these frameworks can enhance data management, provide robust functionalities, and create more streamlined pathways for developing applications. Particularly, combining POI with established frameworks like Spring and Hibernate brings forth several benefits, such as increased efficiency in handling data, easier integration of relational databases, and leveraging dependency injection for cleaner code. In this section, we delve deeply into how these integrations work and why they matter.
Using POI with Spring Framework
The Spring Framework is a juggernaut in the world of Java development. By integrating POI with Spring, developers can take advantage of the framework's powerful features while handling Excel files effortlessly. Imagine a scenario where a business needs to pull reports from an Excel sheet and display or manipulate that data in real-time web applications. Integrating POI with Spring resolves this issue smoothly.
- Dependency Management: With Spring, managing dependencies is as straightforward as pie. You can include the POI dependencies in your if you are using Maven. Here's a snippet showing what it might look like:
- Seamless Data Handling: Once you have the dependencies sorted, you can easily read and write Excel files using POI's API inside your Spring services. For instance, you can use POI to read data from an Excel file, process it, and then save it to your database using Spring's repository features.
- Spring Boot Harmony: If you're working with Spring Boot, integrating POI becomes even more streamlined. You can take advantage of Spring Boot's autoconfiguration, making your application startup quicker and easier. Just throw in a Controller that handles file upload and processing, and youāre all set!
This integration not only increases productivity but also allows for better maintainability of code. Combining these two powerful tools means your development process can flow like a well-oiled machine.
Combining POI with Hibernate
Hibernate, the popular ORM tool for Java, can really work wonders when paired with POI. This combination is vital when you're dealing with large datasets that need to be read from Excel files and persisted into a relational database. Letās explore some aspects of this integration.
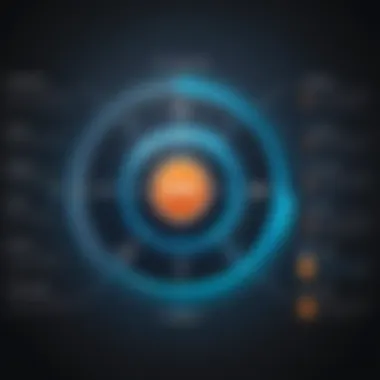
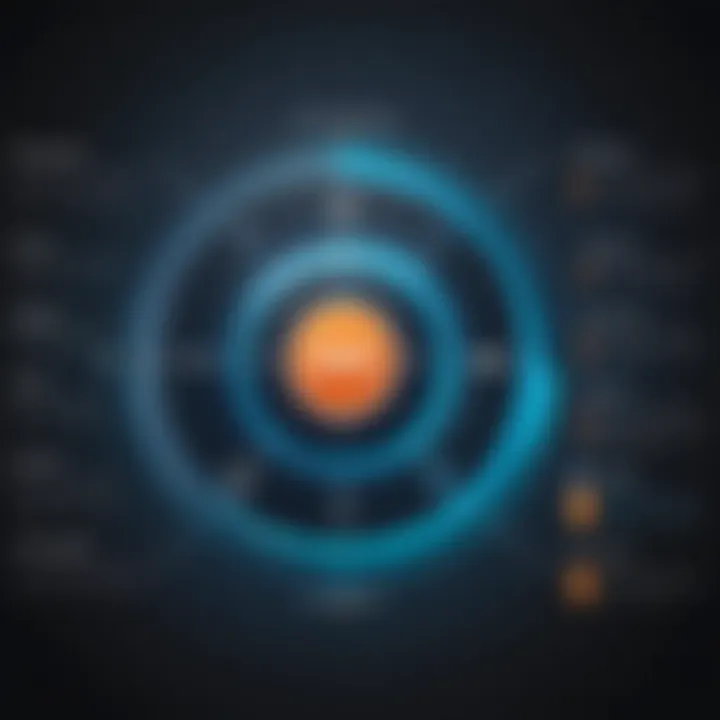
- Loading and Storing Data: When reading data from Excel sheets, you can parse the sheets using POI and then transform these records into Hibernate entities before persisting them to your database. This approach promotes clean separation of concerns and utilizes Hibernate's powerful session management.
- Efficient Querying: Once your data is in the database, Hibernateās querying capabilities come into play. You can execute complex queries against your Excel-imported data with ease. This allows for better data manipulation and retrieval methods that suit your application's needs.
- Transaction Management: Using Hibernate alongside POI means you can wrap the entire read-write cycle in a single transaction. If anything goes awry while inserting data into the database, you can roll back changes easily, saving your application from inconsistent states.
- Performance Optimization: With Hibernate's session caching and batching features, handling bulk data from Excel files becomes more efficient. This results in optimal performance when dealing with large scale applications, allowing for improved user experience.
Integrating POI with other frameworks like Spring and Hibernate not only streamlines your data handling but also enriches the overall architecture of your applications.
Testing and Maintaining Your POI Applications
Testing and maintaining your POI applications is vital for developing robust and efficient Java programs. A well-tested application not only meets functional requirements but also stands strong against unforeseen issues. The importance of this section lies in ensuring the applications remain reliable and effective even as changes are made. As developers, we must embrace a proactive approach to testing and maintenance to safeguard the integrity of our software.
Regular testing can catch bugs early on, making it easier to solve issues before they escalate. Moreover, it enhances code quality, leading to smoother user experiences. Utilizing effective testing methods lays a foundation for reliability, providing end-users with confidence in the applicationās performance.
From unit tests to integration tests, being comprehensive in testing ensures that every piece of the application works harmoniously together. Furthermore, maintaining POI applications is not just a one-time task. It requires ongoing effort to keep up with evolving technologies and user needs, ensuring applications continue to deliver value.
Unit Testing POI Applications
Unit testing is the bedrock of a solid testing strategy. It involves isolating individual components of your application and testing them independently to ensure they perform as required. When working with POI, unit tests can verify that features related to data processing, handling files, or managing annotations function correctly in isolation.
Hereās why unit testing is essential:
- Early Detection of Bugs: Bugs are easier to fix when they are found early in development. Unit tests allow for immediate feedback.
- Code Refactoring Made Easier: With a solid set of unit tests in place, developers can refactor code with confidence, knowing that existing functionality is safeguarded.
- Documentation: Unit tests serve as a form of documentation. They illustrate how parts of the application are expected to function, which is helpful for new team members.
To implement unit testing in your POI applications, consider using frameworks like JUnit or TestNG. Hereās a basic example of a unit test for a POI-related function:
Executing these unit tests regularly is crucial to maintaining a healthy codebase. As new features are added or existing features are modified, keep running tests to confirm everything remains in working order.
Versioning and Maintenance Strategies
Versioning and maintenance strategies are fundamental to the longevity of your POI applications. As the application grows, so do its dependencies, features, and complexity. Using version control systems like Git, you can track changes, collaborate with other developers, and revert to previous versions when necessary.
Here are some strategies for effective versioning and maintenance:
- Adopt Semantic Versioning: This practice involves assigning version numbers based on feature additions and breaking changes. It helps communicate to users the impact of updates.
- Document Changes: Maintain a changelog that records everything from bug fixes to new features. This not only aids in transparency but also assists in diagnosing issues which may arise due to updates.
- Regularly Review Dependencies: As libraries and frameworks evolve, itās essential to keep track of updates. Outdated dependencies can lead to security vulnerabilities and compatibility issues.
- Use Continuous Integration/Continuous Deployment (CI/CD): Implement CI/CD practices to automate testing and deployment, reducing manual intervention and the risk of human error.
Maintaining your application requires diligence and constant engagement with best practices. By combining effective unit testing and intentional versioning, developers ensure that their POI applications remain functional, secure, and adaptive to changing user needs.
"Keeping your code clean and well-tested not only saves time in the long run but makes your development experience far more enjoyable."
Implementing these practices will pay off, especially as your application and user base grow, allowing you to focus on innovation rather than firefighting issues.
Real-World Applications of POI
When it comes to programming, theory is crucial, but without practical application, it often feels hollow. That's where POI truly shines. Understanding the real-world applications of Plain Old Java Objects can help you grasp why they are so vital in developing robust Java applications. By leveraging POI, developers can create intuitive, efficient systems that align with user expectations and business goals.
POI provides a clean, simple model for data manipulation. It sits at the intersection of ease of use and powerful functionality. Whether it's for enhancing mobile apps or powering backend systems, POI has diverse applications that resonate across various industries. Here are some specific elements to consider:
- Flexibility: POI can easily adapt to multiple use cases, from basic CRUD operations (Create, Read, Update, Delete) to more complex interactions. This flexibility is important for businesses looking to maintain agility in their digital solutions.
- Maintainability: Using POI, developers often create code that is easy to maintain. A clean POI implementation helps prevent technical debt, allowing more adjustments over time without the headache of infrastructure overhauls.
- Integration: POI can seamlessly integrate with various Java frameworks, enhancing its utility in broader software ecosystems. This integration capability simplifies accessing data across different platforms.
In summary, understanding the real-world applications of POI not only broadens horizons but also equips developers with the tools they need to tackle real challenges. Letās break it down further by looking at specific case studies and practical implementations.
Case Study: POI in Business Applications
Businesses today heavily rely on well-structured data to drive decisions and processes. Integrating POI into enterprise applications enables organizations to manage data effectively. A notable example is a financial institution using POI to handle customer data. By utilizing POI's features, they managed to:
- Streamline data entry: With POI, they could create a user-friendly interface for entering complex financial details. This saved time and energy for both employees and customers.
- Maintain accuracy: The validation features in POI helped ensure that data entered was both valid and consistent, reducing errors that could occur with manual entry.
- Efficient reporting: Using POI, the reporting process was automated, allowing reports to be generated quickly. This brought down the turnaround time for daily reports significantly.
- Data analysis: By transforming the raw data into actionable insights through POIās capabilities, better business strategies emerged, ultimately enhancing profitability.
In short, this case study illustrates just how vital POI can be in optimizing business processes. The clear benefits coupled with adaptability make POI an asset in any business-centric application environment.
POI in Web Development
The web has transformed how applications function, and POI plays a pivotal role here too. In the domain of web development, POI provides a foundation for creating RESTful APIs that can serve dynamic content to websites. Hereās how:
- Data Handling: POI effectively manages the state of objects exchanged between the client and server. This guarantees that data can be sent across networks securely and quickly, which is paramount in the fast-paced world of web applications.
- Scalability: As web applications grow, the need for scalable solutions pops up. POIās design allows developers to build applications that can expand seamlesslyāsomething critical for handling increasing user loads.
- Framework Integration: POI seamlessly works with front-end frameworks like Angular or React. This ensures that the data flow within the application remains smooth, providing a better user experience.
- Support for JSON: Many web applications rely on JSON for data interchange. POI can easily integrate with serialization libraries that convert POI objects into JSON, facilitating communication between server and client.
By leveraging POI in web development, developers can create more responsive, data-driven applications that truly enhance user experience. As organizations shift towards online platforms, the relevance of POI grows ever more significant.
End and Further Resources
As we wrap up this guide, it's essential to understand the significance of consolidating the knowledge you've gathered on POI. Itās not merely about learning a handful of concepts but rather weaving those threads into a robust understanding. When you look back at what you've absorbed, it serves not just as a refresher but as a springboard for the next steps in your programming journey. This section is not the end; it's more like a pivot that could lead to countless avenues for exploration, whether you're diving deeper into POI or branching out into related Java frameworks.
Recap of Key POI Concepts
In the context of this journey through POI, emphasizing key concepts becomes crucial. Hereās a brief recap of the pivotal points you should keep in mind:
- Plain Old Java Objects (POJOs): At the heart of POI lies the idea of simplicity ā leveraging POJOs allows developers to create models that mirror data without adding unnecessary complexity. Itās straightforward but powerful.
- Annotations: You learned how annotations help in metadata configuration, essentially guiding how POJOs behave under various scenarios, benefiting both performance and readability.
- Interoperability: One of the significant advantages highlighted is how POI jives seamlessly with Java frameworks like Spring and Hibernate, creating more versatile applications. This harmony means youāre not working in isolation; your tools work together beautifully.
Reflecting on these elements can reinforce your understanding and give you a clearer path to improving your projects and skills.
Recommended Reading and Tutorials
To further your mastery of POI and related areas, diving into curated reading materials and tutorials can be a gamechanger. Here are a few suggestions:
- Books: Look for resources like "Java Persistence with Hibernate" or "Spring in Action". These texts provide comprehensive insights into how POI fits within the broader Java ecosystem.
- Online Tutorials: Websites like Tutorialspoint and Baeldung offer step-by-step guides that break down complex topics into bite-sized pieces.
- Forums and Communities: Engage with fellow learners and experienced developers on platforms like Reddit or Stack Overflow. These spaces can be invaluable for getting answers to specific questions and exchanging ideas.
- Video Tutorials: Consider platforms like YouTube where many creators provide free content. Channels that focus on Java programming can be particularly useful for visual learners.
Remember, honing your skills is a continuous process. Whether you are cracking open a book, following a video, or simply participating in a discussion, each action adds another layer to your expertise.