Mastering PHP Database Connections for Developers
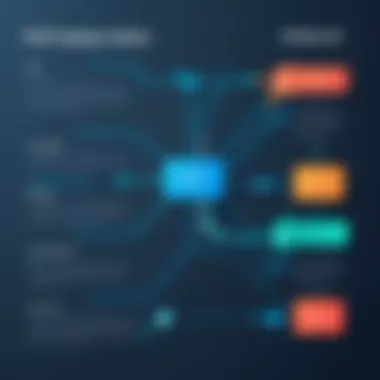
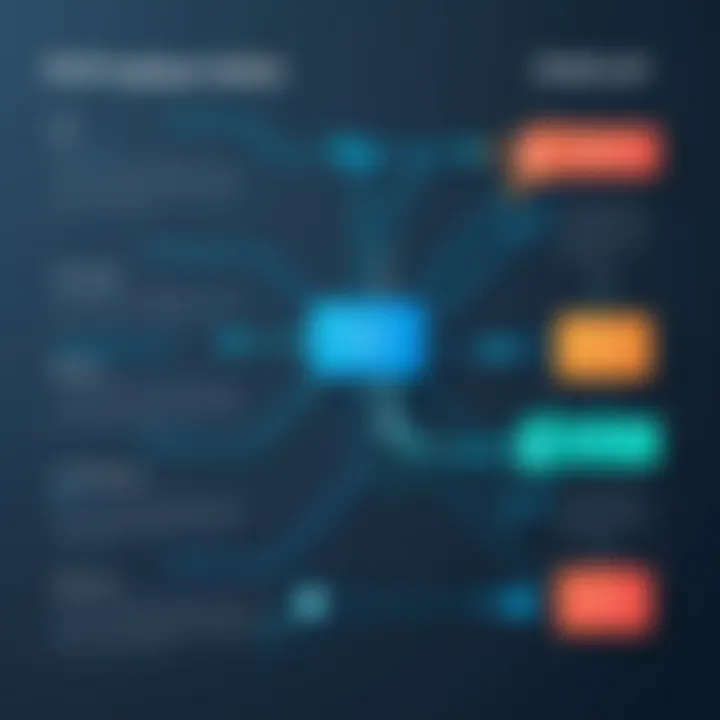
Intro
In today's digital landscape, database connections play a pivotal role in web development, particularly when utilizing programming languages like PHP. PHP, originally created in 1994 by Rasmus Lerdorf, has evolved into one of the most popular server-side scripting languages. Its versatility and deep integration with databases make it a preferred choice among developers for building dynamic websites and applications.
Understanding how to establish and manage connections with databases is crucial for effective data handling. This article aims to demystify PHP database interactions, starting from essential concepts and progressing towards complex implementations, ensuring a comprehensive grasp of procedures.
Following this introduction, we will cover vital topics, including the history of PHP, its key features, and ultimately, how it interacts with various database systems. Furthermore, we will delve into practical coding examples and troubleshooting techniques that facilitate the process of establishing connections securely and efficiently.
Preface to PHP Database Connections
In the realm of web development, the ability to interact with databases is central to creating dynamic applications. This necessity is particularly evident in projects where data storage, modification, and retrieval are essential. PHP, as a prevalent server-side scripting language, facilitates these interactions seamlessly. The process of establishing connections to a database is foundational for any PHP developer.
Understanding how to connect PHP with databases is not merely a technical requirement; it involves unraveling how the two elements integrate to produce functional applications. A correct connection lays the groundwork for performing tasks such as querying for information, executing transactions, and maintaining user data effectively.
Importance of Database Connections
Database connections serve several key purposes:
- Data Integrity: A successful connection ensures that the data can be accurately inserted, updated, or deleted without any loss.
- Performance: Optimized connections can boost the performance of applications. Reducing the load time in accessing databases enhances user experience.
- Security: Understanding how connections work helps in implementing security measures against common vulnerabilities, such as SQL injections.
- Scalability: Knowing how to manage database connections effectively allows applications to scale better, addressing increased load and user requests efficiently.
Establishing PHP database connections is just the beginning. This article will explore various connection methods, dive into basic and advanced coding practices, and offer insights into common pitfalls to avoid. With practical knowledge, readers can capitalize on the benefits that proper database connection management provides.
"Understanding how to establish and manage database connections is crucial for developers looking to create robust applications."
The process extends beyond just functional connections; it engages with error handling, executing queries, and ensuring security best practices. These facets are critical to a robust development process and will be addressed throughout this guide.
As we delve into the upcoming sections, readers will gain a rich understanding of PHP database connections, enhancing their ability to work proficiently in dynamic web environments.
Understanding Databases
In the realm of PHP and web development, a solid grasp of databases is fundamental. Databases serve as the backbone for storing, retrieving, and managing data efficiently. They are critical not just for dynamic website functionality but also for ensuring data integrity and security. Understanding the different types of databases and their respective management systems is vital for developers. This knowledge facilitates informed decisions that can lead to optimized performance and security.
Types of Databases
Databases can be classified into several categories, each serving distinct needs and use cases. Here, we will discuss three primary types of databases that are noteworthy for PHP developers.
Relational Databases
Relational databases, such as MySQL, operate based on a structured query language. They organize data into tables, which consist of rows and columns. The key characteristic of relational databases is their use of relationships between tables to link relevant data. This design allows for complex queries and efficient data management. Their benefits include data integrity and the enforcement of data types and constraints. However, they require careful schema design, which can be a disadvantage for rapid development scenarios where flexibility is essential.
NoSQL Databases
NoSQL databases, on the other hand, offer a different approach. They are not restricted to a fixed schema, allowing for greater flexibility in data storage. This characteristic makes NoSQL databases particularly beneficial for projects dealing with large volumes of unstructured or semi-structured data. Common examples include MongoDB and CouchDB. The unique feature of NoSQL databases lies in their ability to scale horizontally, making them ideal for applications needing quick data access and high availability. However, they may lack some of the transactional safety features inherent in relational databases, which could be a crucial consideration for certain applications.
In-memory Databases
In-memory databases keep data in the main memory rather than on disk, providing faster data retrieval and processing. This is a significant advantage when high performance and low latency are required. Systems like Redis and Memcached highlight this capability. The main characteristic is their ability to handle large volumes of transactions in real time, which is critical for applications like financial services. Nevertheless, the trade-off is that data persistence may be compromised if the database is not configured correctly, as data might be lost during a failure.
Database Management Systems
Database Management Systems or DBMS are software that interact with databases to handle data. Choosing the right DBMS is crucial for integrating it with PHP effectively. It impacts both performance and usability.
MySQL
MySQL is an open-source relational database system widely used for web applications. Its efficiency and reliability contribute to its popularity. The primary characteristic of MySQL is its robustness in handling concurrent requests and large datasets. A significant advantage of MySQL is its extensive documentation and supportive community, making it accessible for learners. However, advanced features may be lacking when compared to other commercial offerings.
PostgreSQL
PostgreSQL is another relational database system, known for its advanced features and compliance with SQL standards. It supports complex queries and various data types, which makes it versatile for different applications. PostgreSQL shines in scenarios where data integrity and complex relationships are crucial. Its strong feature set can be a double-edged sword, as the learning curve may be steeper for new users compared to more straightforward systems like MySQL.
SQLite
SQLite is a lightweight, self-contained database system. Its autonomy means it doesn't require a server setup, making it an ideal choice for smaller applications or development environments. The key characteristic of SQLite is its ease of installation and use, along with its minimal setup overhead. However, its limitations in concurrent access can hinder performance for larger applications. This duality makes it a popular choice for development but perhaps less so for production scenarios.
Connecting to a Database using PHP
Connecting to a database using PHP is a critical step in web development. A connection allows PHP scripts to interact with databases, an integral component of data-driven applications. The ability to fetch, insert, update, and delete data forms the backbone of dynamic web applications. This aspect emphasizes the synergy between PHP and databases, facilitating the creation of responsive applications that cater to user needs.
When engaging with databases through PHP, developers face distinct options, primarily MySQLi and PDO. Each option brings unique features, catering to different coding styles and requirements. Understanding these differences is crucial for making informed decisions about which method to utilize.
Adopting the right connection method influences not only code maintainability but also security and performance. This article will delve deeper into MySQLi and PDO, exploring their specific characteristics and benefits. Following this, developers can evaluate their specific needs and choose a method aligning with their project goals.
Using MySQLi
MySQLi, or MySQL Improved, is a database driver designed specifically for MySQL databases. It presents two styles of use: procedural and object-oriented. Developers often favor it for its ease of use and powerful features.
Procedural Style
The procedural style of MySQLi is straightforward, especially for beginners. This approach emphasizes a sequence of function calls, which many find intuitive. A key characteristic of the procedural style is its simplicity; developers can grasp it quickly and see results almost immediately. Using the procedural style promotes rapid application development.
However, with simplicity comes limitations. The procedural style may lead to less organized code, especially in larger applications. This can affect maintainability, making it harder to scale applications effectively in the long run. Despite this, many developers appreciate the ease of integration with existing procedural code, making it a popular choice for small projects.
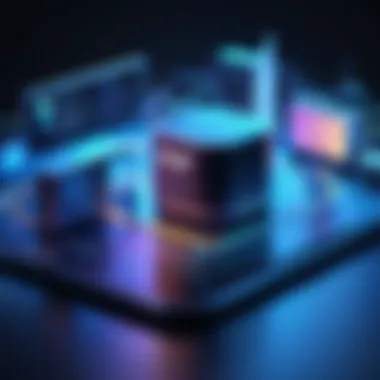
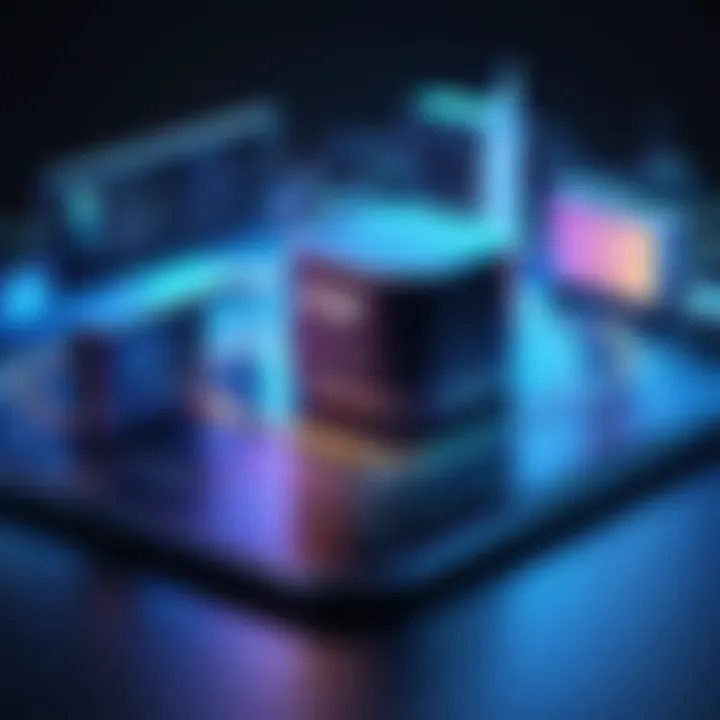
OOP Style
The object-oriented style of MySQLi incorporates principles such as encapsulation and inheritance. By using classes and objects, this approach makes code more structured and reusable. A primary advantage of the OOP style is its ability to manage complex applications more efficiently. This attribute fosters collaboration among developers, enabling multiple team members to work on the same codebase seamlessly.
One unique feature of the OOP style is the implementation of prepared statements, which strengthen security by preventing SQL injection attacks. Despite its benefits, the OOP style may present a steeper learning curve for those new to object-oriented programming. Nevertheless, for developers seeking to build scalable applications, the OOP style proves beneficial.
Using PDO
PDO, or PHP Data Objects, is a database access layer providing a more flexible and robust method for database interactions. PDO supports multiple database types, making it versatile.
Benefits of PDO
One of the standout benefits of PDO is its portability across different database systems. Once developers master PDO, they can switch databases with minimal code changes, enhancing flexibility. This characteristic makes it a preferred choice for developers aiming for future-proof applications.
Another advantage of using PDO is its support for prepared statements, which not only improves security but also enhances application performance. This adaptability attracts many developers shifting towards database abstraction layers that optimize development.
Despite its many advantages, some developers may find PDO requires a more complex setup compared to MySQLi. However, the long-term benefits often outweigh initial challenges.
Basic Syntax
The basic syntax for PDO is relatively straightforward, making it accessible for new developers. Establishing a connection typically involves creating a new instance of the PDO class. Hereโs how that may look in code:
This snippet provides a clear example of a basic PDO connection with error handling. The ease of modifying the connection parameters demonstrates the flexibility of PDO.
The choice between MySQLi and PDO will largely depend on the project requirements and personal preference. Each offers benefits and drawbacks that developers must weigh carefully. The goal remains to create secure, maintainable, and efficient applications.
Basic Connection Syntax
The Basic Connection Syntax is a critical element in establishing a functional interaction between PHP scripts and a database. It serves as the foundation for successfully initiating database connections, executing queries, and efficiently handling data. Mastery of this syntax enhances one's ability to work with PHP and databases, minimizing errors and unnecessary complications during application development.
Establishing a Connection
To establish a connection to a database, you need the correct syntax. The most common methods in PHP for establishing a connection include MySQLi and PDO. Below are essential elements involved when using these extensions:
- Server Name: Refers to the address of the server where the database is hosted, like or a remote server's IP.
- Username: The account name used to connect to the database.
- Password: The password corresponding to the username for authentication purposes.
- Database Name: This indicates the specific database you wish to connect to.
Hereโs a simple code example using MySQLi in procedural style:
This code attempts to connect to the database. If the connection fails, it outputs an error message. Understanding this syntax allows developers to verify connections efficiently and troubleshoot issues that may arise.
Closing the Connection
Closing the database connection is as significant as opening it. It helps in freeing up server resources and preventing potential data corruption. Failing to close connections can lead to performance issues in your application over time.
In PHP, the syntax to close a MySQLi connection is straightforward:
Effective management of database connections contributes to the stability and security of a web application. Properly closing connections ensures that resources are allocated appropriately and minimizes the risk of exceeding database connection limits.
Properly managing database connections is essential for an efficient and secure application. Always remember to close connections after use.
Error Handling in Database Connections
Handling errors effectively in database connections is essential for maintaining the integrity and reliability of applications. In this section, we will discuss why error handling is critical and how it can improve the overall experience when working with PHP and databases.
Errors related to database connections can arise from various sources. These can include issues related to server configuration, invalid credentials, or network problems. Understanding how to handle these errors helps developers pinpoint issues quickly. This knowledge reduces downtime and helps in horizontal scaling in applications. By anticipating potential problems and implementing appropriate error handling strategies, developers can maintain a smooth user experience.
Common Connection Errors
Authentication Failures
Authentication failures occur when the provided credentials do not match the records in the database. This often results from typos in the username or password, or sometimes an incorrect database name. The key characteristic of authentication failures is their immediate feedback. When a connection attempt fails due to authentication issues, the error message is usually straightforward.
This clarity makes them a popular choice for learning about error handling, as it provides a tangible example of what can go wrong. Moreover, authentication failures underscore the importance of securely managing database credentials. Mismanagement can lead to unauthorized access, making this a crucial consideration in database security.
Advantages of focusing on authentication failures include straightforward troubleshooting steps and the opportunity to review best practices for credential management in environments such as production. However, developers must also consider that excessive failed attempts can block user access and can be a gateway for malicious attacks.
Host Unreachable
The host unreachable error points to situations where the application cannot connect to the database server. This may occur due to network issues, incorrect server addresses, or configurations that restrict access. The key characteristic of this error is its association with the broader application environment, requiring attention to both server status and network configuration.
Host unreachable is particularly significant when discussing web applications hosted on remote servers. Understanding this aspect can aid in troubleshooting, especially in scenarios involving cloud services or containerized environments.
The unique feature of unreachable hosts is the potential for it to stem from multiple factors, such as firewall settings or incorrect routing protocols. Addressing this error can involve several layers of network diagnostics and resource verification, making it somewhat complex. This situation illustrates the interconnected nature of modern web applications and the various layers that can introduce issues.
Using try-catch Blocks
In PHP, the try-catch block is a fundamental construct used to handle exceptions gracefully. This method allows developers to anticipate possible errors during database operations. When an error occurs, the code inside the catch block executes, providing a way to manage the issue without crashing the application.
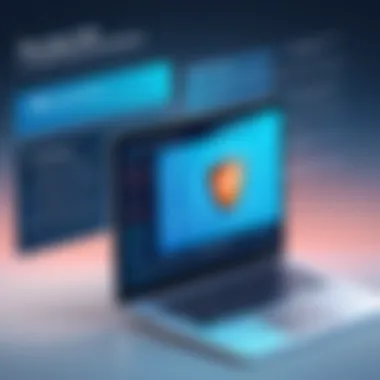
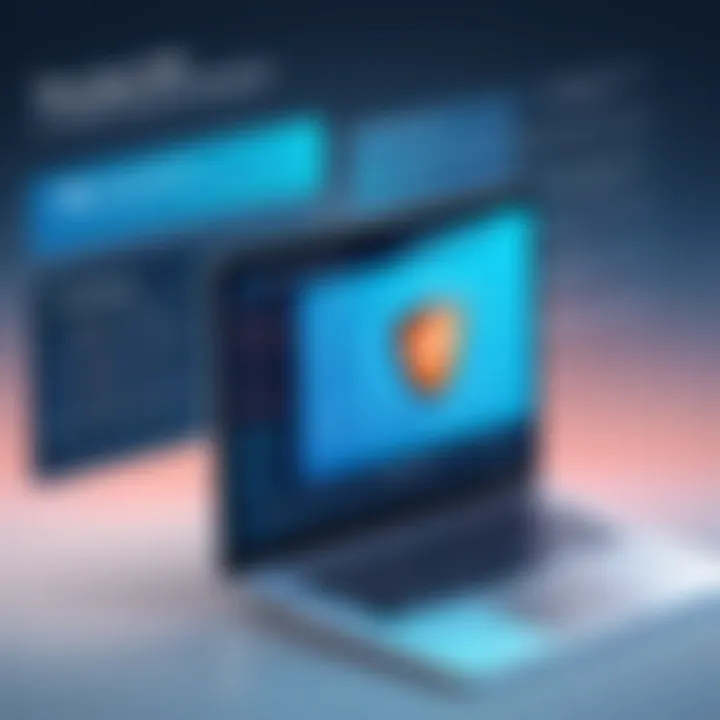
Using try-catch blocks enables cleaner, more organized code. It ensures that developers can log errors or display user-friendly messages without affecting the application's flow. It enhances the user experience by preventing unexpected termination of processes.
Implementing try-catch for database connections might look like this:
This construct not only captures the error but also allows for further actions, such as logging the error message without exposing sensitive information to the end user. This ability to control error visibility exemplifies the balance between robustness and security essential for modern applications.
Executing Queries
Executing queries is a crucial aspect of interacting with databases when using PHP. This process allows developers to retrieve, insert, update, or delete data stored in the database. Understanding how to execute queries effectively enhances the capability of PHP applications to manage data efficiently. Moreover, the effectiveness of query execution directly impacts application performance and user experience.
When you send a query to the database, you are making an instruction. The database management system interprets these instructions, carrying out tasks such as data retrieval or modification. Failing to properly execute these queries can lead to errors, inefficiencies, and potentially insecure practices that expose data to risks. Thus, mastering query execution is indispensable for maintaining the integrity and efficiency of an application.
Select Queries
Select queries are fundamental in database operations. They allow you to retrieve data from one or more tables. The data returned can be further processed or displayed within an application. The general syntax for a select query in PHP using MySQLi might look like this:
Benefits of Select Queries:
- Data Retrieval: The primary function is to extract data needed for applications.
- Flexibility: You can specify conditions, join multiple tables, and select specific columns, making select queries adaptable to various needs.
- Performance: Efficient select queries enhance the speed of applications by minimizing data load.
Itโs important to handle select queries carefully. Improperly structured queries can lead to performance bottlenecks or data errors. Additionally, using techniques like pagination is advisable when dealing with large datasets to avoid overwhelming the application and maintain responsiveness.
Insert, Update, Delete Queries
Insert, update, and delete queries work together to manage data in a database effectively. These operations allow the creation of new records, modification of existing ones, and removal of unwanted data, respectively.
- Insert Queries: To add new entries, the SQL syntax typically resembles:
- Update Queries: When modifying existing records, the syntax can look like:
- Delete Queries: To remove entries, the command would be:
Considerations:
- Data Integrity: Ensure that you validate data before executing these queries to maintain the quality of the database.
- Error Handling: Always implement error handling to capture any issues that may arise during execution. Failing to do so can lead to unexpected behavior in applications.
- Security Measures: Be aware of SQL injection attacks, and apply prepared statements or parameterized queries to safeguard against such attacks.
Fetching Results
Fetching results from a database is a crucial aspect of database interactions in PHP. Once data is retrieved via a query, efficiently handling and presenting this data determines the success of any application or website. It holds immense significance, particularly when outcomes are to be displayed to users or further processed within the application. Understanding how to fetch data correctly allows developers to optimize performance, reduce resource usage, and improve user experience.
Fetching Single Results
Fetching a single result from a database involves using a query that is designed to return a single record. This is paramount when you need specific information about an entry. The most common scenario is selecting a user by their ID or retrieving the details of a particular product. Using MySQLi or PDO in PHP, you can execute a query and manage the outcomes effectively.
For instance, consider the following example using PDO:
In this snippet, a prepared statement is used for security and efficiency. Once the query executes, the method retrieves a single record. This approach minimizes the risk of SQL injection and optimizes speed and resource usage. Fetching a single result is often all that is needed when displaying detailed pages that require specific information.
Fetching Multiple Results
On the other hand, fetching multiple results is critical when an array of records is needed. This is often the case when displaying a list of items, such as products in an e-commerce application or user listings in an admin panel. Using MySQLi and PDO allows for straightforward retrieval of these datasets.
An example of fetching multiple results can be seen in the following code using MySQLi:
Here, the method returns all records as an associative array, making it easier to process and display in a loop. Handling multiple results efficiently is crucial, as it affects both front-end and back-end performance. It is important to manage the size of data fetched, as overly large datasets can cause delays and increase loading times.
Security Best Practices
When working with PHP and database connections, security must be a primary consideration. Protecting data from unauthorized access and malicious attacks is essential to maintaining integrity and user trust. Secure coding practices, such as sanitizing inputs and using secure connections, can mitigate risks significantly.
Effective security practices encompass various strategies and techniques. Implementing these measures not only safeguards the data but also protects the application from common vulnerabilities. Understanding the importance of security in database interactions is crucial for developers, especially for those early in their coding journeys.
SQL Injection Prevention
SQL Injection is one of the most prevalent security threats that can affect databases. This type of attack occurs when an attacker injects malicious SQL statements into input fields, potentially allowing unauthorized access to sensitive data. Therefore, prevention should be a top priority. There are several strategies you can deploy to prevent SQL injection attacks:
- Input Validation: Always validate and sanitize user inputs. Ensure that inputs conform to expected formats. For example, if an input is expected to be an integer, do not accept anything else.
- Use Parameterized Queries: This is a favored approach as it separates SQL logic from user data. For example, using prepared statements can automatically handle input sanitization.
- Limit Database Permissions: Ensure that the database user has only the necessary permissions for the application. Avoid granting excessive rights that could be exploited by attackers.
By implementing these strategies, you are not just protecting data; you are also fostering a secure development culture.
Using Prepared Statements
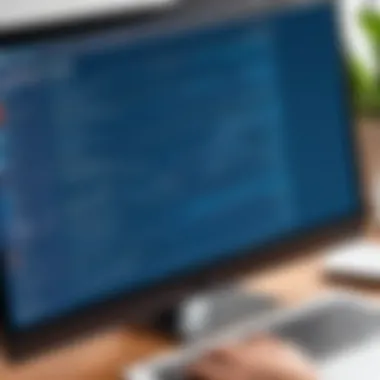
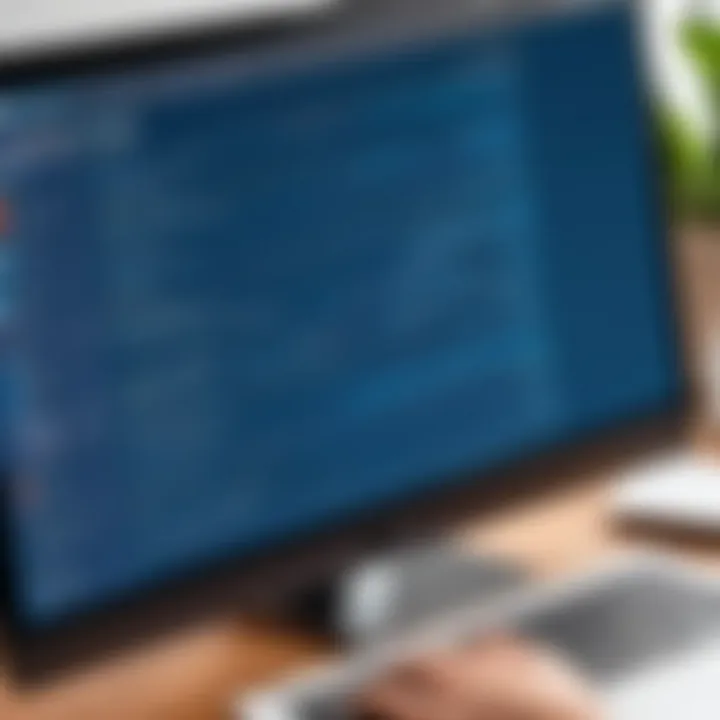
Prepared statements are an integral part of writing secure database code. They fundamentally change how queries are formed. By separating SQL code from data, they render injection attacks ineffective. Prepared statements allow developers to define the structure of the query beforehand. Variables can then be bound to the query in a secure manner.
Here is a general layout of how prepared statements work in PHP:
The use of as placeholders in prepared statements maintains the logical integrity of SQL commands while offering resilience against injection attempts. Always ensure to execute the statements and handle the results properly.
In summary, utilizing prepared statements not only enhances the security of your PHP applications but also improves code readability and maintainability. These practices collectively build a strong defense against one of the most serious threats in the field today.
Common Pitfalls and How to Avoid Them
Understanding common pitfalls during PHP database connections can greatly enhance application reliability and security. Avoiding these pitfalls allows developers to create robust applications that perform consistently, minimizing the risk of errors and vulnerabilities. This section explores two primary issues: hardcoding credentials and ignoring error codes.
Hardcoding Credentials
Hardcoding database credentials directly within the source code is a frequent mistake made by developers. This practice not only exposes sensitive information but also complicates the process of maintaining code in various environments, such as development, testing, and production.
Benefits of Avoiding Hardcoding:
- Enhanced Security: Keeping credentials out of the code reduces the risk of unauthorized access. If someone gains access to the source code, they may learn the database username and password.
- Easier Configuration Management: Using environment variables or configuration files separates sensitive information from the codebase, making it easier to change credentials without modifying the code.
- Better Version Control: When credentials are not included in code, it simplifies versioning and sharing code through repositories, minimizing the risk of unintentionally disclosing sensitive data.
Consider the following sample code that demonstrates a better approach using environment variables:
This technique ensures that the credentials are stored securely outside of the application code.
Ignoring Error Codes
Neglecting to check or respond to error codes is another common pitfall when working with PHP database connections. Each database interaction can produce error codes, which, if ignored, can lead to unhandled exceptions and application crashes or improper data handling.
Why Error Codes Matter:
- Debugging: Error codes provide critical insights into what went wrong during a database operation. Understanding the nature of the error helps in troubleshooting effectively.
- User Experience: By properly handling errors, developers can ensure that users receive helpful feedback instead of generic error messages or blank screens. This responsiveness can significantly enhance user experience.
- System Stability: Addressing potential errors improves overall stability. Applications that handle errors properly are less likely to fail or experience unexpected behavior in production.
An example of effective error handling in PHP using try-catch looks like this:
Properly handling error codes not only aids in troubleshooting but also contributes to a smoother user experience.
In summary, avoiding the hardcoding of credentials and promptly addressing error codes can significantly improve the development of PHP applications. By implementing best practices, developers ensure their applications are secure, maintainable, and user-friendly.
Testing Database Connections
Testing database connections is a critical aspect of database management that ensures the integrity and reliability of your applications. Establishing a successful connection is just the beginning. Knowing how to test these connections effectively can save time and prevent errors that can lead to data loss or application crashes. By thoroughly testing your connections, you can catch issues early and ensure that your application interacts with its database as expected.
Using PHP Scripts for Testing
Using PHP scripts for testing database connections provides a direct and efficient method to verify if your setup is working. A simple testing script can quickly identify connection issues, such as wrong credentials or unreachable hosts. Below is an example of a PHP script that tests a MySQL database connection:
This script attempts to connect to the MySQL server using provided parameters. If the connection fails, it outputs the error message, allowing for quick diagnosis. Therefore, running a test script like this after setting up a database helps ensure that everything is configured correctly.
Tools and Libraries
Various tools and libraries are available for testing database connections effectively. They can streamline the testing process, offer additional features, or enhance your coding experience. Some notable tools and libraries include:
- PHPUnit: Primarily a framework for unit testing, PHPUnit can also be adapted to test database connections.
- DBA Tools: Many hosting providers offer inbuilt databases management interfaces which can be used to test and manage connections.
- DBVisualizer: This is a universal database tool for developers that can also verify connections across different databases.
Using dedicated tools can simplify the testing process further, as they often provide visual interfaces and logs to assist in diagnosing connection problems. Good practices include testing under various conditions and ensuring your applications can handle possible connection failures without significant issues.
Proper testing of database connections is crucial for application stability and reliability.
Culmination
The conclusion section holds substantial importance in addressing the overarching theme present throughout this article. It serves as a synthesis of the fundamental concepts explored in depth regarding PHP database connections. By summarizing critical points, this section reinforces the significance of establishing reliable connections and maintaining a secure interaction between the PHP application and the database.
One primary element to consider is the practical application of the knowledge acquired. Users of this guide can implement various connection methods, such as MySQLi and PDO, with increased confidence after understanding their unique benefits and syntax. Additionally, recognizing the importance of error handling and security practices, like prepared statements, helps to safeguard against vulnerabilities, particularly SQL injection. This enhances not only the integrity of the data but also builds the applicationโs resilience against potential threats.
Moreover, reflecting on common pitfalls reiterates the importance of diligent programming practices. By avoiding hardcoded credentials and taking the time to handle error codes appropriately, developers can create a more adaptable and secure application environment.
"Proper testing and connection management form the backbone of effective database interaction in PHP."
In essence, this guide serves as an essential resource for students and beginners in programming languages. Not only does it offer a structured approach, but it also encourages a strategic mindset towards database management with PHP. Familiarity with these processes can lead to better coding practices and ultimately, a more robust application. The knowledge gained from this article should empower readers to confidently navigate the complexities of PHP database connections and engage deeply with their projects. By fostering a comprehensive understanding, this conclusion confirms the article's objective: to prepare readers for proficient database interactions within PHP.
Further Reading
Engaging in further reading on PHP database connections is an essential step for learners who wish to deepen their understanding of the topic. While this guide provides a strong foundation, expanding one's knowledge through additional resources can offer new insights and refined skills. Here are some specific elements and considerations when pursuing further reading:
- Diversity of Sources: Different resources, such as online tutorials, documentation, and forums, can provide various perspectives on PHP database operations. Websites like Wikipedia and Britannica present accurate, concise information which can clarify complex topics.
- Practical Implementation: Many resources include real-world examples, which can be instrumental in grasping how theoretical concepts play out in actual coding scenarios. Understanding the practical application of different methods, such as PDO or MySQLi, strengthens programming skills.
- Staying Updated: The landscape of programming evolves continuously. Engaging with online communities on platforms like Reddit can keep learners informed about the latest trends and updates in PHP development.
- Troubleshooting Techniques: Advanced reading material often includes detailed troubleshooting techniques. These resources can elucidate common pitfalls that programmers encounter, as well as best practices to resolve them effectively.
- Security Awareness: As security is paramount in database connections, further reading can illuminate vulnerabilities faced by PHP applications. Focusing on topics such as SQL injection or best practices for secure coding will enhance oneโs ability to maintain data integrity.
"The more you read, the more you will know; the more you know, the more you will do."
Incorporating diverse resources enables learners to witness how experienced developers approach challenges, ultimately fostering a more comprehensive skill set.
In summary, further reading on PHP database connections offers a multitude of benefits, ranging from enhancing technical know-how to interpreting contemporary programming challenges. It is vital for anyone serious about excelling in web development to recognize the value that continuous learning provides in a field that demands both flexibility and depth.