Perl Web Development: A Comprehensive Guide for Programmers
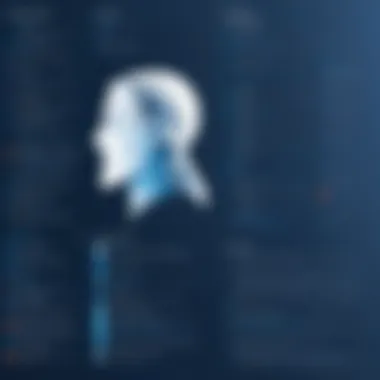
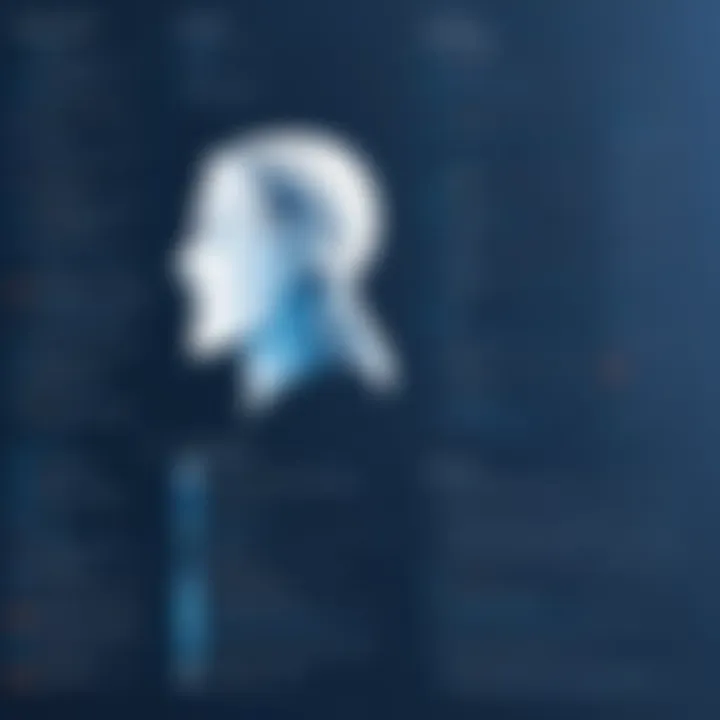
Prologue to Programming Language
Perl is a high-level programming language known for its versatility and power. Originally developed by Larry Wall in 1987, Perl has a rich history that reflects its adaptability. It started as a tool for text manipulation and reporting, but it later expanded into other domains such as web development, system administration, and network programming. It is particularly valued for its capability to handle regular expressions, making it ideal for parsing and generating text.
History and Background
The roots of Perl trace back to the late 1980s. It arose from a necessity for a practical programming solution that could bridge various scripting languages. Over the years, Perl evolved through several versions, each introducing new features and capabilities. The release of Perl 5 in the mid-1990s marked a significant turning point, with the introduction of a fully object-oriented programming system, making Perl more suitable for larger projects and applications.
Features and Uses
Perl possesses distinct features that contribute to its popularity among developers:
- Text processing capabilities: Perl excels in dealing with text, allowing complex string manipulations and pattern matching.
- Cross-platform support: Perl code can run on various operating systems, ensuring flexibility.
- Extensive libraries: CPAN (Comprehensive Perl Archive Network) provides thousands of modules to extend Perl's functionality.
These traits render Perl a preferred choice for web development, particularly in scenarios requiring dynamic content and database interactions.
Popularity and Scope
Despite the rise of newer languages, Perl maintains a loyal community. Its longstanding presence in CGI scripting and web frameworks like Dancer and Mojolicious showcases its capability to stay relevant. While its usage may have shifted, it remains a valuable tool in web development. Its extensive documentation and active user communities foster continuous learning and collaboration, making it suitable for both beginners and experienced programmers.
"Perl's flexibility allows developers to express complex tasks succinctly and efficiently."
Basic Syntax and Concepts
Understanding the basic syntax and concepts of Perl is essential for effective programming. These foundational elements are crucial in building more complex applications and can be often compared to other languages like Python or JavaScript.
Variables and Data Types
Perl provides a rich set of variable types. Variables can be scalar, arrays, or hashes:
- Scalar Variables: Represent a single value. Denoted by a dollar sign, e.g., .
- Arrays: Ordered lists of scalars, denoted by an at sign, e.g., .
- Hashes: Unordered sets of key-value pairs, denoted by a percent sign, e.g., .
Operators and Expressions
Perl supports a variety of operators, from arithmetic to string manipulation. For example, the addition operator can be used to compute sums, while the concatenation operator joins strings. Understanding these operators allows programmers to craft intricate expressions that manipulate data efficiently.
Control Structures
Control structures in Perl facilitate decision-making and looping. Common constructs are:
- If Statements: Allow conditional execution of code blocks.
- Loops (for, while): Enable repetitive tasks over an array or condition.
Advanced Topics
To fully utilize Perl for web development, one must delve into advanced topics that enhance its capabilities.
Functions and Methods
Functions are reusable blocks of code that serve specific tasks. In Perl, defining a function is straightforward, using the keyword. Example:
This function takes a name as input and returns a greeting.
Object-Oriented Programming
Perl provides support for object-oriented programming, allowing programmers to create objects from classes. This enables encapsulation and inheritance, essential concepts for large applications.
Exception Handling
Exception handling in Perl uses blocks to catch and handle errors gracefully. This is crucial for maintaining robust web applications, ensuring that errors do not crash the site.
Hands-On Examples
Applying knowledge through hands-on examples solidifies understanding and enhances skills.
Simple Programs
Starting with simple scripts can help beginners grasp Perl's syntax and built-in functions. A basic "Hello World" script would look like this:
Intermediate Projects
As proficiency grows, programmers can tackle intermediate projects, such as building a simple web application using CGI.
Code Snippets
Utilizing code snippets from existing projects can accelerate development. For instance, connecting to a database using DBI can streamline data management in web applications:
Resources and Further Learning
To enhance Perl programming skills, various resources are available.
Recommended Books and Tutorials
Books like Learning Perl and Programming Perl are essential reads for programmers wanting a solid foundation. Online tutorials can also provide hands-on practice and guidance.
Online Courses and Platforms
Platforms such as Udemy and Coursera offer structured courses on Perl programming, beneficial for learners at all levels.
Community Forums and Groups
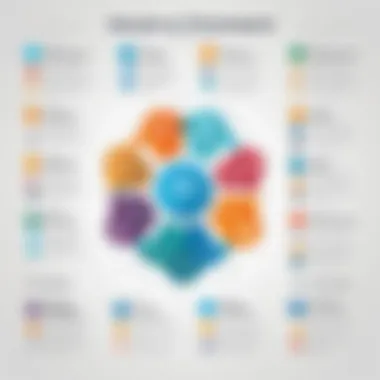
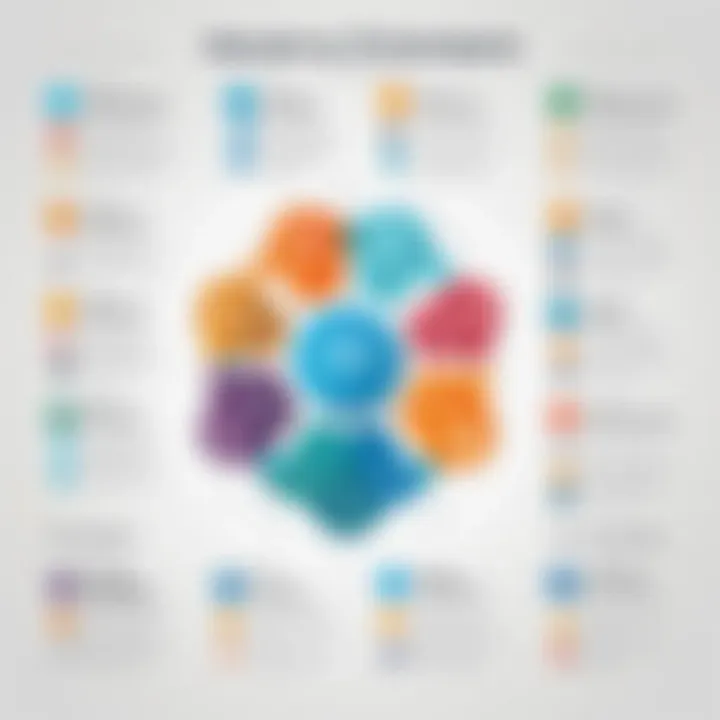
Engaging with community forums like Reddit can facilitate knowledge sharing and support. Additionally, groups on Facebook cater to Perl developers looking to network and collaborate.
By exploring these resources, programmers can deepen their understanding of Perl and its application in web development.
Preface to Perl and Its Role in Web Development
Perl is a programming language known for its flexibility and power, especially in web development settings. As we explore its contributions to creating dynamic web applications, it is essential to appreciate why Perl remains relevant despite the emergence of newer languages. This section introduces the core aspects of Perl's applicability in web development. Understanding these components is crucial for developers looking to navigate the complexities of building modern web applications.
Understanding Perl as a Programming Language
Perl was created by Larry Wall in the late 1980s and has since evolved into a robust scripting language. It is particularly notable for its text processing capabilities, making it a suitable choice for web development tasks. Perl syntax is straightforward, enabling both novice and experienced programmers to write clear code. Its object-oriented features, combined with support for procedural programming, allow for diverse application structures.
One of the main benefits of using Perl is its extensive CPAN (Comprehensive Perl Archive Network) library, which contains numerous modules for varied functionalities. Developers can leverage these pre-built modules to add intricate features without starting from scratch. This makes Perl an efficient and resourceful choice when building complex web applications. Additionally, the strong community support ensures that developers have access to resources and help when encountering challenges.
Historical Context of Perl in Web Applications
Perl first gained popularity in web development during the 1990s, thanks to its ability to generate dynamic content. The advent of technologies like CGI (Common Gateway Interface) allowed Perl scripts to run on servers, enabling the creation of interactive web pages. During its peak, sites like Amazon used Perl for their backend services, showcasing how the language could manage heavy traffic and data.
As the internet evolved, Perl adapted as well. Frameworks such as CGI.pm emerged to simplify the development of web applications. However, with the growth of other languages and frameworks like PHP and Python, Perl's dominance began to wane. Despite this, Perl persists as a valuable tool for specific use cases, particularly where text manipulation and data processing are key.
"The strength of Perl lies in its comprehensive nature; it empowers developers to solve complex problems with simplicity and efficiency."
Frameworks for Perl Web Development
The utilization of frameworks in Perl web development is pivotal as they provide the necessary structure for building applications efficiently. Frameworks assist developers in streamlining their workflow, ensuring scalability and maintainability in their code. They offer a set of reusable components and libraries that can simplify the development process, making it easier to integrate various functionalities without reinventing the wheel. This leads to quicker development cycles as developers can focus on the unique aspects of their applications rather than basic functionalities.
Additionally, frameworks often come with built-in support for common tasks such as routing, templating, and database interactions. This allows developers to adopt best practices right out of the box. When choosing a framework, consider aspects like community support, documentation quality, and compatibility with existing codebases. Below are brief overviews of three major Perl frameworks that each offer unique advantages.
Mojolicious: A Modern Perl Web Framework
Mojolicious positions itself as a contemporary web framework that emphasizes simplicity and developer happiness. Its non-blocking architecture makes it an attractive choice for applications requiring real-time features, such as chat applications or dynamic dashboards. Developers benefit from built-in features like WebSocket support and a powerful routing system, which enables easy handling of HTTP requests.
One distinctive aspect of Mojolicious is its user-friendly environment. It offers both an interactive command-line application and a lightweight web server for testing. This ensures that developers can prototype quickly and iterate on their ideas.
Moreover, Mojolicious comes with a minimalistic approach that avoids adding unnecessary complexity to projects. It focuses on rapid development and straightforward syntax, making it suitable for both new and experienced developers alike. The community around Mojolicious is active, which fosters continuous improvement and regular updates.
Dancer: Simplicity Meets Functionality
Dancer is another prominent framework in the Perl ecosystem, specifically designed for developers who favor simplicity and speed. It is lightweight and encourages a straightforward development style, which can be appealing for small to medium-sized applications. Dancer's architecture allows for rapid prototyping, making it easy to test ideas without substantial overhead.
The framework includes features like middleware support and pluggable components, providing flexibility in extending functionalities. It supports various template engines, assisting developers in creating clean and maintainable views. Furthermore, Dancer's focus on convention over configuration reduces the amount of boilerplate code developers must write, enhancing productivity.
Dancer’s strong emphasis on community contributions means that there is a wealth of plugins available, accommodating diverse needs and preferences. The learning curve is gentle, allowing new developers to become productive quickly while still offering enough depth for advanced users.
Catalyst: A Comprehensive Framework
Catalyst stands out as a more robust framework that adopts the Model-View-Controller (MVC) pattern. It is a powerful choice for larger, more complex web applications. Catalyst’s design allows for clear separation of concerns, which facilitates better organization and management of code. This makes it an ideal solution for projects that demand scalability and maintainability.
One key feature of Catalyst is its support for a wide array of plugins, which can improve functionalities like authentication, authorization, and session management. This modular system allows teams to build tailored solutions without encumbering the core application extensibility.
Although Catalyst may come with a steeper learning curve compared to Dancer or Mojolicious, its comprehensive features and extensive documentation help to mitigate this challenge. There is a dedicated community that actively contributes to its development, ensuring that Catalyst remains relevant in the evolving landscape of web technologies.
In summary, Perl frameworks like Mojolicious, Dancer, and Catalyst each offer unique advantages tailored to different project requirements, making them valuable tools for web developers.
Setting Up a Perl Development Environment
Setting up a Perl development environment is a critical first step in any web development project using Perl. This process involves selecting the appropriate tools and configuring the system in a way that maximizes efficiency and productivity. The right setup can lead to quicker development cycles, effective debugging, and easier maintenance of code.
Choosing the Right Text Editor for Perl
Selecting a text editor is the starting point for writing Perl code. A good text editor enhances the coding experience and can improve overall productivity. Popular text editors among Perl developers include Visual Studio Code, Atom, and Sublime Text. Each of these editors offers features such as syntax highlighting, code completion, and debugging support.
When choosing a text editor, consider its compatibility with Perl and available plugins. Some plugins can provide code linting or integration with version control systems, such as Git. Preferences can vary widely among developers. A text-based editor can work for some, while others may prefer a more graphical interface.
Key Features to Look For in a Text Editor:
- Syntax Highlighting: Makes the code more readable.
- Code Completion: Speeds up the writing process by suggesting code snippets.
- Integrated Terminal: Allows executing scripts without leaving the editor.
- Version Control Integration: Simplifies managing code changes over time.
Installing Perl and Required Modules
After selecting a text editor, the next step is to install Perl and any required modules. Perl can be obtained from different sources, such as the official Perl website, or through package managers like for Debian-based systems or for macOS.
Once Perl is installed, it is crucial to manage dependencies effectively. Perl uses modules to extend its functionality, and these can be installed via CPAN (Comprehensive Perl Archive Network). CPAN is an excellent resource for finding and downloading Perl modules.
Installation Steps for Perl Modules:
- Open your terminal or command prompt.
- Launch the CPAN shell by typing .
- Use the command to install a specific module.
It's essential to check compatibility of the modules with your Perl version. Some modules may not function correctly with certain versions of Perl. Therefore, it is wise to keep the environment updated and manage dependencies with tools like for a more straightforward installation process.
Core Concepts in Perl Web Development
Understanding core concepts is crucial for developing applications using Perl. This section emphasizes the essential elements needed for effective web development. It covers how Perl handles HTTP transactions and how dynamic content generation is achieved through template systems. By grasping these concepts, developers can build robust web applications with better efficiency and reliability.
Handling HTTP Requests and Responses
Handling HTTP requests and responses is a fundamental aspect of web development. When a user navigates to a website, their browser sends an HTTP request to the server. Perl provides several ways to handle these requests effectively, primarily through its web frameworks.
Perl's built-in modules, like LWP::UserAgent, allow you to create scripts that can send HTTP requests and receive responses. On the server-side, frameworks such as Mojolicious and Dancer simplify the process. They enable programmers to manage incoming requests seamlessly and send back the appropriate responses.
Here’s a brief overview of the process:
- A user makes an HTTP request to a Perl web application.
- The server receives the request and interprets it based on the route defined within the application.
- The application processes the request, possibly interacting with a database or another service.
- Finally, it constructs a response, which is sent back to the user’s browser.
This request-response cycle is foundational for any web application. Understanding it enhances a developer's ability to create dynamic applications that respond appropriately to user actions.
Working with Templates for Dynamic Content
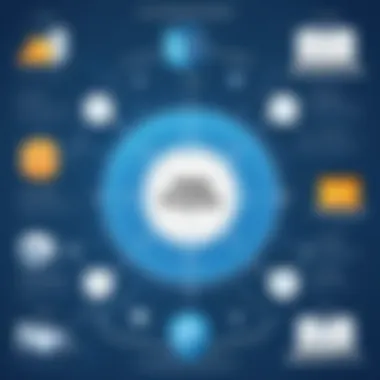
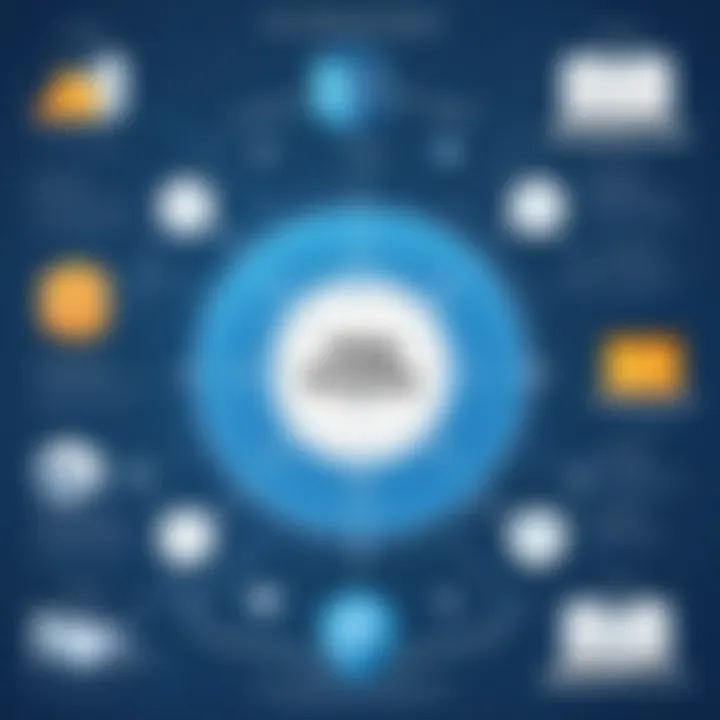
Generating dynamic content is a key feature in modern web applications. Templates allow developers to separate the presentation layer from the application logic, promoting cleaner code and better maintenance. Perl offers several template systems, which help in rendering HTML alongside embedded Perl code.
One popular template engine is Template Toolkit. It allows for easy creation of dynamic web pages by merging data with template files. This process involves:
- Defining Templates: Create a template file that determines how the output should look.
- Data Preparation: Prepare the data that needs to be displayed to the user.
- Merging: Use a Perl script to combine the template with the data.
- Rendering: Finally, output the combined result as HTML to the client’s browser.
Use of templates not only simplifies the development process but also facilitates better performance. By enabling caching and reusing templates, applications can serve requests faster, providing a better user experience.
Integrating templates with your Perl application can significantly reduce code clutter and increase clarity.
Database Interaction with Perl
Database interaction is a core component of modern web applications. In the context of Perl web development, understanding how to efficiently communicate with databases is essential for building dynamic and responsive applications. Many web applications rely heavily on databases for storing and retrieving data. As a programmer, mastering database interaction with Perl empowers you to create applications that can support complex functionality, improve performance, and enhance user experience.
When working with databases, important considerations include the choice of database, the method of connectivity, and how you approach data manipulation. Perl provides several tools and modules to simplify these tasks, offering varying levels of abstraction and control. Using these tools effectively can increase development speed and reliability.
Using DBI for Database Connectivity
DBI, which stands for Database Interface, is a crucial module in Perl for database connectivity. It acts as a database abstraction layer that allows for easy interaction with various database systems, such as MySQL, PostgreSQL, or SQLite. This module standardizes how you connect to different databases, making your code portable and reducing the need for database-specific syntax.
To begin using DBI, you first need to install the module if it's not already available. You can do so easily using the CPAN module manager. Here’s how you might set up a simple database connection:
This straightforward code snippet connects to a SQLite database. Once connected, you can execute SQL queries against the database using DBI’s methods. Additionally, the module handles error reporting gracefully, which is vital for diagnosing issues during development.
DBI also facilitates the execution of prepared statements, which enhances both performance and security. Prepared statements prevent SQL injection attacks by separating query execution from data input. Here’s an example of using a prepared statement:
ORM Solutions: DBIx::Class
While DBI is powerful, direct SQL can become cumbersome for larger applications. This is where Object-Relational Mapping (ORM) solutions like DBIx::Class come into play. DBIx::Class simplifies data handling by representing database tables as classes and rows as objects. This allows developers to interact with the database using Perl object syntax, promoting cleaner and more maintainable code.
One key benefit of using DBIx::Class is its ability to abstract database interactions. Developers can focus on the application logic rather than dealing with the intricacies of SQL queries. Here is a simple example of how DBIx::Class can manage a database entry:
This code defines a User model in DBIx::Class. Once this is set up, you can create a new user object and save it to the database without writing raw SQL:
Using DBIx::Class not only boosts developer productivity but also enhances code readability. Furthermore, it provides powerful features like relationship handling, which allows for easy association between different data models.
Working with APIs in Perl
Working with APIs is an essential aspect of modern web development. As applications increasingly rely on external services and data sources, understanding how to effectively handle API interactions becomes crucial. In the context of Perl, APIs provide a way for developers to create dynamic and responsive applications that can communicate with other systems, whether they are internal services or third-party endpoints.
Using APIs in Perl allows developers to tap into existing services, streamlining development processes. For instance, integrating payment processing, geolocation services, or social media functionalities can be accomplished efficiently through APIs. This capability enhances the scalability and functionality of web applications. Additionally, the use of APIs promotes a modular approach to development, enabling teams to focus on specific functionalities without needing to build every component from scratch.
RESTful APIs: A Practical Approach
RESTful APIs are a key standard in web services today. They follow the principles of Representational State Transfer, making use of HTTP requests to access and manipulate data. This approach is particularly advantageous because it aligns well with web technologies and is both simple and effective.
In Perl, handling RESTful APIs often involves using modules like LWP::UserAgent or HTTP::Tiny for HTTP requests. Here is a basic example of making a GET request with LWP::UserAgent:
This snippet demonstrates how to initiate a request to an API and process the response. RESTful APIs return data in a structured format, typically JSON or XML. Perl provides excellent support for JSON through modules like JSON::MaybeXS, making it simple to decode and work with responses.
Integrating Third-Party APIs
Integrating third-party APIs expands the capabilities of a Perl web application significantly. Many services, like Twitter, Google Maps, or Stripe, offer APIs that can be integrated easily. Each API comes with its own set of requirements—most commonly, authentication tokens or API keys.
When integrating with these APIs, developers should carefully review the API documentation provided by the service. This documentation usually outlines the endpoints, request formats, and data return structures.
Here’s a general approach to integrating a third-party API:
- Get API Keys: Register for the API to obtain necessary access credentials.
- Read Documentation: Understand the endpoints, parameters, and data formats required.
- Set Up Requests: Use Perl modules to construct requests, managing headers and authentication as needed.
- Handle Responses: Properly parse and utilize the data returned, maintaining error handling.
For example, a simple integration with a weather API might look like this:
By following these steps, developers can integrate rich functionalities into their Perl applications using available APIs, making their projects more powerful and versatile.
"APIs are a critical element in modern web development. They allow developers to enhance their web applications without reinventing the wheel."
In summary, working with APIs in Perl opens up avenues to leverage external systems and services, immensely enriching web application capabilities. Understanding and implementing RESTful APIs, along with integrating third-party APIs, are foundational skills for any programmer looking to succeed in the evolving landscape of web development.
Testing and Debugging Perl Web Applications
Testing and debugging are vital components of programming in any language, including Perl. They play a significant role in ensuring that web applications run smoothly and meet user expectations. In Perl web development, proper testing can catch bugs early, which minimizes costly repairs later. Debugging helps developers understand the behavior of their scripts and locate issues that may not be immediately apparent. Together, these processes enhance the reliability and functionality of applications.
Unit Testing with Test::More
Unit testing is a focused approach that involves testing individual components or functions of a program to verify their correctness. In Perl, the Test::More module provides a robust framework for this purpose. Using Test::More can automate tests, ensuring that any changes to code do not introduce new bugs. Here are some key aspects:
- Simplicity: Writing tests is straightforward with Test::More. The syntax is clean, which makes it easier for developers to articulate their tests.
- Comprehensive Assertions: The module offers numerous assertions to check values, such as , , , among others. This variety allows for thorough validation of components.
- Test Plans: Developers can outline a plan for what tests to run before executing them. This feature ensures that all anticipated scenarios are covered.
Code example:
This sample code demonstrates using Test::More to perform simple mathematical tests. Such tests help confirm the correct functioning of the logic in the application.
Debugging Techniques for Perl Scripts
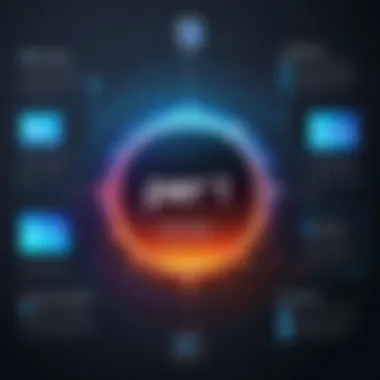
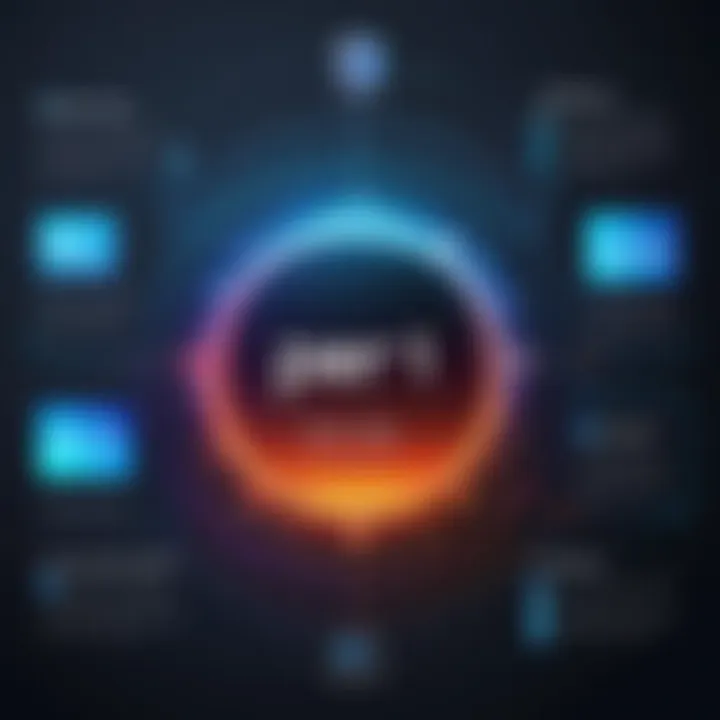
Debugging is as essential as testing, as it allows developers to explore and fix issues in their applications. Perl offers built-in tools to aid in debugging. Here are some effective techniques:
- Use of Perl Debugger: Perl has a debugger that can be invoked with the flag. It allows stepping through the code, inspecting variable values, and identifying where things go wrong.
- Print Statements: While not a formal debugging technique, inserting print statements throughout the code can help track variable values and application flow. This is especially useful for quick checks.
Effective debugging leads to a deeper understanding of the code and enhances overall user experience. Regular practice of these techniques can prevent future complications, keeping the codebase reliable.
Performance Optimization Techniques
Performance optimization is vital in web development, especially when using Perl. A well-optimized application improves user experience and effectively utilizes server resources. This section explores various techniques to enhance the performance of Perl web applications. Key considerations include load times, efficient resource management, and scaling the application as user load increases.
Profiling Perl Applications
Profiling is the process of measuring where time and resources are spent in your application. It helps identify bottlenecks and inefficient code blocks. Tools like Devel::NYTProf can provide deep insights into how your Perl application performs. This module generates detailed reports on the execution time of subroutines and other code segments.
- Choose a Profiling Tool: Start by selecting a tool that fits your needs. Devel::NYTProf is popular for its comprehensive output, while DProf is simpler.
- Analyze Performance Metrics: After profiling, review the generated reports. Focus on the sections with high execution times. This allows you to detect slow database queries or heavy processing tasks.
- Optimize Bottlenecks: Once identified, modify or refactor the slow parts of code. Splitting large functions into smaller, manageable pieces or utilizing more efficient algorithms can yield significant improvements.
Profiling is not a one-time task. Regular profiling should be part of the development workflow to keep performance in check.
Caching Strategies for Web Applications
Caching is a technique used to store copies of files or data to serve subsequent requests quickly. In Perl web development, effective caching strategies can reduce load times and server strain. Here are essential caching methods:
- Page Caching: Store HTML output of pages. When a user requests the page, the server retrieves the pre-generated HTML instead of processing the Perl code.
- Database Query Caching: Store results of frequent database queries. Tools like Cache::Memcached allow quick access to database results without repetitive queries.
- Object Caching: Utilize objects, files, or other data structures that are expensive to create. Storing them in a cache reduces load times for future accesses.
Implementing caching requires careful consideration. Overusing cache can lead to stale data issues. Always update the cache appropriately when underlying data changes.
Regularly evaluate your caching strategy for effectiveness. Adjust as needed to align with user behavior and application requirements.
Combining profiling with robust caching strategies can significantly enhance the performance of Perl web applications, ensuring swift responses to users while optimizing resource usage.
Deployment Strategies for Perl Applications
Deployment is a crucial component in the life cycle of a web application. It directly affects how users interact with the application and how developers maintain it. Understanding deployment strategies for Perl applications can lead to enhanced performance, security, and scalability. An effective deployment strategy ensures that an application is available, reliable, and easy to update.
When planning deployment, consider the environment your application will run in. Factors such as server specifications, configuration, and load balancing play important roles. Different strategies can optimize resource usage and reduce downtime. In this section, we will discuss two main approaches: traditional hosting options and modern containerization techniques.
Hosting Options for Perl-based Applications
Several hosting options exist for deploying Perl applications. Selecting the right one depends on the unique needs of a project. Some common hosting options include:
- Shared Hosting: With shared hosting, multiple websites coexist on a single server. It is a cost-effective solution for small applications but may suffer from performance issues due to resource sharing.
- Virtual Private Servers (VPS): A VPS provides a dedicated environment within a shared server. This allows for better performance and security compared to shared hosting. It is suitable for growing applications that require more control.
- Dedicated Servers: For larger applications, dedicated servers offer maximum control and resources. They are suitable for high-traffic websites. However, managing a dedicated server requires technical expertise.
- Cloud Hosting: Services like Amazon Web Services and Google Cloud provide scalable resources on demand. Cloud hosting is flexible and can adjust to changing traffic patterns. It offers the potential for cost savings as you only pay for what you use.
Each of these options comes with its benefits and challenges. It is important to evaluate the specific requirements of your application before making a choice.
Using Docker for Perl Deployment
Docker has revolutionized how applications are deployed in recent years. It allows developers to package applications and their dependencies into isolated containers. This ensures consistency across different environments.
Deploying Perl applications using Docker offers several advantages:
- Environment Consistency: Docker containers standardize development, testing, and production environments. This reduces surprises when deploying across different platforms.
- Scalability: Containers are lightweight. This means they can be replicated quickly to handle increased loads, making scaling more manageable.
- Isolation: Each container runs in its own environment. This isolation prevents conflicts between different applications and their dependencies.
To deploy a Perl application with Docker, start by creating a Dockerfile. This file defines the environment and configurations for your application. A simple Dockerfile for a Perl application might look like this:
In this example, the Dockerfile uses the latest version of Perl, sets the working directory, copies the application files, and specifies the command to run the Perl script.
Deploying with Docker simplifies many aspects of management and scaling. However, it does require familiarity with container technologies and best practices.
Security Considerations in Perl Web Development
In the realm of web development, security is paramount. This is especially true for applications built with Perl, where vulnerabilities can lead to significant data breaches, illegal access, or service interruptions. Understanding security considerations is crucial for any developer. Good practice in security not only protects end-users but also enhances the reputation and reliability of the applications being developed.
Perl has unique characteristics that both help and hinder secure web development. By recognizing these aspects, developers can effectively guard against common threats, ensuring their applications are robust and resilient. The following sections will go deeper into common vulnerabilities and strategies to mitigate them.
Common Security Vulnerabilities
Security vulnerabilities come in various forms, and Perl web applications are not immune. Here are some prevalent ones that developers need to be aware of:
- SQL Injection: This vulnerability allows attackers to execute arbitrary SQL queries through manipulated user input. This can result in unauthorized access to databases, data leaks, or data manipulation.
- Cross-Site Scripting (XSS): XSS allows attackers to inject malicious scripts into web pages viewed by users. This can lead to session hijacking, data theft, or misleading content.
- Cross-Site Request Forgery (CSRF): An attacker tricks a user into executing unwanted actions on a web application in which they are authenticated. This can lead to unauthorized actions without the user's awareness.
- File Inclusion Vulnerabilities: If user input is not sanitized properly, attackers may include files from the server, leading to arbitrary code execution.
- Exposure of Sensitive Data: Failure to enforce proper encryption and data protection measures can expose sensitive information, risking user privacy and compliance violations.
Being aware of these vulnerabilities is the first step in securing applications built with Perl. It is essential for developers to regularly update their knowledge and tools to prevent these forms of attacks.
Best Practices for Securing Perl Applications
Securing a Perl web application requires proactive steps and adherence to best practices. Here are some key strategies:
- Input Validation: Validate and sanitize all user inputs. Use built-in Perl functions to ensure user data is safe before processing.
- Parameterized Queries: When interacting with databases, use parameterized queries with . This prevents SQL injection, forcing input data to be treated as data rather than executable code.
- XSS Prevention: Implement output encoding to protect against XSS attacks. Libraries like HTML::Escape can help sanitize output data.
- CSRF Tokens: Use unique tokens for forms to prevent unauthorized requests. These tokens should be validated for each state-changing action in your application.
- File Access Controls: Restrict file access and ensure that user inputs cannot alter file paths. Regularly audit file permissions to ensure they align with least privilege principles.
- Regular Security Updates: Stay informed about security updates and patches for libraries and frameworks used in your application. Outdated software can have known vulnerabilities that are easily exploitable.
By implementing these practices, developers can significantly reduce the risk of severe security incidents.
"Good security is not just about tools, but about a culture of safety. Keeping the mind sharp and always learning offers the strongest defense against potential threats."
The Future of Perl in Web Development
The landscape of web development is changing rapidly. Understanding these shifts is important for developers using Perl. Perl, a language known for its text-processing power and flexibility, must continue to evolve to meet the demands of modern web applications. This section explores crucial future trends and considerations that shape Perl's role in web development.
Trends Influencing Perl Development
Current trends have notable implications for the future of Perl. Several factors influence how developers approach web development with this programming language. Some of the prominent trends include:
- Microservices Architecture: This approach promotes small, independent services that can work together. Perl is adapting to this model by providing lightweight frameworks.
- Serverless Computing: Using functions in the cloud without managing servers is growing. Perl can integrate with cloud services, allowing for flexibility and scalability.
- DevOps Practices: Automation and collaboration in software development are important. Perl scripts can facilitate automated processes, making them valuable in DevOps pipelines.
- APIs and Integration: As web applications become more interconnected, using Perl to build and consume APIs is essential. With a focus on RESTful architectures, Perl offers tools for integrating various services seamlessly.
While these trends hold promise, they require continued adaptation from the Perl community. Developers must remain aware of the shifting landscape and utilize Perl's strengths in new ways.
Perl Community and Ecosystem Growth
The growth of the Perl community plays a significant role in shaping its future in web development. The community is known for its collaborative spirit, fostering learning and resource sharing.
- Conferences and Meetings: Events such as YAPC (Yet Another Perl Conference) and workshops are platforms where developers exchange ideas. This interaction helps keep the language relevant and innovative.
- Open Source Contributions: Many Perl modules are open-source. Encouraging contributions can enhance Perl's ecosystem, leading to more robust frameworks and tools.
- Online Resources: Platforms like Reddit and PerlMonks provide support and knowledge-sharing. These online forums are vital for new learners and experienced developers alike, ensuring that Perl remains accessible.
"A vibrant community is essential for any programming language's evolution. The continuous exchange of ideas is what drives Perl forward."
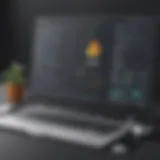
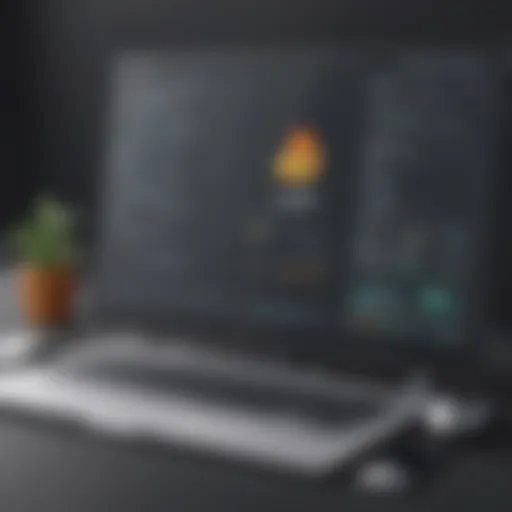