Integrating Node.js with React for Scalable Web Apps
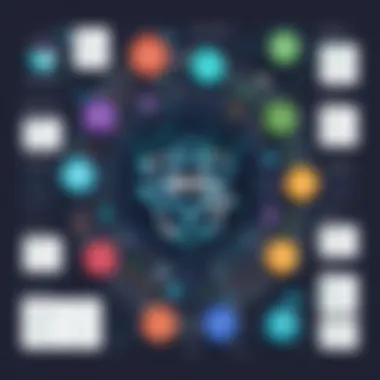
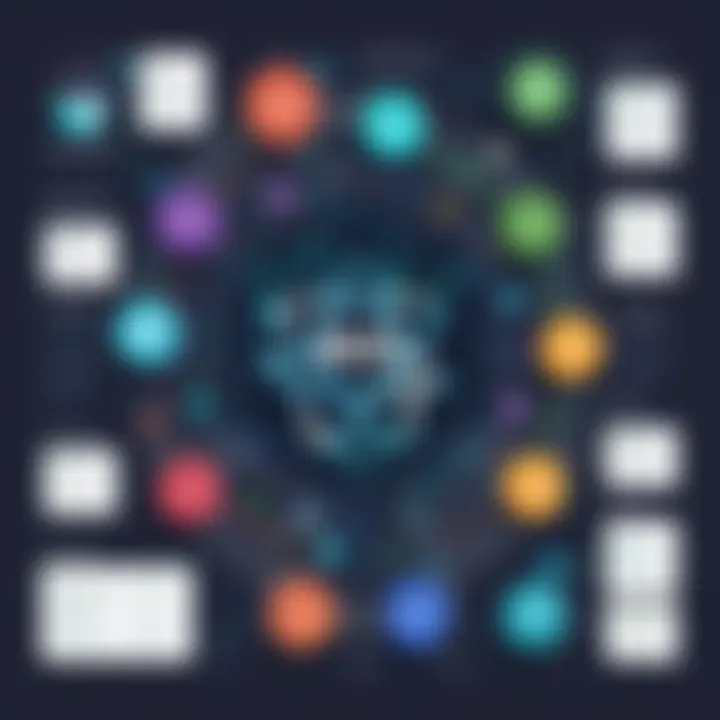
Intro
As the digital landscape continues to evolve, understanding how different web technologies can work in tandem is becoming critical. Two of the most prominent players in this arena are Node.js and React. Node.js is a powerful runtime for executing JavaScript on the server side, while React is a popular library for building interactive user interfaces. Together, they present a robust solution for developing dynamic and scalable web applications. This article aims to furnish readers with a comprehensive guide on integrating these technologies effectively.
Why Node.js with React?
Utilizing Node.js alongside React offers numerous advantages. Both technologies revolve around JavaScript, enabling seamless communication and data transfer between the client and server. For instance, with Node.js handling the backend and React managing the front end, developers can streamline their workflow through shared libraries and consistent data structures.
But why should developers consider this combination?
- Single Language: There's no need to juggle multiple languages. This allows for a more coherent development process.
- Performance: Node.js's non-blocking architecture serves well when handling numerous connections, while React's virtual DOM enhances user experience by minimizing DOM manipulations.
- Scalability: Building applications that can scale is easier when utilizing both technologies together. You can easily handle growing data loads and user requests.
"When building modern applications, choosing the right tools is half the battle."
Initial Setup
Before diving into coding, it's essential to set up the environment properly. Install Node.js from Node.js official website, and you’ll also want a package manager like npm (which comes bundled with Node.js). For React, you'll typically use the Create React App boilerplate. This setup simplifies your development process, letting you focus on enhancing your application.
Core Concepts
Node.js and React Architecture
Understanding the architectural framework of both technologies is fundamental. Node.js operates on a single-threaded event loop, allowing it to handle multiple requests with minimal overhead. On the other hand, React's component-based architecture promotes reusability and separation of concerns, enhancing maintainability.
Data Handling
Data is at the heart of modern applications. Integrating Node.js with React typically involves API interactions. Developers often utilize Express.js on the Node side to create RESTful APIs. Here’s a simple example of an Express route:
This snippet serves a basic JSON response that React can easily fetch and display.
Common Challenges
Integrating Node.js and React may not always be smooth sailing. Some common challenges include:
- CORS Issues: Cross-Origin Resource Sharing can lead to difficulties when the frontend and backend are hosted on different domains.
- State Management: As applications grow, managing state becomes critical. Libraries like Redux can aid in maintaining a predictable state across your application.
Final Thoughts
The fusion of Node.js and React holds the promise of creating scalable, maintainable, and efficient web applications. By grasping the underlying concepts and components involved in this integration, developers can significantly enhance their productivity and craft better user experiences. As you embark on this journey, remember that the learning never stops, and there's always something new in this dynamic landscape.
For further learning, consider delving into Wikipedia's page about Node.js, and to engage with fellow programmers, platforms like Reddit can be invaluable.
Whether you are just starting or looking to refine your expertise, the synergy between Node.js and React is a pathway to mastering modern web development.
Preface to Node.js and React
In today's fast-paced digital landscape, the stakes for web developers have never been higher. Building responsive, interactive applications is no longer just a nice-to-have; it's a necessity. This creates a compelling case for understanding Node.js and React, two cornerstone technologies that, when combined, equip developers with the tools to craft modern web applications effectively.
Let’s consider the integration of these two technologies. Node.js provides a robust server-side environment based on JavaScript, enabling developers to build scalable network applications. Meanwhile, React, a declarative JavaScript library maintained by Facebook, focuses on building user interfaces that are not just reactive but also efficient. The juxtaposition of these two allows for fluid communication between the client and server, resulting in a seamless user experience.
As we delve deeper into this article, the aim is to unveil the specific elements that make the integration of Node.js and React vital: their benefits, considerations, and practical implications.
The Evolution of Web Development
Web development has come a long way since the days of static web pages. Initially, developers relied heavily on back-end languages like PHP or Ruby on Rails, but as user expectations evolved, so did the technological landscape. The shift towards dynamic content became apparent; users wanted real-time interactions which static pages just couldn’t provide.
Enter AJAX and then the rise of single-page applications (SPA). Technologies such as JavaScript frameworks emerged to support these requirements, providing users with a smooth experience. Node.js and React represent the culmination of this evolution, where developers can wield the same language across both the server and client sides, tightening the loop and simplifying the overall architecture.
Overview of Node.js
Node.js has carved its niche in web development due to its event-driven, non-blocking I/O model. This architecture makes it lightweight and efficient, a prime candidate for data-intensive real-time applications that run across distributed devices. In less technical terms, it means that Node.js can handle multiple connections simultaneously without breaking a sweat.
What sets Node apart is its JavaScript runtime built on Chrome's V8 engine, which ensures top-notch performance. Developers are finding it intuitive since they can use JavaScript for both front-end and back-end development, effectively homogenizing the development stack. This leads to reduced context switching when working on projects and can speed up development timelines significantly.
Overview of React
On the front-end side, React shines brightly. Its component-based architecture allows developers to break down their applications into small, reusable pieces. This approach not only enhances maintainability but also encourages a more organized structure. Each component can manage its own state, making data flow easier to manage and debug.
React’s rendering model, particularly the concept of a virtual DOM, optimizes performance. Changes in state lead to efficient updates rather than whole-page refreshes, which can slow down user experiences. Furthermore, with the thriving ecosystem of React libraries, such as Redux for state management, developers have a broad canvas to paint their applications.
In summary, Node.js and React together form a synergistic relationship that allows developers to create seamless web applications that meet modern users’ expectations. Strengthening the linkages between server-side processing and frontend user experience is what makes diving into their integration not merely relevant but essential.
"Combining Node.js and React not only streamlines development but also enriches the web experience for users, ultimately leading to more robust applications."
As we proceed through the following sections of this article, expect a comprehensive exploration of these frameworks, delving into their setup, architecture, and effective integration practices.
Understanding the Roles of Node.js and React
Integrating Node.js with React provides a clearer picture of modern web application development. Each technology plays a distinct but complementary role that enhances the overall functionality of web applications. Understanding these roles is crucial not just for developers, but also for anyone who’s looking to grasp how contemporary web systems are built.
Node.js as a Backend Technology
Node.js has carved its niche as a powerful backend technology for building scalable applications. Notably, its event-driven architecture is particularly well-suited for handling asynchronous operations, which is essential in today’s fast-paced web environment. When a request is made, Node.js efficiently serves it without blocking the main thread, making it capable of handling numerous simultaneous connections. This non-blocking characteristic is a game-changer, especially for real-time applications like chat platforms or online gaming.
Another compelling factor is Node.js’s JavaScript runtime, which allows developers to use the same language on both the client and server sides. This uniformity simplifies development as it reduces the cognitive load associated with switching between different languages. If you’re already well-versed in JavaScript, diving into the backend side with Node.js feels like second nature.
The NPM (Node Package Manager) ecosystem further enriches the capabilities of Node.js by providing access to a vast array of modules and packages. Thousands of open-source libraries are at developers’ fingertips, helping to accelerate the development process. For anyone looking to build RESTful APIs, Node.js becomes an attractive choice: it naturally integrates with other JavaScript frameworks and libraries.
React as a Frontend Library
On the flip side, React has transformed the way developers build user interfaces. Its component-based architecture allows for the creation of reusable UI elements that can be utilized across different parts of an application. This promotes consistency and reduces redundancy, making your codebase cleaner and easier to maintain. React efficiently updates and renders only the components necessary, leading to optimized performance, especially in applications with complex user interactions.
React also excels at managing the state of an application through its state management tools, like React Context or even external libraries like Redux. Understanding how React manages state is essential for developers, as this can significantly impact the overall user experience. When combined with Node.js, you can effectively manage the flow of data between the backend and frontend, enhancing interactivity and responsiveness.
By marrying Node.js’s powerful server capabilities with React’s dynamic rendering on the client side, developers have the flexibility to create applications that are not only functional but also engaging. Thus, comprehending the roles played by both technologies is pivotal, as it highlights how they complement each other to create robust, scalable web applications.
"In the digital age, understanding how the tools work together is as vital as knowing the tools themselves."
Setting Up Your Development Environment
Setting up your development environment is a crucial step in integrating Node.js with React. It’s the stage where everything starts to take shape. You can think of it as laying the foundation for a house. If the groundwork isn’t solid, then no matter how beautiful the structure on top might be, it'll always be at risk of crumbling. An effective development environment not only ensures that you have the tools you need but also sets the stage for a smooth workflow.
When you're dealing with both Node.js and React, there are several specific elements you need to consider. Here’s what you should think about:
- Compatibility of the tools: Ensuring that you are using the right versions of Node.js and React is key. An incompatible setup can lead to unexpected issues and headaches.
- Project Structure: Defining a clear project structure right from the get-go helps keep your code organized. This is especially important in larger projects where chaos can easily ensue without a clear plan.
- Version Control: Implementing version control through tools like Git is essential. It allows you to keep track of changes and revert back if necessary, which is a lifesaver during the development process.
With these points in mind, let’s delve deeper into the first steps of establishing your environment with details about installing Node.js and setting up React.
Installing Node.js
Installing Node.js is typically the first action to take in setting up your environment. Node.js serves as the server-side runtime allowing JavaScript to run outside the browser. This makes it an indispensable tool when integrating with React, which is primarily client-side.
To begin the installation:
- Download the Installer: Head over to the Node.js official website and choose the version that best suits your needs. The LTS version is often recommended for stability.
- Run the Installer: Follow the setup wizard steps. This generally means agreeing to the license, choosing your installation path, and selecting the necessary components.
- Verify Installation: After installation, open your command prompt or terminal and run the following commands:This will show you the installed versions of Node.js and npm (Node Package Manager), ensuring everything is correctly installed.
- Set Up Environment Variables (if necessary): Sometimes, you need to configure environment variables, especially on Windows, to ensure that Node.js is recognized globally.
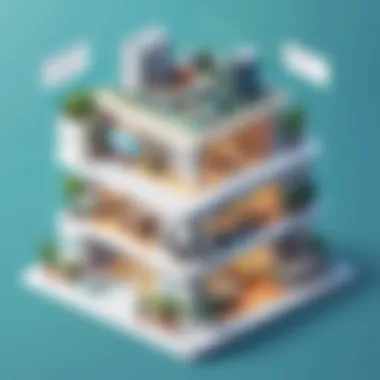
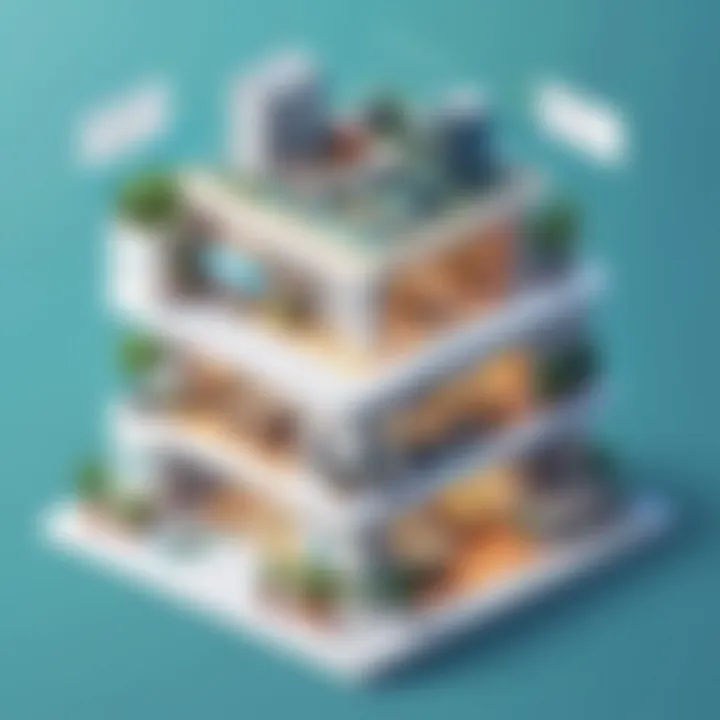
By completing these steps, you’ll have Node.js installed and ready for your React projects.
Setting Up React Using Create React App
Next up is setting up your React environment, and one of the easiest ways to do this is by using Create React App. This command-line tool simplifies the process of setting up the React application by creating a suitable project structure and automatically configuring the build settings needed for development.
Here’s a step-by-step process:
- Open Terminal or Command Prompt: Navigate to the directory where you want to create your new application.
- Run Create React App Command: Input the following command:Replace with your desired application name. Running this command initializes a new React application and installs the necessary dependencies.
- Navigate into Your Project Directory: Once the installation completes, you can navigate into your new app folder using:
- Start the Development Server: Finally, to see your new React app in action, run:This command starts a development server and opens your new app in the default browser.
By using Create React App, you have an excellent starting point without needing to worry about various build configurations. This process greatly enhances productivity and makes it easier for newcomers to dive into React development.
"Setting up your development environment is like sharpening your tools before crafting your masterpiece".
Proper installation and initial setup of tools play a significant role in easing future development tasks. With Node.js and React ready to go, you're equipped to build modern web applications that leverage the power of both technologies.
Basic Architecture for Combining Node.js with React
In the realm of modern web development, understanding the basic architecture for combining Node.js with React is essential. This synergy facilitates the development of dynamic, responsive, and scalable applications. It allows developers to harness the strengths of both technologies, enabling a seamless flow of data and optimized performance.
The architecture generally follows a client-server model, where React operates on the client side while Node.js acts as the server-side backbone. This separation of concerns not only enhances efficiency but also promotes maintainability of the code. By establishing clear boundaries between the frontend and backend, developers can work independently on each component, which leads to faster development cycles and easier debugging.
Key Benefits
- Scalability: With Node.js handling multiple concurrent connections efficiently and React rendering views fluidly, this architecture is robust enough to scale as your application grows.
- Speed: React's virtual DOM minimizes page update times, while Node.js can manage numerous requests without bogging down the server, making the full stack performance snappy.
- Unified JavaScript Development: Using JavaScript in both the frontend and backend streamlines the development process. Developers can share code and libraries between the two, reducing redundancy and enhancing collaboration.
Considerations
There are certain factors to keep in mind when designing a basic architecture for Node.js and React applications. The first is ensuring that RESTful principles are adhered to when constructing APIs. This keeps the architecture clean and understandable. Additionally, developers should account for authentication and data security from the outset. A comprehensive security strategy ensures that user data is protected and that communication between the client and server is secure.
Ending
In sum, understanding the basic architecture is a stepping stone in the journey to effectively integrating Node.js with React. Developers who grasp these foundational concepts can build not only effective applications but also foster better collaboration within their teams.
"An architecture that blends the responsiveness of React with the scalability of Node.js can significantly improve the user experience."
By establishing a strong architectural foundation, developers can delve deeper and harness the true potential of these two powerful technologies.
Client-Server Architecture Overview
The client-server architecture provides the framework through which Node.js and React communicate, enabling a structured exchange of data. At its core, the architecture delineates two distinct roles: the client, which is primarily handled by React, and the server, typically managed by Node.js.
In this setup, the client sends requests to the server for data or actions. This is where RESTful APIs come into play. They act as intermediaries, transmitting requests from the client to the server and delivering responses back.
Key Roles in Client-Server Architecture:
- Client (React): Responsible for the user interface, handling user inputs, and rendering content dynamically based on data received from the server.
- Server (Node.js): Processes incoming requests, fetches data from databases, and sends back the appropriate responses. This could involve CRUD operations and more complex logic.
Communication Between Frontend and Backend
Establishing robust communication between the frontend and backend is vital for any successful web application. In a typical React and Node.js setup, this interaction is managed through a series of well-defined API endpoints.
- HTTP Protocol: Most interactions between React and Node.js use the HTTP protocol. This simplicity allows for straightforward requests and responses, typically in JSON format.
- Asynchronous Requests: Using libraries like Axios or Fetch API within React, developers can make asynchronous requests to the Node.js server without blocking the user interface. This ensures a smoother user experience, as users can continue to interact with the application while waiting for data.
- WebSockets for Real-Time Communication: For applications that require immediate data updates—like chat applications or live notifications—using WebSockets provides a persistent connection between the client and server. Node.js excels at this thanks to its non-blocking I/O model.
Data Handling and State Management
Data handling and state management are crucial components in the realm of web development, particularly when integrating Node.js with React. The way data flows between the backend and frontend determines not only the efficiency of the application but also its responsiveness and user experience. Understanding the nuances of managing state and fetching data lays the groundwork for building applications that are both performant and scalable.
Correctly managing state helps in tracking the changes over the lifecycle of a component and ensures the application reflects those changes in real-time. This is important, as a poorly managed state can lead to inconsistent UI behavior, making the application feel sluggish or unresponsive. Similarly, effective data handling practices ensure that applications can easily communicate with the server, managing requests and responses efficiently.
Managing State with React
In React, state management can take various forms. One common approach is using React’s built-in state management through the and hooks. These allow developers to keep track of local component states without much hassle. Additionally, when the complexity of the application increases, managing state with libraries like Redux or Context API becomes pertinent.
For instance, consider a shopping cart application:
- When a user adds an item, the state is updated to reflect this change.
- If you want to open a modal displaying the cart contents, the state managing whether the modal is open or not needs to be efficiently handled.
In this scenario, if state changes are not handled properly, it could lead to a frustrating user experience. Components might re-render more times than necessary, causing performance hiccups. A clear understanding of which state changes trigger what parts of the UI to update is essential. This can optimize rendering cycles and enhance performance.
Fetching Data from Node.js
Fetching data in a React application usually involves making requests to a Node.js backend. This process typically involves HTTP requests using libraries like Axios or the Fetch API. A common flow is as follows:
- Sending a Request: As soon as a component mounts or based on user interaction, a request is sent to the Node.js server to fetch, say, user profile data.
- Handling Responses: Once the response is received, the React state is updated accordingly.
- Displaying Data: Finally, the relevant data is rendered within the component.
Here’s an example using :
This code snippet exemplifies how a GET request can be initiated to a Node.js server and how the result is stored in the component’s state. However, it’s important to handle potential errors, such as connection issues or unexpected data formats, to ensure the application remains robust and user-friendly.
In summary, effective data handling and state management strategies play a pivotal role in developing efficient applications with Node.js and React. They not only improve the user experience but also enhance the maintainability of the application by creating predictable, easy-to-understand code.
Creating RESTful APIs with Node.js
When it comes to developing dynamic web applications, understanding how to create RESTful APIs with Node.js is nothing short of essential. REST, which stands for Representational State Transfer, is an architectural style that governs how data is exchanged over the web. Building APIs that adhere to REST principles allows developers to create applications that are not just functional but also efficient and scalable.
Understanding REST Principles
To grasp RESTful APIs, it's first important to understand what REST involves. It revolves around a few core principles that impact design and usability:
- Statelessness: Each HTTP request from a client must contain all the information the server needs to fulfill that request. This means no client context should be stored on the server, allowing for improved scalability.
- Resource-Based: Everything in a REST API is considered a resource. Resources are accessed via URIs, typically using standard HTTP methods like GET, POST, PUT, and DELETE.
- Representation: Clients interact with these resources by exchanging representations, typically in formats like JSON or XML. For Node.js applications, JSON is a common choice due to its lightweight nature and compatibility with JavaScript.
These principles guide the construction of APIs that are intuitive and maintainable. They facilitate communication between the frontend, often built with React, and the backend powered by Node.js, creating a smooth development workflow.
Building a Simple API
The process of building a simple RESTful API using Node.js is straightforward yet powerful. With the help of frameworks like Express.js, developers can easily set up an API that serves various client requests. Here’s a look at how to create a basic RESTful API:
- Setting Up Your Node Environment: Start by creating a new directory for your project. Run to create a new package.json file.
- Installing Dependencies: Use npm to install Express by running:Express is a minimal and flexible Node.js web application framework that provides a robust set of features for building web and mobile applications.
- Creating the API: Create a file called , where your Express application will reside. Here’s a basic template to get started:This simple code creates a server that listens on port 3000 and responds with a JSON message when a GET request is made to .
- Testing the API: To validate your API, you can use tools like Postman or curl. Making a simple GET request to should return:
Using these steps, you can build out more complex APIs by adding additional routes and logic, enhancing data processing, and integrating with databases.
Key Takeaway: Creating RESTful APIs with Node.js streamlines the interaction between your backend and frontend technologies, empowering you to develop scalable and efficient web applications. Understanding the principles of REST is only the beginning; mastering them opens doors to enhanced development workflows and better user experiences.
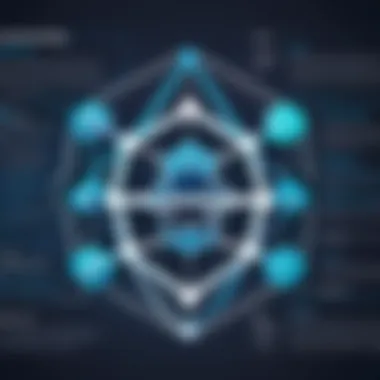
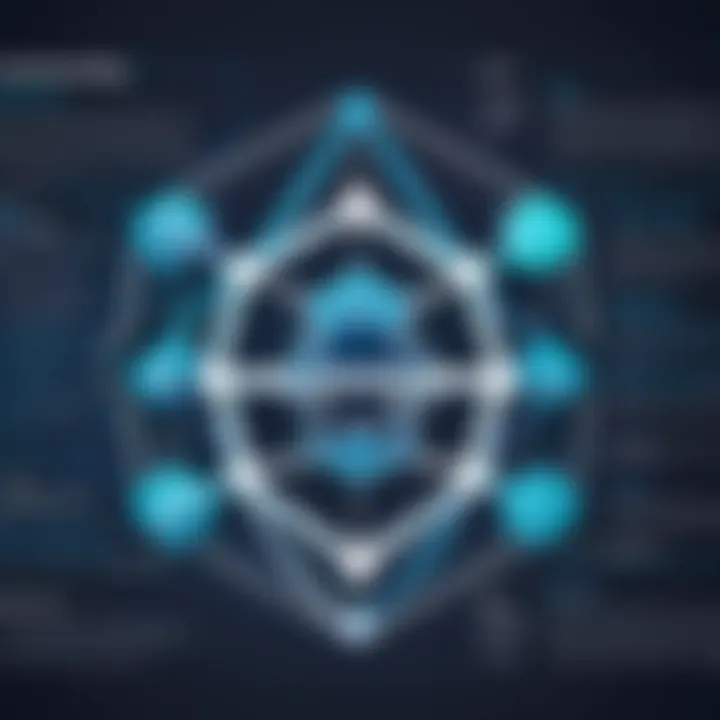
Overall, this section highlights both the significance of RESTful APIs and provides a framework for building them with Node.js, setting the stage for deeper exploration of technologies that can interconnect with React.
Authentication and Security
In the realm of modern web applications, the intersection of Node.js and React brings both vast opportunities and significant challenges, particularly in the domain of authentication and security. With web applications routinely handling sensitive user data—from credit card numbers to personal identity information—ensuring a robust authentication mechanism is paramount. This section delves into the essentials of authentication strategies in Node.js and explores measures to secure React applications, aiming to build trust in users while safeguarding their information.
Implementing Authentication in Node.js
Authentication in Node.js usually revolves around identifying users effectively while allowing secure access to various resources. Here are key aspects to consider:
- User Verification: Users need a way to identify themselves, commonly through a username and password. Using libraries like Passport.js can simplify this process, providing a range of strategies to authenticate users.
- Tokens for Session Management: Instead of traditional session cookies, many applications today employ JSON Web Tokens (JWT). When a user successfully logs in, a JWT is generated and sent to the client. On subsequent requests, this token is sent back for verification, granting access to secured routes.
- Hashing Passwords: It’s vital to never store plain-text passwords. Instead, use hashing algorithms like bcrypt to encrypt user passwords before they are saved to the database. Implementing salting techniques here is equally important.
- Role-based Access Control (RBAC): Define roles for users based on their responsibilities within the application. This can range from admin rights to regular user permissions. Such a strategy helps control access at various levels, reinforcing the security perimeter.
Implementing robust authentication can feel like preparing a fortress against attackers, yet failing to follow these practices could leave a wide-open gateway.
Securing React Applications
As much as Node.js forms the backbone of your web application, React is its face. However, with great power comes significant duties; hence, security on this front is crucial too. Here are some best practices for securing React applications:
- Environment Configurations: Keep sensitive information like API keys and secrets outside of your frontend code. Store such data in environment variables and access it via your backend.
- Cross-Site Scripting (XSS) Protection: Ensure that user input is sanitized and validate data before rendering it on the UI. Using libraries like DOMPurify helps strip out any malicious script tags from user inputs.
- CSRF Protection: Implement Cross-Site Request Forgery (CSRF) tokens to ensure that requests to your backend are legitimate. Libraries like can aid in setting this up.
- Use HTTPS: Ensure your applications are served over HTTPS. This encrypts the data between the client and server, making it extremely difficult for attackers to intercept sensitive information.
- Regular Dependency Audits: Conduct audits of your npm packages to identify and mitigate vulnerabilities. Utilizing tools like npm audit can help flag potential security issues in your dependencies.
Remember, the cost of a security breach is often higher than the investment made in implementing proper security measures.
By weaving these strategies into the fabric of your Node.js and React integration, you will be taking significant strides toward creating a more secure application, thereby fostering trust with your users.
Deploying Node.js with React Applications
Deploying applications built on Node.js and React brings a new set of challenges and considerations that developers need to face head-on. Missteps in this stage can lead to performance bottlenecks, security vulnerabilities, or even downtime. Thus, understanding how to deploy Node.js with React effectively is crucial for sustaining an application’s lifecycle and enhancing user experience. This section delves into the essential elements of deployment, emphasizing critical benefits and practical considerations that can streamline the process, ensuring a seamless transition from development to production.
Choosing the Right Hosting Solution
When it comes to hosting a combined Node.js and React application, the choices made can either catapult the performance or hinder it. It’s akin to picking the right vehicle for a long journey—certain options can make the trip enjoyable and efficient, while others can turn it into a slog.
Key Factors to Consider:
- Scalability: Your hosting provider should support easy scalability—whether that’s vertical or horizontal. As your user base grows, you don’t want to hit a wall.
- Performance: Low-server latency and quick response times are non-negotiable.
- Cost: Balancing performance with budget is vital. Some platforms might offer premium features at a price that could break the bank.
- Ease of Use: The hosting solution should offer simple deployment processes and good documentation for fast resolution of issues.
Popular hosting platforms include Heroku, AWS, DigitalOcean, and Vercel, each presenting unique advantages depending on your needs. Heroku stands out for its user-friendliness, while AWS often wins the scalability game. It’s about matching the capabilities of the hosting platform to the demands of your application.
Note: Always review the latest guidelines and compare features before making a decision.
Continuous Integration and Delivery
Implementing continuous integration (CI) and continuous delivery (CD) in your Node.js and React deployment process can't be overstated. It’s the linchpin that helps you automate the workflow—from code integration through testing to deployment—making the entire process smoother and more reliable.
Benefits of /CD:
- Fewer Bugs: Regular testing can uncover issues sooner rather than later.
- Faster Release Cycles: You can roll out updates more frequently, capturing user feedback in real-time.
- Increased Efficiency: Automation minimizes human error and manual work, freeing developers to focus on core features.
A typical CI/CD pipeline for a Node.js and React application might look something like this:
- Source Code Management: Version control (like Git) helps manage changes and keeps the project organized.
- Build and Test Automation: Every change triggers automated builds and tests, ensuring code stability.
- Deployment Automation: Successful tests lead to automated deployments, eliminating manual overhead.
Tools like Jenkins, Travis CI, or CircleCI work wonders for managing CI/CD processes. They can hook up directly with your Git repositories, simplifying the integration with various API and deployment workflows.
In summary, deploying Node.js applications with React efficiently elevates your development game and enhances the ecosystem you’re working in. Selecting a hosting solution and implementing effective CI/CD practices aren’t just operational necessities—they’re pillars for achieving a robust, scalable, and modern web application.
Testing Strategies for Node.js and React
In the world of software development, testing is the backbone that supports the stability and robustness of applications. With the rise of Node.js and React in modern web development, understanding and implementing effective testing strategies has never been more crucial. Testing not only helps in identifying bugs or issues early in the development process but also enhances the overall quality of the application. In this section, we delve into the various aspects of testing these technologies, focusing on unit testing with Jest and integration testing scenarios, ultimately helping you build a resilient application.
When integrating Node.js with React, establishing a comprehensive testing strategy is essential. It promotes confidence among developers and stakeholders. Many consider thorough testing as a safety net that allows for rapid changes and feature additions without the impending dread of breaking existing functionality. Therefore, the commitment to testing should be embedded into the development process from day one.
Unit Testing with Jest
Unit testing is a fundamental aspect of the testing lifecycle. It involves testing individual components or functions in isolation to ensure they work as expected. When it comes to Node.js and React, Jest is a standout framework that has gained popularity due to its simplicity and power. This testing framework is particularly beneficial for its ease of setup and built-in mocking capabilities, allowing developers to conduct tests without needing to worry about the behavior of external dependencies.
For instance, let’s consider a simple scenario in which you have a React component that displays a user’s name. Using Jest, you can write a unit test to verify if this component renders the name correctly. The following is a basic illustration of how this unit test might look:
This straightforward code checks that the component renders the correct content based on the name prop passed to it. Such tests not only ensure that your components behave as intended but also serve as documentation for future developers who might look at your code later.
Maintaining a suite of unit tests means that as your application evolves, existing features remain intact while new functionality is introduced. Developers don’t have to fear breaking something that already works; instead, they can move ahead with confidence.
Integration Testing Scenarios
While unit tests focus on individual components, integration testing assesses how these components behave when combined. It evaluates the interaction between different parts of the application, particularly the frontend managed by React and the backend powered by Node.js.
Integration testing ensures that all the pieces fit together correctly and that the data flows as expected between the client and server. For example, if you have an API endpoint in Node.js that sends user data, integration tests can verify that your React components correctly receive and display this data.
When you get into integration testing, tools like Jest along with libraries such as Enzyme or React Testing Library come in handy. To illustrate, let's assume there is an API that fetches user information when a component mounts. Your integration test might look like this:
This test checks not only if your component correctly makes a request to the API but also whether it handles the response properly. It validates the connection between your frontend and backend, something that unit tests alone can’t accomplish.
Effective testing strategies are the hallmark of robust applications. They provide a safety net, ensuring that developers can innovate and iterate without risk. It's not just about finding bugs; it's about embracing quality as a culture in your development workflow.
Common Challenges and Solutions
In the realm of web development, integrating Node.js with React can feel like navigating a ship through a stormy sea. Sure, the end goal is to build a robust application, but there are quite a few waves to ride along the way. From handling asynchronous practices to addressing error management, developers often encounter multiple hurdles that can throw a wrench in the works. This section delves into some common challenges developers face and presents practical solutions to keep your project afloat.
Dealing with Asynchronous Practices
Asynchronous programming is a cornerstone of modern JavaScript and plays a vital role in Node.js and React. At its core, it allows developers to write non-blocking code, enabling multiple operations to run simultaneously. However, mastering async behavior can be tricky. One minute you're sailing smooth, and the next, you're tangled in a mess of callbacks, promises, and async/await—all of which can complicate flow.
Here are a few elements you need to consider when dealing with asynchronous practices:
- Callback Hell: When multiple functions call one another, the code can become cluttered and hard to read. This results in what's known as "callback hell." To tackle this, use promises or opt for async/await syntax, which enhance readability and make debugging easier.
- Error Handling in Async Code: Mistakes can happen—it's the nature of coding. When dealing with asynchronous functions, ensure you catch errors effectively. Within a block in async/await, you can manage errors in a cleaner manner than chaining on promises.
- Data Fetching: React components often rely on asynchronous data fetching from your Node.js backend. Using hooks like and dependency arrays can help manage the timing of when your data is fetched. This keeps your application responsive and avoids unnecessary state updates.
Remember: Effective management of asynchronous practices ensures your application runs like a well-oiled machine.
Error Handling Strategies
Error handling should never be an afterthought. It deserves its spot in the spotlight. Whether you're working within Node.js or React, having a solid error-handling strategy can save you a heap of trouble down the line.
Common strategies include:
- Centralized Error Handling: Set up a centralized error-handling middleware in your Node.js application. This way, you can streamline how errors are managed and presented to users. Instead of scattering error handling throughout your code, a single entry point can enhance maintainability.
- Graceful Failures: Make it a habit to anticipate possible failure scenarios and design your app to fail gracefully. This can involve returning meaningful messages back to the user, instead of a generic error page. For instance, notifying users when there's an issue with data fetching is often better than leaving them in the dark.
- Logging Errors: Consider logging errors for monitoring and debugging purposes. Implement tools like Winston for Node.js to capture errors and log them effectively. This not only helps in diagnosing issues but also aids in improving application performance.
- React Error Boundaries: In React, employ error boundaries to catch rendering errors in child components. They act as a safety net that keeps the rest of your application functional even if one part breaks.
In summary, addressing challenges in Node.js and React integration isn't just about quick fixes; it's about establishing a framework that anticipates issues and handles them in a systematic way. By doing so, not only do you ensure higher quality code but also offer a better experience for users engaging with your application.
Performance Optimization
Performance optimization is a critical aspect of developing applications with Node.js and React. As the digital landscape continues to expand, users expect applications to be fast and responsive. When integrating these technologies, developers must emphasize optimizing both the server-side and client-side performance to ensure a seamless user experience.
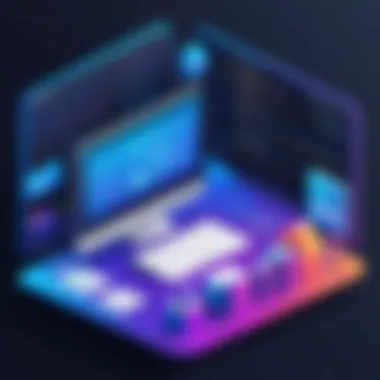
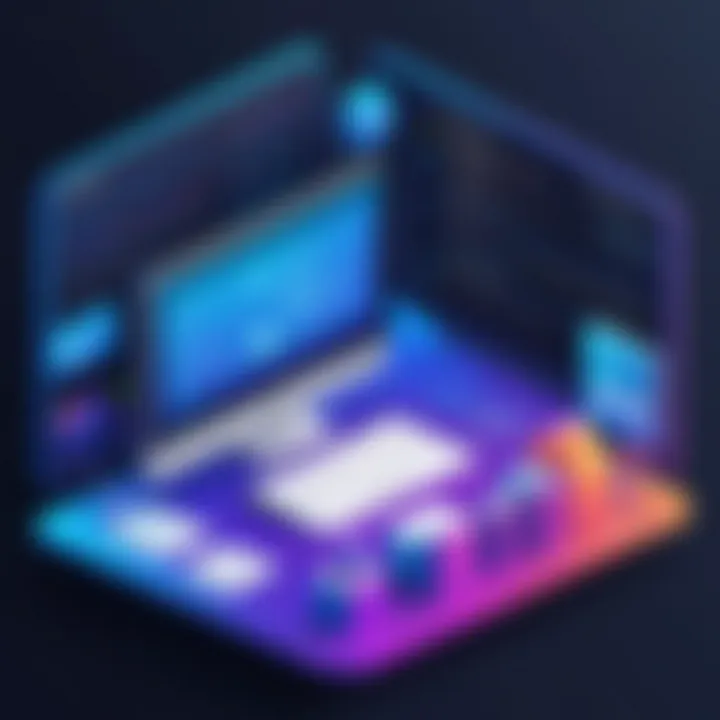
The key benefits of performance optimization include reduced load times, improved user experience, and better resource utilization. With faster applications, users are more likely to stay engaged, which can lead to higher conversion rates. It's imperative to consider how optimizations at one level can impact the other; for instance, a delay in backend processing can lead to sluggish frontend performance. Therefore, honing in on optimizing for performance should be a priority throughout the development process.
Optimizing Server Performance
When it comes to optimizing server performance in Node.js, there are several strategies developers can implement. To start, leveraging asynchronous programming can immensely enhance the way your server processes requests. Node.js operates on a single-threaded architecture, which is efficient but can become overwhelmed by blocking operations. Using callbacks, promises, or the more modern async/await syntax allows developers to handle multiple requests without the dreaded bottleneck.
Besides that, it’s also fundamental to make use of caching mechanisms. Caching prevents the application from needing to perform expensive computations or database queries for frequently requested data. Technologies like Redis or even in-memory caching can significantly reduce response times.
It's also wise to understand how your application performs under various loads. Load testing tools, such as Apache JMeter or Artillery, can help gauge server responsiveness and uncover potential performance pitfalls before they affect users. By addressing these performance concerns proactively, you can create a more resilient application that stands up to user demands.
"A stitch in time saves nine" – addressing performance issues early on can prevent larger problems down the line.
Optimizing React for Performance
On the frontend, when working with React, there are numerous ways to ensure the application runs smoothly. One prominent method is the use of React’s built-in features like and . These tools allow developers to minimize unnecessary re-renders, which can significantly speed up the app’s responsiveness, resulting in a snappier user experience.
Moreover, code-splitting is an excellent technique for improving load times. By breaking large bundles into smaller, more manageable chunks, React applications can load only the necessary components when needed. Libraries like and make implementing code-splitting straightforward and effective.
Another vital aspect to consider is optimizing asset delivery. Using tools such as Webpack, developers can gzip their files or utilize tree-shaking to eliminate unused code. This practice minimizes the size of the JavaScript bundles, which can lead to faster download times and improve overall performance.
Additionally, effective management of state using tools like Redux or Context API should not be overlooked. Maintaining a predictable state can help avoid redundancy and unnecessary updates, allowing for a more efficient rendering process.
In summary, optimizing both server and React performance not only enhances the user experience but also positively impacts how the application interacts with users. By integrating these optimizations across the full stack, developers position themselves to create fast and competitive applications in today’s fast-paced environment.
Scaling Applications Effectively
Scaling applications effectively is a hot topic in the realm of web development, especially when you're looking to enhance performance and maintainability. As your application grows, you’ll find that what worked for a small user base may not hold up when the traffic ramps up. This section delves into why understanding scalability is pivotal in your Node.js and React integration journey.
The importance of scaling lies in ensuring that your application can handle growth without sacrificing performance. Scalability is not just about being able to handle more users; it encompasses the ability to manage increased loads on resources such as servers, databases, and network bandwidth. Plus, a well-scaled application does wonders for user experience. Slow loading times or outages can drive users away faster than you can say "buffering."
Some specific elements that contribute to effective scaling include:
- Load Balancers: These nifty tools distribute incoming traffic across multiple servers, helping to prevent any single server from becoming a bottleneck.
- Caching Mechanisms: By storing frequently accessed data in a temporary storage area, caching reduces the time it takes to retrieve that data, thus speeding up response times for users.
- Database Optimization: Databases can become quite the overhead if not designed for scale. Employing techniques like indexing, partitioning, or moving to a NoSQL solution can help.
- Microservices: Splitting your application into smaller, independent services allows for easier management and scaling.
In practice, effective scaling allows you to deploy updates with minimal disruption. Imagine a mobile app that becomes popular overnight. If your backend isn’t prepared for the surge, users could experience downtime, leading to negative reviews. Good scalability isn’t merely desirable; in a competitive market, it’s essential.
"The biggest pitfall for a growing application is underestimating the traffic it could face."
Understanding Scalability Concepts
Now, let’s unpack what scalability actually means. Scalability can be thought of in terms of two primary types: vertical and horizontal. Vertical scaling, or scaling up, involves adding more power (CPU, RAM) to an existing machine. It’s simple but has limits, as physical servers can only support so much upgrade.
On the other hand, horizontal scaling, or scaling out, involves adding more machines into your pool of resources. This method is generally more sustainable in the long run as it aligns better with distributed computing practices. Horizontal scaling is the direction that modern architectures, particularly in cloud environments, are headed.
Ultimately, a well-structured understanding of these concepts translates into applying best practices in your Node.js and React integration. Think of it this way: if you aim for horizontal scaling, your application architecture must be suited for distributed computing from the get-go.
Microservices Architecture Considerations
When considering scaling, diving into microservices architecture could be a game-changer. Rather than having one monolithic app, microservices break functionalities into smaller, manageable services. Each service can then be developed, deployed, and scaled independently.
Some considerations to keep in mind include:
- Service Independence: Failure in one microservice doesn’t cripple the entire system, allowing for better fault tolerance.
- Technology Diversity: Different microservices can be built using different languages or frameworks, which gives you the flexibility to use the right tool for the job.
- Data Management: Each microservice usually manages its own data, which can lead to a more efficient handling of data-related tasks. However, it does come with its own set of challenges around ensuring data consistency across services.
- Deployment Complexity: With more moving parts comes the need for sophisticated deployment strategies. Using services like Kubernetes can help automate these deployments.
Exploring Real-World Use Cases
In the realm of web development, bridging the gap between theory and application is vital. This is where exploring real-world use cases becomes a cornerstone in understanding the integration of Node.js with React. Real-world examples not only illustrate the practical utility of the combined technologies, but they also offer insights into best practices and pitfalls that developers commonly face.
Successful Application Examples
Many organizations have harnessed the power of Node.js and React to create robust applications that drive engagement and improve user experience. For instance, Netflix employs Node.js to handle their server-side operations, while React is pivotal in delivering a seamless viewing experience. This synergy allows Netflix to efficiently manage massive volumes of data while providing users with an interactive and dynamic interface.
Another notable example is Airbnb. The company leverages Node.js to support the back-end services that manage bookings and user interactions, ensuring that the system can scale as user demand fluctuates. React, on the other hand, is used to build their client-side interfaces, facilitating a fluid experience for users as they navigate listings and communicate with hosts.
These examples underscore how enterprises capitalize on the strengths of both technologies. Using Node.js for server-side performance combined with React's responsive UI capabilities can drastically enhance the speed and accessibility of applications while accommodating significant traffic.
Industry Adaptations
The adaptability of Node.js and React is evident across numerous industries, including e-commerce, education, and healthcare. In the e-commerce sector, platforms like eBay and Shopify have integrated Node.js and React to create faster, more responsive online shopping experiences. By managing backend processes through Node.js, these businesses can handle numerous transactions simultaneously, ensuring quick load times and efficient inventory management. React further enhances this by creating interactive product pages that elevate user engagement and satisfaction.
In education, institutions are increasingly using this combination for learning management systems. For example, Coursera employs Node.js on its backend to support user registrations and course management. The frontend, built with React, provides learners with a clean and immersive interface that makes accessing educational content straightforward and intuitive.
The integration of Node.js and React showcases a flexible approach to development, addressing various needs across different sectors while enhancing user engagement.
As seen, the benefits of integrating Node.js with React are vast and evident in real-world applications. They allow developers to build scalable, efficient, and user-friendly systems. Understanding these applications can inspire developers to innovate and push the boundaries of what's possible in their projects.
As developers dive into the practicalities of integration, these examples and adaptations provide a blueprint for harnessing the potential of these technologies in their work.
Future Trends in Node.js and React.
The landscape of web development is a fast-changing arena, especially with technologies like Node.js and React at the forefront. Understanding the future trends in these technologies is crucial for developers who want to remain relevant and competitive in the field. As the demand for interactive applications grows, so do the expectations around performance, scalability, and developer experience. In this section, we explore how emerging technologies and frameworks are shaping these ecosystems alongside the expanding community that supports them.
Emerging Technologies and Frameworks
Emerging technologies are continually reinventing how Node.js and React function together. New frameworks and tools are appearing all the time, each adding layers of functionality that can enhance productivity and streamline development processes. For instance, consider frameworks like Next.js. This framework enables developers to create server-rendered React applications seamlessly, which is paramount for improving user experience by loading pages faster.
- Typescript: Developers are leaning more towards TypeScript for type safety in large applications, resulting in more maintainable code and fewer runtime errors.
- GraphQL: This API query language presents an alternative to REST. It allows for more efficient data retrieval by fetching only the necessary data, thus optimizing the communication between Node.js and React applications.
- Serverless architecture: Using platforms like AWS Lambda, developers can run backend code without managing servers, leading to greater scalability and cost-effectiveness.
These technologies not only streamline the development process but also open up possibilities for more advanced applications that can adapt to user demands. With tools like React's hooks and Node.js middleware, building powerful applications is becoming increasingly achievable—if one stays informed about these cutting-edge solutions.
The Growing Community and Ecosystem
As Node.js and React continue to evolve, so does the size and dynamism of their communities. The mutual support within these communities fosters an environment ripe for innovation and learning. Developers are now more than just users of technology; they are contributors, helping to identify issues, suggesting features, and enhancing frameworks.
Utilizing platforms like GitHub and Reddit, developers can share knowledge and access a wealth of resources, such as:
- Open-source libraries: These add-ons help in solving common problems efficiently without needing to reinvent the wheel.
- Forums and discussion groups: Engaging in conversations allows developers to stay updated on best practices, and bug fixes, and share insights about new trends.
Moreover, the growing ecosystem composed of various libraries, plugins, and tools builds on the community’s contributions. This creates a cycle of continuous improvement and enrichment for both Node.js and React users. Environments like Facebook's React Native also exemplify this growth as it encourages mobile app developers to explore familiar concepts in a new setting.
"Staying engaged with the community can provide not just solutions but also inspiration to tackle complex problems with innovative approaches."
In summary, the future of Node.js and React is intertwined with the rise of emerging technologies and a collaborative community spirit. Both aspects are essential for any developer keen on mastering these tools and preparing their skill sets for what lies ahead.
Culmination and Next Steps
In wrapping up this comprehensive exploration of integrating Node.js with React, it's clear that understanding how these technologies complement each other is crucial in today’s fast-paced web development landscape. Node.js serves as a robust backbone, allowing for efficient and scalable server-side operations. Conversely, React shines brightly on the frontend, handling user interactions with flair and efficiency. Merging these two not just streamlines the development process but also enhances user experience, making it paramount for developers to grasp their integration thoroughly.
Moving forward, one must consider a few key points. First, it's all about developing a solid grasp of each platform individually. Without understanding the strengths and limitations of both Node.js and React, it can be like trying to mix oil and water. Next, as you experiment and build applications, be mindful of best practices, especially in managing state and optimizing performance—these are the breadcrumbs that lead to a well-oiled machine.
Furthermore, as technology continues to evolve, so should your approaches and understanding. Staying abreast of new libraries, frameworks, and tools will keep your skills relevant. Overall, this journey doesn't end here; think of it as an evolving road where you continually learn and adapt.
"Programming isn't just about writing code; it's about solving problems and creating solutions that improve lives."
What’s next? Engage with the community on platforms such as reddit.com where you’ll find discussions, advice, and shared experiences about integrating Node.js with React. Connect with likeminded individuals who share a passion for technology.
Lastly, don’t forget to apply your knowledge practically. Build projects, contribute to open-source, and challenge yourself to create more complex applications. This hands-on experience is invaluable and brings all the concepts discussed throughout this guide to life.
Summary of Key Takeaways
- Node.js and React are complimentary: Understanding how Node.js facilitates backend operations while React takes charge of the user interface can help create efficient applications.
- Best Practices Matter: State management, performance optimization, and error handling are all critical for smooth user experience and application reliability.
- Keep Learning: The landscape is in constant flux; immersing yourself in community-driven platforms and staying updated on emerging tools could set you apart.
Resources for Continued Learning
- Node.js Official Documentation: A comprehensive resource for all things Node.js, including API reference and guides.
- React Official Documentation: The best starting point to dive deeper into React’s components and architecture.
- FreeCodeCamp: Offers valuable exercises and projects centered on both Node.js and React, great for hands-on learning.
- W3Schools: For quick and understandable tutorials covering web development essentials, including both frameworks.
By utilizing these resources, aspiring developers can not only solidify their knowledge but also explore new concepts and innovations within web development.