Integrating Node.js with MySQL for Modern Development
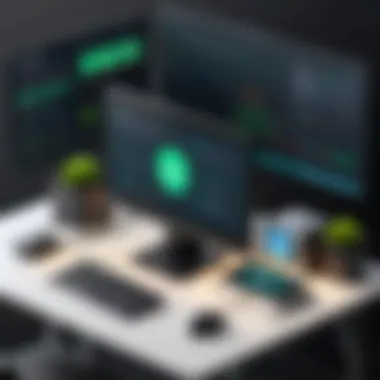
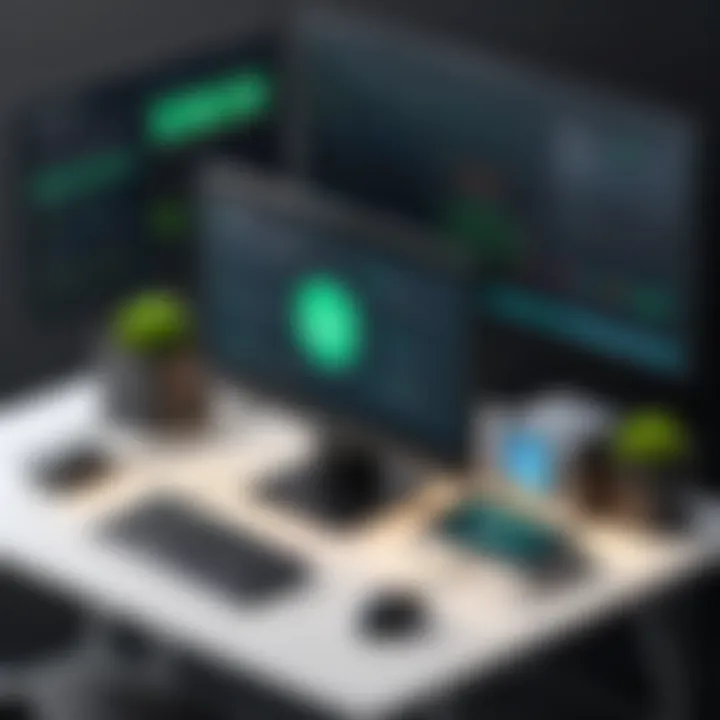
Prolusion to Node.js and MySQL Integration
In the fast-paced world of web development, a robust back-end setup is crucial for ensuring seamless data management and application performance. Node.js has become a powerful choice for server-side programming, allowing developers to build scalable network applications with ease. When paired with MySQL, a popular relational database management system, they form a strong duo capable of handling various data needs in an application.
Integrating Node.js with MySQL offers several advantages, including non-blocking I/O capabilities, a rich ecosystem of libraries, and the efficiency of JavaScript across both front-end and back-end development. This means developers can employ a single language throughout the stack, promoting consistency and simplifying the learning curve.
But how do you get started? In this article, we’ll navigate through setting up your Node.js environment, connecting to a MySQL database, and executing essential database operations—all while considering error management and optimization techniques. Let's delve into the topic to uncover the depth of Node.js and MySQL integration for aspiring programmers.
Setting Up Node.js
Before diving into the connection with MySQL, you'll need to establish a suitable Node.js environment. Here’s a simplified path:
- Download and Install Node.js:
- Verify Installation:
- Create Your Project Directory:
- Initialize Node Project:
- Head over to the Node.js website and choose the version that suits your operating system. The LTS version is typically recommended for stability.
- Open your command line interface and run:
- This ensures that both Node.js and npm (Node Package Manager) are installed properly.
- Organize your workspace by creating a dedicated folder for your project:
- Use npm to create a package.json file, which helps manage dependencies:
With your Node.js environment freshly minted, it’s time to bring in MySQL.
Connecting to MySQL
Connecting Node.js with a MySQL database requires the use of a library. The most common choice is or . To install the library, run:
Next, create a connection to your MySQL database:
This sets the stage for you to start interacting with your MySQL database through Node.js, enabling you to run queries and manage data directly within your application.
CRUD Operations
Once you’re hooked up with MySQL, it’s time to implement basic CRUD (Create, Read, Update, Delete) operations. Here’s a quick overview:
- Create: Inserting new entries into a table.
- Read: Fetching data from a table.
- Update: Modifying existing records.
- Delete: Removing records from a table.
Example of a simple CRUD operation:
Handling these operations effectively is vital to ensuring your application runs smoothly, especially as it scales.
Managing Errors
Error management is a crucial aspect of development. Understanding how to handle errors will make your application more resilient and user-friendly. Instead of crashing your app, provide informative outputs for easier debugging:
- Use callback functions to handle errors in queries.
- Utilize try-catch statements when dealing with promises or async/await patterns.
For example:
Culmination
By now, you should have a sturdy foundation for integrating Node.js with MySQL. Armed with knowledge about setting up your environment, connecting to the database, performing CRUD operations, and managing errors, you're well on your way to becoming more adept in back-end development.
Further learning will only deepen your understanding and enhance your skills. Stay curious, explore additional resources, and keep pushing your boundaries in the fascinating realm of web development.
Prologue to Node.js and MySQL
In today's digital landscape, where responsiveness and agility are paramount, understanding the relationship between Node.js and MySQL is critical for anyone delving into back-end development. This introduction highlights the seamless integration of a JavaScript runtime environment with a robust relational database management system. Together, they form a powerful combination that has garnered widespread adoption across various industries.
Node.js is designed to facilitate the execution of JavaScript code on the server side, thereby enhancing the efficiency of web applications. Meanwhile, MySQL is one of the most popular database systems, providing a reliable means to store, manage, and retrieve data with efficiency and speed. The synergy between these two technologies opens up a multitude of possibilities, allowing developers to build responsive and scalable applications.
This section will address key aspects of both technologies, examining their functionalities, benefits, and relevance in modern web applications.
Understanding Node.js
Node.js operates on a non-blocking, event-driven architecture, which makes it an excellent choice for building scalable network applications. Many programmers appreciate its use of JavaScript, a language they may already know from client-side scripting. A distinguishing feature of Node.js is its ability to handle a high number of connections concurrently, making it well-suited for real-time applications.
However, while Node.js may seem straightforward to grasp due to its JavaScript foundation, it brings along unique concepts and paradigms that can be quite different from traditional programming models. For instance, understanding how to efficiently manage asynchronous operations becomes vital to harnessing its true potential.
What is MySQL?
Let's switch gears and look at MySQL, an efficient open-source database that plays a central role in many applications. As a relational database management system, it organizes data into tables using structured query language (SQL) for interaction. This organization allows for complex querying capabilities, supporting data integrity and relationships through robust ACID compliance.
User friendliness is one of MySQL's strong suits. The extensive documentation, alongside a plethora of community support, means developers can quickly get started. However, there's gumption involved, as one must also dive deep into database design principles and optimization techniques to maximize performance.
The Role of Databases in Web Applications
Databases serve as the backbone of nearly every web application. Specifically, they enable applications to persist data, allowing for dynamic interactions and user engagement. Imagine an e-commerce website: it wouldn’t be possible to track orders or store user profiles without the backing of a solid database.
In terms of data integrity and security, having a reliable database system like MySQL is critical. Applications that manage sensitive information, such as personal data or payment details, must knowingly prioritize securing that data. Furthermore, the performance dynamics between Node.js and MySQL can significantly impact application responsiveness and user satisfaction.
Setting Up Your Development Environment
Setting up your development environment is like laying a solid foundation before building a house. Without a robust framework in place, your programming endeavors might face unexpected hurdles down the road. When integrating Node.js and MySQL, having the right setup is crucial because it not only enhances your productivity but also minimizes errors and streamlines the development process. This ensures that you spend more time focusing on building features rather than troubleshooting configuration issues.
Installing Node.js
Installing Node.js is the first step in your setup journey. Node.js provides a runtime environment allowing you to execute JavaScript on the server side. This is significant because JavaScript is traditionally a front-end language, and Node.js opens up a whole new world for backend programming.
To install Node.js, follow these steps:
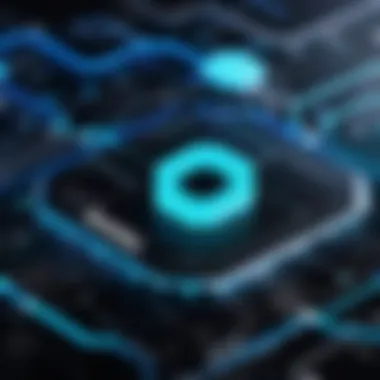
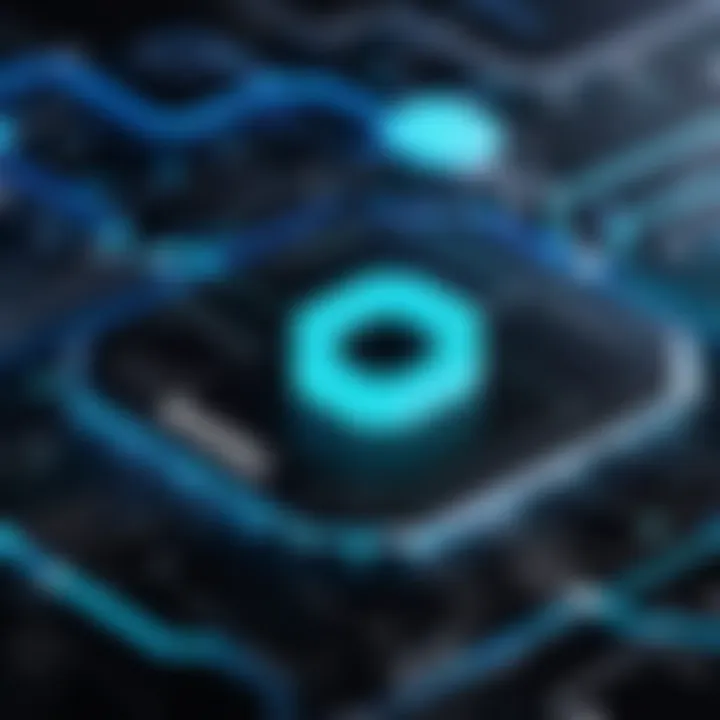
- Go to the official Node.js website: nodejs.org.
- Download the installer suitable for your operating system (Windows, macOS, or Linux).
- Run the installer and follow the prompts. This typically involves accepting the license agreement and choosing the installation path.
- Verify your installation by opening a command line interface and typing . If you see a version number, congratulations, you’ve got Node.js up and running!
"The only limit to your impact is your imagination and commitment." - Tony Robbins
Setting Up MySQL
The next puzzle piece is MySQL, the relational database management system that will handle your data storage needs. Setting it up is straightforward; however, it’s vital to configure it correctly. First, you’ll need to install MySQL:
- Navigate to the MySQL Community Server page: mysql.com.
- Download the appropriate version for your operating system.
- Run the installer, opting for the Custom Setup if you want to tweak installation options such as choosing your install location or adding specific components like MySQL Workbench.
- When prompted, create a root password and remember it. This will be your administrative access point.
Once installed, test your MySQL installation. You can do this by opening a command line or terminal and typing , then entering the password you just set. If you get access to the MySQL command prompt, you’re in business.
Configuring Your IDE for Node.js Development
With Node.js and MySQL installed, the final step in the setup process is configuring your Integrated Development Environment (IDE). An IDE is a software suite that consolidates basic tools required for software development. Choosing the right IDE can greatly influence your coding efficiency and ease of use. Popular IDEs for Node.js development include Visual Studio Code, WebStorm, and Atom.
Here’s how to configure Visual Studio Code for Node.js development:
- Install Visual Studio Code from code.visualstudio.com.
- Once installed, open Visual Studio Code and navigate to the Extensions tab (you can find it in the sidebar or by pressing ).
- Search for and install the following extensions:
- Customize settings and preferences according to your workflow. Adjusting themes, font sizes, and accessibility options can significantly improve your working experience.
- Node.js Extension Pack: This includes essential tools for Node.js development.
- MySQL: Use this if you want integrated MySQL support to run SQL queries directly from your IDE.
Having a well-configured IDE means a smoother coding journey, less clutter, and a focus on what really matters—writing high-quality code that interacts seamlessly with Node.js and MySQL.
Connecting Node.js to MySQL Database
Connecting Node.js to MySQL is an essential step in developing modern web applications. This connection enables dynamic data handling, allowing applications to interact with a database and perform various operations efficiently. In this section, we will explore the MySQL Node.js driver, how to establish a connection, and the concept of connection pooling.
Using MySQL Node.js Driver
The MySQL Node.js driver acts as the bridge between Node.js and MySQL, facilitating seamless communication between the two. Various drivers are available, but one of the most widely used is , which offers a promise-based interface, supporting both callbacks and promises. To install it, simply run:
This driver allows developers to execute SQL statements and retrieve data effectively. Compared to other libraries, the driver is known for its performance and efficiency. It supports prepared statements, a feature that helps prevent SQL injection, showing that security is not an afterthought but an integral part of the design.
Establishing a Connection
Once the driver is installed, the next step is to establish a connection to the MySQL database. Here’s how you can create a connection using Node.js:
In the snippet above, you must replace yourUsername, yourPassword, and yourDatabase with your database credentials. When the method is called, it attempts to establish a connection. If successful, a message is logged; otherwise, the error is captured and printed. This straightforward approach is the first step to enabling data communication.
Connection Pooling
Connection pooling is an important concept when operating with databases in a production environment. Instead of opening and closing connections for each request, which can be resource-intensive, a pool of connections can be maintained. Here’s a brief look at how to implement a connection pool using the driver:
In this code, the method allows for managing multiple connections efficiently. Each time a connection is needed, one is drawn from the pool. After its usage, returns it back to the pool. This not only enhances performance but also reduces the overhead of establishing a connection repeatedly.
Key Takeaway: By connecting Node.js with MySQL through the use of the MySQL Node.js driver, developers can efficiently manage database interactions, ultimately leading to more robust and scalable applications.
Implementing CRUD Operations
Implementing CRUD operations—short for Create, Read, Update, and Delete—is a cornerstone of interacting with databases, and it holds particular significance for applications built using Node.js and MySQL. This section serves to illuminate the functionality and fluid nature of these operations, laying the groundwork for any web application where data manipulation is essential. Without the ability to handle these basic operations, the application would be devoid of dynamic data exchange and interactivity, akin to a ship with no sails.
Creating Data
In the realm of back-end development, creating data represents the entry point of information into the database. It's like planting seeds in fertile soil; without this step, there would be nothing to tend to later. Using Node.js, developers employ libraries such as MySQL to facilitate the insertion of new records into the database.
The SQL command for inserting data is fairly straightforward. Below is a simple example using MySQL's SQL syntax:
This command inserts a new user into the table, showcasing how easily Node.js can interface with MySQL to accomplish this critical task. It’s important to note that successful data creation often relies on validating input data beforehand to avoid potential pitfalls, such as SQL injection attacks.
Reading Data
The ability to retrieve or read data is equally vital. It enables your application to access information stored in the database, allowing users to view whatever details are pertinent to them—much like flipping through pages of a book to find your favorite story. Using Node.js, fetching records from a MySQL database is accomplished using the statement.
An example request might look something like this:
This query will fetch the user's details from the table based on the given email, thus demonstrating how Node.js simplifies the process of data retrieval. This operation is often complemented by pagination techniques when dealing with large datasets, allowing applications to manage resource allocation effectively.
Updating Data
Updating data follows closely behind reading data in terms of importance. It’s like tuning an instrument to ensure it sounds just right. If users are allowed to modify their input, like updating their profile information or preferences, it's crucial that applications facilitate these operations seamlessly. In SQL, updating records is conducted with the statement:
This command shows how straightforward it is to change existing user data. One must be careful here, as a poorly crafted update could unintentionally alter more records than intended. Implementing validation checks is advisable to ensure updates occur accurately and securely.
Deleting Data
Finally, the ability to delete data provides a clean approach to removing unnecessary or outdated information from the database. Think of it as decluttering your workspace; it creates a more efficient environment. For Node.js applications that interface with MySQL, the statement does the trick. An example follows:
This command removes the user associated with the provided email. While deleting data may seem straightforward, it’s vital to implement safeguards. Accidental deletions can be devastating, and typically, developers will include confirmation prompts to mitigate such risks.
"CRUD operations form the very backbone of data manipulation in web applications. Understanding their functions can greatly enhance a developer's skill set."
In summary, mastering CRUD operations is essential when working with Node.js and MySQL. These operations not only empower applications through dynamic data handling but also impose responsibility on developers to ensure secure and reliable data management practices as they continue to build increasingly complex applications.
Handling Asynchronous Operations
In the world of Node.js, the handling of asynchronous operations stands as a foundational pillar. This is crucial, especially when it comes to web applications, where user experience hinges on responsiveness. Understanding how to manage asynchronous activities effectively allows developers to create non-blocking applications, which means the server can handle multiple requests without waiting for one to finish before starting another. This not only boosts performance but also enhances user satisfaction.
Understanding Callbacks
Callbacks are perhaps the earliest form of asynchronous operation in Node.js. A callback is simply a function that is passed as an argument to another function and gets called after the completion of a certain task. It’s like asking a friend to call you back after they’ve finished a task. The downside, however, is what’s often termed as "callback hell," where callbacks are nested within callbacks, leading to complex and unreadable code.
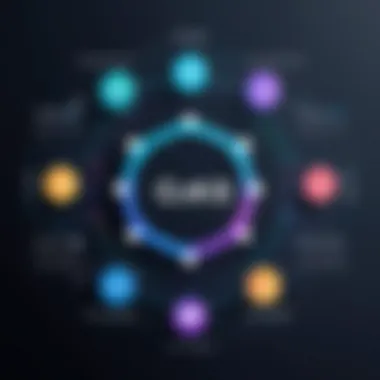
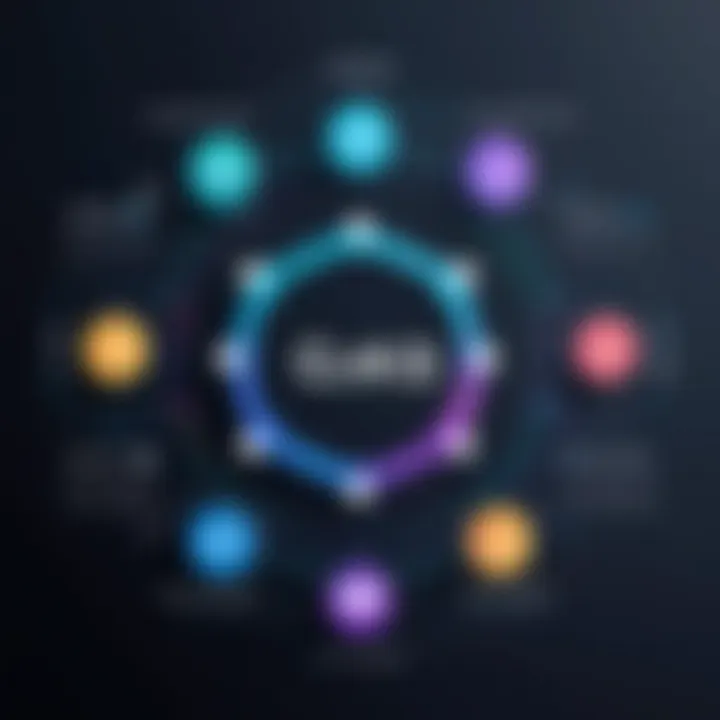
For example:
Here, if you needed to read multiple files one after another, each read operation would require another callback nested inside the previous one. This can quickly become unmanageable.
Promises and Async/Await
To combat the drawbacks of callbacks, JavaScript introduced Promises, which represent the eventual completion (or failure) of an asynchronous operation. A promise can be in one of three states: pending, fulfilled, or rejected. This leads to clearer and more manageable code. You can chain multiple and use for error handling, streamlining your asynchronous logic.
With the introduction of Async/Await, working with promises became even more straightforward. functions can pause at any point with , making asynchronous code look like synchronous code. This drastically boosts readability and maintainability.
For instance:
Managing Concurrency
When dealing with multiple asynchronous operations, managing concurrency becomes a vital consideration. Node.js operates on a single-threaded event loop, meaning it can execute many operations concurrently without blocking the execution thread. This requires careful handling to ensure that operations don’t step on each other's toes.
There are several techniques to manage concurrency effectively:
- Throttle requests: Control the number of simultaneous operations being processed at any given time to avoid overwhelming resources.
- Debounce: Especially useful for user-input events, this technique waits for a pause in activity before executing a function, ensuring it’s called only once after the user has stopped interacting for a brief period.
- Using libraries: Libraries like async can facilitate easier management through various utilities designed for handling collections of asynchronous operations with a bit more sophistication than native JavaScript.
"Managing asynchronous behavior effectively is akin to orchestrating a symphony; every element must harmonize for the masterpiece to resonate."
In summary, mastering asynchronous operations in Node.js is paramount for creating robust applications that can scale and perform effectively under user load. Whether by leveraging callbacks, adopting promises, or utilizing async/await, each step enhances your ability to handle concurrent processes fluidly.
Error Handling in Node.js and MySQL
In the realm of software development, particularly while working with Node.js and MySQL, error handling holds significant weight. An application that overlooks error management can be like a ship sailing without a compass—lost and prone to catastrophe. Errors can arise from multiple fronts: network issues, invalid data input, or even unexpected server responses. This chapter delves into why handling these errors effectively is paramount in any application, safeguarding user experience and maintaining data integrity.
With proper error handling, developers can anticipate potential pitfalls and implement appropriate mitigation strategies. It elevates the application's reliability, making it robust against the unpredictable nature of web environments. This segment will unfold into specific common errors and their solutions, utilization of try/catch blocks, and strategies for logging errors during debugging to bolster this essential aspect of development.
Common Errors and Their Solutions
When navigating through Node.js and MySQL interaction, several errors frequently rear their heads. Understanding them, alongside their remedies, is critical for seamless functionality.
- Connection Errors: Often occur if the database address is incorrect or the MySQL server is down. Verify your connection strings and ensure the server is accessible.
- Syntax Errors: d SQL queries can trigger this. Validate query syntax using a testing tool or MySQL shell.
- Null Value Exceptions: Attempting to insert or update a record with null where it's not permitted will result in an error. Utilize checks to avoid such conditions beforehand.
- Data Type Mismatches: Feeding data of one type into a field expecting another can lead to errors. Always confirm that data types are compatible.
These errors can be frustrating, but with an understanding of their origins and practical responses, developers can swiftly navigate around them.
Using Try/Catch Blocks
In Node.js, try/catch blocks serve as a safety net, allowing developers to catch exceptions and handle them gracefully rather than letting the application crash. Here's a simple implementation:
Why Use Try/Catch?
- Enhanced Stability: It can stop an application from crashing unexpectedly.
- User Experience: Provides better feedback to users instead of a generic error page.
- Debugging Advantages: Offers specific feedback on where the error occurred, aiding in quicker resolution.
Using try/catch effectively can safeguard against unhandled promise rejections and runtime errors, making Node.js applications a lot sturdier.
Logging Errors for Debugging
Beyond handling errors, logging them is a crucial step that should not be overlooked. Logs serve as the wisdom archive of an application, allowing developers to track issues, analyze trends, and preempt failures. Employing a logging library, such as Winston, or even simpler methods using the built-in , can help capture useful information for troubleshooting.
"If you can't measure it, you can't improve it." — Peter Drucker
Techniques for Logging:
- Error Level Segmentation: Differentiate error types such as info, warn, error, etc. This helps prioritize responses.
- Timestamping Entries: Record when an error occurred so overall trends can be observed over time.
- Storing Logs Externally: Utilize services like Loggly or ELK Stack for centralized logging, making it easier to audit and monitor logs.
Optimizing Performance
Optimizing performance in the context of Node.js and MySQL is paramount to ensure that applications run smoothly and can handle high traffic efficiently. As applications grow, bottlenecks can slow things down, frustrating users and degrading the experience. Performance optimization is not just about quick fixes; it involves methodical strategies and considerations that impact various layers of the application stack. When Node.js interfaces with MySQL, careful attention must be given to how data is retrieved, manipulated, and served back to users.
Indexing in MySQL
Indexing can be likened to creating an index at the back of a book—it saves time. In MySQL, indexes are essential for speeding up the retrieval of records. Without them, MySQL has to scan the entire table to find the desired data, which can be painfully slow, especially with large datasets. Indexes work by providing a structured way to access rows, enabling faster searches.
- Types of Indexes: MySQL supports different kinds of indexes, such as:
- Primary Keys: Unique identifiers for each record.
- Unique Indexes: Ensures that all the values in a column are different.
- Full-text Indexes: Useful for searching within textual data.
Creating indexes should be approached with care. While they can significantly enhance read performance, having too many indexes can slow down write operations like inserts or updates. The trade-off is essential to consider when designing your schema, balancing speed for reads versus writes.
This simple command can turn a sluggish query into a speedy operation, so long as you choose the right columns for indexing.
Query Optimization Techniques
Optimizing queries in MySQL is crucial to ensure the application runs like a well-oiled machine. Here are some practical techniques:
- Use SELECT Specific Columns: Instead of , always specify the columns needed. This reduces data transfer overhead.
- Avoid Subqueries: Sometimes, subqueries are necessary, but they can often be replaced with JOINs which are typically faster.
- Limit Results: When possible, always use the clause to reduce the amount of data fetched, especially during initial stages of data processing.
Implementing these adjustments can save valuable processing time and network bandwidth, which ultimately translates to a snappier user experience. Testing different queries for execution time can help pinpoint inefficiencies. The command in MySQL provides insight into how MySQL executes a query and how indices are being utilized.
Load Balancing and Scaling
Load balancing and scaling are crucial as your Node.js application grows. Load balancing distributes traffic across multiple servers so that no single server becomes a bottleneck. This is essential for maintaining performance during peak usage times.
- Horizontal Scaling: This involves adding more machines to handle the increased load. It’s often seen as being more effective than vertical scaling (adding more power to a single machine) in a distributed environment.
- Using a Load Balancer: Tools like Nginx or HAProxy can be deployed to manage requests and send them to the least busy Node.js instance.
Also, when working with MySQL, scaling can be achieved through clustering or replication.
By keeping your application architecture in mind from the get-go, you’ll set yourself up for success as demand increases. Combined with good caching strategies, it’s feasible to serve thousands of users seamlessly without a hitch.
Proper performance optimization is key to sustaining high traffic levels in Node.js applications while maintaining a harmonious relationship with MySQL.
Security Considerations
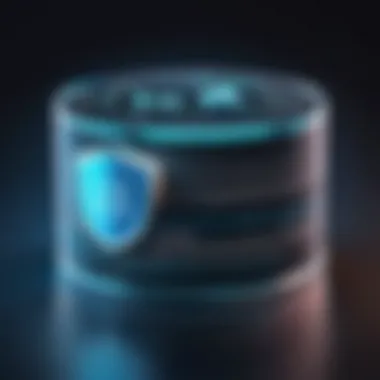
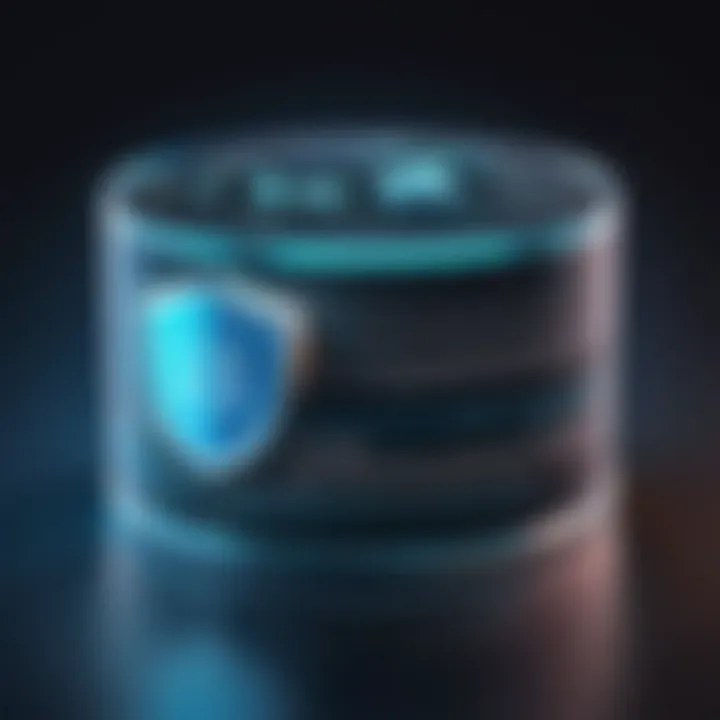
In today's rapidly evolving digital landscape, protecting sensitive data is paramount. Security considerations are central to the integration of Node.js and MySQL. With the increasing intersection of technology and data, breaches can have dire consequences. This is particularly critical in applications handling user information, financial data, and personal identifiers.
By effectively addressing security issues, developers can bolster the trustworthiness of their applications and ensure compliance with regulations. This section examines key aspects related to web security within the Node.js and MySQL ecosystem, focusing on preventing SQL injection, securing Node.js applications, and utilizing environment variables in configurations.
Preventing SQL Injection
SQL injection is one of the oldest and most common forms of cyber attacks. An attacker may exploit vulnerabilities in an application's software to manipulate SQL queries. This can lead to unauthorized access to data, data loss, or even system compromise.
To prevent SQL injection, it’s crucial to employ the principle of least privilege when interacting with the database. Always use parameterized queries or prepared statements. For example, instead of constructing SQL queries by concatenating strings, using a library like or , you can write:
This practice not only safeguards against attacks but also enhances code clarity, making it easier for future modifications.
Securing Node.js Applications
Node.js applications must have security at their core. With numerous libraries and frameworks available, developers should prioritize those that are actively maintained and widely vetted by the community. Adopting HTTPS is essential, encrypting data in transit and establishing trust with users. By implementing security headers like Content Security Policy (CSP) and X-Content-Type-Options, you limit the vectors available to an attacker.
Another effective strategy is keeping dependencies up to date. Tools like or can identify vulnerabilities in libraries you may be using, ensuring your application’s security stance remains robust over time.
"An ounce of prevention is worth a pound of cure." – Benjamin Franklin
Using Environment Variables for Configuration
Environment variables are a simple yet effective way to secure sensitive information like database credentials, API keys, and other configuration data. Hardcoding this information may lead to leaks, especially if the codebase is shared or open-sourced.
Utilize a package such as to manage environment variables seamlessly. In your file, you would store your configurations:
Then, in your Node.js application, access these variables:
In this way, even if your code is exposed, your sensitive data remains secure.
In summary, security considerations are non-negotiable when integrating Node.js with MySQL. Through proactive measures, developers can create resilient applications that stand the test of time, safeguarding users’ data and fostering a secure digital environment.
Best Practices in Node.js and MySQL Development
In the world of web development, adhering to best practices is not just a nicety, it’s a necessity. When it comes to integrating Node.js with MySQL, following established guidelines and methods can drastically improve not just the performance of your application but also its maintainability, security, and user experience. Think of these best practices as the foundation of a well-built house; if the foundation is shaky, everything above it is bound to have cracks.
Code Structure and Organization
A well-organized codebase goes a long way in making the development process smooth. Node.js applications often become complex as they grow. Therefore, organizing your files and directories logically is crucial. Here are some tips for maintaining clean code:
- Modularity: Break your application into modules. Each module should encapsulate a particular functionality or feature, making it easier to manage and test.
- Consistent Naming Conventions: Use consistent naming conventions for files and variables. Whether you opt for camelCase or snake_case, be uniform to avoid confusion.
- Directory Structure: Separate your routes, models, and controllers! A good directory structure might include folders like /routes, /models, and /controllers. This makes it easier for anyone who reads your code to understand the flow of data and handling logic.
By following these practices, you not only make it easier to navigate through your code, but you also set a standard for team members, which is vital for collaboration.
Documentation and Comments
Documentation is the unsung hero of software development. Taking the time to document your code may seem tedious, but it makes a world of difference. Here are key points to consider:
- In-line Comments: Use comments judiciously to explain the logic behind complex sections of code. Simple, clear explanations can save hours of confusion later on.
- API Documentation: Use tools like Swagger to document your APIs. It helps others understand how to interact with your application’s endpoints without digging through the codebase.
- README Files: A well-crafted README can serve as a guide for anyone inheriting your project. It should include setup instructions, usage examples, and an overview of the application.
Good documentation ensures that anyone can jump into the project and grasp the fundamentals quickly, which is especially beneficial in collaborative environments. It's worth spending that extra hour to document your work properly.
Continuous Integration and Deployment
Adopting continuous integration (CI) and continuous deployment (CD) practices can significantly enhance your development workflow. Here's why they matter:
- Automated Testing: CI allows you to run tests each time you make a change to your code. It catches errors early, preventing broken deployments that can frustrate users.
- Rapid Feedback Loop: By getting immediate feedback on new code, developers can make adjustments quickly, ensuring a more fluid development cycle.
- Reliable Deployments: Using CD practices makes the deployment process less error-prone. Tools like Jenkins or GitLab CI can automate the deployment pipeline, allowing you to deploy with confidence.
Incorporating CI/CD can propel your project into the fast lane, allowing for frequent updates and innovations without the fear of ruining the user experience.
Best practices in coding and deployment cut down risks and boost efficiency.
Real-world Applications of Node.js and MySQL
Node.js, combined with MySQL, has carved out a significant niche in the world of web development. This duo is instrumental in various industries, facilitating the rapid development of applications that are high-performance and scalable. The integration of Node.js and MySQL can be a game-changer for businesses, enabling streamlined data management and user interaction. When exploring these applications, focusing on practical implementations sheds light on the versatility and efficiency that this technology stack brings.
E-commerce Platforms
In the realm of e-commerce, the efficiency of Node.js paired with the data handling capabilities of MySQL can lead to significant advantages. Online businesses are constantly on the lookout for seamless user experiences, and Node.js is designed to handle numerous concurrent connections, making it perfect for high-traffic online stores. With real-time updates of inventory levels and user data management, this setup allows for rapid response times.
- User Authentication: Secure management of customer data is a priority. Node.js facilitates fast and secure login processes while MySQL ensures data integrity and consistency.
- Inventory Management: MySQL's ability to efficiently manage large datasets helps e-commerce platforms keep track of stock levels, allowing for automatic updates when purchases are made.
- Transaction Handling: Node.js excels in handling multiple transactions simultaneously without lag, while MySQL organizes transaction records for easy retrieval and oversight.
The benefits are clear. Businesses can expect enhanced user experience through faster load times, broad data management, and effective transaction handling.
Content Management Systems
Content Management Systems (CMS) built on Node.js and MySQL benefit from the speed and scalability this combo offers. These systems require flexibility and user accessibility, enabling users to create, modify, and publish content quickly and efficiently.
- Dynamic Content Delivery: Node.js powers real-time services in CMS, delivering quick updates and content delivery.
- User Engagement: With MySQL effectively organizing user-generated content, site administrators can easily curate and manage content, from blog posts to multimedia.
- Scalability: Node.js applications can grow rapidly with the business's needs, accommodating increasing amounts of content and users without performance degradation.
Hence, utilizing this setup gives developers the tools to build flexible and robust content systems.
Data Analysis Tools
In a world that increasingly relies on data, Node.js and MySQL together form a formidable combination for data analysis tools. Businesses need real-time insights from their data, and this duo provides a structure where data can be collected, analyzed, and visualized efficiently.
- Rapid Data Retrieval: Node.js allows for quick processing of queries, providing fast insights, while MySQL handles data storage effectively.
- Dashboard Creation: Utilizing tools built on this platform to create interactive dashboards enables organizations to visualize their data intuitively.
- Integration with Other Technologies: Node.js easily integrates with various front-end frameworks and libraries, enhancing the user experience in data tools.
Through this framework, organizations can turn raw data into actionable insights swiftly, making strategic decisions based on real-time analysis.
Ultimately, the use of Node.js and MySQL across various applications illustrates the potential of this technology stack. Whether improving customer experience in e-commerce or empowering organizations with data analysis tools, the real-world implications are profound.
Culmination
As we wrap up our insights on integrating Node.js and MySQL, it’s essential to reflect on the significance of the relationship between these two technologies. Understanding how Node.js interacts with MySQL is not just beneficial—it's critical for anyone stepping into the world of back-end development. This conclusion will explore the vivid elements we've covered, the benefits of this integration, and vital considerations for future projects.
Key Takeaways
- Integration Simplicity: Node.js provides a lean architecture to connect seamlessly with MySQL, allowing developers to manage databases efficiently.
- Asynchronous Handling: Utilizing Node.js's event-driven architecture enables quick and effective management of multiple database requests without bogging down the server.
- CRUD Operations Mastery: Implementing CRUD (Create, Read, Update, Delete) operations in a Node.js application is straightforward and can lead to rapid application development.
- Performance Optimization: Techniques such as connection pooling and indexing in MySQL optimize responses, ensuring applications run smooth.
- Security Protocols: Addressing urgent security matters like SQL injection and environment variable management fortifies the applications, making them robust and resilient.
The Future of Node.js and MySQL
Looking ahead, the evolution of Node.js and MySQL holds immense potential. The continued growth in demand for real-time applications positions Node.js as a fundamental pillar in web development. Likewise, advancements in MySQL's capabilities, especially with cloud database services, provide robust solutions to handle intricate data relationships effortlessly.
Furthermore, as the programming landscape shifts towards microservices architectures, the compatibility of Node.js with MySQL will help developers embrace scalable solutions without a hitch. The increasing focus on full-stack JavaScript development enhances this synergy, as developers can harness the power of a singular language across the board.
"With great power comes great responsibility." In this context, mastering Node.js and MySQL not only enhances your skill set but also equips you to create impactful software solutions that can meet modern demands.