Node.js Assessment Questions: A Comprehensive Guide
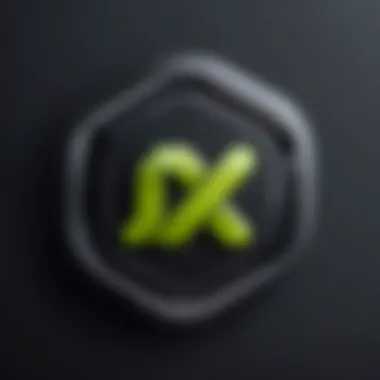
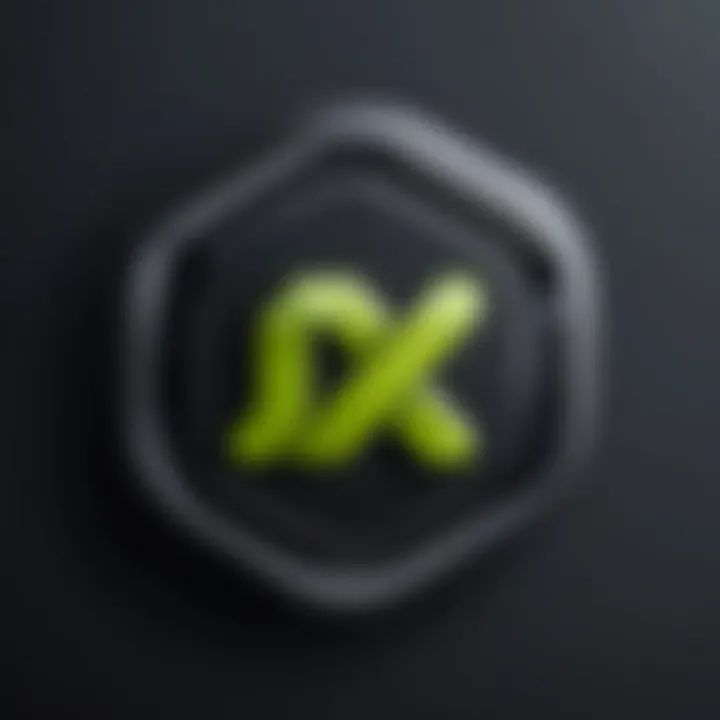
Foreword to Programming Language
Node.js has become a staple for developers who rely on JavaScript to build scalable applications. Unlike traditional programming environments, Node.js surprises most when users realize it allows developers to run JavaScript on the server side. This shift means that web applications can reach new heights of efficiency and functionality.
History and Background
Node.js emerged in 2009, crafted by Ryan Dahl. The fundamental idea behind Node.js was to create a framework that leverages the non-blocking I/O model, enabling developers to handle multiple connections with high throughput. This approach has changed how we conceive server-side programming in simple yet powerful ways. Node.js runs on the V8 JavaScript engine, which was initially engineered by Google for its Chrome browser. Since its inception, Node.js has forked a life of its own, maturing into a robust environment with frequent updates and a thriving package ecosystem managed by npm (Node Package Manager).
Features and Uses
One of the primary features of Node.js is its asynchronous nature. This allows developers to enjoy high performance and responsiveness, making it an excellent choice for I/O-heavy applications, like those that involve real-time data.
Common uses for Node.js include:
- Web applications, such as online shopping platforms.
- Real-time applications, like chat applications and collaboration tools.
- RESTful APIs, providing easy access to data.
With rapid development cycles in mind, many find its architecture conducive to Agile methodologies and DevOps practices, genuinely catering to teams looking for efficiency.
Popularity and Scope
Node.js has achieved a significant foothold in the programming landscape. According to various surveys, it consistently ranks among the top choices for server-side development, attracting both startups and established enterprises. Its growing popularity can also be attributed to vibrant community support and a wealth of resources, meaning new learners can quickly find help when tackling challenges.
By facilitating JavaScript use throughout the stack, Node.js empowers developers to write both front-end and back-end code with the same language. This unification has led many companies to adopt it widely, enabling a seamless and versatile development experience.
"Node.js provides a powerful platform where performance meets convenience, making it effective for both novice and expert developers."
In understanding Node.js principles, one lays the groundwork for tackling more complex subjects later in the programming journey. The abundance of resources and real-world applications surrounding it only solidifies its place in the annals of modern software development.
Looking ahead, exploring basic syntax, functions, and advanced topics can further enhance oneās command of this transformative tool.
Prelims to Node.js Assessments
Node.js has made waves in the software development sea, providing a robust platform for building scalable applications. As the demand for Node.js skills surges, so too does the necessity for effective assessment tools. Understanding Node.js assessments is crucial not only for individuals looking to hone their abilities but also for employers on the lookout for proficient candidates. These assessments serve both as a reflection of oneās capabilities and as a route to identify areas ripe for improvement.
Purpose of Node.js Assessments
The purpose behind Node.js assessments is multifaceted. They aim to evaluate a candidateās grasp of key concepts, from the basics of how Node.js operates to the intricacies of its architecture. When crafted thoughtfully, these assessments can reveal whether an individual can effectively leverage Node.js to tackle real-world problems. They aren't just about rote memorization of syntax, but rather about demonstrating critical thinking and problem-solving skills in practical scenarios.
Node.js assessments can take many forms; from simple quizzes that test foundational knowledge to comprehensive practical assignments where candidates need to build an application or feature. Each format has its own benefits:
- Quizzes help gauge immediate understanding and retention of concepts.
- Practical projects provide a better perspective on a candidate's coding style and approach to problem-solving.
In essence, these assessments serve to bridge the gap between theoretical knowledge and practical application.
Importance of Skill Evaluation
Evaluating skills in Node.js is about more than just numbers and scoresāitās about fostering growth and development. With the tech landscape evolving at breakneck speed, ongoing evaluations can help individuals keep pace with new trends, best practices, and critical updates in Node.js.
Consider the following points regarding the significance of skill evaluation:
- Identifying Strengths and Weaknesses: Individuals can pinpoint what they excel at and where they may need extra help or training.
- Staying Relevant: Regular assessments help developers adapt to new libraries, frameworks, and methodologies within the Node.js ecosystem.
- Enhancing Job Prospects: For job seekers, showcasing a solid track record in assessments can make them stand out in a crowded job market.
"Regular evaluations cultivate a culture of continuous improvement."
Fundamental Concepts
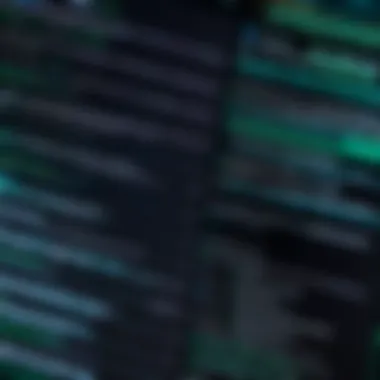
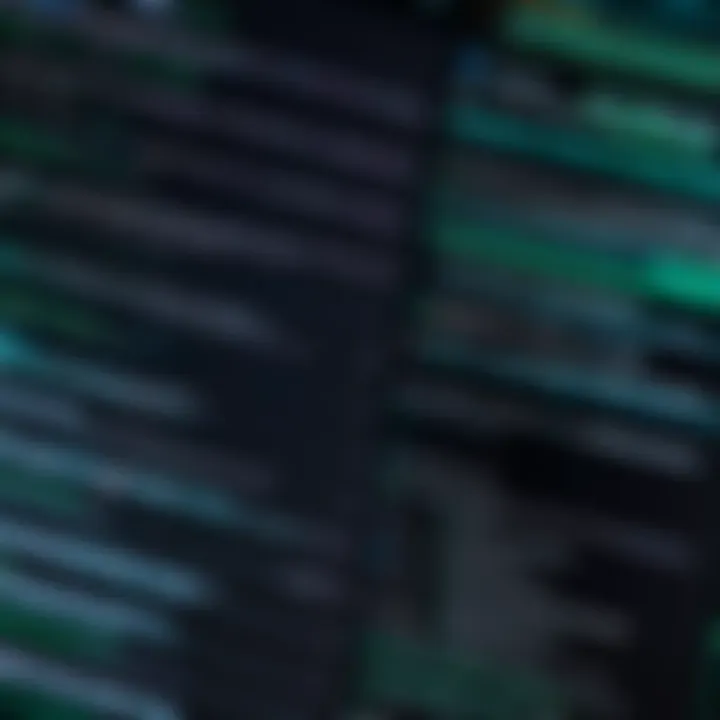
When diving into the world of Node.js, understanding fundamental concepts is not merely beneficial; it's essential. These concepts form the backbone of how Node.js operates, enabling developers to write efficient and scalable applications. Below are critical areas that underscore the importance of these fundamentals.
Understanding Asynchronous Programming
Asynchronous programming is a core feature of Node.js, setting it apart from traditional synchronous models. In a nutshell, this approach allows the execution of tasks without waiting for previous tasks to complete. This is particularly useful in scenarios where input/output operations are involvedālike reading files or calling APIsāthat can introduce significant delays.
For instance, if a developer writes a function to fetch user data from a database, an async implementation means the function can continue executing subsequent code while the data is being retrieved. This leads to enhanced performance in applications, particularly when dealing with numerous simultaneous requests. To illustrate:
Here, the keyword pauses the code at that line until the data is fetched, yet the event loop allows other operations to progress in the meantime, promoting an efficient runtime environment. Understanding this concept is crucial for evaluating candidates in assessments, as it showcases their grasp of real-world applications in Node.js.
Event Loop Mechanism
Another fundamental aspect of Node.js is its event loop mechanism. At its foundation, the event loop is what allows Node.js to perform non-blocking I/O operations. Instead of waiting for an operation to complete, the event loop puts it aside and moves on to process other tasks, efficiently handling multiple requests at once.
To visualize this, imagine a chef in a busy kitchen. When the chef puts a pot of water on to boil, he doesnāt just stand there waiting. Instead, he preps other ingredients. This multitasking ensures that all orders are served promptly, paralleling how the event loop operates. The event loop checks the queue, processes any callbacks waiting in line, and continues its workāmaximizing productivity.
Understanding this mechanism is invaluable during assessments, as it reveals a deep knowledge of how Node.js optimizes performance through its architecture. The event loop not only makes Node.js efficient but also shapes how developers design applications.
Node.js Architecture
Lastly, delving into Node.js architecture provides insight into how all these moving parts come together to create a powerful runtime. Node.js employs a non-blocking I/O model based on Google Chrome's V8 JavaScript engine. This architecture comprises several layers, including the core modules, the libuv library, and the event-driven philosophy.
- Core Modules: These are essential functions that come out of the box, like file system operations and creating web servers.
- Libuv: This library allows Node.js to handle asynchronous operations across environments, working with the event loop and managing multi-threading.
- Single-threaded: Despite the use of asynchronous calls, Node.js runs in a single-threaded environment. This means developers must carefully design their application flow to avoid performance hits.
Grasping the architecture not only enables developers to write better code but also positions them to troubleshoot issues effectively. In assessments, this knowledge demonstrates a candidate's ability to architect solid Node.js applications, enhancing their overall proficiency.
Understanding these fundamental aspects of Node.js enhances a developer's ability to create scalable applications. As candidates prepare for their assessments, this foundational knowledge will allow them to tackle both theoretical and practical challenges with confidence. The stronger their grasp of these concepts, the better equipped they are to excel in their careers.
Common Assessment Questions
The realm of Node.js is vast and ever-evolving, making common assessment questions essential for gauging oneās grasp of its core aspects. These questions serve as touchstones for understanding not merely the functionality of Node.js but also the thought processes that underpin effective programming and development practices. Whatās the big deal, you might wonder? Well, having a repository of well-thought-out questions allows both learners and professionals to identify their strengths and weaknesses in a structured manner. This is invaluable, especially in a job market where every second counts, and technical interviews can be the deciding factor between two equally qualified candidates.
Basic Questions on Node.js
Whether you're just starting out or youāve dabbled in a few projects, basic questions provide a solid grounding to ensure that your understanding of Node.js is on the right track. These inquiries often center around the fundamental concepts that every Node.js developer should grasp. For example, a question like "What is Node.js, and how does it differ from traditional web servers?" helps to clarify its non-blocking architecture and single-threaded nature.
Beyond definitions, basic questions can include:
- How do you install Node.js on your machine?
- What are some core modules in Node.js?
- What is a package.json file, and why is it important?
These questions allow learners to step back and evaluate their foundational knowledge while also preparing them for more complex concepts down the line.
Intermediate Questions on Node.js
As one moves further along the Node.js learning curve, intermediate questions begin to explore more intricate topics, often focusing on concurrency, modules, and the Node Package Manager (NPM). At this level of understanding, developers should be ready to tackle queries that can really test their grasp of the ecosystem. Questions like "What is the difference between require() and import()?" or "How does asynchronous programming work in Node.js?" encourage deeper thought about code and its execution.
Possible intermediate questions could be:
- How do you handle events in Node.js?
- What is middleware, and how does it work in Express?
- Can you explain what the Event Loop is?
These questions help build a bridge from basic knowledge to advanced concepts, further solidifying understanding of how Node.js operates under the hood.
Advanced Questions on Node.js
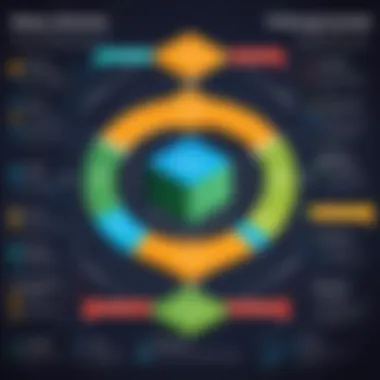
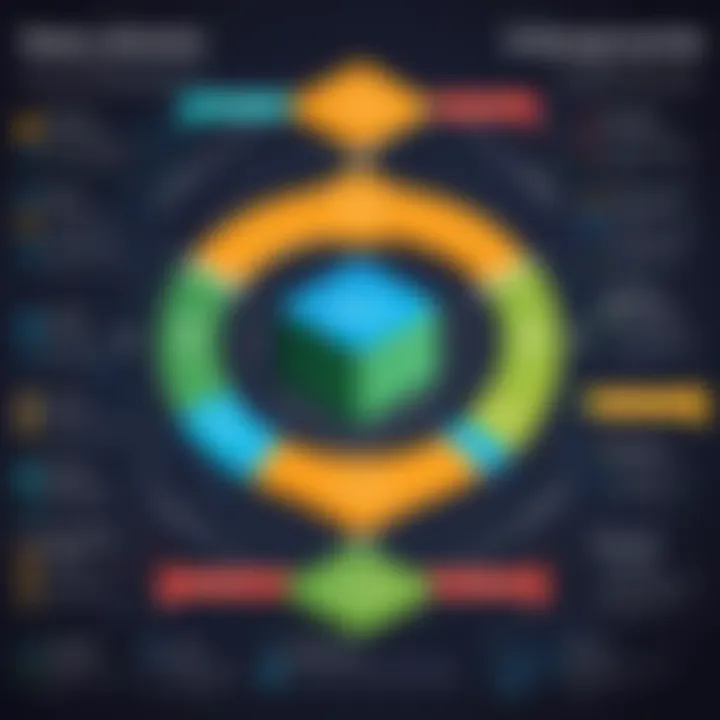
For those ready to demonstrate their expertise, advanced questions dive into complex mechanisms and design patterns that truly showcase a developer's skills. Queries put forward here often challenge the learner to think critically about real-world applications and best practices. An example might be, "How would you implement a WebSocket server in Node.js?" or "Can you explain how you would manage database connections efficiently?"
Advanced questions could also include:
- What are streams in Node.js and how do they function?
- Explain the concept of Promises and async/await.
- What are the potential pitfalls of using callbacks?
These questions not only probe into a candidate's technical proficiency but also encourage them to think strategically about their problem-solving approaches.
"A clear understanding of assessment questions can guide developers in honing their skills and identifying gaps in knowledge that need to be addressed."
In sum, assessment questionsāwhether basic, intermediate, or advancedāplay a crucial role in the continual growth of a Node.js developer. They align learning paths with necessary skills and encourage self-evaluation, which ultimately leads to more effective and successful programmers.
Best Practices in Node.js Development
When delving into Node.js development, understanding and adhering to best practices can significantly influence the robustness and efficiency of your code. This section spotlights various essential practices that developers should integrate into their workflow. It's not just about writing code; itās also about maintaining clarity, performance, and scalability. In the fast-paced world of technology, a strong foundation in best practices is invaluable.
Key Benefits of Best Practices
- Improved Code Quality: Adopting best practices fosters clean, manageable, and efficient code. This translates to fewer bugs and a more robust application.
- Enhanced Collaboration: With established conventions, teams can collaborate effectively, streamlining the development process.
- Better Performance: Knowing and applying optimization strategies can greatly improve the execution speed and resource management of your application.
- Long-term Maintenance: Well-structured code allows for easier updates and maintenance, extending the application's lifecycle.
Error Handling Techniques
Error handling is often overlooked until something goes wrong. However, in Node.js, where callbacks and promises reign supreme, solid error handling techniques are crucial. The asynchronous nature of Node.js means that errors can easily propagate, resulting in unexpected behaviors if not handled properly.
- Use Try-Catch with Async/Await: When using async/await syntax, always encapsulate your asynchronous code within a try-catch block. This provides a clear mechanism for handling errors without leaving them unaddressed.
- Implement Global Error Handlers: Setting up centralized error handling across your application ensures that errors are logged and managed in a consistent manner. Express.js, for example, allows for middleware to catch errors that occur during the request/response cycle.
- Utilize Proper Error Types: Instead of sending generic error messages, categorize your errors (e.g., ValidationErrors, DatabaseErrors) to help with debugging and understanding issues effectively.
āPrevention is better than cure.ā
ā Desmond Tutu
Performance Optimization Strategies
Performance optimization in Node.js can mean the difference between an application that scales and one that collapses under user demand. Here are some strategies that can help squeeze the most out of your Node.js applications.
- Use Cluster Module: Node.js runs on a single thread by default. Implementing clustering can help utilize multi-core systems efficiently, spreading requests across multiple instances of your app.
- Optimize Database Queries: Slow database queries can bottleneck performance. Make sure to optimize your database interactions, indexing your tables appropriately and minimizing the data fetched where possible.
- Leverage Caching: Utilizing caching mechanisms, such as Redis or in-memory caching, can drastically reduce response times for frequently requested data.
- Monitor Performance: Use monitoring tools like New Relic or APM to keep an eye on application performance in real time. Identify slow requests and bottlenecks to proactively solve problems.
By integrating these error handling and performance optimization practices, developers can write high-quality Node.js applications that are resilient under pressure and easier to maintain over time.
Practical Applications
In the realm of software development, the practical applications of Node.js are paramount. By arming oneself with a solid grasp of Node.js, developers open the door to a myriad of opportunities that extend beyond mere theory. Understanding how to effectively apply Node.js in real-world scenarios is essential for creating efficient applications that can handle high workloads.
When coding with Node.js, emphasis on practical application particularly enhances oneās employability. Companies are on the hunt for individuals who can not only answer technical questions but also demonstrate the ability to solve real issues. This helps bridge the gap between academic knowledge and practical skills, ensuring that skills are put to effective use in production environments.
Building RESTful APIs with Node.js
One of the most significant applications of Node.js is in creating RESTful APIs. Leveraging Node.js for API development allows for an asynchronous, event-driven architecture that can simplify the handling of multiple requests simultaneously. With tools like Express.js, developers can craft APIs that are both powerful and scalable.
RESTful APIs, in essence, follow a set of conventions that allow services to communicate reliably over HTTP. Here are some key considerations when building these APIs with Node.js:
- Understanding HTTP methods: Familiarize yourself with GET, POST, PUT, and DELETE. Each method serves a specific purpose and knowing when to use each is essential.
- Middleware Usage: Middleware functions play an important role in processing requests and responses. Functions like bodyParser and cors help manage data and keep applications secure.
- Consistent URL structures: Crafting intuitive and consistent URL endpoints ensures that anyone using the API can navigate with ease.
Hereās a simple illustration of setting up a RESTful API in Node.js using Express:
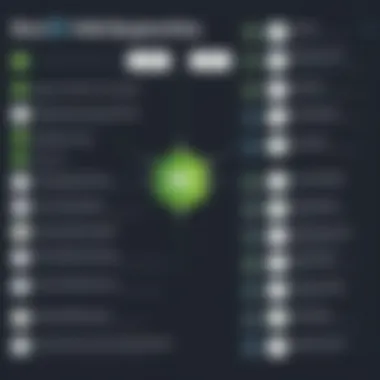
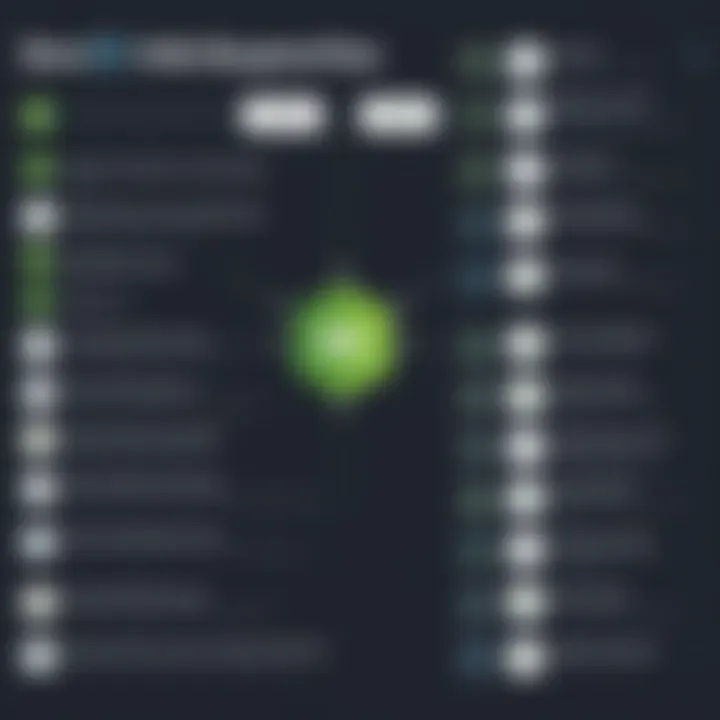
In this snippet, a basic server setup highlights the core functionality of handling GET requests. This practice showcases Node.js's efficiency in managing APIs, marking the first step in mastering practical applications of Node.js.
Using Middleware in Express
Webhook mechanisms in Express.js deploy middleware that intercepts requests and responses. Middleware serves as a tool to augment the functionality of your application without tightly coupling various layers. This is where the beauty of modular programming comes to life.
Consider these vital aspects when working with middleware in Express:
- Optimizing Request Processing: Middleware can be used to compress response sizes, manage requests arrays through pagination, or handle sessions, enhancing performance and user experience.
- Error Handling: Implementing custom error-handling middleware allows for smoother debugging and improved responses. Handling errors at a specific stage helps pinpoint issues without sending back uninformative messages to the user.
- Third-Party Middleware: Tools like Morgan for logging and Helmet for security can be integrated effortlessly, strengthening your applicationās capabilities.
"Good middleware allows developers to build robust applications while keeping the code clean and manageable."
As developers deepen their understanding and application of middleware, they will find it's a crucial element in Node.js development. This knowledge is not only theoretical; rather, it transforms into practical know-how that enhances the overall functionality and resilience of applications. Understanding how to implement and utilize middleware can define the success of a developer navigating the complexities of web technologies.
Evaluating Node.js Knowledge
Evaluating oneās knowledge of Node.js isn't just a walk in the park; itās more akin to navigating a labyrinth of intricate concepts and hands-on skills. This evaluation process helps in pinpointing the strengths and weaknesses of developers, providing them with valuable insights into where they stand in their learning journey. For those engaging with Node.js, understanding the nuances of this evaluation can facilitate targeted improvement, ultimately sharpening their coding skills and broadening their practical toolkit.
Assessments can range from straightforward quizzes to complex projects, each designed to approach different learning outcomes. They hold a vital place in not just preparing for job interviews but also in self-driven development. Emphasizing practical applications alongside theoretical knowledge ensures a well-rounded grasp of how Node.js functions as a backend engine.
"Evaluating knowledge is like sharpening a blade; the more you refine, the better it cuts through challenges."
Self-Assessment Tools
Self-assessment tools occupy a significant niche in the realm of Node.js evaluations. They enable learners to measure their grasp of concepts without the pressure of comparisons or judgment from others. Various platforms offer tailored quizzes and coding challenges that allow users to focus on their understanding and recall. By employing these tools, developers can learn effectively on their own timeline, reinforcing topics that may feel fuzzy.
Here are some popular self-assessment tools and resources:
- Codecademy: Offers interactive courses with quizzes to evaluate understanding.
- LeetCode: Provides a range of coding problems to practice and assess algorithm skills.
- Exercism: Focused on coding challenges that allow for practice in diverse programming languages, including JavaScript.
Depending on their current level, developers can select from beginner to advanced assessments. Tracking improvements over time can often be enlightening, giving a sense of progress that inspires continual learning.
Peer Review Mechanisms
Peer review mechanisms stand as pillars in the journey of assessing Node.js proficiency. Collaboration and feedback are crucial in software development, making this evaluation method especially pertinent. In this environment, learning takes on a communal approach where developers can critique each other's code and share insights about best practices.
Some avenues for peer review include:
- GitHub: A widely-used platform where code can be shared and reviewed openly. Engaging in code reviews helps cultivate best practices.
- Stack Overflow: Not strictly a peer review platform, but asking questions and receiving feedback fosters a community of learning where developers can refine their understanding through shared knowledge.
- Meetup Groups: Local or virtual gatherings where developers come together, often working through challenges and sharing expertise.
Introducing a systematic approach to peer feedback can help individuals confront their assumptions and understand the reasoning behind certain coding decisions. In such evaluative mechanisms, not only does one gain insights into their work, but they also build a network that can ultimately lead to more opportunities within the tech landscape.
Resources for Further Learning
Diving deep into Node.js can be akin to navigating a labyrinthāchallenging yet rewarding. One of the best ways to ensure one stays on the right path is by utilizing the right resources. Resources for Further Learning provide not only the theoretical background but also practical knowledge that can prove invaluable during assessments or real-world applications. Engaging with high-quality materials can amplify the understanding of Node.js and sharpen skills. In this section, we will explore online courses, certifications, books, and documentation that can help learners at various levels to fortify their grasp on Node.js.
Online Courses and Certifications
For those eager to grasp Node.js comprehensively, online courses offer a structured environment for learning. Platforms like Udemy and Coursera are treasure troves filled with a variety of courses tailored for different skill levels.
- Visual Learning: Many courses include video tutorials where concepts are demonstrated practically. Seeing Node.js in action can clarify complex ideas, making them more digestible.
- Certifications: Obtaining a certification can be quite beneficial if someone wishes to showcase their skills to potential employers. Microsoft and Node.js Certification offer credentials that can serve as proof of oneās capabilities.
- Flexibility: Online courses allow learners to study at their own pace, revisiting tricky topics until theyāre well understood.
Additionally, engaging in community forums related to these courses can provide a deeper insight through interaction with instructors and peers.
Books and Documentation
Books remain an evergreen resource for learners desiring an extensive understanding of Node.js. Texts like "Node.js Design Patterns" and "Learning Node.js Development" offer detailed explorations into the workings of this environment.
- Comprehensive Guides: Books often cover topics extensively, providing context and examples. They often go beyond the basics, diving into intricate concepts that may come up during assessments.
- Official Documentation: The official Node.js documentation (available at nodejs.org) is a goldmine of knowledge. Itās kept up-to-date with the latest changes and features. Browsing through the docs can be your go-to when looking for specifics.
- Interactive Learning: Some books come with accompanying online resources or exercise problems to practice coding while learning theory.
"A good book can be a guide for a lifetime, opening doors to uncharted knowledge."
The significance of sourcing reliable materials cannot be understated in todayās tech world. By integrating both online courses and robust books into their study regimen, learners are better equipped to understand and excel in Node.js. Regardless of where one finds themselves on their Node.js journey, these resources can pave the way to a more rounded, confident skill set.