Mastering TypeScript: A Transition Guide for JavaScript Developers
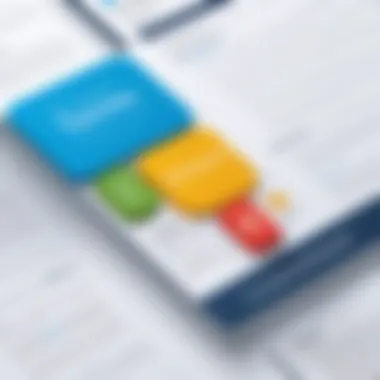
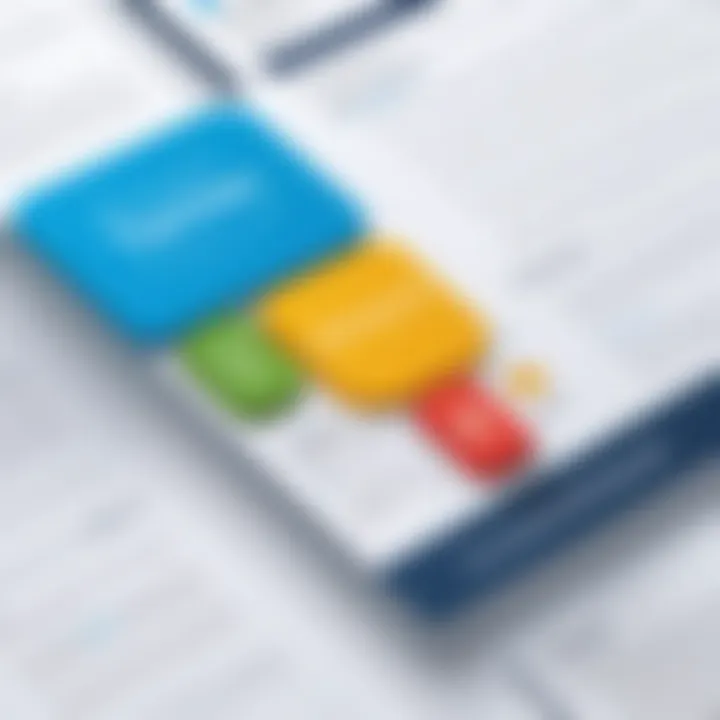
Prelims to Programming Language
History and Background
TypeScript was first introduced by Microsoft in 2012, aiming to address some of the challenges faced in large-scale JavaScript applications. As JavaScript grew in popularity, developers started encountering issues mainly related to type safety and code scalability. TypeScript emerged as a solution, providing a statically typed superset of JavaScript. This allows developers to add type annotations to their code, simplifying the detection of errors during development rather than at runtime.
Features and Uses
TypeScript captures the essence of JavaScript while introducing additional features. Here are some standout aspects:
- Static Typing: Type annotations help in catching errors early. This reduces debugging time and enhances code quality.
- Enhanced Tooling: Integrated development environments (IDEs) can provide better autocompletion, navigation, and refactoring tools.
- Object-Oriented Programming: TypeScript leverages classes, interfaces, and inheritance, making it more aligned with traditional programming paradigms.
- Compatibility with JavaScript: Every valid JavaScript code is also valid TypeScript code, ensuring that developers can adopt it incrementally.
Developers utilize TypeScript for various applications, from small projects to complex enterprise systems. Its use in frameworks like Angular has also surged its adoption across the development community.
Popularity and Scope
TypeScript has gained a significant foothold in the programming world. According to the Stack Overflow Developer Survey in 2023, TypeScript ranked among the top languages that developers want to work with. The growing interest corresponds with the need for more robust tools in web development, especially as applications scale.
"TypeScript provides a bridge between the flexibility of JavaScript and the strictness of strongly typed languages, enabling developers to write cleaner, maintainable code."
In summary, the allure of TypeScript resides in its balancing act between enabling rapid development while fostering better code practices. For JavaScript developers seeking to deepen their programming knowledge, understanding TypeScript offers a strategic advantage in both project execution and career advancement.
Prelude to TypeScript
In the landscape of modern programming, TypeScript has emerged as a powerful tool, especially for developers familiar with JavaScript. Understanding TypeScript is crucial for efficiently handling complex applications. It serves as a robust bridge between JavaScript's flexibility and the structured programming approach preferred in large-scale development. This section outlines the significance of TypeScript, illustrating how it enhances the coding experience and contributes to cleaner, more maintainable code.
What is TypeScript?
TypeScript is an open-source language developed by Microsoft. It is a superset of JavaScript, meaning any valid JavaScript code is also valid TypeScript code. The core feature of TypeScript is its static typing. This optional feature allows developers to define types for variables, function parameters, and return values, which can help catch errors at compile time rather than at runtime. By offering this layer of type-checking, TypeScript aims to improve development efficiency and code quality.
Historical Context
TypeScript was introduced in 2012, a response to the limitations in JavaScript as projects grew in size and complexity. Since JavaScript is a dynamically typed language, this can lead to unexpected issues during execution. The increasing demand for more structured coding practices in large teams or projects prompted developers to seek alternatives. TypeScript gained traction because it allows developers to write more robust code while still enabling them to utilize existing JavaScript libraries and frameworks. Its growing adoption can be attributed to frameworks like Angular, which fully embraced TypeScript in their architecture.
The Necessity of TypeScript
The importance of adopting TypeScript cannot be overstated. For JavaScript developers, transitioning to TypeScript offers significant advantages:
- Error Reduction: By catching errors early through static typing, developers can avoid many common pitfalls associated with dynamic typing.
- Improved Code Maintainability: Clear type annotations lead to better self-documenting code. This is especially beneficial when collaborating within large teams.
- Enhanced Tooling: TypeScript integrates well with modern IDEs, providing features such as auto-completion and improved refactoring capabilities.
Consider the long-term impact on a codebase. An initial investment in learning TypeScript can lead to fewer bugs, clearer interfaces, and ultimately, reduced development time as the project evolves. Therefore, TypeScript is not just a helpful tool; it becomes a necessity for serious JavaScript developers aiming for scalability and robustness in their applications.
"By embracing TypeScript, developers can turn chaos into order, enhancing both productivity and project clarity."
Understanding these foundational aspects of TypeScript will prepare developers to explore its core features, leading to greater effectiveness in their coding practices.
Comparing JavaScript and TypeScript
Understanding the differences between JavaScript and TypeScript is crucial for developers transitioning from one to the other. This section aims to illuminate key aspects that define both languages, stressing important elements that enhance programming effectiveness. As TypeScript evolves from JavaScript, its capabilities not only build on the existing structure of JavaScript but also introduce additional functionality that caters to larger scale applications. The clear delineation of type systems, compilation process, and language features not only supports developers in coding decisions but also enriches their programming toolkit.
Core Language Features
JavaScript, as a dynamic and flexible language, allows for rapid development and prototyping due to its permissive syntax. However, this flexibility comes with a price. TypeScript adds a layer of structure by introducing static types. This is fundamentally different, as TypeScript enables developers to define interfaces, enums, and generics, which are not present in JavaScript. Understanding these features enhances the control a developer has over their code.
Core language features of TypeScript include:
- Static Typing: This allows for earlier detection of errors during development, enhancing reliability.
- Interfaces: They provide a way to define contracts in code, which improves maintainability and documentation.
- Enums: Enums introduce a way to define a set of named constants, which can make the code more descriptive.
- Generics: They offer a method of creating reusable and adaptable components that can handle a variety of types.
These features are designed to improve the coding experience, especially in larger team environments where multiple developers work on the same codebase.
Static Typing vs. Dynamic Typing
The fundamental distinction between JavaScript and TypeScript lies in the nature of their typing systems. JavaScript employs dynamic typing, meaning that variable types are determined at runtime. This allows for greater flexibility but also increases the potential for runtime errors. A wrongly assigned type may only be caught during execution, which can complicate debugging.
In contrast, TypeScript employs static typing, where variable types are defined at compile time. Developers benefit from this system in several ways:
- Error Detection: Many errors can be caught before the code runs, reducing debugging time.
- IntelliSense Support: IDEs can provide better autocomplete features and suggestions due to known types.
- Code Clarity: By explicitly defining types, code becomes significantly clearer, fostering better team collaboration.
Compilation Process
Another significant difference is the compilation process. JavaScript is an interpreted language and runs directly in the browser. This means developers can test their code instantly. However, such immediacy can obscure errors that need to be addressed before deployment.
TypeScript, on the other hand, is a compiled language. TypeScript code is first transpiled into plain JavaScript, which is then run in the target environment. This compilation step can be beneficial for developers, as it:
- Provides Feedback: Errors can be identified during compilation, giving developers the opportunity to correct them before runtime.
- Generates JavaScript: The resulting JavaScript can be optimized for performance, as TypeScript can leverage advanced features from ES6 and beyond.
- Compatibility: The transpiled code ensures that it runs on all JavaScript environments, making TypeScript projects versatile.
Getting Started with TypeScript
Getting started with TypeScript is essential for JavaScript developers aiming to enhance their coding skills. It provides a strong foundation that bridges JavaScript’s dynamic nature with TypeScript’s static typing. This section covers the necessary steps to set up the development environment and start writing TypeScript code effectively.
Setting Up Your Environment
Setting up your environment is crucial because it ensures that you have the right tools and configuration to leverage TypeScript's features fully. This process consists of two main components: installing Node.js and configuring TypeScript.
Installing Node.js
Installing Node.js is the first step for any JavaScript developer. Node.js allows you to run JavaScript on the server side and serves as a runtime for many development tools. Its popularity comes from the extensive ecosystem of packages available through npm, the node package manager.
Node.js is particularly beneficial because it enables the seamless execution of TypeScript’s build steps. Once installed, it facilitates the installation of TypeScript through npm.
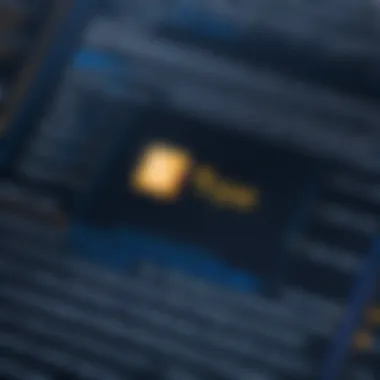
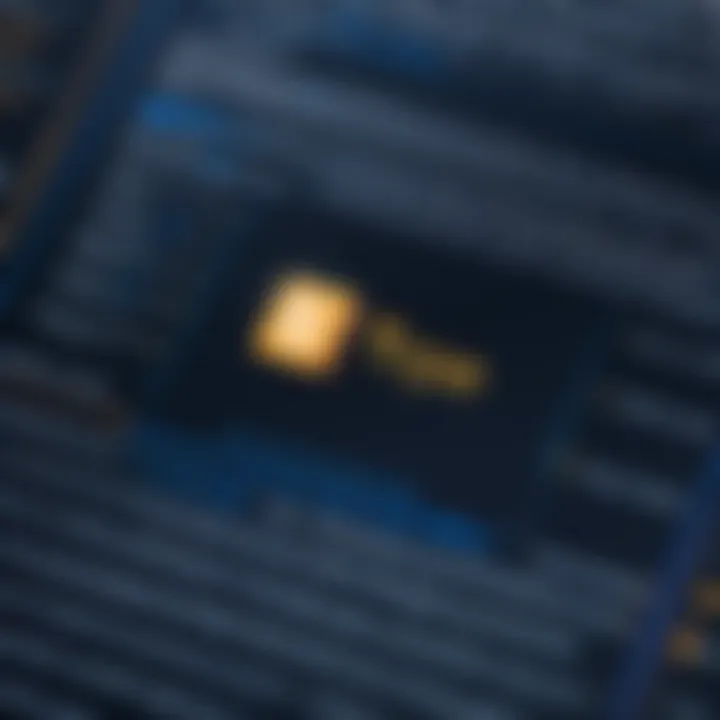
Moreover, one unique characteristic of Node.js is its non-blocking, event-driven architecture, making it efficient for developing applications with concurrent connections. However, a downside might be its reliance on JavaScript for certain aspects of configuration. In this context, learning to use Node.js becomes a vital step in the process of adopting TypeScript.
Configuring TypeScript
Configuring TypeScript involves setting up the TypeScript compiler and modifying its configuration files to suit project needs. This configuration is especially important since it determines how TypeScript files are compiled into JavaScript, which is ultimately run in browsers or Node.js.
TypeScript's configuration is often done using a file. This file allows developers to specify compiler options, types, and other project settings in a structured manner. The flexibility and customizability of this setup make it a popular choice among developers.
One unique feature of configuring TypeScript is the ability to include type definitions for third-party libraries, enhancing compatibility and minimizing runtime errors. However, configuring TypeScript properly might come with a learning curve, particularly for developers new to its static typing and compiler options.
Writing Your First TypeScript Code
Once the environment is set up, the next exciting step is writing your first TypeScript code. This process not only familiarizes you with the syntax but also highlights the advantages of TypeScript's features over traditional JavaScript. From simple functions to more complex class structures, writing TypeScript code helps in better understanding its functionality.
"Transitioning from JavaScript to TypeScript can feel daunting, but mastering the setup and initial coding will pave the way for a smoother transition."
This section will guide you through writing and executing your first TypeScript script, illustrating its enhanced readability and maintainability.
Key TypeScript Features for JavaScript Developers
Understanding TypeScript is crucial for JavaScript developers seeking to elevate their coding capabilities. TypeScript introduces several key features that enhance the development experience and improve code quality. This section explores features such as interfaces, enums, and generics, each vital for structuring larger applications and managing complex data more effectively. By grasping these concepts, developers can leverage TypeScript's advantages to build more robust and maintainable applications.
Interfaces
Interfaces in TypeScript serve as a blueprint for objects. They define the structure of an object by specifying what properties and methods it should have. This structure is fundamental for TypeScript's type system and allows developers to enforce contracts within their code. When working in a team or on large projects, utilizing interfaces can significantly enhance code clarity and reduce potential errors.
For instance, consider the following example of an interface defining a user object:
Using the defined interface helps ensure that any object conforming to follows the specified structure. This promotes consistency, especially when dealing with complex data structures.
Moreover, interfaces can extend other interfaces, allowing for more reusable and flexible code. This feature aids in maintaining clean architecture and eases the transition for developers familiar with object-oriented principles.
Enums
Enums, or enumerations, are another powerful feature provided by TypeScript. They allow developers to create a set of named constants. This makes the codebase more readable and manageable, especially when dealing with values that have a fixed set of options, such as status codes or user roles.
Here is an example of an enum for user roles:
By utilizing enums, developers can use descriptive names instead of primitive values, reducing the chance of errors caused by typos and improving code clarity. Enums can also be combined with interfaces, providing a structured approach to managing complex state and data.
Generics
Generics in TypeScript allow developers to create reusable functions and classes that can work with various data types while maintaining type safety. This capability is vital in developing flexible applications without losing the advantages of a statically typed language.
For example, a generic function can be defined as follows:
In this example, represents a type variable that allows the function to accept any type of argument while ensuring that the return type matches the input type. This flexibility makes generics extremely useful for creating data structures, such as stacks or queues, and writing libraries that can be utilized across different applications.
By mastering interfaces, enums, and generics, JavaScript developers can fully exploit TypeScript's potential, leading to better code quality and maintainability.
Type Safety and Type Inference
Type safety and type inference are crucial components when transitioning from JavaScript to TypeScript. Understanding these concepts allows developers to write more robust code, reducing bugs and improving maintainability. As JavaScript lacks strict type definitions, it can lead to unexpected behaviors in applications. TypeScript addresses this gap, offering a structured approach to types that benefits developers in numerous ways.
Understanding Type Safety
Type safety refers to a programming language's ability to prevent type errors. In TypeScript, this concept is taken seriously, providing a system that allows developers to specify the type of data that can be stored in variables, function parameters, and return types. This guarantees that the code behaves as intended, significantly reducing runtime errors.
When a developer defines types explicitly, TypeScript performs checks during compilation. For example, if a variable is declared as a string, assigning a number to it will result in a compile-time error, rather than a potentially confusing runtime error. This immediate feedback helps catch issues early in the development cycle.
Benefits of Type Safety:
- Forces clearer contracts in code.
- Improves self-documentation, making it easier for teams to understand code structure.
- Enhances refactoring process, as type information is preserved.
- Reduces potential for bugs that arise from incorrect data handling.
To better illustrate this, consider the following TypeScript snippet:
This kind of strictness promotes better coding practices and leads to higher-quality applications.
Using Type Inference
Type inference is a powerful feature in TypeScript that provides flexibility while ensuring type safety. It allows the TypeScript compiler to automatically determine the types of variables when they are created. This means that developers can write code without always explicitly declaring types, simplifying the coding experience while still benefiting from TypeScript’s safety features.
For example, when you assign a value to a variable without specifying a type, TypeScript infers the type based on the value assigned:
Even though is not annotated with a type, TypeScript recognizes that it is a number based on the assignment. This allows for both clean code and type safety.
Considerations for Type Inference:
- Type inference works best when the initial value is clear and unambiguous.
- When combined with defined types, it can create a more readable codebase.
- It is important to understand inferred types, especially in complex scenarios.
By utilizing type inference, developers can enjoy the best of both worlds: concise syntax and maintainable code, thus driving efficiency in development.
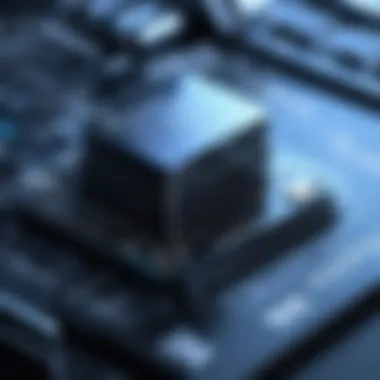
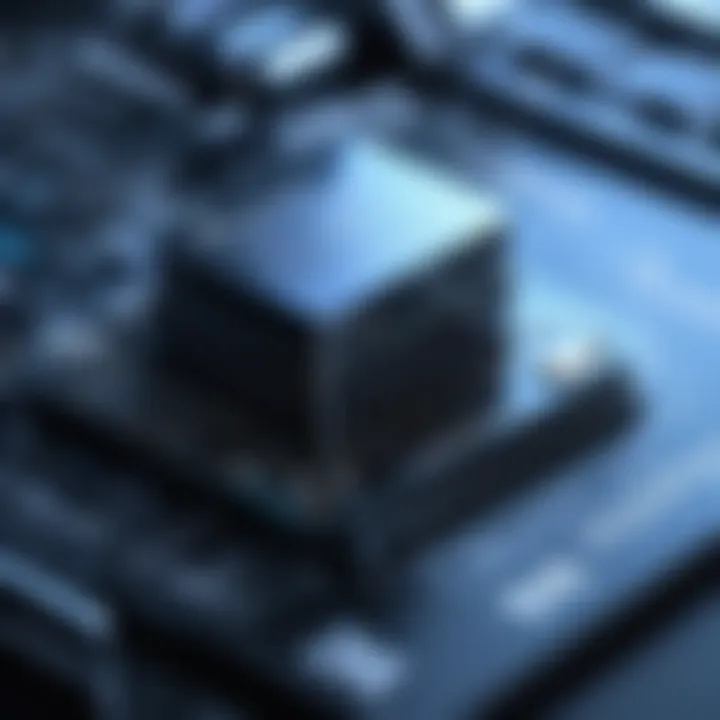
"Understanding type safety and inference allows developers to avoid common pitfalls and boosts confidence in the reliability of their code."
Advanced TypeScript Concepts
Advanced TypeScript concepts are crucial for developers aiming to fully leverage the language's potential. Understanding these features not only enhances code quality but also improves maintainability and scalability. A firm grasp of these concepts allows developers to take full advantage of TypeScript’s capabilities, especially when moving from the more flexible JavaScript environment.
Decorators
Decorators are a powerful feature in TypeScript that enable developers to modify classes and class members at the time of their definition. This metaprogramming tool can seem complex at first but brings significant functionality to your codebase. Decorators are essentially a function that can be attached to a class, method, accessor, property, or parameter. This provides a way to annotate and modify the behavior of the target, thereby enhancing the development experience.
For instance, imagine needing to log information about method calls in a class. Rather than modifying every method directly, one could use a decorator to systematically apply logging across an entire class.
Example of a simple method decorator:
In this example, the decorator wraps the original method to include logging functionality seamlessly. This concise yet profound capability makes decorators a valuable asset in any TypeScript project.
Mapped Types
Mapped types allow for powerful type transformations in TypeScript. They make it easy to create new types based on existing ones, providing a way to derive new types within code bases without repetitive typing. For instance, if you have a type for user profiles and you wish to create a read-only version of it, you can use a mapped type for efficiency.
In this case, is a built-in utility type that transforms all properties of to be read-only. With mapped types, developers can maintain DRY principles and create more flexible codebases. They also help in extending existing types, allowing you to maintain type safety while making essential changes.
Conditional Types
Conditional types provide a way to express non-uniform type relationships in TypeScript. They help in defining types in a way that depends on a condition. This allows developers to apply type logic dynamically. The syntax is similar to a ternary operator in JavaScript and operates based on whether a condition is true or false.
An example can illustrate this:
In this case, checks if is a . If it is, it returns the string "It's a string!". Otherwise, it returns "It's not a string!". This aspect of TypeScript enhances type manipulation, making it a powerful tool for developers seeking to apply conditions in their typings.
In summation, advanced TypeScript concepts enrich the programming landscape for developers transitioning from JavaScript. Grasping decorators, mapped types, and conditional types can significantly elevate one's coding practices. By incorporating these tools, developers can create robust, effective, and maintainable code.
Working with TypeScript in Existing JavaScript Projects
Transitioning from JavaScript to TypeScript requires careful consideration when working within existing projects. Integrating TypeScript can enhance code quality and introduce new features without discarding the established JavaScript ecosystem. This section focuses on how to approach adopting TypeScript incrementally and how it aligns with popular build tools such as Webpack and Babel.
Incremental Adoption
Adopting TypeScript incrementally means that developers can introduce TypeScript into existing JavaScript projects step by step. This approach minimizes disruption to current workflows and allows teams to maintain their timeline for deliverables. First, ensure that TypeScript is installed in your project.
Once installed, rename your JavaScript files from to . TypeScript is quite tolerant and can handle existing JavaScript code gracefully. You can start using type annotations in your code where it makes sense, and gradually refactor JavaScript files to TypeScript. This method encourages teams to learn TypeScript effectively over time while maintaining productivity. Additionally, TypeScript can coexist with JavaScript files; therefore, one can mix and match based on team comfort.
Integrating TypeScript with Build Tools
Integrating TypeScript with build tools like Webpack and Babel is essential for achieving an efficient development workflow. These tools manage the build process, allowing code to be compiled and bundled smoothly.
Webpack
Webpack is a popular module bundler that allows developers to bundle JavaScript files effectively. When integrating TypeScript with Webpack, developers benefit from features like code splitting, which improves load time and performance. One key characteristic of Webpack is its ability to support a wide array of loaders and plugins, enhancing functionality.
A unique feature of Webpack is its hot module replacement. This allows developers to see changes in real-time during development, significantly speeding up the programming cycle. While this is beneficial, developers must be mindful of configuring Webpack correctly to avoid issues down the line. Proper setup ensures that TypeScript is compiled accordingly, and errors are captured during development rather than at runtime.
Babel
Babel is another tool widely recognized for its ability to transpile modern JavaScript into backward-compatible versions. Its capability to transform TypeScript into JavaScript while enabling modern features makes it a strong asset in any development stack. One of its main advantages is the plugins system, allowing for extensive customization based on project requirements.
A distinct feature of Babel is its ease of use combined with compatibility. It works seamlessly with TypeScript, ensuring that developers do not face extensive setup issues. However, it should be noted that Babel does not perform type checking but focuses on converting syntax. Therefore, teams may still need TypeScript's own compiler for thorough type inspections.
Integrating TypeScript with build tools such as Webpack and Babel enhances both development speed and project maintainability, ensuring the transition to TypeScript is smooth and efficient.
In summary, working with TypeScript in existing JavaScript projects is an essential step toward harnessing the power of type safety without discarding established practices. By adopting TypeScript gradually and utilizing tools like Webpack and Babel, developers can seamlessly enhance their existing codebases.
Best Practices for TypeScript Development
The importance of adhering to best practices in TypeScript development cannot be overstated. These practices not only streamline the coding process but also enhance the readability and maintainability of your code. For developers transitioning from JavaScript to TypeScript, implementing these methodologies ensures a smoother adoption of TypeScript's additional features and benefits. Understanding them can reduce bugs and improve collaboration when working in teams.
Code Organization
Effective code organization is a foundational aspect of successful TypeScript development. Organizing code properly helps in separating concerns, making it easier to manage as projects grow. Here are some key considerations for good code organization:
- Modularization: Break your code into smaller modules. Each module should have a specific responsibility. This improves reusability and reduces dependencies between sections of code.
- File Structure: Maintain a logical file structure that reflects the functionality of your modules. For example, placing all type definitions in a folder can help in easily locating them.
- Naming Conventions: Use consistent and meaningful names for your files, functions, and variables. This practice enhances readability and makes it easier for others to understand your code quickly.
Such structures help in avoiding confusion and promote clarity. When revisiting older code, it becomes easier to recall the purpose of each module.
Type Annotations and Usage
Type annotations are one of the standout features of TypeScript. By indicating the expected data types, type annotations significantly improve the quality of code. This feature provides several benefits:
- Error Detection: Type annotations allow TypeScript to catch errors at compile time rather than during runtime. This capability helps identify and fix potential bugs earlier in the development process.
- Code Documentation: When types are explicitly declared, the code becomes self-documenting. This means other developers can quickly understand what types of data are expected in each function or variable, facilitating better collaboration.
- Improved IDE Support: Most modern Integrated Development Environments (IDEs) can leverage type annotations for better autocompletion and refactoring tools. This improves developer productivity by reducing the time spent navigating code.
Here is a simple example demonstrating type annotations in a function:
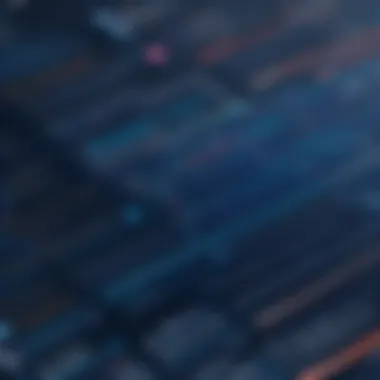
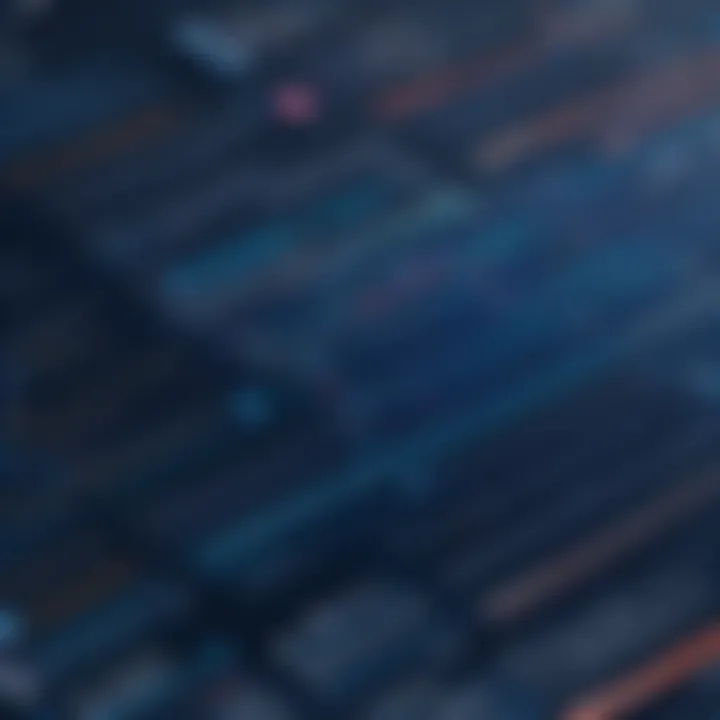
In this example, adding the types to the parameters and return type enhances the function's clarity and reliability.
By incorporating best practices in TypeScript, developers not only write better code but also set a strong foundation for any future projects. Adopting these strategies will yield long-term benefits in development efficiency and project maintainability.
Common Challenges and Debugging in TypeScript
When transitioning from JavaScript to TypeScript, developers will inevitably encounter various challenges. Understanding how to effectively handle errors and debug code is essential. This section will cover how TypeScript's features relate to common programming issues and the best strategies for debugging. The goal is to equip learners with practical knowledge that they can immediately apply to overcome obstacles in their development process.
Error Handling
TypeScript enhances error handling compared to JavaScript by utilizing strict type checking. This feature allows developers to catch potential errors at compile time rather than runtime. If a variable is assigned a type incompatible with its declaration, TypeScript will raise an error during the compilation process.
Key aspects of error handling in TypeScript include:
- Type Annotations: Clearly define the expected types of variables, function parameters, and return values. This clarity enables developers to identify discrepancies before executing code.
- Union Types: Use union types to specify a variable that can hold multiple types. This approach mitigates errors by making sure the code handles different scenarios appropriately.
- Custom Error Types: By creating custom error classes, developers can craft tailored error messages that provide more context and clarity when something goes wrong.
Leveraging these features maximizes code reliability and minimizes unexpected behaviors, which is a significant advantage over traditional JavaScript practices.
Debugging Strategies
Debugging TypeScript can initially seem daunting, but several strategies can streamline the process. Here are effective approaches:
- Using Source Maps: Enable source maps in your TypeScript configuration to improve your debugging experience. Source maps map compiled code back to the original TypeScript source, which makes it easier to identify issues in the actual codebase instead of the transpiled JavaScript.
- TypeScript Compiler Options: Utilize compiler options like , which prevents output if there are errors in the code. This ensures that developers don't run a version of the code that may contain bugs.
- Console Logging: While this may seem basic, effective logging strategies can reveal much about program state and flow. Purposeful logging around critical code, especially conditional branches, can highlight where issues arise.
- Debugging Tools: Use tools like Visual Studio Code, which has robust debugging support for TypeScript. This IDE allows setting breakpoints and inspecting variable states directly from the editor, making it more efficient to pinpoint problems.
- Community Resources: Engage with platforms such as Reddit or Stack Overflow when stuck. Many developers share insights and solutions to common errors, which can provide direction for troubleshooting.
Debugging is not just fixing errors but understanding the reasons behind those errors. Knowledge of TypeScript features can elevate this understanding.
Incorporating these strategies will create a more manageable debugging environment, leading to improved efficiency and better overall productivity in TypeScript development.
Real-World Applications of TypeScript
TypeScript has become increasingly important in modern development environments. As JavaScript continues to dominate the web, TypeScript introduces strong typing, enabling developers to build more scalable and maintainable applications. This section explores the real-world applications of TypeScript, focusing on frameworks and notable case studies that demonstrate its capabilities.
Popular Frameworks Using TypeScript
Many popular frameworks and libraries have embraced TypeScript, enhancing their robustness and developer experience. Some notable frameworks include:
- Angular: Google’s Angular framework is primarily built using TypeScript. Its architecture promotes modularity and testability, with TypeScript facilitating clearer and safer code.
- React: Although originally a JavaScript library, React supports TypeScript for building complex user interfaces. TypeScript helps manage props and state more efficiently, reducing runtime errors.
- Vue.js: While Vue itself is JavaScript-based, TypeScript has a growing presence in its ecosystem, offering improved type definitions that streamline development.
- NestJS: A progressive Node.js framework built with TypeScript, it is designed for building efficient, reliable, and scalable server-side applications.
These frameworks utilize TypeScript's features to improve maintainability, enhance productivity, and reduce bugs, making them preferable for many developers.
Case Studies
Real-world implementations of TypeScript show its effectiveness in various scenarios. Consider the following case studies:
- Microsoft Office Online: The Office suite has incorporated TypeScript for its web applications. This transition allowed for better organization of complex code, significantly enhancing development speed and minimizing bugs.
- Slack: The Slack web application utilizes TypeScript to manage its extensive codebase. This decision improved the team’s ability to quickly implement new features while maintaining stability and performance.
- Asana: A project management tool that adopted TypeScript for its front-end. The type system reduced bugs and increased developer confidence, resulting in faster development cycles and better software quality.
"Adopting TypeScript in projects can lead to improved code quality, fewer bugs, and enhanced collaboration among teams."
In summary, TypeScript plays a crucial role in a variety of real-world applications. Its integration into popular frameworks and successful case studies highlight its benefits in modern software development. By understanding these applications, developers can appreciate how TypeScript enhances their workflow and the overall quality of their projects.
Resources for Further Learning
When transitioning from JavaScript to TypeScript, having access to the correct resources is paramount. These resources not only help reinforce concepts learned but also introduce more advanced topics that complement foundational knowledge. Utilizing a variety of materials can lead to a deeper comprehension and proficiency in TypeScript.
One significant element to consider is that diverse learning styles often influence how effectively someone grasps new information. For visual learners, videos or visual tutorials can be highly engaging. On the other hand, detailed books tend to benefit those who prefer going at their own pace, allowing them to absorb information thoroughly. A mix of formats may often be ideal.
The benefits of leveraging these resources include:
- Depth of Understanding: Books and tutorials often provide comprehensive explanations of topics.
- Practical Application: Online courses typically incorporate project-based learning, enhancing the ability to implement TypeScript in real-world applications.
- Community Support: Engaging with platforms like Reddit allows learners to connect with others facing similar challenges.
- Continuous Learning: As TypeScript evolves, participants can keep updated with current best practices and advanced techniques.
Ultimately, the right resources empower learners to tackle TypeScript confidently and apply it effectively in projects.
Books and Tutorials
Books and tutorials serve as a foundation for understanding TypeScript’s core principles. They provide structured content that guides learners through the initial concepts to more complex topics.
- Effective TypeScript by Dan Vanderkam offers 62 specific ways to harness TypeScript’s capabilities effectively.
- Programming TypeScript by Boris Cherny gives a comprehensive look at using TypeScript in a practical context, emphasizing type-driven techniques.
In addition to books, online tutorials can simplify learning. Websites like freeCodeCamp and Codecademy feature interactive tutorials that allow you to practice coding in real-time.
Online Courses
Online courses are particularly effective for Learning TypeScript. They usually combine video lectures, hands-on exercises, and community forums, providing learners with several resources in one platform.
- Udemy offers a range of courses, like "Understanding TypeScript" by Maximilian Schwarzmüller, which breaks down concepts step-by-step with practical examples.
- Coursera features courses from reputable institutions, allowing learners to gain insights from experts and potentially earn certificates that add credibility to their skillset.
Another advantage of these platforms is their flexibility. Learners can study at their own pace, revisit difficult sections, and even download resources for offline use. This adaptability makes online courses an inviting approach for many.
End
In this article, we explored the transition from JavaScript to TypeScript, a journey that can greatly enhance a developer's abilities. This conclusion is significant because it ties together the critical insights and key principles discussed throughout the article. Adopting TypeScript brings several essential benefits that can improve both coding standards and team collaboration.
Recap of Key Points
First, we highlighted the distinctive attributes of TypeScript compared to JavaScript. TypeScript introduces static typing, which helps catch errors at compile time. This feature fosters a more robust coding environment. We also explored the importance of interfaces and how they facilitate clear contract definitions within the code.
Moreover, the compilation process was detailed, explaining how TypeScript is transpiled into JavaScript, thus maintaining cross-browser compatibility. The incremental adoption in existing JavaScript projects was discussed as a pragmatic approach toward TypeScript integration.
In terms of best practices for TypeScript development, we covered code organization and efficient use of type annotations. These practices significantly contribute to maintainable and scalable code.
Future of TypeScript
Looking ahead, TypeScript is poised for continued growth within the programming community. Its adaptability and the ongoing support from Microsoft ensure its relevancy. The demand for TypeScript will likely increase as more developers recognize its advantages, particularly for larger codebases where type safety becomes crucial.
The community around TypeScript is expanding rapidly, leading to extensive resources, frameworks, and libraries. Adoption by popular frameworks such as Angular and React illustrates its importance in modern web development. Additionally, the continuous updates and enhancements will likely attract even more developers.